Terminating docker container on SIGTERM on warping script
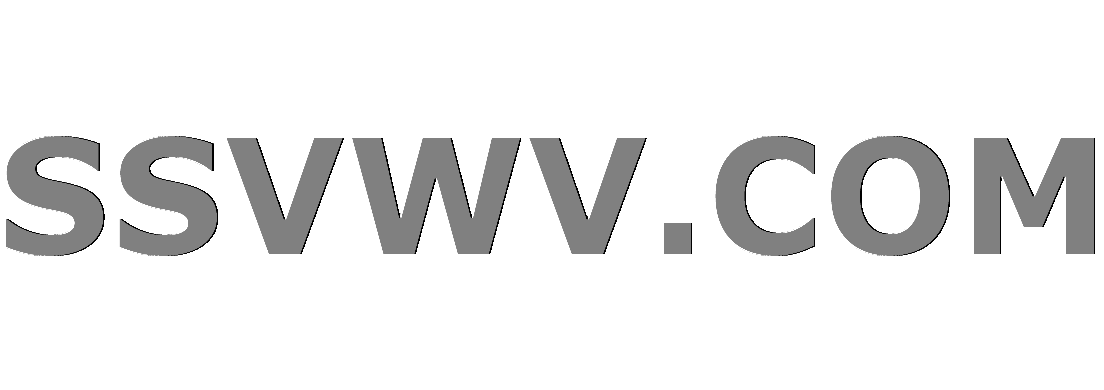
Multi tool use
up vote
0
down vote
favorite
I have the following Python script that warps a docker container run:
import subprocess
import sys
import signal
container_id = None
def catch_term(signum, frame):
sys.stderr.write('Caught SIGTERM. Stopping containern')
if container_id != None:
cmd = ['docker', 'rm', '-f', container_id]
subprocess.call(cmd)
sys.exit(143)
signal.signal(signal.SIGTERM, catch_term)
cmd = ['docker', 'run', '--cidfile', cidfile, '-i', image_name, container_process_cmd]
p = subprocess.Popen(cmd)
# This function waits for the file to contain 64 bytes and then reads the container id from it
container_id = get_container_id_from_cidfile(cidfile)
p.communicate()
sys.exit(p.returncode)
When I run the Python process on interactive mode, and kill that warping script during the run (kill <PID>
), the catch_term
function is called and the container is removed. Then my process exits.
However, when I run the warping process on the background, i.e. my_warping_script_above.py &
, and kill it in the same way, the catch_term
function is not called at all and my script keeps running, including the container.
Any idea how to make the
catch_term
function be called even when the script runs on background?I think it has something to do with the fact that
docker run
is a "protected" process. It should only be able to get killed using a docker command, such asdocker rm -f <CONTAINER ID>
, or usingCtrl+C
if called with-i
. That's the reason why I'm trying to catch the TERM signal and calldocker rm
, but my function is not called when the script runs on background... (I think it tries to first kill the child process, then as it fails, it does not reach to stage of calling my registered function).
python docker subprocess background-process
add a comment |
up vote
0
down vote
favorite
I have the following Python script that warps a docker container run:
import subprocess
import sys
import signal
container_id = None
def catch_term(signum, frame):
sys.stderr.write('Caught SIGTERM. Stopping containern')
if container_id != None:
cmd = ['docker', 'rm', '-f', container_id]
subprocess.call(cmd)
sys.exit(143)
signal.signal(signal.SIGTERM, catch_term)
cmd = ['docker', 'run', '--cidfile', cidfile, '-i', image_name, container_process_cmd]
p = subprocess.Popen(cmd)
# This function waits for the file to contain 64 bytes and then reads the container id from it
container_id = get_container_id_from_cidfile(cidfile)
p.communicate()
sys.exit(p.returncode)
When I run the Python process on interactive mode, and kill that warping script during the run (kill <PID>
), the catch_term
function is called and the container is removed. Then my process exits.
However, when I run the warping process on the background, i.e. my_warping_script_above.py &
, and kill it in the same way, the catch_term
function is not called at all and my script keeps running, including the container.
Any idea how to make the
catch_term
function be called even when the script runs on background?I think it has something to do with the fact that
docker run
is a "protected" process. It should only be able to get killed using a docker command, such asdocker rm -f <CONTAINER ID>
, or usingCtrl+C
if called with-i
. That's the reason why I'm trying to catch the TERM signal and calldocker rm
, but my function is not called when the script runs on background... (I think it tries to first kill the child process, then as it fails, it does not reach to stage of calling my registered function).
python docker subprocess background-process
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have the following Python script that warps a docker container run:
import subprocess
import sys
import signal
container_id = None
def catch_term(signum, frame):
sys.stderr.write('Caught SIGTERM. Stopping containern')
if container_id != None:
cmd = ['docker', 'rm', '-f', container_id]
subprocess.call(cmd)
sys.exit(143)
signal.signal(signal.SIGTERM, catch_term)
cmd = ['docker', 'run', '--cidfile', cidfile, '-i', image_name, container_process_cmd]
p = subprocess.Popen(cmd)
# This function waits for the file to contain 64 bytes and then reads the container id from it
container_id = get_container_id_from_cidfile(cidfile)
p.communicate()
sys.exit(p.returncode)
When I run the Python process on interactive mode, and kill that warping script during the run (kill <PID>
), the catch_term
function is called and the container is removed. Then my process exits.
However, when I run the warping process on the background, i.e. my_warping_script_above.py &
, and kill it in the same way, the catch_term
function is not called at all and my script keeps running, including the container.
Any idea how to make the
catch_term
function be called even when the script runs on background?I think it has something to do with the fact that
docker run
is a "protected" process. It should only be able to get killed using a docker command, such asdocker rm -f <CONTAINER ID>
, or usingCtrl+C
if called with-i
. That's the reason why I'm trying to catch the TERM signal and calldocker rm
, but my function is not called when the script runs on background... (I think it tries to first kill the child process, then as it fails, it does not reach to stage of calling my registered function).
python docker subprocess background-process
I have the following Python script that warps a docker container run:
import subprocess
import sys
import signal
container_id = None
def catch_term(signum, frame):
sys.stderr.write('Caught SIGTERM. Stopping containern')
if container_id != None:
cmd = ['docker', 'rm', '-f', container_id]
subprocess.call(cmd)
sys.exit(143)
signal.signal(signal.SIGTERM, catch_term)
cmd = ['docker', 'run', '--cidfile', cidfile, '-i', image_name, container_process_cmd]
p = subprocess.Popen(cmd)
# This function waits for the file to contain 64 bytes and then reads the container id from it
container_id = get_container_id_from_cidfile(cidfile)
p.communicate()
sys.exit(p.returncode)
When I run the Python process on interactive mode, and kill that warping script during the run (kill <PID>
), the catch_term
function is called and the container is removed. Then my process exits.
However, when I run the warping process on the background, i.e. my_warping_script_above.py &
, and kill it in the same way, the catch_term
function is not called at all and my script keeps running, including the container.
Any idea how to make the
catch_term
function be called even when the script runs on background?I think it has something to do with the fact that
docker run
is a "protected" process. It should only be able to get killed using a docker command, such asdocker rm -f <CONTAINER ID>
, or usingCtrl+C
if called with-i
. That's the reason why I'm trying to catch the TERM signal and calldocker rm
, but my function is not called when the script runs on background... (I think it tries to first kill the child process, then as it fails, it does not reach to stage of calling my registered function).
python docker subprocess background-process
python docker subprocess background-process
edited Nov 11 at 16:24
asked Nov 7 at 20:47


SomethingSomething
4,08173465
4,08173465
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
accepted
Answering myself:
This trick solved my problem: adding preexec_fn=os.setsid
to the subprocess.Popen
function:
p = subprocess.Popen(cmd, preexec_fn=os.setsid)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Answering myself:
This trick solved my problem: adding preexec_fn=os.setsid
to the subprocess.Popen
function:
p = subprocess.Popen(cmd, preexec_fn=os.setsid)
add a comment |
up vote
0
down vote
accepted
Answering myself:
This trick solved my problem: adding preexec_fn=os.setsid
to the subprocess.Popen
function:
p = subprocess.Popen(cmd, preexec_fn=os.setsid)
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Answering myself:
This trick solved my problem: adding preexec_fn=os.setsid
to the subprocess.Popen
function:
p = subprocess.Popen(cmd, preexec_fn=os.setsid)
Answering myself:
This trick solved my problem: adding preexec_fn=os.setsid
to the subprocess.Popen
function:
p = subprocess.Popen(cmd, preexec_fn=os.setsid)
answered Nov 7 at 20:55


SomethingSomething
4,08173465
4,08173465
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53197564%2fterminating-docker-container-on-sigterm-on-warping-script%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
u1AE56JjnbTArSlIDvruSrgoCd3H9,You,0b7o,4DPhmvUfK6CwBtLWXmMmZb1uADuZmXTANPQnyWf,MMYo