How to handle back button when TexInput is focused
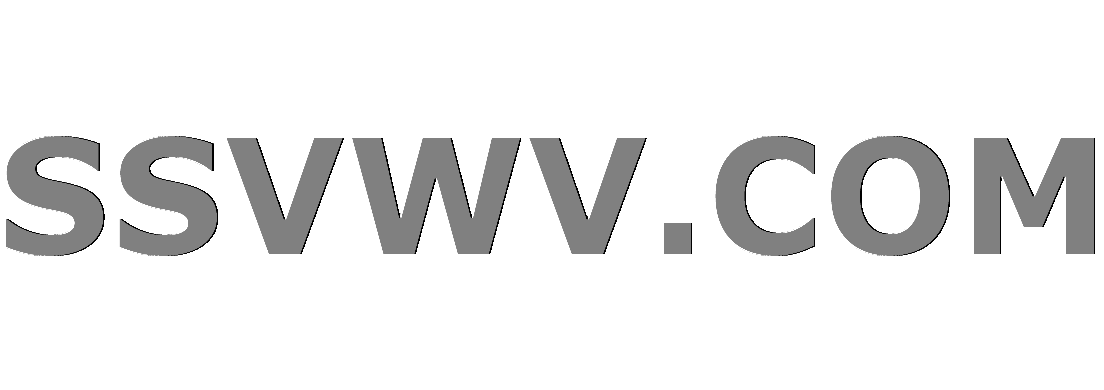
Multi tool use
up vote
0
down vote
favorite
This is a react-native question for Android.
How can I handle the back button in Android when a TextInput is focused?
BackHandler.addEventListener('hardwareBackPress'. () => {})
does not catch any event if TextInput is focused. It automatically dismisses the keyboard.
(Actually what I am trying to achieve is to remove the cursor when Back Button is pressed and the keyboard is dismissed)
You can play with this expo snack to understand what I am talking about:

add a comment |
up vote
0
down vote
favorite
This is a react-native question for Android.
How can I handle the back button in Android when a TextInput is focused?
BackHandler.addEventListener('hardwareBackPress'. () => {})
does not catch any event if TextInput is focused. It automatically dismisses the keyboard.
(Actually what I am trying to achieve is to remove the cursor when Back Button is pressed and the keyboard is dismissed)
You can play with this expo snack to understand what I am talking about:

No need to add the tags to the title of the question
– perissf
Nov 7 at 16:54
Never add code from external links. Instead, provide a mvce inside the question. Don't add the answer in the question. If the answers provided were not helpful enough, add your own answer.
– perissf
Nov 7 at 19:57
Thanks for your feedback @perissf. I edited the question accordingly. I left the link to expo snack because my intention with the link is not to show the code but to allow anyone to scan the QR code with Expo app and actually see the behavior in its own Android phone.
– Francisco Sarmento
Nov 7 at 20:40
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
This is a react-native question for Android.
How can I handle the back button in Android when a TextInput is focused?
BackHandler.addEventListener('hardwareBackPress'. () => {})
does not catch any event if TextInput is focused. It automatically dismisses the keyboard.
(Actually what I am trying to achieve is to remove the cursor when Back Button is pressed and the keyboard is dismissed)
You can play with this expo snack to understand what I am talking about:

This is a react-native question for Android.
How can I handle the back button in Android when a TextInput is focused?
BackHandler.addEventListener('hardwareBackPress'. () => {})
does not catch any event if TextInput is focused. It automatically dismisses the keyboard.
(Actually what I am trying to achieve is to remove the cursor when Back Button is pressed and the keyboard is dismissed)
You can play with this expo snack to understand what I am talking about:


edited Nov 7 at 20:45
asked Nov 7 at 13:41


Francisco Sarmento
587
587
No need to add the tags to the title of the question
– perissf
Nov 7 at 16:54
Never add code from external links. Instead, provide a mvce inside the question. Don't add the answer in the question. If the answers provided were not helpful enough, add your own answer.
– perissf
Nov 7 at 19:57
Thanks for your feedback @perissf. I edited the question accordingly. I left the link to expo snack because my intention with the link is not to show the code but to allow anyone to scan the QR code with Expo app and actually see the behavior in its own Android phone.
– Francisco Sarmento
Nov 7 at 20:40
add a comment |
No need to add the tags to the title of the question
– perissf
Nov 7 at 16:54
Never add code from external links. Instead, provide a mvce inside the question. Don't add the answer in the question. If the answers provided were not helpful enough, add your own answer.
– perissf
Nov 7 at 19:57
Thanks for your feedback @perissf. I edited the question accordingly. I left the link to expo snack because my intention with the link is not to show the code but to allow anyone to scan the QR code with Expo app and actually see the behavior in its own Android phone.
– Francisco Sarmento
Nov 7 at 20:40
No need to add the tags to the title of the question
– perissf
Nov 7 at 16:54
No need to add the tags to the title of the question
– perissf
Nov 7 at 16:54
Never add code from external links. Instead, provide a mvce inside the question. Don't add the answer in the question. If the answers provided were not helpful enough, add your own answer.
– perissf
Nov 7 at 19:57
Never add code from external links. Instead, provide a mvce inside the question. Don't add the answer in the question. If the answers provided were not helpful enough, add your own answer.
– perissf
Nov 7 at 19:57
Thanks for your feedback @perissf. I edited the question accordingly. I left the link to expo snack because my intention with the link is not to show the code but to allow anyone to scan the QR code with Expo app and actually see the behavior in its own Android phone.
– Francisco Sarmento
Nov 7 at 20:40
Thanks for your feedback @perissf. I edited the question accordingly. I left the link to expo snack because my intention with the link is not to show the code but to allow anyone to scan the QR code with Expo app and actually see the behavior in its own Android phone.
– Francisco Sarmento
Nov 7 at 20:40
add a comment |
3 Answers
3
active
oldest
votes
up vote
1
down vote
I believe that is the correct behavior, but to make what you want, you may detect the keyboard itself hiding instead by using Keyboard (docs at https://facebook.github.io/react-native/docs/keyboard)
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
this.input.blur();
};
//Rest of component...
}
I prefer this method than using the onKeyPress event from TextInput because onKeyPress won't read hardware keyboard back presses, so if the user has a device with hardware back buttons, like some Android devices have, the onKeyPress won't work, this provides a more consistent experience.
This seems like the best workaround. Still, what I was looking for is more like an option in Android to ignore the back button handler that the keyboard seems to have, so I can be able to control the event myself.
– Francisco Sarmento
Nov 7 at 16:56
That's the thing @FranciscoSarmento, I don't think you can do that in React Native directly, as this is sometimes a problem even in pure Android. When you press back and a keyboard is open, the Input Media Editor (IME for short, also known as the keyboard open) receives that press first before your app, and the only way to surpass the system is to use a listener specific for this, called onKeyPreIme. Once the IME listens to a back press, it closes itself and consumes it. Up to RN v0.57.4, there isn't a way to access this listener.
– Danilo
Nov 7 at 17:50
add a comment |
up vote
0
down vote
You'd handle it on the TextInput
itself instead of using a BackHandler
. You can do this via the onKeyPress
prop
constructor(props){
super(props)
this.inputRef = React.createRef()
}
<TextInput
onKeyPress={({ nativeEvent }) => {
if(nativeEvent.key === 'Backspace'){
//your code
// if you want to remove focus then you can use a ref
Keyboard.dismiss();
this.inputRef.blur()
}
}}
ref={this.inputRef}
/>
Also it's important to note that on Android this event will only fire on the software keyboard, so if you're running on an emulator and use the backspace key on your computer's keyboard this will not work.
This will fire on keyboard backspace press and not on Android back button.
– Francisco Sarmento
Nov 7 at 16:47
add a comment |
up vote
0
down vote
@Danilo answer does work, but it has to be applied to all text inputs. I ended up using Danilo's solution with a small twist.
Keyboard.dismiss()
does blur any active TextInput, so on keyboardDidHide event I just call Keyboard.dismiss() (although the keyboard just got dismissed by pressing back button).
I just need to add this to my main component.
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
Keyboard.dismiss();
};
//Rest of component...
}
You can teste this solution in this expo snack.
In case your app has multiple TextInputs that onSubmitEditing focus the next TextInput, this is how I made it work:
...
keyboardDidHide = () => {
this.keyboardTimeout = setTimeout(() => Keyboard.dismiss(), 300)
};
keyboardDidShow = () => {
clearTimeout(this.keyboardTimeout)
};
...
add a comment |
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
I believe that is the correct behavior, but to make what you want, you may detect the keyboard itself hiding instead by using Keyboard (docs at https://facebook.github.io/react-native/docs/keyboard)
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
this.input.blur();
};
//Rest of component...
}
I prefer this method than using the onKeyPress event from TextInput because onKeyPress won't read hardware keyboard back presses, so if the user has a device with hardware back buttons, like some Android devices have, the onKeyPress won't work, this provides a more consistent experience.
This seems like the best workaround. Still, what I was looking for is more like an option in Android to ignore the back button handler that the keyboard seems to have, so I can be able to control the event myself.
– Francisco Sarmento
Nov 7 at 16:56
That's the thing @FranciscoSarmento, I don't think you can do that in React Native directly, as this is sometimes a problem even in pure Android. When you press back and a keyboard is open, the Input Media Editor (IME for short, also known as the keyboard open) receives that press first before your app, and the only way to surpass the system is to use a listener specific for this, called onKeyPreIme. Once the IME listens to a back press, it closes itself and consumes it. Up to RN v0.57.4, there isn't a way to access this listener.
– Danilo
Nov 7 at 17:50
add a comment |
up vote
1
down vote
I believe that is the correct behavior, but to make what you want, you may detect the keyboard itself hiding instead by using Keyboard (docs at https://facebook.github.io/react-native/docs/keyboard)
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
this.input.blur();
};
//Rest of component...
}
I prefer this method than using the onKeyPress event from TextInput because onKeyPress won't read hardware keyboard back presses, so if the user has a device with hardware back buttons, like some Android devices have, the onKeyPress won't work, this provides a more consistent experience.
This seems like the best workaround. Still, what I was looking for is more like an option in Android to ignore the back button handler that the keyboard seems to have, so I can be able to control the event myself.
– Francisco Sarmento
Nov 7 at 16:56
That's the thing @FranciscoSarmento, I don't think you can do that in React Native directly, as this is sometimes a problem even in pure Android. When you press back and a keyboard is open, the Input Media Editor (IME for short, also known as the keyboard open) receives that press first before your app, and the only way to surpass the system is to use a listener specific for this, called onKeyPreIme. Once the IME listens to a back press, it closes itself and consumes it. Up to RN v0.57.4, there isn't a way to access this listener.
– Danilo
Nov 7 at 17:50
add a comment |
up vote
1
down vote
up vote
1
down vote
I believe that is the correct behavior, but to make what you want, you may detect the keyboard itself hiding instead by using Keyboard (docs at https://facebook.github.io/react-native/docs/keyboard)
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
this.input.blur();
};
//Rest of component...
}
I prefer this method than using the onKeyPress event from TextInput because onKeyPress won't read hardware keyboard back presses, so if the user has a device with hardware back buttons, like some Android devices have, the onKeyPress won't work, this provides a more consistent experience.
I believe that is the correct behavior, but to make what you want, you may detect the keyboard itself hiding instead by using Keyboard (docs at https://facebook.github.io/react-native/docs/keyboard)
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
this.input.blur();
};
//Rest of component...
}
I prefer this method than using the onKeyPress event from TextInput because onKeyPress won't read hardware keyboard back presses, so if the user has a device with hardware back buttons, like some Android devices have, the onKeyPress won't work, this provides a more consistent experience.
edited Nov 7 at 14:30
answered Nov 7 at 14:15
Danilo
1217
1217
This seems like the best workaround. Still, what I was looking for is more like an option in Android to ignore the back button handler that the keyboard seems to have, so I can be able to control the event myself.
– Francisco Sarmento
Nov 7 at 16:56
That's the thing @FranciscoSarmento, I don't think you can do that in React Native directly, as this is sometimes a problem even in pure Android. When you press back and a keyboard is open, the Input Media Editor (IME for short, also known as the keyboard open) receives that press first before your app, and the only way to surpass the system is to use a listener specific for this, called onKeyPreIme. Once the IME listens to a back press, it closes itself and consumes it. Up to RN v0.57.4, there isn't a way to access this listener.
– Danilo
Nov 7 at 17:50
add a comment |
This seems like the best workaround. Still, what I was looking for is more like an option in Android to ignore the back button handler that the keyboard seems to have, so I can be able to control the event myself.
– Francisco Sarmento
Nov 7 at 16:56
That's the thing @FranciscoSarmento, I don't think you can do that in React Native directly, as this is sometimes a problem even in pure Android. When you press back and a keyboard is open, the Input Media Editor (IME for short, also known as the keyboard open) receives that press first before your app, and the only way to surpass the system is to use a listener specific for this, called onKeyPreIme. Once the IME listens to a back press, it closes itself and consumes it. Up to RN v0.57.4, there isn't a way to access this listener.
– Danilo
Nov 7 at 17:50
This seems like the best workaround. Still, what I was looking for is more like an option in Android to ignore the back button handler that the keyboard seems to have, so I can be able to control the event myself.
– Francisco Sarmento
Nov 7 at 16:56
This seems like the best workaround. Still, what I was looking for is more like an option in Android to ignore the back button handler that the keyboard seems to have, so I can be able to control the event myself.
– Francisco Sarmento
Nov 7 at 16:56
That's the thing @FranciscoSarmento, I don't think you can do that in React Native directly, as this is sometimes a problem even in pure Android. When you press back and a keyboard is open, the Input Media Editor (IME for short, also known as the keyboard open) receives that press first before your app, and the only way to surpass the system is to use a listener specific for this, called onKeyPreIme. Once the IME listens to a back press, it closes itself and consumes it. Up to RN v0.57.4, there isn't a way to access this listener.
– Danilo
Nov 7 at 17:50
That's the thing @FranciscoSarmento, I don't think you can do that in React Native directly, as this is sometimes a problem even in pure Android. When you press back and a keyboard is open, the Input Media Editor (IME for short, also known as the keyboard open) receives that press first before your app, and the only way to surpass the system is to use a listener specific for this, called onKeyPreIme. Once the IME listens to a back press, it closes itself and consumes it. Up to RN v0.57.4, there isn't a way to access this listener.
– Danilo
Nov 7 at 17:50
add a comment |
up vote
0
down vote
You'd handle it on the TextInput
itself instead of using a BackHandler
. You can do this via the onKeyPress
prop
constructor(props){
super(props)
this.inputRef = React.createRef()
}
<TextInput
onKeyPress={({ nativeEvent }) => {
if(nativeEvent.key === 'Backspace'){
//your code
// if you want to remove focus then you can use a ref
Keyboard.dismiss();
this.inputRef.blur()
}
}}
ref={this.inputRef}
/>
Also it's important to note that on Android this event will only fire on the software keyboard, so if you're running on an emulator and use the backspace key on your computer's keyboard this will not work.
This will fire on keyboard backspace press and not on Android back button.
– Francisco Sarmento
Nov 7 at 16:47
add a comment |
up vote
0
down vote
You'd handle it on the TextInput
itself instead of using a BackHandler
. You can do this via the onKeyPress
prop
constructor(props){
super(props)
this.inputRef = React.createRef()
}
<TextInput
onKeyPress={({ nativeEvent }) => {
if(nativeEvent.key === 'Backspace'){
//your code
// if you want to remove focus then you can use a ref
Keyboard.dismiss();
this.inputRef.blur()
}
}}
ref={this.inputRef}
/>
Also it's important to note that on Android this event will only fire on the software keyboard, so if you're running on an emulator and use the backspace key on your computer's keyboard this will not work.
This will fire on keyboard backspace press and not on Android back button.
– Francisco Sarmento
Nov 7 at 16:47
add a comment |
up vote
0
down vote
up vote
0
down vote
You'd handle it on the TextInput
itself instead of using a BackHandler
. You can do this via the onKeyPress
prop
constructor(props){
super(props)
this.inputRef = React.createRef()
}
<TextInput
onKeyPress={({ nativeEvent }) => {
if(nativeEvent.key === 'Backspace'){
//your code
// if you want to remove focus then you can use a ref
Keyboard.dismiss();
this.inputRef.blur()
}
}}
ref={this.inputRef}
/>
Also it's important to note that on Android this event will only fire on the software keyboard, so if you're running on an emulator and use the backspace key on your computer's keyboard this will not work.
You'd handle it on the TextInput
itself instead of using a BackHandler
. You can do this via the onKeyPress
prop
constructor(props){
super(props)
this.inputRef = React.createRef()
}
<TextInput
onKeyPress={({ nativeEvent }) => {
if(nativeEvent.key === 'Backspace'){
//your code
// if you want to remove focus then you can use a ref
Keyboard.dismiss();
this.inputRef.blur()
}
}}
ref={this.inputRef}
/>
Also it's important to note that on Android this event will only fire on the software keyboard, so if you're running on an emulator and use the backspace key on your computer's keyboard this will not work.
edited Nov 7 at 14:50


Praveen
813
813
answered Nov 7 at 14:14
Robbie Milejczak
1,9121625
1,9121625
This will fire on keyboard backspace press and not on Android back button.
– Francisco Sarmento
Nov 7 at 16:47
add a comment |
This will fire on keyboard backspace press and not on Android back button.
– Francisco Sarmento
Nov 7 at 16:47
This will fire on keyboard backspace press and not on Android back button.
– Francisco Sarmento
Nov 7 at 16:47
This will fire on keyboard backspace press and not on Android back button.
– Francisco Sarmento
Nov 7 at 16:47
add a comment |
up vote
0
down vote
@Danilo answer does work, but it has to be applied to all text inputs. I ended up using Danilo's solution with a small twist.
Keyboard.dismiss()
does blur any active TextInput, so on keyboardDidHide event I just call Keyboard.dismiss() (although the keyboard just got dismissed by pressing back button).
I just need to add this to my main component.
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
Keyboard.dismiss();
};
//Rest of component...
}
You can teste this solution in this expo snack.
In case your app has multiple TextInputs that onSubmitEditing focus the next TextInput, this is how I made it work:
...
keyboardDidHide = () => {
this.keyboardTimeout = setTimeout(() => Keyboard.dismiss(), 300)
};
keyboardDidShow = () => {
clearTimeout(this.keyboardTimeout)
};
...
add a comment |
up vote
0
down vote
@Danilo answer does work, but it has to be applied to all text inputs. I ended up using Danilo's solution with a small twist.
Keyboard.dismiss()
does blur any active TextInput, so on keyboardDidHide event I just call Keyboard.dismiss() (although the keyboard just got dismissed by pressing back button).
I just need to add this to my main component.
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
Keyboard.dismiss();
};
//Rest of component...
}
You can teste this solution in this expo snack.
In case your app has multiple TextInputs that onSubmitEditing focus the next TextInput, this is how I made it work:
...
keyboardDidHide = () => {
this.keyboardTimeout = setTimeout(() => Keyboard.dismiss(), 300)
};
keyboardDidShow = () => {
clearTimeout(this.keyboardTimeout)
};
...
add a comment |
up vote
0
down vote
up vote
0
down vote
@Danilo answer does work, but it has to be applied to all text inputs. I ended up using Danilo's solution with a small twist.
Keyboard.dismiss()
does blur any active TextInput, so on keyboardDidHide event I just call Keyboard.dismiss() (although the keyboard just got dismissed by pressing back button).
I just need to add this to my main component.
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
Keyboard.dismiss();
};
//Rest of component...
}
You can teste this solution in this expo snack.
In case your app has multiple TextInputs that onSubmitEditing focus the next TextInput, this is how I made it work:
...
keyboardDidHide = () => {
this.keyboardTimeout = setTimeout(() => Keyboard.dismiss(), 300)
};
keyboardDidShow = () => {
clearTimeout(this.keyboardTimeout)
};
...
@Danilo answer does work, but it has to be applied to all text inputs. I ended up using Danilo's solution with a small twist.
Keyboard.dismiss()
does blur any active TextInput, so on keyboardDidHide event I just call Keyboard.dismiss() (although the keyboard just got dismissed by pressing back button).
I just need to add this to my main component.
import * as React from 'react';
import { Keyboard } from 'react-native';
class MyComponent extends React.Component {
componentDidMount() {
this.keyboardDidHideListener = Keyboard.addListener('keyboardDidHide', this.keyboardDidHide);
}
componentWillUnmount() {
this.keyboardDidHideListener.remove();
}
keyboardDidHide = () => {
Keyboard.dismiss();
};
//Rest of component...
}
You can teste this solution in this expo snack.
In case your app has multiple TextInputs that onSubmitEditing focus the next TextInput, this is how I made it work:
...
keyboardDidHide = () => {
this.keyboardTimeout = setTimeout(() => Keyboard.dismiss(), 300)
};
keyboardDidShow = () => {
clearTimeout(this.keyboardTimeout)
};
...
edited Nov 7 at 20:47
answered Nov 7 at 20:34


Francisco Sarmento
587
587
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53190621%2fhow-to-handle-back-button-when-texinput-is-focused%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Uk9itbT1qKSmAueSWT1
No need to add the tags to the title of the question
– perissf
Nov 7 at 16:54
Never add code from external links. Instead, provide a mvce inside the question. Don't add the answer in the question. If the answers provided were not helpful enough, add your own answer.
– perissf
Nov 7 at 19:57
Thanks for your feedback @perissf. I edited the question accordingly. I left the link to expo snack because my intention with the link is not to show the code but to allow anyone to scan the QR code with Expo app and actually see the behavior in its own Android phone.
– Francisco Sarmento
Nov 7 at 20:40