How do I set a custom meta value when creating a post using the Wordpress REST api
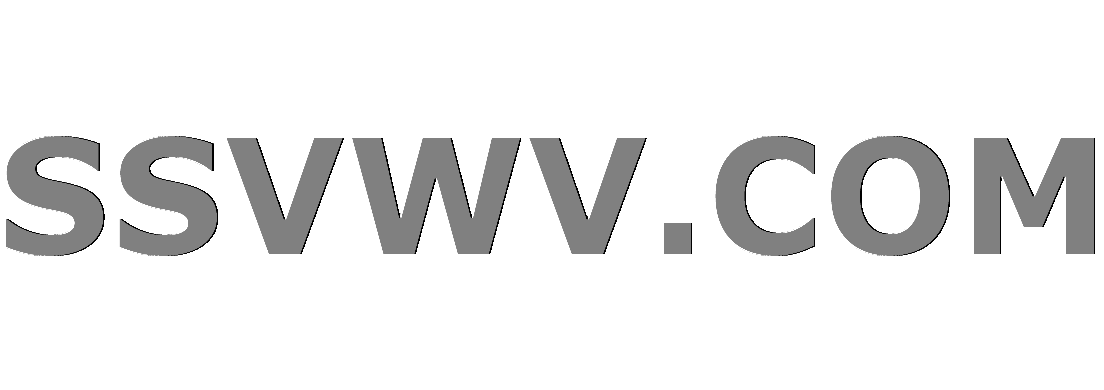
Multi tool use
up vote
0
down vote
favorite
I am currently using the Wordpress REST API to read and write posts.
I have created a custom post type called property
:
register_post_type( 'property', array(
'labels' => array(
'name' => 'Properties',
'singular_name' => 'Property',
),
'description' => '',
'show_in_rest' => true,
'public' => true,
'menu_position' => 20,
'supports' => array( 'title', 'editor')
));
add_action( 'init', 'property_cpt' );
And I have added a custom metabox called property_name
so the it will appear in the edit post admin page:
function add_property_metaboxes(){
add_meta_box(
'property_name', // id
'Name', // title
'property_name_callback', // callback
'property', // page
'normal', // context
'default' // priority
);
}
function property_name_callback() {
global $post;
wp_nonce_field( basename( __FILE__ ), 'property_fields' );
$value = get_post_meta( $post->ID, 'property_name', true );
echo '<input name="property_name" type="text" value="' . $value . '" />';
}
add_action( 'add_meta_boxes', 'add_property_metaboxes' );
I am handling the save function for this post type:
function property_save_meta( $post_id, $post ) {
if ( ! current_user_can( 'edit_post', $post_id ) ) {
return $post_id;
}
if ( !isset( $_POST['property_name'] ) ||
!wp_verify_nonce( $_POST['property_fields'], basename(__FILE__) ) ) {
return $post_id;
}
$events_meta['property_name'] = esc_textarea( $_POST['property_name'] );
foreach ( $events_meta as $key => $value ) :
if ( 'revision' === $post->post_type ) {
return;
}
if ( get_post_meta( $post_id, $key, false ) ) {
update_post_meta( $post_id, $key, $value );
} else {
add_post_meta( $post_id, $key, $value);
}
if ( ! $value ) {
delete_post_meta( $post_id, $key );
}
endforeach;
}
add_action( 'save_post', 'property_save_meta', 1, 2 );
And finally I have registered property_name
as a rest field:
function register_rest_fields() {
register_rest_field('property', 'property_name',
array(
'get_callback' => 'get_post_meta_cb',
'update_callback' => 'update_post_meta_cb',
'schema' => null
)
);
}
function get_post_meta_cb($object, $field_name, $request){
return get_post_meta($object['id'], $field_name, true);
}
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
add_action('rest_api_init', 'register_rest_fields');
On the front-end, I am able to fetch 'property' posts using the API and the property_name
field appears correctly in the response.
I am also able to create a new post easily but I am not able to set the property_name
meta field value.
Currently I am trying:
request(`${url}/wp-json/wp/v2/property`, {
method: "POST",
body:JSON.stringify({
title: 'Lorem ipsum dolor sit amet',
content: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
status: 'publish',
meta: {
property_name: 'Testing'
}
})
});
javascript wordpress wordpress-rest-api
add a comment |
up vote
0
down vote
favorite
I am currently using the Wordpress REST API to read and write posts.
I have created a custom post type called property
:
register_post_type( 'property', array(
'labels' => array(
'name' => 'Properties',
'singular_name' => 'Property',
),
'description' => '',
'show_in_rest' => true,
'public' => true,
'menu_position' => 20,
'supports' => array( 'title', 'editor')
));
add_action( 'init', 'property_cpt' );
And I have added a custom metabox called property_name
so the it will appear in the edit post admin page:
function add_property_metaboxes(){
add_meta_box(
'property_name', // id
'Name', // title
'property_name_callback', // callback
'property', // page
'normal', // context
'default' // priority
);
}
function property_name_callback() {
global $post;
wp_nonce_field( basename( __FILE__ ), 'property_fields' );
$value = get_post_meta( $post->ID, 'property_name', true );
echo '<input name="property_name" type="text" value="' . $value . '" />';
}
add_action( 'add_meta_boxes', 'add_property_metaboxes' );
I am handling the save function for this post type:
function property_save_meta( $post_id, $post ) {
if ( ! current_user_can( 'edit_post', $post_id ) ) {
return $post_id;
}
if ( !isset( $_POST['property_name'] ) ||
!wp_verify_nonce( $_POST['property_fields'], basename(__FILE__) ) ) {
return $post_id;
}
$events_meta['property_name'] = esc_textarea( $_POST['property_name'] );
foreach ( $events_meta as $key => $value ) :
if ( 'revision' === $post->post_type ) {
return;
}
if ( get_post_meta( $post_id, $key, false ) ) {
update_post_meta( $post_id, $key, $value );
} else {
add_post_meta( $post_id, $key, $value);
}
if ( ! $value ) {
delete_post_meta( $post_id, $key );
}
endforeach;
}
add_action( 'save_post', 'property_save_meta', 1, 2 );
And finally I have registered property_name
as a rest field:
function register_rest_fields() {
register_rest_field('property', 'property_name',
array(
'get_callback' => 'get_post_meta_cb',
'update_callback' => 'update_post_meta_cb',
'schema' => null
)
);
}
function get_post_meta_cb($object, $field_name, $request){
return get_post_meta($object['id'], $field_name, true);
}
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
add_action('rest_api_init', 'register_rest_fields');
On the front-end, I am able to fetch 'property' posts using the API and the property_name
field appears correctly in the response.
I am also able to create a new post easily but I am not able to set the property_name
meta field value.
Currently I am trying:
request(`${url}/wp-json/wp/v2/property`, {
method: "POST",
body:JSON.stringify({
title: 'Lorem ipsum dolor sit amet',
content: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
status: 'publish',
meta: {
property_name: 'Testing'
}
})
});
javascript wordpress wordpress-rest-api
1
In your "update" callback (update_post_meta_cb()
), you usedupdate_term_meta()
and notupdate_post_meta()
.
– Sally CJ
Nov 4 at 13:02
@SallyCJ Thank you! I have spent hours on this. You could put that as an answer
– Jackson
Nov 4 at 13:42
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am currently using the Wordpress REST API to read and write posts.
I have created a custom post type called property
:
register_post_type( 'property', array(
'labels' => array(
'name' => 'Properties',
'singular_name' => 'Property',
),
'description' => '',
'show_in_rest' => true,
'public' => true,
'menu_position' => 20,
'supports' => array( 'title', 'editor')
));
add_action( 'init', 'property_cpt' );
And I have added a custom metabox called property_name
so the it will appear in the edit post admin page:
function add_property_metaboxes(){
add_meta_box(
'property_name', // id
'Name', // title
'property_name_callback', // callback
'property', // page
'normal', // context
'default' // priority
);
}
function property_name_callback() {
global $post;
wp_nonce_field( basename( __FILE__ ), 'property_fields' );
$value = get_post_meta( $post->ID, 'property_name', true );
echo '<input name="property_name" type="text" value="' . $value . '" />';
}
add_action( 'add_meta_boxes', 'add_property_metaboxes' );
I am handling the save function for this post type:
function property_save_meta( $post_id, $post ) {
if ( ! current_user_can( 'edit_post', $post_id ) ) {
return $post_id;
}
if ( !isset( $_POST['property_name'] ) ||
!wp_verify_nonce( $_POST['property_fields'], basename(__FILE__) ) ) {
return $post_id;
}
$events_meta['property_name'] = esc_textarea( $_POST['property_name'] );
foreach ( $events_meta as $key => $value ) :
if ( 'revision' === $post->post_type ) {
return;
}
if ( get_post_meta( $post_id, $key, false ) ) {
update_post_meta( $post_id, $key, $value );
} else {
add_post_meta( $post_id, $key, $value);
}
if ( ! $value ) {
delete_post_meta( $post_id, $key );
}
endforeach;
}
add_action( 'save_post', 'property_save_meta', 1, 2 );
And finally I have registered property_name
as a rest field:
function register_rest_fields() {
register_rest_field('property', 'property_name',
array(
'get_callback' => 'get_post_meta_cb',
'update_callback' => 'update_post_meta_cb',
'schema' => null
)
);
}
function get_post_meta_cb($object, $field_name, $request){
return get_post_meta($object['id'], $field_name, true);
}
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
add_action('rest_api_init', 'register_rest_fields');
On the front-end, I am able to fetch 'property' posts using the API and the property_name
field appears correctly in the response.
I am also able to create a new post easily but I am not able to set the property_name
meta field value.
Currently I am trying:
request(`${url}/wp-json/wp/v2/property`, {
method: "POST",
body:JSON.stringify({
title: 'Lorem ipsum dolor sit amet',
content: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
status: 'publish',
meta: {
property_name: 'Testing'
}
})
});
javascript wordpress wordpress-rest-api
I am currently using the Wordpress REST API to read and write posts.
I have created a custom post type called property
:
register_post_type( 'property', array(
'labels' => array(
'name' => 'Properties',
'singular_name' => 'Property',
),
'description' => '',
'show_in_rest' => true,
'public' => true,
'menu_position' => 20,
'supports' => array( 'title', 'editor')
));
add_action( 'init', 'property_cpt' );
And I have added a custom metabox called property_name
so the it will appear in the edit post admin page:
function add_property_metaboxes(){
add_meta_box(
'property_name', // id
'Name', // title
'property_name_callback', // callback
'property', // page
'normal', // context
'default' // priority
);
}
function property_name_callback() {
global $post;
wp_nonce_field( basename( __FILE__ ), 'property_fields' );
$value = get_post_meta( $post->ID, 'property_name', true );
echo '<input name="property_name" type="text" value="' . $value . '" />';
}
add_action( 'add_meta_boxes', 'add_property_metaboxes' );
I am handling the save function for this post type:
function property_save_meta( $post_id, $post ) {
if ( ! current_user_can( 'edit_post', $post_id ) ) {
return $post_id;
}
if ( !isset( $_POST['property_name'] ) ||
!wp_verify_nonce( $_POST['property_fields'], basename(__FILE__) ) ) {
return $post_id;
}
$events_meta['property_name'] = esc_textarea( $_POST['property_name'] );
foreach ( $events_meta as $key => $value ) :
if ( 'revision' === $post->post_type ) {
return;
}
if ( get_post_meta( $post_id, $key, false ) ) {
update_post_meta( $post_id, $key, $value );
} else {
add_post_meta( $post_id, $key, $value);
}
if ( ! $value ) {
delete_post_meta( $post_id, $key );
}
endforeach;
}
add_action( 'save_post', 'property_save_meta', 1, 2 );
And finally I have registered property_name
as a rest field:
function register_rest_fields() {
register_rest_field('property', 'property_name',
array(
'get_callback' => 'get_post_meta_cb',
'update_callback' => 'update_post_meta_cb',
'schema' => null
)
);
}
function get_post_meta_cb($object, $field_name, $request){
return get_post_meta($object['id'], $field_name, true);
}
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
add_action('rest_api_init', 'register_rest_fields');
On the front-end, I am able to fetch 'property' posts using the API and the property_name
field appears correctly in the response.
I am also able to create a new post easily but I am not able to set the property_name
meta field value.
Currently I am trying:
request(`${url}/wp-json/wp/v2/property`, {
method: "POST",
body:JSON.stringify({
title: 'Lorem ipsum dolor sit amet',
content: 'Lorem ipsum dolor sit amet, consectetur adipiscing elit.',
status: 'publish',
meta: {
property_name: 'Testing'
}
})
});
javascript wordpress wordpress-rest-api
javascript wordpress wordpress-rest-api
asked Nov 4 at 11:16


Jackson
2,7101818
2,7101818
1
In your "update" callback (update_post_meta_cb()
), you usedupdate_term_meta()
and notupdate_post_meta()
.
– Sally CJ
Nov 4 at 13:02
@SallyCJ Thank you! I have spent hours on this. You could put that as an answer
– Jackson
Nov 4 at 13:42
add a comment |
1
In your "update" callback (update_post_meta_cb()
), you usedupdate_term_meta()
and notupdate_post_meta()
.
– Sally CJ
Nov 4 at 13:02
@SallyCJ Thank you! I have spent hours on this. You could put that as an answer
– Jackson
Nov 4 at 13:42
1
1
In your "update" callback (
update_post_meta_cb()
), you used update_term_meta()
and not update_post_meta()
.– Sally CJ
Nov 4 at 13:02
In your "update" callback (
update_post_meta_cb()
), you used update_term_meta()
and not update_post_meta()
.– Sally CJ
Nov 4 at 13:02
@SallyCJ Thank you! I have spent hours on this. You could put that as an answer
– Jackson
Nov 4 at 13:42
@SallyCJ Thank you! I have spent hours on this. You could put that as an answer
– Jackson
Nov 4 at 13:42
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
(As pointed in my comment,) the problem is here:
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
where you're actually saving a post meta, but then used update_term_meta()
which is for saving a term meta. :)
And (I forgot to say that) to fix it, just replace the update_term_meta()
with update_post_meta()
. ;)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
(As pointed in my comment,) the problem is here:
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
where you're actually saving a post meta, but then used update_term_meta()
which is for saving a term meta. :)
And (I forgot to say that) to fix it, just replace the update_term_meta()
with update_post_meta()
. ;)
add a comment |
up vote
1
down vote
accepted
(As pointed in my comment,) the problem is here:
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
where you're actually saving a post meta, but then used update_term_meta()
which is for saving a term meta. :)
And (I forgot to say that) to fix it, just replace the update_term_meta()
with update_post_meta()
. ;)
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
(As pointed in my comment,) the problem is here:
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
where you're actually saving a post meta, but then used update_term_meta()
which is for saving a term meta. :)
And (I forgot to say that) to fix it, just replace the update_term_meta()
with update_post_meta()
. ;)
(As pointed in my comment,) the problem is here:
function update_post_meta_cb($value, $object, $field_name){
if ( ! $value || ! is_string( $value ) ) {
return;
}
return update_term_meta( $object->ID, $field_name, $value );
}
where you're actually saving a post meta, but then used update_term_meta()
which is for saving a term meta. :)
And (I forgot to say that) to fix it, just replace the update_term_meta()
with update_post_meta()
. ;)
edited Nov 5 at 4:06
answered Nov 5 at 3:53
Sally CJ
7,0172416
7,0172416
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53140228%2fhow-do-i-set-a-custom-meta-value-when-creating-a-post-using-the-wordpress-rest-a%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
PPJXIkL4d,UJvrIu,cS bKwq3YSVUUGpBBpSkrkDYWUcoHUBJU6kXQ,pbDob23EszK9kRWvJdsACFpbfyGK ZVAkibw
1
In your "update" callback (
update_post_meta_cb()
), you usedupdate_term_meta()
and notupdate_post_meta()
.– Sally CJ
Nov 4 at 13:02
@SallyCJ Thank you! I have spent hours on this. You could put that as an answer
– Jackson
Nov 4 at 13:42