Python: How to get rid of timeout errors when you're using external network APIs?
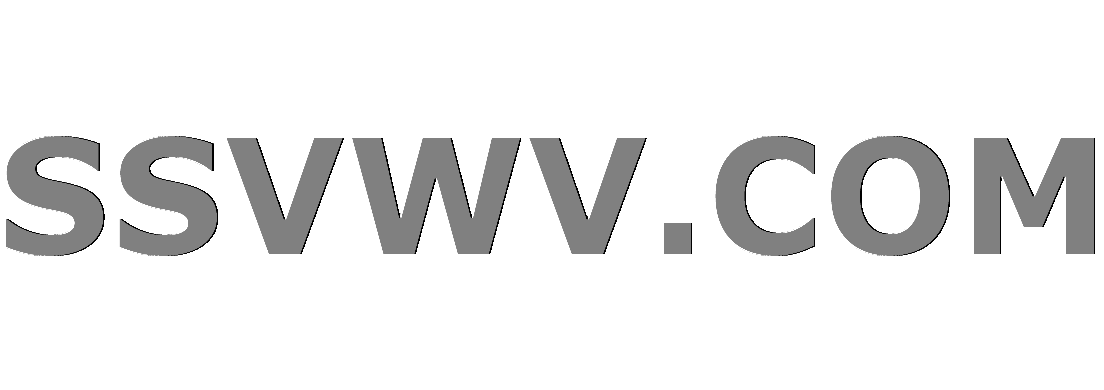
Multi tool use
up vote
1
down vote
favorite
When I am using postman tool everything is working fine but when I am trying to get access token using Python http client / requests. both times ending up following Errors.
When I use http.client
TimeoutError: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond
or when I use requests , session thing
ConnectionError: HTTPSConnectionPool(host='identity.trimble.com', port=443): Max retries exceeded with url: /token (Caused by NewConnectionError('<urllib3.connection.VerifiedHTTPSConnection object at 0x00000000088F49E8>: Failed to establish a new connection: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond',))
this is my code
import http.client, urllib.request, urllib.parse, urllib.error, base64
url = 'https://someurl'
client_id = 'some key' #Consumer Key
client_secret = 'secret' #Consumer Secret
Auth = base64.b64encode("{id}:{secrete}".format(**{'id' : client_id,
'secrete': client_secret}).encode('utf-8'))
header = {
'authorization': 'Basic %s' % Auth.decode('utf-8'),
'content-type' : 'application/x-www-form-urlencoded',
'user-agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/62.0.3202.94 Safari/537.36'
}
body = urllib.parse.urlencode({
'grant_type' : 'password',
'username' : 'usr@gmail.com',
'password' : 'somepwd',
'scope' : 'scope'
})
conn = http.client.HTTPSConnection('identity.trimble.com')
conn.request("POST", "/token", body=body, headers=header)
response = conn.getresponse()
print(response.code)
if response.code == 200:
data = json.loads(response.read())
print(data.get('access_token', None))
#access_token['ttl'] = datetime.now() + timedelta(seconds=data.get('expires_in', 10))
conn.close()
I am in my company VPN does this have any effect? I am wondering because it's working fine with Postman tool.
Thanks
python python-3.x python-requests
add a comment |
up vote
1
down vote
favorite
When I am using postman tool everything is working fine but when I am trying to get access token using Python http client / requests. both times ending up following Errors.
When I use http.client
TimeoutError: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond
or when I use requests , session thing
ConnectionError: HTTPSConnectionPool(host='identity.trimble.com', port=443): Max retries exceeded with url: /token (Caused by NewConnectionError('<urllib3.connection.VerifiedHTTPSConnection object at 0x00000000088F49E8>: Failed to establish a new connection: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond',))
this is my code
import http.client, urllib.request, urllib.parse, urllib.error, base64
url = 'https://someurl'
client_id = 'some key' #Consumer Key
client_secret = 'secret' #Consumer Secret
Auth = base64.b64encode("{id}:{secrete}".format(**{'id' : client_id,
'secrete': client_secret}).encode('utf-8'))
header = {
'authorization': 'Basic %s' % Auth.decode('utf-8'),
'content-type' : 'application/x-www-form-urlencoded',
'user-agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/62.0.3202.94 Safari/537.36'
}
body = urllib.parse.urlencode({
'grant_type' : 'password',
'username' : 'usr@gmail.com',
'password' : 'somepwd',
'scope' : 'scope'
})
conn = http.client.HTTPSConnection('identity.trimble.com')
conn.request("POST", "/token", body=body, headers=header)
response = conn.getresponse()
print(response.code)
if response.code == 200:
data = json.loads(response.read())
print(data.get('access_token', None))
#access_token['ttl'] = datetime.now() + timedelta(seconds=data.get('expires_in', 10))
conn.close()
I am in my company VPN does this have any effect? I am wondering because it's working fine with Postman tool.
Thanks
python python-3.x python-requests
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
When I am using postman tool everything is working fine but when I am trying to get access token using Python http client / requests. both times ending up following Errors.
When I use http.client
TimeoutError: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond
or when I use requests , session thing
ConnectionError: HTTPSConnectionPool(host='identity.trimble.com', port=443): Max retries exceeded with url: /token (Caused by NewConnectionError('<urllib3.connection.VerifiedHTTPSConnection object at 0x00000000088F49E8>: Failed to establish a new connection: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond',))
this is my code
import http.client, urllib.request, urllib.parse, urllib.error, base64
url = 'https://someurl'
client_id = 'some key' #Consumer Key
client_secret = 'secret' #Consumer Secret
Auth = base64.b64encode("{id}:{secrete}".format(**{'id' : client_id,
'secrete': client_secret}).encode('utf-8'))
header = {
'authorization': 'Basic %s' % Auth.decode('utf-8'),
'content-type' : 'application/x-www-form-urlencoded',
'user-agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/62.0.3202.94 Safari/537.36'
}
body = urllib.parse.urlencode({
'grant_type' : 'password',
'username' : 'usr@gmail.com',
'password' : 'somepwd',
'scope' : 'scope'
})
conn = http.client.HTTPSConnection('identity.trimble.com')
conn.request("POST", "/token", body=body, headers=header)
response = conn.getresponse()
print(response.code)
if response.code == 200:
data = json.loads(response.read())
print(data.get('access_token', None))
#access_token['ttl'] = datetime.now() + timedelta(seconds=data.get('expires_in', 10))
conn.close()
I am in my company VPN does this have any effect? I am wondering because it's working fine with Postman tool.
Thanks
python python-3.x python-requests
When I am using postman tool everything is working fine but when I am trying to get access token using Python http client / requests. both times ending up following Errors.
When I use http.client
TimeoutError: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond
or when I use requests , session thing
ConnectionError: HTTPSConnectionPool(host='identity.trimble.com', port=443): Max retries exceeded with url: /token (Caused by NewConnectionError('<urllib3.connection.VerifiedHTTPSConnection object at 0x00000000088F49E8>: Failed to establish a new connection: [WinError 10060] A connection attempt failed because the connected party did not properly respond after a period of time, or established connection failed because connected host has failed to respond',))
this is my code
import http.client, urllib.request, urllib.parse, urllib.error, base64
url = 'https://someurl'
client_id = 'some key' #Consumer Key
client_secret = 'secret' #Consumer Secret
Auth = base64.b64encode("{id}:{secrete}".format(**{'id' : client_id,
'secrete': client_secret}).encode('utf-8'))
header = {
'authorization': 'Basic %s' % Auth.decode('utf-8'),
'content-type' : 'application/x-www-form-urlencoded',
'user-agent':'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/62.0.3202.94 Safari/537.36'
}
body = urllib.parse.urlencode({
'grant_type' : 'password',
'username' : 'usr@gmail.com',
'password' : 'somepwd',
'scope' : 'scope'
})
conn = http.client.HTTPSConnection('identity.trimble.com')
conn.request("POST", "/token", body=body, headers=header)
response = conn.getresponse()
print(response.code)
if response.code == 200:
data = json.loads(response.read())
print(data.get('access_token', None))
#access_token['ttl'] = datetime.now() + timedelta(seconds=data.get('expires_in', 10))
conn.close()
I am in my company VPN does this have any effect? I am wondering because it's working fine with Postman tool.
Thanks
python python-3.x python-requests
python python-3.x python-requests
edited Nov 7 at 7:51
asked Nov 7 at 7:43


CSMaverick
1,2081328
1,2081328
add a comment |
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53185280%2fpython-how-to-get-rid-of-timeout-errors-when-youre-using-external-network-apis%23new-answer', 'question_page');
}
);
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
LTYf94L,XgM fD PGmCc,687DBWffdYU tqd jQqbo3Epl1yV4O4FUA0WME1,tu nibP1n 71m