How to add an ImageView outside and to the left of a Card?
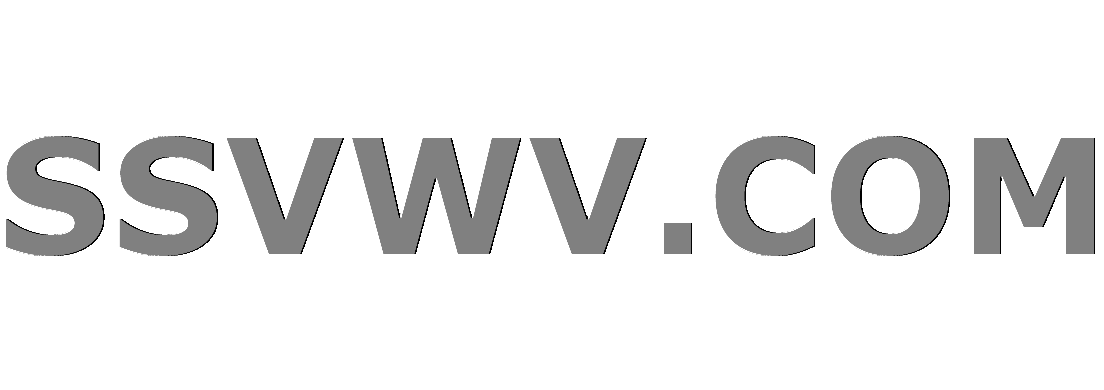
Multi tool use
up vote
0
down vote
favorite
This is what I'm trying to achieve
And this is what I have so far and the code below is the code for this screenshot
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:layout_width="270dp"
android:layout_height="wrap_content"
android:layout_gravity="right"
android:layout_margin="10dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="80dp"
android:layout_height="80dp"
android:layout_centerVertical="true" />
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:layout_toRightOf="@id/imageView"
android:textColor="#000000"
android:textSize="40sp" />
</RelativeLayout>
</android.support.v7.widget.CardView>
Basically, I can't figure out how to get the image outside but still touching the card. Any help is appreciated, thanks.

add a comment |
up vote
0
down vote
favorite
This is what I'm trying to achieve
And this is what I have so far and the code below is the code for this screenshot
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:layout_width="270dp"
android:layout_height="wrap_content"
android:layout_gravity="right"
android:layout_margin="10dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="80dp"
android:layout_height="80dp"
android:layout_centerVertical="true" />
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:layout_toRightOf="@id/imageView"
android:textColor="#000000"
android:textSize="40sp" />
</RelativeLayout>
</android.support.v7.widget.CardView>
Basically, I can't figure out how to get the image outside but still touching the card. Any help is appreciated, thanks.

add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
This is what I'm trying to achieve
And this is what I have so far and the code below is the code for this screenshot
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:layout_width="270dp"
android:layout_height="wrap_content"
android:layout_gravity="right"
android:layout_margin="10dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="80dp"
android:layout_height="80dp"
android:layout_centerVertical="true" />
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:layout_toRightOf="@id/imageView"
android:textColor="#000000"
android:textSize="40sp" />
</RelativeLayout>
</android.support.v7.widget.CardView>
Basically, I can't figure out how to get the image outside but still touching the card. Any help is appreciated, thanks.

This is what I'm trying to achieve
And this is what I have so far and the code below is the code for this screenshot
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<android.support.v7.widget.CardView
android:layout_width="270dp"
android:layout_height="wrap_content"
android:layout_gravity="right"
android:layout_margin="10dp">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:padding="16dp">
<ImageView
android:id="@+id/imageView"
android:layout_width="80dp"
android:layout_height="80dp"
android:layout_centerVertical="true" />
<TextView
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:layout_toRightOf="@id/imageView"
android:textColor="#000000"
android:textSize="40sp" />
</RelativeLayout>
</android.support.v7.widget.CardView>
Basically, I can't figure out how to get the image outside but still touching the card. Any help is appreciated, thanks.


edited Nov 9 at 3:19


Ayush Khare
1,101620
1,101620
asked Nov 9 at 3:15


Sixteen
104
104
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
accepted
You can use FrameLayout
and elevation
to fulfill your target.
According to the documentation of FrameLayout
Child views are drawn in a stack, with the most recently added child
on top
You need to play with the elevation of the ImageView
to bring it above the CardView
.
This is what I have achieved: You can then modify your paddings/margins according to design.
Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
app:cardCornerRadius="4dp"
app:cardBackgroundColor="@android:color/holo_blue_bright"
android:layout_width="308dp"
android:layout_height="112dp"
android:layout_gravity="right"
android:layout_margin="10dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_marginStart="40dp"
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:paddingLeft="8dp"
android:text="EARTH"
android:textColor="#000000"
android:textSize="70sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
<ImageView
android:src="@drawable/planet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:elevation="2dp" />
</FrameLayout>
</LinearLayout>
Thanks, but I changed one line in your code so it could work with my app which was I replaced android:src="@drawable/planet" with android:id="@+id/imageView" and when I run the app it now only displays one card! How would this work with lots of cards?
– Sixteen
Nov 9 at 5:30
You can very well keep the android:id="@+id/imageView" to have a handle to the image view. My android:src="@drawable/planet" was just a static place holder drawable (the pink circle image) for the image view. These two are entirely different. You can programmatically set the drawable/image resource on the imageView later in your code. This layout is a child layout of your recyclerView. You have to have a adapter for populating your recyclerView with a number of items
– mukut bhattacharjee
Nov 9 at 5:52
add a comment |
up vote
0
down vote
Try this layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:card_view="http://schemas.android.com/apk/res-auto"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
android:id="@+id/first"
app:cardBackgroundColor="@android:color/white"
app:cardCornerRadius="@dimen/cardview_default_radius"
app:cardElevation="4dp"
android:layout_width="match_parent"
android:layout_height="80dp"
android:layout_marginLeft="50dp"
card_view:cardUseCompatPadding="true">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="32dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="TEXT"
android:textSize="18sp"/>
</RelativeLayout>
</android.support.v7.widget.CardView>
</FrameLayout>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_marginLeft="32dp"
android:background="#000000"
android:layout_marginTop="15dp"
android:contentDescription="@null"/>
</RelativeLayout>
Result:
add a comment |
up vote
0
down vote
You can use ConstraintLayout cool property for doing this easily
Xml snippet :
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_margin="10dp"
app:cardUseCompatPadding="true">
<android.support.v7.widget.CardView
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="35dp"
app:cardBackgroundColor="@color/cardview_dark_background"
app:cardUseCompatPadding="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="70dp"
android:layout_height="70dp"
android:src="@android:drawable/ic_dialog_info"
android:translationZ="2dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
Output View :
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
You can use FrameLayout
and elevation
to fulfill your target.
According to the documentation of FrameLayout
Child views are drawn in a stack, with the most recently added child
on top
You need to play with the elevation of the ImageView
to bring it above the CardView
.
This is what I have achieved: You can then modify your paddings/margins according to design.
Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
app:cardCornerRadius="4dp"
app:cardBackgroundColor="@android:color/holo_blue_bright"
android:layout_width="308dp"
android:layout_height="112dp"
android:layout_gravity="right"
android:layout_margin="10dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_marginStart="40dp"
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:paddingLeft="8dp"
android:text="EARTH"
android:textColor="#000000"
android:textSize="70sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
<ImageView
android:src="@drawable/planet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:elevation="2dp" />
</FrameLayout>
</LinearLayout>
Thanks, but I changed one line in your code so it could work with my app which was I replaced android:src="@drawable/planet" with android:id="@+id/imageView" and when I run the app it now only displays one card! How would this work with lots of cards?
– Sixteen
Nov 9 at 5:30
You can very well keep the android:id="@+id/imageView" to have a handle to the image view. My android:src="@drawable/planet" was just a static place holder drawable (the pink circle image) for the image view. These two are entirely different. You can programmatically set the drawable/image resource on the imageView later in your code. This layout is a child layout of your recyclerView. You have to have a adapter for populating your recyclerView with a number of items
– mukut bhattacharjee
Nov 9 at 5:52
add a comment |
up vote
1
down vote
accepted
You can use FrameLayout
and elevation
to fulfill your target.
According to the documentation of FrameLayout
Child views are drawn in a stack, with the most recently added child
on top
You need to play with the elevation of the ImageView
to bring it above the CardView
.
This is what I have achieved: You can then modify your paddings/margins according to design.
Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
app:cardCornerRadius="4dp"
app:cardBackgroundColor="@android:color/holo_blue_bright"
android:layout_width="308dp"
android:layout_height="112dp"
android:layout_gravity="right"
android:layout_margin="10dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_marginStart="40dp"
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:paddingLeft="8dp"
android:text="EARTH"
android:textColor="#000000"
android:textSize="70sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
<ImageView
android:src="@drawable/planet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:elevation="2dp" />
</FrameLayout>
</LinearLayout>
Thanks, but I changed one line in your code so it could work with my app which was I replaced android:src="@drawable/planet" with android:id="@+id/imageView" and when I run the app it now only displays one card! How would this work with lots of cards?
– Sixteen
Nov 9 at 5:30
You can very well keep the android:id="@+id/imageView" to have a handle to the image view. My android:src="@drawable/planet" was just a static place holder drawable (the pink circle image) for the image view. These two are entirely different. You can programmatically set the drawable/image resource on the imageView later in your code. This layout is a child layout of your recyclerView. You have to have a adapter for populating your recyclerView with a number of items
– mukut bhattacharjee
Nov 9 at 5:52
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
You can use FrameLayout
and elevation
to fulfill your target.
According to the documentation of FrameLayout
Child views are drawn in a stack, with the most recently added child
on top
You need to play with the elevation of the ImageView
to bring it above the CardView
.
This is what I have achieved: You can then modify your paddings/margins according to design.
Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
app:cardCornerRadius="4dp"
app:cardBackgroundColor="@android:color/holo_blue_bright"
android:layout_width="308dp"
android:layout_height="112dp"
android:layout_gravity="right"
android:layout_margin="10dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_marginStart="40dp"
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:paddingLeft="8dp"
android:text="EARTH"
android:textColor="#000000"
android:textSize="70sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
<ImageView
android:src="@drawable/planet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:elevation="2dp" />
</FrameLayout>
</LinearLayout>
You can use FrameLayout
and elevation
to fulfill your target.
According to the documentation of FrameLayout
Child views are drawn in a stack, with the most recently added child
on top
You need to play with the elevation of the ImageView
to bring it above the CardView
.
This is what I have achieved: You can then modify your paddings/margins according to design.
Code:
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:orientation="vertical">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
app:cardCornerRadius="4dp"
app:cardBackgroundColor="@android:color/holo_blue_bright"
android:layout_width="308dp"
android:layout_height="112dp"
android:layout_gravity="right"
android:layout_margin="10dp">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical">
<TextView
android:layout_marginStart="40dp"
android:id="@+id/title"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="5dp"
android:paddingLeft="8dp"
android:text="EARTH"
android:textColor="#000000"
android:textSize="70sp" />
</LinearLayout>
</android.support.v7.widget.CardView>
<ImageView
android:src="@drawable/planet"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_margin="16dp"
android:elevation="2dp" />
</FrameLayout>
</LinearLayout>
edited Nov 9 at 4:22
answered Nov 9 at 4:01


mukut bhattacharjee
877
877
Thanks, but I changed one line in your code so it could work with my app which was I replaced android:src="@drawable/planet" with android:id="@+id/imageView" and when I run the app it now only displays one card! How would this work with lots of cards?
– Sixteen
Nov 9 at 5:30
You can very well keep the android:id="@+id/imageView" to have a handle to the image view. My android:src="@drawable/planet" was just a static place holder drawable (the pink circle image) for the image view. These two are entirely different. You can programmatically set the drawable/image resource on the imageView later in your code. This layout is a child layout of your recyclerView. You have to have a adapter for populating your recyclerView with a number of items
– mukut bhattacharjee
Nov 9 at 5:52
add a comment |
Thanks, but I changed one line in your code so it could work with my app which was I replaced android:src="@drawable/planet" with android:id="@+id/imageView" and when I run the app it now only displays one card! How would this work with lots of cards?
– Sixteen
Nov 9 at 5:30
You can very well keep the android:id="@+id/imageView" to have a handle to the image view. My android:src="@drawable/planet" was just a static place holder drawable (the pink circle image) for the image view. These two are entirely different. You can programmatically set the drawable/image resource on the imageView later in your code. This layout is a child layout of your recyclerView. You have to have a adapter for populating your recyclerView with a number of items
– mukut bhattacharjee
Nov 9 at 5:52
Thanks, but I changed one line in your code so it could work with my app which was I replaced android:src="@drawable/planet" with android:id="@+id/imageView" and when I run the app it now only displays one card! How would this work with lots of cards?
– Sixteen
Nov 9 at 5:30
Thanks, but I changed one line in your code so it could work with my app which was I replaced android:src="@drawable/planet" with android:id="@+id/imageView" and when I run the app it now only displays one card! How would this work with lots of cards?
– Sixteen
Nov 9 at 5:30
You can very well keep the android:id="@+id/imageView" to have a handle to the image view. My android:src="@drawable/planet" was just a static place holder drawable (the pink circle image) for the image view. These two are entirely different. You can programmatically set the drawable/image resource on the imageView later in your code. This layout is a child layout of your recyclerView. You have to have a adapter for populating your recyclerView with a number of items
– mukut bhattacharjee
Nov 9 at 5:52
You can very well keep the android:id="@+id/imageView" to have a handle to the image view. My android:src="@drawable/planet" was just a static place holder drawable (the pink circle image) for the image view. These two are entirely different. You can programmatically set the drawable/image resource on the imageView later in your code. This layout is a child layout of your recyclerView. You have to have a adapter for populating your recyclerView with a number of items
– mukut bhattacharjee
Nov 9 at 5:52
add a comment |
up vote
0
down vote
Try this layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:card_view="http://schemas.android.com/apk/res-auto"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
android:id="@+id/first"
app:cardBackgroundColor="@android:color/white"
app:cardCornerRadius="@dimen/cardview_default_radius"
app:cardElevation="4dp"
android:layout_width="match_parent"
android:layout_height="80dp"
android:layout_marginLeft="50dp"
card_view:cardUseCompatPadding="true">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="32dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="TEXT"
android:textSize="18sp"/>
</RelativeLayout>
</android.support.v7.widget.CardView>
</FrameLayout>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_marginLeft="32dp"
android:background="#000000"
android:layout_marginTop="15dp"
android:contentDescription="@null"/>
</RelativeLayout>
Result:
add a comment |
up vote
0
down vote
Try this layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:card_view="http://schemas.android.com/apk/res-auto"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
android:id="@+id/first"
app:cardBackgroundColor="@android:color/white"
app:cardCornerRadius="@dimen/cardview_default_radius"
app:cardElevation="4dp"
android:layout_width="match_parent"
android:layout_height="80dp"
android:layout_marginLeft="50dp"
card_view:cardUseCompatPadding="true">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="32dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="TEXT"
android:textSize="18sp"/>
</RelativeLayout>
</android.support.v7.widget.CardView>
</FrameLayout>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_marginLeft="32dp"
android:background="#000000"
android:layout_marginTop="15dp"
android:contentDescription="@null"/>
</RelativeLayout>
Result:
add a comment |
up vote
0
down vote
up vote
0
down vote
Try this layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:card_view="http://schemas.android.com/apk/res-auto"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
android:id="@+id/first"
app:cardBackgroundColor="@android:color/white"
app:cardCornerRadius="@dimen/cardview_default_radius"
app:cardElevation="4dp"
android:layout_width="match_parent"
android:layout_height="80dp"
android:layout_marginLeft="50dp"
card_view:cardUseCompatPadding="true">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="32dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="TEXT"
android:textSize="18sp"/>
</RelativeLayout>
</android.support.v7.widget.CardView>
</FrameLayout>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_marginLeft="32dp"
android:background="#000000"
android:layout_marginTop="15dp"
android:contentDescription="@null"/>
</RelativeLayout>
Result:
Try this layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:card_view="http://schemas.android.com/apk/res-auto"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent">
<FrameLayout
android:layout_width="match_parent"
android:layout_height="wrap_content">
<android.support.v7.widget.CardView
android:id="@+id/first"
app:cardBackgroundColor="@android:color/white"
app:cardCornerRadius="@dimen/cardview_default_radius"
app:cardElevation="4dp"
android:layout_width="match_parent"
android:layout_height="80dp"
android:layout_marginLeft="50dp"
card_view:cardUseCompatPadding="true">
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginLeft="32dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="TEXT"
android:textSize="18sp"/>
</RelativeLayout>
</android.support.v7.widget.CardView>
</FrameLayout>
<ImageView
android:layout_width="50dp"
android:layout_height="50dp"
android:layout_marginLeft="32dp"
android:background="#000000"
android:layout_marginTop="15dp"
android:contentDescription="@null"/>
</RelativeLayout>
Result:
answered Nov 9 at 3:43


Ayush Khare
1,101620
1,101620
add a comment |
add a comment |
up vote
0
down vote
You can use ConstraintLayout cool property for doing this easily
Xml snippet :
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_margin="10dp"
app:cardUseCompatPadding="true">
<android.support.v7.widget.CardView
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="35dp"
app:cardBackgroundColor="@color/cardview_dark_background"
app:cardUseCompatPadding="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="70dp"
android:layout_height="70dp"
android:src="@android:drawable/ic_dialog_info"
android:translationZ="2dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
Output View :
add a comment |
up vote
0
down vote
You can use ConstraintLayout cool property for doing this easily
Xml snippet :
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_margin="10dp"
app:cardUseCompatPadding="true">
<android.support.v7.widget.CardView
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="35dp"
app:cardBackgroundColor="@color/cardview_dark_background"
app:cardUseCompatPadding="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="70dp"
android:layout_height="70dp"
android:src="@android:drawable/ic_dialog_info"
android:translationZ="2dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
Output View :
add a comment |
up vote
0
down vote
up vote
0
down vote
You can use ConstraintLayout cool property for doing this easily
Xml snippet :
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_margin="10dp"
app:cardUseCompatPadding="true">
<android.support.v7.widget.CardView
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="35dp"
app:cardBackgroundColor="@color/cardview_dark_background"
app:cardUseCompatPadding="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="70dp"
android:layout_height="70dp"
android:src="@android:drawable/ic_dialog_info"
android:translationZ="2dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
Output View :
You can use ConstraintLayout cool property for doing this easily
Xml snippet :
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
android:layout_width="match_parent"
android:layout_height="100dp"
android:layout_margin="10dp"
app:cardUseCompatPadding="true">
<android.support.v7.widget.CardView
android:layout_width="0dp"
android:layout_height="0dp"
android:layout_marginStart="35dp"
app:cardBackgroundColor="@color/cardview_dark_background"
app:cardUseCompatPadding="true"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<de.hdodenhof.circleimageview.CircleImageView
android:layout_width="70dp"
android:layout_height="70dp"
android:src="@android:drawable/ic_dialog_info"
android:translationZ="2dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
</android.support.constraint.ConstraintLayout>
Output View :
edited Nov 9 at 4:32
answered Nov 9 at 4:02
Aslam Hossin
679715
679715
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53219343%2fhow-to-add-an-imageview-outside-and-to-the-left-of-a-card%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wUQIXHGRl84T4K6OgtY5W5g2wsmWWDgS6P1y1LyiZs8Taupd4BhFCDALYO457zscwRPRGB a8h