Cloud Functions - Updating Timestamps Based on Warning
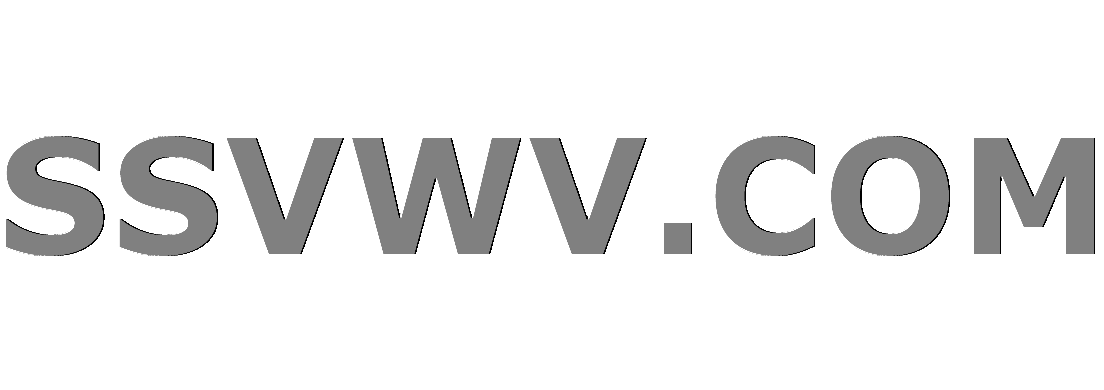
Multi tool use
up vote
0
down vote
favorite
I have the following setup for Firebase Cloud Functions:
index.js
const functions = require('firebase-functions');
const trackVote = require('./trackVote')
const admin = require('firebase-admin');
admin.initializeApp();
exports.trackVote = trackVote.handler;
trackvote.js
const functions = require('firebase-functions');
const admin = require('firebase-admin');
exports.handler = functions.firestore.document('/Polls/{pollId}/responses/{userId}').onCreate((data, context) => {
const answerSelected = data.data().answer;
const answerRef = admin.firestore().doc(`Polls/${context.params.pollId}/answers/${answerSelected}`);
const voteCountRef = admin.firestore().doc(`Polls/${context.params.pollId}`);
return admin.firestore().runTransaction(t => {
return t.get(answerRef)
.then(doc => {
if (doc.data()) {
t.update(answerRef, { vote_count: doc.data().vote_count + 1 });
}
})
}).then(result => {
return admin.firestore().runTransaction(t => {
return t.get(voteCountRef)
.then(doc => {
if (doc.data()) {
t.update(voteCountRef, {vote_count:doc.data().vote_count+1});
}
});
});
});
});
I received the below warning and was curious what changes I need to make within my code on both the cloud functions side and the client side:
TypeError: db.settings is not a function
at Object.<anonymous> (/Users/troychuinard/Code/FanPolls/functions/index.js:13:4)
at Module._compile (module.js:643:30)
at Object.Module._extensions..js (module.js:654:10)
at Module.load (module.js:556:32)
at tryModuleLoad (module.js:499:12)
at Function.Module._load (module.js:491:3)
at Module.require (module.js:587:17)
at require (internal/module.js:11:18)
at /usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:21:11
at Object.<anonymous> (/usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:61:3)
Note: I may need to update my Cloud Functions, which I can do, however I am curious how that would impact the code in both my index.js and trackVote.js
node.js

add a comment |
up vote
0
down vote
favorite
I have the following setup for Firebase Cloud Functions:
index.js
const functions = require('firebase-functions');
const trackVote = require('./trackVote')
const admin = require('firebase-admin');
admin.initializeApp();
exports.trackVote = trackVote.handler;
trackvote.js
const functions = require('firebase-functions');
const admin = require('firebase-admin');
exports.handler = functions.firestore.document('/Polls/{pollId}/responses/{userId}').onCreate((data, context) => {
const answerSelected = data.data().answer;
const answerRef = admin.firestore().doc(`Polls/${context.params.pollId}/answers/${answerSelected}`);
const voteCountRef = admin.firestore().doc(`Polls/${context.params.pollId}`);
return admin.firestore().runTransaction(t => {
return t.get(answerRef)
.then(doc => {
if (doc.data()) {
t.update(answerRef, { vote_count: doc.data().vote_count + 1 });
}
})
}).then(result => {
return admin.firestore().runTransaction(t => {
return t.get(voteCountRef)
.then(doc => {
if (doc.data()) {
t.update(voteCountRef, {vote_count:doc.data().vote_count+1});
}
});
});
});
});
I received the below warning and was curious what changes I need to make within my code on both the cloud functions side and the client side:
TypeError: db.settings is not a function
at Object.<anonymous> (/Users/troychuinard/Code/FanPolls/functions/index.js:13:4)
at Module._compile (module.js:643:30)
at Object.Module._extensions..js (module.js:654:10)
at Module.load (module.js:556:32)
at tryModuleLoad (module.js:499:12)
at Function.Module._load (module.js:491:3)
at Module.require (module.js:587:17)
at require (internal/module.js:11:18)
at /usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:21:11
at Object.<anonymous> (/usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:61:3)
Note: I may need to update my Cloud Functions, which I can do, however I am curious how that would impact the code in both my index.js and trackVote.js
node.js

1
Please don't show pictures of error messages or code. It's better to copy an paste them into the question so they're easier to read and search.
– Doug Stevenson
Nov 8 at 5:45
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the following setup for Firebase Cloud Functions:
index.js
const functions = require('firebase-functions');
const trackVote = require('./trackVote')
const admin = require('firebase-admin');
admin.initializeApp();
exports.trackVote = trackVote.handler;
trackvote.js
const functions = require('firebase-functions');
const admin = require('firebase-admin');
exports.handler = functions.firestore.document('/Polls/{pollId}/responses/{userId}').onCreate((data, context) => {
const answerSelected = data.data().answer;
const answerRef = admin.firestore().doc(`Polls/${context.params.pollId}/answers/${answerSelected}`);
const voteCountRef = admin.firestore().doc(`Polls/${context.params.pollId}`);
return admin.firestore().runTransaction(t => {
return t.get(answerRef)
.then(doc => {
if (doc.data()) {
t.update(answerRef, { vote_count: doc.data().vote_count + 1 });
}
})
}).then(result => {
return admin.firestore().runTransaction(t => {
return t.get(voteCountRef)
.then(doc => {
if (doc.data()) {
t.update(voteCountRef, {vote_count:doc.data().vote_count+1});
}
});
});
});
});
I received the below warning and was curious what changes I need to make within my code on both the cloud functions side and the client side:
TypeError: db.settings is not a function
at Object.<anonymous> (/Users/troychuinard/Code/FanPolls/functions/index.js:13:4)
at Module._compile (module.js:643:30)
at Object.Module._extensions..js (module.js:654:10)
at Module.load (module.js:556:32)
at tryModuleLoad (module.js:499:12)
at Function.Module._load (module.js:491:3)
at Module.require (module.js:587:17)
at require (internal/module.js:11:18)
at /usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:21:11
at Object.<anonymous> (/usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:61:3)
Note: I may need to update my Cloud Functions, which I can do, however I am curious how that would impact the code in both my index.js and trackVote.js
node.js

I have the following setup for Firebase Cloud Functions:
index.js
const functions = require('firebase-functions');
const trackVote = require('./trackVote')
const admin = require('firebase-admin');
admin.initializeApp();
exports.trackVote = trackVote.handler;
trackvote.js
const functions = require('firebase-functions');
const admin = require('firebase-admin');
exports.handler = functions.firestore.document('/Polls/{pollId}/responses/{userId}').onCreate((data, context) => {
const answerSelected = data.data().answer;
const answerRef = admin.firestore().doc(`Polls/${context.params.pollId}/answers/${answerSelected}`);
const voteCountRef = admin.firestore().doc(`Polls/${context.params.pollId}`);
return admin.firestore().runTransaction(t => {
return t.get(answerRef)
.then(doc => {
if (doc.data()) {
t.update(answerRef, { vote_count: doc.data().vote_count + 1 });
}
})
}).then(result => {
return admin.firestore().runTransaction(t => {
return t.get(voteCountRef)
.then(doc => {
if (doc.data()) {
t.update(voteCountRef, {vote_count:doc.data().vote_count+1});
}
});
});
});
});
I received the below warning and was curious what changes I need to make within my code on both the cloud functions side and the client side:
TypeError: db.settings is not a function
at Object.<anonymous> (/Users/troychuinard/Code/FanPolls/functions/index.js:13:4)
at Module._compile (module.js:643:30)
at Object.Module._extensions..js (module.js:654:10)
at Module.load (module.js:556:32)
at tryModuleLoad (module.js:499:12)
at Function.Module._load (module.js:491:3)
at Module.require (module.js:587:17)
at require (internal/module.js:11:18)
at /usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:21:11
at Object.<anonymous> (/usr/local/lib/node_modules/firebase-tools/lib/triggerParser.js:61:3)
Note: I may need to update my Cloud Functions, which I can do, however I am curious how that would impact the code in both my index.js and trackVote.js
node.js

node.js

edited Nov 10 at 0:14
asked Nov 8 at 2:44
tccpg288
1,14511533
1,14511533
1
Please don't show pictures of error messages or code. It's better to copy an paste them into the question so they're easier to read and search.
– Doug Stevenson
Nov 8 at 5:45
add a comment |
1
Please don't show pictures of error messages or code. It's better to copy an paste them into the question so they're easier to read and search.
– Doug Stevenson
Nov 8 at 5:45
1
1
Please don't show pictures of error messages or code. It's better to copy an paste them into the question so they're easier to read and search.
– Doug Stevenson
Nov 8 at 5:45
Please don't show pictures of error messages or code. It's better to copy an paste them into the question so they're easier to read and search.
– Doug Stevenson
Nov 8 at 5:45
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
const admin = require('firebase-admin');
const functions = require('firebase-functions');
let firebaseApp;
if (admin.apps.length > 0) {
firebaseApp = admin.app()
} else {
firebaseApp = admin.initializeApp(functions.config().firebase)
}
let db = firebaseApp.firestore()
db.settings({ timestampsInSnapshots: true })
Thanks, so this code would go in the index.js, below admin.initializeApp() but above exports.trackVote = trackVote.handler? Also, would I have to change all of the dates in my client code?
– tccpg288
Nov 8 at 3:12
Yes. You don't need to change dates in your client code, as long as it fits your needs.
– Socrates Tuas
Nov 8 at 3:18
Much appreciated
– tccpg288
Nov 8 at 3:20
Actually I encountered the error above, not sure if I should delete the first admin.initializeApp()
– tccpg288
Nov 8 at 3:40
Thanks, I tried your code and am still getting the above error. I realize I may need to update my cloud functions, just unsure how it will impact trackVote.js, if that will change at all.
– tccpg288
Nov 10 at 0:14
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
const admin = require('firebase-admin');
const functions = require('firebase-functions');
let firebaseApp;
if (admin.apps.length > 0) {
firebaseApp = admin.app()
} else {
firebaseApp = admin.initializeApp(functions.config().firebase)
}
let db = firebaseApp.firestore()
db.settings({ timestampsInSnapshots: true })
Thanks, so this code would go in the index.js, below admin.initializeApp() but above exports.trackVote = trackVote.handler? Also, would I have to change all of the dates in my client code?
– tccpg288
Nov 8 at 3:12
Yes. You don't need to change dates in your client code, as long as it fits your needs.
– Socrates Tuas
Nov 8 at 3:18
Much appreciated
– tccpg288
Nov 8 at 3:20
Actually I encountered the error above, not sure if I should delete the first admin.initializeApp()
– tccpg288
Nov 8 at 3:40
Thanks, I tried your code and am still getting the above error. I realize I may need to update my cloud functions, just unsure how it will impact trackVote.js, if that will change at all.
– tccpg288
Nov 10 at 0:14
add a comment |
up vote
2
down vote
const admin = require('firebase-admin');
const functions = require('firebase-functions');
let firebaseApp;
if (admin.apps.length > 0) {
firebaseApp = admin.app()
} else {
firebaseApp = admin.initializeApp(functions.config().firebase)
}
let db = firebaseApp.firestore()
db.settings({ timestampsInSnapshots: true })
Thanks, so this code would go in the index.js, below admin.initializeApp() but above exports.trackVote = trackVote.handler? Also, would I have to change all of the dates in my client code?
– tccpg288
Nov 8 at 3:12
Yes. You don't need to change dates in your client code, as long as it fits your needs.
– Socrates Tuas
Nov 8 at 3:18
Much appreciated
– tccpg288
Nov 8 at 3:20
Actually I encountered the error above, not sure if I should delete the first admin.initializeApp()
– tccpg288
Nov 8 at 3:40
Thanks, I tried your code and am still getting the above error. I realize I may need to update my cloud functions, just unsure how it will impact trackVote.js, if that will change at all.
– tccpg288
Nov 10 at 0:14
add a comment |
up vote
2
down vote
up vote
2
down vote
const admin = require('firebase-admin');
const functions = require('firebase-functions');
let firebaseApp;
if (admin.apps.length > 0) {
firebaseApp = admin.app()
} else {
firebaseApp = admin.initializeApp(functions.config().firebase)
}
let db = firebaseApp.firestore()
db.settings({ timestampsInSnapshots: true })
const admin = require('firebase-admin');
const functions = require('firebase-functions');
let firebaseApp;
if (admin.apps.length > 0) {
firebaseApp = admin.app()
} else {
firebaseApp = admin.initializeApp(functions.config().firebase)
}
let db = firebaseApp.firestore()
db.settings({ timestampsInSnapshots: true })
edited Nov 8 at 3:58
answered Nov 8 at 3:07


Socrates Tuas
1146
1146
Thanks, so this code would go in the index.js, below admin.initializeApp() but above exports.trackVote = trackVote.handler? Also, would I have to change all of the dates in my client code?
– tccpg288
Nov 8 at 3:12
Yes. You don't need to change dates in your client code, as long as it fits your needs.
– Socrates Tuas
Nov 8 at 3:18
Much appreciated
– tccpg288
Nov 8 at 3:20
Actually I encountered the error above, not sure if I should delete the first admin.initializeApp()
– tccpg288
Nov 8 at 3:40
Thanks, I tried your code and am still getting the above error. I realize I may need to update my cloud functions, just unsure how it will impact trackVote.js, if that will change at all.
– tccpg288
Nov 10 at 0:14
add a comment |
Thanks, so this code would go in the index.js, below admin.initializeApp() but above exports.trackVote = trackVote.handler? Also, would I have to change all of the dates in my client code?
– tccpg288
Nov 8 at 3:12
Yes. You don't need to change dates in your client code, as long as it fits your needs.
– Socrates Tuas
Nov 8 at 3:18
Much appreciated
– tccpg288
Nov 8 at 3:20
Actually I encountered the error above, not sure if I should delete the first admin.initializeApp()
– tccpg288
Nov 8 at 3:40
Thanks, I tried your code and am still getting the above error. I realize I may need to update my cloud functions, just unsure how it will impact trackVote.js, if that will change at all.
– tccpg288
Nov 10 at 0:14
Thanks, so this code would go in the index.js, below admin.initializeApp() but above exports.trackVote = trackVote.handler? Also, would I have to change all of the dates in my client code?
– tccpg288
Nov 8 at 3:12
Thanks, so this code would go in the index.js, below admin.initializeApp() but above exports.trackVote = trackVote.handler? Also, would I have to change all of the dates in my client code?
– tccpg288
Nov 8 at 3:12
Yes. You don't need to change dates in your client code, as long as it fits your needs.
– Socrates Tuas
Nov 8 at 3:18
Yes. You don't need to change dates in your client code, as long as it fits your needs.
– Socrates Tuas
Nov 8 at 3:18
Much appreciated
– tccpg288
Nov 8 at 3:20
Much appreciated
– tccpg288
Nov 8 at 3:20
Actually I encountered the error above, not sure if I should delete the first admin.initializeApp()
– tccpg288
Nov 8 at 3:40
Actually I encountered the error above, not sure if I should delete the first admin.initializeApp()
– tccpg288
Nov 8 at 3:40
Thanks, I tried your code and am still getting the above error. I realize I may need to update my cloud functions, just unsure how it will impact trackVote.js, if that will change at all.
– tccpg288
Nov 10 at 0:14
Thanks, I tried your code and am still getting the above error. I realize I may need to update my cloud functions, just unsure how it will impact trackVote.js, if that will change at all.
– tccpg288
Nov 10 at 0:14
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53200823%2fcloud-functions-updating-timestamps-based-on-warning%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
14sfyEuBNS4 UiJ5rBGCIWFLK h,zLh2,JFzpdR,EojYxahGRdGA CBZNadcRZ
1
Please don't show pictures of error messages or code. It's better to copy an paste them into the question so they're easier to read and search.
– Doug Stevenson
Nov 8 at 5:45