Servlets & JSPs basics: Use one or two servlets to design a website login?
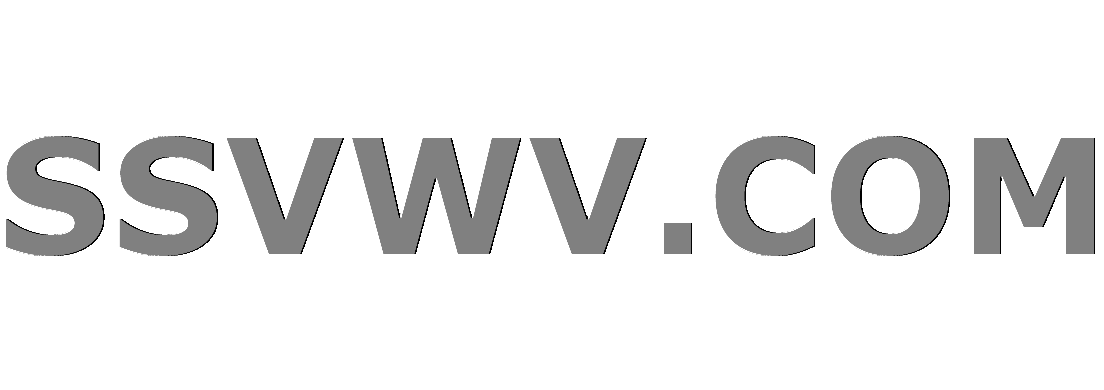
Multi tool use
up vote
0
down vote
favorite
I'm learning about servlets and JSPs and have what is probably a very basic question. I understand a servlet has URL mappings so that when a URL is requested, the associated servlet takes the request, processes it and possibly forwards to a JSP.
Now I want to do a Login page. When I request the URL ending with ".../Login" I want to display a form with username and password fields and a Login button. When the button is clicked, I want a success or failure page to be displayed.
My question is do I need 2 URLs for this operation, one of which would be ".../Login" and the other ".../Login/Result" for instance, and when the button is clicked, a post request would be sent to the second one? In that case I would need two servlets. The first one would simply forward to the JSP which contains the form, and that form would have:
<form action="/Result" method="post">
and the second one would receive the request containing the form data and do the actual login logic and forward to a success or fail view (JSP).
Is that the way to do it or is there a simpler way to do a login, using one servlet? Is there a way for a single servlet to display an initial view containing a form then when that form is submitted, display a subsequent view (success/failure)?
jsp servlets login
add a comment |
up vote
0
down vote
favorite
I'm learning about servlets and JSPs and have what is probably a very basic question. I understand a servlet has URL mappings so that when a URL is requested, the associated servlet takes the request, processes it and possibly forwards to a JSP.
Now I want to do a Login page. When I request the URL ending with ".../Login" I want to display a form with username and password fields and a Login button. When the button is clicked, I want a success or failure page to be displayed.
My question is do I need 2 URLs for this operation, one of which would be ".../Login" and the other ".../Login/Result" for instance, and when the button is clicked, a post request would be sent to the second one? In that case I would need two servlets. The first one would simply forward to the JSP which contains the form, and that form would have:
<form action="/Result" method="post">
and the second one would receive the request containing the form data and do the actual login logic and forward to a success or fail view (JSP).
Is that the way to do it or is there a simpler way to do a login, using one servlet? Is there a way for a single servlet to display an initial view containing a form then when that form is submitted, display a subsequent view (success/failure)?
jsp servlets login
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm learning about servlets and JSPs and have what is probably a very basic question. I understand a servlet has URL mappings so that when a URL is requested, the associated servlet takes the request, processes it and possibly forwards to a JSP.
Now I want to do a Login page. When I request the URL ending with ".../Login" I want to display a form with username and password fields and a Login button. When the button is clicked, I want a success or failure page to be displayed.
My question is do I need 2 URLs for this operation, one of which would be ".../Login" and the other ".../Login/Result" for instance, and when the button is clicked, a post request would be sent to the second one? In that case I would need two servlets. The first one would simply forward to the JSP which contains the form, and that form would have:
<form action="/Result" method="post">
and the second one would receive the request containing the form data and do the actual login logic and forward to a success or fail view (JSP).
Is that the way to do it or is there a simpler way to do a login, using one servlet? Is there a way for a single servlet to display an initial view containing a form then when that form is submitted, display a subsequent view (success/failure)?
jsp servlets login
I'm learning about servlets and JSPs and have what is probably a very basic question. I understand a servlet has URL mappings so that when a URL is requested, the associated servlet takes the request, processes it and possibly forwards to a JSP.
Now I want to do a Login page. When I request the URL ending with ".../Login" I want to display a form with username and password fields and a Login button. When the button is clicked, I want a success or failure page to be displayed.
My question is do I need 2 URLs for this operation, one of which would be ".../Login" and the other ".../Login/Result" for instance, and when the button is clicked, a post request would be sent to the second one? In that case I would need two servlets. The first one would simply forward to the JSP which contains the form, and that form would have:
<form action="/Result" method="post">
and the second one would receive the request containing the form data and do the actual login logic and forward to a success or fail view (JSP).
Is that the way to do it or is there a simpler way to do a login, using one servlet? Is there a way for a single servlet to display an initial view containing a form then when that form is submitted, display a subsequent view (success/failure)?
jsp servlets login
jsp servlets login
asked Nov 7 at 4:18
Andrew S.
124
124
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
0
down vote
accepted
I understand a servlet has URL mappings so that when a URL is
requested, the associated servlet takes the request, processes it and
possibly forwards to a JSP.
Correct but remember that only the doGet method is accessible directly. (i.e. you can run the doGet method of a servlet by typing it into the browser)
When I request the URL ending with ".../Login" I want to display a
form with username and password fields and a Login button.
You can use the doGet method of the Login servlet to forward to the jsp:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
RequestDispatcher rd = request.getRequestDispatcher("login.jsp");
rd.forward(request, response);
}
So you can now either access the login page form either directly /login.jsp
or via the doGet method of our Login Servlet /Login
For example you could have a link somewhere like this:
<a href="/Login">Login</a>
My question is do I need 2 URLs for this operation, one of which would
be ".../Login" and the other ".../Login/Result" for instance, and when
the button is clicked, a post request would be sent to the second one?
In that case I would need two servlets. The first one would simply
forward to the JSP which contains the form, and that form would have:
No, that would be over complicating it and would make your application harder to manage. You can use the same Login Servlet to both redirect the user to the login page (doGet) and handle the results (doPost).
When the button is clicked, I want a success or failure page to be displayed.
Instead of having a failure jsp, why not just keep it on the login page? If a user types in their password incorrectly by mistake more than once, it would be annoying for them to keep having to go back to the login page no? What most websites do is that they will show the error message on the login page itself, so they can easily just type their details in again without having to click back. And you don't need a dedicated success jsp, the success page would just be the dashboard/homepage/welcomepage.
Here's a nice example of the above:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//get the parameters submitted by the form
String user_name = request.getParameter("username");
String user_pass = request.getParameter("password");
String url = ""; //Create a string literal that we will modify based on if the details are correct or not.
UserDao udao = new UserDao(); //class containing all the database methods pertaining to the user table.
if(udao.isValid(user_name, user_pass)){ //UserDao has a method called isValid which returns a boolean.
//details are valid so forward to success page
url = "welcome.jsp"; //set the url welcome.jsp because the details are correct.
//here you set your session variables and do whatever else you want to do
HttpSession session = (request.getSession());
session.setAttribute("username", user_name); // i can access this variable now in any jsp with: ${username}
}else{
//details are not valid so lets forward them back to the login page with an error message
String error = "Username or password is incorrect!";
request.setAttribute("error", error);
url = "login.jsp"; //set the url login.jsp because the details are incorrect.
}
RequestDispatcher rd = request.getRequestDispatcher(url); //the url we set based on if details are correct or not
rd.forward(request, response);
}
Your jsp might look something like this:
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<jsp:include flush="true" page="includes/header.jsp"></jsp:include>
<form action="../Login" method="post">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="Submit">
</form>
<!-- you can handle the error here also -->
<h1>${error}</h1>
<jsp:include flush="true" page="includes/footer.jsp"></jsp:include>
The isValid method inside the UserDao class would look something like this:
public boolean isValid(String username, String userpass){
boolean status = false;
PreparedStatement pst = null;
ResultSet rs = null;
try(Connection conn= DBConnection.getConnection()){
pst = conn.prepareStatement("select * from user where username=? and userpass=?;");
pst.setString(1, username);
pst.setString(2, userpass);
rs = pst.executeQuery();
status = rs.next();
} catch (SQLException e) {
e.printStackTrace();
}
return status;
}
Hope that helps understand it better, if you have any questions feel free to ask.
Note: one of the answers uses scriptlets in their jsp (those <% %>
things) . This is highly discouraged! If you want to start on a good path do not use scriptlets! More info on why here:
How to avoid Java code in JSP files?
add a comment |
up vote
0
down vote
As a general rule, you will want to use multiple URLs.
This is because you'll want to follow the basic idiom of POST
, REDIRECT
, GET
for the majority of your transactions. This helps reinforce the "stateless" nature of web applications.
So, for your example, you could do something like this:
GET /Login
(mapped to LoginServlet) -> dispatcher.forward("/login.jsp") which displays the login form.
The form will then:
POST /Login
with the user credentials. Note that the URL is the same but the request is different, a POST
instead of a GET
.
At this point, if the login succeeds, then the LoginServlet will send a HttpServletResponse.sendRedirect to, say, "/Welcome" (which is your WelcomeServlet).
If the login FAILS, then you need to REDIRECT to a login failure. This can be as simple as your LoginServlet with a "failed" parameter.
So, .sendRedirect("/Login?failed"). When you LoginServlet sees the "failed" parameter, it .forwards to "/loginFailed.jsp".
Why do you do this?
Simply, think of what happens should the user do a refresh button on their browser. If you just POST
and then forward to the JSP, when you refresh, the browser will POST
again (or ask if you want to submit the form again, which is annoying).
By sending the redirect, which causes a new GET, the URL changes, and it just reloads the last page they saw.
Finally, you don't want to GET
JSPs directly, but route them through your servlet layer. The servlets are the actions in the system, the JSPs are just the views. Send the requests to the servlets, let them figure out what the views are.
Once you've done this a couple times, you'll want to potentially look in to "action frameworks" which makes this type of web programming easier.
Thanks for the thorough response. I hadn't thought of using a single servlet and single URL for 2 different requests, each having its own logic, since in this case one is a get and the other one is a post.
– Andrew S.
Nov 7 at 7:03
add a comment |
up vote
0
down vote
This is opinion based. What I would do is,
- Have a folder named
login
and have anindex.jsp
page inside it, containing the login form design (.../login/index.jsp
). So I can call.../login/
as it is not necessary to mentionindex.jsp
in the url.
Then I'll have a servlet to pass the information for verification. I would prefer the URL of the servlet to be
.../login/controller
.
form tag of the
/login/index.jsp
would look like this.
<form action="controller" method="POST">
But, you can do this even with a single jsp page. At the beginning of the page you can check whether the login information is being passed.
<%
String username = request.getParameter("username"),
password = request.getParameter("password");
if(username != null && password != null){
//validation part
response.sendRedirect("profile.jsp"); //if login successful
return; //make sure you add this after redirecting.
}
%>
<!-- html part of the login page continues -->
<form action="" method="POST">
Side note: If you want to remove the .jsp
part from your URL when sending a request to a jsp page. You can add an URL mapping for a jsp page in your deployment descriptor(web.xml
).
<servlet>
<servlet-name>Login</servlet-name>
<jsp-file>login.jsp</jsp-file>
</servlet>
<servlet-mapping>
<servlet-name>Login</servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>
Read Url Mapping For Jsp for more info.
Ok so no servlet for the first part, just a jsp page. A servlet would be needed just for taking the post request, but you're suggesting it's not even necessary and I can have the login logic inside the jsp? But isn't it better to separate code from the jsp (which is presentation)? Also, I have a stupid question: where do you put your "login" folder? Under WebContent? And is there a way for Tomcat to automatically map a newly created jsp to a url, just like for servlets it's automatically done when I use Eclipse's create New Servlet?
– Andrew S.
Nov 7 at 7:16
@AndrewS. isn't it better to separate code from the jsp? like I said it is primarily opinion-based. According to my opinion, yes it is better to have a jsp and a servlet, I was just saying that you can even do it in the same jsp page. I've updated my answer with an image of the folder tree (I'm using NetBeans, the folder name maybe different in Eclipse). Tomcat will give you an url automatically to your newly created jsp page. In my case it's../login/index.jsp
. No manual mapping is needed unless you need to get rid of that.jsp
part in the URL.
– Roshana Pitigala
Nov 7 at 8:02
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
I understand a servlet has URL mappings so that when a URL is
requested, the associated servlet takes the request, processes it and
possibly forwards to a JSP.
Correct but remember that only the doGet method is accessible directly. (i.e. you can run the doGet method of a servlet by typing it into the browser)
When I request the URL ending with ".../Login" I want to display a
form with username and password fields and a Login button.
You can use the doGet method of the Login servlet to forward to the jsp:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
RequestDispatcher rd = request.getRequestDispatcher("login.jsp");
rd.forward(request, response);
}
So you can now either access the login page form either directly /login.jsp
or via the doGet method of our Login Servlet /Login
For example you could have a link somewhere like this:
<a href="/Login">Login</a>
My question is do I need 2 URLs for this operation, one of which would
be ".../Login" and the other ".../Login/Result" for instance, and when
the button is clicked, a post request would be sent to the second one?
In that case I would need two servlets. The first one would simply
forward to the JSP which contains the form, and that form would have:
No, that would be over complicating it and would make your application harder to manage. You can use the same Login Servlet to both redirect the user to the login page (doGet) and handle the results (doPost).
When the button is clicked, I want a success or failure page to be displayed.
Instead of having a failure jsp, why not just keep it on the login page? If a user types in their password incorrectly by mistake more than once, it would be annoying for them to keep having to go back to the login page no? What most websites do is that they will show the error message on the login page itself, so they can easily just type their details in again without having to click back. And you don't need a dedicated success jsp, the success page would just be the dashboard/homepage/welcomepage.
Here's a nice example of the above:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//get the parameters submitted by the form
String user_name = request.getParameter("username");
String user_pass = request.getParameter("password");
String url = ""; //Create a string literal that we will modify based on if the details are correct or not.
UserDao udao = new UserDao(); //class containing all the database methods pertaining to the user table.
if(udao.isValid(user_name, user_pass)){ //UserDao has a method called isValid which returns a boolean.
//details are valid so forward to success page
url = "welcome.jsp"; //set the url welcome.jsp because the details are correct.
//here you set your session variables and do whatever else you want to do
HttpSession session = (request.getSession());
session.setAttribute("username", user_name); // i can access this variable now in any jsp with: ${username}
}else{
//details are not valid so lets forward them back to the login page with an error message
String error = "Username or password is incorrect!";
request.setAttribute("error", error);
url = "login.jsp"; //set the url login.jsp because the details are incorrect.
}
RequestDispatcher rd = request.getRequestDispatcher(url); //the url we set based on if details are correct or not
rd.forward(request, response);
}
Your jsp might look something like this:
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<jsp:include flush="true" page="includes/header.jsp"></jsp:include>
<form action="../Login" method="post">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="Submit">
</form>
<!-- you can handle the error here also -->
<h1>${error}</h1>
<jsp:include flush="true" page="includes/footer.jsp"></jsp:include>
The isValid method inside the UserDao class would look something like this:
public boolean isValid(String username, String userpass){
boolean status = false;
PreparedStatement pst = null;
ResultSet rs = null;
try(Connection conn= DBConnection.getConnection()){
pst = conn.prepareStatement("select * from user where username=? and userpass=?;");
pst.setString(1, username);
pst.setString(2, userpass);
rs = pst.executeQuery();
status = rs.next();
} catch (SQLException e) {
e.printStackTrace();
}
return status;
}
Hope that helps understand it better, if you have any questions feel free to ask.
Note: one of the answers uses scriptlets in their jsp (those <% %>
things) . This is highly discouraged! If you want to start on a good path do not use scriptlets! More info on why here:
How to avoid Java code in JSP files?
add a comment |
up vote
0
down vote
accepted
I understand a servlet has URL mappings so that when a URL is
requested, the associated servlet takes the request, processes it and
possibly forwards to a JSP.
Correct but remember that only the doGet method is accessible directly. (i.e. you can run the doGet method of a servlet by typing it into the browser)
When I request the URL ending with ".../Login" I want to display a
form with username and password fields and a Login button.
You can use the doGet method of the Login servlet to forward to the jsp:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
RequestDispatcher rd = request.getRequestDispatcher("login.jsp");
rd.forward(request, response);
}
So you can now either access the login page form either directly /login.jsp
or via the doGet method of our Login Servlet /Login
For example you could have a link somewhere like this:
<a href="/Login">Login</a>
My question is do I need 2 URLs for this operation, one of which would
be ".../Login" and the other ".../Login/Result" for instance, and when
the button is clicked, a post request would be sent to the second one?
In that case I would need two servlets. The first one would simply
forward to the JSP which contains the form, and that form would have:
No, that would be over complicating it and would make your application harder to manage. You can use the same Login Servlet to both redirect the user to the login page (doGet) and handle the results (doPost).
When the button is clicked, I want a success or failure page to be displayed.
Instead of having a failure jsp, why not just keep it on the login page? If a user types in their password incorrectly by mistake more than once, it would be annoying for them to keep having to go back to the login page no? What most websites do is that they will show the error message on the login page itself, so they can easily just type their details in again without having to click back. And you don't need a dedicated success jsp, the success page would just be the dashboard/homepage/welcomepage.
Here's a nice example of the above:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//get the parameters submitted by the form
String user_name = request.getParameter("username");
String user_pass = request.getParameter("password");
String url = ""; //Create a string literal that we will modify based on if the details are correct or not.
UserDao udao = new UserDao(); //class containing all the database methods pertaining to the user table.
if(udao.isValid(user_name, user_pass)){ //UserDao has a method called isValid which returns a boolean.
//details are valid so forward to success page
url = "welcome.jsp"; //set the url welcome.jsp because the details are correct.
//here you set your session variables and do whatever else you want to do
HttpSession session = (request.getSession());
session.setAttribute("username", user_name); // i can access this variable now in any jsp with: ${username}
}else{
//details are not valid so lets forward them back to the login page with an error message
String error = "Username or password is incorrect!";
request.setAttribute("error", error);
url = "login.jsp"; //set the url login.jsp because the details are incorrect.
}
RequestDispatcher rd = request.getRequestDispatcher(url); //the url we set based on if details are correct or not
rd.forward(request, response);
}
Your jsp might look something like this:
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<jsp:include flush="true" page="includes/header.jsp"></jsp:include>
<form action="../Login" method="post">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="Submit">
</form>
<!-- you can handle the error here also -->
<h1>${error}</h1>
<jsp:include flush="true" page="includes/footer.jsp"></jsp:include>
The isValid method inside the UserDao class would look something like this:
public boolean isValid(String username, String userpass){
boolean status = false;
PreparedStatement pst = null;
ResultSet rs = null;
try(Connection conn= DBConnection.getConnection()){
pst = conn.prepareStatement("select * from user where username=? and userpass=?;");
pst.setString(1, username);
pst.setString(2, userpass);
rs = pst.executeQuery();
status = rs.next();
} catch (SQLException e) {
e.printStackTrace();
}
return status;
}
Hope that helps understand it better, if you have any questions feel free to ask.
Note: one of the answers uses scriptlets in their jsp (those <% %>
things) . This is highly discouraged! If you want to start on a good path do not use scriptlets! More info on why here:
How to avoid Java code in JSP files?
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
I understand a servlet has URL mappings so that when a URL is
requested, the associated servlet takes the request, processes it and
possibly forwards to a JSP.
Correct but remember that only the doGet method is accessible directly. (i.e. you can run the doGet method of a servlet by typing it into the browser)
When I request the URL ending with ".../Login" I want to display a
form with username and password fields and a Login button.
You can use the doGet method of the Login servlet to forward to the jsp:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
RequestDispatcher rd = request.getRequestDispatcher("login.jsp");
rd.forward(request, response);
}
So you can now either access the login page form either directly /login.jsp
or via the doGet method of our Login Servlet /Login
For example you could have a link somewhere like this:
<a href="/Login">Login</a>
My question is do I need 2 URLs for this operation, one of which would
be ".../Login" and the other ".../Login/Result" for instance, and when
the button is clicked, a post request would be sent to the second one?
In that case I would need two servlets. The first one would simply
forward to the JSP which contains the form, and that form would have:
No, that would be over complicating it and would make your application harder to manage. You can use the same Login Servlet to both redirect the user to the login page (doGet) and handle the results (doPost).
When the button is clicked, I want a success or failure page to be displayed.
Instead of having a failure jsp, why not just keep it on the login page? If a user types in their password incorrectly by mistake more than once, it would be annoying for them to keep having to go back to the login page no? What most websites do is that they will show the error message on the login page itself, so they can easily just type their details in again without having to click back. And you don't need a dedicated success jsp, the success page would just be the dashboard/homepage/welcomepage.
Here's a nice example of the above:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//get the parameters submitted by the form
String user_name = request.getParameter("username");
String user_pass = request.getParameter("password");
String url = ""; //Create a string literal that we will modify based on if the details are correct or not.
UserDao udao = new UserDao(); //class containing all the database methods pertaining to the user table.
if(udao.isValid(user_name, user_pass)){ //UserDao has a method called isValid which returns a boolean.
//details are valid so forward to success page
url = "welcome.jsp"; //set the url welcome.jsp because the details are correct.
//here you set your session variables and do whatever else you want to do
HttpSession session = (request.getSession());
session.setAttribute("username", user_name); // i can access this variable now in any jsp with: ${username}
}else{
//details are not valid so lets forward them back to the login page with an error message
String error = "Username or password is incorrect!";
request.setAttribute("error", error);
url = "login.jsp"; //set the url login.jsp because the details are incorrect.
}
RequestDispatcher rd = request.getRequestDispatcher(url); //the url we set based on if details are correct or not
rd.forward(request, response);
}
Your jsp might look something like this:
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<jsp:include flush="true" page="includes/header.jsp"></jsp:include>
<form action="../Login" method="post">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="Submit">
</form>
<!-- you can handle the error here also -->
<h1>${error}</h1>
<jsp:include flush="true" page="includes/footer.jsp"></jsp:include>
The isValid method inside the UserDao class would look something like this:
public boolean isValid(String username, String userpass){
boolean status = false;
PreparedStatement pst = null;
ResultSet rs = null;
try(Connection conn= DBConnection.getConnection()){
pst = conn.prepareStatement("select * from user where username=? and userpass=?;");
pst.setString(1, username);
pst.setString(2, userpass);
rs = pst.executeQuery();
status = rs.next();
} catch (SQLException e) {
e.printStackTrace();
}
return status;
}
Hope that helps understand it better, if you have any questions feel free to ask.
Note: one of the answers uses scriptlets in their jsp (those <% %>
things) . This is highly discouraged! If you want to start on a good path do not use scriptlets! More info on why here:
How to avoid Java code in JSP files?
I understand a servlet has URL mappings so that when a URL is
requested, the associated servlet takes the request, processes it and
possibly forwards to a JSP.
Correct but remember that only the doGet method is accessible directly. (i.e. you can run the doGet method of a servlet by typing it into the browser)
When I request the URL ending with ".../Login" I want to display a
form with username and password fields and a Login button.
You can use the doGet method of the Login servlet to forward to the jsp:
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
RequestDispatcher rd = request.getRequestDispatcher("login.jsp");
rd.forward(request, response);
}
So you can now either access the login page form either directly /login.jsp
or via the doGet method of our Login Servlet /Login
For example you could have a link somewhere like this:
<a href="/Login">Login</a>
My question is do I need 2 URLs for this operation, one of which would
be ".../Login" and the other ".../Login/Result" for instance, and when
the button is clicked, a post request would be sent to the second one?
In that case I would need two servlets. The first one would simply
forward to the JSP which contains the form, and that form would have:
No, that would be over complicating it and would make your application harder to manage. You can use the same Login Servlet to both redirect the user to the login page (doGet) and handle the results (doPost).
When the button is clicked, I want a success or failure page to be displayed.
Instead of having a failure jsp, why not just keep it on the login page? If a user types in their password incorrectly by mistake more than once, it would be annoying for them to keep having to go back to the login page no? What most websites do is that they will show the error message on the login page itself, so they can easily just type their details in again without having to click back. And you don't need a dedicated success jsp, the success page would just be the dashboard/homepage/welcomepage.
Here's a nice example of the above:
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
//get the parameters submitted by the form
String user_name = request.getParameter("username");
String user_pass = request.getParameter("password");
String url = ""; //Create a string literal that we will modify based on if the details are correct or not.
UserDao udao = new UserDao(); //class containing all the database methods pertaining to the user table.
if(udao.isValid(user_name, user_pass)){ //UserDao has a method called isValid which returns a boolean.
//details are valid so forward to success page
url = "welcome.jsp"; //set the url welcome.jsp because the details are correct.
//here you set your session variables and do whatever else you want to do
HttpSession session = (request.getSession());
session.setAttribute("username", user_name); // i can access this variable now in any jsp with: ${username}
}else{
//details are not valid so lets forward them back to the login page with an error message
String error = "Username or password is incorrect!";
request.setAttribute("error", error);
url = "login.jsp"; //set the url login.jsp because the details are incorrect.
}
RequestDispatcher rd = request.getRequestDispatcher(url); //the url we set based on if details are correct or not
rd.forward(request, response);
}
Your jsp might look something like this:
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<jsp:include flush="true" page="includes/header.jsp"></jsp:include>
<form action="../Login" method="post">
<input type="text" name="username">
<input type="password" name="password">
<input type="submit" value="Submit">
</form>
<!-- you can handle the error here also -->
<h1>${error}</h1>
<jsp:include flush="true" page="includes/footer.jsp"></jsp:include>
The isValid method inside the UserDao class would look something like this:
public boolean isValid(String username, String userpass){
boolean status = false;
PreparedStatement pst = null;
ResultSet rs = null;
try(Connection conn= DBConnection.getConnection()){
pst = conn.prepareStatement("select * from user where username=? and userpass=?;");
pst.setString(1, username);
pst.setString(2, userpass);
rs = pst.executeQuery();
status = rs.next();
} catch (SQLException e) {
e.printStackTrace();
}
return status;
}
Hope that helps understand it better, if you have any questions feel free to ask.
Note: one of the answers uses scriptlets in their jsp (those <% %>
things) . This is highly discouraged! If you want to start on a good path do not use scriptlets! More info on why here:
How to avoid Java code in JSP files?
answered Nov 7 at 10:24


Jonathan Laliberte
1,6022824
1,6022824
add a comment |
add a comment |
up vote
0
down vote
As a general rule, you will want to use multiple URLs.
This is because you'll want to follow the basic idiom of POST
, REDIRECT
, GET
for the majority of your transactions. This helps reinforce the "stateless" nature of web applications.
So, for your example, you could do something like this:
GET /Login
(mapped to LoginServlet) -> dispatcher.forward("/login.jsp") which displays the login form.
The form will then:
POST /Login
with the user credentials. Note that the URL is the same but the request is different, a POST
instead of a GET
.
At this point, if the login succeeds, then the LoginServlet will send a HttpServletResponse.sendRedirect to, say, "/Welcome" (which is your WelcomeServlet).
If the login FAILS, then you need to REDIRECT to a login failure. This can be as simple as your LoginServlet with a "failed" parameter.
So, .sendRedirect("/Login?failed"). When you LoginServlet sees the "failed" parameter, it .forwards to "/loginFailed.jsp".
Why do you do this?
Simply, think of what happens should the user do a refresh button on their browser. If you just POST
and then forward to the JSP, when you refresh, the browser will POST
again (or ask if you want to submit the form again, which is annoying).
By sending the redirect, which causes a new GET, the URL changes, and it just reloads the last page they saw.
Finally, you don't want to GET
JSPs directly, but route them through your servlet layer. The servlets are the actions in the system, the JSPs are just the views. Send the requests to the servlets, let them figure out what the views are.
Once you've done this a couple times, you'll want to potentially look in to "action frameworks" which makes this type of web programming easier.
Thanks for the thorough response. I hadn't thought of using a single servlet and single URL for 2 different requests, each having its own logic, since in this case one is a get and the other one is a post.
– Andrew S.
Nov 7 at 7:03
add a comment |
up vote
0
down vote
As a general rule, you will want to use multiple URLs.
This is because you'll want to follow the basic idiom of POST
, REDIRECT
, GET
for the majority of your transactions. This helps reinforce the "stateless" nature of web applications.
So, for your example, you could do something like this:
GET /Login
(mapped to LoginServlet) -> dispatcher.forward("/login.jsp") which displays the login form.
The form will then:
POST /Login
with the user credentials. Note that the URL is the same but the request is different, a POST
instead of a GET
.
At this point, if the login succeeds, then the LoginServlet will send a HttpServletResponse.sendRedirect to, say, "/Welcome" (which is your WelcomeServlet).
If the login FAILS, then you need to REDIRECT to a login failure. This can be as simple as your LoginServlet with a "failed" parameter.
So, .sendRedirect("/Login?failed"). When you LoginServlet sees the "failed" parameter, it .forwards to "/loginFailed.jsp".
Why do you do this?
Simply, think of what happens should the user do a refresh button on their browser. If you just POST
and then forward to the JSP, when you refresh, the browser will POST
again (or ask if you want to submit the form again, which is annoying).
By sending the redirect, which causes a new GET, the URL changes, and it just reloads the last page they saw.
Finally, you don't want to GET
JSPs directly, but route them through your servlet layer. The servlets are the actions in the system, the JSPs are just the views. Send the requests to the servlets, let them figure out what the views are.
Once you've done this a couple times, you'll want to potentially look in to "action frameworks" which makes this type of web programming easier.
Thanks for the thorough response. I hadn't thought of using a single servlet and single URL for 2 different requests, each having its own logic, since in this case one is a get and the other one is a post.
– Andrew S.
Nov 7 at 7:03
add a comment |
up vote
0
down vote
up vote
0
down vote
As a general rule, you will want to use multiple URLs.
This is because you'll want to follow the basic idiom of POST
, REDIRECT
, GET
for the majority of your transactions. This helps reinforce the "stateless" nature of web applications.
So, for your example, you could do something like this:
GET /Login
(mapped to LoginServlet) -> dispatcher.forward("/login.jsp") which displays the login form.
The form will then:
POST /Login
with the user credentials. Note that the URL is the same but the request is different, a POST
instead of a GET
.
At this point, if the login succeeds, then the LoginServlet will send a HttpServletResponse.sendRedirect to, say, "/Welcome" (which is your WelcomeServlet).
If the login FAILS, then you need to REDIRECT to a login failure. This can be as simple as your LoginServlet with a "failed" parameter.
So, .sendRedirect("/Login?failed"). When you LoginServlet sees the "failed" parameter, it .forwards to "/loginFailed.jsp".
Why do you do this?
Simply, think of what happens should the user do a refresh button on their browser. If you just POST
and then forward to the JSP, when you refresh, the browser will POST
again (or ask if you want to submit the form again, which is annoying).
By sending the redirect, which causes a new GET, the URL changes, and it just reloads the last page they saw.
Finally, you don't want to GET
JSPs directly, but route them through your servlet layer. The servlets are the actions in the system, the JSPs are just the views. Send the requests to the servlets, let them figure out what the views are.
Once you've done this a couple times, you'll want to potentially look in to "action frameworks" which makes this type of web programming easier.
As a general rule, you will want to use multiple URLs.
This is because you'll want to follow the basic idiom of POST
, REDIRECT
, GET
for the majority of your transactions. This helps reinforce the "stateless" nature of web applications.
So, for your example, you could do something like this:
GET /Login
(mapped to LoginServlet) -> dispatcher.forward("/login.jsp") which displays the login form.
The form will then:
POST /Login
with the user credentials. Note that the URL is the same but the request is different, a POST
instead of a GET
.
At this point, if the login succeeds, then the LoginServlet will send a HttpServletResponse.sendRedirect to, say, "/Welcome" (which is your WelcomeServlet).
If the login FAILS, then you need to REDIRECT to a login failure. This can be as simple as your LoginServlet with a "failed" parameter.
So, .sendRedirect("/Login?failed"). When you LoginServlet sees the "failed" parameter, it .forwards to "/loginFailed.jsp".
Why do you do this?
Simply, think of what happens should the user do a refresh button on their browser. If you just POST
and then forward to the JSP, when you refresh, the browser will POST
again (or ask if you want to submit the form again, which is annoying).
By sending the redirect, which causes a new GET, the URL changes, and it just reloads the last page they saw.
Finally, you don't want to GET
JSPs directly, but route them through your servlet layer. The servlets are the actions in the system, the JSPs are just the views. Send the requests to the servlets, let them figure out what the views are.
Once you've done this a couple times, you'll want to potentially look in to "action frameworks" which makes this type of web programming easier.
answered Nov 7 at 4:49
Will Hartung
92.2k17106188
92.2k17106188
Thanks for the thorough response. I hadn't thought of using a single servlet and single URL for 2 different requests, each having its own logic, since in this case one is a get and the other one is a post.
– Andrew S.
Nov 7 at 7:03
add a comment |
Thanks for the thorough response. I hadn't thought of using a single servlet and single URL for 2 different requests, each having its own logic, since in this case one is a get and the other one is a post.
– Andrew S.
Nov 7 at 7:03
Thanks for the thorough response. I hadn't thought of using a single servlet and single URL for 2 different requests, each having its own logic, since in this case one is a get and the other one is a post.
– Andrew S.
Nov 7 at 7:03
Thanks for the thorough response. I hadn't thought of using a single servlet and single URL for 2 different requests, each having its own logic, since in this case one is a get and the other one is a post.
– Andrew S.
Nov 7 at 7:03
add a comment |
up vote
0
down vote
This is opinion based. What I would do is,
- Have a folder named
login
and have anindex.jsp
page inside it, containing the login form design (.../login/index.jsp
). So I can call.../login/
as it is not necessary to mentionindex.jsp
in the url.
Then I'll have a servlet to pass the information for verification. I would prefer the URL of the servlet to be
.../login/controller
.
form tag of the
/login/index.jsp
would look like this.
<form action="controller" method="POST">
But, you can do this even with a single jsp page. At the beginning of the page you can check whether the login information is being passed.
<%
String username = request.getParameter("username"),
password = request.getParameter("password");
if(username != null && password != null){
//validation part
response.sendRedirect("profile.jsp"); //if login successful
return; //make sure you add this after redirecting.
}
%>
<!-- html part of the login page continues -->
<form action="" method="POST">
Side note: If you want to remove the .jsp
part from your URL when sending a request to a jsp page. You can add an URL mapping for a jsp page in your deployment descriptor(web.xml
).
<servlet>
<servlet-name>Login</servlet-name>
<jsp-file>login.jsp</jsp-file>
</servlet>
<servlet-mapping>
<servlet-name>Login</servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>
Read Url Mapping For Jsp for more info.
Ok so no servlet for the first part, just a jsp page. A servlet would be needed just for taking the post request, but you're suggesting it's not even necessary and I can have the login logic inside the jsp? But isn't it better to separate code from the jsp (which is presentation)? Also, I have a stupid question: where do you put your "login" folder? Under WebContent? And is there a way for Tomcat to automatically map a newly created jsp to a url, just like for servlets it's automatically done when I use Eclipse's create New Servlet?
– Andrew S.
Nov 7 at 7:16
@AndrewS. isn't it better to separate code from the jsp? like I said it is primarily opinion-based. According to my opinion, yes it is better to have a jsp and a servlet, I was just saying that you can even do it in the same jsp page. I've updated my answer with an image of the folder tree (I'm using NetBeans, the folder name maybe different in Eclipse). Tomcat will give you an url automatically to your newly created jsp page. In my case it's../login/index.jsp
. No manual mapping is needed unless you need to get rid of that.jsp
part in the URL.
– Roshana Pitigala
Nov 7 at 8:02
add a comment |
up vote
0
down vote
This is opinion based. What I would do is,
- Have a folder named
login
and have anindex.jsp
page inside it, containing the login form design (.../login/index.jsp
). So I can call.../login/
as it is not necessary to mentionindex.jsp
in the url.
Then I'll have a servlet to pass the information for verification. I would prefer the URL of the servlet to be
.../login/controller
.
form tag of the
/login/index.jsp
would look like this.
<form action="controller" method="POST">
But, you can do this even with a single jsp page. At the beginning of the page you can check whether the login information is being passed.
<%
String username = request.getParameter("username"),
password = request.getParameter("password");
if(username != null && password != null){
//validation part
response.sendRedirect("profile.jsp"); //if login successful
return; //make sure you add this after redirecting.
}
%>
<!-- html part of the login page continues -->
<form action="" method="POST">
Side note: If you want to remove the .jsp
part from your URL when sending a request to a jsp page. You can add an URL mapping for a jsp page in your deployment descriptor(web.xml
).
<servlet>
<servlet-name>Login</servlet-name>
<jsp-file>login.jsp</jsp-file>
</servlet>
<servlet-mapping>
<servlet-name>Login</servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>
Read Url Mapping For Jsp for more info.
Ok so no servlet for the first part, just a jsp page. A servlet would be needed just for taking the post request, but you're suggesting it's not even necessary and I can have the login logic inside the jsp? But isn't it better to separate code from the jsp (which is presentation)? Also, I have a stupid question: where do you put your "login" folder? Under WebContent? And is there a way for Tomcat to automatically map a newly created jsp to a url, just like for servlets it's automatically done when I use Eclipse's create New Servlet?
– Andrew S.
Nov 7 at 7:16
@AndrewS. isn't it better to separate code from the jsp? like I said it is primarily opinion-based. According to my opinion, yes it is better to have a jsp and a servlet, I was just saying that you can even do it in the same jsp page. I've updated my answer with an image of the folder tree (I'm using NetBeans, the folder name maybe different in Eclipse). Tomcat will give you an url automatically to your newly created jsp page. In my case it's../login/index.jsp
. No manual mapping is needed unless you need to get rid of that.jsp
part in the URL.
– Roshana Pitigala
Nov 7 at 8:02
add a comment |
up vote
0
down vote
up vote
0
down vote
This is opinion based. What I would do is,
- Have a folder named
login
and have anindex.jsp
page inside it, containing the login form design (.../login/index.jsp
). So I can call.../login/
as it is not necessary to mentionindex.jsp
in the url.
Then I'll have a servlet to pass the information for verification. I would prefer the URL of the servlet to be
.../login/controller
.
form tag of the
/login/index.jsp
would look like this.
<form action="controller" method="POST">
But, you can do this even with a single jsp page. At the beginning of the page you can check whether the login information is being passed.
<%
String username = request.getParameter("username"),
password = request.getParameter("password");
if(username != null && password != null){
//validation part
response.sendRedirect("profile.jsp"); //if login successful
return; //make sure you add this after redirecting.
}
%>
<!-- html part of the login page continues -->
<form action="" method="POST">
Side note: If you want to remove the .jsp
part from your URL when sending a request to a jsp page. You can add an URL mapping for a jsp page in your deployment descriptor(web.xml
).
<servlet>
<servlet-name>Login</servlet-name>
<jsp-file>login.jsp</jsp-file>
</servlet>
<servlet-mapping>
<servlet-name>Login</servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>
Read Url Mapping For Jsp for more info.
This is opinion based. What I would do is,
- Have a folder named
login
and have anindex.jsp
page inside it, containing the login form design (.../login/index.jsp
). So I can call.../login/
as it is not necessary to mentionindex.jsp
in the url.
Then I'll have a servlet to pass the information for verification. I would prefer the URL of the servlet to be
.../login/controller
.
form tag of the
/login/index.jsp
would look like this.
<form action="controller" method="POST">
But, you can do this even with a single jsp page. At the beginning of the page you can check whether the login information is being passed.
<%
String username = request.getParameter("username"),
password = request.getParameter("password");
if(username != null && password != null){
//validation part
response.sendRedirect("profile.jsp"); //if login successful
return; //make sure you add this after redirecting.
}
%>
<!-- html part of the login page continues -->
<form action="" method="POST">
Side note: If you want to remove the .jsp
part from your URL when sending a request to a jsp page. You can add an URL mapping for a jsp page in your deployment descriptor(web.xml
).
<servlet>
<servlet-name>Login</servlet-name>
<jsp-file>login.jsp</jsp-file>
</servlet>
<servlet-mapping>
<servlet-name>Login</servlet-name>
<url-pattern>/login</url-pattern>
</servlet-mapping>
Read Url Mapping For Jsp for more info.
edited Nov 7 at 8:06
answered Nov 7 at 6:10


Roshana Pitigala
4,53562346
4,53562346
Ok so no servlet for the first part, just a jsp page. A servlet would be needed just for taking the post request, but you're suggesting it's not even necessary and I can have the login logic inside the jsp? But isn't it better to separate code from the jsp (which is presentation)? Also, I have a stupid question: where do you put your "login" folder? Under WebContent? And is there a way for Tomcat to automatically map a newly created jsp to a url, just like for servlets it's automatically done when I use Eclipse's create New Servlet?
– Andrew S.
Nov 7 at 7:16
@AndrewS. isn't it better to separate code from the jsp? like I said it is primarily opinion-based. According to my opinion, yes it is better to have a jsp and a servlet, I was just saying that you can even do it in the same jsp page. I've updated my answer with an image of the folder tree (I'm using NetBeans, the folder name maybe different in Eclipse). Tomcat will give you an url automatically to your newly created jsp page. In my case it's../login/index.jsp
. No manual mapping is needed unless you need to get rid of that.jsp
part in the URL.
– Roshana Pitigala
Nov 7 at 8:02
add a comment |
Ok so no servlet for the first part, just a jsp page. A servlet would be needed just for taking the post request, but you're suggesting it's not even necessary and I can have the login logic inside the jsp? But isn't it better to separate code from the jsp (which is presentation)? Also, I have a stupid question: where do you put your "login" folder? Under WebContent? And is there a way for Tomcat to automatically map a newly created jsp to a url, just like for servlets it's automatically done when I use Eclipse's create New Servlet?
– Andrew S.
Nov 7 at 7:16
@AndrewS. isn't it better to separate code from the jsp? like I said it is primarily opinion-based. According to my opinion, yes it is better to have a jsp and a servlet, I was just saying that you can even do it in the same jsp page. I've updated my answer with an image of the folder tree (I'm using NetBeans, the folder name maybe different in Eclipse). Tomcat will give you an url automatically to your newly created jsp page. In my case it's../login/index.jsp
. No manual mapping is needed unless you need to get rid of that.jsp
part in the URL.
– Roshana Pitigala
Nov 7 at 8:02
Ok so no servlet for the first part, just a jsp page. A servlet would be needed just for taking the post request, but you're suggesting it's not even necessary and I can have the login logic inside the jsp? But isn't it better to separate code from the jsp (which is presentation)? Also, I have a stupid question: where do you put your "login" folder? Under WebContent? And is there a way for Tomcat to automatically map a newly created jsp to a url, just like for servlets it's automatically done when I use Eclipse's create New Servlet?
– Andrew S.
Nov 7 at 7:16
Ok so no servlet for the first part, just a jsp page. A servlet would be needed just for taking the post request, but you're suggesting it's not even necessary and I can have the login logic inside the jsp? But isn't it better to separate code from the jsp (which is presentation)? Also, I have a stupid question: where do you put your "login" folder? Under WebContent? And is there a way for Tomcat to automatically map a newly created jsp to a url, just like for servlets it's automatically done when I use Eclipse's create New Servlet?
– Andrew S.
Nov 7 at 7:16
@AndrewS. isn't it better to separate code from the jsp? like I said it is primarily opinion-based. According to my opinion, yes it is better to have a jsp and a servlet, I was just saying that you can even do it in the same jsp page. I've updated my answer with an image of the folder tree (I'm using NetBeans, the folder name maybe different in Eclipse). Tomcat will give you an url automatically to your newly created jsp page. In my case it's
../login/index.jsp
. No manual mapping is needed unless you need to get rid of that .jsp
part in the URL.– Roshana Pitigala
Nov 7 at 8:02
@AndrewS. isn't it better to separate code from the jsp? like I said it is primarily opinion-based. According to my opinion, yes it is better to have a jsp and a servlet, I was just saying that you can even do it in the same jsp page. I've updated my answer with an image of the folder tree (I'm using NetBeans, the folder name maybe different in Eclipse). Tomcat will give you an url automatically to your newly created jsp page. In my case it's
../login/index.jsp
. No manual mapping is needed unless you need to get rid of that .jsp
part in the URL.– Roshana Pitigala
Nov 7 at 8:02
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53183502%2fservlets-jsps-basics-use-one-or-two-servlets-to-design-a-website-login%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
initZ8Q2UyMgM,kjDBBi7Xl2iI,g8InXwikoePhy3IexkHPlixLlM,EaI tM 0G NIiW0aS3JiNWK5DmYyHpsu3,Q ej