comparing a date string with current Date()
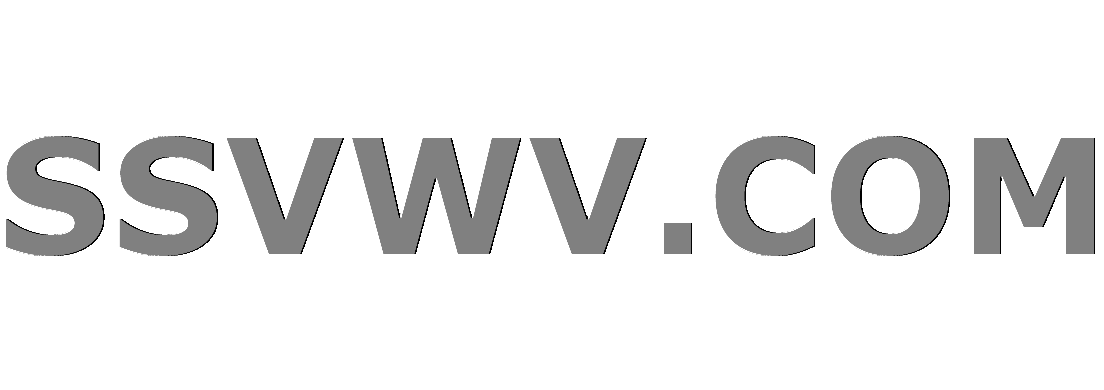
Multi tool use
So, I already have a variable that holds all the cells in a certain column.
each cell contains, as it's innerText, a timestamp formatted like such, yyyy-mm-dd hh:mm in 24h format.
How do I go about comparing the string that I have with Date() to see if the string is within the next hour?
I was thinking a for loop to go through the array with an if function inside saying "if the time shown is within an hour of the current time then change the background color of the cell to red.
for(var i=0; i<column.length;i++){
if(column[i].innerText - Date() < 1hr){ //this line needs work
column[i].style.backgroundColor='#ff000';
} else(){
}
};
I'm sure there probably needs to be some parse method used or something but I'm not too familiar with it.
note: I'm using Tampermonkey to inject the code into a page I have no control over and so the timestamps are as the come.
javascript date string-comparison tampermonkey
add a comment |
So, I already have a variable that holds all the cells in a certain column.
each cell contains, as it's innerText, a timestamp formatted like such, yyyy-mm-dd hh:mm in 24h format.
How do I go about comparing the string that I have with Date() to see if the string is within the next hour?
I was thinking a for loop to go through the array with an if function inside saying "if the time shown is within an hour of the current time then change the background color of the cell to red.
for(var i=0; i<column.length;i++){
if(column[i].innerText - Date() < 1hr){ //this line needs work
column[i].style.backgroundColor='#ff000';
} else(){
}
};
I'm sure there probably needs to be some parse method used or something but I'm not too familiar with it.
note: I'm using Tampermonkey to inject the code into a page I have no control over and so the timestamps are as the come.
javascript date string-comparison tampermonkey
add a comment |
So, I already have a variable that holds all the cells in a certain column.
each cell contains, as it's innerText, a timestamp formatted like such, yyyy-mm-dd hh:mm in 24h format.
How do I go about comparing the string that I have with Date() to see if the string is within the next hour?
I was thinking a for loop to go through the array with an if function inside saying "if the time shown is within an hour of the current time then change the background color of the cell to red.
for(var i=0; i<column.length;i++){
if(column[i].innerText - Date() < 1hr){ //this line needs work
column[i].style.backgroundColor='#ff000';
} else(){
}
};
I'm sure there probably needs to be some parse method used or something but I'm not too familiar with it.
note: I'm using Tampermonkey to inject the code into a page I have no control over and so the timestamps are as the come.
javascript date string-comparison tampermonkey
So, I already have a variable that holds all the cells in a certain column.
each cell contains, as it's innerText, a timestamp formatted like such, yyyy-mm-dd hh:mm in 24h format.
How do I go about comparing the string that I have with Date() to see if the string is within the next hour?
I was thinking a for loop to go through the array with an if function inside saying "if the time shown is within an hour of the current time then change the background color of the cell to red.
for(var i=0; i<column.length;i++){
if(column[i].innerText - Date() < 1hr){ //this line needs work
column[i].style.backgroundColor='#ff000';
} else(){
}
};
I'm sure there probably needs to be some parse method used or something but I'm not too familiar with it.
note: I'm using Tampermonkey to inject the code into a page I have no control over and so the timestamps are as the come.
javascript date string-comparison tampermonkey
javascript date string-comparison tampermonkey
asked Nov 18 '18 at 1:43


Pshock13Pshock13
345
345
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Date constructor does the job of parsing for you. So something like this would be all you need:
hour = 3600000 //1 hour in ms
nextHour = new Date(column[i].innerText) - new Date()
if(nextHour <= hour && nextHour >= 0) {
//Your code here
}
Explanation:
Since Javascript Date is based on milliseconds since midnight January 1, 1970, - (minus) operator allows you to treat it as a Number and returns the resulting number as a Number.
is there a way to compare the DAY as well, because I dont see how using .getHours() would help if the day in the column is after the current date.
– Pshock13
Nov 18 '18 at 2:47
You are correct. However the second case should fully cover this as it measures the time difference between two dates.
– Burak
Nov 18 '18 at 2:48
@Pshock13 I have now removed the first solution as it was faulty in so many ways. The current solution should work as it is though.
– Burak
Nov 18 '18 at 2:52
you needvar
in front of declaring your variables, but also, I tried making a newvar test = new Date(column[i].innerText)
. doingconsole.log(test)
inside my for loop returns invalid date for each one.
– Pshock13
Nov 18 '18 at 3:01
1
@Pshock13 that shouldn't happen if your dates are in the format you say. It might help to post the actual date string in the question.
– Mark Meyer
Nov 18 '18 at 3:02
|
show 6 more comments
Change this:
if(column[i].innerText - Date() < 1hr){
To this:
var hourNow = new Date().getHours();
var hourColumn = Number(column.innerText.split("")[11] + "" + column.innerText.split("")[12]);
if (hourNow + 1 >= hourColumn || hourColumn + 1 <= hourNow) {
And it should work.
How does this work over midnight?
– Mark Meyer
Nov 18 '18 at 2:35
1st: i expect you mean column[i] when splitting and not just column. 2nd: this doesn't work correctly if the date in the column isn't the same as the current date. ie column:4th while current date: 3rd.
– Pshock13
Nov 18 '18 at 2:35
add a comment |
You can go with below approach. Here I have used getUTCHours()
, because new Date(new Date(columns[i].innerText) - new Date())
will give UTC timestamp. You can find the explanation about UTC timestamp from here
var columns;
function changecolors() {
columns = document.getElementsByClassName('column');
for (var i = 0; i < columns.length; i++) {
if (new Date(new Date(columns[i].innerText) - new Date()).getUTCHours() < 1) {
columns[i].style.backgroundColor = '#ff0000';
}
};
}
<div class="column">2018-11-18 09:30</div>
<div class="column">2018-11-18 11:00</div>
<button onclick="changecolors()">Change Colors</button>
1
"yyyy-mm-dd hh:mm" is not a format supported by ECMAScript so parsing is implementation dependent. At least one current browser will return an invalid Date.
– RobG
Nov 18 '18 at 8:25
@RobG thank you for your explanation, got it : -)
– Javascript Lover - SKT
Nov 20 '18 at 6:10
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53357194%2fcomparing-a-date-string-with-current-date%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Date constructor does the job of parsing for you. So something like this would be all you need:
hour = 3600000 //1 hour in ms
nextHour = new Date(column[i].innerText) - new Date()
if(nextHour <= hour && nextHour >= 0) {
//Your code here
}
Explanation:
Since Javascript Date is based on milliseconds since midnight January 1, 1970, - (minus) operator allows you to treat it as a Number and returns the resulting number as a Number.
is there a way to compare the DAY as well, because I dont see how using .getHours() would help if the day in the column is after the current date.
– Pshock13
Nov 18 '18 at 2:47
You are correct. However the second case should fully cover this as it measures the time difference between two dates.
– Burak
Nov 18 '18 at 2:48
@Pshock13 I have now removed the first solution as it was faulty in so many ways. The current solution should work as it is though.
– Burak
Nov 18 '18 at 2:52
you needvar
in front of declaring your variables, but also, I tried making a newvar test = new Date(column[i].innerText)
. doingconsole.log(test)
inside my for loop returns invalid date for each one.
– Pshock13
Nov 18 '18 at 3:01
1
@Pshock13 that shouldn't happen if your dates are in the format you say. It might help to post the actual date string in the question.
– Mark Meyer
Nov 18 '18 at 3:02
|
show 6 more comments
Date constructor does the job of parsing for you. So something like this would be all you need:
hour = 3600000 //1 hour in ms
nextHour = new Date(column[i].innerText) - new Date()
if(nextHour <= hour && nextHour >= 0) {
//Your code here
}
Explanation:
Since Javascript Date is based on milliseconds since midnight January 1, 1970, - (minus) operator allows you to treat it as a Number and returns the resulting number as a Number.
is there a way to compare the DAY as well, because I dont see how using .getHours() would help if the day in the column is after the current date.
– Pshock13
Nov 18 '18 at 2:47
You are correct. However the second case should fully cover this as it measures the time difference between two dates.
– Burak
Nov 18 '18 at 2:48
@Pshock13 I have now removed the first solution as it was faulty in so many ways. The current solution should work as it is though.
– Burak
Nov 18 '18 at 2:52
you needvar
in front of declaring your variables, but also, I tried making a newvar test = new Date(column[i].innerText)
. doingconsole.log(test)
inside my for loop returns invalid date for each one.
– Pshock13
Nov 18 '18 at 3:01
1
@Pshock13 that shouldn't happen if your dates are in the format you say. It might help to post the actual date string in the question.
– Mark Meyer
Nov 18 '18 at 3:02
|
show 6 more comments
Date constructor does the job of parsing for you. So something like this would be all you need:
hour = 3600000 //1 hour in ms
nextHour = new Date(column[i].innerText) - new Date()
if(nextHour <= hour && nextHour >= 0) {
//Your code here
}
Explanation:
Since Javascript Date is based on milliseconds since midnight January 1, 1970, - (minus) operator allows you to treat it as a Number and returns the resulting number as a Number.
Date constructor does the job of parsing for you. So something like this would be all you need:
hour = 3600000 //1 hour in ms
nextHour = new Date(column[i].innerText) - new Date()
if(nextHour <= hour && nextHour >= 0) {
//Your code here
}
Explanation:
Since Javascript Date is based on milliseconds since midnight January 1, 1970, - (minus) operator allows you to treat it as a Number and returns the resulting number as a Number.
edited Nov 18 '18 at 15:45
answered Nov 18 '18 at 2:44
BurakBurak
736
736
is there a way to compare the DAY as well, because I dont see how using .getHours() would help if the day in the column is after the current date.
– Pshock13
Nov 18 '18 at 2:47
You are correct. However the second case should fully cover this as it measures the time difference between two dates.
– Burak
Nov 18 '18 at 2:48
@Pshock13 I have now removed the first solution as it was faulty in so many ways. The current solution should work as it is though.
– Burak
Nov 18 '18 at 2:52
you needvar
in front of declaring your variables, but also, I tried making a newvar test = new Date(column[i].innerText)
. doingconsole.log(test)
inside my for loop returns invalid date for each one.
– Pshock13
Nov 18 '18 at 3:01
1
@Pshock13 that shouldn't happen if your dates are in the format you say. It might help to post the actual date string in the question.
– Mark Meyer
Nov 18 '18 at 3:02
|
show 6 more comments
is there a way to compare the DAY as well, because I dont see how using .getHours() would help if the day in the column is after the current date.
– Pshock13
Nov 18 '18 at 2:47
You are correct. However the second case should fully cover this as it measures the time difference between two dates.
– Burak
Nov 18 '18 at 2:48
@Pshock13 I have now removed the first solution as it was faulty in so many ways. The current solution should work as it is though.
– Burak
Nov 18 '18 at 2:52
you needvar
in front of declaring your variables, but also, I tried making a newvar test = new Date(column[i].innerText)
. doingconsole.log(test)
inside my for loop returns invalid date for each one.
– Pshock13
Nov 18 '18 at 3:01
1
@Pshock13 that shouldn't happen if your dates are in the format you say. It might help to post the actual date string in the question.
– Mark Meyer
Nov 18 '18 at 3:02
is there a way to compare the DAY as well, because I dont see how using .getHours() would help if the day in the column is after the current date.
– Pshock13
Nov 18 '18 at 2:47
is there a way to compare the DAY as well, because I dont see how using .getHours() would help if the day in the column is after the current date.
– Pshock13
Nov 18 '18 at 2:47
You are correct. However the second case should fully cover this as it measures the time difference between two dates.
– Burak
Nov 18 '18 at 2:48
You are correct. However the second case should fully cover this as it measures the time difference between two dates.
– Burak
Nov 18 '18 at 2:48
@Pshock13 I have now removed the first solution as it was faulty in so many ways. The current solution should work as it is though.
– Burak
Nov 18 '18 at 2:52
@Pshock13 I have now removed the first solution as it was faulty in so many ways. The current solution should work as it is though.
– Burak
Nov 18 '18 at 2:52
you need
var
in front of declaring your variables, but also, I tried making a new var test = new Date(column[i].innerText)
. doing console.log(test)
inside my for loop returns invalid date for each one.– Pshock13
Nov 18 '18 at 3:01
you need
var
in front of declaring your variables, but also, I tried making a new var test = new Date(column[i].innerText)
. doing console.log(test)
inside my for loop returns invalid date for each one.– Pshock13
Nov 18 '18 at 3:01
1
1
@Pshock13 that shouldn't happen if your dates are in the format you say. It might help to post the actual date string in the question.
– Mark Meyer
Nov 18 '18 at 3:02
@Pshock13 that shouldn't happen if your dates are in the format you say. It might help to post the actual date string in the question.
– Mark Meyer
Nov 18 '18 at 3:02
|
show 6 more comments
Change this:
if(column[i].innerText - Date() < 1hr){
To this:
var hourNow = new Date().getHours();
var hourColumn = Number(column.innerText.split("")[11] + "" + column.innerText.split("")[12]);
if (hourNow + 1 >= hourColumn || hourColumn + 1 <= hourNow) {
And it should work.
How does this work over midnight?
– Mark Meyer
Nov 18 '18 at 2:35
1st: i expect you mean column[i] when splitting and not just column. 2nd: this doesn't work correctly if the date in the column isn't the same as the current date. ie column:4th while current date: 3rd.
– Pshock13
Nov 18 '18 at 2:35
add a comment |
Change this:
if(column[i].innerText - Date() < 1hr){
To this:
var hourNow = new Date().getHours();
var hourColumn = Number(column.innerText.split("")[11] + "" + column.innerText.split("")[12]);
if (hourNow + 1 >= hourColumn || hourColumn + 1 <= hourNow) {
And it should work.
How does this work over midnight?
– Mark Meyer
Nov 18 '18 at 2:35
1st: i expect you mean column[i] when splitting and not just column. 2nd: this doesn't work correctly if the date in the column isn't the same as the current date. ie column:4th while current date: 3rd.
– Pshock13
Nov 18 '18 at 2:35
add a comment |
Change this:
if(column[i].innerText - Date() < 1hr){
To this:
var hourNow = new Date().getHours();
var hourColumn = Number(column.innerText.split("")[11] + "" + column.innerText.split("")[12]);
if (hourNow + 1 >= hourColumn || hourColumn + 1 <= hourNow) {
And it should work.
Change this:
if(column[i].innerText - Date() < 1hr){
To this:
var hourNow = new Date().getHours();
var hourColumn = Number(column.innerText.split("")[11] + "" + column.innerText.split("")[12]);
if (hourNow + 1 >= hourColumn || hourColumn + 1 <= hourNow) {
And it should work.
answered Nov 18 '18 at 1:53
Jack BashfordJack Bashford
7,46931338
7,46931338
How does this work over midnight?
– Mark Meyer
Nov 18 '18 at 2:35
1st: i expect you mean column[i] when splitting and not just column. 2nd: this doesn't work correctly if the date in the column isn't the same as the current date. ie column:4th while current date: 3rd.
– Pshock13
Nov 18 '18 at 2:35
add a comment |
How does this work over midnight?
– Mark Meyer
Nov 18 '18 at 2:35
1st: i expect you mean column[i] when splitting and not just column. 2nd: this doesn't work correctly if the date in the column isn't the same as the current date. ie column:4th while current date: 3rd.
– Pshock13
Nov 18 '18 at 2:35
How does this work over midnight?
– Mark Meyer
Nov 18 '18 at 2:35
How does this work over midnight?
– Mark Meyer
Nov 18 '18 at 2:35
1st: i expect you mean column[i] when splitting and not just column. 2nd: this doesn't work correctly if the date in the column isn't the same as the current date. ie column:4th while current date: 3rd.
– Pshock13
Nov 18 '18 at 2:35
1st: i expect you mean column[i] when splitting and not just column. 2nd: this doesn't work correctly if the date in the column isn't the same as the current date. ie column:4th while current date: 3rd.
– Pshock13
Nov 18 '18 at 2:35
add a comment |
You can go with below approach. Here I have used getUTCHours()
, because new Date(new Date(columns[i].innerText) - new Date())
will give UTC timestamp. You can find the explanation about UTC timestamp from here
var columns;
function changecolors() {
columns = document.getElementsByClassName('column');
for (var i = 0; i < columns.length; i++) {
if (new Date(new Date(columns[i].innerText) - new Date()).getUTCHours() < 1) {
columns[i].style.backgroundColor = '#ff0000';
}
};
}
<div class="column">2018-11-18 09:30</div>
<div class="column">2018-11-18 11:00</div>
<button onclick="changecolors()">Change Colors</button>
1
"yyyy-mm-dd hh:mm" is not a format supported by ECMAScript so parsing is implementation dependent. At least one current browser will return an invalid Date.
– RobG
Nov 18 '18 at 8:25
@RobG thank you for your explanation, got it : -)
– Javascript Lover - SKT
Nov 20 '18 at 6:10
add a comment |
You can go with below approach. Here I have used getUTCHours()
, because new Date(new Date(columns[i].innerText) - new Date())
will give UTC timestamp. You can find the explanation about UTC timestamp from here
var columns;
function changecolors() {
columns = document.getElementsByClassName('column');
for (var i = 0; i < columns.length; i++) {
if (new Date(new Date(columns[i].innerText) - new Date()).getUTCHours() < 1) {
columns[i].style.backgroundColor = '#ff0000';
}
};
}
<div class="column">2018-11-18 09:30</div>
<div class="column">2018-11-18 11:00</div>
<button onclick="changecolors()">Change Colors</button>
1
"yyyy-mm-dd hh:mm" is not a format supported by ECMAScript so parsing is implementation dependent. At least one current browser will return an invalid Date.
– RobG
Nov 18 '18 at 8:25
@RobG thank you for your explanation, got it : -)
– Javascript Lover - SKT
Nov 20 '18 at 6:10
add a comment |
You can go with below approach. Here I have used getUTCHours()
, because new Date(new Date(columns[i].innerText) - new Date())
will give UTC timestamp. You can find the explanation about UTC timestamp from here
var columns;
function changecolors() {
columns = document.getElementsByClassName('column');
for (var i = 0; i < columns.length; i++) {
if (new Date(new Date(columns[i].innerText) - new Date()).getUTCHours() < 1) {
columns[i].style.backgroundColor = '#ff0000';
}
};
}
<div class="column">2018-11-18 09:30</div>
<div class="column">2018-11-18 11:00</div>
<button onclick="changecolors()">Change Colors</button>
You can go with below approach. Here I have used getUTCHours()
, because new Date(new Date(columns[i].innerText) - new Date())
will give UTC timestamp. You can find the explanation about UTC timestamp from here
var columns;
function changecolors() {
columns = document.getElementsByClassName('column');
for (var i = 0; i < columns.length; i++) {
if (new Date(new Date(columns[i].innerText) - new Date()).getUTCHours() < 1) {
columns[i].style.backgroundColor = '#ff0000';
}
};
}
<div class="column">2018-11-18 09:30</div>
<div class="column">2018-11-18 11:00</div>
<button onclick="changecolors()">Change Colors</button>
var columns;
function changecolors() {
columns = document.getElementsByClassName('column');
for (var i = 0; i < columns.length; i++) {
if (new Date(new Date(columns[i].innerText) - new Date()).getUTCHours() < 1) {
columns[i].style.backgroundColor = '#ff0000';
}
};
}
<div class="column">2018-11-18 09:30</div>
<div class="column">2018-11-18 11:00</div>
<button onclick="changecolors()">Change Colors</button>
var columns;
function changecolors() {
columns = document.getElementsByClassName('column');
for (var i = 0; i < columns.length; i++) {
if (new Date(new Date(columns[i].innerText) - new Date()).getUTCHours() < 1) {
columns[i].style.backgroundColor = '#ff0000';
}
};
}
<div class="column">2018-11-18 09:30</div>
<div class="column">2018-11-18 11:00</div>
<button onclick="changecolors()">Change Colors</button>
edited Nov 18 '18 at 3:27
answered Nov 18 '18 at 3:15


Javascript Lover - SKTJavascript Lover - SKT
1,2631525
1,2631525
1
"yyyy-mm-dd hh:mm" is not a format supported by ECMAScript so parsing is implementation dependent. At least one current browser will return an invalid Date.
– RobG
Nov 18 '18 at 8:25
@RobG thank you for your explanation, got it : -)
– Javascript Lover - SKT
Nov 20 '18 at 6:10
add a comment |
1
"yyyy-mm-dd hh:mm" is not a format supported by ECMAScript so parsing is implementation dependent. At least one current browser will return an invalid Date.
– RobG
Nov 18 '18 at 8:25
@RobG thank you for your explanation, got it : -)
– Javascript Lover - SKT
Nov 20 '18 at 6:10
1
1
"yyyy-mm-dd hh:mm" is not a format supported by ECMAScript so parsing is implementation dependent. At least one current browser will return an invalid Date.
– RobG
Nov 18 '18 at 8:25
"yyyy-mm-dd hh:mm" is not a format supported by ECMAScript so parsing is implementation dependent. At least one current browser will return an invalid Date.
– RobG
Nov 18 '18 at 8:25
@RobG thank you for your explanation, got it : -)
– Javascript Lover - SKT
Nov 20 '18 at 6:10
@RobG thank you for your explanation, got it : -)
– Javascript Lover - SKT
Nov 20 '18 at 6:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53357194%2fcomparing-a-date-string-with-current-date%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tYpm8Mnt6Q2jgPmFk831Zf