How to bind inverse boolean properties in WPF?
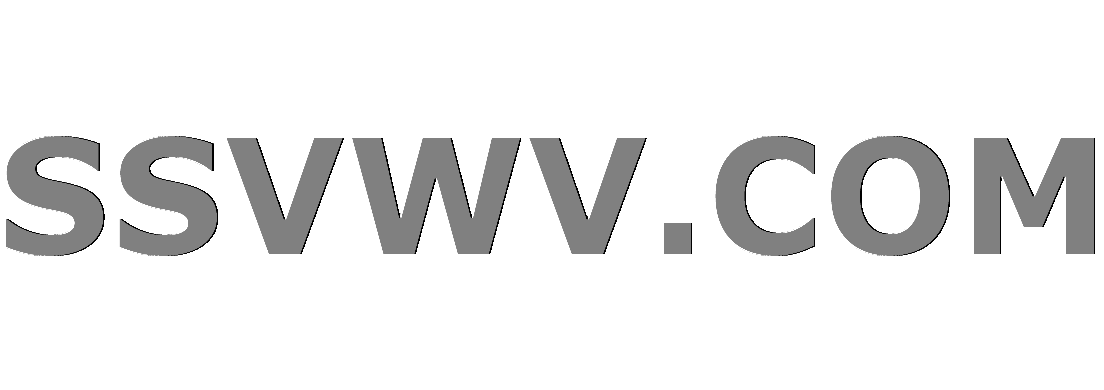
Multi tool use
up vote
317
down vote
favorite
What I have is an object that has an IsReadOnly
property. If this property is true, I would like to set the IsEnabled
property on a Button, ( for example ), to false.
I would like to believe that I can do it as easily as IsEnabled="{Binding Path=!IsReadOnly}"
but that doesn't fly with WPF.
Am I relegated to having to go through all of the style settings? Just seems too wordy for something as simple as setting one bool to the inverse of another bool.
<Button.Style>
<Style TargetType="{x:Type Button}">
<Style.Triggers>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="True">
<Setter Property="IsEnabled" Value="False" />
</DataTrigger>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="False">
<Setter Property="IsEnabled" Value="True" />
</DataTrigger>
</Style.Triggers>
</Style>
</Button.Style>
wpf .net-3.5 styles
add a comment |
up vote
317
down vote
favorite
What I have is an object that has an IsReadOnly
property. If this property is true, I would like to set the IsEnabled
property on a Button, ( for example ), to false.
I would like to believe that I can do it as easily as IsEnabled="{Binding Path=!IsReadOnly}"
but that doesn't fly with WPF.
Am I relegated to having to go through all of the style settings? Just seems too wordy for something as simple as setting one bool to the inverse of another bool.
<Button.Style>
<Style TargetType="{x:Type Button}">
<Style.Triggers>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="True">
<Setter Property="IsEnabled" Value="False" />
</DataTrigger>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="False">
<Setter Property="IsEnabled" Value="True" />
</DataTrigger>
</Style.Triggers>
</Style>
</Button.Style>
wpf .net-3.5 styles
add a comment |
up vote
317
down vote
favorite
up vote
317
down vote
favorite
What I have is an object that has an IsReadOnly
property. If this property is true, I would like to set the IsEnabled
property on a Button, ( for example ), to false.
I would like to believe that I can do it as easily as IsEnabled="{Binding Path=!IsReadOnly}"
but that doesn't fly with WPF.
Am I relegated to having to go through all of the style settings? Just seems too wordy for something as simple as setting one bool to the inverse of another bool.
<Button.Style>
<Style TargetType="{x:Type Button}">
<Style.Triggers>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="True">
<Setter Property="IsEnabled" Value="False" />
</DataTrigger>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="False">
<Setter Property="IsEnabled" Value="True" />
</DataTrigger>
</Style.Triggers>
</Style>
</Button.Style>
wpf .net-3.5 styles
What I have is an object that has an IsReadOnly
property. If this property is true, I would like to set the IsEnabled
property on a Button, ( for example ), to false.
I would like to believe that I can do it as easily as IsEnabled="{Binding Path=!IsReadOnly}"
but that doesn't fly with WPF.
Am I relegated to having to go through all of the style settings? Just seems too wordy for something as simple as setting one bool to the inverse of another bool.
<Button.Style>
<Style TargetType="{x:Type Button}">
<Style.Triggers>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="True">
<Setter Property="IsEnabled" Value="False" />
</DataTrigger>
<DataTrigger Binding="{Binding Path=IsReadOnly}" Value="False">
<Setter Property="IsEnabled" Value="True" />
</DataTrigger>
</Style.Triggers>
</Style>
</Button.Style>
wpf .net-3.5 styles
wpf .net-3.5 styles
edited Oct 8 '15 at 7:53
Tim Pohlmann
1,7101340
1,7101340
asked Jun 24 '09 at 16:59


Russ
5,691175070
5,691175070
add a comment |
add a comment |
11 Answers
11
active
oldest
votes
up vote
413
down vote
accepted
You can use a ValueConverter that inverts a bool property for you.
XAML:
IsEnabled="{Binding Path=IsReadOnly, Converter={StaticResource InverseBooleanConverter}}"
Converter:
[ValueConversion(typeof(bool), typeof(bool))]
public class InverseBooleanConverter: IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
if (targetType != typeof(bool))
throw new InvalidOperationException("The target must be a boolean");
return !(bool)value;
}
public object ConvertBack(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
throw new NotSupportedException();
}
#endregion
}
6
There are a few things I have to consider here, that will likely make me pick @Paul's answer over this one. I am by myself when coding (for now), so I need to go with a solution that "I" will remember, which I will use over and over. I also feel that the less wordy something is the better, and creating an inverse property is very explicit, making it easy for me to remember, as well as future dev's ( I Hope, I Hope ), to be able to quickly see what I was doing, as well as making it easier for them to throw me under the proverbial bus.
– Russ
Jun 24 '09 at 18:07
14
By your own arguments, IMHO the converter solution is better in the long term : you only have to write the converter once, and after that you can reuse it over and over. If you go for the new property, you will have to rewrite it in every class that needs it...
– Thomas Levesque
Jun 26 '09 at 8:30
45
I'm using the same approach... but it makes panda saaad... =(
– Max Galkin
Jan 4 '10 at 21:34
20
Compared to!
, that’s some long-winded code... People go to insane amounts of effort to separate what they feel is "code" from those poor designers. Extra extra painful when I’m both the coder and the designer.
– Roman Starkov
Apr 14 '12 at 14:48
7
many people including myself would consider this a prime example of over-engineering. I suggest using a inverted property as in Paul Alexander post below.
– Christian Westman
Jan 14 '14 at 12:32
|
show 13 more comments
up vote
90
down vote
Have you considered a IsNotReadOnly property? If the object being bound is a ViewModel in a MVVM domain then the additional property makes perfect sense. If it's a direct Entity model, you might consider composition and presenting a specialized viewmodel of your entity to the form.
4
I just solved the same problem using this approach and I agree that not only is it more elegant, but much more maintainable than using a Converter.
– alimbada
Sep 24 '10 at 16:43
25
I would disagree that this approach is better than the value converter. It also produces more code if you need several NotProperty instances.
– Thiru
Jun 26 '12 at 0:31
22
MVVM isn't about not writing code, it's about solving problems declaratively. To that end, the converter is the correct solution.
– Jeff
Dec 31 '12 at 16:41
10
The problem with this solution is that if you have 100 objects, you would have to add an IsNotReadOnly property to all 100 objects. That property would have to be a DependencyProperty. That adds about 10 lines of code to all 100 objects or 1000 lines of code. The Converter is 20 lines of code. 1000 lines or 20 lines. Which would you choose?
– Rhyous
May 13 '14 at 20:59
6
There is a common saying for this: do it once, do it twice, and then automate. In doubt, I would use this answer the first time it's needed in a project, and then if things grow, I'd use the accepted answer. But having the converter snippet pre-made might make it way less difficult to use.
– heltonbiker
Feb 9 '15 at 17:54
|
show 2 more comments
up vote
53
down vote
With standart binding you need to use converters that looks little windy. So, I recommend you to look at my project CalcBinding, which was developed specially to resolve this problem and some others. With advanced binding you can write expressions with many source properties directly in xaml. Say, you can write something like:
<Button IsEnabled="{c:Binding Path=!IsReadOnly}" />
or
<Button Content="{c:Binding ElementName=grid, Path=ActualWidth+Height}"/>
or
<Label Content="{c:Binding A+B+C }" />
or
<Button Visibility="{c:Binding IsChecked, FalseToVisibility=Hidden}" />
where A, B, C, IsChecked - properties of viewModel and it will work properly
Goodluck!
5
Although QuickConverter is more powerful, I find the CalcBinding mode readable - usable.
– xmedeko
Jun 5 '15 at 12:49
This is a great tool. I wish it existed 5 years ago!
– jugg1es
Mar 6 at 16:39
add a comment |
up vote
16
down vote
I would recommend using https://quickconverter.codeplex.com/
Inverting a boolean is then as simple as:
<Button IsEnabled="{qc:Binding '!$P', P={Binding IsReadOnly}}" />
That speeds the time normally needed to write converters.
12
When giving a -1 to someone, it would be nice to explain why.
– Noxxys
Oct 20 '14 at 10:29
add a comment |
up vote
11
down vote
I wanted my XAML to remain as elegant as possible so I created a class to wrap the bool which resides in one of my shared libraries, the implicit operators allow the class to be used as a bool in code-behind seamlessly
public class InvertableBool
{
private bool value = false;
public bool Value { get { return value; } }
public bool Invert { get { return !value; } }
public InvertableBool(bool b)
{
value = b;
}
public static implicit operator InvertableBool(bool b)
{
return new InvertableBool(b);
}
public static implicit operator bool(InvertableBool b)
{
return b.value;
}
}
The only changes needed to your project are to make the property you want to invert return this instead of bool
public InvertableBool IsActive
{
get
{
return true;
}
}
And in the XAML postfix the binding with either Value or Invert
IsEnabled="{Binding IsActive.Value}"
IsEnabled="{Binding IsActive.Invert}"
Downside is that you'd have to change all code that compared it with / assigned it to otherbool
Type Expressions / Variables even not referencing the inverse value. I would instead add a "Not" Extension Method to theBoolean
Struct
.
– Tom
Jan 18 at 0:16
Doh! Never mind. Forgot had to beProperty
vs.Method
forBinding
. My "Downside" statement still applies. Btw, the 'Boolean` "Not" Extension Method is still useful for avoiding the "!" Operator which is easily missed when it (as is often the case) is embedded next to chars that look like it (i.e. one/more "("'s and "l"'s and "I"'s).
– Tom
Jan 18 at 1:54
add a comment |
up vote
7
down vote
This one also works for nullable bools.
[ValueConversion(typeof(bool?), typeof(bool))]
public class InverseBooleanConverter : IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (targetType != typeof(bool?))
{
throw new InvalidOperationException("The target must be a nullable boolean");
}
bool? b = (bool?)value;
return b.HasValue && !b.Value;
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
return !(value as bool?);
}
#endregion
}
add a comment |
up vote
1
down vote
Don't know if this is relevant to XAML, but in my simple Windows app I created the binding manually and added a Format event handler.
public FormMain() {
InitializeComponent();
Binding argBinding = new Binding("Enabled", uxCheckBoxArgsNull, "Checked", false, DataSourceUpdateMode.OnPropertyChanged);
argBinding.Format += new ConvertEventHandler(Binding_Format_BooleanInverse);
uxTextBoxArgs.DataBindings.Add(argBinding);
}
void Binding_Format_BooleanInverse(object sender, ConvertEventArgs e) {
bool boolValue = (bool)e.Value;
e.Value = !boolValue;
}
Seems pretty much the same than the converter approach.Format
andParse
events in WinForms bindings are roughly equivalent of the WPF converter.
– Alejandro
Jan 21 '16 at 13:15
add a comment |
up vote
0
down vote
Add one more property in your view model, which will return reverse value.
And bind that to button.
Like;
in view model:
public bool IsNotReadOnly{get{return !IsReadOnly;}}
in xaml:
IsEnabled="{Binding IsNotReadOnly"}
Great answer. One thing to add, using this you better rise the PropertyChanged event for IsNotReadOnly in the setter for the property IsReadOnly. With this you will make sure the UI gets updated correctly.
– Muhannad
Sep 6 at 21:24
add a comment |
up vote
0
down vote
I had an inversion problem, but a neat solution.
Motivation was that the XAML designer would show an empty control e.g. when there was no datacontext / no MyValues
(itemssource).
Initial code: hide control when MyValues
is empty.
Improved code: show control when MyValues
is NOT null or empty.
Ofcourse the problem is how to express '1 or more items', which is the opposite of 0 items.
<ListBox ItemsSource={Binding MyValues}">
<ListBox.Style x:Uid="F404D7B2-B7D3-11E7-A5A7-97680265A416">
<Style TargetType="{x:Type ListBox}">
<Style.Triggers>
<DataTrigger Binding="{Binding MyValues.Count}">
<Setter Property="Visibility" Value="Collapsed"/>
</DataTrigger>
</Style.Triggers>
</Style>
</ListBox.Style>
</ListBox>
I solved it by adding:
<DataTrigger Binding="{Binding MyValues.Count, FallbackValue=0, TargetNullValue=0}">
Ergo setting the default for the binding. Ofcourse this doesn't work for all kinds of inverse problems, but helped me out with clean code.
add a comment |
up vote
0
down vote
Following @Paul's answer, I wrote the following in the ViewModel:
public bool ShowAtView { get; set; }
public bool InvShowAtView { get { return !ShowAtView; } }
I hope having a snippet here will help someone, probably newbie as I am.
And if there's a mistake, please let me know!
BTW, I also agree with @heltonbiker comment - it's definitely the correct approach only if you don't have to use it more than 3 times...
add a comment |
up vote
0
down vote
I did something very similar. I created my property behind the scenes that enabled the selection of a combobox ONLY if it had finished searching for data. When my window first appears, it launches an async loaded command but I do not want the user to click on the combobox while it is still loading data (would be empty, then would be populated). So by default the property is false so I return the inverse in the getter. Then when I'm searching I set the property to true and back to false when complete.
private bool _isSearching;
public bool IsSearching
{
get { return !_isSearching; }
set
{
if(_isSearching != value)
{
_isSearching = value;
OnPropertyChanged("IsSearching");
}
}
}
public CityViewModel()
{
LoadedCommand = new DelegateCommandAsync(LoadCity, LoadCanExecute);
}
private async Task LoadCity(object pArg)
{
IsSearching = true;
//**Do your searching task here**
IsSearching = false;
}
private bool LoadCanExecute(object pArg)
{
return IsSearching;
}
Then for the combobox I can bind it directly to the IsSearching:
<ComboBox ItemsSource="{Binding Cities}" IsEnabled="{Binding IsSearching}" DisplayMemberPath="City" />
add a comment |
11 Answers
11
active
oldest
votes
11 Answers
11
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
413
down vote
accepted
You can use a ValueConverter that inverts a bool property for you.
XAML:
IsEnabled="{Binding Path=IsReadOnly, Converter={StaticResource InverseBooleanConverter}}"
Converter:
[ValueConversion(typeof(bool), typeof(bool))]
public class InverseBooleanConverter: IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
if (targetType != typeof(bool))
throw new InvalidOperationException("The target must be a boolean");
return !(bool)value;
}
public object ConvertBack(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
throw new NotSupportedException();
}
#endregion
}
6
There are a few things I have to consider here, that will likely make me pick @Paul's answer over this one. I am by myself when coding (for now), so I need to go with a solution that "I" will remember, which I will use over and over. I also feel that the less wordy something is the better, and creating an inverse property is very explicit, making it easy for me to remember, as well as future dev's ( I Hope, I Hope ), to be able to quickly see what I was doing, as well as making it easier for them to throw me under the proverbial bus.
– Russ
Jun 24 '09 at 18:07
14
By your own arguments, IMHO the converter solution is better in the long term : you only have to write the converter once, and after that you can reuse it over and over. If you go for the new property, you will have to rewrite it in every class that needs it...
– Thomas Levesque
Jun 26 '09 at 8:30
45
I'm using the same approach... but it makes panda saaad... =(
– Max Galkin
Jan 4 '10 at 21:34
20
Compared to!
, that’s some long-winded code... People go to insane amounts of effort to separate what they feel is "code" from those poor designers. Extra extra painful when I’m both the coder and the designer.
– Roman Starkov
Apr 14 '12 at 14:48
7
many people including myself would consider this a prime example of over-engineering. I suggest using a inverted property as in Paul Alexander post below.
– Christian Westman
Jan 14 '14 at 12:32
|
show 13 more comments
up vote
413
down vote
accepted
You can use a ValueConverter that inverts a bool property for you.
XAML:
IsEnabled="{Binding Path=IsReadOnly, Converter={StaticResource InverseBooleanConverter}}"
Converter:
[ValueConversion(typeof(bool), typeof(bool))]
public class InverseBooleanConverter: IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
if (targetType != typeof(bool))
throw new InvalidOperationException("The target must be a boolean");
return !(bool)value;
}
public object ConvertBack(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
throw new NotSupportedException();
}
#endregion
}
6
There are a few things I have to consider here, that will likely make me pick @Paul's answer over this one. I am by myself when coding (for now), so I need to go with a solution that "I" will remember, which I will use over and over. I also feel that the less wordy something is the better, and creating an inverse property is very explicit, making it easy for me to remember, as well as future dev's ( I Hope, I Hope ), to be able to quickly see what I was doing, as well as making it easier for them to throw me under the proverbial bus.
– Russ
Jun 24 '09 at 18:07
14
By your own arguments, IMHO the converter solution is better in the long term : you only have to write the converter once, and after that you can reuse it over and over. If you go for the new property, you will have to rewrite it in every class that needs it...
– Thomas Levesque
Jun 26 '09 at 8:30
45
I'm using the same approach... but it makes panda saaad... =(
– Max Galkin
Jan 4 '10 at 21:34
20
Compared to!
, that’s some long-winded code... People go to insane amounts of effort to separate what they feel is "code" from those poor designers. Extra extra painful when I’m both the coder and the designer.
– Roman Starkov
Apr 14 '12 at 14:48
7
many people including myself would consider this a prime example of over-engineering. I suggest using a inverted property as in Paul Alexander post below.
– Christian Westman
Jan 14 '14 at 12:32
|
show 13 more comments
up vote
413
down vote
accepted
up vote
413
down vote
accepted
You can use a ValueConverter that inverts a bool property for you.
XAML:
IsEnabled="{Binding Path=IsReadOnly, Converter={StaticResource InverseBooleanConverter}}"
Converter:
[ValueConversion(typeof(bool), typeof(bool))]
public class InverseBooleanConverter: IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
if (targetType != typeof(bool))
throw new InvalidOperationException("The target must be a boolean");
return !(bool)value;
}
public object ConvertBack(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
throw new NotSupportedException();
}
#endregion
}
You can use a ValueConverter that inverts a bool property for you.
XAML:
IsEnabled="{Binding Path=IsReadOnly, Converter={StaticResource InverseBooleanConverter}}"
Converter:
[ValueConversion(typeof(bool), typeof(bool))]
public class InverseBooleanConverter: IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
if (targetType != typeof(bool))
throw new InvalidOperationException("The target must be a boolean");
return !(bool)value;
}
public object ConvertBack(object value, Type targetType, object parameter,
System.Globalization.CultureInfo culture)
{
throw new NotSupportedException();
}
#endregion
}
answered Jun 24 '09 at 17:06
Chris Nicol
7,03853148
7,03853148
6
There are a few things I have to consider here, that will likely make me pick @Paul's answer over this one. I am by myself when coding (for now), so I need to go with a solution that "I" will remember, which I will use over and over. I also feel that the less wordy something is the better, and creating an inverse property is very explicit, making it easy for me to remember, as well as future dev's ( I Hope, I Hope ), to be able to quickly see what I was doing, as well as making it easier for them to throw me under the proverbial bus.
– Russ
Jun 24 '09 at 18:07
14
By your own arguments, IMHO the converter solution is better in the long term : you only have to write the converter once, and after that you can reuse it over and over. If you go for the new property, you will have to rewrite it in every class that needs it...
– Thomas Levesque
Jun 26 '09 at 8:30
45
I'm using the same approach... but it makes panda saaad... =(
– Max Galkin
Jan 4 '10 at 21:34
20
Compared to!
, that’s some long-winded code... People go to insane amounts of effort to separate what they feel is "code" from those poor designers. Extra extra painful when I’m both the coder and the designer.
– Roman Starkov
Apr 14 '12 at 14:48
7
many people including myself would consider this a prime example of over-engineering. I suggest using a inverted property as in Paul Alexander post below.
– Christian Westman
Jan 14 '14 at 12:32
|
show 13 more comments
6
There are a few things I have to consider here, that will likely make me pick @Paul's answer over this one. I am by myself when coding (for now), so I need to go with a solution that "I" will remember, which I will use over and over. I also feel that the less wordy something is the better, and creating an inverse property is very explicit, making it easy for me to remember, as well as future dev's ( I Hope, I Hope ), to be able to quickly see what I was doing, as well as making it easier for them to throw me under the proverbial bus.
– Russ
Jun 24 '09 at 18:07
14
By your own arguments, IMHO the converter solution is better in the long term : you only have to write the converter once, and after that you can reuse it over and over. If you go for the new property, you will have to rewrite it in every class that needs it...
– Thomas Levesque
Jun 26 '09 at 8:30
45
I'm using the same approach... but it makes panda saaad... =(
– Max Galkin
Jan 4 '10 at 21:34
20
Compared to!
, that’s some long-winded code... People go to insane amounts of effort to separate what they feel is "code" from those poor designers. Extra extra painful when I’m both the coder and the designer.
– Roman Starkov
Apr 14 '12 at 14:48
7
many people including myself would consider this a prime example of over-engineering. I suggest using a inverted property as in Paul Alexander post below.
– Christian Westman
Jan 14 '14 at 12:32
6
6
There are a few things I have to consider here, that will likely make me pick @Paul's answer over this one. I am by myself when coding (for now), so I need to go with a solution that "I" will remember, which I will use over and over. I also feel that the less wordy something is the better, and creating an inverse property is very explicit, making it easy for me to remember, as well as future dev's ( I Hope, I Hope ), to be able to quickly see what I was doing, as well as making it easier for them to throw me under the proverbial bus.
– Russ
Jun 24 '09 at 18:07
There are a few things I have to consider here, that will likely make me pick @Paul's answer over this one. I am by myself when coding (for now), so I need to go with a solution that "I" will remember, which I will use over and over. I also feel that the less wordy something is the better, and creating an inverse property is very explicit, making it easy for me to remember, as well as future dev's ( I Hope, I Hope ), to be able to quickly see what I was doing, as well as making it easier for them to throw me under the proverbial bus.
– Russ
Jun 24 '09 at 18:07
14
14
By your own arguments, IMHO the converter solution is better in the long term : you only have to write the converter once, and after that you can reuse it over and over. If you go for the new property, you will have to rewrite it in every class that needs it...
– Thomas Levesque
Jun 26 '09 at 8:30
By your own arguments, IMHO the converter solution is better in the long term : you only have to write the converter once, and after that you can reuse it over and over. If you go for the new property, you will have to rewrite it in every class that needs it...
– Thomas Levesque
Jun 26 '09 at 8:30
45
45
I'm using the same approach... but it makes panda saaad... =(
– Max Galkin
Jan 4 '10 at 21:34
I'm using the same approach... but it makes panda saaad... =(
– Max Galkin
Jan 4 '10 at 21:34
20
20
Compared to
!
, that’s some long-winded code... People go to insane amounts of effort to separate what they feel is "code" from those poor designers. Extra extra painful when I’m both the coder and the designer.– Roman Starkov
Apr 14 '12 at 14:48
Compared to
!
, that’s some long-winded code... People go to insane amounts of effort to separate what they feel is "code" from those poor designers. Extra extra painful when I’m both the coder and the designer.– Roman Starkov
Apr 14 '12 at 14:48
7
7
many people including myself would consider this a prime example of over-engineering. I suggest using a inverted property as in Paul Alexander post below.
– Christian Westman
Jan 14 '14 at 12:32
many people including myself would consider this a prime example of over-engineering. I suggest using a inverted property as in Paul Alexander post below.
– Christian Westman
Jan 14 '14 at 12:32
|
show 13 more comments
up vote
90
down vote
Have you considered a IsNotReadOnly property? If the object being bound is a ViewModel in a MVVM domain then the additional property makes perfect sense. If it's a direct Entity model, you might consider composition and presenting a specialized viewmodel of your entity to the form.
4
I just solved the same problem using this approach and I agree that not only is it more elegant, but much more maintainable than using a Converter.
– alimbada
Sep 24 '10 at 16:43
25
I would disagree that this approach is better than the value converter. It also produces more code if you need several NotProperty instances.
– Thiru
Jun 26 '12 at 0:31
22
MVVM isn't about not writing code, it's about solving problems declaratively. To that end, the converter is the correct solution.
– Jeff
Dec 31 '12 at 16:41
10
The problem with this solution is that if you have 100 objects, you would have to add an IsNotReadOnly property to all 100 objects. That property would have to be a DependencyProperty. That adds about 10 lines of code to all 100 objects or 1000 lines of code. The Converter is 20 lines of code. 1000 lines or 20 lines. Which would you choose?
– Rhyous
May 13 '14 at 20:59
6
There is a common saying for this: do it once, do it twice, and then automate. In doubt, I would use this answer the first time it's needed in a project, and then if things grow, I'd use the accepted answer. But having the converter snippet pre-made might make it way less difficult to use.
– heltonbiker
Feb 9 '15 at 17:54
|
show 2 more comments
up vote
90
down vote
Have you considered a IsNotReadOnly property? If the object being bound is a ViewModel in a MVVM domain then the additional property makes perfect sense. If it's a direct Entity model, you might consider composition and presenting a specialized viewmodel of your entity to the form.
4
I just solved the same problem using this approach and I agree that not only is it more elegant, but much more maintainable than using a Converter.
– alimbada
Sep 24 '10 at 16:43
25
I would disagree that this approach is better than the value converter. It also produces more code if you need several NotProperty instances.
– Thiru
Jun 26 '12 at 0:31
22
MVVM isn't about not writing code, it's about solving problems declaratively. To that end, the converter is the correct solution.
– Jeff
Dec 31 '12 at 16:41
10
The problem with this solution is that if you have 100 objects, you would have to add an IsNotReadOnly property to all 100 objects. That property would have to be a DependencyProperty. That adds about 10 lines of code to all 100 objects or 1000 lines of code. The Converter is 20 lines of code. 1000 lines or 20 lines. Which would you choose?
– Rhyous
May 13 '14 at 20:59
6
There is a common saying for this: do it once, do it twice, and then automate. In doubt, I would use this answer the first time it's needed in a project, and then if things grow, I'd use the accepted answer. But having the converter snippet pre-made might make it way less difficult to use.
– heltonbiker
Feb 9 '15 at 17:54
|
show 2 more comments
up vote
90
down vote
up vote
90
down vote
Have you considered a IsNotReadOnly property? If the object being bound is a ViewModel in a MVVM domain then the additional property makes perfect sense. If it's a direct Entity model, you might consider composition and presenting a specialized viewmodel of your entity to the form.
Have you considered a IsNotReadOnly property? If the object being bound is a ViewModel in a MVVM domain then the additional property makes perfect sense. If it's a direct Entity model, you might consider composition and presenting a specialized viewmodel of your entity to the form.
answered Jun 24 '09 at 17:07
Paul Alexander
24.9k1183139
24.9k1183139
4
I just solved the same problem using this approach and I agree that not only is it more elegant, but much more maintainable than using a Converter.
– alimbada
Sep 24 '10 at 16:43
25
I would disagree that this approach is better than the value converter. It also produces more code if you need several NotProperty instances.
– Thiru
Jun 26 '12 at 0:31
22
MVVM isn't about not writing code, it's about solving problems declaratively. To that end, the converter is the correct solution.
– Jeff
Dec 31 '12 at 16:41
10
The problem with this solution is that if you have 100 objects, you would have to add an IsNotReadOnly property to all 100 objects. That property would have to be a DependencyProperty. That adds about 10 lines of code to all 100 objects or 1000 lines of code. The Converter is 20 lines of code. 1000 lines or 20 lines. Which would you choose?
– Rhyous
May 13 '14 at 20:59
6
There is a common saying for this: do it once, do it twice, and then automate. In doubt, I would use this answer the first time it's needed in a project, and then if things grow, I'd use the accepted answer. But having the converter snippet pre-made might make it way less difficult to use.
– heltonbiker
Feb 9 '15 at 17:54
|
show 2 more comments
4
I just solved the same problem using this approach and I agree that not only is it more elegant, but much more maintainable than using a Converter.
– alimbada
Sep 24 '10 at 16:43
25
I would disagree that this approach is better than the value converter. It also produces more code if you need several NotProperty instances.
– Thiru
Jun 26 '12 at 0:31
22
MVVM isn't about not writing code, it's about solving problems declaratively. To that end, the converter is the correct solution.
– Jeff
Dec 31 '12 at 16:41
10
The problem with this solution is that if you have 100 objects, you would have to add an IsNotReadOnly property to all 100 objects. That property would have to be a DependencyProperty. That adds about 10 lines of code to all 100 objects or 1000 lines of code. The Converter is 20 lines of code. 1000 lines or 20 lines. Which would you choose?
– Rhyous
May 13 '14 at 20:59
6
There is a common saying for this: do it once, do it twice, and then automate. In doubt, I would use this answer the first time it's needed in a project, and then if things grow, I'd use the accepted answer. But having the converter snippet pre-made might make it way less difficult to use.
– heltonbiker
Feb 9 '15 at 17:54
4
4
I just solved the same problem using this approach and I agree that not only is it more elegant, but much more maintainable than using a Converter.
– alimbada
Sep 24 '10 at 16:43
I just solved the same problem using this approach and I agree that not only is it more elegant, but much more maintainable than using a Converter.
– alimbada
Sep 24 '10 at 16:43
25
25
I would disagree that this approach is better than the value converter. It also produces more code if you need several NotProperty instances.
– Thiru
Jun 26 '12 at 0:31
I would disagree that this approach is better than the value converter. It also produces more code if you need several NotProperty instances.
– Thiru
Jun 26 '12 at 0:31
22
22
MVVM isn't about not writing code, it's about solving problems declaratively. To that end, the converter is the correct solution.
– Jeff
Dec 31 '12 at 16:41
MVVM isn't about not writing code, it's about solving problems declaratively. To that end, the converter is the correct solution.
– Jeff
Dec 31 '12 at 16:41
10
10
The problem with this solution is that if you have 100 objects, you would have to add an IsNotReadOnly property to all 100 objects. That property would have to be a DependencyProperty. That adds about 10 lines of code to all 100 objects or 1000 lines of code. The Converter is 20 lines of code. 1000 lines or 20 lines. Which would you choose?
– Rhyous
May 13 '14 at 20:59
The problem with this solution is that if you have 100 objects, you would have to add an IsNotReadOnly property to all 100 objects. That property would have to be a DependencyProperty. That adds about 10 lines of code to all 100 objects or 1000 lines of code. The Converter is 20 lines of code. 1000 lines or 20 lines. Which would you choose?
– Rhyous
May 13 '14 at 20:59
6
6
There is a common saying for this: do it once, do it twice, and then automate. In doubt, I would use this answer the first time it's needed in a project, and then if things grow, I'd use the accepted answer. But having the converter snippet pre-made might make it way less difficult to use.
– heltonbiker
Feb 9 '15 at 17:54
There is a common saying for this: do it once, do it twice, and then automate. In doubt, I would use this answer the first time it's needed in a project, and then if things grow, I'd use the accepted answer. But having the converter snippet pre-made might make it way less difficult to use.
– heltonbiker
Feb 9 '15 at 17:54
|
show 2 more comments
up vote
53
down vote
With standart binding you need to use converters that looks little windy. So, I recommend you to look at my project CalcBinding, which was developed specially to resolve this problem and some others. With advanced binding you can write expressions with many source properties directly in xaml. Say, you can write something like:
<Button IsEnabled="{c:Binding Path=!IsReadOnly}" />
or
<Button Content="{c:Binding ElementName=grid, Path=ActualWidth+Height}"/>
or
<Label Content="{c:Binding A+B+C }" />
or
<Button Visibility="{c:Binding IsChecked, FalseToVisibility=Hidden}" />
where A, B, C, IsChecked - properties of viewModel and it will work properly
Goodluck!
5
Although QuickConverter is more powerful, I find the CalcBinding mode readable - usable.
– xmedeko
Jun 5 '15 at 12:49
This is a great tool. I wish it existed 5 years ago!
– jugg1es
Mar 6 at 16:39
add a comment |
up vote
53
down vote
With standart binding you need to use converters that looks little windy. So, I recommend you to look at my project CalcBinding, which was developed specially to resolve this problem and some others. With advanced binding you can write expressions with many source properties directly in xaml. Say, you can write something like:
<Button IsEnabled="{c:Binding Path=!IsReadOnly}" />
or
<Button Content="{c:Binding ElementName=grid, Path=ActualWidth+Height}"/>
or
<Label Content="{c:Binding A+B+C }" />
or
<Button Visibility="{c:Binding IsChecked, FalseToVisibility=Hidden}" />
where A, B, C, IsChecked - properties of viewModel and it will work properly
Goodluck!
5
Although QuickConverter is more powerful, I find the CalcBinding mode readable - usable.
– xmedeko
Jun 5 '15 at 12:49
This is a great tool. I wish it existed 5 years ago!
– jugg1es
Mar 6 at 16:39
add a comment |
up vote
53
down vote
up vote
53
down vote
With standart binding you need to use converters that looks little windy. So, I recommend you to look at my project CalcBinding, which was developed specially to resolve this problem and some others. With advanced binding you can write expressions with many source properties directly in xaml. Say, you can write something like:
<Button IsEnabled="{c:Binding Path=!IsReadOnly}" />
or
<Button Content="{c:Binding ElementName=grid, Path=ActualWidth+Height}"/>
or
<Label Content="{c:Binding A+B+C }" />
or
<Button Visibility="{c:Binding IsChecked, FalseToVisibility=Hidden}" />
where A, B, C, IsChecked - properties of viewModel and it will work properly
Goodluck!
With standart binding you need to use converters that looks little windy. So, I recommend you to look at my project CalcBinding, which was developed specially to resolve this problem and some others. With advanced binding you can write expressions with many source properties directly in xaml. Say, you can write something like:
<Button IsEnabled="{c:Binding Path=!IsReadOnly}" />
or
<Button Content="{c:Binding ElementName=grid, Path=ActualWidth+Height}"/>
or
<Label Content="{c:Binding A+B+C }" />
or
<Button Visibility="{c:Binding IsChecked, FalseToVisibility=Hidden}" />
where A, B, C, IsChecked - properties of viewModel and it will work properly
Goodluck!
edited Jul 6 '15 at 10:56
answered Dec 5 '14 at 21:14
Alex141
71869
71869
5
Although QuickConverter is more powerful, I find the CalcBinding mode readable - usable.
– xmedeko
Jun 5 '15 at 12:49
This is a great tool. I wish it existed 5 years ago!
– jugg1es
Mar 6 at 16:39
add a comment |
5
Although QuickConverter is more powerful, I find the CalcBinding mode readable - usable.
– xmedeko
Jun 5 '15 at 12:49
This is a great tool. I wish it existed 5 years ago!
– jugg1es
Mar 6 at 16:39
5
5
Although QuickConverter is more powerful, I find the CalcBinding mode readable - usable.
– xmedeko
Jun 5 '15 at 12:49
Although QuickConverter is more powerful, I find the CalcBinding mode readable - usable.
– xmedeko
Jun 5 '15 at 12:49
This is a great tool. I wish it existed 5 years ago!
– jugg1es
Mar 6 at 16:39
This is a great tool. I wish it existed 5 years ago!
– jugg1es
Mar 6 at 16:39
add a comment |
up vote
16
down vote
I would recommend using https://quickconverter.codeplex.com/
Inverting a boolean is then as simple as:
<Button IsEnabled="{qc:Binding '!$P', P={Binding IsReadOnly}}" />
That speeds the time normally needed to write converters.
12
When giving a -1 to someone, it would be nice to explain why.
– Noxxys
Oct 20 '14 at 10:29
add a comment |
up vote
16
down vote
I would recommend using https://quickconverter.codeplex.com/
Inverting a boolean is then as simple as:
<Button IsEnabled="{qc:Binding '!$P', P={Binding IsReadOnly}}" />
That speeds the time normally needed to write converters.
12
When giving a -1 to someone, it would be nice to explain why.
– Noxxys
Oct 20 '14 at 10:29
add a comment |
up vote
16
down vote
up vote
16
down vote
I would recommend using https://quickconverter.codeplex.com/
Inverting a boolean is then as simple as:
<Button IsEnabled="{qc:Binding '!$P', P={Binding IsReadOnly}}" />
That speeds the time normally needed to write converters.
I would recommend using https://quickconverter.codeplex.com/
Inverting a boolean is then as simple as:
<Button IsEnabled="{qc:Binding '!$P', P={Binding IsReadOnly}}" />
That speeds the time normally needed to write converters.
edited Nov 25 '14 at 9:57
answered Aug 7 '14 at 13:28
Noxxys
4511416
4511416
12
When giving a -1 to someone, it would be nice to explain why.
– Noxxys
Oct 20 '14 at 10:29
add a comment |
12
When giving a -1 to someone, it would be nice to explain why.
– Noxxys
Oct 20 '14 at 10:29
12
12
When giving a -1 to someone, it would be nice to explain why.
– Noxxys
Oct 20 '14 at 10:29
When giving a -1 to someone, it would be nice to explain why.
– Noxxys
Oct 20 '14 at 10:29
add a comment |
up vote
11
down vote
I wanted my XAML to remain as elegant as possible so I created a class to wrap the bool which resides in one of my shared libraries, the implicit operators allow the class to be used as a bool in code-behind seamlessly
public class InvertableBool
{
private bool value = false;
public bool Value { get { return value; } }
public bool Invert { get { return !value; } }
public InvertableBool(bool b)
{
value = b;
}
public static implicit operator InvertableBool(bool b)
{
return new InvertableBool(b);
}
public static implicit operator bool(InvertableBool b)
{
return b.value;
}
}
The only changes needed to your project are to make the property you want to invert return this instead of bool
public InvertableBool IsActive
{
get
{
return true;
}
}
And in the XAML postfix the binding with either Value or Invert
IsEnabled="{Binding IsActive.Value}"
IsEnabled="{Binding IsActive.Invert}"
Downside is that you'd have to change all code that compared it with / assigned it to otherbool
Type Expressions / Variables even not referencing the inverse value. I would instead add a "Not" Extension Method to theBoolean
Struct
.
– Tom
Jan 18 at 0:16
Doh! Never mind. Forgot had to beProperty
vs.Method
forBinding
. My "Downside" statement still applies. Btw, the 'Boolean` "Not" Extension Method is still useful for avoiding the "!" Operator which is easily missed when it (as is often the case) is embedded next to chars that look like it (i.e. one/more "("'s and "l"'s and "I"'s).
– Tom
Jan 18 at 1:54
add a comment |
up vote
11
down vote
I wanted my XAML to remain as elegant as possible so I created a class to wrap the bool which resides in one of my shared libraries, the implicit operators allow the class to be used as a bool in code-behind seamlessly
public class InvertableBool
{
private bool value = false;
public bool Value { get { return value; } }
public bool Invert { get { return !value; } }
public InvertableBool(bool b)
{
value = b;
}
public static implicit operator InvertableBool(bool b)
{
return new InvertableBool(b);
}
public static implicit operator bool(InvertableBool b)
{
return b.value;
}
}
The only changes needed to your project are to make the property you want to invert return this instead of bool
public InvertableBool IsActive
{
get
{
return true;
}
}
And in the XAML postfix the binding with either Value or Invert
IsEnabled="{Binding IsActive.Value}"
IsEnabled="{Binding IsActive.Invert}"
Downside is that you'd have to change all code that compared it with / assigned it to otherbool
Type Expressions / Variables even not referencing the inverse value. I would instead add a "Not" Extension Method to theBoolean
Struct
.
– Tom
Jan 18 at 0:16
Doh! Never mind. Forgot had to beProperty
vs.Method
forBinding
. My "Downside" statement still applies. Btw, the 'Boolean` "Not" Extension Method is still useful for avoiding the "!" Operator which is easily missed when it (as is often the case) is embedded next to chars that look like it (i.e. one/more "("'s and "l"'s and "I"'s).
– Tom
Jan 18 at 1:54
add a comment |
up vote
11
down vote
up vote
11
down vote
I wanted my XAML to remain as elegant as possible so I created a class to wrap the bool which resides in one of my shared libraries, the implicit operators allow the class to be used as a bool in code-behind seamlessly
public class InvertableBool
{
private bool value = false;
public bool Value { get { return value; } }
public bool Invert { get { return !value; } }
public InvertableBool(bool b)
{
value = b;
}
public static implicit operator InvertableBool(bool b)
{
return new InvertableBool(b);
}
public static implicit operator bool(InvertableBool b)
{
return b.value;
}
}
The only changes needed to your project are to make the property you want to invert return this instead of bool
public InvertableBool IsActive
{
get
{
return true;
}
}
And in the XAML postfix the binding with either Value or Invert
IsEnabled="{Binding IsActive.Value}"
IsEnabled="{Binding IsActive.Invert}"
I wanted my XAML to remain as elegant as possible so I created a class to wrap the bool which resides in one of my shared libraries, the implicit operators allow the class to be used as a bool in code-behind seamlessly
public class InvertableBool
{
private bool value = false;
public bool Value { get { return value; } }
public bool Invert { get { return !value; } }
public InvertableBool(bool b)
{
value = b;
}
public static implicit operator InvertableBool(bool b)
{
return new InvertableBool(b);
}
public static implicit operator bool(InvertableBool b)
{
return b.value;
}
}
The only changes needed to your project are to make the property you want to invert return this instead of bool
public InvertableBool IsActive
{
get
{
return true;
}
}
And in the XAML postfix the binding with either Value or Invert
IsEnabled="{Binding IsActive.Value}"
IsEnabled="{Binding IsActive.Invert}"
answered Aug 4 '15 at 10:07
jevansio
11112
11112
Downside is that you'd have to change all code that compared it with / assigned it to otherbool
Type Expressions / Variables even not referencing the inverse value. I would instead add a "Not" Extension Method to theBoolean
Struct
.
– Tom
Jan 18 at 0:16
Doh! Never mind. Forgot had to beProperty
vs.Method
forBinding
. My "Downside" statement still applies. Btw, the 'Boolean` "Not" Extension Method is still useful for avoiding the "!" Operator which is easily missed when it (as is often the case) is embedded next to chars that look like it (i.e. one/more "("'s and "l"'s and "I"'s).
– Tom
Jan 18 at 1:54
add a comment |
Downside is that you'd have to change all code that compared it with / assigned it to otherbool
Type Expressions / Variables even not referencing the inverse value. I would instead add a "Not" Extension Method to theBoolean
Struct
.
– Tom
Jan 18 at 0:16
Doh! Never mind. Forgot had to beProperty
vs.Method
forBinding
. My "Downside" statement still applies. Btw, the 'Boolean` "Not" Extension Method is still useful for avoiding the "!" Operator which is easily missed when it (as is often the case) is embedded next to chars that look like it (i.e. one/more "("'s and "l"'s and "I"'s).
– Tom
Jan 18 at 1:54
Downside is that you'd have to change all code that compared it with / assigned it to other
bool
Type Expressions / Variables even not referencing the inverse value. I would instead add a "Not" Extension Method to the Boolean
Struct
.– Tom
Jan 18 at 0:16
Downside is that you'd have to change all code that compared it with / assigned it to other
bool
Type Expressions / Variables even not referencing the inverse value. I would instead add a "Not" Extension Method to the Boolean
Struct
.– Tom
Jan 18 at 0:16
Doh! Never mind. Forgot had to be
Property
vs. Method
for Binding
. My "Downside" statement still applies. Btw, the 'Boolean` "Not" Extension Method is still useful for avoiding the "!" Operator which is easily missed when it (as is often the case) is embedded next to chars that look like it (i.e. one/more "("'s and "l"'s and "I"'s).– Tom
Jan 18 at 1:54
Doh! Never mind. Forgot had to be
Property
vs. Method
for Binding
. My "Downside" statement still applies. Btw, the 'Boolean` "Not" Extension Method is still useful for avoiding the "!" Operator which is easily missed when it (as is often the case) is embedded next to chars that look like it (i.e. one/more "("'s and "l"'s and "I"'s).– Tom
Jan 18 at 1:54
add a comment |
up vote
7
down vote
This one also works for nullable bools.
[ValueConversion(typeof(bool?), typeof(bool))]
public class InverseBooleanConverter : IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (targetType != typeof(bool?))
{
throw new InvalidOperationException("The target must be a nullable boolean");
}
bool? b = (bool?)value;
return b.HasValue && !b.Value;
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
return !(value as bool?);
}
#endregion
}
add a comment |
up vote
7
down vote
This one also works for nullable bools.
[ValueConversion(typeof(bool?), typeof(bool))]
public class InverseBooleanConverter : IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (targetType != typeof(bool?))
{
throw new InvalidOperationException("The target must be a nullable boolean");
}
bool? b = (bool?)value;
return b.HasValue && !b.Value;
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
return !(value as bool?);
}
#endregion
}
add a comment |
up vote
7
down vote
up vote
7
down vote
This one also works for nullable bools.
[ValueConversion(typeof(bool?), typeof(bool))]
public class InverseBooleanConverter : IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (targetType != typeof(bool?))
{
throw new InvalidOperationException("The target must be a nullable boolean");
}
bool? b = (bool?)value;
return b.HasValue && !b.Value;
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
return !(value as bool?);
}
#endregion
}
This one also works for nullable bools.
[ValueConversion(typeof(bool?), typeof(bool))]
public class InverseBooleanConverter : IValueConverter
{
#region IValueConverter Members
public object Convert(object value, Type targetType, object parameter, CultureInfo culture)
{
if (targetType != typeof(bool?))
{
throw new InvalidOperationException("The target must be a nullable boolean");
}
bool? b = (bool?)value;
return b.HasValue && !b.Value;
}
public object ConvertBack(object value, Type targetType, object parameter, System.Globalization.CultureInfo culture)
{
return !(value as bool?);
}
#endregion
}
answered Mar 23 '16 at 11:59
Andreas
2,18612443
2,18612443
add a comment |
add a comment |
up vote
1
down vote
Don't know if this is relevant to XAML, but in my simple Windows app I created the binding manually and added a Format event handler.
public FormMain() {
InitializeComponent();
Binding argBinding = new Binding("Enabled", uxCheckBoxArgsNull, "Checked", false, DataSourceUpdateMode.OnPropertyChanged);
argBinding.Format += new ConvertEventHandler(Binding_Format_BooleanInverse);
uxTextBoxArgs.DataBindings.Add(argBinding);
}
void Binding_Format_BooleanInverse(object sender, ConvertEventArgs e) {
bool boolValue = (bool)e.Value;
e.Value = !boolValue;
}
Seems pretty much the same than the converter approach.Format
andParse
events in WinForms bindings are roughly equivalent of the WPF converter.
– Alejandro
Jan 21 '16 at 13:15
add a comment |
up vote
1
down vote
Don't know if this is relevant to XAML, but in my simple Windows app I created the binding manually and added a Format event handler.
public FormMain() {
InitializeComponent();
Binding argBinding = new Binding("Enabled", uxCheckBoxArgsNull, "Checked", false, DataSourceUpdateMode.OnPropertyChanged);
argBinding.Format += new ConvertEventHandler(Binding_Format_BooleanInverse);
uxTextBoxArgs.DataBindings.Add(argBinding);
}
void Binding_Format_BooleanInverse(object sender, ConvertEventArgs e) {
bool boolValue = (bool)e.Value;
e.Value = !boolValue;
}
Seems pretty much the same than the converter approach.Format
andParse
events in WinForms bindings are roughly equivalent of the WPF converter.
– Alejandro
Jan 21 '16 at 13:15
add a comment |
up vote
1
down vote
up vote
1
down vote
Don't know if this is relevant to XAML, but in my simple Windows app I created the binding manually and added a Format event handler.
public FormMain() {
InitializeComponent();
Binding argBinding = new Binding("Enabled", uxCheckBoxArgsNull, "Checked", false, DataSourceUpdateMode.OnPropertyChanged);
argBinding.Format += new ConvertEventHandler(Binding_Format_BooleanInverse);
uxTextBoxArgs.DataBindings.Add(argBinding);
}
void Binding_Format_BooleanInverse(object sender, ConvertEventArgs e) {
bool boolValue = (bool)e.Value;
e.Value = !boolValue;
}
Don't know if this is relevant to XAML, but in my simple Windows app I created the binding manually and added a Format event handler.
public FormMain() {
InitializeComponent();
Binding argBinding = new Binding("Enabled", uxCheckBoxArgsNull, "Checked", false, DataSourceUpdateMode.OnPropertyChanged);
argBinding.Format += new ConvertEventHandler(Binding_Format_BooleanInverse);
uxTextBoxArgs.DataBindings.Add(argBinding);
}
void Binding_Format_BooleanInverse(object sender, ConvertEventArgs e) {
bool boolValue = (bool)e.Value;
e.Value = !boolValue;
}
answered Mar 4 '15 at 16:35


Simon Dobson
111
111
Seems pretty much the same than the converter approach.Format
andParse
events in WinForms bindings are roughly equivalent of the WPF converter.
– Alejandro
Jan 21 '16 at 13:15
add a comment |
Seems pretty much the same than the converter approach.Format
andParse
events in WinForms bindings are roughly equivalent of the WPF converter.
– Alejandro
Jan 21 '16 at 13:15
Seems pretty much the same than the converter approach.
Format
and Parse
events in WinForms bindings are roughly equivalent of the WPF converter.– Alejandro
Jan 21 '16 at 13:15
Seems pretty much the same than the converter approach.
Format
and Parse
events in WinForms bindings are roughly equivalent of the WPF converter.– Alejandro
Jan 21 '16 at 13:15
add a comment |
up vote
0
down vote
Add one more property in your view model, which will return reverse value.
And bind that to button.
Like;
in view model:
public bool IsNotReadOnly{get{return !IsReadOnly;}}
in xaml:
IsEnabled="{Binding IsNotReadOnly"}
Great answer. One thing to add, using this you better rise the PropertyChanged event for IsNotReadOnly in the setter for the property IsReadOnly. With this you will make sure the UI gets updated correctly.
– Muhannad
Sep 6 at 21:24
add a comment |
up vote
0
down vote
Add one more property in your view model, which will return reverse value.
And bind that to button.
Like;
in view model:
public bool IsNotReadOnly{get{return !IsReadOnly;}}
in xaml:
IsEnabled="{Binding IsNotReadOnly"}
Great answer. One thing to add, using this you better rise the PropertyChanged event for IsNotReadOnly in the setter for the property IsReadOnly. With this you will make sure the UI gets updated correctly.
– Muhannad
Sep 6 at 21:24
add a comment |
up vote
0
down vote
up vote
0
down vote
Add one more property in your view model, which will return reverse value.
And bind that to button.
Like;
in view model:
public bool IsNotReadOnly{get{return !IsReadOnly;}}
in xaml:
IsEnabled="{Binding IsNotReadOnly"}
Add one more property in your view model, which will return reverse value.
And bind that to button.
Like;
in view model:
public bool IsNotReadOnly{get{return !IsReadOnly;}}
in xaml:
IsEnabled="{Binding IsNotReadOnly"}
answered Sep 8 '17 at 13:58
MMM
13
13
Great answer. One thing to add, using this you better rise the PropertyChanged event for IsNotReadOnly in the setter for the property IsReadOnly. With this you will make sure the UI gets updated correctly.
– Muhannad
Sep 6 at 21:24
add a comment |
Great answer. One thing to add, using this you better rise the PropertyChanged event for IsNotReadOnly in the setter for the property IsReadOnly. With this you will make sure the UI gets updated correctly.
– Muhannad
Sep 6 at 21:24
Great answer. One thing to add, using this you better rise the PropertyChanged event for IsNotReadOnly in the setter for the property IsReadOnly. With this you will make sure the UI gets updated correctly.
– Muhannad
Sep 6 at 21:24
Great answer. One thing to add, using this you better rise the PropertyChanged event for IsNotReadOnly in the setter for the property IsReadOnly. With this you will make sure the UI gets updated correctly.
– Muhannad
Sep 6 at 21:24
add a comment |
up vote
0
down vote
I had an inversion problem, but a neat solution.
Motivation was that the XAML designer would show an empty control e.g. when there was no datacontext / no MyValues
(itemssource).
Initial code: hide control when MyValues
is empty.
Improved code: show control when MyValues
is NOT null or empty.
Ofcourse the problem is how to express '1 or more items', which is the opposite of 0 items.
<ListBox ItemsSource={Binding MyValues}">
<ListBox.Style x:Uid="F404D7B2-B7D3-11E7-A5A7-97680265A416">
<Style TargetType="{x:Type ListBox}">
<Style.Triggers>
<DataTrigger Binding="{Binding MyValues.Count}">
<Setter Property="Visibility" Value="Collapsed"/>
</DataTrigger>
</Style.Triggers>
</Style>
</ListBox.Style>
</ListBox>
I solved it by adding:
<DataTrigger Binding="{Binding MyValues.Count, FallbackValue=0, TargetNullValue=0}">
Ergo setting the default for the binding. Ofcourse this doesn't work for all kinds of inverse problems, but helped me out with clean code.
add a comment |
up vote
0
down vote
I had an inversion problem, but a neat solution.
Motivation was that the XAML designer would show an empty control e.g. when there was no datacontext / no MyValues
(itemssource).
Initial code: hide control when MyValues
is empty.
Improved code: show control when MyValues
is NOT null or empty.
Ofcourse the problem is how to express '1 or more items', which is the opposite of 0 items.
<ListBox ItemsSource={Binding MyValues}">
<ListBox.Style x:Uid="F404D7B2-B7D3-11E7-A5A7-97680265A416">
<Style TargetType="{x:Type ListBox}">
<Style.Triggers>
<DataTrigger Binding="{Binding MyValues.Count}">
<Setter Property="Visibility" Value="Collapsed"/>
</DataTrigger>
</Style.Triggers>
</Style>
</ListBox.Style>
</ListBox>
I solved it by adding:
<DataTrigger Binding="{Binding MyValues.Count, FallbackValue=0, TargetNullValue=0}">
Ergo setting the default for the binding. Ofcourse this doesn't work for all kinds of inverse problems, but helped me out with clean code.
add a comment |
up vote
0
down vote
up vote
0
down vote
I had an inversion problem, but a neat solution.
Motivation was that the XAML designer would show an empty control e.g. when there was no datacontext / no MyValues
(itemssource).
Initial code: hide control when MyValues
is empty.
Improved code: show control when MyValues
is NOT null or empty.
Ofcourse the problem is how to express '1 or more items', which is the opposite of 0 items.
<ListBox ItemsSource={Binding MyValues}">
<ListBox.Style x:Uid="F404D7B2-B7D3-11E7-A5A7-97680265A416">
<Style TargetType="{x:Type ListBox}">
<Style.Triggers>
<DataTrigger Binding="{Binding MyValues.Count}">
<Setter Property="Visibility" Value="Collapsed"/>
</DataTrigger>
</Style.Triggers>
</Style>
</ListBox.Style>
</ListBox>
I solved it by adding:
<DataTrigger Binding="{Binding MyValues.Count, FallbackValue=0, TargetNullValue=0}">
Ergo setting the default for the binding. Ofcourse this doesn't work for all kinds of inverse problems, but helped me out with clean code.
I had an inversion problem, but a neat solution.
Motivation was that the XAML designer would show an empty control e.g. when there was no datacontext / no MyValues
(itemssource).
Initial code: hide control when MyValues
is empty.
Improved code: show control when MyValues
is NOT null or empty.
Ofcourse the problem is how to express '1 or more items', which is the opposite of 0 items.
<ListBox ItemsSource={Binding MyValues}">
<ListBox.Style x:Uid="F404D7B2-B7D3-11E7-A5A7-97680265A416">
<Style TargetType="{x:Type ListBox}">
<Style.Triggers>
<DataTrigger Binding="{Binding MyValues.Count}">
<Setter Property="Visibility" Value="Collapsed"/>
</DataTrigger>
</Style.Triggers>
</Style>
</ListBox.Style>
</ListBox>
I solved it by adding:
<DataTrigger Binding="{Binding MyValues.Count, FallbackValue=0, TargetNullValue=0}">
Ergo setting the default for the binding. Ofcourse this doesn't work for all kinds of inverse problems, but helped me out with clean code.
answered Oct 26 '17 at 7:39
EricG
2,96111528
2,96111528
add a comment |
add a comment |
up vote
0
down vote
Following @Paul's answer, I wrote the following in the ViewModel:
public bool ShowAtView { get; set; }
public bool InvShowAtView { get { return !ShowAtView; } }
I hope having a snippet here will help someone, probably newbie as I am.
And if there's a mistake, please let me know!
BTW, I also agree with @heltonbiker comment - it's definitely the correct approach only if you don't have to use it more than 3 times...
add a comment |
up vote
0
down vote
Following @Paul's answer, I wrote the following in the ViewModel:
public bool ShowAtView { get; set; }
public bool InvShowAtView { get { return !ShowAtView; } }
I hope having a snippet here will help someone, probably newbie as I am.
And if there's a mistake, please let me know!
BTW, I also agree with @heltonbiker comment - it's definitely the correct approach only if you don't have to use it more than 3 times...
add a comment |
up vote
0
down vote
up vote
0
down vote
Following @Paul's answer, I wrote the following in the ViewModel:
public bool ShowAtView { get; set; }
public bool InvShowAtView { get { return !ShowAtView; } }
I hope having a snippet here will help someone, probably newbie as I am.
And if there's a mistake, please let me know!
BTW, I also agree with @heltonbiker comment - it's definitely the correct approach only if you don't have to use it more than 3 times...
Following @Paul's answer, I wrote the following in the ViewModel:
public bool ShowAtView { get; set; }
public bool InvShowAtView { get { return !ShowAtView; } }
I hope having a snippet here will help someone, probably newbie as I am.
And if there's a mistake, please let me know!
BTW, I also agree with @heltonbiker comment - it's definitely the correct approach only if you don't have to use it more than 3 times...
answered Jan 9 at 10:37
Ofaim
62
62
add a comment |
add a comment |
up vote
0
down vote
I did something very similar. I created my property behind the scenes that enabled the selection of a combobox ONLY if it had finished searching for data. When my window first appears, it launches an async loaded command but I do not want the user to click on the combobox while it is still loading data (would be empty, then would be populated). So by default the property is false so I return the inverse in the getter. Then when I'm searching I set the property to true and back to false when complete.
private bool _isSearching;
public bool IsSearching
{
get { return !_isSearching; }
set
{
if(_isSearching != value)
{
_isSearching = value;
OnPropertyChanged("IsSearching");
}
}
}
public CityViewModel()
{
LoadedCommand = new DelegateCommandAsync(LoadCity, LoadCanExecute);
}
private async Task LoadCity(object pArg)
{
IsSearching = true;
//**Do your searching task here**
IsSearching = false;
}
private bool LoadCanExecute(object pArg)
{
return IsSearching;
}
Then for the combobox I can bind it directly to the IsSearching:
<ComboBox ItemsSource="{Binding Cities}" IsEnabled="{Binding IsSearching}" DisplayMemberPath="City" />
add a comment |
up vote
0
down vote
I did something very similar. I created my property behind the scenes that enabled the selection of a combobox ONLY if it had finished searching for data. When my window first appears, it launches an async loaded command but I do not want the user to click on the combobox while it is still loading data (would be empty, then would be populated). So by default the property is false so I return the inverse in the getter. Then when I'm searching I set the property to true and back to false when complete.
private bool _isSearching;
public bool IsSearching
{
get { return !_isSearching; }
set
{
if(_isSearching != value)
{
_isSearching = value;
OnPropertyChanged("IsSearching");
}
}
}
public CityViewModel()
{
LoadedCommand = new DelegateCommandAsync(LoadCity, LoadCanExecute);
}
private async Task LoadCity(object pArg)
{
IsSearching = true;
//**Do your searching task here**
IsSearching = false;
}
private bool LoadCanExecute(object pArg)
{
return IsSearching;
}
Then for the combobox I can bind it directly to the IsSearching:
<ComboBox ItemsSource="{Binding Cities}" IsEnabled="{Binding IsSearching}" DisplayMemberPath="City" />
add a comment |
up vote
0
down vote
up vote
0
down vote
I did something very similar. I created my property behind the scenes that enabled the selection of a combobox ONLY if it had finished searching for data. When my window first appears, it launches an async loaded command but I do not want the user to click on the combobox while it is still loading data (would be empty, then would be populated). So by default the property is false so I return the inverse in the getter. Then when I'm searching I set the property to true and back to false when complete.
private bool _isSearching;
public bool IsSearching
{
get { return !_isSearching; }
set
{
if(_isSearching != value)
{
_isSearching = value;
OnPropertyChanged("IsSearching");
}
}
}
public CityViewModel()
{
LoadedCommand = new DelegateCommandAsync(LoadCity, LoadCanExecute);
}
private async Task LoadCity(object pArg)
{
IsSearching = true;
//**Do your searching task here**
IsSearching = false;
}
private bool LoadCanExecute(object pArg)
{
return IsSearching;
}
Then for the combobox I can bind it directly to the IsSearching:
<ComboBox ItemsSource="{Binding Cities}" IsEnabled="{Binding IsSearching}" DisplayMemberPath="City" />
I did something very similar. I created my property behind the scenes that enabled the selection of a combobox ONLY if it had finished searching for data. When my window first appears, it launches an async loaded command but I do not want the user to click on the combobox while it is still loading data (would be empty, then would be populated). So by default the property is false so I return the inverse in the getter. Then when I'm searching I set the property to true and back to false when complete.
private bool _isSearching;
public bool IsSearching
{
get { return !_isSearching; }
set
{
if(_isSearching != value)
{
_isSearching = value;
OnPropertyChanged("IsSearching");
}
}
}
public CityViewModel()
{
LoadedCommand = new DelegateCommandAsync(LoadCity, LoadCanExecute);
}
private async Task LoadCity(object pArg)
{
IsSearching = true;
//**Do your searching task here**
IsSearching = false;
}
private bool LoadCanExecute(object pArg)
{
return IsSearching;
}
Then for the combobox I can bind it directly to the IsSearching:
<ComboBox ItemsSource="{Binding Cities}" IsEnabled="{Binding IsSearching}" DisplayMemberPath="City" />
answered Feb 5 at 19:18


GregN
3410
3410
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f1039636%2fhow-to-bind-inverse-boolean-properties-in-wpf%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zWbW4X0zk2r55hD6Z dCXLtb69W3te34ovJOqUsf TfKz3,w6obdJ1vmZYU9XLK,uIsARkCmW8YEJOIFT mJ PRE aG