error:no appropriate constructor found, even though i have made one,c++
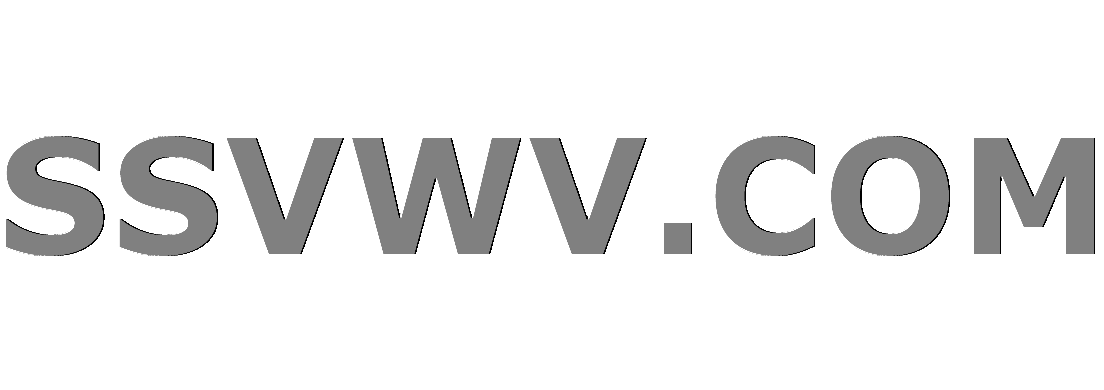
Multi tool use
up vote
2
down vote
favorite
class String
{
private:
static const int SZ = 80;
char str[SZ];
public:
String()
{
strcpy_s(str, " ");
}
String(char s)
{
strcpy_s(str, s);
}
}
This is the constructor i have written, note the second one
and here I am using it:
String s1 = "yes";
String s2 = "no";
String s3;
This is the error message :
Severity Code Description Project File Line Suppression State Error
(active) E0415 no suitable constructor exists to convert from "const
char [4]" to "String" rishabh c++ projects F:rishabh c++
projectsrishabh c++ projectsmain.cpp 35
c++
add a comment |
up vote
2
down vote
favorite
class String
{
private:
static const int SZ = 80;
char str[SZ];
public:
String()
{
strcpy_s(str, " ");
}
String(char s)
{
strcpy_s(str, s);
}
}
This is the constructor i have written, note the second one
and here I am using it:
String s1 = "yes";
String s2 = "no";
String s3;
This is the error message :
Severity Code Description Project File Line Suppression State Error
(active) E0415 no suitable constructor exists to convert from "const
char [4]" to "String" rishabh c++ projects F:rishabh c++
projectsrishabh c++ projectsmain.cpp 35
c++
5
Note the error message saidconst char[4]
.
– songyuanyao
Nov 7 at 9:39
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
class String
{
private:
static const int SZ = 80;
char str[SZ];
public:
String()
{
strcpy_s(str, " ");
}
String(char s)
{
strcpy_s(str, s);
}
}
This is the constructor i have written, note the second one
and here I am using it:
String s1 = "yes";
String s2 = "no";
String s3;
This is the error message :
Severity Code Description Project File Line Suppression State Error
(active) E0415 no suitable constructor exists to convert from "const
char [4]" to "String" rishabh c++ projects F:rishabh c++
projectsrishabh c++ projectsmain.cpp 35
c++
class String
{
private:
static const int SZ = 80;
char str[SZ];
public:
String()
{
strcpy_s(str, " ");
}
String(char s)
{
strcpy_s(str, s);
}
}
This is the constructor i have written, note the second one
and here I am using it:
String s1 = "yes";
String s2 = "no";
String s3;
This is the error message :
Severity Code Description Project File Line Suppression State Error
(active) E0415 no suitable constructor exists to convert from "const
char [4]" to "String" rishabh c++ projects F:rishabh c++
projectsrishabh c++ projectsmain.cpp 35
c++
c++
edited Nov 7 at 10:23


Luboš Turek
2,09442338
2,09442338
asked Nov 7 at 9:36
rishabh jain
133
133
5
Note the error message saidconst char[4]
.
– songyuanyao
Nov 7 at 9:39
add a comment |
5
Note the error message saidconst char[4]
.
– songyuanyao
Nov 7 at 9:39
5
5
Note the error message said
const char[4]
.– songyuanyao
Nov 7 at 9:39
Note the error message said
const char[4]
.– songyuanyao
Nov 7 at 9:39
add a comment |
1 Answer
1
active
oldest
votes
up vote
6
down vote
accepted
The compiler is telling you there is no implicit cast from your string literal to any of the available constructors. The reason behind this is the types that your class can be constructed with:
String(char s);
Note that string literals are constant, as per the C++ standard. That will not be implicitly converted to the non-constant type that your constructor requires. To do that would require const_cast
, but please don't do that!!!
You would also get an error (or at least a warning) if you attempted explicit construction:
String s1("yes"); // error
The simple fix is the change the parameter to const char
(or indeed const char*
is more commonly used).
String(const char *s)
{
strcpy_s(str, s);
}
Whenever passing pointer or reference types as parameters, always ask yourself: "does my function need to modify the value of the parameter?" -- whenever the answer is "no" make it const
.
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
6
down vote
accepted
The compiler is telling you there is no implicit cast from your string literal to any of the available constructors. The reason behind this is the types that your class can be constructed with:
String(char s);
Note that string literals are constant, as per the C++ standard. That will not be implicitly converted to the non-constant type that your constructor requires. To do that would require const_cast
, but please don't do that!!!
You would also get an error (or at least a warning) if you attempted explicit construction:
String s1("yes"); // error
The simple fix is the change the parameter to const char
(or indeed const char*
is more commonly used).
String(const char *s)
{
strcpy_s(str, s);
}
Whenever passing pointer or reference types as parameters, always ask yourself: "does my function need to modify the value of the parameter?" -- whenever the answer is "no" make it const
.
add a comment |
up vote
6
down vote
accepted
The compiler is telling you there is no implicit cast from your string literal to any of the available constructors. The reason behind this is the types that your class can be constructed with:
String(char s);
Note that string literals are constant, as per the C++ standard. That will not be implicitly converted to the non-constant type that your constructor requires. To do that would require const_cast
, but please don't do that!!!
You would also get an error (or at least a warning) if you attempted explicit construction:
String s1("yes"); // error
The simple fix is the change the parameter to const char
(or indeed const char*
is more commonly used).
String(const char *s)
{
strcpy_s(str, s);
}
Whenever passing pointer or reference types as parameters, always ask yourself: "does my function need to modify the value of the parameter?" -- whenever the answer is "no" make it const
.
add a comment |
up vote
6
down vote
accepted
up vote
6
down vote
accepted
The compiler is telling you there is no implicit cast from your string literal to any of the available constructors. The reason behind this is the types that your class can be constructed with:
String(char s);
Note that string literals are constant, as per the C++ standard. That will not be implicitly converted to the non-constant type that your constructor requires. To do that would require const_cast
, but please don't do that!!!
You would also get an error (or at least a warning) if you attempted explicit construction:
String s1("yes"); // error
The simple fix is the change the parameter to const char
(or indeed const char*
is more commonly used).
String(const char *s)
{
strcpy_s(str, s);
}
Whenever passing pointer or reference types as parameters, always ask yourself: "does my function need to modify the value of the parameter?" -- whenever the answer is "no" make it const
.
The compiler is telling you there is no implicit cast from your string literal to any of the available constructors. The reason behind this is the types that your class can be constructed with:
String(char s);
Note that string literals are constant, as per the C++ standard. That will not be implicitly converted to the non-constant type that your constructor requires. To do that would require const_cast
, but please don't do that!!!
You would also get an error (or at least a warning) if you attempted explicit construction:
String s1("yes"); // error
The simple fix is the change the parameter to const char
(or indeed const char*
is more commonly used).
String(const char *s)
{
strcpy_s(str, s);
}
Whenever passing pointer or reference types as parameters, always ask yourself: "does my function need to modify the value of the parameter?" -- whenever the answer is "no" make it const
.
answered Nov 7 at 9:50


paddy
41.8k52976
41.8k52976
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53186774%2ferrorno-appropriate-constructor-found-even-though-i-have-made-one-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
QrpWtj6tOZ qSnyrMOzsiVr1rEYZZF4EX,Bln7fFidMwTf2B i
5
Note the error message said
const char[4]
.– songyuanyao
Nov 7 at 9:39