Angular: Errors with passed @Input values to override button text and add class by using ngClass
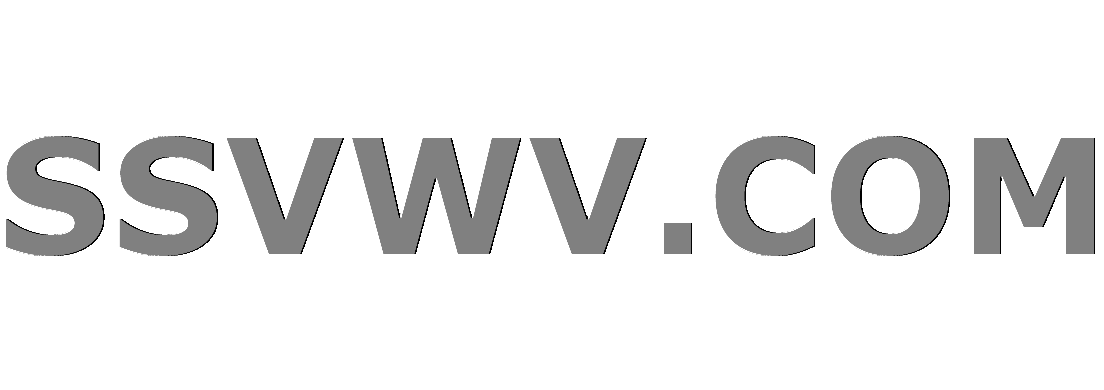
Multi tool use
I've created an angular component
called app-button
.
The template
looks like this:
<div class="app-button">
<button class="app-button__btn">{{text}}</button>
</div>
In the controller I have defined two @Input
variables called modifier
and text
:
export class CanButtonComponent implements OnInit {
@Input() modifier: string;
@Input() text: string = "Default button";
...
}
In the template
of a second component
called sample-page
, I'm reusing my component
app-button
like this:
<app-button></app-button>
At the moment, I got an HTML
button
with my default text
Default button
, so it's working fine.
Now I'm trying to work with my two @Input
variables modifier
and text
.
First of all, I would add a second class, if I pass a value for modifier
:
<app-button [modifier]="test"></app-button>
I tried it in two wayes:
1.) add second class
directly in template
of app-button
with ngClass
condition:
<div class="app-button" [ngClass]="{'app-button--{{modifier}}' modifier != ''}">...</div>
2.) add second class
directly in template
of app-button
with ngClass
calling function
in controller
:
template:
<div class="app-button" [ngClass]="getModifier()">...</div>
controller:
getModifier() {
if (this.modifier) {
return `app-button--${this.modifier}`;
}
}
Both ideas are not working to add this second class
by using the value
of the passed modifier
string
.
Second issue with text
I also have a problem to override the default button
text
also by passing a value for @Input text
:
<app-button [text]="This is a new text">...</app-button>
I've got follow console
error
:
Uncaught Error: Template parse errors:
Parser Error: Unexpected token 'is' at column 6 in [this is a new text]
Hope my two issues are clear.
javascript html angular typescript angular-ng-class
add a comment |
I've created an angular component
called app-button
.
The template
looks like this:
<div class="app-button">
<button class="app-button__btn">{{text}}</button>
</div>
In the controller I have defined two @Input
variables called modifier
and text
:
export class CanButtonComponent implements OnInit {
@Input() modifier: string;
@Input() text: string = "Default button";
...
}
In the template
of a second component
called sample-page
, I'm reusing my component
app-button
like this:
<app-button></app-button>
At the moment, I got an HTML
button
with my default text
Default button
, so it's working fine.
Now I'm trying to work with my two @Input
variables modifier
and text
.
First of all, I would add a second class, if I pass a value for modifier
:
<app-button [modifier]="test"></app-button>
I tried it in two wayes:
1.) add second class
directly in template
of app-button
with ngClass
condition:
<div class="app-button" [ngClass]="{'app-button--{{modifier}}' modifier != ''}">...</div>
2.) add second class
directly in template
of app-button
with ngClass
calling function
in controller
:
template:
<div class="app-button" [ngClass]="getModifier()">...</div>
controller:
getModifier() {
if (this.modifier) {
return `app-button--${this.modifier}`;
}
}
Both ideas are not working to add this second class
by using the value
of the passed modifier
string
.
Second issue with text
I also have a problem to override the default button
text
also by passing a value for @Input text
:
<app-button [text]="This is a new text">...</app-button>
I've got follow console
error
:
Uncaught Error: Template parse errors:
Parser Error: Unexpected token 'is' at column 6 in [this is a new text]
Hope my two issues are clear.
javascript html angular typescript angular-ng-class
add a comment |
I've created an angular component
called app-button
.
The template
looks like this:
<div class="app-button">
<button class="app-button__btn">{{text}}</button>
</div>
In the controller I have defined two @Input
variables called modifier
and text
:
export class CanButtonComponent implements OnInit {
@Input() modifier: string;
@Input() text: string = "Default button";
...
}
In the template
of a second component
called sample-page
, I'm reusing my component
app-button
like this:
<app-button></app-button>
At the moment, I got an HTML
button
with my default text
Default button
, so it's working fine.
Now I'm trying to work with my two @Input
variables modifier
and text
.
First of all, I would add a second class, if I pass a value for modifier
:
<app-button [modifier]="test"></app-button>
I tried it in two wayes:
1.) add second class
directly in template
of app-button
with ngClass
condition:
<div class="app-button" [ngClass]="{'app-button--{{modifier}}' modifier != ''}">...</div>
2.) add second class
directly in template
of app-button
with ngClass
calling function
in controller
:
template:
<div class="app-button" [ngClass]="getModifier()">...</div>
controller:
getModifier() {
if (this.modifier) {
return `app-button--${this.modifier}`;
}
}
Both ideas are not working to add this second class
by using the value
of the passed modifier
string
.
Second issue with text
I also have a problem to override the default button
text
also by passing a value for @Input text
:
<app-button [text]="This is a new text">...</app-button>
I've got follow console
error
:
Uncaught Error: Template parse errors:
Parser Error: Unexpected token 'is' at column 6 in [this is a new text]
Hope my two issues are clear.
javascript html angular typescript angular-ng-class
I've created an angular component
called app-button
.
The template
looks like this:
<div class="app-button">
<button class="app-button__btn">{{text}}</button>
</div>
In the controller I have defined two @Input
variables called modifier
and text
:
export class CanButtonComponent implements OnInit {
@Input() modifier: string;
@Input() text: string = "Default button";
...
}
In the template
of a second component
called sample-page
, I'm reusing my component
app-button
like this:
<app-button></app-button>
At the moment, I got an HTML
button
with my default text
Default button
, so it's working fine.
Now I'm trying to work with my two @Input
variables modifier
and text
.
First of all, I would add a second class, if I pass a value for modifier
:
<app-button [modifier]="test"></app-button>
I tried it in two wayes:
1.) add second class
directly in template
of app-button
with ngClass
condition:
<div class="app-button" [ngClass]="{'app-button--{{modifier}}' modifier != ''}">...</div>
2.) add second class
directly in template
of app-button
with ngClass
calling function
in controller
:
template:
<div class="app-button" [ngClass]="getModifier()">...</div>
controller:
getModifier() {
if (this.modifier) {
return `app-button--${this.modifier}`;
}
}
Both ideas are not working to add this second class
by using the value
of the passed modifier
string
.
Second issue with text
I also have a problem to override the default button
text
also by passing a value for @Input text
:
<app-button [text]="This is a new text">...</app-button>
I've got follow console
error
:
Uncaught Error: Template parse errors:
Parser Error: Unexpected token 'is' at column 6 in [this is a new text]
Hope my two issues are clear.
javascript html angular typescript angular-ng-class
javascript html angular typescript angular-ng-class
asked Nov 19 '18 at 13:16


MrBuggyMrBuggy
1,66211748
1,66211748
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
When you do the following, you are saying that you are passing the "test" property from the parent component to the child:
<app-button [modifier]="test"></app-button>
If you are trying to pass actual test strings, you should do it like this:
<app-button [modifier]="'test'"></app-button>
And:
<app-button [text]="'This is a new text'">...</app-button>
1
Insane, now it works! I thought, if I don't put a property into {{ }}, it would be recognized as a default string, but {{ }} seems to be just for getting the value of a property. Nice to know, thanks mate!
– MrBuggy
Nov 19 '18 at 13:28
You're welcome :)
– Frank Modica
Nov 19 '18 at 13:31
add a comment |
Maybe this
<div class="app-button {{ modifier!== '' ? 'app-button--' + modifier : ''}}">{{text}}</div>
Then
<app-button [text]="'This is a new text'" [modifier]="'test'" >...</app-button>
Hope this will help!
change condition to<div class="app-button {{ modifier !== undefined ? 'app-button--' + modifier : ''}}"
in case ofmodifier !== ' '
. Seems also to be a nice way to solve it
– MrBuggy
Nov 19 '18 at 13:32
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53375481%2fangular-errors-with-passed-input-values-to-override-button-text-and-add-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
When you do the following, you are saying that you are passing the "test" property from the parent component to the child:
<app-button [modifier]="test"></app-button>
If you are trying to pass actual test strings, you should do it like this:
<app-button [modifier]="'test'"></app-button>
And:
<app-button [text]="'This is a new text'">...</app-button>
1
Insane, now it works! I thought, if I don't put a property into {{ }}, it would be recognized as a default string, but {{ }} seems to be just for getting the value of a property. Nice to know, thanks mate!
– MrBuggy
Nov 19 '18 at 13:28
You're welcome :)
– Frank Modica
Nov 19 '18 at 13:31
add a comment |
When you do the following, you are saying that you are passing the "test" property from the parent component to the child:
<app-button [modifier]="test"></app-button>
If you are trying to pass actual test strings, you should do it like this:
<app-button [modifier]="'test'"></app-button>
And:
<app-button [text]="'This is a new text'">...</app-button>
1
Insane, now it works! I thought, if I don't put a property into {{ }}, it would be recognized as a default string, but {{ }} seems to be just for getting the value of a property. Nice to know, thanks mate!
– MrBuggy
Nov 19 '18 at 13:28
You're welcome :)
– Frank Modica
Nov 19 '18 at 13:31
add a comment |
When you do the following, you are saying that you are passing the "test" property from the parent component to the child:
<app-button [modifier]="test"></app-button>
If you are trying to pass actual test strings, you should do it like this:
<app-button [modifier]="'test'"></app-button>
And:
<app-button [text]="'This is a new text'">...</app-button>
When you do the following, you are saying that you are passing the "test" property from the parent component to the child:
<app-button [modifier]="test"></app-button>
If you are trying to pass actual test strings, you should do it like this:
<app-button [modifier]="'test'"></app-button>
And:
<app-button [text]="'This is a new text'">...</app-button>
answered Nov 19 '18 at 13:24


Frank ModicaFrank Modica
6,4442727
6,4442727
1
Insane, now it works! I thought, if I don't put a property into {{ }}, it would be recognized as a default string, but {{ }} seems to be just for getting the value of a property. Nice to know, thanks mate!
– MrBuggy
Nov 19 '18 at 13:28
You're welcome :)
– Frank Modica
Nov 19 '18 at 13:31
add a comment |
1
Insane, now it works! I thought, if I don't put a property into {{ }}, it would be recognized as a default string, but {{ }} seems to be just for getting the value of a property. Nice to know, thanks mate!
– MrBuggy
Nov 19 '18 at 13:28
You're welcome :)
– Frank Modica
Nov 19 '18 at 13:31
1
1
Insane, now it works! I thought, if I don't put a property into {{ }}, it would be recognized as a default string, but {{ }} seems to be just for getting the value of a property. Nice to know, thanks mate!
– MrBuggy
Nov 19 '18 at 13:28
Insane, now it works! I thought, if I don't put a property into {{ }}, it would be recognized as a default string, but {{ }} seems to be just for getting the value of a property. Nice to know, thanks mate!
– MrBuggy
Nov 19 '18 at 13:28
You're welcome :)
– Frank Modica
Nov 19 '18 at 13:31
You're welcome :)
– Frank Modica
Nov 19 '18 at 13:31
add a comment |
Maybe this
<div class="app-button {{ modifier!== '' ? 'app-button--' + modifier : ''}}">{{text}}</div>
Then
<app-button [text]="'This is a new text'" [modifier]="'test'" >...</app-button>
Hope this will help!
change condition to<div class="app-button {{ modifier !== undefined ? 'app-button--' + modifier : ''}}"
in case ofmodifier !== ' '
. Seems also to be a nice way to solve it
– MrBuggy
Nov 19 '18 at 13:32
add a comment |
Maybe this
<div class="app-button {{ modifier!== '' ? 'app-button--' + modifier : ''}}">{{text}}</div>
Then
<app-button [text]="'This is a new text'" [modifier]="'test'" >...</app-button>
Hope this will help!
change condition to<div class="app-button {{ modifier !== undefined ? 'app-button--' + modifier : ''}}"
in case ofmodifier !== ' '
. Seems also to be a nice way to solve it
– MrBuggy
Nov 19 '18 at 13:32
add a comment |
Maybe this
<div class="app-button {{ modifier!== '' ? 'app-button--' + modifier : ''}}">{{text}}</div>
Then
<app-button [text]="'This is a new text'" [modifier]="'test'" >...</app-button>
Hope this will help!
Maybe this
<div class="app-button {{ modifier!== '' ? 'app-button--' + modifier : ''}}">{{text}}</div>
Then
<app-button [text]="'This is a new text'" [modifier]="'test'" >...</app-button>
Hope this will help!
answered Nov 19 '18 at 13:29


freepowderfreepowder
2896
2896
change condition to<div class="app-button {{ modifier !== undefined ? 'app-button--' + modifier : ''}}"
in case ofmodifier !== ' '
. Seems also to be a nice way to solve it
– MrBuggy
Nov 19 '18 at 13:32
add a comment |
change condition to<div class="app-button {{ modifier !== undefined ? 'app-button--' + modifier : ''}}"
in case ofmodifier !== ' '
. Seems also to be a nice way to solve it
– MrBuggy
Nov 19 '18 at 13:32
change condition to
<div class="app-button {{ modifier !== undefined ? 'app-button--' + modifier : ''}}"
in case of modifier !== ' '
. Seems also to be a nice way to solve it– MrBuggy
Nov 19 '18 at 13:32
change condition to
<div class="app-button {{ modifier !== undefined ? 'app-button--' + modifier : ''}}"
in case of modifier !== ' '
. Seems also to be a nice way to solve it– MrBuggy
Nov 19 '18 at 13:32
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53375481%2fangular-errors-with-passed-input-values-to-override-button-text-and-add-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3hxHBZ,GB2ULdhRKtBJrwn6Yg2RFGP3LSfqfId4 W8g4D