Service Layer with multiple validation and other functions
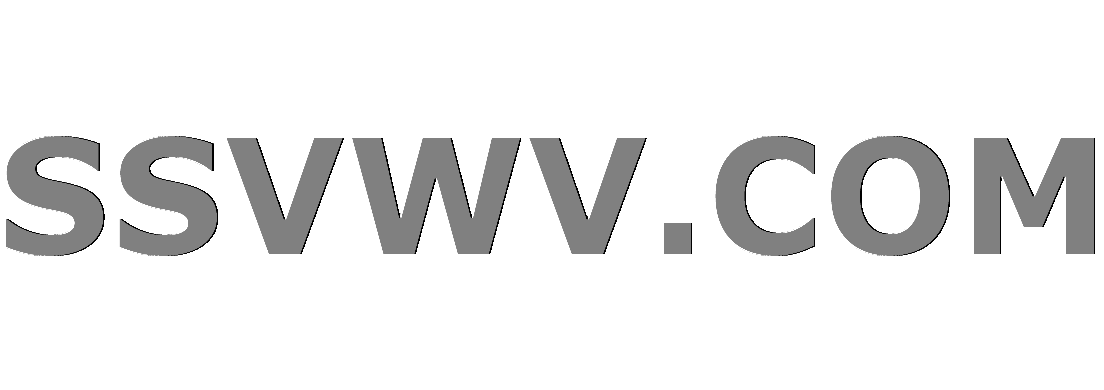
Multi tool use
up vote
0
down vote
favorite
I am currently developing a REST Api using Slim Framework with a Layered Architecture:
Controller->Service->Repository->DB
My problem is the save() function on one of my services contains a collection of complex functions which clearly violates the Separation of Responsibility Principle so I grouped the functions based on their "responsibilities". Here are some of it:
- Data Validation
- Record Locking
- Edit History Logging
- Destination Delegation
These functions are not always executed. It executes depending on the data passed onto the service and some of the functions blocks the saving if a business rule is violated. Currently,I am planning to solve it using the Chain-of-Responsibility Pattern
class PropertyController
{
public function saveAction($request, $response, $args)
{
$data = $req->getParsedBody();
$dataValidation = new DataValidation();
$recordLocking = new RecordLocking();
$editHistoryLogging = new EditHistoryLogging();
$destinationDelegation = new DestinationDelegation();
$dataValidation->next($recordLocking);
$recordLocking->next($editHistoryLogging);
$editHistoryLogging->next($destinationDelegation);
$propertyService = new PropertyService(new PropertyEloquentRepository());
$result = $propertyService->save($data, $dataValidation);
return $response->withStatus(200)
->withHeader('Content-Type','application/json')
->write(json_encode($result));
}
}
Is this implementation fine and is considered a good practice?
php architecture architectural-patterns
add a comment |
up vote
0
down vote
favorite
I am currently developing a REST Api using Slim Framework with a Layered Architecture:
Controller->Service->Repository->DB
My problem is the save() function on one of my services contains a collection of complex functions which clearly violates the Separation of Responsibility Principle so I grouped the functions based on their "responsibilities". Here are some of it:
- Data Validation
- Record Locking
- Edit History Logging
- Destination Delegation
These functions are not always executed. It executes depending on the data passed onto the service and some of the functions blocks the saving if a business rule is violated. Currently,I am planning to solve it using the Chain-of-Responsibility Pattern
class PropertyController
{
public function saveAction($request, $response, $args)
{
$data = $req->getParsedBody();
$dataValidation = new DataValidation();
$recordLocking = new RecordLocking();
$editHistoryLogging = new EditHistoryLogging();
$destinationDelegation = new DestinationDelegation();
$dataValidation->next($recordLocking);
$recordLocking->next($editHistoryLogging);
$editHistoryLogging->next($destinationDelegation);
$propertyService = new PropertyService(new PropertyEloquentRepository());
$result = $propertyService->save($data, $dataValidation);
return $response->withStatus(200)
->withHeader('Content-Type','application/json')
->write(json_encode($result));
}
}
Is this implementation fine and is considered a good practice?
php architecture architectural-patterns
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am currently developing a REST Api using Slim Framework with a Layered Architecture:
Controller->Service->Repository->DB
My problem is the save() function on one of my services contains a collection of complex functions which clearly violates the Separation of Responsibility Principle so I grouped the functions based on their "responsibilities". Here are some of it:
- Data Validation
- Record Locking
- Edit History Logging
- Destination Delegation
These functions are not always executed. It executes depending on the data passed onto the service and some of the functions blocks the saving if a business rule is violated. Currently,I am planning to solve it using the Chain-of-Responsibility Pattern
class PropertyController
{
public function saveAction($request, $response, $args)
{
$data = $req->getParsedBody();
$dataValidation = new DataValidation();
$recordLocking = new RecordLocking();
$editHistoryLogging = new EditHistoryLogging();
$destinationDelegation = new DestinationDelegation();
$dataValidation->next($recordLocking);
$recordLocking->next($editHistoryLogging);
$editHistoryLogging->next($destinationDelegation);
$propertyService = new PropertyService(new PropertyEloquentRepository());
$result = $propertyService->save($data, $dataValidation);
return $response->withStatus(200)
->withHeader('Content-Type','application/json')
->write(json_encode($result));
}
}
Is this implementation fine and is considered a good practice?
php architecture architectural-patterns
I am currently developing a REST Api using Slim Framework with a Layered Architecture:
Controller->Service->Repository->DB
My problem is the save() function on one of my services contains a collection of complex functions which clearly violates the Separation of Responsibility Principle so I grouped the functions based on their "responsibilities". Here are some of it:
- Data Validation
- Record Locking
- Edit History Logging
- Destination Delegation
These functions are not always executed. It executes depending on the data passed onto the service and some of the functions blocks the saving if a business rule is violated. Currently,I am planning to solve it using the Chain-of-Responsibility Pattern
class PropertyController
{
public function saveAction($request, $response, $args)
{
$data = $req->getParsedBody();
$dataValidation = new DataValidation();
$recordLocking = new RecordLocking();
$editHistoryLogging = new EditHistoryLogging();
$destinationDelegation = new DestinationDelegation();
$dataValidation->next($recordLocking);
$recordLocking->next($editHistoryLogging);
$editHistoryLogging->next($destinationDelegation);
$propertyService = new PropertyService(new PropertyEloquentRepository());
$result = $propertyService->save($data, $dataValidation);
return $response->withStatus(200)
->withHeader('Content-Type','application/json')
->write(json_encode($result));
}
}
Is this implementation fine and is considered a good practice?
php architecture architectural-patterns
php architecture architectural-patterns
asked Nov 8 at 4:36


straycat
113
113
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53201617%2fservice-layer-with-multiple-validation-and-other-functions%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8B,RNoer36N0UuzRG6LtpxXl1awT 0