How do I retrieve an IVParameterSpec after base64 decode?
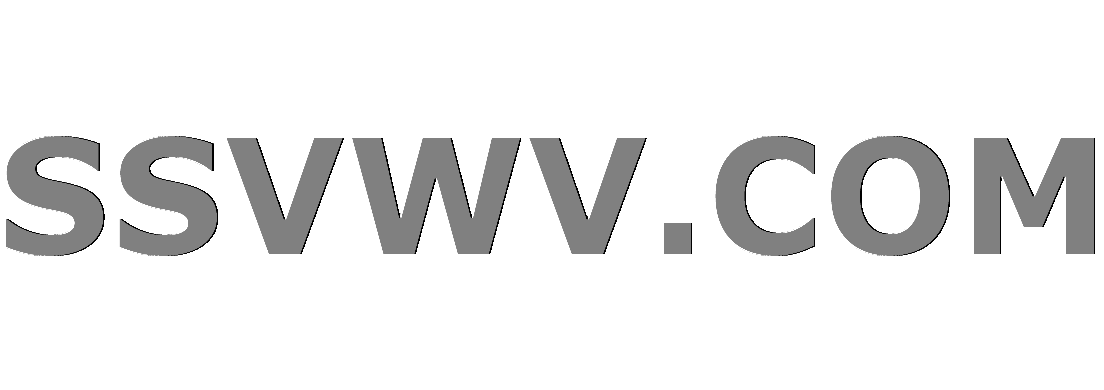
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
EDIT: For those asking to see the entire methods/classes
I have a class Encrypter which creates an IVParameterSpec in a constructor, encodes the IVParameterSpec in the Encoder method and returns the IVParameterSpec in the GetIV method.
This is the Encrypter class
public class Encrypter
{
public IVParameterSpec ctr_iv;
public Encrypter(int keylength)
{
//ctr_iv is created in this constructor
byte counter = new byte[16];
ctr_iv = new IvParameterSpec(counter);
System.out.println("The iv is " + ctr_iv);
}
public String Encoder()
{
String encoded_IV = Base64.getEncoder().encodeToString(ctr_iv.getIV());
return encoded_IV;
}
public IvParameterSpec getIV()
{
return ctr_iv;
}
}
I have a class Decrypter that decodes the IVParameterSpec in a constructor and returns the IVParameterSpec in the GetIV method.
public class Decrypter
{
IvParameterSpec retrieved_iv;
public Decrypter(String iv)
{
byte decodedIV = Base64.getDecoder().decode(iv);
retrieved_iv = new IvParameterSpec(decodedIV);
System.out.println("The iv in this class is " + retrieved_iv);
}
public IvParameterSpec getIV()
{
return retrieved_iv;
}
}
My aim is to make sure that retrieved_iv in the Decryptor class is equal to the ctr_iv in the Encrypter class. I initially thought the constructors above would do the trick. But when I tested their values for equality, I found out that they were not equal. This is how I tested them:
public class Main
{
public static void main(String args)
{
Encrypter encrypter = new Encrypter(); //Initializes ctr_iv
Decrypter decrypter = new Decrypter(encrypter.Encoder()); //Encodes ctr_iv in first object and passes it as an argument to the constructor of the 2nd object
if(encrypter.GetIV().equals(decrypter.GetIV()))
{
System.out.println("IV's are equal");
}else{System.out.println("IV's are not equal");}
}
}
I have a feeling this has something to do with how I'm decoding the string in the Decrypter constructor.
java encryption encoding decode
|
show 1 more comment
EDIT: For those asking to see the entire methods/classes
I have a class Encrypter which creates an IVParameterSpec in a constructor, encodes the IVParameterSpec in the Encoder method and returns the IVParameterSpec in the GetIV method.
This is the Encrypter class
public class Encrypter
{
public IVParameterSpec ctr_iv;
public Encrypter(int keylength)
{
//ctr_iv is created in this constructor
byte counter = new byte[16];
ctr_iv = new IvParameterSpec(counter);
System.out.println("The iv is " + ctr_iv);
}
public String Encoder()
{
String encoded_IV = Base64.getEncoder().encodeToString(ctr_iv.getIV());
return encoded_IV;
}
public IvParameterSpec getIV()
{
return ctr_iv;
}
}
I have a class Decrypter that decodes the IVParameterSpec in a constructor and returns the IVParameterSpec in the GetIV method.
public class Decrypter
{
IvParameterSpec retrieved_iv;
public Decrypter(String iv)
{
byte decodedIV = Base64.getDecoder().decode(iv);
retrieved_iv = new IvParameterSpec(decodedIV);
System.out.println("The iv in this class is " + retrieved_iv);
}
public IvParameterSpec getIV()
{
return retrieved_iv;
}
}
My aim is to make sure that retrieved_iv in the Decryptor class is equal to the ctr_iv in the Encrypter class. I initially thought the constructors above would do the trick. But when I tested their values for equality, I found out that they were not equal. This is how I tested them:
public class Main
{
public static void main(String args)
{
Encrypter encrypter = new Encrypter(); //Initializes ctr_iv
Decrypter decrypter = new Decrypter(encrypter.Encoder()); //Encodes ctr_iv in first object and passes it as an argument to the constructor of the 2nd object
if(encrypter.GetIV().equals(decrypter.GetIV()))
{
System.out.println("IV's are equal");
}else{System.out.println("IV's are not equal");}
}
}
I have a feeling this has something to do with how I'm decoding the string in the Decrypter constructor.
java encryption encoding decode
3
"... it turns out that this just creates a different IVParameterSpec..."? How do you know they're different? Different in what way? They will certainly be different objects, but they will represent (and encapsulate) the same IV.
– James K Polk
Nov 23 '18 at 17:24
I printed out the IVParameterSpec from both methods and they were different.
– Vktr
Nov 23 '18 at 20:29
<Sigh> Printed them out how? Post the code you used that proved to you that they're different.
– James K Polk
Nov 24 '18 at 0:12
1
Finally, we have the source of your confusion.
– James K Polk
Nov 24 '18 at 14:21
1
Possible duplicate of How does an array's equal method work?
– James K Polk
Nov 24 '18 at 14:21
|
show 1 more comment
EDIT: For those asking to see the entire methods/classes
I have a class Encrypter which creates an IVParameterSpec in a constructor, encodes the IVParameterSpec in the Encoder method and returns the IVParameterSpec in the GetIV method.
This is the Encrypter class
public class Encrypter
{
public IVParameterSpec ctr_iv;
public Encrypter(int keylength)
{
//ctr_iv is created in this constructor
byte counter = new byte[16];
ctr_iv = new IvParameterSpec(counter);
System.out.println("The iv is " + ctr_iv);
}
public String Encoder()
{
String encoded_IV = Base64.getEncoder().encodeToString(ctr_iv.getIV());
return encoded_IV;
}
public IvParameterSpec getIV()
{
return ctr_iv;
}
}
I have a class Decrypter that decodes the IVParameterSpec in a constructor and returns the IVParameterSpec in the GetIV method.
public class Decrypter
{
IvParameterSpec retrieved_iv;
public Decrypter(String iv)
{
byte decodedIV = Base64.getDecoder().decode(iv);
retrieved_iv = new IvParameterSpec(decodedIV);
System.out.println("The iv in this class is " + retrieved_iv);
}
public IvParameterSpec getIV()
{
return retrieved_iv;
}
}
My aim is to make sure that retrieved_iv in the Decryptor class is equal to the ctr_iv in the Encrypter class. I initially thought the constructors above would do the trick. But when I tested their values for equality, I found out that they were not equal. This is how I tested them:
public class Main
{
public static void main(String args)
{
Encrypter encrypter = new Encrypter(); //Initializes ctr_iv
Decrypter decrypter = new Decrypter(encrypter.Encoder()); //Encodes ctr_iv in first object and passes it as an argument to the constructor of the 2nd object
if(encrypter.GetIV().equals(decrypter.GetIV()))
{
System.out.println("IV's are equal");
}else{System.out.println("IV's are not equal");}
}
}
I have a feeling this has something to do with how I'm decoding the string in the Decrypter constructor.
java encryption encoding decode
EDIT: For those asking to see the entire methods/classes
I have a class Encrypter which creates an IVParameterSpec in a constructor, encodes the IVParameterSpec in the Encoder method and returns the IVParameterSpec in the GetIV method.
This is the Encrypter class
public class Encrypter
{
public IVParameterSpec ctr_iv;
public Encrypter(int keylength)
{
//ctr_iv is created in this constructor
byte counter = new byte[16];
ctr_iv = new IvParameterSpec(counter);
System.out.println("The iv is " + ctr_iv);
}
public String Encoder()
{
String encoded_IV = Base64.getEncoder().encodeToString(ctr_iv.getIV());
return encoded_IV;
}
public IvParameterSpec getIV()
{
return ctr_iv;
}
}
I have a class Decrypter that decodes the IVParameterSpec in a constructor and returns the IVParameterSpec in the GetIV method.
public class Decrypter
{
IvParameterSpec retrieved_iv;
public Decrypter(String iv)
{
byte decodedIV = Base64.getDecoder().decode(iv);
retrieved_iv = new IvParameterSpec(decodedIV);
System.out.println("The iv in this class is " + retrieved_iv);
}
public IvParameterSpec getIV()
{
return retrieved_iv;
}
}
My aim is to make sure that retrieved_iv in the Decryptor class is equal to the ctr_iv in the Encrypter class. I initially thought the constructors above would do the trick. But when I tested their values for equality, I found out that they were not equal. This is how I tested them:
public class Main
{
public static void main(String args)
{
Encrypter encrypter = new Encrypter(); //Initializes ctr_iv
Decrypter decrypter = new Decrypter(encrypter.Encoder()); //Encodes ctr_iv in first object and passes it as an argument to the constructor of the 2nd object
if(encrypter.GetIV().equals(decrypter.GetIV()))
{
System.out.println("IV's are equal");
}else{System.out.println("IV's are not equal");}
}
}
I have a feeling this has something to do with how I'm decoding the string in the Decrypter constructor.
java encryption encoding decode
java encryption encoding decode
edited Nov 25 '18 at 11:45
Vktr
asked Nov 23 '18 at 15:00
VktrVktr
206
206
3
"... it turns out that this just creates a different IVParameterSpec..."? How do you know they're different? Different in what way? They will certainly be different objects, but they will represent (and encapsulate) the same IV.
– James K Polk
Nov 23 '18 at 17:24
I printed out the IVParameterSpec from both methods and they were different.
– Vktr
Nov 23 '18 at 20:29
<Sigh> Printed them out how? Post the code you used that proved to you that they're different.
– James K Polk
Nov 24 '18 at 0:12
1
Finally, we have the source of your confusion.
– James K Polk
Nov 24 '18 at 14:21
1
Possible duplicate of How does an array's equal method work?
– James K Polk
Nov 24 '18 at 14:21
|
show 1 more comment
3
"... it turns out that this just creates a different IVParameterSpec..."? How do you know they're different? Different in what way? They will certainly be different objects, but they will represent (and encapsulate) the same IV.
– James K Polk
Nov 23 '18 at 17:24
I printed out the IVParameterSpec from both methods and they were different.
– Vktr
Nov 23 '18 at 20:29
<Sigh> Printed them out how? Post the code you used that proved to you that they're different.
– James K Polk
Nov 24 '18 at 0:12
1
Finally, we have the source of your confusion.
– James K Polk
Nov 24 '18 at 14:21
1
Possible duplicate of How does an array's equal method work?
– James K Polk
Nov 24 '18 at 14:21
3
3
"... it turns out that this just creates a different IVParameterSpec..."? How do you know they're different? Different in what way? They will certainly be different objects, but they will represent (and encapsulate) the same IV.
– James K Polk
Nov 23 '18 at 17:24
"... it turns out that this just creates a different IVParameterSpec..."? How do you know they're different? Different in what way? They will certainly be different objects, but they will represent (and encapsulate) the same IV.
– James K Polk
Nov 23 '18 at 17:24
I printed out the IVParameterSpec from both methods and they were different.
– Vktr
Nov 23 '18 at 20:29
I printed out the IVParameterSpec from both methods and they were different.
– Vktr
Nov 23 '18 at 20:29
<Sigh> Printed them out how? Post the code you used that proved to you that they're different.
– James K Polk
Nov 24 '18 at 0:12
<Sigh> Printed them out how? Post the code you used that proved to you that they're different.
– James K Polk
Nov 24 '18 at 0:12
1
1
Finally, we have the source of your confusion.
– James K Polk
Nov 24 '18 at 14:21
Finally, we have the source of your confusion.
– James K Polk
Nov 24 '18 at 14:21
1
1
Possible duplicate of How does an array's equal method work?
– James K Polk
Nov 24 '18 at 14:21
Possible duplicate of How does an array's equal method work?
– James K Polk
Nov 24 '18 at 14:21
|
show 1 more comment
1 Answer
1
active
oldest
votes
Compare by Arrays.equals.
if(Arrays.equals(ctr_iv.getIV(),retrieved_iv.getIV())) {
System.out.println("IV's are equal");
} else {
System.out.println("IV's are not equal");
}
The Encoder and Decoder methods are in separate classes (sorry i didn't mention this earlier. I'll edit the description). So I don't think this would work.
– Vktr
Nov 23 '18 at 20:22
@Vktr when you print the values aren't they same?
– kelalaka
Nov 23 '18 at 20:24
Exactly. I even used a conditional statement that checks if they're equal. If they are equal, print "Pass", otherwise print "Fail". But it prints Fail every time.
– Vktr
Nov 23 '18 at 20:28
@Vktr how you compare? how you transfer the IV between the two classes?
– kelalaka
Nov 23 '18 at 20:30
The Encoder method is in a class called Encrypter and the Decoder method is in a class called Decrypter. In the Encrypter class, I created a method called GetIV(), which simply returns ctr_iv and in the Decrypter class, I have a method called GetIV() which simply returns retrieved_iv. In the Main class, I created objects for both Encryptor (encrypterobject) and Decryptor (decrypterobject). Then I called the Encoder method to encrypt the IV and then I called the Decoder method, where I passed encrypterobject.Encoder() as the string argument.
– Vktr
Nov 23 '18 at 20:37
|
show 9 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448949%2fhow-do-i-retrieve-an-ivparameterspec-after-base64-decode%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Compare by Arrays.equals.
if(Arrays.equals(ctr_iv.getIV(),retrieved_iv.getIV())) {
System.out.println("IV's are equal");
} else {
System.out.println("IV's are not equal");
}
The Encoder and Decoder methods are in separate classes (sorry i didn't mention this earlier. I'll edit the description). So I don't think this would work.
– Vktr
Nov 23 '18 at 20:22
@Vktr when you print the values aren't they same?
– kelalaka
Nov 23 '18 at 20:24
Exactly. I even used a conditional statement that checks if they're equal. If they are equal, print "Pass", otherwise print "Fail". But it prints Fail every time.
– Vktr
Nov 23 '18 at 20:28
@Vktr how you compare? how you transfer the IV between the two classes?
– kelalaka
Nov 23 '18 at 20:30
The Encoder method is in a class called Encrypter and the Decoder method is in a class called Decrypter. In the Encrypter class, I created a method called GetIV(), which simply returns ctr_iv and in the Decrypter class, I have a method called GetIV() which simply returns retrieved_iv. In the Main class, I created objects for both Encryptor (encrypterobject) and Decryptor (decrypterobject). Then I called the Encoder method to encrypt the IV and then I called the Decoder method, where I passed encrypterobject.Encoder() as the string argument.
– Vktr
Nov 23 '18 at 20:37
|
show 9 more comments
Compare by Arrays.equals.
if(Arrays.equals(ctr_iv.getIV(),retrieved_iv.getIV())) {
System.out.println("IV's are equal");
} else {
System.out.println("IV's are not equal");
}
The Encoder and Decoder methods are in separate classes (sorry i didn't mention this earlier. I'll edit the description). So I don't think this would work.
– Vktr
Nov 23 '18 at 20:22
@Vktr when you print the values aren't they same?
– kelalaka
Nov 23 '18 at 20:24
Exactly. I even used a conditional statement that checks if they're equal. If they are equal, print "Pass", otherwise print "Fail". But it prints Fail every time.
– Vktr
Nov 23 '18 at 20:28
@Vktr how you compare? how you transfer the IV between the two classes?
– kelalaka
Nov 23 '18 at 20:30
The Encoder method is in a class called Encrypter and the Decoder method is in a class called Decrypter. In the Encrypter class, I created a method called GetIV(), which simply returns ctr_iv and in the Decrypter class, I have a method called GetIV() which simply returns retrieved_iv. In the Main class, I created objects for both Encryptor (encrypterobject) and Decryptor (decrypterobject). Then I called the Encoder method to encrypt the IV and then I called the Decoder method, where I passed encrypterobject.Encoder() as the string argument.
– Vktr
Nov 23 '18 at 20:37
|
show 9 more comments
Compare by Arrays.equals.
if(Arrays.equals(ctr_iv.getIV(),retrieved_iv.getIV())) {
System.out.println("IV's are equal");
} else {
System.out.println("IV's are not equal");
}
Compare by Arrays.equals.
if(Arrays.equals(ctr_iv.getIV(),retrieved_iv.getIV())) {
System.out.println("IV's are equal");
} else {
System.out.println("IV's are not equal");
}
edited Nov 24 '18 at 18:27
answered Nov 23 '18 at 18:53


kelalakakelalaka
1,61331225
1,61331225
The Encoder and Decoder methods are in separate classes (sorry i didn't mention this earlier. I'll edit the description). So I don't think this would work.
– Vktr
Nov 23 '18 at 20:22
@Vktr when you print the values aren't they same?
– kelalaka
Nov 23 '18 at 20:24
Exactly. I even used a conditional statement that checks if they're equal. If they are equal, print "Pass", otherwise print "Fail". But it prints Fail every time.
– Vktr
Nov 23 '18 at 20:28
@Vktr how you compare? how you transfer the IV between the two classes?
– kelalaka
Nov 23 '18 at 20:30
The Encoder method is in a class called Encrypter and the Decoder method is in a class called Decrypter. In the Encrypter class, I created a method called GetIV(), which simply returns ctr_iv and in the Decrypter class, I have a method called GetIV() which simply returns retrieved_iv. In the Main class, I created objects for both Encryptor (encrypterobject) and Decryptor (decrypterobject). Then I called the Encoder method to encrypt the IV and then I called the Decoder method, where I passed encrypterobject.Encoder() as the string argument.
– Vktr
Nov 23 '18 at 20:37
|
show 9 more comments
The Encoder and Decoder methods are in separate classes (sorry i didn't mention this earlier. I'll edit the description). So I don't think this would work.
– Vktr
Nov 23 '18 at 20:22
@Vktr when you print the values aren't they same?
– kelalaka
Nov 23 '18 at 20:24
Exactly. I even used a conditional statement that checks if they're equal. If they are equal, print "Pass", otherwise print "Fail". But it prints Fail every time.
– Vktr
Nov 23 '18 at 20:28
@Vktr how you compare? how you transfer the IV between the two classes?
– kelalaka
Nov 23 '18 at 20:30
The Encoder method is in a class called Encrypter and the Decoder method is in a class called Decrypter. In the Encrypter class, I created a method called GetIV(), which simply returns ctr_iv and in the Decrypter class, I have a method called GetIV() which simply returns retrieved_iv. In the Main class, I created objects for both Encryptor (encrypterobject) and Decryptor (decrypterobject). Then I called the Encoder method to encrypt the IV and then I called the Decoder method, where I passed encrypterobject.Encoder() as the string argument.
– Vktr
Nov 23 '18 at 20:37
The Encoder and Decoder methods are in separate classes (sorry i didn't mention this earlier. I'll edit the description). So I don't think this would work.
– Vktr
Nov 23 '18 at 20:22
The Encoder and Decoder methods are in separate classes (sorry i didn't mention this earlier. I'll edit the description). So I don't think this would work.
– Vktr
Nov 23 '18 at 20:22
@Vktr when you print the values aren't they same?
– kelalaka
Nov 23 '18 at 20:24
@Vktr when you print the values aren't they same?
– kelalaka
Nov 23 '18 at 20:24
Exactly. I even used a conditional statement that checks if they're equal. If they are equal, print "Pass", otherwise print "Fail". But it prints Fail every time.
– Vktr
Nov 23 '18 at 20:28
Exactly. I even used a conditional statement that checks if they're equal. If they are equal, print "Pass", otherwise print "Fail". But it prints Fail every time.
– Vktr
Nov 23 '18 at 20:28
@Vktr how you compare? how you transfer the IV between the two classes?
– kelalaka
Nov 23 '18 at 20:30
@Vktr how you compare? how you transfer the IV between the two classes?
– kelalaka
Nov 23 '18 at 20:30
The Encoder method is in a class called Encrypter and the Decoder method is in a class called Decrypter. In the Encrypter class, I created a method called GetIV(), which simply returns ctr_iv and in the Decrypter class, I have a method called GetIV() which simply returns retrieved_iv. In the Main class, I created objects for both Encryptor (encrypterobject) and Decryptor (decrypterobject). Then I called the Encoder method to encrypt the IV and then I called the Decoder method, where I passed encrypterobject.Encoder() as the string argument.
– Vktr
Nov 23 '18 at 20:37
The Encoder method is in a class called Encrypter and the Decoder method is in a class called Decrypter. In the Encrypter class, I created a method called GetIV(), which simply returns ctr_iv and in the Decrypter class, I have a method called GetIV() which simply returns retrieved_iv. In the Main class, I created objects for both Encryptor (encrypterobject) and Decryptor (decrypterobject). Then I called the Encoder method to encrypt the IV and then I called the Decoder method, where I passed encrypterobject.Encoder() as the string argument.
– Vktr
Nov 23 '18 at 20:37
|
show 9 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448949%2fhow-do-i-retrieve-an-ivparameterspec-after-base64-decode%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WUlDnvk7,npy5GSrv s2WlWLGVE,B8NfqUCyo0nKkLufJja,TJEMcOyYVqMG Hp NU9vHQbOzn9n1 YCuhgL0E,d
3
"... it turns out that this just creates a different IVParameterSpec..."? How do you know they're different? Different in what way? They will certainly be different objects, but they will represent (and encapsulate) the same IV.
– James K Polk
Nov 23 '18 at 17:24
I printed out the IVParameterSpec from both methods and they were different.
– Vktr
Nov 23 '18 at 20:29
<Sigh> Printed them out how? Post the code you used that proved to you that they're different.
– James K Polk
Nov 24 '18 at 0:12
1
Finally, we have the source of your confusion.
– James K Polk
Nov 24 '18 at 14:21
1
Possible duplicate of How does an array's equal method work?
– James K Polk
Nov 24 '18 at 14:21