PutItem with DynamoDB using lists / arrays
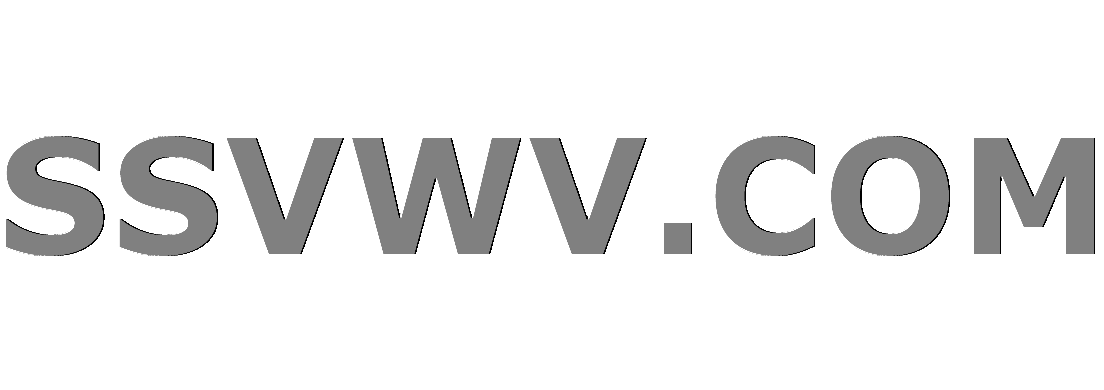
Multi tool use
up vote
1
down vote
favorite
I'm trying to achieve something that I assumed would be pretty standard, but I've hit a wall with it. I have a bunch of data coming at me that I want to store in DynamoDB
const putDataParams = {
TableName: "sensorNodeData2",
Item: {
"deviceId": {"S": event.deviceId},
"timeStamp": {"N": event.time},
"rssi": {"S": event.rssi},
"seq": {"S": event.seqNum},
"payload": {"L": payloadArrayInt16.map(x => x.N)}
}
};
console.log('Data PutItem Params = ' + JSON.stringify(putDataParams));
dynamodb.putItem(putDataParams, function(err, data) {
if (err) {
console.log(err);
context.fail('ERROR: Dynamo failed: ' + err);
}
else {
console.log(data);
context.succeed('SUCCESS');
}
});
The problem is I cannot for the life of me figure out how to get the list part to work. I've defined it first as :
var payloadArrayInt16= new Uint16Array(dataArrayMaxIndex);
and the error is:
"errorMessage": "ERROR: Dynamo failed: InvalidParameterType: Expected params.Item[payload].L to be an Array"
Then I tried :
var payloadArrayInt16= [dataArrayMaxIndex];
Which went through, but obviously doesn't do what I want... when I print out the params, it's not pulled out the contents of the array. It sees:
"Temp":{"L":[null,null,null,null,null,null,null,null,null,null]}
I'm pulling my hair out. There isn't a single example anywhere on how to do this. Can someone please put me out of my misery?!
amazon-web-services amazon-dynamodb aws-sdk-nodejs
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
favorite
I'm trying to achieve something that I assumed would be pretty standard, but I've hit a wall with it. I have a bunch of data coming at me that I want to store in DynamoDB
const putDataParams = {
TableName: "sensorNodeData2",
Item: {
"deviceId": {"S": event.deviceId},
"timeStamp": {"N": event.time},
"rssi": {"S": event.rssi},
"seq": {"S": event.seqNum},
"payload": {"L": payloadArrayInt16.map(x => x.N)}
}
};
console.log('Data PutItem Params = ' + JSON.stringify(putDataParams));
dynamodb.putItem(putDataParams, function(err, data) {
if (err) {
console.log(err);
context.fail('ERROR: Dynamo failed: ' + err);
}
else {
console.log(data);
context.succeed('SUCCESS');
}
});
The problem is I cannot for the life of me figure out how to get the list part to work. I've defined it first as :
var payloadArrayInt16= new Uint16Array(dataArrayMaxIndex);
and the error is:
"errorMessage": "ERROR: Dynamo failed: InvalidParameterType: Expected params.Item[payload].L to be an Array"
Then I tried :
var payloadArrayInt16= [dataArrayMaxIndex];
Which went through, but obviously doesn't do what I want... when I print out the params, it's not pulled out the contents of the array. It sees:
"Temp":{"L":[null,null,null,null,null,null,null,null,null,null]}
I'm pulling my hair out. There isn't a single example anywhere on how to do this. Can someone please put me out of my misery?!
amazon-web-services amazon-dynamodb aws-sdk-nodejs
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm trying to achieve something that I assumed would be pretty standard, but I've hit a wall with it. I have a bunch of data coming at me that I want to store in DynamoDB
const putDataParams = {
TableName: "sensorNodeData2",
Item: {
"deviceId": {"S": event.deviceId},
"timeStamp": {"N": event.time},
"rssi": {"S": event.rssi},
"seq": {"S": event.seqNum},
"payload": {"L": payloadArrayInt16.map(x => x.N)}
}
};
console.log('Data PutItem Params = ' + JSON.stringify(putDataParams));
dynamodb.putItem(putDataParams, function(err, data) {
if (err) {
console.log(err);
context.fail('ERROR: Dynamo failed: ' + err);
}
else {
console.log(data);
context.succeed('SUCCESS');
}
});
The problem is I cannot for the life of me figure out how to get the list part to work. I've defined it first as :
var payloadArrayInt16= new Uint16Array(dataArrayMaxIndex);
and the error is:
"errorMessage": "ERROR: Dynamo failed: InvalidParameterType: Expected params.Item[payload].L to be an Array"
Then I tried :
var payloadArrayInt16= [dataArrayMaxIndex];
Which went through, but obviously doesn't do what I want... when I print out the params, it's not pulled out the contents of the array. It sees:
"Temp":{"L":[null,null,null,null,null,null,null,null,null,null]}
I'm pulling my hair out. There isn't a single example anywhere on how to do this. Can someone please put me out of my misery?!
amazon-web-services amazon-dynamodb aws-sdk-nodejs
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
I'm trying to achieve something that I assumed would be pretty standard, but I've hit a wall with it. I have a bunch of data coming at me that I want to store in DynamoDB
const putDataParams = {
TableName: "sensorNodeData2",
Item: {
"deviceId": {"S": event.deviceId},
"timeStamp": {"N": event.time},
"rssi": {"S": event.rssi},
"seq": {"S": event.seqNum},
"payload": {"L": payloadArrayInt16.map(x => x.N)}
}
};
console.log('Data PutItem Params = ' + JSON.stringify(putDataParams));
dynamodb.putItem(putDataParams, function(err, data) {
if (err) {
console.log(err);
context.fail('ERROR: Dynamo failed: ' + err);
}
else {
console.log(data);
context.succeed('SUCCESS');
}
});
The problem is I cannot for the life of me figure out how to get the list part to work. I've defined it first as :
var payloadArrayInt16= new Uint16Array(dataArrayMaxIndex);
and the error is:
"errorMessage": "ERROR: Dynamo failed: InvalidParameterType: Expected params.Item[payload].L to be an Array"
Then I tried :
var payloadArrayInt16= [dataArrayMaxIndex];
Which went through, but obviously doesn't do what I want... when I print out the params, it's not pulled out the contents of the array. It sees:
"Temp":{"L":[null,null,null,null,null,null,null,null,null,null]}
I'm pulling my hair out. There isn't a single example anywhere on how to do this. Can someone please put me out of my misery?!
const putDataParams = {
TableName: "sensorNodeData2",
Item: {
"deviceId": {"S": event.deviceId},
"timeStamp": {"N": event.time},
"rssi": {"S": event.rssi},
"seq": {"S": event.seqNum},
"payload": {"L": payloadArrayInt16.map(x => x.N)}
}
};
console.log('Data PutItem Params = ' + JSON.stringify(putDataParams));
dynamodb.putItem(putDataParams, function(err, data) {
if (err) {
console.log(err);
context.fail('ERROR: Dynamo failed: ' + err);
}
else {
console.log(data);
context.succeed('SUCCESS');
}
});
const putDataParams = {
TableName: "sensorNodeData2",
Item: {
"deviceId": {"S": event.deviceId},
"timeStamp": {"N": event.time},
"rssi": {"S": event.rssi},
"seq": {"S": event.seqNum},
"payload": {"L": payloadArrayInt16.map(x => x.N)}
}
};
console.log('Data PutItem Params = ' + JSON.stringify(putDataParams));
dynamodb.putItem(putDataParams, function(err, data) {
if (err) {
console.log(err);
context.fail('ERROR: Dynamo failed: ' + err);
}
else {
console.log(data);
context.succeed('SUCCESS');
}
});
amazon-web-services amazon-dynamodb aws-sdk-nodejs
amazon-web-services amazon-dynamodb aws-sdk-nodejs
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
asked Nov 7 at 7:55


monkey
82
82
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
New contributor
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
monkey is a new contributor to this site. Take care in asking for clarification, commenting, and answering.
Check out our Code of Conduct.
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
2
down vote
accepted
DynamoDB array of numeric values is represented like this:
"payload": {
"L": [
{
"N": "1"
},
{
"N": "2"
},
{
"N": "3"
}
]
}
Now if your input array payloadArrayInt16 is like this:
[1,2,3]
You have to transform it to dynamo db format:
payloadArrayInt16.map(x => { return { "N": x.toString() }});
But you can make your life much easier by using this official aws dymanodb js library
It handles all transformations between native js object structures to dynamo db data structures and viceversa.
Also if you are using AWS IoT Core you can write your data to dynamodb directly without any lambda.
Crumbs. You've hit the nail on the head. Thanks heaps!
– monkey
Nov 7 at 9:00
And you're totally right. DocumentClient worked perfectly for putting items into the database. The reason I didn't use it was I couldn't get it working for querying and kind of gave up on it... Maybe I'll give it another whirl.
– monkey
Nov 7 at 19:19
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
DynamoDB array of numeric values is represented like this:
"payload": {
"L": [
{
"N": "1"
},
{
"N": "2"
},
{
"N": "3"
}
]
}
Now if your input array payloadArrayInt16 is like this:
[1,2,3]
You have to transform it to dynamo db format:
payloadArrayInt16.map(x => { return { "N": x.toString() }});
But you can make your life much easier by using this official aws dymanodb js library
It handles all transformations between native js object structures to dynamo db data structures and viceversa.
Also if you are using AWS IoT Core you can write your data to dynamodb directly without any lambda.
Crumbs. You've hit the nail on the head. Thanks heaps!
– monkey
Nov 7 at 9:00
And you're totally right. DocumentClient worked perfectly for putting items into the database. The reason I didn't use it was I couldn't get it working for querying and kind of gave up on it... Maybe I'll give it another whirl.
– monkey
Nov 7 at 19:19
add a comment |
up vote
2
down vote
accepted
DynamoDB array of numeric values is represented like this:
"payload": {
"L": [
{
"N": "1"
},
{
"N": "2"
},
{
"N": "3"
}
]
}
Now if your input array payloadArrayInt16 is like this:
[1,2,3]
You have to transform it to dynamo db format:
payloadArrayInt16.map(x => { return { "N": x.toString() }});
But you can make your life much easier by using this official aws dymanodb js library
It handles all transformations between native js object structures to dynamo db data structures and viceversa.
Also if you are using AWS IoT Core you can write your data to dynamodb directly without any lambda.
Crumbs. You've hit the nail on the head. Thanks heaps!
– monkey
Nov 7 at 9:00
And you're totally right. DocumentClient worked perfectly for putting items into the database. The reason I didn't use it was I couldn't get it working for querying and kind of gave up on it... Maybe I'll give it another whirl.
– monkey
Nov 7 at 19:19
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
DynamoDB array of numeric values is represented like this:
"payload": {
"L": [
{
"N": "1"
},
{
"N": "2"
},
{
"N": "3"
}
]
}
Now if your input array payloadArrayInt16 is like this:
[1,2,3]
You have to transform it to dynamo db format:
payloadArrayInt16.map(x => { return { "N": x.toString() }});
But you can make your life much easier by using this official aws dymanodb js library
It handles all transformations between native js object structures to dynamo db data structures and viceversa.
Also if you are using AWS IoT Core you can write your data to dynamodb directly without any lambda.
DynamoDB array of numeric values is represented like this:
"payload": {
"L": [
{
"N": "1"
},
{
"N": "2"
},
{
"N": "3"
}
]
}
Now if your input array payloadArrayInt16 is like this:
[1,2,3]
You have to transform it to dynamo db format:
payloadArrayInt16.map(x => { return { "N": x.toString() }});
But you can make your life much easier by using this official aws dymanodb js library
It handles all transformations between native js object structures to dynamo db data structures and viceversa.
Also if you are using AWS IoT Core you can write your data to dynamodb directly without any lambda.
edited Nov 7 at 9:02
answered Nov 7 at 8:28


misher
392110
392110
Crumbs. You've hit the nail on the head. Thanks heaps!
– monkey
Nov 7 at 9:00
And you're totally right. DocumentClient worked perfectly for putting items into the database. The reason I didn't use it was I couldn't get it working for querying and kind of gave up on it... Maybe I'll give it another whirl.
– monkey
Nov 7 at 19:19
add a comment |
Crumbs. You've hit the nail on the head. Thanks heaps!
– monkey
Nov 7 at 9:00
And you're totally right. DocumentClient worked perfectly for putting items into the database. The reason I didn't use it was I couldn't get it working for querying and kind of gave up on it... Maybe I'll give it another whirl.
– monkey
Nov 7 at 19:19
Crumbs. You've hit the nail on the head. Thanks heaps!
– monkey
Nov 7 at 9:00
Crumbs. You've hit the nail on the head. Thanks heaps!
– monkey
Nov 7 at 9:00
And you're totally right. DocumentClient worked perfectly for putting items into the database. The reason I didn't use it was I couldn't get it working for querying and kind of gave up on it... Maybe I'll give it another whirl.
– monkey
Nov 7 at 19:19
And you're totally right. DocumentClient worked perfectly for putting items into the database. The reason I didn't use it was I couldn't get it working for querying and kind of gave up on it... Maybe I'll give it another whirl.
– monkey
Nov 7 at 19:19
add a comment |
monkey is a new contributor. Be nice, and check out our Code of Conduct.
monkey is a new contributor. Be nice, and check out our Code of Conduct.
monkey is a new contributor. Be nice, and check out our Code of Conduct.
monkey is a new contributor. Be nice, and check out our Code of Conduct.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53185405%2fputitem-with-dynamodb-using-lists-arrays%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
LM8m,EZpSc9szeRF,mUlq6bV0ZHVsTCS