Fetch request in React: How do I Map through JSON array of objects, setState() & append?
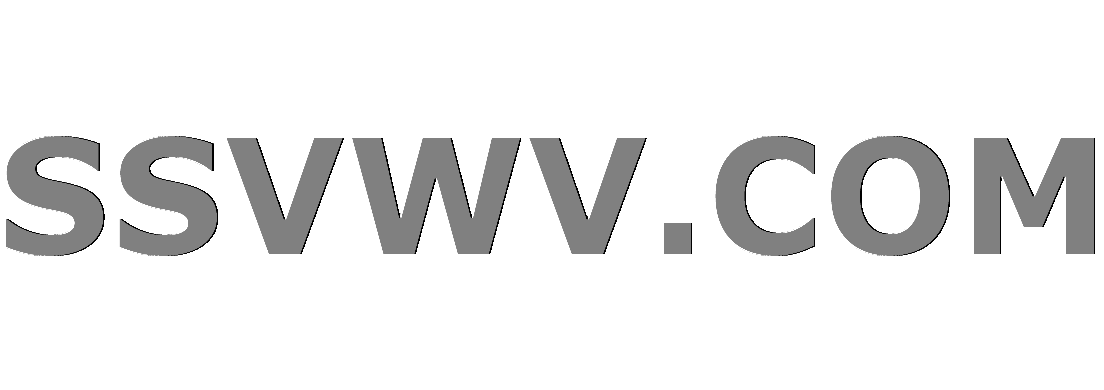
Multi tool use
This API returns a JSON array of objects, but I'm having trouble with setState and appending. Most documentation covers JSON objects with keys. The error I get from my renderItems() func is:
ItemsList.js:76 Uncaught TypeError: Cannot read property 'map' of undefined
in ItemsList.js
import React, { Component } from "react";
import NewSingleItem from './NewSingleItem';
import { findDOMNode } from 'react-dom';
const title_URL = "https://www.healthcare.gov/api/index.json";
class ItemsList extends Component {
constructor(props) {
super(props);
this.state = {
// error: null,
// isLoaded: false,
title: ,
url: ,
descrip:
};
}
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
for (let i = 0; i < data.length; i++) {
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
console.log(data[i])
}
})
.catch(error => console.log(error));
}
renderItems() {
return this.state.title.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
render() {
return <ul>{this.renderItems()}</ul>;
}
}
export default ItemsList;
Above, I'm trying to map through the items, but I'm not quite sure why I cant map through the objects I set in setState(). Note: even if in my setState() I use title: data.title
, it doesnt work. Can someone explain where I'm erroring out? Thanks.
in App.js
import React, { Component } from "react";
import { hot } from "react-hot-loader";
import "./App.css";
import ItemsList from './ItemsList';
class App extends Component {
render() {
return (
<div className="App">
<h1> Hello Healthcare </h1>
<ItemsList />
<article className="main"></article>
</div>
);
}
}
export default App;
in NewSingleItem.js
import React, { Component } from "react";
const NewSingleItem = ({item}) => {
<li>
<p>{item.title}</p>
</li>
};
export default NewSingleItem;
ajax reactjs fetch
add a comment |
This API returns a JSON array of objects, but I'm having trouble with setState and appending. Most documentation covers JSON objects with keys. The error I get from my renderItems() func is:
ItemsList.js:76 Uncaught TypeError: Cannot read property 'map' of undefined
in ItemsList.js
import React, { Component } from "react";
import NewSingleItem from './NewSingleItem';
import { findDOMNode } from 'react-dom';
const title_URL = "https://www.healthcare.gov/api/index.json";
class ItemsList extends Component {
constructor(props) {
super(props);
this.state = {
// error: null,
// isLoaded: false,
title: ,
url: ,
descrip:
};
}
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
for (let i = 0; i < data.length; i++) {
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
console.log(data[i])
}
})
.catch(error => console.log(error));
}
renderItems() {
return this.state.title.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
render() {
return <ul>{this.renderItems()}</ul>;
}
}
export default ItemsList;
Above, I'm trying to map through the items, but I'm not quite sure why I cant map through the objects I set in setState(). Note: even if in my setState() I use title: data.title
, it doesnt work. Can someone explain where I'm erroring out? Thanks.
in App.js
import React, { Component } from "react";
import { hot } from "react-hot-loader";
import "./App.css";
import ItemsList from './ItemsList';
class App extends Component {
render() {
return (
<div className="App">
<h1> Hello Healthcare </h1>
<ItemsList />
<article className="main"></article>
</div>
);
}
}
export default App;
in NewSingleItem.js
import React, { Component } from "react";
const NewSingleItem = ({item}) => {
<li>
<p>{item.title}</p>
</li>
};
export default NewSingleItem;
ajax reactjs fetch
add a comment |
This API returns a JSON array of objects, but I'm having trouble with setState and appending. Most documentation covers JSON objects with keys. The error I get from my renderItems() func is:
ItemsList.js:76 Uncaught TypeError: Cannot read property 'map' of undefined
in ItemsList.js
import React, { Component } from "react";
import NewSingleItem from './NewSingleItem';
import { findDOMNode } from 'react-dom';
const title_URL = "https://www.healthcare.gov/api/index.json";
class ItemsList extends Component {
constructor(props) {
super(props);
this.state = {
// error: null,
// isLoaded: false,
title: ,
url: ,
descrip:
};
}
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
for (let i = 0; i < data.length; i++) {
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
console.log(data[i])
}
})
.catch(error => console.log(error));
}
renderItems() {
return this.state.title.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
render() {
return <ul>{this.renderItems()}</ul>;
}
}
export default ItemsList;
Above, I'm trying to map through the items, but I'm not quite sure why I cant map through the objects I set in setState(). Note: even if in my setState() I use title: data.title
, it doesnt work. Can someone explain where I'm erroring out? Thanks.
in App.js
import React, { Component } from "react";
import { hot } from "react-hot-loader";
import "./App.css";
import ItemsList from './ItemsList';
class App extends Component {
render() {
return (
<div className="App">
<h1> Hello Healthcare </h1>
<ItemsList />
<article className="main"></article>
</div>
);
}
}
export default App;
in NewSingleItem.js
import React, { Component } from "react";
const NewSingleItem = ({item}) => {
<li>
<p>{item.title}</p>
</li>
};
export default NewSingleItem;
ajax reactjs fetch
This API returns a JSON array of objects, but I'm having trouble with setState and appending. Most documentation covers JSON objects with keys. The error I get from my renderItems() func is:
ItemsList.js:76 Uncaught TypeError: Cannot read property 'map' of undefined
in ItemsList.js
import React, { Component } from "react";
import NewSingleItem from './NewSingleItem';
import { findDOMNode } from 'react-dom';
const title_URL = "https://www.healthcare.gov/api/index.json";
class ItemsList extends Component {
constructor(props) {
super(props);
this.state = {
// error: null,
// isLoaded: false,
title: ,
url: ,
descrip:
};
}
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
for (let i = 0; i < data.length; i++) {
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
console.log(data[i])
}
})
.catch(error => console.log(error));
}
renderItems() {
return this.state.title.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
render() {
return <ul>{this.renderItems()}</ul>;
}
}
export default ItemsList;
Above, I'm trying to map through the items, but I'm not quite sure why I cant map through the objects I set in setState(). Note: even if in my setState() I use title: data.title
, it doesnt work. Can someone explain where I'm erroring out? Thanks.
in App.js
import React, { Component } from "react";
import { hot } from "react-hot-loader";
import "./App.css";
import ItemsList from './ItemsList';
class App extends Component {
render() {
return (
<div className="App">
<h1> Hello Healthcare </h1>
<ItemsList />
<article className="main"></article>
</div>
);
}
}
export default App;
in NewSingleItem.js
import React, { Component } from "react";
const NewSingleItem = ({item}) => {
<li>
<p>{item.title}</p>
</li>
};
export default NewSingleItem;
ajax reactjs fetch
ajax reactjs fetch
asked Nov 10 at 23:34
Cat Perry
12
12
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The problem is this line:
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
When you state this.state.title
to data[i].title
, it's no longer an array. You need to ensure it stays an array. You probably don't want to split them up anyway, just keep them all in a self contained array:
this.state = {
// error: null,
// isLoaded: false,
items: ,
};
...
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
this.setState({
items: data.map(item => ({
title: item.title,
url: item.url,
descrip: item.bite,
})
});
console.log(data[i])
}
})
...
renderItems() {
return this.state.items.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
Awesome, thanks, noe the states preserved and I have one neat object to handle. The final issue is rendering still isnt working. I've tried passing {...this.state} and {...this.props} to App.js, but the darn list still wont show. Note: the <ul> from render() shows but not the list from renderItems(). any ideas? thanks!
– Cat Perry
Nov 11 at 18:06
@CatPerry Can you create a reproducible issue on codesandbox.io?
– FrankerZ
Nov 11 at 19:54
Sure thing: link What I have logging to the console is item object, eventually I'll want to display/manipulate item.title, etc, but for now I'm just trying to the get item.title to render in the NewSingleItem.
– Cat Perry
Nov 11 at 20:19
@CatPerry Check out my updated sandbox. Few things: 1. Be wary about curly brackets. When you use them in arrow functionsparam => { ... }
, you don't actually return anything. You need to make sure you either include a return statement, or explicitly start your function off with a(
to say "Return everything following this". 2. The data being returned was kinda messed up (It was returning false for the last item from healthcare.gov), so I added a filter to ensure it only showed elements with a title.
– FrankerZ
Nov 11 at 20:48
It works! Fab. I knew there were a few outstanding issues. I also added a return statement and parens to the NewSingleItem function and that made it work in addition to your fitler() method addition. Thanks for the info about { . . . }. Really appreciate your help!
– Cat Perry
Nov 12 at 0:15
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244476%2ffetch-request-in-react-how-do-i-map-through-json-array-of-objects-setstate%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The problem is this line:
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
When you state this.state.title
to data[i].title
, it's no longer an array. You need to ensure it stays an array. You probably don't want to split them up anyway, just keep them all in a self contained array:
this.state = {
// error: null,
// isLoaded: false,
items: ,
};
...
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
this.setState({
items: data.map(item => ({
title: item.title,
url: item.url,
descrip: item.bite,
})
});
console.log(data[i])
}
})
...
renderItems() {
return this.state.items.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
Awesome, thanks, noe the states preserved and I have one neat object to handle. The final issue is rendering still isnt working. I've tried passing {...this.state} and {...this.props} to App.js, but the darn list still wont show. Note: the <ul> from render() shows but not the list from renderItems(). any ideas? thanks!
– Cat Perry
Nov 11 at 18:06
@CatPerry Can you create a reproducible issue on codesandbox.io?
– FrankerZ
Nov 11 at 19:54
Sure thing: link What I have logging to the console is item object, eventually I'll want to display/manipulate item.title, etc, but for now I'm just trying to the get item.title to render in the NewSingleItem.
– Cat Perry
Nov 11 at 20:19
@CatPerry Check out my updated sandbox. Few things: 1. Be wary about curly brackets. When you use them in arrow functionsparam => { ... }
, you don't actually return anything. You need to make sure you either include a return statement, or explicitly start your function off with a(
to say "Return everything following this". 2. The data being returned was kinda messed up (It was returning false for the last item from healthcare.gov), so I added a filter to ensure it only showed elements with a title.
– FrankerZ
Nov 11 at 20:48
It works! Fab. I knew there were a few outstanding issues. I also added a return statement and parens to the NewSingleItem function and that made it work in addition to your fitler() method addition. Thanks for the info about { . . . }. Really appreciate your help!
– Cat Perry
Nov 12 at 0:15
add a comment |
The problem is this line:
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
When you state this.state.title
to data[i].title
, it's no longer an array. You need to ensure it stays an array. You probably don't want to split them up anyway, just keep them all in a self contained array:
this.state = {
// error: null,
// isLoaded: false,
items: ,
};
...
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
this.setState({
items: data.map(item => ({
title: item.title,
url: item.url,
descrip: item.bite,
})
});
console.log(data[i])
}
})
...
renderItems() {
return this.state.items.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
Awesome, thanks, noe the states preserved and I have one neat object to handle. The final issue is rendering still isnt working. I've tried passing {...this.state} and {...this.props} to App.js, but the darn list still wont show. Note: the <ul> from render() shows but not the list from renderItems(). any ideas? thanks!
– Cat Perry
Nov 11 at 18:06
@CatPerry Can you create a reproducible issue on codesandbox.io?
– FrankerZ
Nov 11 at 19:54
Sure thing: link What I have logging to the console is item object, eventually I'll want to display/manipulate item.title, etc, but for now I'm just trying to the get item.title to render in the NewSingleItem.
– Cat Perry
Nov 11 at 20:19
@CatPerry Check out my updated sandbox. Few things: 1. Be wary about curly brackets. When you use them in arrow functionsparam => { ... }
, you don't actually return anything. You need to make sure you either include a return statement, or explicitly start your function off with a(
to say "Return everything following this". 2. The data being returned was kinda messed up (It was returning false for the last item from healthcare.gov), so I added a filter to ensure it only showed elements with a title.
– FrankerZ
Nov 11 at 20:48
It works! Fab. I knew there were a few outstanding issues. I also added a return statement and parens to the NewSingleItem function and that made it work in addition to your fitler() method addition. Thanks for the info about { . . . }. Really appreciate your help!
– Cat Perry
Nov 12 at 0:15
add a comment |
The problem is this line:
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
When you state this.state.title
to data[i].title
, it's no longer an array. You need to ensure it stays an array. You probably don't want to split them up anyway, just keep them all in a self contained array:
this.state = {
// error: null,
// isLoaded: false,
items: ,
};
...
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
this.setState({
items: data.map(item => ({
title: item.title,
url: item.url,
descrip: item.bite,
})
});
console.log(data[i])
}
})
...
renderItems() {
return this.state.items.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
The problem is this line:
this.setState({
title: data[i].title,
url: data[i].url,
descrip: data[i].bite,
});
When you state this.state.title
to data[i].title
, it's no longer an array. You need to ensure it stays an array. You probably don't want to split them up anyway, just keep them all in a self contained array:
this.state = {
// error: null,
// isLoaded: false,
items: ,
};
...
componentDidMount() {
fetch(title_URL)
.then(response => {
return response.json();
})
.then((data) => {
this.setState({
items: data.map(item => ({
title: item.title,
url: item.url,
descrip: item.bite,
})
});
console.log(data[i])
}
})
...
renderItems() {
return this.state.items.map(item => {
<NewSingleItem key={item.title} item={item.title} />;
});
}
answered Nov 11 at 0:41


FrankerZ
16.1k72859
16.1k72859
Awesome, thanks, noe the states preserved and I have one neat object to handle. The final issue is rendering still isnt working. I've tried passing {...this.state} and {...this.props} to App.js, but the darn list still wont show. Note: the <ul> from render() shows but not the list from renderItems(). any ideas? thanks!
– Cat Perry
Nov 11 at 18:06
@CatPerry Can you create a reproducible issue on codesandbox.io?
– FrankerZ
Nov 11 at 19:54
Sure thing: link What I have logging to the console is item object, eventually I'll want to display/manipulate item.title, etc, but for now I'm just trying to the get item.title to render in the NewSingleItem.
– Cat Perry
Nov 11 at 20:19
@CatPerry Check out my updated sandbox. Few things: 1. Be wary about curly brackets. When you use them in arrow functionsparam => { ... }
, you don't actually return anything. You need to make sure you either include a return statement, or explicitly start your function off with a(
to say "Return everything following this". 2. The data being returned was kinda messed up (It was returning false for the last item from healthcare.gov), so I added a filter to ensure it only showed elements with a title.
– FrankerZ
Nov 11 at 20:48
It works! Fab. I knew there were a few outstanding issues. I also added a return statement and parens to the NewSingleItem function and that made it work in addition to your fitler() method addition. Thanks for the info about { . . . }. Really appreciate your help!
– Cat Perry
Nov 12 at 0:15
add a comment |
Awesome, thanks, noe the states preserved and I have one neat object to handle. The final issue is rendering still isnt working. I've tried passing {...this.state} and {...this.props} to App.js, but the darn list still wont show. Note: the <ul> from render() shows but not the list from renderItems(). any ideas? thanks!
– Cat Perry
Nov 11 at 18:06
@CatPerry Can you create a reproducible issue on codesandbox.io?
– FrankerZ
Nov 11 at 19:54
Sure thing: link What I have logging to the console is item object, eventually I'll want to display/manipulate item.title, etc, but for now I'm just trying to the get item.title to render in the NewSingleItem.
– Cat Perry
Nov 11 at 20:19
@CatPerry Check out my updated sandbox. Few things: 1. Be wary about curly brackets. When you use them in arrow functionsparam => { ... }
, you don't actually return anything. You need to make sure you either include a return statement, or explicitly start your function off with a(
to say "Return everything following this". 2. The data being returned was kinda messed up (It was returning false for the last item from healthcare.gov), so I added a filter to ensure it only showed elements with a title.
– FrankerZ
Nov 11 at 20:48
It works! Fab. I knew there were a few outstanding issues. I also added a return statement and parens to the NewSingleItem function and that made it work in addition to your fitler() method addition. Thanks for the info about { . . . }. Really appreciate your help!
– Cat Perry
Nov 12 at 0:15
Awesome, thanks, noe the states preserved and I have one neat object to handle. The final issue is rendering still isnt working. I've tried passing {...this.state} and {...this.props} to App.js, but the darn list still wont show. Note: the <ul> from render() shows but not the list from renderItems(). any ideas? thanks!
– Cat Perry
Nov 11 at 18:06
Awesome, thanks, noe the states preserved and I have one neat object to handle. The final issue is rendering still isnt working. I've tried passing {...this.state} and {...this.props} to App.js, but the darn list still wont show. Note: the <ul> from render() shows but not the list from renderItems(). any ideas? thanks!
– Cat Perry
Nov 11 at 18:06
@CatPerry Can you create a reproducible issue on codesandbox.io?
– FrankerZ
Nov 11 at 19:54
@CatPerry Can you create a reproducible issue on codesandbox.io?
– FrankerZ
Nov 11 at 19:54
Sure thing: link What I have logging to the console is item object, eventually I'll want to display/manipulate item.title, etc, but for now I'm just trying to the get item.title to render in the NewSingleItem.
– Cat Perry
Nov 11 at 20:19
Sure thing: link What I have logging to the console is item object, eventually I'll want to display/manipulate item.title, etc, but for now I'm just trying to the get item.title to render in the NewSingleItem.
– Cat Perry
Nov 11 at 20:19
@CatPerry Check out my updated sandbox. Few things: 1. Be wary about curly brackets. When you use them in arrow functions
param => { ... }
, you don't actually return anything. You need to make sure you either include a return statement, or explicitly start your function off with a (
to say "Return everything following this". 2. The data being returned was kinda messed up (It was returning false for the last item from healthcare.gov), so I added a filter to ensure it only showed elements with a title.– FrankerZ
Nov 11 at 20:48
@CatPerry Check out my updated sandbox. Few things: 1. Be wary about curly brackets. When you use them in arrow functions
param => { ... }
, you don't actually return anything. You need to make sure you either include a return statement, or explicitly start your function off with a (
to say "Return everything following this". 2. The data being returned was kinda messed up (It was returning false for the last item from healthcare.gov), so I added a filter to ensure it only showed elements with a title.– FrankerZ
Nov 11 at 20:48
It works! Fab. I knew there were a few outstanding issues. I also added a return statement and parens to the NewSingleItem function and that made it work in addition to your fitler() method addition. Thanks for the info about { . . . }. Really appreciate your help!
– Cat Perry
Nov 12 at 0:15
It works! Fab. I knew there were a few outstanding issues. I also added a return statement and parens to the NewSingleItem function and that made it work in addition to your fitler() method addition. Thanks for the info about { . . . }. Really appreciate your help!
– Cat Perry
Nov 12 at 0:15
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244476%2ffetch-request-in-react-how-do-i-map-through-json-array-of-objects-setstate%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Jcd kX6oAOzs,WFlDr bR4DQ3hbr e2J nbVJ7e99pFtxJ8bmx,i5S9oCtJkHWyNV 1DR,gFA8ZNyOGGDp Mj2ok