alembic upgrade head with no output
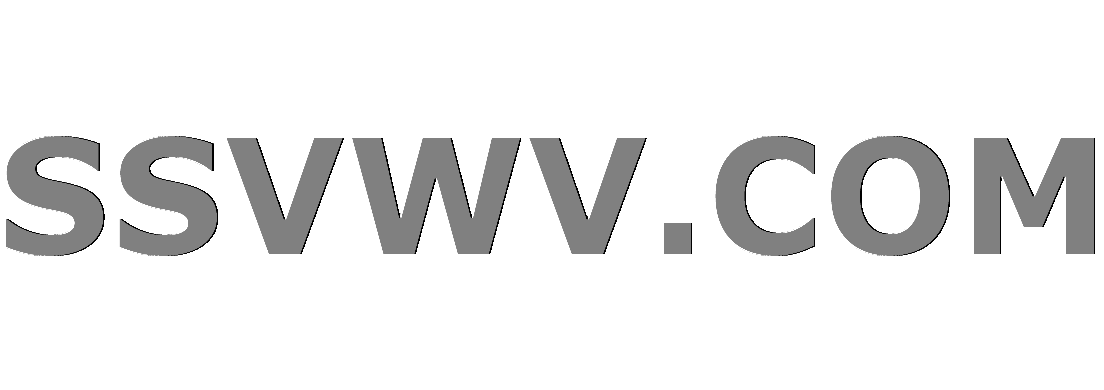
Multi tool use
up vote
0
down vote
favorite
I'm having troubles trying to upgrade the database of my Flask API.
I want to add a new column to table "persons".
I have this structure :
-- app
-- alembic
-- env.py
-- README
-- versions
-- c12d697e5535_add_a_column.py
-- common
-- __init__.py
-- base.py
-- model
-- Persons.py
-- Teams.py
-- __init__.py
-- api.py
This is base.py:
# coding=utf-8
import os
from sqlalchemy import *
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
from sqlalchemy.pool import QueuePool
def get_engine():
connection_string = os.environ['DATABASE_ENGINE'] + '://' +
os.environ['DATABASE_USERNAME'] + ':' +
os.environ['DATABASE_PASSWORD'] + '@' +
os.environ['DATABASE_SERVER'] + '/dsiapi?charset=utf8'
engine = create_engine(connection_string, pool_recycle=3600, poolclass=QueuePool)
return engine
engine = get_engine()
# use session_factory() to get a new Session
_SessionFactory = sessionmaker(bind=engine, autoflush=True, expire_on_commit = False)
Base = declarative_base()
def session_factory():
Base.metadata.create_all(engine)
return _SessionFactory()
def truncate_db():
meta = MetaData(bind=engine, reflect=True)
con = engine.connect()
trans = con.begin()
con.execute('SET FOREIGN_KEY_CHECKS = 0;')
for table in meta.sorted_tables:
con.execute(table.delete())
con.execute("ALTER TABLE "+str(table)+" AUTO_INCREMENT = 1;")
con.execute('SET FOREIGN_KEY_CHECKS = 1;')
trans.commit()
This is model.Persons.py:
# coding=utf-8
from sqlalchemy import Column, String, Date, Integer, Numeric, Enum
from common.base import Base
from sqlalchemy import create_engine, ForeignKey
from sqlalchemy.orm import relationship, deferred
from model.DictSerializable import DictSerializable
class Persons( Base, DictSerializable ):
__tablename__ = 'persons'
id = Column( Integer, primary_key = True )
name = Column( String )
firstname = Column( String )
lastname = Column( String )
fonction = Column( String )
organizations_id = Column( Integer, ForeignKey( "organizations.id" ), nullable = True )
emails = Column( String )
bitbucket_username = Column( String )
persons_teams = relationship( "Teams", secondary = 'assoc_teams_persons', back_populates = 'teams_persons' )
org = relationship( "Organizations", foreign_keys = [organizations_id] )
def __init__( self, name, firstname, lastname, fonction, organizations_id, emails, bitbucket_username):
self.name = name
self.firstname = firstname
self.lastname = lastname
self.fonction = fonction
self.organizations_id = organizations_id
self.emails = emails
self.bitbucket_username = bitbucket_username
This is env.py:
# imprt model.py
import os
import sys
MODEL_PATH = os.path.join(os.path.abspath(os.path.dirname(__file__)), "..")
sys.path.append(MODEL_PATH)
from model import *
from common.base import Base
# edit this line and pass metadata
target_metadata = Base.metadata
this is c12d697e5535_add_a_column.py:
"""Add a column
Revision ID: c12d697e5535
Revises: 20cc36b3a388
Create Date: 2018-11-08 16:14:17.219897
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers, used by Alembic.
revision = 'c12d697e5535'
down_revision = None
branch_labels = None
depends_on = None
def upgrade():
op.add_column('persons', sa.Column('iam_username', sa.String))
def downgrade():
pass
When I run : alembic upgrade head
I get nothing. the column is not added. Am I missing something here?
python sqlalchemy migration alembic
add a comment |
up vote
0
down vote
favorite
I'm having troubles trying to upgrade the database of my Flask API.
I want to add a new column to table "persons".
I have this structure :
-- app
-- alembic
-- env.py
-- README
-- versions
-- c12d697e5535_add_a_column.py
-- common
-- __init__.py
-- base.py
-- model
-- Persons.py
-- Teams.py
-- __init__.py
-- api.py
This is base.py:
# coding=utf-8
import os
from sqlalchemy import *
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
from sqlalchemy.pool import QueuePool
def get_engine():
connection_string = os.environ['DATABASE_ENGINE'] + '://' +
os.environ['DATABASE_USERNAME'] + ':' +
os.environ['DATABASE_PASSWORD'] + '@' +
os.environ['DATABASE_SERVER'] + '/dsiapi?charset=utf8'
engine = create_engine(connection_string, pool_recycle=3600, poolclass=QueuePool)
return engine
engine = get_engine()
# use session_factory() to get a new Session
_SessionFactory = sessionmaker(bind=engine, autoflush=True, expire_on_commit = False)
Base = declarative_base()
def session_factory():
Base.metadata.create_all(engine)
return _SessionFactory()
def truncate_db():
meta = MetaData(bind=engine, reflect=True)
con = engine.connect()
trans = con.begin()
con.execute('SET FOREIGN_KEY_CHECKS = 0;')
for table in meta.sorted_tables:
con.execute(table.delete())
con.execute("ALTER TABLE "+str(table)+" AUTO_INCREMENT = 1;")
con.execute('SET FOREIGN_KEY_CHECKS = 1;')
trans.commit()
This is model.Persons.py:
# coding=utf-8
from sqlalchemy import Column, String, Date, Integer, Numeric, Enum
from common.base import Base
from sqlalchemy import create_engine, ForeignKey
from sqlalchemy.orm import relationship, deferred
from model.DictSerializable import DictSerializable
class Persons( Base, DictSerializable ):
__tablename__ = 'persons'
id = Column( Integer, primary_key = True )
name = Column( String )
firstname = Column( String )
lastname = Column( String )
fonction = Column( String )
organizations_id = Column( Integer, ForeignKey( "organizations.id" ), nullable = True )
emails = Column( String )
bitbucket_username = Column( String )
persons_teams = relationship( "Teams", secondary = 'assoc_teams_persons', back_populates = 'teams_persons' )
org = relationship( "Organizations", foreign_keys = [organizations_id] )
def __init__( self, name, firstname, lastname, fonction, organizations_id, emails, bitbucket_username):
self.name = name
self.firstname = firstname
self.lastname = lastname
self.fonction = fonction
self.organizations_id = organizations_id
self.emails = emails
self.bitbucket_username = bitbucket_username
This is env.py:
# imprt model.py
import os
import sys
MODEL_PATH = os.path.join(os.path.abspath(os.path.dirname(__file__)), "..")
sys.path.append(MODEL_PATH)
from model import *
from common.base import Base
# edit this line and pass metadata
target_metadata = Base.metadata
this is c12d697e5535_add_a_column.py:
"""Add a column
Revision ID: c12d697e5535
Revises: 20cc36b3a388
Create Date: 2018-11-08 16:14:17.219897
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers, used by Alembic.
revision = 'c12d697e5535'
down_revision = None
branch_labels = None
depends_on = None
def upgrade():
op.add_column('persons', sa.Column('iam_username', sa.String))
def downgrade():
pass
When I run : alembic upgrade head
I get nothing. the column is not added. Am I missing something here?
python sqlalchemy migration alembic
Something that has happened to me multiple times: tested a migration, forgot all about it, modified it, and wondered why it does not work.
– Ilja Everilä
Nov 10 at 13:26
@IljaEverilä I don't see what you are saying ?
– Souad
Nov 12 at 8:32
Meaning: I've more or less accidentally applied an upgrade, modified it, and wondered why is it not being applied. Just something to check.
– Ilja Everilä
Nov 12 at 10:35
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I'm having troubles trying to upgrade the database of my Flask API.
I want to add a new column to table "persons".
I have this structure :
-- app
-- alembic
-- env.py
-- README
-- versions
-- c12d697e5535_add_a_column.py
-- common
-- __init__.py
-- base.py
-- model
-- Persons.py
-- Teams.py
-- __init__.py
-- api.py
This is base.py:
# coding=utf-8
import os
from sqlalchemy import *
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
from sqlalchemy.pool import QueuePool
def get_engine():
connection_string = os.environ['DATABASE_ENGINE'] + '://' +
os.environ['DATABASE_USERNAME'] + ':' +
os.environ['DATABASE_PASSWORD'] + '@' +
os.environ['DATABASE_SERVER'] + '/dsiapi?charset=utf8'
engine = create_engine(connection_string, pool_recycle=3600, poolclass=QueuePool)
return engine
engine = get_engine()
# use session_factory() to get a new Session
_SessionFactory = sessionmaker(bind=engine, autoflush=True, expire_on_commit = False)
Base = declarative_base()
def session_factory():
Base.metadata.create_all(engine)
return _SessionFactory()
def truncate_db():
meta = MetaData(bind=engine, reflect=True)
con = engine.connect()
trans = con.begin()
con.execute('SET FOREIGN_KEY_CHECKS = 0;')
for table in meta.sorted_tables:
con.execute(table.delete())
con.execute("ALTER TABLE "+str(table)+" AUTO_INCREMENT = 1;")
con.execute('SET FOREIGN_KEY_CHECKS = 1;')
trans.commit()
This is model.Persons.py:
# coding=utf-8
from sqlalchemy import Column, String, Date, Integer, Numeric, Enum
from common.base import Base
from sqlalchemy import create_engine, ForeignKey
from sqlalchemy.orm import relationship, deferred
from model.DictSerializable import DictSerializable
class Persons( Base, DictSerializable ):
__tablename__ = 'persons'
id = Column( Integer, primary_key = True )
name = Column( String )
firstname = Column( String )
lastname = Column( String )
fonction = Column( String )
organizations_id = Column( Integer, ForeignKey( "organizations.id" ), nullable = True )
emails = Column( String )
bitbucket_username = Column( String )
persons_teams = relationship( "Teams", secondary = 'assoc_teams_persons', back_populates = 'teams_persons' )
org = relationship( "Organizations", foreign_keys = [organizations_id] )
def __init__( self, name, firstname, lastname, fonction, organizations_id, emails, bitbucket_username):
self.name = name
self.firstname = firstname
self.lastname = lastname
self.fonction = fonction
self.organizations_id = organizations_id
self.emails = emails
self.bitbucket_username = bitbucket_username
This is env.py:
# imprt model.py
import os
import sys
MODEL_PATH = os.path.join(os.path.abspath(os.path.dirname(__file__)), "..")
sys.path.append(MODEL_PATH)
from model import *
from common.base import Base
# edit this line and pass metadata
target_metadata = Base.metadata
this is c12d697e5535_add_a_column.py:
"""Add a column
Revision ID: c12d697e5535
Revises: 20cc36b3a388
Create Date: 2018-11-08 16:14:17.219897
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers, used by Alembic.
revision = 'c12d697e5535'
down_revision = None
branch_labels = None
depends_on = None
def upgrade():
op.add_column('persons', sa.Column('iam_username', sa.String))
def downgrade():
pass
When I run : alembic upgrade head
I get nothing. the column is not added. Am I missing something here?
python sqlalchemy migration alembic
I'm having troubles trying to upgrade the database of my Flask API.
I want to add a new column to table "persons".
I have this structure :
-- app
-- alembic
-- env.py
-- README
-- versions
-- c12d697e5535_add_a_column.py
-- common
-- __init__.py
-- base.py
-- model
-- Persons.py
-- Teams.py
-- __init__.py
-- api.py
This is base.py:
# coding=utf-8
import os
from sqlalchemy import *
from sqlalchemy.ext.declarative import declarative_base
from sqlalchemy.orm import sessionmaker
from sqlalchemy.pool import QueuePool
def get_engine():
connection_string = os.environ['DATABASE_ENGINE'] + '://' +
os.environ['DATABASE_USERNAME'] + ':' +
os.environ['DATABASE_PASSWORD'] + '@' +
os.environ['DATABASE_SERVER'] + '/dsiapi?charset=utf8'
engine = create_engine(connection_string, pool_recycle=3600, poolclass=QueuePool)
return engine
engine = get_engine()
# use session_factory() to get a new Session
_SessionFactory = sessionmaker(bind=engine, autoflush=True, expire_on_commit = False)
Base = declarative_base()
def session_factory():
Base.metadata.create_all(engine)
return _SessionFactory()
def truncate_db():
meta = MetaData(bind=engine, reflect=True)
con = engine.connect()
trans = con.begin()
con.execute('SET FOREIGN_KEY_CHECKS = 0;')
for table in meta.sorted_tables:
con.execute(table.delete())
con.execute("ALTER TABLE "+str(table)+" AUTO_INCREMENT = 1;")
con.execute('SET FOREIGN_KEY_CHECKS = 1;')
trans.commit()
This is model.Persons.py:
# coding=utf-8
from sqlalchemy import Column, String, Date, Integer, Numeric, Enum
from common.base import Base
from sqlalchemy import create_engine, ForeignKey
from sqlalchemy.orm import relationship, deferred
from model.DictSerializable import DictSerializable
class Persons( Base, DictSerializable ):
__tablename__ = 'persons'
id = Column( Integer, primary_key = True )
name = Column( String )
firstname = Column( String )
lastname = Column( String )
fonction = Column( String )
organizations_id = Column( Integer, ForeignKey( "organizations.id" ), nullable = True )
emails = Column( String )
bitbucket_username = Column( String )
persons_teams = relationship( "Teams", secondary = 'assoc_teams_persons', back_populates = 'teams_persons' )
org = relationship( "Organizations", foreign_keys = [organizations_id] )
def __init__( self, name, firstname, lastname, fonction, organizations_id, emails, bitbucket_username):
self.name = name
self.firstname = firstname
self.lastname = lastname
self.fonction = fonction
self.organizations_id = organizations_id
self.emails = emails
self.bitbucket_username = bitbucket_username
This is env.py:
# imprt model.py
import os
import sys
MODEL_PATH = os.path.join(os.path.abspath(os.path.dirname(__file__)), "..")
sys.path.append(MODEL_PATH)
from model import *
from common.base import Base
# edit this line and pass metadata
target_metadata = Base.metadata
this is c12d697e5535_add_a_column.py:
"""Add a column
Revision ID: c12d697e5535
Revises: 20cc36b3a388
Create Date: 2018-11-08 16:14:17.219897
"""
from alembic import op
import sqlalchemy as sa
# revision identifiers, used by Alembic.
revision = 'c12d697e5535'
down_revision = None
branch_labels = None
depends_on = None
def upgrade():
op.add_column('persons', sa.Column('iam_username', sa.String))
def downgrade():
pass
When I run : alembic upgrade head
I get nothing. the column is not added. Am I missing something here?
python sqlalchemy migration alembic
python sqlalchemy migration alembic
edited Nov 9 at 9:36
asked Nov 9 at 8:09


Souad
1,81553469
1,81553469
Something that has happened to me multiple times: tested a migration, forgot all about it, modified it, and wondered why it does not work.
– Ilja Everilä
Nov 10 at 13:26
@IljaEverilä I don't see what you are saying ?
– Souad
Nov 12 at 8:32
Meaning: I've more or less accidentally applied an upgrade, modified it, and wondered why is it not being applied. Just something to check.
– Ilja Everilä
Nov 12 at 10:35
add a comment |
Something that has happened to me multiple times: tested a migration, forgot all about it, modified it, and wondered why it does not work.
– Ilja Everilä
Nov 10 at 13:26
@IljaEverilä I don't see what you are saying ?
– Souad
Nov 12 at 8:32
Meaning: I've more or less accidentally applied an upgrade, modified it, and wondered why is it not being applied. Just something to check.
– Ilja Everilä
Nov 12 at 10:35
Something that has happened to me multiple times: tested a migration, forgot all about it, modified it, and wondered why it does not work.
– Ilja Everilä
Nov 10 at 13:26
Something that has happened to me multiple times: tested a migration, forgot all about it, modified it, and wondered why it does not work.
– Ilja Everilä
Nov 10 at 13:26
@IljaEverilä I don't see what you are saying ?
– Souad
Nov 12 at 8:32
@IljaEverilä I don't see what you are saying ?
– Souad
Nov 12 at 8:32
Meaning: I've more or less accidentally applied an upgrade, modified it, and wondered why is it not being applied. Just something to check.
– Ilja Everilä
Nov 12 at 10:35
Meaning: I've more or less accidentally applied an upgrade, modified it, and wondered why is it not being applied. Just something to check.
– Ilja Everilä
Nov 12 at 10:35
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53221938%2falembic-upgrade-head-with-no-output%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g6cKaHbQtH q5L L Wn2 2OLg,J,5
Something that has happened to me multiple times: tested a migration, forgot all about it, modified it, and wondered why it does not work.
– Ilja Everilä
Nov 10 at 13:26
@IljaEverilä I don't see what you are saying ?
– Souad
Nov 12 at 8:32
Meaning: I've more or less accidentally applied an upgrade, modified it, and wondered why is it not being applied. Just something to check.
– Ilja Everilä
Nov 12 at 10:35