Show elements updated in real-time with Spring
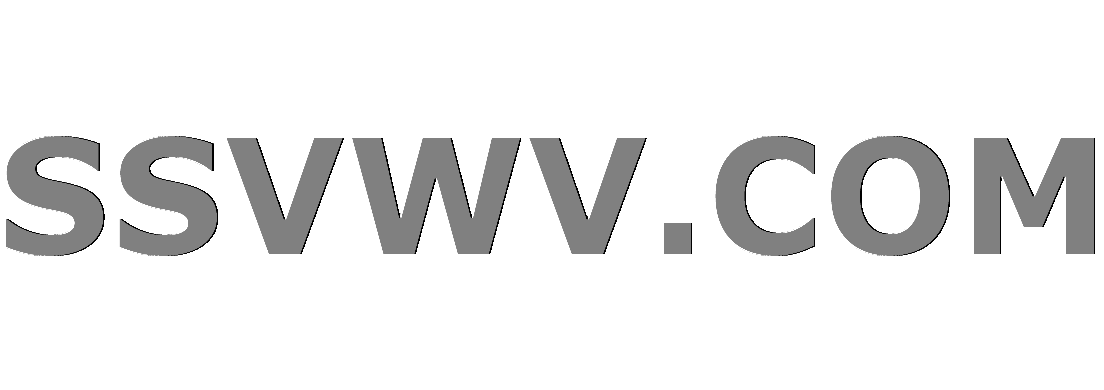
Multi tool use
God afternoon.
I am developing an app which show the places avalaible. In the case of Backend, researched about the SSE (Server-Sent-Event) and TailableCursors with MongoDB. I have done in Spring:
@Repository
public interface Repositorio extends ReactiveMongoRepository<Prueba, String> {
@Tailable
Flux<Prueba> findWithTailableCursorBy();
}
And the controller:
@RestController
@CrossOrigin
@RequestMapping
public class Controlador {
@Autowired
Repositorio repo;
@GetMapping(value = "/prueba", produces = MediaType.TEXT_EVENT_STREAM_VALUE)
public Flux<Prueba> getTodos() {
return repo.findWithTailableCursorBy();
}
@GetMapping("/prueba2")
public Flux<Prueba> prueba() {
return repo.findAll();
}
@PostMapping("/prueba")
public Mono<Prueba> insert(@RequestBody Prueba prueba) {
return repo.save(prueba);
}
}
The items don't change when updated. I thought that with Tailable Cursors I would solve it. However, I see that no...
Is there any way to show the rafters in real time with Spring? So I'm using EventSource on the client-side.
PS: The class model (a capped collection) is:
@Document(collection = "pruebas")
// Lombok's annotations
@Data @NoArgsConstructor @AllArgsConstructor
@EqualsAndHashCode @ToString
public class Prueba {
@Id private String id;
private String nombre;
private boolean activo = false;
}
spring mongodb spring-data-mongodb
add a comment |
God afternoon.
I am developing an app which show the places avalaible. In the case of Backend, researched about the SSE (Server-Sent-Event) and TailableCursors with MongoDB. I have done in Spring:
@Repository
public interface Repositorio extends ReactiveMongoRepository<Prueba, String> {
@Tailable
Flux<Prueba> findWithTailableCursorBy();
}
And the controller:
@RestController
@CrossOrigin
@RequestMapping
public class Controlador {
@Autowired
Repositorio repo;
@GetMapping(value = "/prueba", produces = MediaType.TEXT_EVENT_STREAM_VALUE)
public Flux<Prueba> getTodos() {
return repo.findWithTailableCursorBy();
}
@GetMapping("/prueba2")
public Flux<Prueba> prueba() {
return repo.findAll();
}
@PostMapping("/prueba")
public Mono<Prueba> insert(@RequestBody Prueba prueba) {
return repo.save(prueba);
}
}
The items don't change when updated. I thought that with Tailable Cursors I would solve it. However, I see that no...
Is there any way to show the rafters in real time with Spring? So I'm using EventSource on the client-side.
PS: The class model (a capped collection) is:
@Document(collection = "pruebas")
// Lombok's annotations
@Data @NoArgsConstructor @AllArgsConstructor
@EqualsAndHashCode @ToString
public class Prueba {
@Id private String id;
private String nombre;
private boolean activo = false;
}
spring mongodb spring-data-mongodb
I'm thinking here that "tailable cursors" are not really what you want here. These are actually special things which require "capped collections" in order to work. Modern API and server versions support "change streams" which is probably more like what you are looking for. Note that neither of these "mirrors a collection" if that is what you were expecting.
– Neil Lunn
Nov 13 '18 at 6:37
See also Spring Data MongoDB - Change Streams Example from the spring-mongo repostiory for a typical reactive example.
– Neil Lunn
Nov 13 '18 at 6:39
Oh, I didn't know about this alternative. Thank you.
– Franco Salvador Hernndez Hernn
Nov 15 '18 at 6:04
add a comment |
God afternoon.
I am developing an app which show the places avalaible. In the case of Backend, researched about the SSE (Server-Sent-Event) and TailableCursors with MongoDB. I have done in Spring:
@Repository
public interface Repositorio extends ReactiveMongoRepository<Prueba, String> {
@Tailable
Flux<Prueba> findWithTailableCursorBy();
}
And the controller:
@RestController
@CrossOrigin
@RequestMapping
public class Controlador {
@Autowired
Repositorio repo;
@GetMapping(value = "/prueba", produces = MediaType.TEXT_EVENT_STREAM_VALUE)
public Flux<Prueba> getTodos() {
return repo.findWithTailableCursorBy();
}
@GetMapping("/prueba2")
public Flux<Prueba> prueba() {
return repo.findAll();
}
@PostMapping("/prueba")
public Mono<Prueba> insert(@RequestBody Prueba prueba) {
return repo.save(prueba);
}
}
The items don't change when updated. I thought that with Tailable Cursors I would solve it. However, I see that no...
Is there any way to show the rafters in real time with Spring? So I'm using EventSource on the client-side.
PS: The class model (a capped collection) is:
@Document(collection = "pruebas")
// Lombok's annotations
@Data @NoArgsConstructor @AllArgsConstructor
@EqualsAndHashCode @ToString
public class Prueba {
@Id private String id;
private String nombre;
private boolean activo = false;
}
spring mongodb spring-data-mongodb
God afternoon.
I am developing an app which show the places avalaible. In the case of Backend, researched about the SSE (Server-Sent-Event) and TailableCursors with MongoDB. I have done in Spring:
@Repository
public interface Repositorio extends ReactiveMongoRepository<Prueba, String> {
@Tailable
Flux<Prueba> findWithTailableCursorBy();
}
And the controller:
@RestController
@CrossOrigin
@RequestMapping
public class Controlador {
@Autowired
Repositorio repo;
@GetMapping(value = "/prueba", produces = MediaType.TEXT_EVENT_STREAM_VALUE)
public Flux<Prueba> getTodos() {
return repo.findWithTailableCursorBy();
}
@GetMapping("/prueba2")
public Flux<Prueba> prueba() {
return repo.findAll();
}
@PostMapping("/prueba")
public Mono<Prueba> insert(@RequestBody Prueba prueba) {
return repo.save(prueba);
}
}
The items don't change when updated. I thought that with Tailable Cursors I would solve it. However, I see that no...
Is there any way to show the rafters in real time with Spring? So I'm using EventSource on the client-side.
PS: The class model (a capped collection) is:
@Document(collection = "pruebas")
// Lombok's annotations
@Data @NoArgsConstructor @AllArgsConstructor
@EqualsAndHashCode @ToString
public class Prueba {
@Id private String id;
private String nombre;
private boolean activo = false;
}
spring mongodb spring-data-mongodb
spring mongodb spring-data-mongodb
asked Nov 13 '18 at 6:17


Franco Salvador Hernndez HernnFranco Salvador Hernndez Hernn
1
1
I'm thinking here that "tailable cursors" are not really what you want here. These are actually special things which require "capped collections" in order to work. Modern API and server versions support "change streams" which is probably more like what you are looking for. Note that neither of these "mirrors a collection" if that is what you were expecting.
– Neil Lunn
Nov 13 '18 at 6:37
See also Spring Data MongoDB - Change Streams Example from the spring-mongo repostiory for a typical reactive example.
– Neil Lunn
Nov 13 '18 at 6:39
Oh, I didn't know about this alternative. Thank you.
– Franco Salvador Hernndez Hernn
Nov 15 '18 at 6:04
add a comment |
I'm thinking here that "tailable cursors" are not really what you want here. These are actually special things which require "capped collections" in order to work. Modern API and server versions support "change streams" which is probably more like what you are looking for. Note that neither of these "mirrors a collection" if that is what you were expecting.
– Neil Lunn
Nov 13 '18 at 6:37
See also Spring Data MongoDB - Change Streams Example from the spring-mongo repostiory for a typical reactive example.
– Neil Lunn
Nov 13 '18 at 6:39
Oh, I didn't know about this alternative. Thank you.
– Franco Salvador Hernndez Hernn
Nov 15 '18 at 6:04
I'm thinking here that "tailable cursors" are not really what you want here. These are actually special things which require "capped collections" in order to work. Modern API and server versions support "change streams" which is probably more like what you are looking for. Note that neither of these "mirrors a collection" if that is what you were expecting.
– Neil Lunn
Nov 13 '18 at 6:37
I'm thinking here that "tailable cursors" are not really what you want here. These are actually special things which require "capped collections" in order to work. Modern API and server versions support "change streams" which is probably more like what you are looking for. Note that neither of these "mirrors a collection" if that is what you were expecting.
– Neil Lunn
Nov 13 '18 at 6:37
See also Spring Data MongoDB - Change Streams Example from the spring-mongo repostiory for a typical reactive example.
– Neil Lunn
Nov 13 '18 at 6:39
See also Spring Data MongoDB - Change Streams Example from the spring-mongo repostiory for a typical reactive example.
– Neil Lunn
Nov 13 '18 at 6:39
Oh, I didn't know about this alternative. Thank you.
– Franco Salvador Hernndez Hernn
Nov 15 '18 at 6:04
Oh, I didn't know about this alternative. Thank you.
– Franco Salvador Hernndez Hernn
Nov 15 '18 at 6:04
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53274916%2fshow-elements-updated-in-real-time-with-spring%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53274916%2fshow-elements-updated-in-real-time-with-spring%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
peckKTK 5d9omjH,yeBPDcGG,7iA esKENuTFIo59R6lj,hc wof l5WdQ0HzReX9 DOUB YR 05g3wU05V
I'm thinking here that "tailable cursors" are not really what you want here. These are actually special things which require "capped collections" in order to work. Modern API and server versions support "change streams" which is probably more like what you are looking for. Note that neither of these "mirrors a collection" if that is what you were expecting.
– Neil Lunn
Nov 13 '18 at 6:37
See also Spring Data MongoDB - Change Streams Example from the spring-mongo repostiory for a typical reactive example.
– Neil Lunn
Nov 13 '18 at 6:39
Oh, I didn't know about this alternative. Thank you.
– Franco Salvador Hernndez Hernn
Nov 15 '18 at 6:04