How to send each csv row one at a time over socket
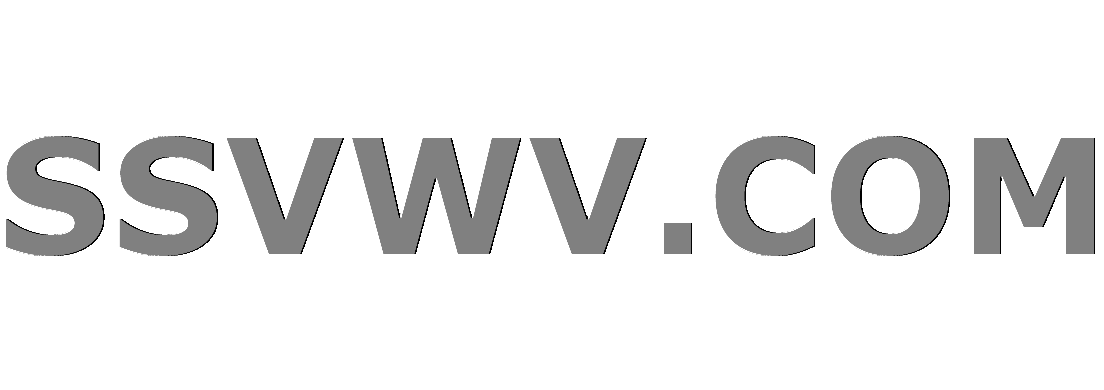
Multi tool use
up vote
0
down vote
favorite
I am writing a code that sends data from client to server. The data I need to send is from a csv file, which has around 1,700 rows. Currently I am sending the data in a large buffer as a whole, but I would prefer to send it row by row and also receive it the same way. I am not sure if I should use the split()
function or there are any better ways to do the same thing.
Note: this question is based off the Python socket to send rows of data
I have the exact same problem. The answer given there for the server portion makes no sense to me. Here is that answer repeated here for clarity.
#server.py
def read_line(sock):
return "".join(iter(lambda:sock.recv(1),"n"))
I need to see how to add this answer into the server code listed below.
The server...
import socket
import ipdb
result = ""
HOST = 'LOCAL_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind((HOST, PORT))
print "Server running", HOST, PORT
s.listen(5)
conn, addr = s.accept()
print'Connected by', addr
while True:
data = conn.recv(409600)
print repr(data.split("' "))
if not data:
break
print "Done Receiving"
conn.close()
The client...
import socket
HOST = 'SERVER_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((HOST, PORT))
while True:
for line in open('target.csv', 'rb'):
s.send(line)
break
print "Done Sending"
s.close()
Thank you for your help.
python python-2.7 sockets
add a comment |
up vote
0
down vote
favorite
I am writing a code that sends data from client to server. The data I need to send is from a csv file, which has around 1,700 rows. Currently I am sending the data in a large buffer as a whole, but I would prefer to send it row by row and also receive it the same way. I am not sure if I should use the split()
function or there are any better ways to do the same thing.
Note: this question is based off the Python socket to send rows of data
I have the exact same problem. The answer given there for the server portion makes no sense to me. Here is that answer repeated here for clarity.
#server.py
def read_line(sock):
return "".join(iter(lambda:sock.recv(1),"n"))
I need to see how to add this answer into the server code listed below.
The server...
import socket
import ipdb
result = ""
HOST = 'LOCAL_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind((HOST, PORT))
print "Server running", HOST, PORT
s.listen(5)
conn, addr = s.accept()
print'Connected by', addr
while True:
data = conn.recv(409600)
print repr(data.split("' "))
if not data:
break
print "Done Receiving"
conn.close()
The client...
import socket
HOST = 'SERVER_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((HOST, PORT))
while True:
for line in open('target.csv', 'rb'):
s.send(line)
break
print "Done Sending"
s.close()
Thank you for your help.
python python-2.7 sockets
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I am writing a code that sends data from client to server. The data I need to send is from a csv file, which has around 1,700 rows. Currently I am sending the data in a large buffer as a whole, but I would prefer to send it row by row and also receive it the same way. I am not sure if I should use the split()
function or there are any better ways to do the same thing.
Note: this question is based off the Python socket to send rows of data
I have the exact same problem. The answer given there for the server portion makes no sense to me. Here is that answer repeated here for clarity.
#server.py
def read_line(sock):
return "".join(iter(lambda:sock.recv(1),"n"))
I need to see how to add this answer into the server code listed below.
The server...
import socket
import ipdb
result = ""
HOST = 'LOCAL_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind((HOST, PORT))
print "Server running", HOST, PORT
s.listen(5)
conn, addr = s.accept()
print'Connected by', addr
while True:
data = conn.recv(409600)
print repr(data.split("' "))
if not data:
break
print "Done Receiving"
conn.close()
The client...
import socket
HOST = 'SERVER_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((HOST, PORT))
while True:
for line in open('target.csv', 'rb'):
s.send(line)
break
print "Done Sending"
s.close()
Thank you for your help.
python python-2.7 sockets
I am writing a code that sends data from client to server. The data I need to send is from a csv file, which has around 1,700 rows. Currently I am sending the data in a large buffer as a whole, but I would prefer to send it row by row and also receive it the same way. I am not sure if I should use the split()
function or there are any better ways to do the same thing.
Note: this question is based off the Python socket to send rows of data
I have the exact same problem. The answer given there for the server portion makes no sense to me. Here is that answer repeated here for clarity.
#server.py
def read_line(sock):
return "".join(iter(lambda:sock.recv(1),"n"))
I need to see how to add this answer into the server code listed below.
The server...
import socket
import ipdb
result = ""
HOST = 'LOCAL_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.bind((HOST, PORT))
print "Server running", HOST, PORT
s.listen(5)
conn, addr = s.accept()
print'Connected by', addr
while True:
data = conn.recv(409600)
print repr(data.split("' "))
if not data:
break
print "Done Receiving"
conn.close()
The client...
import socket
HOST = 'SERVER_IP'
PORT = 42050
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
s.connect((HOST, PORT))
while True:
for line in open('target.csv', 'rb'):
s.send(line)
break
print "Done Sending"
s.close()
Thank you for your help.
python python-2.7 sockets
python python-2.7 sockets
edited Nov 5 at 8:10
Ashok Kumar Jayaraman
900715
900715
asked Nov 5 at 3:41


Jessi
3531618
3531618
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53148019%2fhow-to-send-each-csv-row-one-at-a-time-over-socket%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
8MOhU,Ou4GJYLkGTOrElimOGRVhVy3otPoZ0,j5FCKX3