Angular HttpClient query parameters not showing in prod build
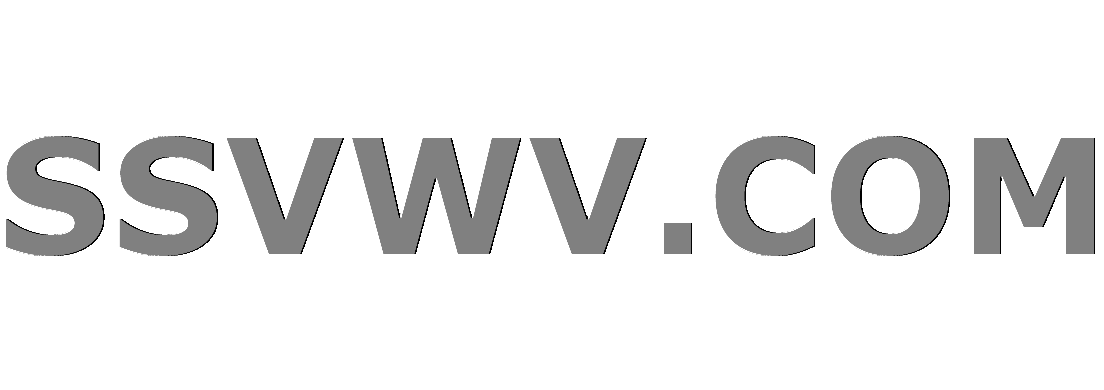
Multi tool use
I have this odd problem where my query parameters of get requests do not work in a prod build, but they do work in a development build.
First I was using the HttpModule, I upgraded to HttpClientModule with hopes the problem might be solved. But unfortunately without success.
When switching to HttpClient I first came across this problem where nested objects were not passed properly. I fixed this by stringifying all nested objects.
Still, the problem remains where no query paramaters are passed when using a prod build.
This is the method that I am using to stringify the data before it is passed to HttpParams:
stringifyObjects() {
for (var prop in this) {
let isObject = typeof this[prop] === 'object' && this[prop] !== null
if(isObject) {
this[prop] = JSON.stringify(this[prop]) as any;
}
}
}
This is the code I am using to pass the params to the request
let params = new HttpParams({
fromObject: data
})
this.http.get(url, {params: params, headers: headers})
Also, I have tried to append every parameter separately:
let httpParams = new HttpParams();
Object.keys(data).forEach(function (key) {
httpParams = httpParams.append(key, data[key]);
});
return this.http.get(url, {params: httpParams, headers: headers})
All with the same result.
Anyone else who came across this issue, or anyone who knows what might be causing this?
Thanks in advance.
EDIT
I added this line to check where the problem starts.
console.log('params.toString()', params.toString());
Found out the this params.toString() returns an empty string (while with a development build, this returns the string correctly). My approach with this was to manually add the string to the url instead of passing is as params.
Still, I have not found a way to fix this or work around this.
angular http angular-http angular-httpclient
add a comment |
I have this odd problem where my query parameters of get requests do not work in a prod build, but they do work in a development build.
First I was using the HttpModule, I upgraded to HttpClientModule with hopes the problem might be solved. But unfortunately without success.
When switching to HttpClient I first came across this problem where nested objects were not passed properly. I fixed this by stringifying all nested objects.
Still, the problem remains where no query paramaters are passed when using a prod build.
This is the method that I am using to stringify the data before it is passed to HttpParams:
stringifyObjects() {
for (var prop in this) {
let isObject = typeof this[prop] === 'object' && this[prop] !== null
if(isObject) {
this[prop] = JSON.stringify(this[prop]) as any;
}
}
}
This is the code I am using to pass the params to the request
let params = new HttpParams({
fromObject: data
})
this.http.get(url, {params: params, headers: headers})
Also, I have tried to append every parameter separately:
let httpParams = new HttpParams();
Object.keys(data).forEach(function (key) {
httpParams = httpParams.append(key, data[key]);
});
return this.http.get(url, {params: httpParams, headers: headers})
All with the same result.
Anyone else who came across this issue, or anyone who knows what might be causing this?
Thanks in advance.
EDIT
I added this line to check where the problem starts.
console.log('params.toString()', params.toString());
Found out the this params.toString() returns an empty string (while with a development build, this returns the string correctly). My approach with this was to manually add the string to the url instead of passing is as params.
Still, I have not found a way to fix this or work around this.
angular http angular-http angular-httpclient
Params and Query params are different for angular. I am unsure what the issue is and if setting is the issue. Alternatively can you check with for...in loop and see if scope resolution is the issue. It's unlikely though. I have face similar issue with append though. Can you check this this might help - stackoverflow.com/questions/45470575/…
– Gary
Nov 18 '18 at 6:01
the best tool for buildingHttpClient.get()
query params is string template:.get(`${url}?param1=${param1}&...`)
, using backward quotes
– WildDev
Nov 18 '18 at 10:43
add a comment |
I have this odd problem where my query parameters of get requests do not work in a prod build, but they do work in a development build.
First I was using the HttpModule, I upgraded to HttpClientModule with hopes the problem might be solved. But unfortunately without success.
When switching to HttpClient I first came across this problem where nested objects were not passed properly. I fixed this by stringifying all nested objects.
Still, the problem remains where no query paramaters are passed when using a prod build.
This is the method that I am using to stringify the data before it is passed to HttpParams:
stringifyObjects() {
for (var prop in this) {
let isObject = typeof this[prop] === 'object' && this[prop] !== null
if(isObject) {
this[prop] = JSON.stringify(this[prop]) as any;
}
}
}
This is the code I am using to pass the params to the request
let params = new HttpParams({
fromObject: data
})
this.http.get(url, {params: params, headers: headers})
Also, I have tried to append every parameter separately:
let httpParams = new HttpParams();
Object.keys(data).forEach(function (key) {
httpParams = httpParams.append(key, data[key]);
});
return this.http.get(url, {params: httpParams, headers: headers})
All with the same result.
Anyone else who came across this issue, or anyone who knows what might be causing this?
Thanks in advance.
EDIT
I added this line to check where the problem starts.
console.log('params.toString()', params.toString());
Found out the this params.toString() returns an empty string (while with a development build, this returns the string correctly). My approach with this was to manually add the string to the url instead of passing is as params.
Still, I have not found a way to fix this or work around this.
angular http angular-http angular-httpclient
I have this odd problem where my query parameters of get requests do not work in a prod build, but they do work in a development build.
First I was using the HttpModule, I upgraded to HttpClientModule with hopes the problem might be solved. But unfortunately without success.
When switching to HttpClient I first came across this problem where nested objects were not passed properly. I fixed this by stringifying all nested objects.
Still, the problem remains where no query paramaters are passed when using a prod build.
This is the method that I am using to stringify the data before it is passed to HttpParams:
stringifyObjects() {
for (var prop in this) {
let isObject = typeof this[prop] === 'object' && this[prop] !== null
if(isObject) {
this[prop] = JSON.stringify(this[prop]) as any;
}
}
}
This is the code I am using to pass the params to the request
let params = new HttpParams({
fromObject: data
})
this.http.get(url, {params: params, headers: headers})
Also, I have tried to append every parameter separately:
let httpParams = new HttpParams();
Object.keys(data).forEach(function (key) {
httpParams = httpParams.append(key, data[key]);
});
return this.http.get(url, {params: httpParams, headers: headers})
All with the same result.
Anyone else who came across this issue, or anyone who knows what might be causing this?
Thanks in advance.
EDIT
I added this line to check where the problem starts.
console.log('params.toString()', params.toString());
Found out the this params.toString() returns an empty string (while with a development build, this returns the string correctly). My approach with this was to manually add the string to the url instead of passing is as params.
Still, I have not found a way to fix this or work around this.
angular http angular-http angular-httpclient
angular http angular-http angular-httpclient
edited Nov 18 '18 at 14:41
TheBurgerShot
asked Nov 18 '18 at 5:22


TheBurgerShotTheBurgerShot
6641715
6641715
Params and Query params are different for angular. I am unsure what the issue is and if setting is the issue. Alternatively can you check with for...in loop and see if scope resolution is the issue. It's unlikely though. I have face similar issue with append though. Can you check this this might help - stackoverflow.com/questions/45470575/…
– Gary
Nov 18 '18 at 6:01
the best tool for buildingHttpClient.get()
query params is string template:.get(`${url}?param1=${param1}&...`)
, using backward quotes
– WildDev
Nov 18 '18 at 10:43
add a comment |
Params and Query params are different for angular. I am unsure what the issue is and if setting is the issue. Alternatively can you check with for...in loop and see if scope resolution is the issue. It's unlikely though. I have face similar issue with append though. Can you check this this might help - stackoverflow.com/questions/45470575/…
– Gary
Nov 18 '18 at 6:01
the best tool for buildingHttpClient.get()
query params is string template:.get(`${url}?param1=${param1}&...`)
, using backward quotes
– WildDev
Nov 18 '18 at 10:43
Params and Query params are different for angular. I am unsure what the issue is and if setting is the issue. Alternatively can you check with for...in loop and see if scope resolution is the issue. It's unlikely though. I have face similar issue with append though. Can you check this this might help - stackoverflow.com/questions/45470575/…
– Gary
Nov 18 '18 at 6:01
Params and Query params are different for angular. I am unsure what the issue is and if setting is the issue. Alternatively can you check with for...in loop and see if scope resolution is the issue. It's unlikely though. I have face similar issue with append though. Can you check this this might help - stackoverflow.com/questions/45470575/…
– Gary
Nov 18 '18 at 6:01
the best tool for building
HttpClient.get()
query params is string template: .get(`${url}?param1=${param1}&...`)
, using backward quotes– WildDev
Nov 18 '18 at 10:43
the best tool for building
HttpClient.get()
query params is string template: .get(`${url}?param1=${param1}&...`)
, using backward quotes– WildDev
Nov 18 '18 at 10:43
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53358144%2fangular-httpclient-query-parameters-not-showing-in-prod-build%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53358144%2fangular-httpclient-query-parameters-not-showing-in-prod-build%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NHlPjvTexT1Or W3NNxkd6pug38
Params and Query params are different for angular. I am unsure what the issue is and if setting is the issue. Alternatively can you check with for...in loop and see if scope resolution is the issue. It's unlikely though. I have face similar issue with append though. Can you check this this might help - stackoverflow.com/questions/45470575/…
– Gary
Nov 18 '18 at 6:01
the best tool for building
HttpClient.get()
query params is string template:.get(`${url}?param1=${param1}&...`)
, using backward quotes– WildDev
Nov 18 '18 at 10:43