UWP ListView Drop get index
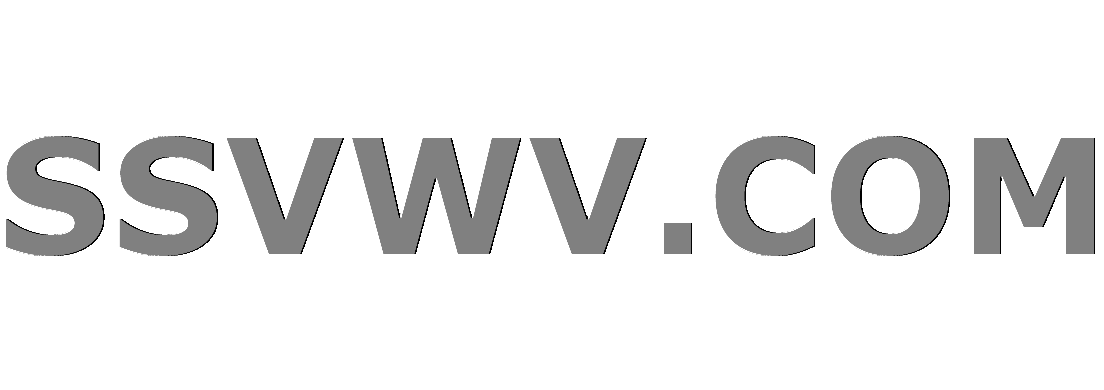
Multi tool use
up vote
0
down vote
favorite
I have 2 ListViews, I can drag an item from ListView1 and drop it on to ListView2. If I drop the item between other items I want the item to be added in that same location.
So the question is: How do I get the position/index where I dropped my item?
I would expect to find the index somewhere in the DragEventArgs of the Drop event, but I can't find it anywhere:
private async void ListView2_Drop(object sender, DragEventArgs e)
{
}
uwp drag-and-drop
add a comment |
up vote
0
down vote
favorite
I have 2 ListViews, I can drag an item from ListView1 and drop it on to ListView2. If I drop the item between other items I want the item to be added in that same location.
So the question is: How do I get the position/index where I dropped my item?
I would expect to find the index somewhere in the DragEventArgs of the Drop event, but I can't find it anywhere:
private async void ListView2_Drop(object sender, DragEventArgs e)
{
}
uwp drag-and-drop
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have 2 ListViews, I can drag an item from ListView1 and drop it on to ListView2. If I drop the item between other items I want the item to be added in that same location.
So the question is: How do I get the position/index where I dropped my item?
I would expect to find the index somewhere in the DragEventArgs of the Drop event, but I can't find it anywhere:
private async void ListView2_Drop(object sender, DragEventArgs e)
{
}
uwp drag-and-drop
I have 2 ListViews, I can drag an item from ListView1 and drop it on to ListView2. If I drop the item between other items I want the item to be added in that same location.
So the question is: How do I get the position/index where I dropped my item?
I would expect to find the index somewhere in the DragEventArgs of the Drop event, but I can't find it anywhere:
private async void ListView2_Drop(object sender, DragEventArgs e)
{
}
uwp drag-and-drop
uwp drag-and-drop
asked Nov 7 at 18:18


Niels
736916
736916
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
2
down vote
accepted
Anyway: I have tinkered around with this code and finally got it to work to my needs:
private async void MyListView_Drop(object sender, DragEventArgs e)
{
var scrollViewer = VisualTreeHelper.GetChild(VisualTreeHelper.GetChild(MyListView, 0), 0) as ScrollViewer;
var position = e.GetPosition((ListView)sender);
var positionY = scrollViewer.VerticalOffset + position.Y;
var index = GetItemIndex(positionY, MyListView);
// do something useful with the index...
}
int GetItemIndex(double positionY, ListView targetListView)
{
var index = 0;
double height = 0;
foreach (var item in targetListView.Items)
{
height += GetRowHeight(item, targetListView);
if (height > positionY) return index;
index++;
}
return index;
}
double GetRowHeight(object listItem, ListView targetListView)
{
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
return marginTop + height;
}
Thank you for pointing me in the right direction!
Well done, you could mark your answer.
– Nico Zhu - MSFT
Nov 9 at 2:14
add a comment |
up vote
0
down vote
How do I get the position/index where I dropped my item?
Currently, Drop
event does not provide the index of your dropped item. But you could get the drop position with GetPosition
method and calculate the index of your current dropped item. I have implement it based on official drag and drop code sample. And you could use the following code directly.
private double rowHeight;
private async void TargetListView_Drop(object sender, DragEventArgs e)
{
// This test is in theory not needed as we returned DataPackageOperation.None if
// the DataPackage did not contained text. However, it is always better if each
// method is robust by itself
if (e.DataView.Contains(StandardDataFormats.Text))
{
var position = e.GetPosition((UIElement)sender);
var targetListView = sender as ListView;
if (targetListView.Items.Count != 0)
{
var listItem = targetListView.Items[0];
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
rowHeight = marginTop + height;
}
if (position.Y > rowHeight * targetListView.Items.Count)
{
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Add(item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
else
{
double index = position.Y / rowHeight;
var mathIndex = Math.Round(index, MidpointRounding.AwayFromZero);
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Insert(Convert.ToInt32(mathIndex), item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
}
}
Hmmm, it doesn't look like this code can actually work: e.GetPosition gives me the relative position above the ListView, so if I have a long list, scrolled down the list and hovering between the 2nd and 3rd item in view, this code will give me an index of 2 but there are many items above that out of view. Second, this code assumes that all rows have the same height, which isn't the case in my app. When I hover over items, the items move out of the way in the listview. Isn't there an event triggered when that happens? That would make a lot more sense.
– Niels
Nov 8 at 15:09
Sure, the above answer is just apply for same row height. for different row height you could use foreach to calculate the total item's row height. The theory is same as the above answer.
– Nico Zhu - MSFT
Nov 9 at 2:49
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Anyway: I have tinkered around with this code and finally got it to work to my needs:
private async void MyListView_Drop(object sender, DragEventArgs e)
{
var scrollViewer = VisualTreeHelper.GetChild(VisualTreeHelper.GetChild(MyListView, 0), 0) as ScrollViewer;
var position = e.GetPosition((ListView)sender);
var positionY = scrollViewer.VerticalOffset + position.Y;
var index = GetItemIndex(positionY, MyListView);
// do something useful with the index...
}
int GetItemIndex(double positionY, ListView targetListView)
{
var index = 0;
double height = 0;
foreach (var item in targetListView.Items)
{
height += GetRowHeight(item, targetListView);
if (height > positionY) return index;
index++;
}
return index;
}
double GetRowHeight(object listItem, ListView targetListView)
{
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
return marginTop + height;
}
Thank you for pointing me in the right direction!
Well done, you could mark your answer.
– Nico Zhu - MSFT
Nov 9 at 2:14
add a comment |
up vote
2
down vote
accepted
Anyway: I have tinkered around with this code and finally got it to work to my needs:
private async void MyListView_Drop(object sender, DragEventArgs e)
{
var scrollViewer = VisualTreeHelper.GetChild(VisualTreeHelper.GetChild(MyListView, 0), 0) as ScrollViewer;
var position = e.GetPosition((ListView)sender);
var positionY = scrollViewer.VerticalOffset + position.Y;
var index = GetItemIndex(positionY, MyListView);
// do something useful with the index...
}
int GetItemIndex(double positionY, ListView targetListView)
{
var index = 0;
double height = 0;
foreach (var item in targetListView.Items)
{
height += GetRowHeight(item, targetListView);
if (height > positionY) return index;
index++;
}
return index;
}
double GetRowHeight(object listItem, ListView targetListView)
{
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
return marginTop + height;
}
Thank you for pointing me in the right direction!
Well done, you could mark your answer.
– Nico Zhu - MSFT
Nov 9 at 2:14
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Anyway: I have tinkered around with this code and finally got it to work to my needs:
private async void MyListView_Drop(object sender, DragEventArgs e)
{
var scrollViewer = VisualTreeHelper.GetChild(VisualTreeHelper.GetChild(MyListView, 0), 0) as ScrollViewer;
var position = e.GetPosition((ListView)sender);
var positionY = scrollViewer.VerticalOffset + position.Y;
var index = GetItemIndex(positionY, MyListView);
// do something useful with the index...
}
int GetItemIndex(double positionY, ListView targetListView)
{
var index = 0;
double height = 0;
foreach (var item in targetListView.Items)
{
height += GetRowHeight(item, targetListView);
if (height > positionY) return index;
index++;
}
return index;
}
double GetRowHeight(object listItem, ListView targetListView)
{
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
return marginTop + height;
}
Thank you for pointing me in the right direction!
Anyway: I have tinkered around with this code and finally got it to work to my needs:
private async void MyListView_Drop(object sender, DragEventArgs e)
{
var scrollViewer = VisualTreeHelper.GetChild(VisualTreeHelper.GetChild(MyListView, 0), 0) as ScrollViewer;
var position = e.GetPosition((ListView)sender);
var positionY = scrollViewer.VerticalOffset + position.Y;
var index = GetItemIndex(positionY, MyListView);
// do something useful with the index...
}
int GetItemIndex(double positionY, ListView targetListView)
{
var index = 0;
double height = 0;
foreach (var item in targetListView.Items)
{
height += GetRowHeight(item, targetListView);
if (height > positionY) return index;
index++;
}
return index;
}
double GetRowHeight(object listItem, ListView targetListView)
{
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
return marginTop + height;
}
Thank you for pointing me in the right direction!
answered Nov 8 at 15:26


Niels
736916
736916
Well done, you could mark your answer.
– Nico Zhu - MSFT
Nov 9 at 2:14
add a comment |
Well done, you could mark your answer.
– Nico Zhu - MSFT
Nov 9 at 2:14
Well done, you could mark your answer.
– Nico Zhu - MSFT
Nov 9 at 2:14
Well done, you could mark your answer.
– Nico Zhu - MSFT
Nov 9 at 2:14
add a comment |
up vote
0
down vote
How do I get the position/index where I dropped my item?
Currently, Drop
event does not provide the index of your dropped item. But you could get the drop position with GetPosition
method and calculate the index of your current dropped item. I have implement it based on official drag and drop code sample. And you could use the following code directly.
private double rowHeight;
private async void TargetListView_Drop(object sender, DragEventArgs e)
{
// This test is in theory not needed as we returned DataPackageOperation.None if
// the DataPackage did not contained text. However, it is always better if each
// method is robust by itself
if (e.DataView.Contains(StandardDataFormats.Text))
{
var position = e.GetPosition((UIElement)sender);
var targetListView = sender as ListView;
if (targetListView.Items.Count != 0)
{
var listItem = targetListView.Items[0];
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
rowHeight = marginTop + height;
}
if (position.Y > rowHeight * targetListView.Items.Count)
{
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Add(item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
else
{
double index = position.Y / rowHeight;
var mathIndex = Math.Round(index, MidpointRounding.AwayFromZero);
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Insert(Convert.ToInt32(mathIndex), item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
}
}
Hmmm, it doesn't look like this code can actually work: e.GetPosition gives me the relative position above the ListView, so if I have a long list, scrolled down the list and hovering between the 2nd and 3rd item in view, this code will give me an index of 2 but there are many items above that out of view. Second, this code assumes that all rows have the same height, which isn't the case in my app. When I hover over items, the items move out of the way in the listview. Isn't there an event triggered when that happens? That would make a lot more sense.
– Niels
Nov 8 at 15:09
Sure, the above answer is just apply for same row height. for different row height you could use foreach to calculate the total item's row height. The theory is same as the above answer.
– Nico Zhu - MSFT
Nov 9 at 2:49
add a comment |
up vote
0
down vote
How do I get the position/index where I dropped my item?
Currently, Drop
event does not provide the index of your dropped item. But you could get the drop position with GetPosition
method and calculate the index of your current dropped item. I have implement it based on official drag and drop code sample. And you could use the following code directly.
private double rowHeight;
private async void TargetListView_Drop(object sender, DragEventArgs e)
{
// This test is in theory not needed as we returned DataPackageOperation.None if
// the DataPackage did not contained text. However, it is always better if each
// method is robust by itself
if (e.DataView.Contains(StandardDataFormats.Text))
{
var position = e.GetPosition((UIElement)sender);
var targetListView = sender as ListView;
if (targetListView.Items.Count != 0)
{
var listItem = targetListView.Items[0];
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
rowHeight = marginTop + height;
}
if (position.Y > rowHeight * targetListView.Items.Count)
{
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Add(item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
else
{
double index = position.Y / rowHeight;
var mathIndex = Math.Round(index, MidpointRounding.AwayFromZero);
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Insert(Convert.ToInt32(mathIndex), item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
}
}
Hmmm, it doesn't look like this code can actually work: e.GetPosition gives me the relative position above the ListView, so if I have a long list, scrolled down the list and hovering between the 2nd and 3rd item in view, this code will give me an index of 2 but there are many items above that out of view. Second, this code assumes that all rows have the same height, which isn't the case in my app. When I hover over items, the items move out of the way in the listview. Isn't there an event triggered when that happens? That would make a lot more sense.
– Niels
Nov 8 at 15:09
Sure, the above answer is just apply for same row height. for different row height you could use foreach to calculate the total item's row height. The theory is same as the above answer.
– Nico Zhu - MSFT
Nov 9 at 2:49
add a comment |
up vote
0
down vote
up vote
0
down vote
How do I get the position/index where I dropped my item?
Currently, Drop
event does not provide the index of your dropped item. But you could get the drop position with GetPosition
method and calculate the index of your current dropped item. I have implement it based on official drag and drop code sample. And you could use the following code directly.
private double rowHeight;
private async void TargetListView_Drop(object sender, DragEventArgs e)
{
// This test is in theory not needed as we returned DataPackageOperation.None if
// the DataPackage did not contained text. However, it is always better if each
// method is robust by itself
if (e.DataView.Contains(StandardDataFormats.Text))
{
var position = e.GetPosition((UIElement)sender);
var targetListView = sender as ListView;
if (targetListView.Items.Count != 0)
{
var listItem = targetListView.Items[0];
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
rowHeight = marginTop + height;
}
if (position.Y > rowHeight * targetListView.Items.Count)
{
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Add(item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
else
{
double index = position.Y / rowHeight;
var mathIndex = Math.Round(index, MidpointRounding.AwayFromZero);
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Insert(Convert.ToInt32(mathIndex), item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
}
}
How do I get the position/index where I dropped my item?
Currently, Drop
event does not provide the index of your dropped item. But you could get the drop position with GetPosition
method and calculate the index of your current dropped item. I have implement it based on official drag and drop code sample. And you could use the following code directly.
private double rowHeight;
private async void TargetListView_Drop(object sender, DragEventArgs e)
{
// This test is in theory not needed as we returned DataPackageOperation.None if
// the DataPackage did not contained text. However, it is always better if each
// method is robust by itself
if (e.DataView.Contains(StandardDataFormats.Text))
{
var position = e.GetPosition((UIElement)sender);
var targetListView = sender as ListView;
if (targetListView.Items.Count != 0)
{
var listItem = targetListView.Items[0];
var listItemContainer = targetListView.ContainerFromItem(listItem) as ListViewItem;
var height = listItemContainer.ActualHeight;
var marginTop = listItemContainer.Margin.Top;
rowHeight = marginTop + height;
}
if (position.Y > rowHeight * targetListView.Items.Count)
{
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Add(item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
else
{
double index = position.Y / rowHeight;
var mathIndex = Math.Round(index, MidpointRounding.AwayFromZero);
var def = e.GetDeferral();
var s = await e.DataView.GetTextAsync();
var items = s.Split('n');
foreach (var item in items)
{
_selection.Insert(Convert.ToInt32(mathIndex), item);
}
e.AcceptedOperation = DataPackageOperation.Copy;
def.Complete();
}
}
}
answered Nov 8 at 7:42


Nico Zhu - MSFT
8,4021321
8,4021321
Hmmm, it doesn't look like this code can actually work: e.GetPosition gives me the relative position above the ListView, so if I have a long list, scrolled down the list and hovering between the 2nd and 3rd item in view, this code will give me an index of 2 but there are many items above that out of view. Second, this code assumes that all rows have the same height, which isn't the case in my app. When I hover over items, the items move out of the way in the listview. Isn't there an event triggered when that happens? That would make a lot more sense.
– Niels
Nov 8 at 15:09
Sure, the above answer is just apply for same row height. for different row height you could use foreach to calculate the total item's row height. The theory is same as the above answer.
– Nico Zhu - MSFT
Nov 9 at 2:49
add a comment |
Hmmm, it doesn't look like this code can actually work: e.GetPosition gives me the relative position above the ListView, so if I have a long list, scrolled down the list and hovering between the 2nd and 3rd item in view, this code will give me an index of 2 but there are many items above that out of view. Second, this code assumes that all rows have the same height, which isn't the case in my app. When I hover over items, the items move out of the way in the listview. Isn't there an event triggered when that happens? That would make a lot more sense.
– Niels
Nov 8 at 15:09
Sure, the above answer is just apply for same row height. for different row height you could use foreach to calculate the total item's row height. The theory is same as the above answer.
– Nico Zhu - MSFT
Nov 9 at 2:49
Hmmm, it doesn't look like this code can actually work: e.GetPosition gives me the relative position above the ListView, so if I have a long list, scrolled down the list and hovering between the 2nd and 3rd item in view, this code will give me an index of 2 but there are many items above that out of view. Second, this code assumes that all rows have the same height, which isn't the case in my app. When I hover over items, the items move out of the way in the listview. Isn't there an event triggered when that happens? That would make a lot more sense.
– Niels
Nov 8 at 15:09
Hmmm, it doesn't look like this code can actually work: e.GetPosition gives me the relative position above the ListView, so if I have a long list, scrolled down the list and hovering between the 2nd and 3rd item in view, this code will give me an index of 2 but there are many items above that out of view. Second, this code assumes that all rows have the same height, which isn't the case in my app. When I hover over items, the items move out of the way in the listview. Isn't there an event triggered when that happens? That would make a lot more sense.
– Niels
Nov 8 at 15:09
Sure, the above answer is just apply for same row height. for different row height you could use foreach to calculate the total item's row height. The theory is same as the above answer.
– Nico Zhu - MSFT
Nov 9 at 2:49
Sure, the above answer is just apply for same row height. for different row height you could use foreach to calculate the total item's row height. The theory is same as the above answer.
– Nico Zhu - MSFT
Nov 9 at 2:49
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53195463%2fuwp-listview-drop-get-index%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ejX5X58RnIV keLCz3P