Meteor Apollo GraphQL Interfaces: return an array of object and invoking resolver in another resolver
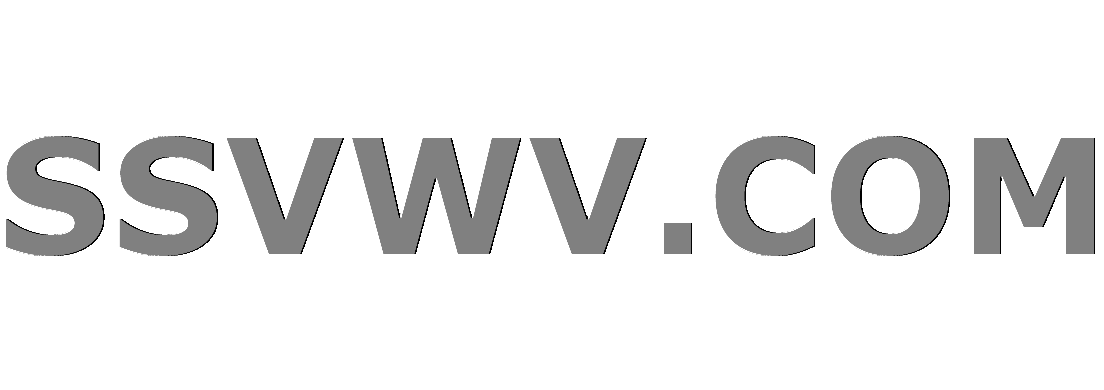
Multi tool use
I'm having some issues with GraphQl interfaces. Let take the example in the doc:
interface Vehicle {
maxSpeed: Int
}
type Airplane implements Vehicle {
maxSpeed: Int
wingspan: Int
}
type Car implements Vehicle {
maxSpeed: Int
licensePlate: String
}
type Query {
vehicle: Vehicle
}
const resolvers = {
Vehicle: {
__resolveType(obj, context, info){
if(obj.wingspan){
return 'Airplane';
}
if(obj.licensePlate){
return 'Car';
}
return null;
},
},
Query: {
vehicle: () => {
// Query Airplane and Car an merge in one single array.
}
},
};
Background: I create 2 different DBs
for each interfaces implementation: one for Car
and one for Airplane
. But I did not create a DB
for Vehicle
since it just an interface
that does not keep data in itself. (I hope this is fine)
The first problem is how to access Car
and Airplane
resolvers
to query their respective DBs
in the Vehicle resolver
? So in Query.vehicle()
, I would like to just call the resolver defined for fetching all cars to get all cars and call the one for fetching airplanes to get all airplanes in the DB. (note using Car.find().fetch()
is not always effective because before doing the query, lots of other query needs to be done also avoid code duplication).
The Second problem is that in the example, obj.wingspan
assume that obj
should be an object. So returning an array from Query.vehicle()
, won't help because this query is used in Vehicle.__resolveType
. So from what I've experienced with GraphQl interfaces, it only returns a single object from the query.
My aim is to obtain with the with the following query:
{
vehicle {
maxSpeed
... on Car {
licensePlate
}
... on Airplane {
wingspan
}
}
}
the following result:
{
"data": {
"car": [
{
"maxSpeed": 200,
"licensePlate": 1.8
},
{
"maxSpeed": 120,
"licensePlate": "String"
},
...
],
"Airplane": [
{
"maxSpeed": 500,
"wingspan": "String"
},
{
"maxSpeed": 700,
"wingspan": 1.5
},
...
]
}
}
meteor graphql apollo apollo-client apollo-server
add a comment |
I'm having some issues with GraphQl interfaces. Let take the example in the doc:
interface Vehicle {
maxSpeed: Int
}
type Airplane implements Vehicle {
maxSpeed: Int
wingspan: Int
}
type Car implements Vehicle {
maxSpeed: Int
licensePlate: String
}
type Query {
vehicle: Vehicle
}
const resolvers = {
Vehicle: {
__resolveType(obj, context, info){
if(obj.wingspan){
return 'Airplane';
}
if(obj.licensePlate){
return 'Car';
}
return null;
},
},
Query: {
vehicle: () => {
// Query Airplane and Car an merge in one single array.
}
},
};
Background: I create 2 different DBs
for each interfaces implementation: one for Car
and one for Airplane
. But I did not create a DB
for Vehicle
since it just an interface
that does not keep data in itself. (I hope this is fine)
The first problem is how to access Car
and Airplane
resolvers
to query their respective DBs
in the Vehicle resolver
? So in Query.vehicle()
, I would like to just call the resolver defined for fetching all cars to get all cars and call the one for fetching airplanes to get all airplanes in the DB. (note using Car.find().fetch()
is not always effective because before doing the query, lots of other query needs to be done also avoid code duplication).
The Second problem is that in the example, obj.wingspan
assume that obj
should be an object. So returning an array from Query.vehicle()
, won't help because this query is used in Vehicle.__resolveType
. So from what I've experienced with GraphQl interfaces, it only returns a single object from the query.
My aim is to obtain with the with the following query:
{
vehicle {
maxSpeed
... on Car {
licensePlate
}
... on Airplane {
wingspan
}
}
}
the following result:
{
"data": {
"car": [
{
"maxSpeed": 200,
"licensePlate": 1.8
},
{
"maxSpeed": 120,
"licensePlate": "String"
},
...
],
"Airplane": [
{
"maxSpeed": 500,
"wingspan": "String"
},
{
"maxSpeed": 700,
"wingspan": 1.5
},
...
]
}
}
meteor graphql apollo apollo-client apollo-server
add a comment |
I'm having some issues with GraphQl interfaces. Let take the example in the doc:
interface Vehicle {
maxSpeed: Int
}
type Airplane implements Vehicle {
maxSpeed: Int
wingspan: Int
}
type Car implements Vehicle {
maxSpeed: Int
licensePlate: String
}
type Query {
vehicle: Vehicle
}
const resolvers = {
Vehicle: {
__resolveType(obj, context, info){
if(obj.wingspan){
return 'Airplane';
}
if(obj.licensePlate){
return 'Car';
}
return null;
},
},
Query: {
vehicle: () => {
// Query Airplane and Car an merge in one single array.
}
},
};
Background: I create 2 different DBs
for each interfaces implementation: one for Car
and one for Airplane
. But I did not create a DB
for Vehicle
since it just an interface
that does not keep data in itself. (I hope this is fine)
The first problem is how to access Car
and Airplane
resolvers
to query their respective DBs
in the Vehicle resolver
? So in Query.vehicle()
, I would like to just call the resolver defined for fetching all cars to get all cars and call the one for fetching airplanes to get all airplanes in the DB. (note using Car.find().fetch()
is not always effective because before doing the query, lots of other query needs to be done also avoid code duplication).
The Second problem is that in the example, obj.wingspan
assume that obj
should be an object. So returning an array from Query.vehicle()
, won't help because this query is used in Vehicle.__resolveType
. So from what I've experienced with GraphQl interfaces, it only returns a single object from the query.
My aim is to obtain with the with the following query:
{
vehicle {
maxSpeed
... on Car {
licensePlate
}
... on Airplane {
wingspan
}
}
}
the following result:
{
"data": {
"car": [
{
"maxSpeed": 200,
"licensePlate": 1.8
},
{
"maxSpeed": 120,
"licensePlate": "String"
},
...
],
"Airplane": [
{
"maxSpeed": 500,
"wingspan": "String"
},
{
"maxSpeed": 700,
"wingspan": 1.5
},
...
]
}
}
meteor graphql apollo apollo-client apollo-server
I'm having some issues with GraphQl interfaces. Let take the example in the doc:
interface Vehicle {
maxSpeed: Int
}
type Airplane implements Vehicle {
maxSpeed: Int
wingspan: Int
}
type Car implements Vehicle {
maxSpeed: Int
licensePlate: String
}
type Query {
vehicle: Vehicle
}
const resolvers = {
Vehicle: {
__resolveType(obj, context, info){
if(obj.wingspan){
return 'Airplane';
}
if(obj.licensePlate){
return 'Car';
}
return null;
},
},
Query: {
vehicle: () => {
// Query Airplane and Car an merge in one single array.
}
},
};
Background: I create 2 different DBs
for each interfaces implementation: one for Car
and one for Airplane
. But I did not create a DB
for Vehicle
since it just an interface
that does not keep data in itself. (I hope this is fine)
The first problem is how to access Car
and Airplane
resolvers
to query their respective DBs
in the Vehicle resolver
? So in Query.vehicle()
, I would like to just call the resolver defined for fetching all cars to get all cars and call the one for fetching airplanes to get all airplanes in the DB. (note using Car.find().fetch()
is not always effective because before doing the query, lots of other query needs to be done also avoid code duplication).
The Second problem is that in the example, obj.wingspan
assume that obj
should be an object. So returning an array from Query.vehicle()
, won't help because this query is used in Vehicle.__resolveType
. So from what I've experienced with GraphQl interfaces, it only returns a single object from the query.
My aim is to obtain with the with the following query:
{
vehicle {
maxSpeed
... on Car {
licensePlate
}
... on Airplane {
wingspan
}
}
}
the following result:
{
"data": {
"car": [
{
"maxSpeed": 200,
"licensePlate": 1.8
},
{
"maxSpeed": 120,
"licensePlate": "String"
},
...
],
"Airplane": [
{
"maxSpeed": 500,
"wingspan": "String"
},
{
"maxSpeed": 700,
"wingspan": 1.5
},
...
]
}
}
meteor graphql apollo apollo-client apollo-server
meteor graphql apollo apollo-client apollo-server
asked Nov 10 at 23:33
danyhiol
100113
100113
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
It sounds like you may be trying to return multiple objects. If so, you'll need to return an array of objects:
type Query {
vehicles: [ Vehicle ]
}
If you're just trying to return a single object by ID, in your vehicle resolver, just try both databases and return the one with the result:
const resolvers = {
Query: {
vehicle: (parent, args) => {
const { id } = args;
const [ car, plane ] = await Promise.all(
await carDB.get(id),
await planeDB.get(id)
)
return car || plane
}
}
}
Thanks for your answer. I already figured that out. What I'm trying to achieve is the result showed above with each DB (Car, Airplane,...) grouped.
– danyhiol
Nov 25 at 19:23
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244470%2fmeteor-apollo-graphql-interfaces-return-an-array-of-object-and-invoking-resolve%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
It sounds like you may be trying to return multiple objects. If so, you'll need to return an array of objects:
type Query {
vehicles: [ Vehicle ]
}
If you're just trying to return a single object by ID, in your vehicle resolver, just try both databases and return the one with the result:
const resolvers = {
Query: {
vehicle: (parent, args) => {
const { id } = args;
const [ car, plane ] = await Promise.all(
await carDB.get(id),
await planeDB.get(id)
)
return car || plane
}
}
}
Thanks for your answer. I already figured that out. What I'm trying to achieve is the result showed above with each DB (Car, Airplane,...) grouped.
– danyhiol
Nov 25 at 19:23
add a comment |
It sounds like you may be trying to return multiple objects. If so, you'll need to return an array of objects:
type Query {
vehicles: [ Vehicle ]
}
If you're just trying to return a single object by ID, in your vehicle resolver, just try both databases and return the one with the result:
const resolvers = {
Query: {
vehicle: (parent, args) => {
const { id } = args;
const [ car, plane ] = await Promise.all(
await carDB.get(id),
await planeDB.get(id)
)
return car || plane
}
}
}
Thanks for your answer. I already figured that out. What I'm trying to achieve is the result showed above with each DB (Car, Airplane,...) grouped.
– danyhiol
Nov 25 at 19:23
add a comment |
It sounds like you may be trying to return multiple objects. If so, you'll need to return an array of objects:
type Query {
vehicles: [ Vehicle ]
}
If you're just trying to return a single object by ID, in your vehicle resolver, just try both databases and return the one with the result:
const resolvers = {
Query: {
vehicle: (parent, args) => {
const { id } = args;
const [ car, plane ] = await Promise.all(
await carDB.get(id),
await planeDB.get(id)
)
return car || plane
}
}
}
It sounds like you may be trying to return multiple objects. If so, you'll need to return an array of objects:
type Query {
vehicles: [ Vehicle ]
}
If you're just trying to return a single object by ID, in your vehicle resolver, just try both databases and return the one with the result:
const resolvers = {
Query: {
vehicle: (parent, args) => {
const { id } = args;
const [ car, plane ] = await Promise.all(
await carDB.get(id),
await planeDB.get(id)
)
return car || plane
}
}
}
answered Nov 19 at 18:48
Dan Crews
1,5091019
1,5091019
Thanks for your answer. I already figured that out. What I'm trying to achieve is the result showed above with each DB (Car, Airplane,...) grouped.
– danyhiol
Nov 25 at 19:23
add a comment |
Thanks for your answer. I already figured that out. What I'm trying to achieve is the result showed above with each DB (Car, Airplane,...) grouped.
– danyhiol
Nov 25 at 19:23
Thanks for your answer. I already figured that out. What I'm trying to achieve is the result showed above with each DB (Car, Airplane,...) grouped.
– danyhiol
Nov 25 at 19:23
Thanks for your answer. I already figured that out. What I'm trying to achieve is the result showed above with each DB (Car, Airplane,...) grouped.
– danyhiol
Nov 25 at 19:23
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53244470%2fmeteor-apollo-graphql-interfaces-return-an-array-of-object-and-invoking-resolve%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
87BCz Z8YPiMIbSXu8,eAu3dy8HHJWz9m0m 3MCL1OLzGwn okU t5,b