return the first non repeating character in a string in javascript
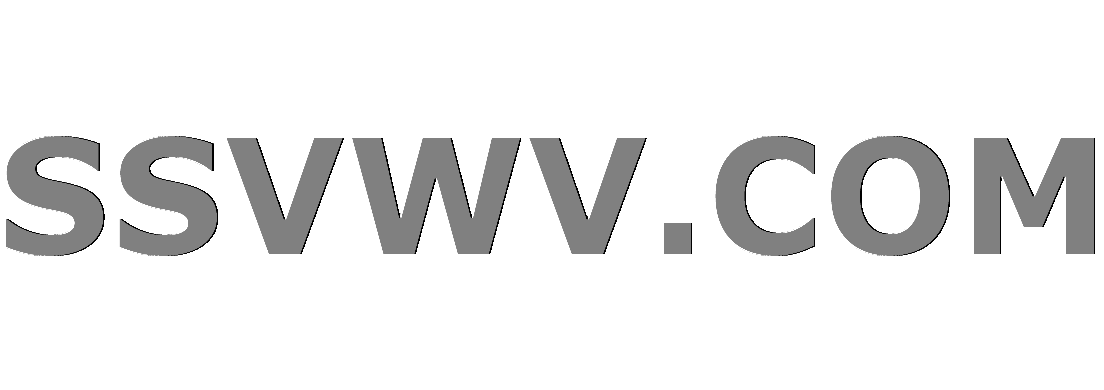
Multi tool use
So I tried looking for this in the search but the closest I could come is a similar answer in several different languages, I would like to use Javascript to do it.
The problem is I have an arbitrary string that I would like to return the first non repeating character. EX: 'aba' -> would return b
'aabcbd' -> would return c.
This is what I have so far, just a simple for loop to start.
var someString = 'aabcbd';
var firstNonRepeatedCharacter = function(string) {
for(var i = 0; i < someString.length; i++){
}
};
http://jsfiddle.net/w7F87/
Not sure where to go from here
javascript
|
show 8 more comments
So I tried looking for this in the search but the closest I could come is a similar answer in several different languages, I would like to use Javascript to do it.
The problem is I have an arbitrary string that I would like to return the first non repeating character. EX: 'aba' -> would return b
'aabcbd' -> would return c.
This is what I have so far, just a simple for loop to start.
var someString = 'aabcbd';
var firstNonRepeatedCharacter = function(string) {
for(var i = 0; i < someString.length; i++){
}
};
http://jsfiddle.net/w7F87/
Not sure where to go from here
javascript
The index of a non-repeating character will be odd and it will be different than the character before it. That should be enough to get you through.
– tvanfosson
Jul 17 '14 at 0:33
I don't understand, how would the index be odd? In my example c is in the third index but what if it was aabbcd instead which means it would be in the 4th index.?
– TaylorAllred
Jul 17 '14 at 0:37
He thinks it has to repeat the character before it
– dave
Jul 17 '14 at 0:38
Are you familiar with map objects in JavaScript? Such as:var charMap = {}; charMap['a'] = 0; charMap['b'] = 1;
? You could iterate through the input string, adding every char into a charMap: jsfiddle.net/w7F87/14
– acdcjunior
Jul 17 '14 at 0:41
@user3806863 - using the logic you outlined, in the sample in your comment the non-repeat would be 'd', the 6th letter at index 5.
– tvanfosson
Jul 17 '14 at 0:47
|
show 8 more comments
So I tried looking for this in the search but the closest I could come is a similar answer in several different languages, I would like to use Javascript to do it.
The problem is I have an arbitrary string that I would like to return the first non repeating character. EX: 'aba' -> would return b
'aabcbd' -> would return c.
This is what I have so far, just a simple for loop to start.
var someString = 'aabcbd';
var firstNonRepeatedCharacter = function(string) {
for(var i = 0; i < someString.length; i++){
}
};
http://jsfiddle.net/w7F87/
Not sure where to go from here
javascript
So I tried looking for this in the search but the closest I could come is a similar answer in several different languages, I would like to use Javascript to do it.
The problem is I have an arbitrary string that I would like to return the first non repeating character. EX: 'aba' -> would return b
'aabcbd' -> would return c.
This is what I have so far, just a simple for loop to start.
var someString = 'aabcbd';
var firstNonRepeatedCharacter = function(string) {
for(var i = 0; i < someString.length; i++){
}
};
http://jsfiddle.net/w7F87/
Not sure where to go from here
javascript
javascript
asked Jul 17 '14 at 0:29
TaylorAllredTaylorAllred
3862417
3862417
The index of a non-repeating character will be odd and it will be different than the character before it. That should be enough to get you through.
– tvanfosson
Jul 17 '14 at 0:33
I don't understand, how would the index be odd? In my example c is in the third index but what if it was aabbcd instead which means it would be in the 4th index.?
– TaylorAllred
Jul 17 '14 at 0:37
He thinks it has to repeat the character before it
– dave
Jul 17 '14 at 0:38
Are you familiar with map objects in JavaScript? Such as:var charMap = {}; charMap['a'] = 0; charMap['b'] = 1;
? You could iterate through the input string, adding every char into a charMap: jsfiddle.net/w7F87/14
– acdcjunior
Jul 17 '14 at 0:41
@user3806863 - using the logic you outlined, in the sample in your comment the non-repeat would be 'd', the 6th letter at index 5.
– tvanfosson
Jul 17 '14 at 0:47
|
show 8 more comments
The index of a non-repeating character will be odd and it will be different than the character before it. That should be enough to get you through.
– tvanfosson
Jul 17 '14 at 0:33
I don't understand, how would the index be odd? In my example c is in the third index but what if it was aabbcd instead which means it would be in the 4th index.?
– TaylorAllred
Jul 17 '14 at 0:37
He thinks it has to repeat the character before it
– dave
Jul 17 '14 at 0:38
Are you familiar with map objects in JavaScript? Such as:var charMap = {}; charMap['a'] = 0; charMap['b'] = 1;
? You could iterate through the input string, adding every char into a charMap: jsfiddle.net/w7F87/14
– acdcjunior
Jul 17 '14 at 0:41
@user3806863 - using the logic you outlined, in the sample in your comment the non-repeat would be 'd', the 6th letter at index 5.
– tvanfosson
Jul 17 '14 at 0:47
The index of a non-repeating character will be odd and it will be different than the character before it. That should be enough to get you through.
– tvanfosson
Jul 17 '14 at 0:33
The index of a non-repeating character will be odd and it will be different than the character before it. That should be enough to get you through.
– tvanfosson
Jul 17 '14 at 0:33
I don't understand, how would the index be odd? In my example c is in the third index but what if it was aabbcd instead which means it would be in the 4th index.?
– TaylorAllred
Jul 17 '14 at 0:37
I don't understand, how would the index be odd? In my example c is in the third index but what if it was aabbcd instead which means it would be in the 4th index.?
– TaylorAllred
Jul 17 '14 at 0:37
He thinks it has to repeat the character before it
– dave
Jul 17 '14 at 0:38
He thinks it has to repeat the character before it
– dave
Jul 17 '14 at 0:38
Are you familiar with map objects in JavaScript? Such as:
var charMap = {}; charMap['a'] = 0; charMap['b'] = 1;
? You could iterate through the input string, adding every char into a charMap: jsfiddle.net/w7F87/14– acdcjunior
Jul 17 '14 at 0:41
Are you familiar with map objects in JavaScript? Such as:
var charMap = {}; charMap['a'] = 0; charMap['b'] = 1;
? You could iterate through the input string, adding every char into a charMap: jsfiddle.net/w7F87/14– acdcjunior
Jul 17 '14 at 0:41
@user3806863 - using the logic you outlined, in the sample in your comment the non-repeat would be 'd', the 6th letter at index 5.
– tvanfosson
Jul 17 '14 at 0:47
@user3806863 - using the logic you outlined, in the sample in your comment the non-repeat would be 'd', the 6th letter at index 5.
– tvanfosson
Jul 17 '14 at 0:47
|
show 8 more comments
12 Answers
12
active
oldest
votes
You can use the indexOf
method to find the non repeating character. If you look for the character in the string, it will be the first one found, and you won't find another after it:
function firstNonRepeatedCharacter(string) {
for (var i = 0; i < string.length; i++) {
var c = string.charAt(i);
if (string.indexOf(c) == i && string.indexOf(c, i + 1) == -1) {
return c;
}
}
return null;
}
Demo: http://jsfiddle.net/Guffa/Se4dD/
Both you and dave used the .charAt method, I am unfamiliar with it.
– TaylorAllred
Jul 17 '14 at 0:45
1
@user3806863: It gets the character at a specific index,string.charAt(i)
does the same asstring[i]
, but it works in all browsers.
– Guffa
Jul 17 '14 at 0:49
add a comment |
If you're looking for the first occurrence of a letter that only occurs once, I would use another data structure to keep track of how many times each letter has been seen. This would let you do it with an O(n) rather than an O(n2) solution, except that n in this case is the larger of the difference between the smallest and largest character code or the length of the string and so not directly comparable.
Note: an earlier version of this used for-in
- which in practice turns out to be incredibly slow. I've updated it to use the character codes as indexes to keep the look up as fast as possible. What we really need is a hash table but given the small values of N and the small, relative speed up, it's probably not worth it for this problem. In fact, you should prefer @Guffa's solution. I'm including mine only because I ended up learning a lot from it.
function firstNonRepeatedCharacter(string) {
var counts = {};
var i, minCode = 999999, maxCode = -1;
for (i = 0; i < string.length; ++i) {
var letter = string.charAt(i);
var letterCode = string.charCodeAt(i);
if (letterCode < minCode) {
minCode = letterCode;
}
if (letterCode > maxCode) {
maxCode = letterCode;
}
var count = counts[letterCode];
if (count) {
count.count = count.count + 1;
}
else {
counts[letterCode] = { letter: letter, count: 1, index: i };
}
}
var smallestIndex = string.length;
for (i = minCode; i <= maxCode; ++i) {
var count = counts[i];
if (count && count.count === 1 && count.index < smallestIndex) {
smallestIndex = count.index;
}
}
return smallestIndex < string.length ? string.charAt(smallestIndex) : '';
}
See fiddle at http://jsfiddle.net/b2dE4/
Also a (slightly different than the comments) performance test at http://jsperf.com/24793051/2
jsperf.com/24793051
– Xotic750
Jul 17 '14 at 1:10
@Xotic750 - that's very interesting. I guess I was assuming that the object index would be an O(1) operation, but it's obviously not. I wonder if converting it to an integer would improve that.
– tvanfosson
Jul 17 '14 at 1:19
Usingcounts = {}
may give a small improvement. It's worth noting how poor Opera is, but your code gets a big boost with that browser.
– Xotic750
Jul 17 '14 at 1:27
1
@Xotic750 - I've got an updated algorithm which runs significantly faster, though probably not on the small string test case. I had always assumed thatfor-in
and the property index operations would be O(1) but apparently they're not. Good learning opportunity.
– tvanfosson
Jul 17 '14 at 2:08
add a comment |
var firstNonRepeatedCharacter = function(string) {
var chars = string.split('');
for (var i = 0; i < string.length; i++) {
if (chars.filter(function(j) {
return j == string.charAt(i);
}).length == 1) return string.charAt(i);
}
};
So we create an array of all the characters, by splitting on anything.
Then, we loop through each character, and we filter the array we created, so we'll get an array of only those characters. If the length is ever 1, we know we have a non-repeated character.
Fiddle: http://jsfiddle.net/2FpZF/
add a comment |
First of all, start your loop at 1, not 0. There is no point in checking the first character to see if its repeating, obviously it can't be.
Now, within your loop, you have someString[i] and someString[i - 1]. They are the current and previous characters.
if someString[i] === someString[i - 1] then the characters are repeating, if someString[i] !== someString[i - 1] then they are not repeating, so you return someString[i]
I won't write the whole thing out for you, but hopefully the thought process behind this will help
That only checks if it repeats the character before it. His example shows it can repeat anywhere -abcdea
has a repeatinga
– dave
Jul 17 '14 at 0:41
Thank you for understanding what I was asking Dave. Thank you for answering anyway Martin
– TaylorAllred
Jul 17 '14 at 0:43
add a comment |
function FirstNotRepeatedChar(str) {
var arr = str.split('');
var result = '';
var ctr = 0;
for (var x = 0; x < arr.length; x++) {
ctr = 0;
for (var y = 0; y < arr.length; y++) {
if (arr[x] === arr[y]) {
ctr+= 1;
}
}
if (ctr < 2) {
result = arr[x];
break;
}
}
return result;
}
console.log(FirstNotRepeatedChar('asif shaik'));
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Subramanyam
Apr 18 '18 at 9:11
add a comment |
Two further possibilities, using ECMA5 array methods. Will return undefined
if none exist.
Javascript
function firstNonRepeatedCharacter(string) {
return string.split('').filter(function (character, index, obj) {
return obj.indexOf(character) === obj.lastIndexOf(character);
}).shift();
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
Or if you want a bit better performance, especially on longer strings.
Javascript
function firstNonRepeatedCharacter(string) {
var first;
string.split('').some(function (character, index, obj) {
if(obj.indexOf(character) === obj.lastIndexOf(character)) {
first = character;
return true;
}
return false;
});
return first;
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
add a comment |
Fill an empty array with zeros, with same length as the string array, and tally up how many times they appear through the loop. Grab the first one in the tallied array with a value of 1.
function firstNotRepeatingCharacter(s) {
const array = s.split("");
let scores = new Array(array.length).fill(0);
for (let char of array) {
scores[array.indexOf(char)]++;
}
const singleChar = array[scores.indexOf(1)];
return singleChar ? singleChar : "_"
}
add a comment |
Here's an O(n) solution with 2 ES6 Sets, one tracking all characters that have appeared and one tracking only chars that have appeared once. This solution takes advantage of the insertion order preserved by Set.
const firstNonRepeating = str => {
const set = new Set();
const finalSet = new Set();
str.split('').forEach(char => {
if (set.has(char)) finalSet.delete(char);
else {
set.add(char);
finalSet.add(char);
}
})
const iter = finalSet.values();
return iter.next().value;
}
add a comment |
I came accross this while facing similar problem. Let me add my 2 lines.
What I did is a similar to the Guffa's answer. But using both indexOf
method and lastIndexOf
.
My mehod:
function nonRepeated(str) {
for(let i = 0; i < str.length; i++) {
let j = str.charAt(i)
if (str.indexOf(j) == str.lastIndexOf(j)) {
return j;
}
}
return null;
}
nonRepeated("aabcbd"); //c
Simply, indexOf()
gets first occurrence of a character & lastIndexOf()
gets the last occurrence. So when the first occurrence is also == the last occurence, it means there's just one the character.
add a comment |
You can iterate through each character to find()
the first letter that returns a single match()
. This will result in the first non-repeated character in the given string:
const first_nonrepeated_character = string => [...string].find(e => string.match(new RegExp(e, 'g')).length === 1);
const string = 'aabcbd';
console.log(first_nonrepeated_character(string)); // c
add a comment |
let arr = [10, 5, 3, 4, 3, 5, 6];
outer:for(let i=0;i<arr.length;i++){
for(let j=0;j<arr.length;j++){
if(arr[i]===arr[j+1]){
console.log(arr[i]);
break outer;
}
}
}
//or else you may try this way...
function firstDuplicate(arr) {
let findFirst = new Set()
for (element of arr)
if (findFirst.has(element ))
return element
else
findFirst.add(element )
}
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Brevity is acceptable, but fuller explanations are better.
– Armali
yesterday
add a comment |
solution: map characters into an object
To begin, an empty object is initialized to be a count
. Each char
of the count
is assigned as each letter from the input string.
If the letter
doesn't already exist in the count
, then the letter
is assigned a value of 1
. Otherwise, if the letter
already exists, increase the count of its occurrence by 1
.
After looping through each letter in the input string and assigning each letter to a key-value pair in the count
, the next step is to loop through each property (key-value pair) in the count
.
While looping through the properties in the count
, the first property equal to 1
is the first non-repeating character in the input string.
The expected and actual output from invoking firstNonRepeatedCharacter(someString)
is c
.
var firstNonRepeatedCharacter = function(string) {
var count = {};
for (var i = 0; i < string.length; i++) {
var letter = string[i];
if (!count[letter]) {
count[letter] = 1;
} else {
count[letter]++;
}
}
for (var letter in count) {
if (count[letter] === 1) {
return letter;
}
}
return null;
}
var someString = 'aabcbd';
console.log(firstNonRepeatedCharacter(someString));
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f24793051%2freturn-the-first-non-repeating-character-in-a-string-in-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
12 Answers
12
active
oldest
votes
12 Answers
12
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use the indexOf
method to find the non repeating character. If you look for the character in the string, it will be the first one found, and you won't find another after it:
function firstNonRepeatedCharacter(string) {
for (var i = 0; i < string.length; i++) {
var c = string.charAt(i);
if (string.indexOf(c) == i && string.indexOf(c, i + 1) == -1) {
return c;
}
}
return null;
}
Demo: http://jsfiddle.net/Guffa/Se4dD/
Both you and dave used the .charAt method, I am unfamiliar with it.
– TaylorAllred
Jul 17 '14 at 0:45
1
@user3806863: It gets the character at a specific index,string.charAt(i)
does the same asstring[i]
, but it works in all browsers.
– Guffa
Jul 17 '14 at 0:49
add a comment |
You can use the indexOf
method to find the non repeating character. If you look for the character in the string, it will be the first one found, and you won't find another after it:
function firstNonRepeatedCharacter(string) {
for (var i = 0; i < string.length; i++) {
var c = string.charAt(i);
if (string.indexOf(c) == i && string.indexOf(c, i + 1) == -1) {
return c;
}
}
return null;
}
Demo: http://jsfiddle.net/Guffa/Se4dD/
Both you and dave used the .charAt method, I am unfamiliar with it.
– TaylorAllred
Jul 17 '14 at 0:45
1
@user3806863: It gets the character at a specific index,string.charAt(i)
does the same asstring[i]
, but it works in all browsers.
– Guffa
Jul 17 '14 at 0:49
add a comment |
You can use the indexOf
method to find the non repeating character. If you look for the character in the string, it will be the first one found, and you won't find another after it:
function firstNonRepeatedCharacter(string) {
for (var i = 0; i < string.length; i++) {
var c = string.charAt(i);
if (string.indexOf(c) == i && string.indexOf(c, i + 1) == -1) {
return c;
}
}
return null;
}
Demo: http://jsfiddle.net/Guffa/Se4dD/
You can use the indexOf
method to find the non repeating character. If you look for the character in the string, it will be the first one found, and you won't find another after it:
function firstNonRepeatedCharacter(string) {
for (var i = 0; i < string.length; i++) {
var c = string.charAt(i);
if (string.indexOf(c) == i && string.indexOf(c, i + 1) == -1) {
return c;
}
}
return null;
}
Demo: http://jsfiddle.net/Guffa/Se4dD/
answered Jul 17 '14 at 0:38
GuffaGuffa
556k77563880
556k77563880
Both you and dave used the .charAt method, I am unfamiliar with it.
– TaylorAllred
Jul 17 '14 at 0:45
1
@user3806863: It gets the character at a specific index,string.charAt(i)
does the same asstring[i]
, but it works in all browsers.
– Guffa
Jul 17 '14 at 0:49
add a comment |
Both you and dave used the .charAt method, I am unfamiliar with it.
– TaylorAllred
Jul 17 '14 at 0:45
1
@user3806863: It gets the character at a specific index,string.charAt(i)
does the same asstring[i]
, but it works in all browsers.
– Guffa
Jul 17 '14 at 0:49
Both you and dave used the .charAt method, I am unfamiliar with it.
– TaylorAllred
Jul 17 '14 at 0:45
Both you and dave used the .charAt method, I am unfamiliar with it.
– TaylorAllred
Jul 17 '14 at 0:45
1
1
@user3806863: It gets the character at a specific index,
string.charAt(i)
does the same as string[i]
, but it works in all browsers.– Guffa
Jul 17 '14 at 0:49
@user3806863: It gets the character at a specific index,
string.charAt(i)
does the same as string[i]
, but it works in all browsers.– Guffa
Jul 17 '14 at 0:49
add a comment |
If you're looking for the first occurrence of a letter that only occurs once, I would use another data structure to keep track of how many times each letter has been seen. This would let you do it with an O(n) rather than an O(n2) solution, except that n in this case is the larger of the difference between the smallest and largest character code or the length of the string and so not directly comparable.
Note: an earlier version of this used for-in
- which in practice turns out to be incredibly slow. I've updated it to use the character codes as indexes to keep the look up as fast as possible. What we really need is a hash table but given the small values of N and the small, relative speed up, it's probably not worth it for this problem. In fact, you should prefer @Guffa's solution. I'm including mine only because I ended up learning a lot from it.
function firstNonRepeatedCharacter(string) {
var counts = {};
var i, minCode = 999999, maxCode = -1;
for (i = 0; i < string.length; ++i) {
var letter = string.charAt(i);
var letterCode = string.charCodeAt(i);
if (letterCode < minCode) {
minCode = letterCode;
}
if (letterCode > maxCode) {
maxCode = letterCode;
}
var count = counts[letterCode];
if (count) {
count.count = count.count + 1;
}
else {
counts[letterCode] = { letter: letter, count: 1, index: i };
}
}
var smallestIndex = string.length;
for (i = minCode; i <= maxCode; ++i) {
var count = counts[i];
if (count && count.count === 1 && count.index < smallestIndex) {
smallestIndex = count.index;
}
}
return smallestIndex < string.length ? string.charAt(smallestIndex) : '';
}
See fiddle at http://jsfiddle.net/b2dE4/
Also a (slightly different than the comments) performance test at http://jsperf.com/24793051/2
jsperf.com/24793051
– Xotic750
Jul 17 '14 at 1:10
@Xotic750 - that's very interesting. I guess I was assuming that the object index would be an O(1) operation, but it's obviously not. I wonder if converting it to an integer would improve that.
– tvanfosson
Jul 17 '14 at 1:19
Usingcounts = {}
may give a small improvement. It's worth noting how poor Opera is, but your code gets a big boost with that browser.
– Xotic750
Jul 17 '14 at 1:27
1
@Xotic750 - I've got an updated algorithm which runs significantly faster, though probably not on the small string test case. I had always assumed thatfor-in
and the property index operations would be O(1) but apparently they're not. Good learning opportunity.
– tvanfosson
Jul 17 '14 at 2:08
add a comment |
If you're looking for the first occurrence of a letter that only occurs once, I would use another data structure to keep track of how many times each letter has been seen. This would let you do it with an O(n) rather than an O(n2) solution, except that n in this case is the larger of the difference between the smallest and largest character code or the length of the string and so not directly comparable.
Note: an earlier version of this used for-in
- which in practice turns out to be incredibly slow. I've updated it to use the character codes as indexes to keep the look up as fast as possible. What we really need is a hash table but given the small values of N and the small, relative speed up, it's probably not worth it for this problem. In fact, you should prefer @Guffa's solution. I'm including mine only because I ended up learning a lot from it.
function firstNonRepeatedCharacter(string) {
var counts = {};
var i, minCode = 999999, maxCode = -1;
for (i = 0; i < string.length; ++i) {
var letter = string.charAt(i);
var letterCode = string.charCodeAt(i);
if (letterCode < minCode) {
minCode = letterCode;
}
if (letterCode > maxCode) {
maxCode = letterCode;
}
var count = counts[letterCode];
if (count) {
count.count = count.count + 1;
}
else {
counts[letterCode] = { letter: letter, count: 1, index: i };
}
}
var smallestIndex = string.length;
for (i = minCode; i <= maxCode; ++i) {
var count = counts[i];
if (count && count.count === 1 && count.index < smallestIndex) {
smallestIndex = count.index;
}
}
return smallestIndex < string.length ? string.charAt(smallestIndex) : '';
}
See fiddle at http://jsfiddle.net/b2dE4/
Also a (slightly different than the comments) performance test at http://jsperf.com/24793051/2
jsperf.com/24793051
– Xotic750
Jul 17 '14 at 1:10
@Xotic750 - that's very interesting. I guess I was assuming that the object index would be an O(1) operation, but it's obviously not. I wonder if converting it to an integer would improve that.
– tvanfosson
Jul 17 '14 at 1:19
Usingcounts = {}
may give a small improvement. It's worth noting how poor Opera is, but your code gets a big boost with that browser.
– Xotic750
Jul 17 '14 at 1:27
1
@Xotic750 - I've got an updated algorithm which runs significantly faster, though probably not on the small string test case. I had always assumed thatfor-in
and the property index operations would be O(1) but apparently they're not. Good learning opportunity.
– tvanfosson
Jul 17 '14 at 2:08
add a comment |
If you're looking for the first occurrence of a letter that only occurs once, I would use another data structure to keep track of how many times each letter has been seen. This would let you do it with an O(n) rather than an O(n2) solution, except that n in this case is the larger of the difference between the smallest and largest character code or the length of the string and so not directly comparable.
Note: an earlier version of this used for-in
- which in practice turns out to be incredibly slow. I've updated it to use the character codes as indexes to keep the look up as fast as possible. What we really need is a hash table but given the small values of N and the small, relative speed up, it's probably not worth it for this problem. In fact, you should prefer @Guffa's solution. I'm including mine only because I ended up learning a lot from it.
function firstNonRepeatedCharacter(string) {
var counts = {};
var i, minCode = 999999, maxCode = -1;
for (i = 0; i < string.length; ++i) {
var letter = string.charAt(i);
var letterCode = string.charCodeAt(i);
if (letterCode < minCode) {
minCode = letterCode;
}
if (letterCode > maxCode) {
maxCode = letterCode;
}
var count = counts[letterCode];
if (count) {
count.count = count.count + 1;
}
else {
counts[letterCode] = { letter: letter, count: 1, index: i };
}
}
var smallestIndex = string.length;
for (i = minCode; i <= maxCode; ++i) {
var count = counts[i];
if (count && count.count === 1 && count.index < smallestIndex) {
smallestIndex = count.index;
}
}
return smallestIndex < string.length ? string.charAt(smallestIndex) : '';
}
See fiddle at http://jsfiddle.net/b2dE4/
Also a (slightly different than the comments) performance test at http://jsperf.com/24793051/2
If you're looking for the first occurrence of a letter that only occurs once, I would use another data structure to keep track of how many times each letter has been seen. This would let you do it with an O(n) rather than an O(n2) solution, except that n in this case is the larger of the difference between the smallest and largest character code or the length of the string and so not directly comparable.
Note: an earlier version of this used for-in
- which in practice turns out to be incredibly slow. I've updated it to use the character codes as indexes to keep the look up as fast as possible. What we really need is a hash table but given the small values of N and the small, relative speed up, it's probably not worth it for this problem. In fact, you should prefer @Guffa's solution. I'm including mine only because I ended up learning a lot from it.
function firstNonRepeatedCharacter(string) {
var counts = {};
var i, minCode = 999999, maxCode = -1;
for (i = 0; i < string.length; ++i) {
var letter = string.charAt(i);
var letterCode = string.charCodeAt(i);
if (letterCode < minCode) {
minCode = letterCode;
}
if (letterCode > maxCode) {
maxCode = letterCode;
}
var count = counts[letterCode];
if (count) {
count.count = count.count + 1;
}
else {
counts[letterCode] = { letter: letter, count: 1, index: i };
}
}
var smallestIndex = string.length;
for (i = minCode; i <= maxCode; ++i) {
var count = counts[i];
if (count && count.count === 1 && count.index < smallestIndex) {
smallestIndex = count.index;
}
}
return smallestIndex < string.length ? string.charAt(smallestIndex) : '';
}
See fiddle at http://jsfiddle.net/b2dE4/
Also a (slightly different than the comments) performance test at http://jsperf.com/24793051/2
edited Jul 17 '14 at 2:06
answered Jul 17 '14 at 1:03
tvanfossontvanfosson
426k80645751
426k80645751
jsperf.com/24793051
– Xotic750
Jul 17 '14 at 1:10
@Xotic750 - that's very interesting. I guess I was assuming that the object index would be an O(1) operation, but it's obviously not. I wonder if converting it to an integer would improve that.
– tvanfosson
Jul 17 '14 at 1:19
Usingcounts = {}
may give a small improvement. It's worth noting how poor Opera is, but your code gets a big boost with that browser.
– Xotic750
Jul 17 '14 at 1:27
1
@Xotic750 - I've got an updated algorithm which runs significantly faster, though probably not on the small string test case. I had always assumed thatfor-in
and the property index operations would be O(1) but apparently they're not. Good learning opportunity.
– tvanfosson
Jul 17 '14 at 2:08
add a comment |
jsperf.com/24793051
– Xotic750
Jul 17 '14 at 1:10
@Xotic750 - that's very interesting. I guess I was assuming that the object index would be an O(1) operation, but it's obviously not. I wonder if converting it to an integer would improve that.
– tvanfosson
Jul 17 '14 at 1:19
Usingcounts = {}
may give a small improvement. It's worth noting how poor Opera is, but your code gets a big boost with that browser.
– Xotic750
Jul 17 '14 at 1:27
1
@Xotic750 - I've got an updated algorithm which runs significantly faster, though probably not on the small string test case. I had always assumed thatfor-in
and the property index operations would be O(1) but apparently they're not. Good learning opportunity.
– tvanfosson
Jul 17 '14 at 2:08
jsperf.com/24793051
– Xotic750
Jul 17 '14 at 1:10
jsperf.com/24793051
– Xotic750
Jul 17 '14 at 1:10
@Xotic750 - that's very interesting. I guess I was assuming that the object index would be an O(1) operation, but it's obviously not. I wonder if converting it to an integer would improve that.
– tvanfosson
Jul 17 '14 at 1:19
@Xotic750 - that's very interesting. I guess I was assuming that the object index would be an O(1) operation, but it's obviously not. I wonder if converting it to an integer would improve that.
– tvanfosson
Jul 17 '14 at 1:19
Using
counts = {}
may give a small improvement. It's worth noting how poor Opera is, but your code gets a big boost with that browser.– Xotic750
Jul 17 '14 at 1:27
Using
counts = {}
may give a small improvement. It's worth noting how poor Opera is, but your code gets a big boost with that browser.– Xotic750
Jul 17 '14 at 1:27
1
1
@Xotic750 - I've got an updated algorithm which runs significantly faster, though probably not on the small string test case. I had always assumed that
for-in
and the property index operations would be O(1) but apparently they're not. Good learning opportunity.– tvanfosson
Jul 17 '14 at 2:08
@Xotic750 - I've got an updated algorithm which runs significantly faster, though probably not on the small string test case. I had always assumed that
for-in
and the property index operations would be O(1) but apparently they're not. Good learning opportunity.– tvanfosson
Jul 17 '14 at 2:08
add a comment |
var firstNonRepeatedCharacter = function(string) {
var chars = string.split('');
for (var i = 0; i < string.length; i++) {
if (chars.filter(function(j) {
return j == string.charAt(i);
}).length == 1) return string.charAt(i);
}
};
So we create an array of all the characters, by splitting on anything.
Then, we loop through each character, and we filter the array we created, so we'll get an array of only those characters. If the length is ever 1, we know we have a non-repeated character.
Fiddle: http://jsfiddle.net/2FpZF/
add a comment |
var firstNonRepeatedCharacter = function(string) {
var chars = string.split('');
for (var i = 0; i < string.length; i++) {
if (chars.filter(function(j) {
return j == string.charAt(i);
}).length == 1) return string.charAt(i);
}
};
So we create an array of all the characters, by splitting on anything.
Then, we loop through each character, and we filter the array we created, so we'll get an array of only those characters. If the length is ever 1, we know we have a non-repeated character.
Fiddle: http://jsfiddle.net/2FpZF/
add a comment |
var firstNonRepeatedCharacter = function(string) {
var chars = string.split('');
for (var i = 0; i < string.length; i++) {
if (chars.filter(function(j) {
return j == string.charAt(i);
}).length == 1) return string.charAt(i);
}
};
So we create an array of all the characters, by splitting on anything.
Then, we loop through each character, and we filter the array we created, so we'll get an array of only those characters. If the length is ever 1, we know we have a non-repeated character.
Fiddle: http://jsfiddle.net/2FpZF/
var firstNonRepeatedCharacter = function(string) {
var chars = string.split('');
for (var i = 0; i < string.length; i++) {
if (chars.filter(function(j) {
return j == string.charAt(i);
}).length == 1) return string.charAt(i);
}
};
So we create an array of all the characters, by splitting on anything.
Then, we loop through each character, and we filter the array we created, so we'll get an array of only those characters. If the length is ever 1, we know we have a non-repeated character.
Fiddle: http://jsfiddle.net/2FpZF/
answered Jul 17 '14 at 0:37


davedave
35.2k13764
35.2k13764
add a comment |
add a comment |
First of all, start your loop at 1, not 0. There is no point in checking the first character to see if its repeating, obviously it can't be.
Now, within your loop, you have someString[i] and someString[i - 1]. They are the current and previous characters.
if someString[i] === someString[i - 1] then the characters are repeating, if someString[i] !== someString[i - 1] then they are not repeating, so you return someString[i]
I won't write the whole thing out for you, but hopefully the thought process behind this will help
That only checks if it repeats the character before it. His example shows it can repeat anywhere -abcdea
has a repeatinga
– dave
Jul 17 '14 at 0:41
Thank you for understanding what I was asking Dave. Thank you for answering anyway Martin
– TaylorAllred
Jul 17 '14 at 0:43
add a comment |
First of all, start your loop at 1, not 0. There is no point in checking the first character to see if its repeating, obviously it can't be.
Now, within your loop, you have someString[i] and someString[i - 1]. They are the current and previous characters.
if someString[i] === someString[i - 1] then the characters are repeating, if someString[i] !== someString[i - 1] then they are not repeating, so you return someString[i]
I won't write the whole thing out for you, but hopefully the thought process behind this will help
That only checks if it repeats the character before it. His example shows it can repeat anywhere -abcdea
has a repeatinga
– dave
Jul 17 '14 at 0:41
Thank you for understanding what I was asking Dave. Thank you for answering anyway Martin
– TaylorAllred
Jul 17 '14 at 0:43
add a comment |
First of all, start your loop at 1, not 0. There is no point in checking the first character to see if its repeating, obviously it can't be.
Now, within your loop, you have someString[i] and someString[i - 1]. They are the current and previous characters.
if someString[i] === someString[i - 1] then the characters are repeating, if someString[i] !== someString[i - 1] then they are not repeating, so you return someString[i]
I won't write the whole thing out for you, but hopefully the thought process behind this will help
First of all, start your loop at 1, not 0. There is no point in checking the first character to see if its repeating, obviously it can't be.
Now, within your loop, you have someString[i] and someString[i - 1]. They are the current and previous characters.
if someString[i] === someString[i - 1] then the characters are repeating, if someString[i] !== someString[i - 1] then they are not repeating, so you return someString[i]
I won't write the whole thing out for you, but hopefully the thought process behind this will help
answered Jul 17 '14 at 0:40
Martin BoothMartin Booth
7,3942429
7,3942429
That only checks if it repeats the character before it. His example shows it can repeat anywhere -abcdea
has a repeatinga
– dave
Jul 17 '14 at 0:41
Thank you for understanding what I was asking Dave. Thank you for answering anyway Martin
– TaylorAllred
Jul 17 '14 at 0:43
add a comment |
That only checks if it repeats the character before it. His example shows it can repeat anywhere -abcdea
has a repeatinga
– dave
Jul 17 '14 at 0:41
Thank you for understanding what I was asking Dave. Thank you for answering anyway Martin
– TaylorAllred
Jul 17 '14 at 0:43
That only checks if it repeats the character before it. His example shows it can repeat anywhere -
abcdea
has a repeating a
– dave
Jul 17 '14 at 0:41
That only checks if it repeats the character before it. His example shows it can repeat anywhere -
abcdea
has a repeating a
– dave
Jul 17 '14 at 0:41
Thank you for understanding what I was asking Dave. Thank you for answering anyway Martin
– TaylorAllred
Jul 17 '14 at 0:43
Thank you for understanding what I was asking Dave. Thank you for answering anyway Martin
– TaylorAllred
Jul 17 '14 at 0:43
add a comment |
function FirstNotRepeatedChar(str) {
var arr = str.split('');
var result = '';
var ctr = 0;
for (var x = 0; x < arr.length; x++) {
ctr = 0;
for (var y = 0; y < arr.length; y++) {
if (arr[x] === arr[y]) {
ctr+= 1;
}
}
if (ctr < 2) {
result = arr[x];
break;
}
}
return result;
}
console.log(FirstNotRepeatedChar('asif shaik'));
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Subramanyam
Apr 18 '18 at 9:11
add a comment |
function FirstNotRepeatedChar(str) {
var arr = str.split('');
var result = '';
var ctr = 0;
for (var x = 0; x < arr.length; x++) {
ctr = 0;
for (var y = 0; y < arr.length; y++) {
if (arr[x] === arr[y]) {
ctr+= 1;
}
}
if (ctr < 2) {
result = arr[x];
break;
}
}
return result;
}
console.log(FirstNotRepeatedChar('asif shaik'));
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Subramanyam
Apr 18 '18 at 9:11
add a comment |
function FirstNotRepeatedChar(str) {
var arr = str.split('');
var result = '';
var ctr = 0;
for (var x = 0; x < arr.length; x++) {
ctr = 0;
for (var y = 0; y < arr.length; y++) {
if (arr[x] === arr[y]) {
ctr+= 1;
}
}
if (ctr < 2) {
result = arr[x];
break;
}
}
return result;
}
console.log(FirstNotRepeatedChar('asif shaik'));
function FirstNotRepeatedChar(str) {
var arr = str.split('');
var result = '';
var ctr = 0;
for (var x = 0; x < arr.length; x++) {
ctr = 0;
for (var y = 0; y < arr.length; y++) {
if (arr[x] === arr[y]) {
ctr+= 1;
}
}
if (ctr < 2) {
result = arr[x];
break;
}
}
return result;
}
console.log(FirstNotRepeatedChar('asif shaik'));
edited Apr 18 '18 at 9:11
answered Apr 18 '18 at 9:02


SubramanyamSubramanyam
217
217
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Subramanyam
Apr 18 '18 at 9:11
add a comment |
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Subramanyam
Apr 18 '18 at 9:11
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Subramanyam
Apr 18 '18 at 9:11
developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/…
– Subramanyam
Apr 18 '18 at 9:11
add a comment |
Two further possibilities, using ECMA5 array methods. Will return undefined
if none exist.
Javascript
function firstNonRepeatedCharacter(string) {
return string.split('').filter(function (character, index, obj) {
return obj.indexOf(character) === obj.lastIndexOf(character);
}).shift();
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
Or if you want a bit better performance, especially on longer strings.
Javascript
function firstNonRepeatedCharacter(string) {
var first;
string.split('').some(function (character, index, obj) {
if(obj.indexOf(character) === obj.lastIndexOf(character)) {
first = character;
return true;
}
return false;
});
return first;
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
add a comment |
Two further possibilities, using ECMA5 array methods. Will return undefined
if none exist.
Javascript
function firstNonRepeatedCharacter(string) {
return string.split('').filter(function (character, index, obj) {
return obj.indexOf(character) === obj.lastIndexOf(character);
}).shift();
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
Or if you want a bit better performance, especially on longer strings.
Javascript
function firstNonRepeatedCharacter(string) {
var first;
string.split('').some(function (character, index, obj) {
if(obj.indexOf(character) === obj.lastIndexOf(character)) {
first = character;
return true;
}
return false;
});
return first;
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
add a comment |
Two further possibilities, using ECMA5 array methods. Will return undefined
if none exist.
Javascript
function firstNonRepeatedCharacter(string) {
return string.split('').filter(function (character, index, obj) {
return obj.indexOf(character) === obj.lastIndexOf(character);
}).shift();
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
Or if you want a bit better performance, especially on longer strings.
Javascript
function firstNonRepeatedCharacter(string) {
var first;
string.split('').some(function (character, index, obj) {
if(obj.indexOf(character) === obj.lastIndexOf(character)) {
first = character;
return true;
}
return false;
});
return first;
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
Two further possibilities, using ECMA5 array methods. Will return undefined
if none exist.
Javascript
function firstNonRepeatedCharacter(string) {
return string.split('').filter(function (character, index, obj) {
return obj.indexOf(character) === obj.lastIndexOf(character);
}).shift();
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
Or if you want a bit better performance, especially on longer strings.
Javascript
function firstNonRepeatedCharacter(string) {
var first;
string.split('').some(function (character, index, obj) {
if(obj.indexOf(character) === obj.lastIndexOf(character)) {
first = character;
return true;
}
return false;
});
return first;
}
console.log(firstNonRepeatedCharacter('aabcbd'));
On jsFiddle
edited Jul 17 '14 at 1:01
answered Jul 17 '14 at 0:46
Xotic750Xotic750
16.1k63963
16.1k63963
add a comment |
add a comment |
Fill an empty array with zeros, with same length as the string array, and tally up how many times they appear through the loop. Grab the first one in the tallied array with a value of 1.
function firstNotRepeatingCharacter(s) {
const array = s.split("");
let scores = new Array(array.length).fill(0);
for (let char of array) {
scores[array.indexOf(char)]++;
}
const singleChar = array[scores.indexOf(1)];
return singleChar ? singleChar : "_"
}
add a comment |
Fill an empty array with zeros, with same length as the string array, and tally up how many times they appear through the loop. Grab the first one in the tallied array with a value of 1.
function firstNotRepeatingCharacter(s) {
const array = s.split("");
let scores = new Array(array.length).fill(0);
for (let char of array) {
scores[array.indexOf(char)]++;
}
const singleChar = array[scores.indexOf(1)];
return singleChar ? singleChar : "_"
}
add a comment |
Fill an empty array with zeros, with same length as the string array, and tally up how many times they appear through the loop. Grab the first one in the tallied array with a value of 1.
function firstNotRepeatingCharacter(s) {
const array = s.split("");
let scores = new Array(array.length).fill(0);
for (let char of array) {
scores[array.indexOf(char)]++;
}
const singleChar = array[scores.indexOf(1)];
return singleChar ? singleChar : "_"
}
Fill an empty array with zeros, with same length as the string array, and tally up how many times they appear through the loop. Grab the first one in the tallied array with a value of 1.
function firstNotRepeatingCharacter(s) {
const array = s.split("");
let scores = new Array(array.length).fill(0);
for (let char of array) {
scores[array.indexOf(char)]++;
}
const singleChar = array[scores.indexOf(1)];
return singleChar ? singleChar : "_"
}
answered May 28 '18 at 22:43


muninn9muninn9
3682719
3682719
add a comment |
add a comment |
Here's an O(n) solution with 2 ES6 Sets, one tracking all characters that have appeared and one tracking only chars that have appeared once. This solution takes advantage of the insertion order preserved by Set.
const firstNonRepeating = str => {
const set = new Set();
const finalSet = new Set();
str.split('').forEach(char => {
if (set.has(char)) finalSet.delete(char);
else {
set.add(char);
finalSet.add(char);
}
})
const iter = finalSet.values();
return iter.next().value;
}
add a comment |
Here's an O(n) solution with 2 ES6 Sets, one tracking all characters that have appeared and one tracking only chars that have appeared once. This solution takes advantage of the insertion order preserved by Set.
const firstNonRepeating = str => {
const set = new Set();
const finalSet = new Set();
str.split('').forEach(char => {
if (set.has(char)) finalSet.delete(char);
else {
set.add(char);
finalSet.add(char);
}
})
const iter = finalSet.values();
return iter.next().value;
}
add a comment |
Here's an O(n) solution with 2 ES6 Sets, one tracking all characters that have appeared and one tracking only chars that have appeared once. This solution takes advantage of the insertion order preserved by Set.
const firstNonRepeating = str => {
const set = new Set();
const finalSet = new Set();
str.split('').forEach(char => {
if (set.has(char)) finalSet.delete(char);
else {
set.add(char);
finalSet.add(char);
}
})
const iter = finalSet.values();
return iter.next().value;
}
Here's an O(n) solution with 2 ES6 Sets, one tracking all characters that have appeared and one tracking only chars that have appeared once. This solution takes advantage of the insertion order preserved by Set.
const firstNonRepeating = str => {
const set = new Set();
const finalSet = new Set();
str.split('').forEach(char => {
if (set.has(char)) finalSet.delete(char);
else {
set.add(char);
finalSet.add(char);
}
})
const iter = finalSet.values();
return iter.next().value;
}
answered Oct 18 '18 at 15:25


Andrew HeekinAndrew Heekin
3781213
3781213
add a comment |
add a comment |
I came accross this while facing similar problem. Let me add my 2 lines.
What I did is a similar to the Guffa's answer. But using both indexOf
method and lastIndexOf
.
My mehod:
function nonRepeated(str) {
for(let i = 0; i < str.length; i++) {
let j = str.charAt(i)
if (str.indexOf(j) == str.lastIndexOf(j)) {
return j;
}
}
return null;
}
nonRepeated("aabcbd"); //c
Simply, indexOf()
gets first occurrence of a character & lastIndexOf()
gets the last occurrence. So when the first occurrence is also == the last occurence, it means there's just one the character.
add a comment |
I came accross this while facing similar problem. Let me add my 2 lines.
What I did is a similar to the Guffa's answer. But using both indexOf
method and lastIndexOf
.
My mehod:
function nonRepeated(str) {
for(let i = 0; i < str.length; i++) {
let j = str.charAt(i)
if (str.indexOf(j) == str.lastIndexOf(j)) {
return j;
}
}
return null;
}
nonRepeated("aabcbd"); //c
Simply, indexOf()
gets first occurrence of a character & lastIndexOf()
gets the last occurrence. So when the first occurrence is also == the last occurence, it means there's just one the character.
add a comment |
I came accross this while facing similar problem. Let me add my 2 lines.
What I did is a similar to the Guffa's answer. But using both indexOf
method and lastIndexOf
.
My mehod:
function nonRepeated(str) {
for(let i = 0; i < str.length; i++) {
let j = str.charAt(i)
if (str.indexOf(j) == str.lastIndexOf(j)) {
return j;
}
}
return null;
}
nonRepeated("aabcbd"); //c
Simply, indexOf()
gets first occurrence of a character & lastIndexOf()
gets the last occurrence. So when the first occurrence is also == the last occurence, it means there's just one the character.
I came accross this while facing similar problem. Let me add my 2 lines.
What I did is a similar to the Guffa's answer. But using both indexOf
method and lastIndexOf
.
My mehod:
function nonRepeated(str) {
for(let i = 0; i < str.length; i++) {
let j = str.charAt(i)
if (str.indexOf(j) == str.lastIndexOf(j)) {
return j;
}
}
return null;
}
nonRepeated("aabcbd"); //c
Simply, indexOf()
gets first occurrence of a character & lastIndexOf()
gets the last occurrence. So when the first occurrence is also == the last occurence, it means there's just one the character.
answered Nov 4 '18 at 21:39


Abubakar SamboAbubakar Sambo
286
286
add a comment |
add a comment |
You can iterate through each character to find()
the first letter that returns a single match()
. This will result in the first non-repeated character in the given string:
const first_nonrepeated_character = string => [...string].find(e => string.match(new RegExp(e, 'g')).length === 1);
const string = 'aabcbd';
console.log(first_nonrepeated_character(string)); // c
add a comment |
You can iterate through each character to find()
the first letter that returns a single match()
. This will result in the first non-repeated character in the given string:
const first_nonrepeated_character = string => [...string].find(e => string.match(new RegExp(e, 'g')).length === 1);
const string = 'aabcbd';
console.log(first_nonrepeated_character(string)); // c
add a comment |
You can iterate through each character to find()
the first letter that returns a single match()
. This will result in the first non-repeated character in the given string:
const first_nonrepeated_character = string => [...string].find(e => string.match(new RegExp(e, 'g')).length === 1);
const string = 'aabcbd';
console.log(first_nonrepeated_character(string)); // c
You can iterate through each character to find()
the first letter that returns a single match()
. This will result in the first non-repeated character in the given string:
const first_nonrepeated_character = string => [...string].find(e => string.match(new RegExp(e, 'g')).length === 1);
const string = 'aabcbd';
console.log(first_nonrepeated_character(string)); // c
const first_nonrepeated_character = string => [...string].find(e => string.match(new RegExp(e, 'g')).length === 1);
const string = 'aabcbd';
console.log(first_nonrepeated_character(string)); // c
const first_nonrepeated_character = string => [...string].find(e => string.match(new RegExp(e, 'g')).length === 1);
const string = 'aabcbd';
console.log(first_nonrepeated_character(string)); // c
answered Nov 18 '18 at 6:45


Grant MillerGrant Miller
5,767132952
5,767132952
add a comment |
add a comment |
let arr = [10, 5, 3, 4, 3, 5, 6];
outer:for(let i=0;i<arr.length;i++){
for(let j=0;j<arr.length;j++){
if(arr[i]===arr[j+1]){
console.log(arr[i]);
break outer;
}
}
}
//or else you may try this way...
function firstDuplicate(arr) {
let findFirst = new Set()
for (element of arr)
if (findFirst.has(element ))
return element
else
findFirst.add(element )
}
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Brevity is acceptable, but fuller explanations are better.
– Armali
yesterday
add a comment |
let arr = [10, 5, 3, 4, 3, 5, 6];
outer:for(let i=0;i<arr.length;i++){
for(let j=0;j<arr.length;j++){
if(arr[i]===arr[j+1]){
console.log(arr[i]);
break outer;
}
}
}
//or else you may try this way...
function firstDuplicate(arr) {
let findFirst = new Set()
for (element of arr)
if (findFirst.has(element ))
return element
else
findFirst.add(element )
}
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Brevity is acceptable, but fuller explanations are better.
– Armali
yesterday
add a comment |
let arr = [10, 5, 3, 4, 3, 5, 6];
outer:for(let i=0;i<arr.length;i++){
for(let j=0;j<arr.length;j++){
if(arr[i]===arr[j+1]){
console.log(arr[i]);
break outer;
}
}
}
//or else you may try this way...
function firstDuplicate(arr) {
let findFirst = new Set()
for (element of arr)
if (findFirst.has(element ))
return element
else
findFirst.add(element )
}
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
let arr = [10, 5, 3, 4, 3, 5, 6];
outer:for(let i=0;i<arr.length;i++){
for(let j=0;j<arr.length;j++){
if(arr[i]===arr[j+1]){
console.log(arr[i]);
break outer;
}
}
}
//or else you may try this way...
function firstDuplicate(arr) {
let findFirst = new Set()
for (element of arr)
if (findFirst.has(element ))
return element
else
findFirst.add(element )
}
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
answered yesterday


Vinit KumarVinit Kumar
11
11
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Vinit Kumar is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
Brevity is acceptable, but fuller explanations are better.
– Armali
yesterday
add a comment |
Brevity is acceptable, but fuller explanations are better.
– Armali
yesterday
Brevity is acceptable, but fuller explanations are better.
– Armali
yesterday
Brevity is acceptable, but fuller explanations are better.
– Armali
yesterday
add a comment |
solution: map characters into an object
To begin, an empty object is initialized to be a count
. Each char
of the count
is assigned as each letter from the input string.
If the letter
doesn't already exist in the count
, then the letter
is assigned a value of 1
. Otherwise, if the letter
already exists, increase the count of its occurrence by 1
.
After looping through each letter in the input string and assigning each letter to a key-value pair in the count
, the next step is to loop through each property (key-value pair) in the count
.
While looping through the properties in the count
, the first property equal to 1
is the first non-repeating character in the input string.
The expected and actual output from invoking firstNonRepeatedCharacter(someString)
is c
.
var firstNonRepeatedCharacter = function(string) {
var count = {};
for (var i = 0; i < string.length; i++) {
var letter = string[i];
if (!count[letter]) {
count[letter] = 1;
} else {
count[letter]++;
}
}
for (var letter in count) {
if (count[letter] === 1) {
return letter;
}
}
return null;
}
var someString = 'aabcbd';
console.log(firstNonRepeatedCharacter(someString));
add a comment |
solution: map characters into an object
To begin, an empty object is initialized to be a count
. Each char
of the count
is assigned as each letter from the input string.
If the letter
doesn't already exist in the count
, then the letter
is assigned a value of 1
. Otherwise, if the letter
already exists, increase the count of its occurrence by 1
.
After looping through each letter in the input string and assigning each letter to a key-value pair in the count
, the next step is to loop through each property (key-value pair) in the count
.
While looping through the properties in the count
, the first property equal to 1
is the first non-repeating character in the input string.
The expected and actual output from invoking firstNonRepeatedCharacter(someString)
is c
.
var firstNonRepeatedCharacter = function(string) {
var count = {};
for (var i = 0; i < string.length; i++) {
var letter = string[i];
if (!count[letter]) {
count[letter] = 1;
} else {
count[letter]++;
}
}
for (var letter in count) {
if (count[letter] === 1) {
return letter;
}
}
return null;
}
var someString = 'aabcbd';
console.log(firstNonRepeatedCharacter(someString));
add a comment |
solution: map characters into an object
To begin, an empty object is initialized to be a count
. Each char
of the count
is assigned as each letter from the input string.
If the letter
doesn't already exist in the count
, then the letter
is assigned a value of 1
. Otherwise, if the letter
already exists, increase the count of its occurrence by 1
.
After looping through each letter in the input string and assigning each letter to a key-value pair in the count
, the next step is to loop through each property (key-value pair) in the count
.
While looping through the properties in the count
, the first property equal to 1
is the first non-repeating character in the input string.
The expected and actual output from invoking firstNonRepeatedCharacter(someString)
is c
.
var firstNonRepeatedCharacter = function(string) {
var count = {};
for (var i = 0; i < string.length; i++) {
var letter = string[i];
if (!count[letter]) {
count[letter] = 1;
} else {
count[letter]++;
}
}
for (var letter in count) {
if (count[letter] === 1) {
return letter;
}
}
return null;
}
var someString = 'aabcbd';
console.log(firstNonRepeatedCharacter(someString));
solution: map characters into an object
To begin, an empty object is initialized to be a count
. Each char
of the count
is assigned as each letter from the input string.
If the letter
doesn't already exist in the count
, then the letter
is assigned a value of 1
. Otherwise, if the letter
already exists, increase the count of its occurrence by 1
.
After looping through each letter in the input string and assigning each letter to a key-value pair in the count
, the next step is to loop through each property (key-value pair) in the count
.
While looping through the properties in the count
, the first property equal to 1
is the first non-repeating character in the input string.
The expected and actual output from invoking firstNonRepeatedCharacter(someString)
is c
.
var firstNonRepeatedCharacter = function(string) {
var count = {};
for (var i = 0; i < string.length; i++) {
var letter = string[i];
if (!count[letter]) {
count[letter] = 1;
} else {
count[letter]++;
}
}
for (var letter in count) {
if (count[letter] === 1) {
return letter;
}
}
return null;
}
var someString = 'aabcbd';
console.log(firstNonRepeatedCharacter(someString));
var firstNonRepeatedCharacter = function(string) {
var count = {};
for (var i = 0; i < string.length; i++) {
var letter = string[i];
if (!count[letter]) {
count[letter] = 1;
} else {
count[letter]++;
}
}
for (var letter in count) {
if (count[letter] === 1) {
return letter;
}
}
return null;
}
var someString = 'aabcbd';
console.log(firstNonRepeatedCharacter(someString));
var firstNonRepeatedCharacter = function(string) {
var count = {};
for (var i = 0; i < string.length; i++) {
var letter = string[i];
if (!count[letter]) {
count[letter] = 1;
} else {
count[letter]++;
}
}
for (var letter in count) {
if (count[letter] === 1) {
return letter;
}
}
return null;
}
var someString = 'aabcbd';
console.log(firstNonRepeatedCharacter(someString));
edited 14 hours ago
answered yesterday


underthecodeunderthecode
295
295
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f24793051%2freturn-the-first-non-repeating-character-in-a-string-in-javascript%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NUx563,SWJv
The index of a non-repeating character will be odd and it will be different than the character before it. That should be enough to get you through.
– tvanfosson
Jul 17 '14 at 0:33
I don't understand, how would the index be odd? In my example c is in the third index but what if it was aabbcd instead which means it would be in the 4th index.?
– TaylorAllred
Jul 17 '14 at 0:37
He thinks it has to repeat the character before it
– dave
Jul 17 '14 at 0:38
Are you familiar with map objects in JavaScript? Such as:
var charMap = {}; charMap['a'] = 0; charMap['b'] = 1;
? You could iterate through the input string, adding every char into a charMap: jsfiddle.net/w7F87/14– acdcjunior
Jul 17 '14 at 0:41
@user3806863 - using the logic you outlined, in the sample in your comment the non-repeat would be 'd', the 6th letter at index 5.
– tvanfosson
Jul 17 '14 at 0:47