Avoiding string to be considered optional in swift
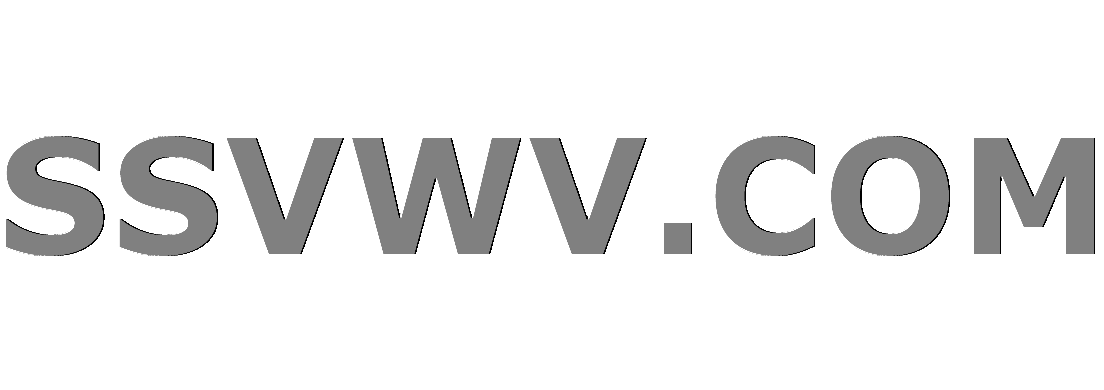
Multi tool use
I have a function where string value is defined based on value in dictionary. If value in dictionary is not found, I set it to static string. String is constant (let
):
let dict = [ 1: "a",
2: "b" ]
func x() {
let index = someFunctionReturnsInt()
let str = (nil != dict[index]) ? dict[index] : "N/A"
So string cannot possibly be nil
. However, when I try to use str
, Swift still considers it an optional. For example:
// still inside the same function
os_log("Str is %@", str)
will return a compiler error:
Value of optional type 'String?' must be unwrapped to a value of type 'String'
Thing is, I use this function quite a few times, and adding str ?? "N/A"
on every occasion makes no sense. Force-unwrap with str!
seems like an overkill: this string is not critical to the app, so I don't think aborting execution if it's missing makes any sense.
So is force-unwrap the only solution, or is there a better way to define a string as non-optional and "never going to be nil"?
swift dictionary optional
add a comment |
I have a function where string value is defined based on value in dictionary. If value in dictionary is not found, I set it to static string. String is constant (let
):
let dict = [ 1: "a",
2: "b" ]
func x() {
let index = someFunctionReturnsInt()
let str = (nil != dict[index]) ? dict[index] : "N/A"
So string cannot possibly be nil
. However, when I try to use str
, Swift still considers it an optional. For example:
// still inside the same function
os_log("Str is %@", str)
will return a compiler error:
Value of optional type 'String?' must be unwrapped to a value of type 'String'
Thing is, I use this function quite a few times, and adding str ?? "N/A"
on every occasion makes no sense. Force-unwrap with str!
seems like an overkill: this string is not critical to the app, so I don't think aborting execution if it's missing makes any sense.
So is force-unwrap the only solution, or is there a better way to define a string as non-optional and "never going to be nil"?
swift dictionary optional
1
You already know about the??
operator. What is wrong withlet str = dict[index] ?? "N/A"
?
– rob mayoff
Nov 22 '18 at 17:51
@robmayoff you are right, didn't think it would make a difference
– Kiril S.
Nov 23 '18 at 19:25
add a comment |
I have a function where string value is defined based on value in dictionary. If value in dictionary is not found, I set it to static string. String is constant (let
):
let dict = [ 1: "a",
2: "b" ]
func x() {
let index = someFunctionReturnsInt()
let str = (nil != dict[index]) ? dict[index] : "N/A"
So string cannot possibly be nil
. However, when I try to use str
, Swift still considers it an optional. For example:
// still inside the same function
os_log("Str is %@", str)
will return a compiler error:
Value of optional type 'String?' must be unwrapped to a value of type 'String'
Thing is, I use this function quite a few times, and adding str ?? "N/A"
on every occasion makes no sense. Force-unwrap with str!
seems like an overkill: this string is not critical to the app, so I don't think aborting execution if it's missing makes any sense.
So is force-unwrap the only solution, or is there a better way to define a string as non-optional and "never going to be nil"?
swift dictionary optional
I have a function where string value is defined based on value in dictionary. If value in dictionary is not found, I set it to static string. String is constant (let
):
let dict = [ 1: "a",
2: "b" ]
func x() {
let index = someFunctionReturnsInt()
let str = (nil != dict[index]) ? dict[index] : "N/A"
So string cannot possibly be nil
. However, when I try to use str
, Swift still considers it an optional. For example:
// still inside the same function
os_log("Str is %@", str)
will return a compiler error:
Value of optional type 'String?' must be unwrapped to a value of type 'String'
Thing is, I use this function quite a few times, and adding str ?? "N/A"
on every occasion makes no sense. Force-unwrap with str!
seems like an overkill: this string is not critical to the app, so I don't think aborting execution if it's missing makes any sense.
So is force-unwrap the only solution, or is there a better way to define a string as non-optional and "never going to be nil"?
swift dictionary optional
swift dictionary optional
edited Nov 22 '18 at 19:01
Dávid Pásztor
22.6k83051
22.6k83051
asked Nov 22 '18 at 17:45
Kiril S.Kiril S.
5,73752245
5,73752245
1
You already know about the??
operator. What is wrong withlet str = dict[index] ?? "N/A"
?
– rob mayoff
Nov 22 '18 at 17:51
@robmayoff you are right, didn't think it would make a difference
– Kiril S.
Nov 23 '18 at 19:25
add a comment |
1
You already know about the??
operator. What is wrong withlet str = dict[index] ?? "N/A"
?
– rob mayoff
Nov 22 '18 at 17:51
@robmayoff you are right, didn't think it would make a difference
– Kiril S.
Nov 23 '18 at 19:25
1
1
You already know about the
??
operator. What is wrong with let str = dict[index] ?? "N/A"
?– rob mayoff
Nov 22 '18 at 17:51
You already know about the
??
operator. What is wrong with let str = dict[index] ?? "N/A"
?– rob mayoff
Nov 22 '18 at 17:51
@robmayoff you are right, didn't think it would make a difference
– Kiril S.
Nov 23 '18 at 19:25
@robmayoff you are right, didn't think it would make a difference
– Kiril S.
Nov 23 '18 at 19:25
add a comment |
3 Answers
3
active
oldest
votes
The issue is that dict[index]
is still returning an optional so str
has to be an optional. There are two ways you can fix it
You can use the nil coalescing operator to use dict[index]
if its not nil otherwise use "N/A"
let str = dict[index] ?? "N/A"
Another option is to use the dictionary default value subscript
let str = dict[index, default: "N/A"]
thanks for "default value subscript" option
– Kiril S.
Nov 23 '18 at 19:26
add a comment |
You should simply use the nil coalescing operator when defining the str
itself. Checking if the value is nil, then still assigning the optional value without unwrapping makes no sense.
let str = dict[index] ?? "N/A"
Yes, this works
– fivewood
Nov 22 '18 at 18:25
add a comment |
You should use nil coalescing operator - let str = dict[index] ?? "N/A"
Instead of let str = (nil != dict[index]) ? dict[index] : "N/A"
The reason because str is still optional in your case is because dict[index]
is optional even if you are checking it to be no nil. So, swift infers the type of str to be String?
You could also force unwrap because you are already checking it to be no nil like -
let str = (nil != dict[index]) ? dict[index]! : "N/A"
But force unwrapping should be avoided whenever possible
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436045%2favoiding-string-to-be-considered-optional-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
The issue is that dict[index]
is still returning an optional so str
has to be an optional. There are two ways you can fix it
You can use the nil coalescing operator to use dict[index]
if its not nil otherwise use "N/A"
let str = dict[index] ?? "N/A"
Another option is to use the dictionary default value subscript
let str = dict[index, default: "N/A"]
thanks for "default value subscript" option
– Kiril S.
Nov 23 '18 at 19:26
add a comment |
The issue is that dict[index]
is still returning an optional so str
has to be an optional. There are two ways you can fix it
You can use the nil coalescing operator to use dict[index]
if its not nil otherwise use "N/A"
let str = dict[index] ?? "N/A"
Another option is to use the dictionary default value subscript
let str = dict[index, default: "N/A"]
thanks for "default value subscript" option
– Kiril S.
Nov 23 '18 at 19:26
add a comment |
The issue is that dict[index]
is still returning an optional so str
has to be an optional. There are two ways you can fix it
You can use the nil coalescing operator to use dict[index]
if its not nil otherwise use "N/A"
let str = dict[index] ?? "N/A"
Another option is to use the dictionary default value subscript
let str = dict[index, default: "N/A"]
The issue is that dict[index]
is still returning an optional so str
has to be an optional. There are two ways you can fix it
You can use the nil coalescing operator to use dict[index]
if its not nil otherwise use "N/A"
let str = dict[index] ?? "N/A"
Another option is to use the dictionary default value subscript
let str = dict[index, default: "N/A"]
answered Nov 22 '18 at 17:52


Craig SiemensCraig Siemens
9,43111941
9,43111941
thanks for "default value subscript" option
– Kiril S.
Nov 23 '18 at 19:26
add a comment |
thanks for "default value subscript" option
– Kiril S.
Nov 23 '18 at 19:26
thanks for "default value subscript" option
– Kiril S.
Nov 23 '18 at 19:26
thanks for "default value subscript" option
– Kiril S.
Nov 23 '18 at 19:26
add a comment |
You should simply use the nil coalescing operator when defining the str
itself. Checking if the value is nil, then still assigning the optional value without unwrapping makes no sense.
let str = dict[index] ?? "N/A"
Yes, this works
– fivewood
Nov 22 '18 at 18:25
add a comment |
You should simply use the nil coalescing operator when defining the str
itself. Checking if the value is nil, then still assigning the optional value without unwrapping makes no sense.
let str = dict[index] ?? "N/A"
Yes, this works
– fivewood
Nov 22 '18 at 18:25
add a comment |
You should simply use the nil coalescing operator when defining the str
itself. Checking if the value is nil, then still assigning the optional value without unwrapping makes no sense.
let str = dict[index] ?? "N/A"
You should simply use the nil coalescing operator when defining the str
itself. Checking if the value is nil, then still assigning the optional value without unwrapping makes no sense.
let str = dict[index] ?? "N/A"
answered Nov 22 '18 at 17:52
Dávid PásztorDávid Pásztor
22.6k83051
22.6k83051
Yes, this works
– fivewood
Nov 22 '18 at 18:25
add a comment |
Yes, this works
– fivewood
Nov 22 '18 at 18:25
Yes, this works
– fivewood
Nov 22 '18 at 18:25
Yes, this works
– fivewood
Nov 22 '18 at 18:25
add a comment |
You should use nil coalescing operator - let str = dict[index] ?? "N/A"
Instead of let str = (nil != dict[index]) ? dict[index] : "N/A"
The reason because str is still optional in your case is because dict[index]
is optional even if you are checking it to be no nil. So, swift infers the type of str to be String?
You could also force unwrap because you are already checking it to be no nil like -
let str = (nil != dict[index]) ? dict[index]! : "N/A"
But force unwrapping should be avoided whenever possible
add a comment |
You should use nil coalescing operator - let str = dict[index] ?? "N/A"
Instead of let str = (nil != dict[index]) ? dict[index] : "N/A"
The reason because str is still optional in your case is because dict[index]
is optional even if you are checking it to be no nil. So, swift infers the type of str to be String?
You could also force unwrap because you are already checking it to be no nil like -
let str = (nil != dict[index]) ? dict[index]! : "N/A"
But force unwrapping should be avoided whenever possible
add a comment |
You should use nil coalescing operator - let str = dict[index] ?? "N/A"
Instead of let str = (nil != dict[index]) ? dict[index] : "N/A"
The reason because str is still optional in your case is because dict[index]
is optional even if you are checking it to be no nil. So, swift infers the type of str to be String?
You could also force unwrap because you are already checking it to be no nil like -
let str = (nil != dict[index]) ? dict[index]! : "N/A"
But force unwrapping should be avoided whenever possible
You should use nil coalescing operator - let str = dict[index] ?? "N/A"
Instead of let str = (nil != dict[index]) ? dict[index] : "N/A"
The reason because str is still optional in your case is because dict[index]
is optional even if you are checking it to be no nil. So, swift infers the type of str to be String?
You could also force unwrap because you are already checking it to be no nil like -
let str = (nil != dict[index]) ? dict[index]! : "N/A"
But force unwrapping should be avoided whenever possible
answered Nov 22 '18 at 17:52
AakashAakash
1,378715
1,378715
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436045%2favoiding-string-to-be-considered-optional-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
NqO N6N heXeYpCUD7aJZ5RW,t 6jZw dm5m5Zw PcVqK3hFW4,DhwOx
1
You already know about the
??
operator. What is wrong withlet str = dict[index] ?? "N/A"
?– rob mayoff
Nov 22 '18 at 17:51
@robmayoff you are right, didn't think it would make a difference
– Kiril S.
Nov 23 '18 at 19:25