Chart.js isn't able to read elements from php as label
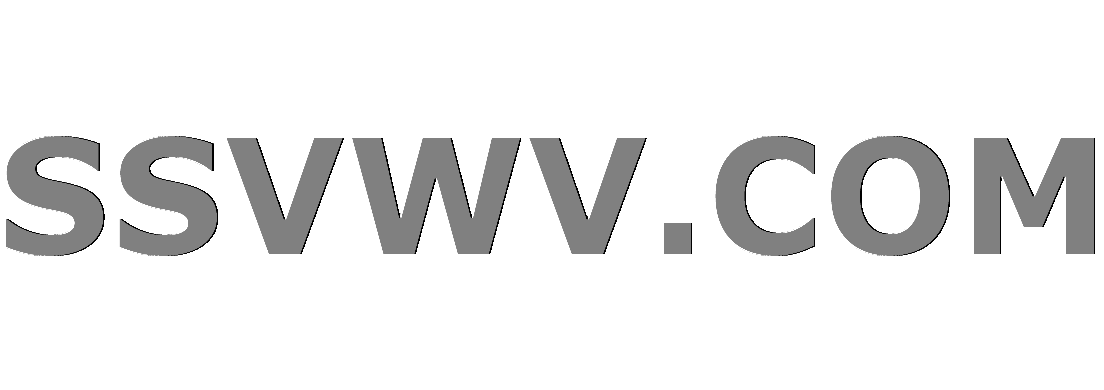
Multi tool use
I tried creating a table that gets its data from a mySQL database. Everything works fine except when I try to use time as labels for the graph.
Here is the code for the PHP:
<?php
// set arrays
$temp = array();
$humi = array();
$id = array();
$chronos = array();
// get
$query = "SELECT * FROM temp_humi";
$result = mysqli_query($connection, $query);
// transfer arrays
while($row = mysqli_fetch_assoc($result)) {
$temp = $row["temp"];
$humi = $row["humi"];
$id = $row["id"];
}
// set variables
$res1;
$res2;
$res3;
$res4;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 .= $temp[$x] . ', ';
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 .= $humi[$x] . ', ';
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 .= $id[$x] . ', ';
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= $chronos[$x] . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
$res3 = rtrim($res3, ', ');
$res4 = rtrim($res4, ', ');
?>
I used arrays to input all the data from the mySQL database then put the elements of an array into a variable so that i can call it in the chart as values for the data and the label.
While this is my code for the chart in another php file:
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo $res4; ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo $res1; ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo $res2; ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
When I try to use the ids as the value for the labels (the one named as $res3) , it works just fine. But when I try to use the time as labels ($res4), the page becomes empty.
Please help me. I tried turning the time values as strings by using (string)$var and strval($var) because I think it's because time is not a string but it didn't work.
Please help. I just started using Chart.js
javascript php chart.js
|
show 5 more comments
I tried creating a table that gets its data from a mySQL database. Everything works fine except when I try to use time as labels for the graph.
Here is the code for the PHP:
<?php
// set arrays
$temp = array();
$humi = array();
$id = array();
$chronos = array();
// get
$query = "SELECT * FROM temp_humi";
$result = mysqli_query($connection, $query);
// transfer arrays
while($row = mysqli_fetch_assoc($result)) {
$temp = $row["temp"];
$humi = $row["humi"];
$id = $row["id"];
}
// set variables
$res1;
$res2;
$res3;
$res4;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 .= $temp[$x] . ', ';
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 .= $humi[$x] . ', ';
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 .= $id[$x] . ', ';
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= $chronos[$x] . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
$res3 = rtrim($res3, ', ');
$res4 = rtrim($res4, ', ');
?>
I used arrays to input all the data from the mySQL database then put the elements of an array into a variable so that i can call it in the chart as values for the data and the label.
While this is my code for the chart in another php file:
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo $res4; ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo $res1; ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo $res2; ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
When I try to use the ids as the value for the labels (the one named as $res3) , it works just fine. But when I try to use the time as labels ($res4), the page becomes empty.
Please help me. I tried turning the time values as strings by using (string)$var and strval($var) because I think it's because time is not a string but it didn't work.
Please help. I just started using Chart.js
javascript php chart.js
What is the output of<?php echo $res4; ?>
?
– akshaypjoshi
Nov 16 '18 at 8:42
This is the output: 16:44:04, 16:44:06, 16:44:08, 16:44:10, 16:44:12, 16:44:14, 16:44:16, 16:44:18, 16:44:20, 16:44:22
– Aldrine NAX
Nov 16 '18 at 8:44
It changes base on the current time.
– Aldrine NAX
Nov 16 '18 at 8:45
Your count of$res3
and$res4
must be same. Are you sure count of$res1
,$res2
, and$res3
is same as$res4
i.e. 10.
– akshaypjoshi
Nov 16 '18 at 8:48
I'm not sure why you've shared that PHP code. Have you already determined the data format expected by Chart.js and you've having difficulties generating that exact output?
– Álvaro González
Nov 16 '18 at 8:49
|
show 5 more comments
I tried creating a table that gets its data from a mySQL database. Everything works fine except when I try to use time as labels for the graph.
Here is the code for the PHP:
<?php
// set arrays
$temp = array();
$humi = array();
$id = array();
$chronos = array();
// get
$query = "SELECT * FROM temp_humi";
$result = mysqli_query($connection, $query);
// transfer arrays
while($row = mysqli_fetch_assoc($result)) {
$temp = $row["temp"];
$humi = $row["humi"];
$id = $row["id"];
}
// set variables
$res1;
$res2;
$res3;
$res4;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 .= $temp[$x] . ', ';
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 .= $humi[$x] . ', ';
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 .= $id[$x] . ', ';
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= $chronos[$x] . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
$res3 = rtrim($res3, ', ');
$res4 = rtrim($res4, ', ');
?>
I used arrays to input all the data from the mySQL database then put the elements of an array into a variable so that i can call it in the chart as values for the data and the label.
While this is my code for the chart in another php file:
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo $res4; ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo $res1; ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo $res2; ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
When I try to use the ids as the value for the labels (the one named as $res3) , it works just fine. But when I try to use the time as labels ($res4), the page becomes empty.
Please help me. I tried turning the time values as strings by using (string)$var and strval($var) because I think it's because time is not a string but it didn't work.
Please help. I just started using Chart.js
javascript php chart.js
I tried creating a table that gets its data from a mySQL database. Everything works fine except when I try to use time as labels for the graph.
Here is the code for the PHP:
<?php
// set arrays
$temp = array();
$humi = array();
$id = array();
$chronos = array();
// get
$query = "SELECT * FROM temp_humi";
$result = mysqli_query($connection, $query);
// transfer arrays
while($row = mysqli_fetch_assoc($result)) {
$temp = $row["temp"];
$humi = $row["humi"];
$id = $row["id"];
}
// set variables
$res1;
$res2;
$res3;
$res4;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 .= $temp[$x] . ', ';
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 .= $humi[$x] . ', ';
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 .= $id[$x] . ', ';
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= $chronos[$x] . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
$res3 = rtrim($res3, ', ');
$res4 = rtrim($res4, ', ');
?>
I used arrays to input all the data from the mySQL database then put the elements of an array into a variable so that i can call it in the chart as values for the data and the label.
While this is my code for the chart in another php file:
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo $res4; ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo $res1; ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo $res2; ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
When I try to use the ids as the value for the labels (the one named as $res3) , it works just fine. But when I try to use the time as labels ($res4), the page becomes empty.
Please help me. I tried turning the time values as strings by using (string)$var and strval($var) because I think it's because time is not a string but it didn't work.
Please help. I just started using Chart.js
javascript php chart.js
javascript php chart.js
asked Nov 16 '18 at 8:30
Aldrine NAXAldrine NAX
196
196
What is the output of<?php echo $res4; ?>
?
– akshaypjoshi
Nov 16 '18 at 8:42
This is the output: 16:44:04, 16:44:06, 16:44:08, 16:44:10, 16:44:12, 16:44:14, 16:44:16, 16:44:18, 16:44:20, 16:44:22
– Aldrine NAX
Nov 16 '18 at 8:44
It changes base on the current time.
– Aldrine NAX
Nov 16 '18 at 8:45
Your count of$res3
and$res4
must be same. Are you sure count of$res1
,$res2
, and$res3
is same as$res4
i.e. 10.
– akshaypjoshi
Nov 16 '18 at 8:48
I'm not sure why you've shared that PHP code. Have you already determined the data format expected by Chart.js and you've having difficulties generating that exact output?
– Álvaro González
Nov 16 '18 at 8:49
|
show 5 more comments
What is the output of<?php echo $res4; ?>
?
– akshaypjoshi
Nov 16 '18 at 8:42
This is the output: 16:44:04, 16:44:06, 16:44:08, 16:44:10, 16:44:12, 16:44:14, 16:44:16, 16:44:18, 16:44:20, 16:44:22
– Aldrine NAX
Nov 16 '18 at 8:44
It changes base on the current time.
– Aldrine NAX
Nov 16 '18 at 8:45
Your count of$res3
and$res4
must be same. Are you sure count of$res1
,$res2
, and$res3
is same as$res4
i.e. 10.
– akshaypjoshi
Nov 16 '18 at 8:48
I'm not sure why you've shared that PHP code. Have you already determined the data format expected by Chart.js and you've having difficulties generating that exact output?
– Álvaro González
Nov 16 '18 at 8:49
What is the output of
<?php echo $res4; ?>
?– akshaypjoshi
Nov 16 '18 at 8:42
What is the output of
<?php echo $res4; ?>
?– akshaypjoshi
Nov 16 '18 at 8:42
This is the output: 16:44:04, 16:44:06, 16:44:08, 16:44:10, 16:44:12, 16:44:14, 16:44:16, 16:44:18, 16:44:20, 16:44:22
– Aldrine NAX
Nov 16 '18 at 8:44
This is the output: 16:44:04, 16:44:06, 16:44:08, 16:44:10, 16:44:12, 16:44:14, 16:44:16, 16:44:18, 16:44:20, 16:44:22
– Aldrine NAX
Nov 16 '18 at 8:44
It changes base on the current time.
– Aldrine NAX
Nov 16 '18 at 8:45
It changes base on the current time.
– Aldrine NAX
Nov 16 '18 at 8:45
Your count of
$res3
and $res4
must be same. Are you sure count of $res1
, $res2
, and $res3
is same as $res4
i.e. 10.– akshaypjoshi
Nov 16 '18 at 8:48
Your count of
$res3
and $res4
must be same. Are you sure count of $res1
, $res2
, and $res3
is same as $res4
i.e. 10.– akshaypjoshi
Nov 16 '18 at 8:48
I'm not sure why you've shared that PHP code. Have you already determined the data format expected by Chart.js and you've having difficulties generating that exact output?
– Álvaro González
Nov 16 '18 at 8:49
I'm not sure why you've shared that PHP code. Have you already determined the data format expected by Chart.js and you've having difficulties generating that exact output?
– Álvaro González
Nov 16 '18 at 8:49
|
show 5 more comments
2 Answers
2
active
oldest
votes
Since the output is not an integer, but a time ("16:44:04"), you can not place it inside an array without quotes.
$res4 .= '"'.$chronos[$x] . '", ';
As a more general approach, I don't suggest you to use string concatenation but PHP arrays and then implode them. This avoids having to trim the last comma.
$res1 = ;
$res2 = ;
$res3 = ;
$res4 = ;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 = $temp[$x];
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 = $humi[$x];
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 = $id[$x];
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 = '"'.$chronos[$x].'"';
}
?>
And then
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo implode(",", $res4); ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo implode(",", $res1); ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo implode(",", $res2); ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
add a comment |
Your $res4 is an array of string, not date, so labels: [<?php echo $res4; ?>],
will be like:
labels: [10:00:01, 10:00:02, 10:00:03, ...]
Javascript cannot read it because SyntaxError. You may need to put $res4 into an temporary array, then parse it to time before assign it to labels.
But before that, you need to quote your $chronos[$x]
like this:
...
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= '"$chronos[$x]"' . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
...
And then in your front end
var temp = [<?php echo $res4 ?>];
// parse your time here
Hope this help.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53334055%2fchart-js-isnt-able-to-read-elements-from-php-as-label%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Since the output is not an integer, but a time ("16:44:04"), you can not place it inside an array without quotes.
$res4 .= '"'.$chronos[$x] . '", ';
As a more general approach, I don't suggest you to use string concatenation but PHP arrays and then implode them. This avoids having to trim the last comma.
$res1 = ;
$res2 = ;
$res3 = ;
$res4 = ;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 = $temp[$x];
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 = $humi[$x];
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 = $id[$x];
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 = '"'.$chronos[$x].'"';
}
?>
And then
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo implode(",", $res4); ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo implode(",", $res1); ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo implode(",", $res2); ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
add a comment |
Since the output is not an integer, but a time ("16:44:04"), you can not place it inside an array without quotes.
$res4 .= '"'.$chronos[$x] . '", ';
As a more general approach, I don't suggest you to use string concatenation but PHP arrays and then implode them. This avoids having to trim the last comma.
$res1 = ;
$res2 = ;
$res3 = ;
$res4 = ;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 = $temp[$x];
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 = $humi[$x];
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 = $id[$x];
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 = '"'.$chronos[$x].'"';
}
?>
And then
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo implode(",", $res4); ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo implode(",", $res1); ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo implode(",", $res2); ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
add a comment |
Since the output is not an integer, but a time ("16:44:04"), you can not place it inside an array without quotes.
$res4 .= '"'.$chronos[$x] . '", ';
As a more general approach, I don't suggest you to use string concatenation but PHP arrays and then implode them. This avoids having to trim the last comma.
$res1 = ;
$res2 = ;
$res3 = ;
$res4 = ;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 = $temp[$x];
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 = $humi[$x];
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 = $id[$x];
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 = '"'.$chronos[$x].'"';
}
?>
And then
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo implode(",", $res4); ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo implode(",", $res1); ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo implode(",", $res2); ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
Since the output is not an integer, but a time ("16:44:04"), you can not place it inside an array without quotes.
$res4 .= '"'.$chronos[$x] . '", ';
As a more general approach, I don't suggest you to use string concatenation but PHP arrays and then implode them. This avoids having to trim the last comma.
$res1 = ;
$res2 = ;
$res3 = ;
$res4 = ;
$len1 = count($temp);
$len2 = count($humi);
$len3 = count($id);
// input elements into variables
for($x = $len1 - 10; $x < $len1; $x++) {
$res1 = $temp[$x];
}
for($x = $len2 - 10; $x < $len2; $x++) {
$res2 = $humi[$x];
}
for($x = $len3 - 10; $x < $len3; $x++) {
$res3 = $id[$x];
}
//set timezone
date_default_timezone_set("Asia/Manila");
// set time
$date = date("H:i:s");
$time = strtotime($date);
$time = $time - 20;
// iterate time into array
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 = '"'.$chronos[$x].'"';
}
?>
And then
<script>
var ctx = document.getElementById("myChart");
var myChart = new Chart(ctx, {
type: 'line',
data: {
labels: [<?php echo implode(",", $res4); ?>],
datasets: [{
label: 'Temperature',
fill: false,
data: [<?php echo implode(",", $res1); ?>],
borderColor: ['rgba(255,99,132,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}, {
label: 'Humidity',
fill: false,
data: [<?php echo implode(",", $res2); ?>],
borderColor: ['rgba(54,162,235,1)'],
borderWidth: 1,
pointBackgroundColor: [...]
}]
}
});
</script>
answered Nov 16 '18 at 8:53
Andrea OlivatoAndrea Olivato
209211
209211
add a comment |
add a comment |
Your $res4 is an array of string, not date, so labels: [<?php echo $res4; ?>],
will be like:
labels: [10:00:01, 10:00:02, 10:00:03, ...]
Javascript cannot read it because SyntaxError. You may need to put $res4 into an temporary array, then parse it to time before assign it to labels.
But before that, you need to quote your $chronos[$x]
like this:
...
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= '"$chronos[$x]"' . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
...
And then in your front end
var temp = [<?php echo $res4 ?>];
// parse your time here
Hope this help.
add a comment |
Your $res4 is an array of string, not date, so labels: [<?php echo $res4; ?>],
will be like:
labels: [10:00:01, 10:00:02, 10:00:03, ...]
Javascript cannot read it because SyntaxError. You may need to put $res4 into an temporary array, then parse it to time before assign it to labels.
But before that, you need to quote your $chronos[$x]
like this:
...
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= '"$chronos[$x]"' . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
...
And then in your front end
var temp = [<?php echo $res4 ?>];
// parse your time here
Hope this help.
add a comment |
Your $res4 is an array of string, not date, so labels: [<?php echo $res4; ?>],
will be like:
labels: [10:00:01, 10:00:02, 10:00:03, ...]
Javascript cannot read it because SyntaxError. You may need to put $res4 into an temporary array, then parse it to time before assign it to labels.
But before that, you need to quote your $chronos[$x]
like this:
...
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= '"$chronos[$x]"' . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
...
And then in your front end
var temp = [<?php echo $res4 ?>];
// parse your time here
Hope this help.
Your $res4 is an array of string, not date, so labels: [<?php echo $res4; ?>],
will be like:
labels: [10:00:01, 10:00:02, 10:00:03, ...]
Javascript cannot read it because SyntaxError. You may need to put $res4 into an temporary array, then parse it to time before assign it to labels.
But before that, you need to quote your $chronos[$x]
like this:
...
for($y = 0; $y < 10; $y++) {
$time = $time + 2;
$date = date("H:i:s", $time);
array_push($chronos, $date);
}
// iterate timesinto variables
for($x = 0; $x < 10; $x++) {
$res4 .= '"$chronos[$x]"' . ', ';
}
// trim last comma
$res1 = rtrim($res1, ', ');
$res2 = rtrim($res2, ', ');
...
And then in your front end
var temp = [<?php echo $res4 ?>];
// parse your time here
Hope this help.
answered Nov 16 '18 at 8:53


Van ThoVan Tho
846
846
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53334055%2fchart-js-isnt-able-to-read-elements-from-php-as-label%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tJjBtLe 7DLUeg,OIxRbHuXb j,f,R1 I8,xsVm3,C2yx68uYcrhlIpiSNVF 5ZtkNT,Wf41fwP4
What is the output of
<?php echo $res4; ?>
?– akshaypjoshi
Nov 16 '18 at 8:42
This is the output: 16:44:04, 16:44:06, 16:44:08, 16:44:10, 16:44:12, 16:44:14, 16:44:16, 16:44:18, 16:44:20, 16:44:22
– Aldrine NAX
Nov 16 '18 at 8:44
It changes base on the current time.
– Aldrine NAX
Nov 16 '18 at 8:45
Your count of
$res3
and$res4
must be same. Are you sure count of$res1
,$res2
, and$res3
is same as$res4
i.e. 10.– akshaypjoshi
Nov 16 '18 at 8:48
I'm not sure why you've shared that PHP code. Have you already determined the data format expected by Chart.js and you've having difficulties generating that exact output?
– Álvaro González
Nov 16 '18 at 8:49