Can I write FileWriter and bufferedwriter in a same class in Java?
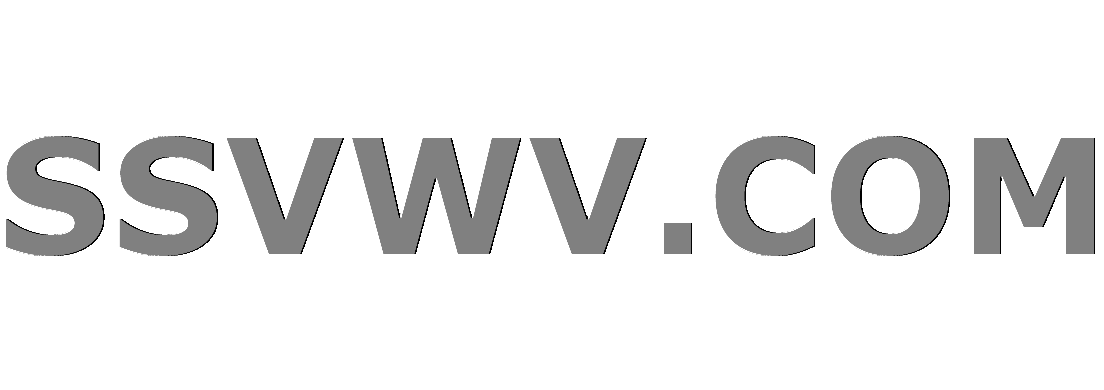
Multi tool use
up vote
0
down vote
favorite
Here is the code, in which I'm trying to write using Filewriter. This is working fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
writing.flush();
In the following code, I'm trying to write using bufferedwriter. This is not adding any text into the same file. For different file, it is working.
BufferedWriter buffwrite = new BufferedWriter(writing); buffwrite.write("java");
writing.flush();
java filewriter bufferedwriter
add a comment |
up vote
0
down vote
favorite
Here is the code, in which I'm trying to write using Filewriter. This is working fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
writing.flush();
In the following code, I'm trying to write using bufferedwriter. This is not adding any text into the same file. For different file, it is working.
BufferedWriter buffwrite = new BufferedWriter(writing); buffwrite.write("java");
writing.flush();
java filewriter bufferedwriter
2
trybuffwrite.flush()
you are flushing the FileWriter.
– Vikram Singh
Nov 9 at 4:49
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Here is the code, in which I'm trying to write using Filewriter. This is working fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
writing.flush();
In the following code, I'm trying to write using bufferedwriter. This is not adding any text into the same file. For different file, it is working.
BufferedWriter buffwrite = new BufferedWriter(writing); buffwrite.write("java");
writing.flush();
java filewriter bufferedwriter
Here is the code, in which I'm trying to write using Filewriter. This is working fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
writing.flush();
In the following code, I'm trying to write using bufferedwriter. This is not adding any text into the same file. For different file, it is working.
BufferedWriter buffwrite = new BufferedWriter(writing); buffwrite.write("java");
writing.flush();
java filewriter bufferedwriter
java filewriter bufferedwriter
edited Nov 9 at 4:45


Pang
6,8311563101
6,8311563101
asked Nov 9 at 4:33
sharan shetty
62
62
2
trybuffwrite.flush()
you are flushing the FileWriter.
– Vikram Singh
Nov 9 at 4:49
add a comment |
2
trybuffwrite.flush()
you are flushing the FileWriter.
– Vikram Singh
Nov 9 at 4:49
2
2
try
buffwrite.flush()
you are flushing the FileWriter.– Vikram Singh
Nov 9 at 4:49
try
buffwrite.flush()
you are flushing the FileWriter.– Vikram Singh
Nov 9 at 4:49
add a comment |
4 Answers
4
active
oldest
votes
up vote
1
down vote
Yes, definitely you can write both in same class.
Your code is not adding any text into the same file because you are Flushing out FileWriter before BufferedWriter. I just edited your code as below and it worked fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
BufferedWriter buffwrite = new BufferedWriter(writing);
buffwrite.write("java");
buffwrite.flush();//flush BufferedWriter first followed by FileWriter
writing.flush();
thanks for the simple solution..
– sharan shetty
Nov 22 at 18:46
add a comment |
up vote
0
down vote
These IO related classes are designed based on decorator pattern.
If you refer to the BufferedWriter
class javadoc, you'd find a constructor that takes a Writer
type object. Writer
is an abstract class which is extended by FileWriter
among other classes. Pass your FileWriter
object in the constructor and then call the write (...)
and flush
methods of BufferedWriter
.
All IO classes work in this pattern.
add a comment |
up vote
0
down vote
Yes you can write.Please checkout the below usecases for writing file in java using FileWriter, BufferedWriter, FileOutputStream and Files in java.
package com.journaldev.files;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteFile {
/**
* This class shows how to write file in java
* @param args
* @throws IOException
*/
public static void main(String args) {
String data = "I will write this String to File in Java";
int noOfLines = 10000;
writeUsingFileWriter(data);
writeUsingBufferedWriter(data, noOfLines);
writeUsingFiles(data);
writeUsingOutputStream(data);
System.out.println("DONE");
}
/**
* Use Streams when you are dealing with raw data
* @param data
*/
private static void writeUsingOutputStream(String data) {
OutputStream os = null;
try {
os = new FileOutputStream(new File("/Users/pankaj/os.txt"));
os.write(data.getBytes(), 0, data.length());
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use Files class from Java 1.7 to write files, internally uses OutputStream
* @param data
*/
private static void writeUsingFiles(String data) {
try {
Files.write(Paths.get("/Users/pankaj/files.txt"), data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* Use BufferedWriter when number of write operations are more
* It uses internal buffer to reduce real IO operations and saves time
* @param data
* @param noOfLines
*/
private static void writeUsingBufferedWriter(String data, int noOfLines) {
File file = new File("/Users/pankaj/BufferedWriter.txt");
FileWriter fr = null;
BufferedWriter br = null;
String dataWithNewLine=data+System.getProperty("line.separator");
try{
fr = new FileWriter(file);
br = new BufferedWriter(fr);
for(int i = noOfLines; i>0; i--){
br.write(dataWithNewLine);
}
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
br.close();
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use FileWriter when number of write operations are less
* @param data
*/
private static void writeUsingFileWriter(String data) {
File file = new File("/Users/pankaj/FileWriter.txt");
FileWriter fr = null;
try {
fr = new FileWriter(file);
fr.write(data);
} catch (IOException e) {
e.printStackTrace();
}finally{
//close resources
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
add a comment |
up vote
0
down vote
You should close
the resources your opened: FileWriter writing
and BufferedWriter buffwrite
. This (JavaDoc)
Flushes the stream. If the stream has saved any characters from the
various write() methods in a buffer, write them immediately to their
intended destination. Then, if that destination is another character
or byte stream, flush it. Thus one flush() invocation will flush all
the buffers in a chain of Writers and OutputStreams.
The right way is to use the try-resource statement. This will tale care to close opened resources.
Or use the java.nio.file.Files.write
methods which will do the resource handling.
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
Yes, definitely you can write both in same class.
Your code is not adding any text into the same file because you are Flushing out FileWriter before BufferedWriter. I just edited your code as below and it worked fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
BufferedWriter buffwrite = new BufferedWriter(writing);
buffwrite.write("java");
buffwrite.flush();//flush BufferedWriter first followed by FileWriter
writing.flush();
thanks for the simple solution..
– sharan shetty
Nov 22 at 18:46
add a comment |
up vote
1
down vote
Yes, definitely you can write both in same class.
Your code is not adding any text into the same file because you are Flushing out FileWriter before BufferedWriter. I just edited your code as below and it worked fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
BufferedWriter buffwrite = new BufferedWriter(writing);
buffwrite.write("java");
buffwrite.flush();//flush BufferedWriter first followed by FileWriter
writing.flush();
thanks for the simple solution..
– sharan shetty
Nov 22 at 18:46
add a comment |
up vote
1
down vote
up vote
1
down vote
Yes, definitely you can write both in same class.
Your code is not adding any text into the same file because you are Flushing out FileWriter before BufferedWriter. I just edited your code as below and it worked fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
BufferedWriter buffwrite = new BufferedWriter(writing);
buffwrite.write("java");
buffwrite.flush();//flush BufferedWriter first followed by FileWriter
writing.flush();
Yes, definitely you can write both in same class.
Your code is not adding any text into the same file because you are Flushing out FileWriter before BufferedWriter. I just edited your code as below and it worked fine.
File f2 = new File("Path");
f2.createNewFile();
FileWriter writing = new FileWriter(f2);
writing.write("i'm into you , i'm into you");
BufferedWriter buffwrite = new BufferedWriter(writing);
buffwrite.write("java");
buffwrite.flush();//flush BufferedWriter first followed by FileWriter
writing.flush();
answered Nov 9 at 6:30


Ameera Najah Kv
94
94
thanks for the simple solution..
– sharan shetty
Nov 22 at 18:46
add a comment |
thanks for the simple solution..
– sharan shetty
Nov 22 at 18:46
thanks for the simple solution..
– sharan shetty
Nov 22 at 18:46
thanks for the simple solution..
– sharan shetty
Nov 22 at 18:46
add a comment |
up vote
0
down vote
These IO related classes are designed based on decorator pattern.
If you refer to the BufferedWriter
class javadoc, you'd find a constructor that takes a Writer
type object. Writer
is an abstract class which is extended by FileWriter
among other classes. Pass your FileWriter
object in the constructor and then call the write (...)
and flush
methods of BufferedWriter
.
All IO classes work in this pattern.
add a comment |
up vote
0
down vote
These IO related classes are designed based on decorator pattern.
If you refer to the BufferedWriter
class javadoc, you'd find a constructor that takes a Writer
type object. Writer
is an abstract class which is extended by FileWriter
among other classes. Pass your FileWriter
object in the constructor and then call the write (...)
and flush
methods of BufferedWriter
.
All IO classes work in this pattern.
add a comment |
up vote
0
down vote
up vote
0
down vote
These IO related classes are designed based on decorator pattern.
If you refer to the BufferedWriter
class javadoc, you'd find a constructor that takes a Writer
type object. Writer
is an abstract class which is extended by FileWriter
among other classes. Pass your FileWriter
object in the constructor and then call the write (...)
and flush
methods of BufferedWriter
.
All IO classes work in this pattern.
These IO related classes are designed based on decorator pattern.
If you refer to the BufferedWriter
class javadoc, you'd find a constructor that takes a Writer
type object. Writer
is an abstract class which is extended by FileWriter
among other classes. Pass your FileWriter
object in the constructor and then call the write (...)
and flush
methods of BufferedWriter
.
All IO classes work in this pattern.
answered Nov 9 at 4:46


Saptarshi Basu
1,0641220
1,0641220
add a comment |
add a comment |
up vote
0
down vote
Yes you can write.Please checkout the below usecases for writing file in java using FileWriter, BufferedWriter, FileOutputStream and Files in java.
package com.journaldev.files;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteFile {
/**
* This class shows how to write file in java
* @param args
* @throws IOException
*/
public static void main(String args) {
String data = "I will write this String to File in Java";
int noOfLines = 10000;
writeUsingFileWriter(data);
writeUsingBufferedWriter(data, noOfLines);
writeUsingFiles(data);
writeUsingOutputStream(data);
System.out.println("DONE");
}
/**
* Use Streams when you are dealing with raw data
* @param data
*/
private static void writeUsingOutputStream(String data) {
OutputStream os = null;
try {
os = new FileOutputStream(new File("/Users/pankaj/os.txt"));
os.write(data.getBytes(), 0, data.length());
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use Files class from Java 1.7 to write files, internally uses OutputStream
* @param data
*/
private static void writeUsingFiles(String data) {
try {
Files.write(Paths.get("/Users/pankaj/files.txt"), data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* Use BufferedWriter when number of write operations are more
* It uses internal buffer to reduce real IO operations and saves time
* @param data
* @param noOfLines
*/
private static void writeUsingBufferedWriter(String data, int noOfLines) {
File file = new File("/Users/pankaj/BufferedWriter.txt");
FileWriter fr = null;
BufferedWriter br = null;
String dataWithNewLine=data+System.getProperty("line.separator");
try{
fr = new FileWriter(file);
br = new BufferedWriter(fr);
for(int i = noOfLines; i>0; i--){
br.write(dataWithNewLine);
}
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
br.close();
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use FileWriter when number of write operations are less
* @param data
*/
private static void writeUsingFileWriter(String data) {
File file = new File("/Users/pankaj/FileWriter.txt");
FileWriter fr = null;
try {
fr = new FileWriter(file);
fr.write(data);
} catch (IOException e) {
e.printStackTrace();
}finally{
//close resources
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
add a comment |
up vote
0
down vote
Yes you can write.Please checkout the below usecases for writing file in java using FileWriter, BufferedWriter, FileOutputStream and Files in java.
package com.journaldev.files;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteFile {
/**
* This class shows how to write file in java
* @param args
* @throws IOException
*/
public static void main(String args) {
String data = "I will write this String to File in Java";
int noOfLines = 10000;
writeUsingFileWriter(data);
writeUsingBufferedWriter(data, noOfLines);
writeUsingFiles(data);
writeUsingOutputStream(data);
System.out.println("DONE");
}
/**
* Use Streams when you are dealing with raw data
* @param data
*/
private static void writeUsingOutputStream(String data) {
OutputStream os = null;
try {
os = new FileOutputStream(new File("/Users/pankaj/os.txt"));
os.write(data.getBytes(), 0, data.length());
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use Files class from Java 1.7 to write files, internally uses OutputStream
* @param data
*/
private static void writeUsingFiles(String data) {
try {
Files.write(Paths.get("/Users/pankaj/files.txt"), data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* Use BufferedWriter when number of write operations are more
* It uses internal buffer to reduce real IO operations and saves time
* @param data
* @param noOfLines
*/
private static void writeUsingBufferedWriter(String data, int noOfLines) {
File file = new File("/Users/pankaj/BufferedWriter.txt");
FileWriter fr = null;
BufferedWriter br = null;
String dataWithNewLine=data+System.getProperty("line.separator");
try{
fr = new FileWriter(file);
br = new BufferedWriter(fr);
for(int i = noOfLines; i>0; i--){
br.write(dataWithNewLine);
}
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
br.close();
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use FileWriter when number of write operations are less
* @param data
*/
private static void writeUsingFileWriter(String data) {
File file = new File("/Users/pankaj/FileWriter.txt");
FileWriter fr = null;
try {
fr = new FileWriter(file);
fr.write(data);
} catch (IOException e) {
e.printStackTrace();
}finally{
//close resources
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
add a comment |
up vote
0
down vote
up vote
0
down vote
Yes you can write.Please checkout the below usecases for writing file in java using FileWriter, BufferedWriter, FileOutputStream and Files in java.
package com.journaldev.files;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteFile {
/**
* This class shows how to write file in java
* @param args
* @throws IOException
*/
public static void main(String args) {
String data = "I will write this String to File in Java";
int noOfLines = 10000;
writeUsingFileWriter(data);
writeUsingBufferedWriter(data, noOfLines);
writeUsingFiles(data);
writeUsingOutputStream(data);
System.out.println("DONE");
}
/**
* Use Streams when you are dealing with raw data
* @param data
*/
private static void writeUsingOutputStream(String data) {
OutputStream os = null;
try {
os = new FileOutputStream(new File("/Users/pankaj/os.txt"));
os.write(data.getBytes(), 0, data.length());
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use Files class from Java 1.7 to write files, internally uses OutputStream
* @param data
*/
private static void writeUsingFiles(String data) {
try {
Files.write(Paths.get("/Users/pankaj/files.txt"), data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* Use BufferedWriter when number of write operations are more
* It uses internal buffer to reduce real IO operations and saves time
* @param data
* @param noOfLines
*/
private static void writeUsingBufferedWriter(String data, int noOfLines) {
File file = new File("/Users/pankaj/BufferedWriter.txt");
FileWriter fr = null;
BufferedWriter br = null;
String dataWithNewLine=data+System.getProperty("line.separator");
try{
fr = new FileWriter(file);
br = new BufferedWriter(fr);
for(int i = noOfLines; i>0; i--){
br.write(dataWithNewLine);
}
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
br.close();
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use FileWriter when number of write operations are less
* @param data
*/
private static void writeUsingFileWriter(String data) {
File file = new File("/Users/pankaj/FileWriter.txt");
FileWriter fr = null;
try {
fr = new FileWriter(file);
fr.write(data);
} catch (IOException e) {
e.printStackTrace();
}finally{
//close resources
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Yes you can write.Please checkout the below usecases for writing file in java using FileWriter, BufferedWriter, FileOutputStream and Files in java.
package com.journaldev.files;
import java.io.BufferedWriter;
import java.io.File;
import java.io.FileOutputStream;
import java.io.FileWriter;
import java.io.IOException;
import java.io.OutputStream;
import java.nio.file.Files;
import java.nio.file.Paths;
public class WriteFile {
/**
* This class shows how to write file in java
* @param args
* @throws IOException
*/
public static void main(String args) {
String data = "I will write this String to File in Java";
int noOfLines = 10000;
writeUsingFileWriter(data);
writeUsingBufferedWriter(data, noOfLines);
writeUsingFiles(data);
writeUsingOutputStream(data);
System.out.println("DONE");
}
/**
* Use Streams when you are dealing with raw data
* @param data
*/
private static void writeUsingOutputStream(String data) {
OutputStream os = null;
try {
os = new FileOutputStream(new File("/Users/pankaj/os.txt"));
os.write(data.getBytes(), 0, data.length());
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use Files class from Java 1.7 to write files, internally uses OutputStream
* @param data
*/
private static void writeUsingFiles(String data) {
try {
Files.write(Paths.get("/Users/pankaj/files.txt"), data.getBytes());
} catch (IOException e) {
e.printStackTrace();
}
}
/**
* Use BufferedWriter when number of write operations are more
* It uses internal buffer to reduce real IO operations and saves time
* @param data
* @param noOfLines
*/
private static void writeUsingBufferedWriter(String data, int noOfLines) {
File file = new File("/Users/pankaj/BufferedWriter.txt");
FileWriter fr = null;
BufferedWriter br = null;
String dataWithNewLine=data+System.getProperty("line.separator");
try{
fr = new FileWriter(file);
br = new BufferedWriter(fr);
for(int i = noOfLines; i>0; i--){
br.write(dataWithNewLine);
}
} catch (IOException e) {
e.printStackTrace();
}finally{
try {
br.close();
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
/**
* Use FileWriter when number of write operations are less
* @param data
*/
private static void writeUsingFileWriter(String data) {
File file = new File("/Users/pankaj/FileWriter.txt");
FileWriter fr = null;
try {
fr = new FileWriter(file);
fr.write(data);
} catch (IOException e) {
e.printStackTrace();
}finally{
//close resources
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
answered Nov 9 at 4:47


Aman sharma
776
776
add a comment |
add a comment |
up vote
0
down vote
You should close
the resources your opened: FileWriter writing
and BufferedWriter buffwrite
. This (JavaDoc)
Flushes the stream. If the stream has saved any characters from the
various write() methods in a buffer, write them immediately to their
intended destination. Then, if that destination is another character
or byte stream, flush it. Thus one flush() invocation will flush all
the buffers in a chain of Writers and OutputStreams.
The right way is to use the try-resource statement. This will tale care to close opened resources.
Or use the java.nio.file.Files.write
methods which will do the resource handling.
add a comment |
up vote
0
down vote
You should close
the resources your opened: FileWriter writing
and BufferedWriter buffwrite
. This (JavaDoc)
Flushes the stream. If the stream has saved any characters from the
various write() methods in a buffer, write them immediately to their
intended destination. Then, if that destination is another character
or byte stream, flush it. Thus one flush() invocation will flush all
the buffers in a chain of Writers and OutputStreams.
The right way is to use the try-resource statement. This will tale care to close opened resources.
Or use the java.nio.file.Files.write
methods which will do the resource handling.
add a comment |
up vote
0
down vote
up vote
0
down vote
You should close
the resources your opened: FileWriter writing
and BufferedWriter buffwrite
. This (JavaDoc)
Flushes the stream. If the stream has saved any characters from the
various write() methods in a buffer, write them immediately to their
intended destination. Then, if that destination is another character
or byte stream, flush it. Thus one flush() invocation will flush all
the buffers in a chain of Writers and OutputStreams.
The right way is to use the try-resource statement. This will tale care to close opened resources.
Or use the java.nio.file.Files.write
methods which will do the resource handling.
You should close
the resources your opened: FileWriter writing
and BufferedWriter buffwrite
. This (JavaDoc)
Flushes the stream. If the stream has saved any characters from the
various write() methods in a buffer, write them immediately to their
intended destination. Then, if that destination is another character
or byte stream, flush it. Thus one flush() invocation will flush all
the buffers in a chain of Writers and OutputStreams.
The right way is to use the try-resource statement. This will tale care to close opened resources.
Or use the java.nio.file.Files.write
methods which will do the resource handling.
edited Nov 9 at 8:03
answered Nov 9 at 7:26
LuCio
2,6441722
2,6441722
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53219926%2fcan-i-write-filewriter-and-bufferedwriter-in-a-same-class-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
RC XJZ,hFUNdyUuggsMLjW,QSeFpDafUAJ
2
try
buffwrite.flush()
you are flushing the FileWriter.– Vikram Singh
Nov 9 at 4:49