Increase/Set vertex label in JUNG graph visualization
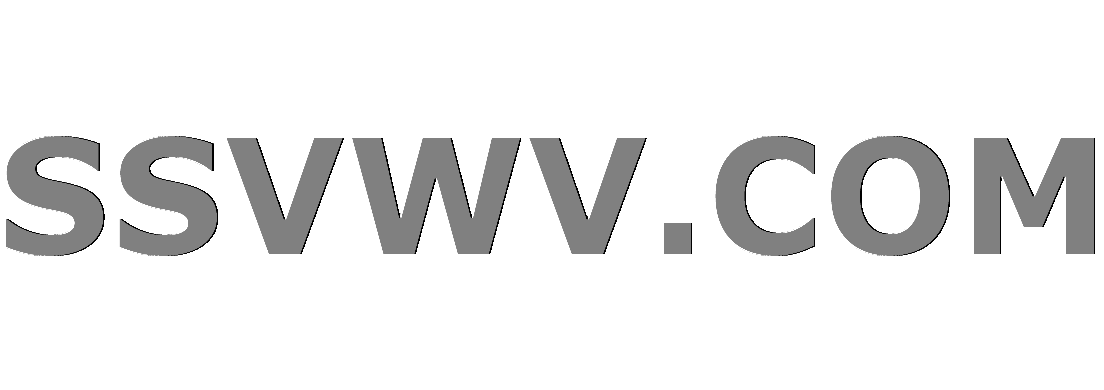
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Looking for a way to increase the size of the vertexLabel using JUNG Graph Visualization. The vertex's themselves are larger than the text label within them and I would like to balance them for a more aesthetically pleasing visual without having to decrease the size of the vertex itself. Below is some code I've been fooling around with:
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Paint;
import java.awt.Shape;
import java.awt.geom.Ellipse2D;
import javax.swing.JComponent;
import javax.swing.JFrame;
import org.apache.commons.collections15.Transformer;
import edu.uci.ics.jung.algorithms.layout.ISOMLayout;
import edu.uci.ics.jung.algorithms.layout.Layout;
import edu.uci.ics.jung.graph.DirectedSparseMultigraph;
import edu.uci.ics.jung.graph.Graph;
import edu.uci.ics.jung.visualization.BasicVisualizationServer;
import edu.uci.ics.jung.visualization.decorators.ToStringLabeller;
import edu.uci.ics.jung.visualization.renderers.DefaultVertexLabelRenderer;
import edu.uci.ics.jung.visualization.renderers.Renderer.VertexLabel.Position;
public class GraphViewer extends JFrame {
private static final long serialVersionUID = 1L;
public GraphViewer(CustomObject user) {
final Graph<CustomObject, String> graph = new DirectedSparseMultigraph<>();
graph.addVertex(user);
for (CustomObject friend: user.getFriends()) {
graph.addVertex(friend);
graph.addEdge(user.toString() + "-" + friend.toString(), user, friend);
}
Layout<CustomObject, String> layout = new ISOMLayout<>(graph);
layout.setSize(new Dimension(1000, 800));
BasicVisualizationServer<CustomObject,String> vv = new BasicVisualizationServer<>(layout);
vv.setPreferredSize(new Dimension(1100,900));
vv.getRenderContext().setVertexLabelTransformer(new ToStringLabeller<CustomObject>());
vv.getRenderer().getVertexLabelRenderer().setPosition(Position.CNTR);
final Color vertexLabelColor = Color.WHITE;
DefaultVertexLabelRenderer vertexLabelRenderer = new DefaultVertexLabelRenderer(vertexLabelColor) {
@Override
public <V> Component getVertexLabelRendererComponent(
JComponent vv, Object value, Font font,
boolean isSelected, V vertex)
{
super.getVertexLabelRendererComponent(vv, value, font, isSelected, vertex);
setForeground(vertexLabelColor);
return this;
}
};
Transformer<CustomObject,Paint> vertexColor = new Transformer<CustomObject,Paint>() {
public Color transform(CustomObject u) {
// First user colored red
// Any user other than first colored blue
if(u.getUsername().equals(user.getUsername()))
return Color.RED;
return Color.BLUE;
}
};
Transformer<CustomObject,Shape> vertexSize = new Transformer<CustomObject,Shape>(){
public Shape transform(CustomObject u) {
Graphics graphics = getGraphics();
FontMetrics metrics = graphics.getFontMetrics();
// Ellipse size is calculated by multiplying the username by a value
int radius = (int) (metrics.stringWidth(u.toString()) * 4.5);
return new Ellipse2D.Double(-15, -15, radius, radius);
}
};
vv.getRenderContext().setVertexLabelRenderer(vertexLabelRenderer);
vv.getRenderContext().setVertexFillPaintTransformer(vertexColor);
vv.getRenderContext().setVertexShapeTransformer(vertexSize);
setTitle(user.toString() + "'s Graph");
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
getContentPane().add(vv);
pack();
setVisible(true);
}
}
Here is an Example Graph of the current output. I would like the font to fill the vertex's a bit more or even be able to manipulate the properties of them (Bold, Italic, etc..)
Also, If you know how to increase the weight of the edge lines I would appreciate any info on that as well.
Thanks for looking. Any feedback is appreciated. Sorry its a mess, its a work in progress and far from finished (obviously).
java graph data-visualization jung graph-visualization
add a comment |
Looking for a way to increase the size of the vertexLabel using JUNG Graph Visualization. The vertex's themselves are larger than the text label within them and I would like to balance them for a more aesthetically pleasing visual without having to decrease the size of the vertex itself. Below is some code I've been fooling around with:
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Paint;
import java.awt.Shape;
import java.awt.geom.Ellipse2D;
import javax.swing.JComponent;
import javax.swing.JFrame;
import org.apache.commons.collections15.Transformer;
import edu.uci.ics.jung.algorithms.layout.ISOMLayout;
import edu.uci.ics.jung.algorithms.layout.Layout;
import edu.uci.ics.jung.graph.DirectedSparseMultigraph;
import edu.uci.ics.jung.graph.Graph;
import edu.uci.ics.jung.visualization.BasicVisualizationServer;
import edu.uci.ics.jung.visualization.decorators.ToStringLabeller;
import edu.uci.ics.jung.visualization.renderers.DefaultVertexLabelRenderer;
import edu.uci.ics.jung.visualization.renderers.Renderer.VertexLabel.Position;
public class GraphViewer extends JFrame {
private static final long serialVersionUID = 1L;
public GraphViewer(CustomObject user) {
final Graph<CustomObject, String> graph = new DirectedSparseMultigraph<>();
graph.addVertex(user);
for (CustomObject friend: user.getFriends()) {
graph.addVertex(friend);
graph.addEdge(user.toString() + "-" + friend.toString(), user, friend);
}
Layout<CustomObject, String> layout = new ISOMLayout<>(graph);
layout.setSize(new Dimension(1000, 800));
BasicVisualizationServer<CustomObject,String> vv = new BasicVisualizationServer<>(layout);
vv.setPreferredSize(new Dimension(1100,900));
vv.getRenderContext().setVertexLabelTransformer(new ToStringLabeller<CustomObject>());
vv.getRenderer().getVertexLabelRenderer().setPosition(Position.CNTR);
final Color vertexLabelColor = Color.WHITE;
DefaultVertexLabelRenderer vertexLabelRenderer = new DefaultVertexLabelRenderer(vertexLabelColor) {
@Override
public <V> Component getVertexLabelRendererComponent(
JComponent vv, Object value, Font font,
boolean isSelected, V vertex)
{
super.getVertexLabelRendererComponent(vv, value, font, isSelected, vertex);
setForeground(vertexLabelColor);
return this;
}
};
Transformer<CustomObject,Paint> vertexColor = new Transformer<CustomObject,Paint>() {
public Color transform(CustomObject u) {
// First user colored red
// Any user other than first colored blue
if(u.getUsername().equals(user.getUsername()))
return Color.RED;
return Color.BLUE;
}
};
Transformer<CustomObject,Shape> vertexSize = new Transformer<CustomObject,Shape>(){
public Shape transform(CustomObject u) {
Graphics graphics = getGraphics();
FontMetrics metrics = graphics.getFontMetrics();
// Ellipse size is calculated by multiplying the username by a value
int radius = (int) (metrics.stringWidth(u.toString()) * 4.5);
return new Ellipse2D.Double(-15, -15, radius, radius);
}
};
vv.getRenderContext().setVertexLabelRenderer(vertexLabelRenderer);
vv.getRenderContext().setVertexFillPaintTransformer(vertexColor);
vv.getRenderContext().setVertexShapeTransformer(vertexSize);
setTitle(user.toString() + "'s Graph");
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
getContentPane().add(vv);
pack();
setVisible(true);
}
}
Here is an Example Graph of the current output. I would like the font to fill the vertex's a bit more or even be able to manipulate the properties of them (Bold, Italic, etc..)
Also, If you know how to increase the weight of the edge lines I would appreciate any info on that as well.
Thanks for looking. Any feedback is appreciated. Sorry its a mess, its a work in progress and far from finished (obviously).
java graph data-visualization jung graph-visualization
add a comment |
Looking for a way to increase the size of the vertexLabel using JUNG Graph Visualization. The vertex's themselves are larger than the text label within them and I would like to balance them for a more aesthetically pleasing visual without having to decrease the size of the vertex itself. Below is some code I've been fooling around with:
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Paint;
import java.awt.Shape;
import java.awt.geom.Ellipse2D;
import javax.swing.JComponent;
import javax.swing.JFrame;
import org.apache.commons.collections15.Transformer;
import edu.uci.ics.jung.algorithms.layout.ISOMLayout;
import edu.uci.ics.jung.algorithms.layout.Layout;
import edu.uci.ics.jung.graph.DirectedSparseMultigraph;
import edu.uci.ics.jung.graph.Graph;
import edu.uci.ics.jung.visualization.BasicVisualizationServer;
import edu.uci.ics.jung.visualization.decorators.ToStringLabeller;
import edu.uci.ics.jung.visualization.renderers.DefaultVertexLabelRenderer;
import edu.uci.ics.jung.visualization.renderers.Renderer.VertexLabel.Position;
public class GraphViewer extends JFrame {
private static final long serialVersionUID = 1L;
public GraphViewer(CustomObject user) {
final Graph<CustomObject, String> graph = new DirectedSparseMultigraph<>();
graph.addVertex(user);
for (CustomObject friend: user.getFriends()) {
graph.addVertex(friend);
graph.addEdge(user.toString() + "-" + friend.toString(), user, friend);
}
Layout<CustomObject, String> layout = new ISOMLayout<>(graph);
layout.setSize(new Dimension(1000, 800));
BasicVisualizationServer<CustomObject,String> vv = new BasicVisualizationServer<>(layout);
vv.setPreferredSize(new Dimension(1100,900));
vv.getRenderContext().setVertexLabelTransformer(new ToStringLabeller<CustomObject>());
vv.getRenderer().getVertexLabelRenderer().setPosition(Position.CNTR);
final Color vertexLabelColor = Color.WHITE;
DefaultVertexLabelRenderer vertexLabelRenderer = new DefaultVertexLabelRenderer(vertexLabelColor) {
@Override
public <V> Component getVertexLabelRendererComponent(
JComponent vv, Object value, Font font,
boolean isSelected, V vertex)
{
super.getVertexLabelRendererComponent(vv, value, font, isSelected, vertex);
setForeground(vertexLabelColor);
return this;
}
};
Transformer<CustomObject,Paint> vertexColor = new Transformer<CustomObject,Paint>() {
public Color transform(CustomObject u) {
// First user colored red
// Any user other than first colored blue
if(u.getUsername().equals(user.getUsername()))
return Color.RED;
return Color.BLUE;
}
};
Transformer<CustomObject,Shape> vertexSize = new Transformer<CustomObject,Shape>(){
public Shape transform(CustomObject u) {
Graphics graphics = getGraphics();
FontMetrics metrics = graphics.getFontMetrics();
// Ellipse size is calculated by multiplying the username by a value
int radius = (int) (metrics.stringWidth(u.toString()) * 4.5);
return new Ellipse2D.Double(-15, -15, radius, radius);
}
};
vv.getRenderContext().setVertexLabelRenderer(vertexLabelRenderer);
vv.getRenderContext().setVertexFillPaintTransformer(vertexColor);
vv.getRenderContext().setVertexShapeTransformer(vertexSize);
setTitle(user.toString() + "'s Graph");
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
getContentPane().add(vv);
pack();
setVisible(true);
}
}
Here is an Example Graph of the current output. I would like the font to fill the vertex's a bit more or even be able to manipulate the properties of them (Bold, Italic, etc..)
Also, If you know how to increase the weight of the edge lines I would appreciate any info on that as well.
Thanks for looking. Any feedback is appreciated. Sorry its a mess, its a work in progress and far from finished (obviously).
java graph data-visualization jung graph-visualization
Looking for a way to increase the size of the vertexLabel using JUNG Graph Visualization. The vertex's themselves are larger than the text label within them and I would like to balance them for a more aesthetically pleasing visual without having to decrease the size of the vertex itself. Below is some code I've been fooling around with:
import java.awt.Color;
import java.awt.Component;
import java.awt.Dimension;
import java.awt.Font;
import java.awt.FontMetrics;
import java.awt.Graphics;
import java.awt.Paint;
import java.awt.Shape;
import java.awt.geom.Ellipse2D;
import javax.swing.JComponent;
import javax.swing.JFrame;
import org.apache.commons.collections15.Transformer;
import edu.uci.ics.jung.algorithms.layout.ISOMLayout;
import edu.uci.ics.jung.algorithms.layout.Layout;
import edu.uci.ics.jung.graph.DirectedSparseMultigraph;
import edu.uci.ics.jung.graph.Graph;
import edu.uci.ics.jung.visualization.BasicVisualizationServer;
import edu.uci.ics.jung.visualization.decorators.ToStringLabeller;
import edu.uci.ics.jung.visualization.renderers.DefaultVertexLabelRenderer;
import edu.uci.ics.jung.visualization.renderers.Renderer.VertexLabel.Position;
public class GraphViewer extends JFrame {
private static final long serialVersionUID = 1L;
public GraphViewer(CustomObject user) {
final Graph<CustomObject, String> graph = new DirectedSparseMultigraph<>();
graph.addVertex(user);
for (CustomObject friend: user.getFriends()) {
graph.addVertex(friend);
graph.addEdge(user.toString() + "-" + friend.toString(), user, friend);
}
Layout<CustomObject, String> layout = new ISOMLayout<>(graph);
layout.setSize(new Dimension(1000, 800));
BasicVisualizationServer<CustomObject,String> vv = new BasicVisualizationServer<>(layout);
vv.setPreferredSize(new Dimension(1100,900));
vv.getRenderContext().setVertexLabelTransformer(new ToStringLabeller<CustomObject>());
vv.getRenderer().getVertexLabelRenderer().setPosition(Position.CNTR);
final Color vertexLabelColor = Color.WHITE;
DefaultVertexLabelRenderer vertexLabelRenderer = new DefaultVertexLabelRenderer(vertexLabelColor) {
@Override
public <V> Component getVertexLabelRendererComponent(
JComponent vv, Object value, Font font,
boolean isSelected, V vertex)
{
super.getVertexLabelRendererComponent(vv, value, font, isSelected, vertex);
setForeground(vertexLabelColor);
return this;
}
};
Transformer<CustomObject,Paint> vertexColor = new Transformer<CustomObject,Paint>() {
public Color transform(CustomObject u) {
// First user colored red
// Any user other than first colored blue
if(u.getUsername().equals(user.getUsername()))
return Color.RED;
return Color.BLUE;
}
};
Transformer<CustomObject,Shape> vertexSize = new Transformer<CustomObject,Shape>(){
public Shape transform(CustomObject u) {
Graphics graphics = getGraphics();
FontMetrics metrics = graphics.getFontMetrics();
// Ellipse size is calculated by multiplying the username by a value
int radius = (int) (metrics.stringWidth(u.toString()) * 4.5);
return new Ellipse2D.Double(-15, -15, radius, radius);
}
};
vv.getRenderContext().setVertexLabelRenderer(vertexLabelRenderer);
vv.getRenderContext().setVertexFillPaintTransformer(vertexColor);
vv.getRenderContext().setVertexShapeTransformer(vertexSize);
setTitle(user.toString() + "'s Graph");
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
getContentPane().add(vv);
pack();
setVisible(true);
}
}
Here is an Example Graph of the current output. I would like the font to fill the vertex's a bit more or even be able to manipulate the properties of them (Bold, Italic, etc..)
Also, If you know how to increase the weight of the edge lines I would appreciate any info on that as well.
Thanks for looking. Any feedback is appreciated. Sorry its a mess, its a work in progress and far from finished (obviously).
java graph data-visualization jung graph-visualization
java graph data-visualization jung graph-visualization
asked Nov 25 '18 at 1:23
string userNamestring userName
61
61
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
You can set the VertexFontTransformer (for example):
vv.getRenderContext().setVertexFontTransformer(new Transformer<CustomObject, Font>(){
@Override
public Font transform(CustomObject customObject) {
return new Font("Helvetica", Font.BOLD, 30);
}
});
You may want to experiment with your vertexShapeTransformer since you are using the size of the label (affected by the font size) to determine the size of the vertices.
To increase the weight of the edge lines, do something like this:
vv.getRenderContext().setEdgeStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
vv.getRenderContext().setEdgeArrowStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
And finally, to place your vertices in better position relative to the edges, you may want to do this:
return new Ellipse2D.Double(-radius/2, -radius/2, radius, radius);
instead of this:
return new Ellipse2D.Double(-15, -15, radius, radius);
(centering the vertices at the origin before they are transformed to their desired position)
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463904%2fincrease-set-vertex-label-in-jung-graph-visualization%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can set the VertexFontTransformer (for example):
vv.getRenderContext().setVertexFontTransformer(new Transformer<CustomObject, Font>(){
@Override
public Font transform(CustomObject customObject) {
return new Font("Helvetica", Font.BOLD, 30);
}
});
You may want to experiment with your vertexShapeTransformer since you are using the size of the label (affected by the font size) to determine the size of the vertices.
To increase the weight of the edge lines, do something like this:
vv.getRenderContext().setEdgeStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
vv.getRenderContext().setEdgeArrowStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
And finally, to place your vertices in better position relative to the edges, you may want to do this:
return new Ellipse2D.Double(-radius/2, -radius/2, radius, radius);
instead of this:
return new Ellipse2D.Double(-15, -15, radius, radius);
(centering the vertices at the origin before they are transformed to their desired position)
add a comment |
You can set the VertexFontTransformer (for example):
vv.getRenderContext().setVertexFontTransformer(new Transformer<CustomObject, Font>(){
@Override
public Font transform(CustomObject customObject) {
return new Font("Helvetica", Font.BOLD, 30);
}
});
You may want to experiment with your vertexShapeTransformer since you are using the size of the label (affected by the font size) to determine the size of the vertices.
To increase the weight of the edge lines, do something like this:
vv.getRenderContext().setEdgeStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
vv.getRenderContext().setEdgeArrowStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
And finally, to place your vertices in better position relative to the edges, you may want to do this:
return new Ellipse2D.Double(-radius/2, -radius/2, radius, radius);
instead of this:
return new Ellipse2D.Double(-15, -15, radius, radius);
(centering the vertices at the origin before they are transformed to their desired position)
add a comment |
You can set the VertexFontTransformer (for example):
vv.getRenderContext().setVertexFontTransformer(new Transformer<CustomObject, Font>(){
@Override
public Font transform(CustomObject customObject) {
return new Font("Helvetica", Font.BOLD, 30);
}
});
You may want to experiment with your vertexShapeTransformer since you are using the size of the label (affected by the font size) to determine the size of the vertices.
To increase the weight of the edge lines, do something like this:
vv.getRenderContext().setEdgeStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
vv.getRenderContext().setEdgeArrowStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
And finally, to place your vertices in better position relative to the edges, you may want to do this:
return new Ellipse2D.Double(-radius/2, -radius/2, radius, radius);
instead of this:
return new Ellipse2D.Double(-15, -15, radius, radius);
(centering the vertices at the origin before they are transformed to their desired position)
You can set the VertexFontTransformer (for example):
vv.getRenderContext().setVertexFontTransformer(new Transformer<CustomObject, Font>(){
@Override
public Font transform(CustomObject customObject) {
return new Font("Helvetica", Font.BOLD, 30);
}
});
You may want to experiment with your vertexShapeTransformer since you are using the size of the label (affected by the font size) to determine the size of the vertices.
To increase the weight of the edge lines, do something like this:
vv.getRenderContext().setEdgeStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
vv.getRenderContext().setEdgeArrowStrokeTransformer(new Transformer<String,Stroke>(){
@Override
public Stroke transform(String s) {
return new BasicStroke(5);
}
});
And finally, to place your vertices in better position relative to the edges, you may want to do this:
return new Ellipse2D.Double(-radius/2, -radius/2, radius, radius);
instead of this:
return new Ellipse2D.Double(-15, -15, radius, radius);
(centering the vertices at the origin before they are transformed to their desired position)
edited Nov 27 '18 at 14:44
answered Nov 25 '18 at 15:42


Tom NelsonTom Nelson
1815
1815
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53463904%2fincrease-set-vertex-label-in-jung-graph-visualization%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
p1,8kGPe p0BKOFbmEs,RGN2Fy0CEkrE3qX I