Conflict between captcha and CSRF
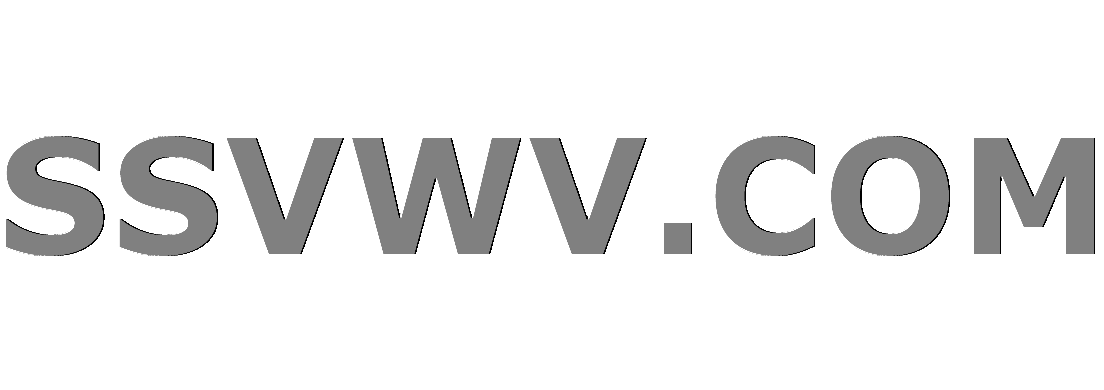
Multi tool use
up vote
0
down vote
favorite
For my login page, I have enable a captcha where user has to enter a 4 digits as displayed in the image. I'm using flask-session-captcha module for this. I followed exactly the same steps as in the instruction.
In the same login page, I also have a hidden CSRF token.
<input type="hidden" name="csrf_token" value="{{ csrf_token() }}"/>
It's working fine when I developed and tested it in my local environment (my laptop) but it doesn't work when I tested it in the test server. It will give errors such as "The CSRF tokens do not match." or "Invalid captcha" (even though I answered correctly) or sometimes "Missing CSRF token".
I have tried so many things like using decorator @csrf_exempt for that particular login page. I also added this:
@app.after_request
def apply_caching(response):
response.headers["X-Frame-Options"] = "SAMEORIGIN"
response.headers["Cache-Control"] = "public, max-age=0, no-cache, no-store, must-revalidate"
response.headers["Pragma"] = "no-cache"
response.headers["Expires"] = "0"
return response
But nothing seems to work. It really puzzles me why it works on local but not on test server. I tried not to use csrf_token() in the login page but it gave me error of missing csrf token. Using Google ReCaptcha is not an option because the user doesn't want to use that.
I wonder if it's because the captcha token is conflicted with the csrf token?
python flask csrf captcha
add a comment |
up vote
0
down vote
favorite
For my login page, I have enable a captcha where user has to enter a 4 digits as displayed in the image. I'm using flask-session-captcha module for this. I followed exactly the same steps as in the instruction.
In the same login page, I also have a hidden CSRF token.
<input type="hidden" name="csrf_token" value="{{ csrf_token() }}"/>
It's working fine when I developed and tested it in my local environment (my laptop) but it doesn't work when I tested it in the test server. It will give errors such as "The CSRF tokens do not match." or "Invalid captcha" (even though I answered correctly) or sometimes "Missing CSRF token".
I have tried so many things like using decorator @csrf_exempt for that particular login page. I also added this:
@app.after_request
def apply_caching(response):
response.headers["X-Frame-Options"] = "SAMEORIGIN"
response.headers["Cache-Control"] = "public, max-age=0, no-cache, no-store, must-revalidate"
response.headers["Pragma"] = "no-cache"
response.headers["Expires"] = "0"
return response
But nothing seems to work. It really puzzles me why it works on local but not on test server. I tried not to use csrf_token() in the login page but it gave me error of missing csrf token. Using Google ReCaptcha is not an option because the user doesn't want to use that.
I wonder if it's because the captcha token is conflicted with the csrf token?
python flask csrf captcha
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
For my login page, I have enable a captcha where user has to enter a 4 digits as displayed in the image. I'm using flask-session-captcha module for this. I followed exactly the same steps as in the instruction.
In the same login page, I also have a hidden CSRF token.
<input type="hidden" name="csrf_token" value="{{ csrf_token() }}"/>
It's working fine when I developed and tested it in my local environment (my laptop) but it doesn't work when I tested it in the test server. It will give errors such as "The CSRF tokens do not match." or "Invalid captcha" (even though I answered correctly) or sometimes "Missing CSRF token".
I have tried so many things like using decorator @csrf_exempt for that particular login page. I also added this:
@app.after_request
def apply_caching(response):
response.headers["X-Frame-Options"] = "SAMEORIGIN"
response.headers["Cache-Control"] = "public, max-age=0, no-cache, no-store, must-revalidate"
response.headers["Pragma"] = "no-cache"
response.headers["Expires"] = "0"
return response
But nothing seems to work. It really puzzles me why it works on local but not on test server. I tried not to use csrf_token() in the login page but it gave me error of missing csrf token. Using Google ReCaptcha is not an option because the user doesn't want to use that.
I wonder if it's because the captcha token is conflicted with the csrf token?
python flask csrf captcha
For my login page, I have enable a captcha where user has to enter a 4 digits as displayed in the image. I'm using flask-session-captcha module for this. I followed exactly the same steps as in the instruction.
In the same login page, I also have a hidden CSRF token.
<input type="hidden" name="csrf_token" value="{{ csrf_token() }}"/>
It's working fine when I developed and tested it in my local environment (my laptop) but it doesn't work when I tested it in the test server. It will give errors such as "The CSRF tokens do not match." or "Invalid captcha" (even though I answered correctly) or sometimes "Missing CSRF token".
I have tried so many things like using decorator @csrf_exempt for that particular login page. I also added this:
@app.after_request
def apply_caching(response):
response.headers["X-Frame-Options"] = "SAMEORIGIN"
response.headers["Cache-Control"] = "public, max-age=0, no-cache, no-store, must-revalidate"
response.headers["Pragma"] = "no-cache"
response.headers["Expires"] = "0"
return response
But nothing seems to work. It really puzzles me why it works on local but not on test server. I tried not to use csrf_token() in the login page but it gave me error of missing csrf token. Using Google ReCaptcha is not an option because the user doesn't want to use that.
I wonder if it's because the captcha token is conflicted with the csrf token?
python flask csrf captcha
python flask csrf captcha
asked Nov 7 at 10:14
sw2
107112
107112
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
Can you try flask_recaptcha?
Below are minimized example.
#app/__init__.py
from flask_recaptcha import ReCaptcha
recaptcha = ReCaptcha(app=app)
#app/views/your_view.py
@app.route('/a_view_for_demo/', methods=['GET', 'POST'])
def a_view_for_demo():
a_form = AForm()
if a_form.validate_on_submit() and recaptcha.verify():
# do your thing after validation.
return render_template('a_template.html', a_form=a_form)
#app_config
RECAPTCHA_ENABLED=True
RECAPTCHA_SITE_KEY="get this key from Google"
RECAPTCHA_SECRET_KEY="get this key from Google"
# a_template.html
<form .....>
{{ a_form.csrf_token }}
{{ recaptcha }}
</form>
I've tried this before but like I mentioned in my post, the user does not want me to use google recaptcha.
– sw2
Nov 8 at 1:03
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
Can you try flask_recaptcha?
Below are minimized example.
#app/__init__.py
from flask_recaptcha import ReCaptcha
recaptcha = ReCaptcha(app=app)
#app/views/your_view.py
@app.route('/a_view_for_demo/', methods=['GET', 'POST'])
def a_view_for_demo():
a_form = AForm()
if a_form.validate_on_submit() and recaptcha.verify():
# do your thing after validation.
return render_template('a_template.html', a_form=a_form)
#app_config
RECAPTCHA_ENABLED=True
RECAPTCHA_SITE_KEY="get this key from Google"
RECAPTCHA_SECRET_KEY="get this key from Google"
# a_template.html
<form .....>
{{ a_form.csrf_token }}
{{ recaptcha }}
</form>
I've tried this before but like I mentioned in my post, the user does not want me to use google recaptcha.
– sw2
Nov 8 at 1:03
add a comment |
up vote
0
down vote
Can you try flask_recaptcha?
Below are minimized example.
#app/__init__.py
from flask_recaptcha import ReCaptcha
recaptcha = ReCaptcha(app=app)
#app/views/your_view.py
@app.route('/a_view_for_demo/', methods=['GET', 'POST'])
def a_view_for_demo():
a_form = AForm()
if a_form.validate_on_submit() and recaptcha.verify():
# do your thing after validation.
return render_template('a_template.html', a_form=a_form)
#app_config
RECAPTCHA_ENABLED=True
RECAPTCHA_SITE_KEY="get this key from Google"
RECAPTCHA_SECRET_KEY="get this key from Google"
# a_template.html
<form .....>
{{ a_form.csrf_token }}
{{ recaptcha }}
</form>
I've tried this before but like I mentioned in my post, the user does not want me to use google recaptcha.
– sw2
Nov 8 at 1:03
add a comment |
up vote
0
down vote
up vote
0
down vote
Can you try flask_recaptcha?
Below are minimized example.
#app/__init__.py
from flask_recaptcha import ReCaptcha
recaptcha = ReCaptcha(app=app)
#app/views/your_view.py
@app.route('/a_view_for_demo/', methods=['GET', 'POST'])
def a_view_for_demo():
a_form = AForm()
if a_form.validate_on_submit() and recaptcha.verify():
# do your thing after validation.
return render_template('a_template.html', a_form=a_form)
#app_config
RECAPTCHA_ENABLED=True
RECAPTCHA_SITE_KEY="get this key from Google"
RECAPTCHA_SECRET_KEY="get this key from Google"
# a_template.html
<form .....>
{{ a_form.csrf_token }}
{{ recaptcha }}
</form>
Can you try flask_recaptcha?
Below are minimized example.
#app/__init__.py
from flask_recaptcha import ReCaptcha
recaptcha = ReCaptcha(app=app)
#app/views/your_view.py
@app.route('/a_view_for_demo/', methods=['GET', 'POST'])
def a_view_for_demo():
a_form = AForm()
if a_form.validate_on_submit() and recaptcha.verify():
# do your thing after validation.
return render_template('a_template.html', a_form=a_form)
#app_config
RECAPTCHA_ENABLED=True
RECAPTCHA_SITE_KEY="get this key from Google"
RECAPTCHA_SECRET_KEY="get this key from Google"
# a_template.html
<form .....>
{{ a_form.csrf_token }}
{{ recaptcha }}
</form>
answered Nov 7 at 22:56
Jessi
288619
288619
I've tried this before but like I mentioned in my post, the user does not want me to use google recaptcha.
– sw2
Nov 8 at 1:03
add a comment |
I've tried this before but like I mentioned in my post, the user does not want me to use google recaptcha.
– sw2
Nov 8 at 1:03
I've tried this before but like I mentioned in my post, the user does not want me to use google recaptcha.
– sw2
Nov 8 at 1:03
I've tried this before but like I mentioned in my post, the user does not want me to use google recaptcha.
– sw2
Nov 8 at 1:03
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53187383%2fconflict-between-captcha-and-csrf%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
N6vhJ Y9GU9pofwWtUML4lVoyMD QwDT qz6p5 cmv