Bitronix PoolingConnectionFactory making multiple connections that won't close
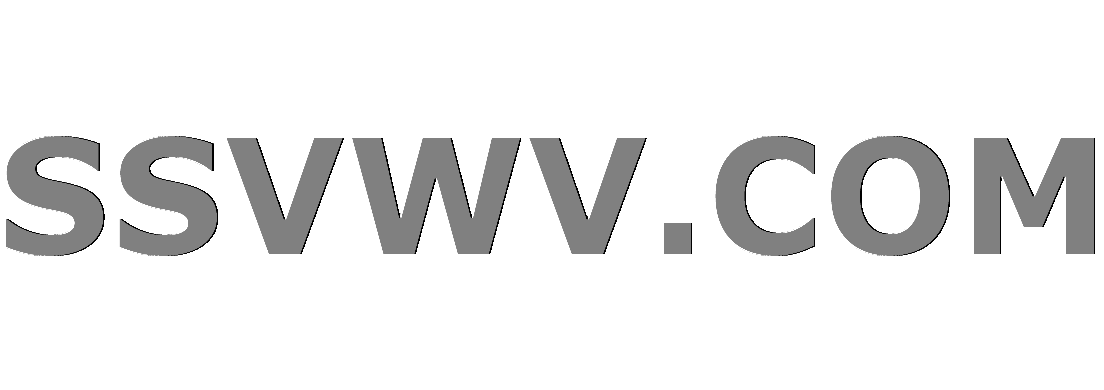
Multi tool use
We are using Bitronix XA as the jta transaction manager in our spring boot app. Problem is that it is making multiple connections that eats up resources on the MQ server. We are using IBM websphere as MQ server. For some reason Bitronix is not honouring the poolsize that we have given. Another observation is that connection leaks happen when we receive or send a burst of messages concurrently. Below are the configurations in code. Please advise how to solve the connection leak problem.
PoolingConnectionFactory:
PoolingConnectionFactory connectionFactory = new PoolingConnectionFactory();
connectionFactory.setClassName("com.ibm.mq.jms.MQXAQueueConnectionFactory");
connectionFactory.setUniqueName(XA_FACTORY_NAME);
connectionFactory.setAllowLocalTransactions(false);
connectionFactory.setTestConnections(true);
connectionFactory.setUser(user);
connectionFactory.setPassword(password);
connectionFactory.setMaxPoolSize(20);
Properties driverProperties = connectionFactory.getDriverProperties();
driverProperties.setProperty("port", ...);
driverProperties.setProperty("transportType", ...);
driverProperties.setProperty("channel", ...);
driverProperties.setProperty("hostName", ...);
driverProperties.setProperty("queueManager", ...);
Btm configs:
@Bean
@Profile({"cloud", "local"})
@DependsOn("instanceInfo")
public bitronix.tm.Configuration btmConfig() {
bitronix.tm.Configuration btmConfig = TransactionManagerServices.getConfiguration();
btmConfig.setDisableJmx(true);
btmConfig.setServerId(instanceInfo);
return btmConfig;
}
@Bean
@DependsOn("btmConfig")
public BitronixTransactionManager bitronixTransactionManager() {
BitronixTransactionManager transactionManager = TransactionManagerServices.getTransactionManager();
return transactionManager;
}
Messagecontainer used in SpringIntegrationFlows:
private DefaultMessageListenerContainer createMessageContainer(MessageListenerAdapter messageListenerAdapter,
ConnectionFactory connectionFactory, String queue, JtaTransactionManager jtaTransactionManager) {
DefaultMessageListenerContainer container = new DefaultMessageListenerContainer();
container.setMessageListener(messageListenerAdapter);
container.setConnectionFactory(connectionFactory);
container.setDestinationName(queue);
container.setSessionTransacted(true);
container.setTransactionManager(jtaTransactionManager);
container.setErrorHandler(containerErrorHandler);
return container;
}
PoolingDataSource for DB connection:
public PoolingDataSource createPooledDataSource(String driverClassName, String userName, String password, String jdbcURL, String validationQuery) {
PoolingDataSource dataSource = new PoolingDataSource();
dataSource.setUniqueName("dataSource");
dataSource.setMaxIdleTime(8);
dataSource.setMinPoolSize(1);
dataSource.setMaxPoolSize(20);
dataSource.setTestQuery(validationQuery);
dataSource.getDriverProperties().setProperty("user", userName);
dataSource.getDriverProperties().setProperty("password", password);
dataSource.getDriverProperties().setProperty("URL", jdbcURL);
dataSource.setClassName(driverClassName);
dataSource.setTestQuery(validationQuery);
dataSource.setAllowLocalTransactions(true);
dataSource.init();
return dataSource;
}
spring-boot ibm-mq xa bitronix
add a comment |
We are using Bitronix XA as the jta transaction manager in our spring boot app. Problem is that it is making multiple connections that eats up resources on the MQ server. We are using IBM websphere as MQ server. For some reason Bitronix is not honouring the poolsize that we have given. Another observation is that connection leaks happen when we receive or send a burst of messages concurrently. Below are the configurations in code. Please advise how to solve the connection leak problem.
PoolingConnectionFactory:
PoolingConnectionFactory connectionFactory = new PoolingConnectionFactory();
connectionFactory.setClassName("com.ibm.mq.jms.MQXAQueueConnectionFactory");
connectionFactory.setUniqueName(XA_FACTORY_NAME);
connectionFactory.setAllowLocalTransactions(false);
connectionFactory.setTestConnections(true);
connectionFactory.setUser(user);
connectionFactory.setPassword(password);
connectionFactory.setMaxPoolSize(20);
Properties driverProperties = connectionFactory.getDriverProperties();
driverProperties.setProperty("port", ...);
driverProperties.setProperty("transportType", ...);
driverProperties.setProperty("channel", ...);
driverProperties.setProperty("hostName", ...);
driverProperties.setProperty("queueManager", ...);
Btm configs:
@Bean
@Profile({"cloud", "local"})
@DependsOn("instanceInfo")
public bitronix.tm.Configuration btmConfig() {
bitronix.tm.Configuration btmConfig = TransactionManagerServices.getConfiguration();
btmConfig.setDisableJmx(true);
btmConfig.setServerId(instanceInfo);
return btmConfig;
}
@Bean
@DependsOn("btmConfig")
public BitronixTransactionManager bitronixTransactionManager() {
BitronixTransactionManager transactionManager = TransactionManagerServices.getTransactionManager();
return transactionManager;
}
Messagecontainer used in SpringIntegrationFlows:
private DefaultMessageListenerContainer createMessageContainer(MessageListenerAdapter messageListenerAdapter,
ConnectionFactory connectionFactory, String queue, JtaTransactionManager jtaTransactionManager) {
DefaultMessageListenerContainer container = new DefaultMessageListenerContainer();
container.setMessageListener(messageListenerAdapter);
container.setConnectionFactory(connectionFactory);
container.setDestinationName(queue);
container.setSessionTransacted(true);
container.setTransactionManager(jtaTransactionManager);
container.setErrorHandler(containerErrorHandler);
return container;
}
PoolingDataSource for DB connection:
public PoolingDataSource createPooledDataSource(String driverClassName, String userName, String password, String jdbcURL, String validationQuery) {
PoolingDataSource dataSource = new PoolingDataSource();
dataSource.setUniqueName("dataSource");
dataSource.setMaxIdleTime(8);
dataSource.setMinPoolSize(1);
dataSource.setMaxPoolSize(20);
dataSource.setTestQuery(validationQuery);
dataSource.getDriverProperties().setProperty("user", userName);
dataSource.getDriverProperties().setProperty("password", password);
dataSource.getDriverProperties().setProperty("URL", jdbcURL);
dataSource.setClassName(driverClassName);
dataSource.setTestQuery(validationQuery);
dataSource.setAllowLocalTransactions(true);
dataSource.init();
return dataSource;
}
spring-boot ibm-mq xa bitronix
add a comment |
We are using Bitronix XA as the jta transaction manager in our spring boot app. Problem is that it is making multiple connections that eats up resources on the MQ server. We are using IBM websphere as MQ server. For some reason Bitronix is not honouring the poolsize that we have given. Another observation is that connection leaks happen when we receive or send a burst of messages concurrently. Below are the configurations in code. Please advise how to solve the connection leak problem.
PoolingConnectionFactory:
PoolingConnectionFactory connectionFactory = new PoolingConnectionFactory();
connectionFactory.setClassName("com.ibm.mq.jms.MQXAQueueConnectionFactory");
connectionFactory.setUniqueName(XA_FACTORY_NAME);
connectionFactory.setAllowLocalTransactions(false);
connectionFactory.setTestConnections(true);
connectionFactory.setUser(user);
connectionFactory.setPassword(password);
connectionFactory.setMaxPoolSize(20);
Properties driverProperties = connectionFactory.getDriverProperties();
driverProperties.setProperty("port", ...);
driverProperties.setProperty("transportType", ...);
driverProperties.setProperty("channel", ...);
driverProperties.setProperty("hostName", ...);
driverProperties.setProperty("queueManager", ...);
Btm configs:
@Bean
@Profile({"cloud", "local"})
@DependsOn("instanceInfo")
public bitronix.tm.Configuration btmConfig() {
bitronix.tm.Configuration btmConfig = TransactionManagerServices.getConfiguration();
btmConfig.setDisableJmx(true);
btmConfig.setServerId(instanceInfo);
return btmConfig;
}
@Bean
@DependsOn("btmConfig")
public BitronixTransactionManager bitronixTransactionManager() {
BitronixTransactionManager transactionManager = TransactionManagerServices.getTransactionManager();
return transactionManager;
}
Messagecontainer used in SpringIntegrationFlows:
private DefaultMessageListenerContainer createMessageContainer(MessageListenerAdapter messageListenerAdapter,
ConnectionFactory connectionFactory, String queue, JtaTransactionManager jtaTransactionManager) {
DefaultMessageListenerContainer container = new DefaultMessageListenerContainer();
container.setMessageListener(messageListenerAdapter);
container.setConnectionFactory(connectionFactory);
container.setDestinationName(queue);
container.setSessionTransacted(true);
container.setTransactionManager(jtaTransactionManager);
container.setErrorHandler(containerErrorHandler);
return container;
}
PoolingDataSource for DB connection:
public PoolingDataSource createPooledDataSource(String driverClassName, String userName, String password, String jdbcURL, String validationQuery) {
PoolingDataSource dataSource = new PoolingDataSource();
dataSource.setUniqueName("dataSource");
dataSource.setMaxIdleTime(8);
dataSource.setMinPoolSize(1);
dataSource.setMaxPoolSize(20);
dataSource.setTestQuery(validationQuery);
dataSource.getDriverProperties().setProperty("user", userName);
dataSource.getDriverProperties().setProperty("password", password);
dataSource.getDriverProperties().setProperty("URL", jdbcURL);
dataSource.setClassName(driverClassName);
dataSource.setTestQuery(validationQuery);
dataSource.setAllowLocalTransactions(true);
dataSource.init();
return dataSource;
}
spring-boot ibm-mq xa bitronix
We are using Bitronix XA as the jta transaction manager in our spring boot app. Problem is that it is making multiple connections that eats up resources on the MQ server. We are using IBM websphere as MQ server. For some reason Bitronix is not honouring the poolsize that we have given. Another observation is that connection leaks happen when we receive or send a burst of messages concurrently. Below are the configurations in code. Please advise how to solve the connection leak problem.
PoolingConnectionFactory:
PoolingConnectionFactory connectionFactory = new PoolingConnectionFactory();
connectionFactory.setClassName("com.ibm.mq.jms.MQXAQueueConnectionFactory");
connectionFactory.setUniqueName(XA_FACTORY_NAME);
connectionFactory.setAllowLocalTransactions(false);
connectionFactory.setTestConnections(true);
connectionFactory.setUser(user);
connectionFactory.setPassword(password);
connectionFactory.setMaxPoolSize(20);
Properties driverProperties = connectionFactory.getDriverProperties();
driverProperties.setProperty("port", ...);
driverProperties.setProperty("transportType", ...);
driverProperties.setProperty("channel", ...);
driverProperties.setProperty("hostName", ...);
driverProperties.setProperty("queueManager", ...);
Btm configs:
@Bean
@Profile({"cloud", "local"})
@DependsOn("instanceInfo")
public bitronix.tm.Configuration btmConfig() {
bitronix.tm.Configuration btmConfig = TransactionManagerServices.getConfiguration();
btmConfig.setDisableJmx(true);
btmConfig.setServerId(instanceInfo);
return btmConfig;
}
@Bean
@DependsOn("btmConfig")
public BitronixTransactionManager bitronixTransactionManager() {
BitronixTransactionManager transactionManager = TransactionManagerServices.getTransactionManager();
return transactionManager;
}
Messagecontainer used in SpringIntegrationFlows:
private DefaultMessageListenerContainer createMessageContainer(MessageListenerAdapter messageListenerAdapter,
ConnectionFactory connectionFactory, String queue, JtaTransactionManager jtaTransactionManager) {
DefaultMessageListenerContainer container = new DefaultMessageListenerContainer();
container.setMessageListener(messageListenerAdapter);
container.setConnectionFactory(connectionFactory);
container.setDestinationName(queue);
container.setSessionTransacted(true);
container.setTransactionManager(jtaTransactionManager);
container.setErrorHandler(containerErrorHandler);
return container;
}
PoolingDataSource for DB connection:
public PoolingDataSource createPooledDataSource(String driverClassName, String userName, String password, String jdbcURL, String validationQuery) {
PoolingDataSource dataSource = new PoolingDataSource();
dataSource.setUniqueName("dataSource");
dataSource.setMaxIdleTime(8);
dataSource.setMinPoolSize(1);
dataSource.setMaxPoolSize(20);
dataSource.setTestQuery(validationQuery);
dataSource.getDriverProperties().setProperty("user", userName);
dataSource.getDriverProperties().setProperty("password", password);
dataSource.getDriverProperties().setProperty("URL", jdbcURL);
dataSource.setClassName(driverClassName);
dataSource.setTestQuery(validationQuery);
dataSource.setAllowLocalTransactions(true);
dataSource.init();
return dataSource;
}
spring-boot ibm-mq xa bitronix
spring-boot ibm-mq xa bitronix
edited Dec 16 '18 at 6:37
JoshMc
5,3841623
5,3841623
asked Nov 16 '18 at 8:31
ramitramit
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Sounds like IBMMQ pulled connection and session pooling out of their client jar, as described in this technote. This could explain higher than maxPoolSize
connection counts, especially if you don't pool xaSession
s explicitly. BTM maxPoolSize
applies to JmsPooledConnection
, but those addtional connections could come from xaSession
s and not controlled by maxPoolSize
.
I'm not familiar Spring or you setup, but read recommendations of using CachingConnectionFactory
(eg. ref-1, ref-2), and seems like IBMMQ added certain support for this (github link, especially how
section. I don't have any experience using them though....).
Btw, ShareConversation can be used to control number of connections as well, which defaults to 10 on server side. IBM doc (link) mentions a potential 15% perf penalty using it.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53334069%2fbitronix-poolingconnectionfactory-making-multiple-connections-that-wont-close%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sounds like IBMMQ pulled connection and session pooling out of their client jar, as described in this technote. This could explain higher than maxPoolSize
connection counts, especially if you don't pool xaSession
s explicitly. BTM maxPoolSize
applies to JmsPooledConnection
, but those addtional connections could come from xaSession
s and not controlled by maxPoolSize
.
I'm not familiar Spring or you setup, but read recommendations of using CachingConnectionFactory
(eg. ref-1, ref-2), and seems like IBMMQ added certain support for this (github link, especially how
section. I don't have any experience using them though....).
Btw, ShareConversation can be used to control number of connections as well, which defaults to 10 on server side. IBM doc (link) mentions a potential 15% perf penalty using it.
add a comment |
Sounds like IBMMQ pulled connection and session pooling out of their client jar, as described in this technote. This could explain higher than maxPoolSize
connection counts, especially if you don't pool xaSession
s explicitly. BTM maxPoolSize
applies to JmsPooledConnection
, but those addtional connections could come from xaSession
s and not controlled by maxPoolSize
.
I'm not familiar Spring or you setup, but read recommendations of using CachingConnectionFactory
(eg. ref-1, ref-2), and seems like IBMMQ added certain support for this (github link, especially how
section. I don't have any experience using them though....).
Btw, ShareConversation can be used to control number of connections as well, which defaults to 10 on server side. IBM doc (link) mentions a potential 15% perf penalty using it.
add a comment |
Sounds like IBMMQ pulled connection and session pooling out of their client jar, as described in this technote. This could explain higher than maxPoolSize
connection counts, especially if you don't pool xaSession
s explicitly. BTM maxPoolSize
applies to JmsPooledConnection
, but those addtional connections could come from xaSession
s and not controlled by maxPoolSize
.
I'm not familiar Spring or you setup, but read recommendations of using CachingConnectionFactory
(eg. ref-1, ref-2), and seems like IBMMQ added certain support for this (github link, especially how
section. I don't have any experience using them though....).
Btw, ShareConversation can be used to control number of connections as well, which defaults to 10 on server side. IBM doc (link) mentions a potential 15% perf penalty using it.
Sounds like IBMMQ pulled connection and session pooling out of their client jar, as described in this technote. This could explain higher than maxPoolSize
connection counts, especially if you don't pool xaSession
s explicitly. BTM maxPoolSize
applies to JmsPooledConnection
, but those addtional connections could come from xaSession
s and not controlled by maxPoolSize
.
I'm not familiar Spring or you setup, but read recommendations of using CachingConnectionFactory
(eg. ref-1, ref-2), and seems like IBMMQ added certain support for this (github link, especially how
section. I don't have any experience using them though....).
Btw, ShareConversation can be used to control number of connections as well, which defaults to 10 on server side. IBM doc (link) mentions a potential 15% perf penalty using it.
edited Dec 7 '18 at 19:13
answered Nov 16 '18 at 19:22
fall14123fall14123
6318
6318
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53334069%2fbitronix-poolingconnectionfactory-making-multiple-connections-that-wont-close%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
CD1q0RQ5 RfPjyo5jotLw,W7cX,E44j0PkqGIaobN39yphRxJJD