Do loop keeps on repeating
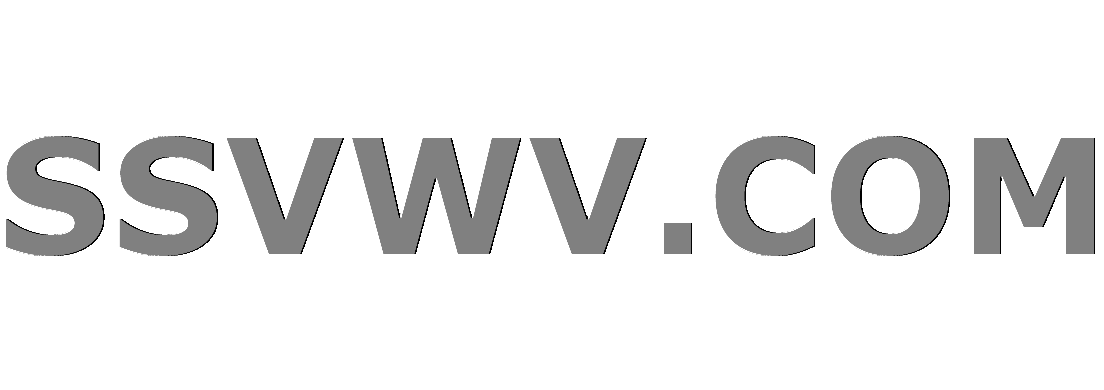
Multi tool use
So my alternative version of this code is in Java, the logic is fairly similar although in JavaScript the userinput is repeated infinitely rather than carrying until the user loses. This is my working Java code for reference:
int stop =0;
Scanner scan = new Scanner(System.in);
Random rand = new Random();
do {
int card;
int upcommingcard;
String userinput;
card= rand.nextInt(13)+1;
System.out.println("Card is "+card);
System.out.println("Higher or Lower?");
userinput = scan.next();
upcommingcard = rand.nextInt(13)+1;
if(!userinput.equalsIgnoreCase("H")&&(!userinput.equalsIgnoreCase("L"))){
System.out.println("Invalid Input ");
}
else if((userinput.equalsIgnoreCase("H")) && (upcommingcard > card)){
System.out.println("Correct!");
}
else if(userinput.equalsIgnoreCase("L") && upcommingcard < card){
System.out.println("Correct!l ");
}
else {
System.out.println("You lost it was " + upcommingcard);
stop=1;
}
}while (stop != 1);
}
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
JavaScript - Not working
var max=13;
var min=1;
var stop=0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is "+card+"... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do{
if((userinput !="H")&&(userinput !="L")){
console.log("Invalid input");
}
else if((userinput ="H")&&(upcommingcard > card)){
console.log("Correct!");
}
else if((userinput ="L")&&(upcommingcard < card)){
console.log("Correct!");
}
else{
console.log("You lost, it was "+ upcommingcard);
stop=1;
}
}
while(stop !=1);
Just to mention also that it registers that the user's input is correct although it fails to continue and just keeps on spitting out the same output until the browser crashes.
EDIT: Thanks for the responses! the loop works perfectly now, my only issue is that the logic is a bit flawed since sometimes I Input 'L' for 8 and upcoming int is 10.. Dispite this I get the Incorrect response.
javascript
|
show 3 more comments
So my alternative version of this code is in Java, the logic is fairly similar although in JavaScript the userinput is repeated infinitely rather than carrying until the user loses. This is my working Java code for reference:
int stop =0;
Scanner scan = new Scanner(System.in);
Random rand = new Random();
do {
int card;
int upcommingcard;
String userinput;
card= rand.nextInt(13)+1;
System.out.println("Card is "+card);
System.out.println("Higher or Lower?");
userinput = scan.next();
upcommingcard = rand.nextInt(13)+1;
if(!userinput.equalsIgnoreCase("H")&&(!userinput.equalsIgnoreCase("L"))){
System.out.println("Invalid Input ");
}
else if((userinput.equalsIgnoreCase("H")) && (upcommingcard > card)){
System.out.println("Correct!");
}
else if(userinput.equalsIgnoreCase("L") && upcommingcard < card){
System.out.println("Correct!l ");
}
else {
System.out.println("You lost it was " + upcommingcard);
stop=1;
}
}while (stop != 1);
}
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
JavaScript - Not working
var max=13;
var min=1;
var stop=0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is "+card+"... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do{
if((userinput !="H")&&(userinput !="L")){
console.log("Invalid input");
}
else if((userinput ="H")&&(upcommingcard > card)){
console.log("Correct!");
}
else if((userinput ="L")&&(upcommingcard < card)){
console.log("Correct!");
}
else{
console.log("You lost, it was "+ upcommingcard);
stop=1;
}
}
while(stop !=1);
Just to mention also that it registers that the user's input is correct although it fails to continue and just keeps on spitting out the same output until the browser crashes.
EDIT: Thanks for the responses! the loop works perfectly now, my only issue is that the logic is a bit flawed since sometimes I Input 'L' for 8 and upcoming int is 10.. Dispite this I get the Incorrect response.
javascript
What is the question?
– cmprogram
Nov 22 '18 at 17:44
2
You're only callingprompt
once before thedo...while
. Put it a the top of the do while.
– pmkro
Nov 22 '18 at 17:47
Essentially My java script version doesn't work and keeps on outputting the same answer rather than continue and eventually crashes. Where as in my java version it stops when the user loses.
– Thomas Hall
Nov 22 '18 at 17:47
2
I see you are using=
instead of==
for your "equal to" comparisons. At the moment you are reassigning the values instead of comparing them.
– Christheoreo
Nov 22 '18 at 17:49
The Java version has the "logic"/"function" in the loop. Why did you partly move it out of the loop in the JavaScript part? And there's a huge difference between=
and==
/===
– Andreas
Nov 22 '18 at 17:49
|
show 3 more comments
So my alternative version of this code is in Java, the logic is fairly similar although in JavaScript the userinput is repeated infinitely rather than carrying until the user loses. This is my working Java code for reference:
int stop =0;
Scanner scan = new Scanner(System.in);
Random rand = new Random();
do {
int card;
int upcommingcard;
String userinput;
card= rand.nextInt(13)+1;
System.out.println("Card is "+card);
System.out.println("Higher or Lower?");
userinput = scan.next();
upcommingcard = rand.nextInt(13)+1;
if(!userinput.equalsIgnoreCase("H")&&(!userinput.equalsIgnoreCase("L"))){
System.out.println("Invalid Input ");
}
else if((userinput.equalsIgnoreCase("H")) && (upcommingcard > card)){
System.out.println("Correct!");
}
else if(userinput.equalsIgnoreCase("L") && upcommingcard < card){
System.out.println("Correct!l ");
}
else {
System.out.println("You lost it was " + upcommingcard);
stop=1;
}
}while (stop != 1);
}
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
JavaScript - Not working
var max=13;
var min=1;
var stop=0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is "+card+"... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do{
if((userinput !="H")&&(userinput !="L")){
console.log("Invalid input");
}
else if((userinput ="H")&&(upcommingcard > card)){
console.log("Correct!");
}
else if((userinput ="L")&&(upcommingcard < card)){
console.log("Correct!");
}
else{
console.log("You lost, it was "+ upcommingcard);
stop=1;
}
}
while(stop !=1);
Just to mention also that it registers that the user's input is correct although it fails to continue and just keeps on spitting out the same output until the browser crashes.
EDIT: Thanks for the responses! the loop works perfectly now, my only issue is that the logic is a bit flawed since sometimes I Input 'L' for 8 and upcoming int is 10.. Dispite this I get the Incorrect response.
javascript
So my alternative version of this code is in Java, the logic is fairly similar although in JavaScript the userinput is repeated infinitely rather than carrying until the user loses. This is my working Java code for reference:
int stop =0;
Scanner scan = new Scanner(System.in);
Random rand = new Random();
do {
int card;
int upcommingcard;
String userinput;
card= rand.nextInt(13)+1;
System.out.println("Card is "+card);
System.out.println("Higher or Lower?");
userinput = scan.next();
upcommingcard = rand.nextInt(13)+1;
if(!userinput.equalsIgnoreCase("H")&&(!userinput.equalsIgnoreCase("L"))){
System.out.println("Invalid Input ");
}
else if((userinput.equalsIgnoreCase("H")) && (upcommingcard > card)){
System.out.println("Correct!");
}
else if(userinput.equalsIgnoreCase("L") && upcommingcard < card){
System.out.println("Correct!l ");
}
else {
System.out.println("You lost it was " + upcommingcard);
stop=1;
}
}while (stop != 1);
}
++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++++
JavaScript - Not working
var max=13;
var min=1;
var stop=0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is "+card+"... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do{
if((userinput !="H")&&(userinput !="L")){
console.log("Invalid input");
}
else if((userinput ="H")&&(upcommingcard > card)){
console.log("Correct!");
}
else if((userinput ="L")&&(upcommingcard < card)){
console.log("Correct!");
}
else{
console.log("You lost, it was "+ upcommingcard);
stop=1;
}
}
while(stop !=1);
Just to mention also that it registers that the user's input is correct although it fails to continue and just keeps on spitting out the same output until the browser crashes.
EDIT: Thanks for the responses! the loop works perfectly now, my only issue is that the logic is a bit flawed since sometimes I Input 'L' for 8 and upcoming int is 10.. Dispite this I get the Incorrect response.
javascript
javascript
edited Nov 22 '18 at 18:02
Thomas Hall
asked Nov 22 '18 at 17:43


Thomas HallThomas Hall
35
35
What is the question?
– cmprogram
Nov 22 '18 at 17:44
2
You're only callingprompt
once before thedo...while
. Put it a the top of the do while.
– pmkro
Nov 22 '18 at 17:47
Essentially My java script version doesn't work and keeps on outputting the same answer rather than continue and eventually crashes. Where as in my java version it stops when the user loses.
– Thomas Hall
Nov 22 '18 at 17:47
2
I see you are using=
instead of==
for your "equal to" comparisons. At the moment you are reassigning the values instead of comparing them.
– Christheoreo
Nov 22 '18 at 17:49
The Java version has the "logic"/"function" in the loop. Why did you partly move it out of the loop in the JavaScript part? And there's a huge difference between=
and==
/===
– Andreas
Nov 22 '18 at 17:49
|
show 3 more comments
What is the question?
– cmprogram
Nov 22 '18 at 17:44
2
You're only callingprompt
once before thedo...while
. Put it a the top of the do while.
– pmkro
Nov 22 '18 at 17:47
Essentially My java script version doesn't work and keeps on outputting the same answer rather than continue and eventually crashes. Where as in my java version it stops when the user loses.
– Thomas Hall
Nov 22 '18 at 17:47
2
I see you are using=
instead of==
for your "equal to" comparisons. At the moment you are reassigning the values instead of comparing them.
– Christheoreo
Nov 22 '18 at 17:49
The Java version has the "logic"/"function" in the loop. Why did you partly move it out of the loop in the JavaScript part? And there's a huge difference between=
and==
/===
– Andreas
Nov 22 '18 at 17:49
What is the question?
– cmprogram
Nov 22 '18 at 17:44
What is the question?
– cmprogram
Nov 22 '18 at 17:44
2
2
You're only calling
prompt
once before the do...while
. Put it a the top of the do while.– pmkro
Nov 22 '18 at 17:47
You're only calling
prompt
once before the do...while
. Put it a the top of the do while.– pmkro
Nov 22 '18 at 17:47
Essentially My java script version doesn't work and keeps on outputting the same answer rather than continue and eventually crashes. Where as in my java version it stops when the user loses.
– Thomas Hall
Nov 22 '18 at 17:47
Essentially My java script version doesn't work and keeps on outputting the same answer rather than continue and eventually crashes. Where as in my java version it stops when the user loses.
– Thomas Hall
Nov 22 '18 at 17:47
2
2
I see you are using
=
instead of ==
for your "equal to" comparisons. At the moment you are reassigning the values instead of comparing them.– Christheoreo
Nov 22 '18 at 17:49
I see you are using
=
instead of ==
for your "equal to" comparisons. At the moment you are reassigning the values instead of comparing them.– Christheoreo
Nov 22 '18 at 17:49
The Java version has the "logic"/"function" in the loop. Why did you partly move it out of the loop in the JavaScript part? And there's a huge difference between
=
and ==
/===
– Andreas
Nov 22 '18 at 17:49
The Java version has the "logic"/"function" in the loop. Why did you partly move it out of the loop in the JavaScript part? And there's a huge difference between
=
and ==
/===
– Andreas
Nov 22 '18 at 17:49
|
show 3 more comments
2 Answers
2
active
oldest
votes
It's not that your console isn't updating, it's that you never exit your loop if the input is incorrect, and you never offer them the option to try again.
Thus if they are incorrect, the loop will never end, the console won't be updated, and they can't retry.
I would recommend changing the code to the following, to alert the user to try again.
var max = 13;
var min = 1;
var stop = 0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do {
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input!");
userinput = prompt("Card is " + card + "... Higher or lower?");
} else if ((userinput == "H") && (upcommingcard > card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Here I thought the game is supposed to go on until the user makes a wrong guess.
– ionizer
Nov 22 '18 at 17:58
@ionizer That'd certainly be a way to improve it, and increment a score, but since OP didn't include a score I didn't want to alter their intention too much.
– cmprogram
Nov 22 '18 at 18:00
Ahh thanks for your response! not what I was quite looking for although was beneficial towards me fixing my issue ! I would upvote if I could although my rep stops me until I hit 15.
– Thomas Hall
Nov 22 '18 at 18:04
Regardless, it does not change the fact that theif
comparison is invalid and will endlessly repeat if thatstop = 1
is removed from the guess checks.
– ionizer
Nov 22 '18 at 18:16
add a comment |
There are some points I want to make on this:
- Your Java and Javascript code logic differs. You had the variables and input reads inside
do while
in Java but outside in Javascript. - As your
prompt
right now is outside the loop, it will keep having the same input value everytime and not asking for another one, and will carry on until it's a wrong guess, or forever if it's an invalid input. And the next point worsens your problem: - Your
if
comparison operators are invalid. What you did, as mentioned in the comments, is a data assignment touserinput
and will always return correct
That being said, I corrected it below while adding alert popups instead of console.log
only:
var stop = 0;
do {
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input");
stop = 1; //Currently stopping if having invalid input, you can remove this later
} else if ((userinput == "H") && (upcommingcard > card)) {
//Note the '==' above, and also the next one for comparing equal values
console.log("Correct!");
alert("Correct");
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Now, do compare the JS snippet above with your working Java code you've posted. If you compare again with your JS code, you should be able see what I meant by having different logic.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436025%2fdo-loop-keeps-on-repeating%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
It's not that your console isn't updating, it's that you never exit your loop if the input is incorrect, and you never offer them the option to try again.
Thus if they are incorrect, the loop will never end, the console won't be updated, and they can't retry.
I would recommend changing the code to the following, to alert the user to try again.
var max = 13;
var min = 1;
var stop = 0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do {
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input!");
userinput = prompt("Card is " + card + "... Higher or lower?");
} else if ((userinput == "H") && (upcommingcard > card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Here I thought the game is supposed to go on until the user makes a wrong guess.
– ionizer
Nov 22 '18 at 17:58
@ionizer That'd certainly be a way to improve it, and increment a score, but since OP didn't include a score I didn't want to alter their intention too much.
– cmprogram
Nov 22 '18 at 18:00
Ahh thanks for your response! not what I was quite looking for although was beneficial towards me fixing my issue ! I would upvote if I could although my rep stops me until I hit 15.
– Thomas Hall
Nov 22 '18 at 18:04
Regardless, it does not change the fact that theif
comparison is invalid and will endlessly repeat if thatstop = 1
is removed from the guess checks.
– ionizer
Nov 22 '18 at 18:16
add a comment |
It's not that your console isn't updating, it's that you never exit your loop if the input is incorrect, and you never offer them the option to try again.
Thus if they are incorrect, the loop will never end, the console won't be updated, and they can't retry.
I would recommend changing the code to the following, to alert the user to try again.
var max = 13;
var min = 1;
var stop = 0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do {
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input!");
userinput = prompt("Card is " + card + "... Higher or lower?");
} else if ((userinput == "H") && (upcommingcard > card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Here I thought the game is supposed to go on until the user makes a wrong guess.
– ionizer
Nov 22 '18 at 17:58
@ionizer That'd certainly be a way to improve it, and increment a score, but since OP didn't include a score I didn't want to alter their intention too much.
– cmprogram
Nov 22 '18 at 18:00
Ahh thanks for your response! not what I was quite looking for although was beneficial towards me fixing my issue ! I would upvote if I could although my rep stops me until I hit 15.
– Thomas Hall
Nov 22 '18 at 18:04
Regardless, it does not change the fact that theif
comparison is invalid and will endlessly repeat if thatstop = 1
is removed from the guess checks.
– ionizer
Nov 22 '18 at 18:16
add a comment |
It's not that your console isn't updating, it's that you never exit your loop if the input is incorrect, and you never offer them the option to try again.
Thus if they are incorrect, the loop will never end, the console won't be updated, and they can't retry.
I would recommend changing the code to the following, to alert the user to try again.
var max = 13;
var min = 1;
var stop = 0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do {
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input!");
userinput = prompt("Card is " + card + "... Higher or lower?");
} else if ((userinput == "H") && (upcommingcard > card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
It's not that your console isn't updating, it's that you never exit your loop if the input is incorrect, and you never offer them the option to try again.
Thus if they are incorrect, the loop will never end, the console won't be updated, and they can't retry.
I would recommend changing the code to the following, to alert the user to try again.
var max = 13;
var min = 1;
var stop = 0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do {
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input!");
userinput = prompt("Card is " + card + "... Higher or lower?");
} else if ((userinput == "H") && (upcommingcard > card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
var max = 13;
var min = 1;
var stop = 0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do {
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input!");
userinput = prompt("Card is " + card + "... Higher or lower?");
} else if ((userinput == "H") && (upcommingcard > card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
var max = 13;
var min = 1;
var stop = 0;
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
do {
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input!");
userinput = prompt("Card is " + card + "... Higher or lower?");
} else if ((userinput == "H") && (upcommingcard > card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
stop = 1;
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
edited Nov 23 '18 at 9:29
answered Nov 22 '18 at 17:55
cmprogramcmprogram
1,289720
1,289720
Here I thought the game is supposed to go on until the user makes a wrong guess.
– ionizer
Nov 22 '18 at 17:58
@ionizer That'd certainly be a way to improve it, and increment a score, but since OP didn't include a score I didn't want to alter their intention too much.
– cmprogram
Nov 22 '18 at 18:00
Ahh thanks for your response! not what I was quite looking for although was beneficial towards me fixing my issue ! I would upvote if I could although my rep stops me until I hit 15.
– Thomas Hall
Nov 22 '18 at 18:04
Regardless, it does not change the fact that theif
comparison is invalid and will endlessly repeat if thatstop = 1
is removed from the guess checks.
– ionizer
Nov 22 '18 at 18:16
add a comment |
Here I thought the game is supposed to go on until the user makes a wrong guess.
– ionizer
Nov 22 '18 at 17:58
@ionizer That'd certainly be a way to improve it, and increment a score, but since OP didn't include a score I didn't want to alter their intention too much.
– cmprogram
Nov 22 '18 at 18:00
Ahh thanks for your response! not what I was quite looking for although was beneficial towards me fixing my issue ! I would upvote if I could although my rep stops me until I hit 15.
– Thomas Hall
Nov 22 '18 at 18:04
Regardless, it does not change the fact that theif
comparison is invalid and will endlessly repeat if thatstop = 1
is removed from the guess checks.
– ionizer
Nov 22 '18 at 18:16
Here I thought the game is supposed to go on until the user makes a wrong guess.
– ionizer
Nov 22 '18 at 17:58
Here I thought the game is supposed to go on until the user makes a wrong guess.
– ionizer
Nov 22 '18 at 17:58
@ionizer That'd certainly be a way to improve it, and increment a score, but since OP didn't include a score I didn't want to alter their intention too much.
– cmprogram
Nov 22 '18 at 18:00
@ionizer That'd certainly be a way to improve it, and increment a score, but since OP didn't include a score I didn't want to alter their intention too much.
– cmprogram
Nov 22 '18 at 18:00
Ahh thanks for your response! not what I was quite looking for although was beneficial towards me fixing my issue ! I would upvote if I could although my rep stops me until I hit 15.
– Thomas Hall
Nov 22 '18 at 18:04
Ahh thanks for your response! not what I was quite looking for although was beneficial towards me fixing my issue ! I would upvote if I could although my rep stops me until I hit 15.
– Thomas Hall
Nov 22 '18 at 18:04
Regardless, it does not change the fact that the
if
comparison is invalid and will endlessly repeat if that stop = 1
is removed from the guess checks.– ionizer
Nov 22 '18 at 18:16
Regardless, it does not change the fact that the
if
comparison is invalid and will endlessly repeat if that stop = 1
is removed from the guess checks.– ionizer
Nov 22 '18 at 18:16
add a comment |
There are some points I want to make on this:
- Your Java and Javascript code logic differs. You had the variables and input reads inside
do while
in Java but outside in Javascript. - As your
prompt
right now is outside the loop, it will keep having the same input value everytime and not asking for another one, and will carry on until it's a wrong guess, or forever if it's an invalid input. And the next point worsens your problem: - Your
if
comparison operators are invalid. What you did, as mentioned in the comments, is a data assignment touserinput
and will always return correct
That being said, I corrected it below while adding alert popups instead of console.log
only:
var stop = 0;
do {
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input");
stop = 1; //Currently stopping if having invalid input, you can remove this later
} else if ((userinput == "H") && (upcommingcard > card)) {
//Note the '==' above, and also the next one for comparing equal values
console.log("Correct!");
alert("Correct");
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Now, do compare the JS snippet above with your working Java code you've posted. If you compare again with your JS code, you should be able see what I meant by having different logic.
add a comment |
There are some points I want to make on this:
- Your Java and Javascript code logic differs. You had the variables and input reads inside
do while
in Java but outside in Javascript. - As your
prompt
right now is outside the loop, it will keep having the same input value everytime and not asking for another one, and will carry on until it's a wrong guess, or forever if it's an invalid input. And the next point worsens your problem: - Your
if
comparison operators are invalid. What you did, as mentioned in the comments, is a data assignment touserinput
and will always return correct
That being said, I corrected it below while adding alert popups instead of console.log
only:
var stop = 0;
do {
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input");
stop = 1; //Currently stopping if having invalid input, you can remove this later
} else if ((userinput == "H") && (upcommingcard > card)) {
//Note the '==' above, and also the next one for comparing equal values
console.log("Correct!");
alert("Correct");
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Now, do compare the JS snippet above with your working Java code you've posted. If you compare again with your JS code, you should be able see what I meant by having different logic.
add a comment |
There are some points I want to make on this:
- Your Java and Javascript code logic differs. You had the variables and input reads inside
do while
in Java but outside in Javascript. - As your
prompt
right now is outside the loop, it will keep having the same input value everytime and not asking for another one, and will carry on until it's a wrong guess, or forever if it's an invalid input. And the next point worsens your problem: - Your
if
comparison operators are invalid. What you did, as mentioned in the comments, is a data assignment touserinput
and will always return correct
That being said, I corrected it below while adding alert popups instead of console.log
only:
var stop = 0;
do {
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input");
stop = 1; //Currently stopping if having invalid input, you can remove this later
} else if ((userinput == "H") && (upcommingcard > card)) {
//Note the '==' above, and also the next one for comparing equal values
console.log("Correct!");
alert("Correct");
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Now, do compare the JS snippet above with your working Java code you've posted. If you compare again with your JS code, you should be able see what I meant by having different logic.
There are some points I want to make on this:
- Your Java and Javascript code logic differs. You had the variables and input reads inside
do while
in Java but outside in Javascript. - As your
prompt
right now is outside the loop, it will keep having the same input value everytime and not asking for another one, and will carry on until it's a wrong guess, or forever if it's an invalid input. And the next point worsens your problem: - Your
if
comparison operators are invalid. What you did, as mentioned in the comments, is a data assignment touserinput
and will always return correct
That being said, I corrected it below while adding alert popups instead of console.log
only:
var stop = 0;
do {
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input");
stop = 1; //Currently stopping if having invalid input, you can remove this later
} else if ((userinput == "H") && (upcommingcard > card)) {
//Note the '==' above, and also the next one for comparing equal values
console.log("Correct!");
alert("Correct");
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
Now, do compare the JS snippet above with your working Java code you've posted. If you compare again with your JS code, you should be able see what I meant by having different logic.
var stop = 0;
do {
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input");
stop = 1; //Currently stopping if having invalid input, you can remove this later
} else if ((userinput == "H") && (upcommingcard > card)) {
//Note the '==' above, and also the next one for comparing equal values
console.log("Correct!");
alert("Correct");
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
var stop = 0;
do {
var card = Math.floor((Math.random() * (13 - 1) + 1));
var userinput = prompt("Card is " + card + "... Higher or lower?");
var upcommingcard = Math.floor((Math.random() * (13 - 1) + 1));
if ((userinput != "H") && (userinput != "L")) {
console.log("Invalid input");
alert("Invalid input");
stop = 1; //Currently stopping if having invalid input, you can remove this later
} else if ((userinput == "H") && (upcommingcard > card)) {
//Note the '==' above, and also the next one for comparing equal values
console.log("Correct!");
alert("Correct");
} else if ((userinput == "L") && (upcommingcard < card)) {
console.log("Correct!");
alert("Correct!");
} else {
console.log("You lost, it was " + upcommingcard);
alert("You lost, it was " + upcommingcard);
stop = 1;
}
}
while (stop != 1);
edited Nov 23 '18 at 10:26
answered Nov 22 '18 at 18:10
ionizerionizer
576216
576216
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436025%2fdo-loop-keeps-on-repeating%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8Aoa,OD d 4xdmVyPNnzU1Eko2WSynbs,5rWC7rZB8Vqe2LJA2v2IU1qrVi9JU2XYK49T xz1FPP1sRL LhLAEgaQQQ
What is the question?
– cmprogram
Nov 22 '18 at 17:44
2
You're only calling
prompt
once before thedo...while
. Put it a the top of the do while.– pmkro
Nov 22 '18 at 17:47
Essentially My java script version doesn't work and keeps on outputting the same answer rather than continue and eventually crashes. Where as in my java version it stops when the user loses.
– Thomas Hall
Nov 22 '18 at 17:47
2
I see you are using
=
instead of==
for your "equal to" comparisons. At the moment you are reassigning the values instead of comparing them.– Christheoreo
Nov 22 '18 at 17:49
The Java version has the "logic"/"function" in the loop. Why did you partly move it out of the loop in the JavaScript part? And there's a huge difference between
=
and==
/===
– Andreas
Nov 22 '18 at 17:49