Error: float() argument must be a string or a number, not 'Polygon' when trying to find points in polygons
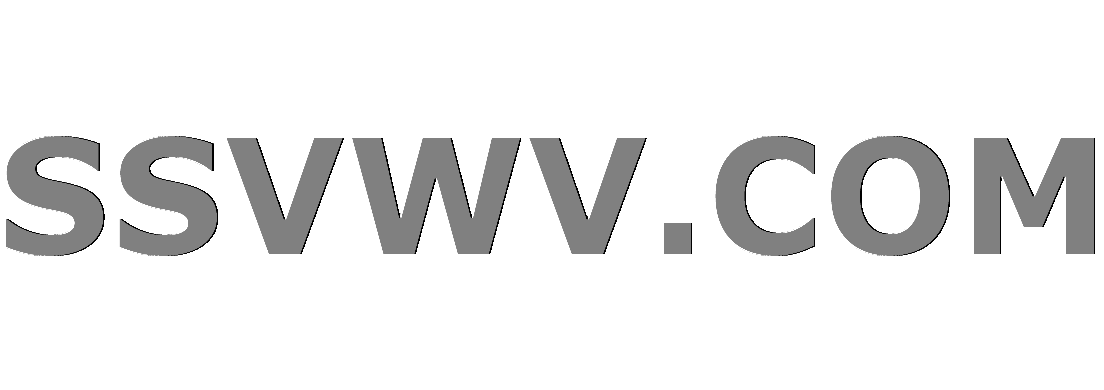
Multi tool use
I have a list of named polygons:
import pandas as pd
import geopandas as gp
from shapely.geometry import Polygon
from shapely.geometry import Point
import matplotlib.path as mpltPath
df = gp.GeoDataFrame([['a', Polygon([(1, 0), (1, 1), (2,2), (1,2)])],
['b', Polygon([(1, 1), (2,2), (3,1)])]],
columns = ['name','geometry'])
df = gp.GeoDataFrame(df, geometry = 'geometry')
and a list of points:
points = gp.GeoDataFrame( [['box', Point(1.5, 1.75)],
['cone', Point(3.0,2.0)],
['triangle', Point(2.5,1.25)]],
columns=['id', 'geometry'],
geometry='geometry')
I am trying to find out what points are in what polygons and add a column to the point dataframe with 'True' or 'False'
I've previously been shown the methods here which show some ways of quickly doing this and have got the script:
point = points['geometry']
path = mpltPath.Path(df['geometry'])
points['inside'] = path.contains_points(point)
but I get the error: float() argument must be a string or a number, not 'Polygon'
How do I fix this?
Alternatively I have been trying this method:
points['inside'] =
for geo1 in df['geometry']:
for geo2 in points['geometry']:
if geo1.contains(geo2):
points['inside'].append('True')
however here I also get an error: Length of values des not match length of index
Any help with either of these would be greatly appreciated!
python python-3.x pandas matplotlib
add a comment |
I have a list of named polygons:
import pandas as pd
import geopandas as gp
from shapely.geometry import Polygon
from shapely.geometry import Point
import matplotlib.path as mpltPath
df = gp.GeoDataFrame([['a', Polygon([(1, 0), (1, 1), (2,2), (1,2)])],
['b', Polygon([(1, 1), (2,2), (3,1)])]],
columns = ['name','geometry'])
df = gp.GeoDataFrame(df, geometry = 'geometry')
and a list of points:
points = gp.GeoDataFrame( [['box', Point(1.5, 1.75)],
['cone', Point(3.0,2.0)],
['triangle', Point(2.5,1.25)]],
columns=['id', 'geometry'],
geometry='geometry')
I am trying to find out what points are in what polygons and add a column to the point dataframe with 'True' or 'False'
I've previously been shown the methods here which show some ways of quickly doing this and have got the script:
point = points['geometry']
path = mpltPath.Path(df['geometry'])
points['inside'] = path.contains_points(point)
but I get the error: float() argument must be a string or a number, not 'Polygon'
How do I fix this?
Alternatively I have been trying this method:
points['inside'] =
for geo1 in df['geometry']:
for geo2 in points['geometry']:
if geo1.contains(geo2):
points['inside'].append('True')
however here I also get an error: Length of values des not match length of index
Any help with either of these would be greatly appreciated!
python python-3.x pandas matplotlib
add a comment |
I have a list of named polygons:
import pandas as pd
import geopandas as gp
from shapely.geometry import Polygon
from shapely.geometry import Point
import matplotlib.path as mpltPath
df = gp.GeoDataFrame([['a', Polygon([(1, 0), (1, 1), (2,2), (1,2)])],
['b', Polygon([(1, 1), (2,2), (3,1)])]],
columns = ['name','geometry'])
df = gp.GeoDataFrame(df, geometry = 'geometry')
and a list of points:
points = gp.GeoDataFrame( [['box', Point(1.5, 1.75)],
['cone', Point(3.0,2.0)],
['triangle', Point(2.5,1.25)]],
columns=['id', 'geometry'],
geometry='geometry')
I am trying to find out what points are in what polygons and add a column to the point dataframe with 'True' or 'False'
I've previously been shown the methods here which show some ways of quickly doing this and have got the script:
point = points['geometry']
path = mpltPath.Path(df['geometry'])
points['inside'] = path.contains_points(point)
but I get the error: float() argument must be a string or a number, not 'Polygon'
How do I fix this?
Alternatively I have been trying this method:
points['inside'] =
for geo1 in df['geometry']:
for geo2 in points['geometry']:
if geo1.contains(geo2):
points['inside'].append('True')
however here I also get an error: Length of values des not match length of index
Any help with either of these would be greatly appreciated!
python python-3.x pandas matplotlib
I have a list of named polygons:
import pandas as pd
import geopandas as gp
from shapely.geometry import Polygon
from shapely.geometry import Point
import matplotlib.path as mpltPath
df = gp.GeoDataFrame([['a', Polygon([(1, 0), (1, 1), (2,2), (1,2)])],
['b', Polygon([(1, 1), (2,2), (3,1)])]],
columns = ['name','geometry'])
df = gp.GeoDataFrame(df, geometry = 'geometry')
and a list of points:
points = gp.GeoDataFrame( [['box', Point(1.5, 1.75)],
['cone', Point(3.0,2.0)],
['triangle', Point(2.5,1.25)]],
columns=['id', 'geometry'],
geometry='geometry')
I am trying to find out what points are in what polygons and add a column to the point dataframe with 'True' or 'False'
I've previously been shown the methods here which show some ways of quickly doing this and have got the script:
point = points['geometry']
path = mpltPath.Path(df['geometry'])
points['inside'] = path.contains_points(point)
but I get the error: float() argument must be a string or a number, not 'Polygon'
How do I fix this?
Alternatively I have been trying this method:
points['inside'] =
for geo1 in df['geometry']:
for geo2 in points['geometry']:
if geo1.contains(geo2):
points['inside'].append('True')
however here I also get an error: Length of values des not match length of index
Any help with either of these would be greatly appreciated!
python python-3.x pandas matplotlib
python python-3.x pandas matplotlib
asked Nov 21 '18 at 14:17
tom91tom91
16611
16611
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I didn't get the "true/false column", but this should do the job:
points.apply(lambda row: (row['id'], list(map(lambda e: e[0], list(filter(lambda p: p[1].contains(row['geometry']), df.values))))), axis=1)
For each point you get the polygons that contains it, output:
0 (box, [a])
1 (cone, )
2 (triangle, [b])
dtype: object
Thank you for this. The 'True, False column' would basically be a column that is added to the points dataframe that would have true next to a point if it fell within either polygon a or b. This is because in the actual data set I have 1000s of points to check within 100s of polygons and don't want to be adding a column manually
– tom91
Nov 21 '18 at 15:37
1
Oh, got it. Then this:points['inside'] = points.geometry.map(lambda x: True if reduce(lambda a,b: a+b, list(map(lambda p: p.contains(x), df.geometry.values))) else False)
– Federico Pucci
Nov 21 '18 at 15:49
YES! Thats got it, thank you so much!
– tom91
Nov 21 '18 at 16:07
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414065%2ferror-float-argument-must-be-a-string-or-a-number-not-polygon-when-trying%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I didn't get the "true/false column", but this should do the job:
points.apply(lambda row: (row['id'], list(map(lambda e: e[0], list(filter(lambda p: p[1].contains(row['geometry']), df.values))))), axis=1)
For each point you get the polygons that contains it, output:
0 (box, [a])
1 (cone, )
2 (triangle, [b])
dtype: object
Thank you for this. The 'True, False column' would basically be a column that is added to the points dataframe that would have true next to a point if it fell within either polygon a or b. This is because in the actual data set I have 1000s of points to check within 100s of polygons and don't want to be adding a column manually
– tom91
Nov 21 '18 at 15:37
1
Oh, got it. Then this:points['inside'] = points.geometry.map(lambda x: True if reduce(lambda a,b: a+b, list(map(lambda p: p.contains(x), df.geometry.values))) else False)
– Federico Pucci
Nov 21 '18 at 15:49
YES! Thats got it, thank you so much!
– tom91
Nov 21 '18 at 16:07
add a comment |
I didn't get the "true/false column", but this should do the job:
points.apply(lambda row: (row['id'], list(map(lambda e: e[0], list(filter(lambda p: p[1].contains(row['geometry']), df.values))))), axis=1)
For each point you get the polygons that contains it, output:
0 (box, [a])
1 (cone, )
2 (triangle, [b])
dtype: object
Thank you for this. The 'True, False column' would basically be a column that is added to the points dataframe that would have true next to a point if it fell within either polygon a or b. This is because in the actual data set I have 1000s of points to check within 100s of polygons and don't want to be adding a column manually
– tom91
Nov 21 '18 at 15:37
1
Oh, got it. Then this:points['inside'] = points.geometry.map(lambda x: True if reduce(lambda a,b: a+b, list(map(lambda p: p.contains(x), df.geometry.values))) else False)
– Federico Pucci
Nov 21 '18 at 15:49
YES! Thats got it, thank you so much!
– tom91
Nov 21 '18 at 16:07
add a comment |
I didn't get the "true/false column", but this should do the job:
points.apply(lambda row: (row['id'], list(map(lambda e: e[0], list(filter(lambda p: p[1].contains(row['geometry']), df.values))))), axis=1)
For each point you get the polygons that contains it, output:
0 (box, [a])
1 (cone, )
2 (triangle, [b])
dtype: object
I didn't get the "true/false column", but this should do the job:
points.apply(lambda row: (row['id'], list(map(lambda e: e[0], list(filter(lambda p: p[1].contains(row['geometry']), df.values))))), axis=1)
For each point you get the polygons that contains it, output:
0 (box, [a])
1 (cone, )
2 (triangle, [b])
dtype: object
answered Nov 21 '18 at 15:18
Federico PucciFederico Pucci
513
513
Thank you for this. The 'True, False column' would basically be a column that is added to the points dataframe that would have true next to a point if it fell within either polygon a or b. This is because in the actual data set I have 1000s of points to check within 100s of polygons and don't want to be adding a column manually
– tom91
Nov 21 '18 at 15:37
1
Oh, got it. Then this:points['inside'] = points.geometry.map(lambda x: True if reduce(lambda a,b: a+b, list(map(lambda p: p.contains(x), df.geometry.values))) else False)
– Federico Pucci
Nov 21 '18 at 15:49
YES! Thats got it, thank you so much!
– tom91
Nov 21 '18 at 16:07
add a comment |
Thank you for this. The 'True, False column' would basically be a column that is added to the points dataframe that would have true next to a point if it fell within either polygon a or b. This is because in the actual data set I have 1000s of points to check within 100s of polygons and don't want to be adding a column manually
– tom91
Nov 21 '18 at 15:37
1
Oh, got it. Then this:points['inside'] = points.geometry.map(lambda x: True if reduce(lambda a,b: a+b, list(map(lambda p: p.contains(x), df.geometry.values))) else False)
– Federico Pucci
Nov 21 '18 at 15:49
YES! Thats got it, thank you so much!
– tom91
Nov 21 '18 at 16:07
Thank you for this. The 'True, False column' would basically be a column that is added to the points dataframe that would have true next to a point if it fell within either polygon a or b. This is because in the actual data set I have 1000s of points to check within 100s of polygons and don't want to be adding a column manually
– tom91
Nov 21 '18 at 15:37
Thank you for this. The 'True, False column' would basically be a column that is added to the points dataframe that would have true next to a point if it fell within either polygon a or b. This is because in the actual data set I have 1000s of points to check within 100s of polygons and don't want to be adding a column manually
– tom91
Nov 21 '18 at 15:37
1
1
Oh, got it. Then this:
points['inside'] = points.geometry.map(lambda x: True if reduce(lambda a,b: a+b, list(map(lambda p: p.contains(x), df.geometry.values))) else False)
– Federico Pucci
Nov 21 '18 at 15:49
Oh, got it. Then this:
points['inside'] = points.geometry.map(lambda x: True if reduce(lambda a,b: a+b, list(map(lambda p: p.contains(x), df.geometry.values))) else False)
– Federico Pucci
Nov 21 '18 at 15:49
YES! Thats got it, thank you so much!
– tom91
Nov 21 '18 at 16:07
YES! Thats got it, thank you so much!
– tom91
Nov 21 '18 at 16:07
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53414065%2ferror-float-argument-must-be-a-string-or-a-number-not-polygon-when-trying%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g4,OyXmJlqZhvEAeC3kF E