Adding manually an entry to the Django queryset
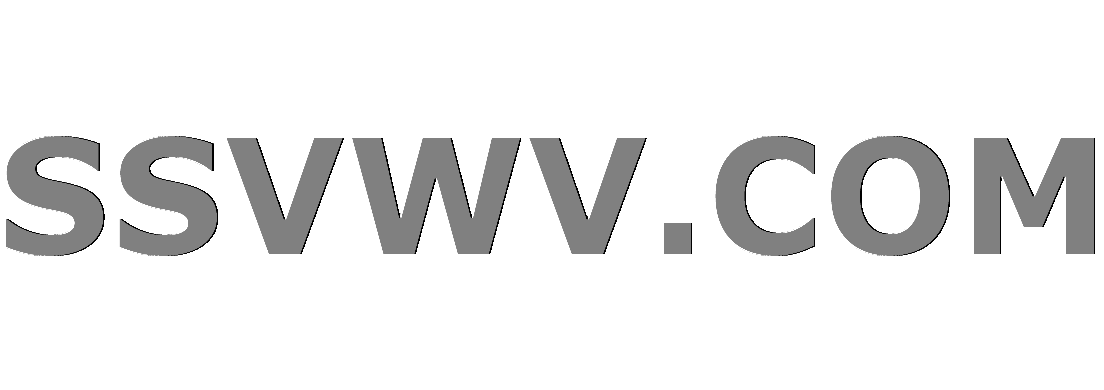
Multi tool use
up vote
0
down vote
favorite
This is my model (the relevant part):
class Country(models.Model):
name = models.CharField(max_length=500, choices=COUNTRY_NAME_CHOICE)
country_id = models.CharField(max_length=500,choices=COUNTRY_ID_CHOICE,primary_key=True,unique=True)
def __str__(self):
return self.name
class City(models.Model):
name = models.CharField(max_length=500,choices=CITY_NAME_CHOICE)
country = models.ForeignKey('Country',on_delete=models.CASCADE)
zip= models.CharField(max_length=500, choices=ZIP_CHOICE, primary_key=True,unique=True)
def __str__(self):
return self.name
class Cost(models.Model):
date = models.ForeignKey('Date' ,on_delete=models.CASCADE)
city= models.ForeignKey('City' ,on_delete=models.CASCADE)
value = models.FloatField(validators=[MinValueValidator(0)])
entry_time = models.DateTimeField(auto_now_add=True)
user = models.ForeignKey(User, on_delete=models.CASCADE)
def __str__(self):
return self.city.name + ' ' +str(self.value)
For model Cost, I have a Django form CostForm that I am listing out on a template, so that users can insert new costs.
class CostForm(ModelForm):
class Meta:
model = Cost
exclude = ['user','entry_time']
#Makes only FR, NO, and RU cities available
def __init__(self, *args, **kwargs):
super(CostForm, self).__init__(*args, **kwargs)
self.fields['city'].queryset = City.objects.filter(country__name__in=['France','Norway','Russia'])
On the template city field is shown as a dropdown. I have already managed to limit this field to the values I want in the form above (user can input costs only for Russia, Norway and France, because their cities are the only options they see in the dropdown).
Here is my problem: I need to make another option available for the
users in the city dropdown. A city that doesn't exist for real in the
database. Lets call it "X". When user chooses city X, that would be a
sign that I need to do some additional calculations in the backend, and
split up cost between some other cities.
I thought I could manually add this city X to the queryset in the CostForm, but after some extensive research I hit the wall. Everyone is saying that the queryset object is not there to be edited, but only represents the result of a query.
Then I tried editing the dropdown in javascript and adding a new option for city X there, and I managed, BUT, I cannot work with this entry with city X, because the form is not valid. So, the user just gets the message similar to "Please choose a valid option for the field".
So now I am back to exploring the possibility of adding this city X manually to the queryset in the CostForm... Does anyone know how?? Also, if you have any other ideas on how I could solve this problem, please share.
django django-queryset
|
show 1 more comment
up vote
0
down vote
favorite
This is my model (the relevant part):
class Country(models.Model):
name = models.CharField(max_length=500, choices=COUNTRY_NAME_CHOICE)
country_id = models.CharField(max_length=500,choices=COUNTRY_ID_CHOICE,primary_key=True,unique=True)
def __str__(self):
return self.name
class City(models.Model):
name = models.CharField(max_length=500,choices=CITY_NAME_CHOICE)
country = models.ForeignKey('Country',on_delete=models.CASCADE)
zip= models.CharField(max_length=500, choices=ZIP_CHOICE, primary_key=True,unique=True)
def __str__(self):
return self.name
class Cost(models.Model):
date = models.ForeignKey('Date' ,on_delete=models.CASCADE)
city= models.ForeignKey('City' ,on_delete=models.CASCADE)
value = models.FloatField(validators=[MinValueValidator(0)])
entry_time = models.DateTimeField(auto_now_add=True)
user = models.ForeignKey(User, on_delete=models.CASCADE)
def __str__(self):
return self.city.name + ' ' +str(self.value)
For model Cost, I have a Django form CostForm that I am listing out on a template, so that users can insert new costs.
class CostForm(ModelForm):
class Meta:
model = Cost
exclude = ['user','entry_time']
#Makes only FR, NO, and RU cities available
def __init__(self, *args, **kwargs):
super(CostForm, self).__init__(*args, **kwargs)
self.fields['city'].queryset = City.objects.filter(country__name__in=['France','Norway','Russia'])
On the template city field is shown as a dropdown. I have already managed to limit this field to the values I want in the form above (user can input costs only for Russia, Norway and France, because their cities are the only options they see in the dropdown).
Here is my problem: I need to make another option available for the
users in the city dropdown. A city that doesn't exist for real in the
database. Lets call it "X". When user chooses city X, that would be a
sign that I need to do some additional calculations in the backend, and
split up cost between some other cities.
I thought I could manually add this city X to the queryset in the CostForm, but after some extensive research I hit the wall. Everyone is saying that the queryset object is not there to be edited, but only represents the result of a query.
Then I tried editing the dropdown in javascript and adding a new option for city X there, and I managed, BUT, I cannot work with this entry with city X, because the form is not valid. So, the user just gets the message similar to "Please choose a valid option for the field".
So now I am back to exploring the possibility of adding this city X manually to the queryset in the CostForm... Does anyone know how?? Also, if you have any other ideas on how I could solve this problem, please share.
django django-queryset
1
You can try making a new Queryset and set it tomutable=True
then update the queryset with the new city data and then save that updated data to the database. If this sounds close to what you're looking for I can provide a snippet as an answer.
– Kevin Hernandez
Nov 8 at 14:24
1
Try this discussion. It contains some interesting ideas.
– Fotis Sk
Nov 8 at 17:10
Thanks for commenting! @KevinHernandez The thing is that I don't want to save this city X in the database. I am connecting this city table to other tables in my db, and I wouldn't want it to show X as an option anywhere else. It should just be a sign for me to do the calculations in the backend when the user chooses the city X. But, this mutable=True does sound interesting, maybe it could help...
– GileBrt
Nov 8 at 17:40
@FotisSk I saw this discussion, but I am looking for a way to edit the queryset in the code, not to create a union of two querysets.
– GileBrt
Nov 8 at 17:40
@GileBrt You can try importing QueryDict fromdjango.http
asfrom django.http import QueryDict
Then creating your own QueryDict withmyquery = QueryDict(mutable=True)
and then updating that mutable query with the new data withmyquery.update(newdata)
.newdata
being the modified data where you add your city.
– Kevin Hernandez
Nov 8 at 18:15
|
show 1 more comment
up vote
0
down vote
favorite
up vote
0
down vote
favorite
This is my model (the relevant part):
class Country(models.Model):
name = models.CharField(max_length=500, choices=COUNTRY_NAME_CHOICE)
country_id = models.CharField(max_length=500,choices=COUNTRY_ID_CHOICE,primary_key=True,unique=True)
def __str__(self):
return self.name
class City(models.Model):
name = models.CharField(max_length=500,choices=CITY_NAME_CHOICE)
country = models.ForeignKey('Country',on_delete=models.CASCADE)
zip= models.CharField(max_length=500, choices=ZIP_CHOICE, primary_key=True,unique=True)
def __str__(self):
return self.name
class Cost(models.Model):
date = models.ForeignKey('Date' ,on_delete=models.CASCADE)
city= models.ForeignKey('City' ,on_delete=models.CASCADE)
value = models.FloatField(validators=[MinValueValidator(0)])
entry_time = models.DateTimeField(auto_now_add=True)
user = models.ForeignKey(User, on_delete=models.CASCADE)
def __str__(self):
return self.city.name + ' ' +str(self.value)
For model Cost, I have a Django form CostForm that I am listing out on a template, so that users can insert new costs.
class CostForm(ModelForm):
class Meta:
model = Cost
exclude = ['user','entry_time']
#Makes only FR, NO, and RU cities available
def __init__(self, *args, **kwargs):
super(CostForm, self).__init__(*args, **kwargs)
self.fields['city'].queryset = City.objects.filter(country__name__in=['France','Norway','Russia'])
On the template city field is shown as a dropdown. I have already managed to limit this field to the values I want in the form above (user can input costs only for Russia, Norway and France, because their cities are the only options they see in the dropdown).
Here is my problem: I need to make another option available for the
users in the city dropdown. A city that doesn't exist for real in the
database. Lets call it "X". When user chooses city X, that would be a
sign that I need to do some additional calculations in the backend, and
split up cost between some other cities.
I thought I could manually add this city X to the queryset in the CostForm, but after some extensive research I hit the wall. Everyone is saying that the queryset object is not there to be edited, but only represents the result of a query.
Then I tried editing the dropdown in javascript and adding a new option for city X there, and I managed, BUT, I cannot work with this entry with city X, because the form is not valid. So, the user just gets the message similar to "Please choose a valid option for the field".
So now I am back to exploring the possibility of adding this city X manually to the queryset in the CostForm... Does anyone know how?? Also, if you have any other ideas on how I could solve this problem, please share.
django django-queryset
This is my model (the relevant part):
class Country(models.Model):
name = models.CharField(max_length=500, choices=COUNTRY_NAME_CHOICE)
country_id = models.CharField(max_length=500,choices=COUNTRY_ID_CHOICE,primary_key=True,unique=True)
def __str__(self):
return self.name
class City(models.Model):
name = models.CharField(max_length=500,choices=CITY_NAME_CHOICE)
country = models.ForeignKey('Country',on_delete=models.CASCADE)
zip= models.CharField(max_length=500, choices=ZIP_CHOICE, primary_key=True,unique=True)
def __str__(self):
return self.name
class Cost(models.Model):
date = models.ForeignKey('Date' ,on_delete=models.CASCADE)
city= models.ForeignKey('City' ,on_delete=models.CASCADE)
value = models.FloatField(validators=[MinValueValidator(0)])
entry_time = models.DateTimeField(auto_now_add=True)
user = models.ForeignKey(User, on_delete=models.CASCADE)
def __str__(self):
return self.city.name + ' ' +str(self.value)
For model Cost, I have a Django form CostForm that I am listing out on a template, so that users can insert new costs.
class CostForm(ModelForm):
class Meta:
model = Cost
exclude = ['user','entry_time']
#Makes only FR, NO, and RU cities available
def __init__(self, *args, **kwargs):
super(CostForm, self).__init__(*args, **kwargs)
self.fields['city'].queryset = City.objects.filter(country__name__in=['France','Norway','Russia'])
On the template city field is shown as a dropdown. I have already managed to limit this field to the values I want in the form above (user can input costs only for Russia, Norway and France, because their cities are the only options they see in the dropdown).
Here is my problem: I need to make another option available for the
users in the city dropdown. A city that doesn't exist for real in the
database. Lets call it "X". When user chooses city X, that would be a
sign that I need to do some additional calculations in the backend, and
split up cost between some other cities.
I thought I could manually add this city X to the queryset in the CostForm, but after some extensive research I hit the wall. Everyone is saying that the queryset object is not there to be edited, but only represents the result of a query.
Then I tried editing the dropdown in javascript and adding a new option for city X there, and I managed, BUT, I cannot work with this entry with city X, because the form is not valid. So, the user just gets the message similar to "Please choose a valid option for the field".
So now I am back to exploring the possibility of adding this city X manually to the queryset in the CostForm... Does anyone know how?? Also, if you have any other ideas on how I could solve this problem, please share.
django django-queryset
django django-queryset
asked Nov 8 at 14:15
GileBrt
5751815
5751815
1
You can try making a new Queryset and set it tomutable=True
then update the queryset with the new city data and then save that updated data to the database. If this sounds close to what you're looking for I can provide a snippet as an answer.
– Kevin Hernandez
Nov 8 at 14:24
1
Try this discussion. It contains some interesting ideas.
– Fotis Sk
Nov 8 at 17:10
Thanks for commenting! @KevinHernandez The thing is that I don't want to save this city X in the database. I am connecting this city table to other tables in my db, and I wouldn't want it to show X as an option anywhere else. It should just be a sign for me to do the calculations in the backend when the user chooses the city X. But, this mutable=True does sound interesting, maybe it could help...
– GileBrt
Nov 8 at 17:40
@FotisSk I saw this discussion, but I am looking for a way to edit the queryset in the code, not to create a union of two querysets.
– GileBrt
Nov 8 at 17:40
@GileBrt You can try importing QueryDict fromdjango.http
asfrom django.http import QueryDict
Then creating your own QueryDict withmyquery = QueryDict(mutable=True)
and then updating that mutable query with the new data withmyquery.update(newdata)
.newdata
being the modified data where you add your city.
– Kevin Hernandez
Nov 8 at 18:15
|
show 1 more comment
1
You can try making a new Queryset and set it tomutable=True
then update the queryset with the new city data and then save that updated data to the database. If this sounds close to what you're looking for I can provide a snippet as an answer.
– Kevin Hernandez
Nov 8 at 14:24
1
Try this discussion. It contains some interesting ideas.
– Fotis Sk
Nov 8 at 17:10
Thanks for commenting! @KevinHernandez The thing is that I don't want to save this city X in the database. I am connecting this city table to other tables in my db, and I wouldn't want it to show X as an option anywhere else. It should just be a sign for me to do the calculations in the backend when the user chooses the city X. But, this mutable=True does sound interesting, maybe it could help...
– GileBrt
Nov 8 at 17:40
@FotisSk I saw this discussion, but I am looking for a way to edit the queryset in the code, not to create a union of two querysets.
– GileBrt
Nov 8 at 17:40
@GileBrt You can try importing QueryDict fromdjango.http
asfrom django.http import QueryDict
Then creating your own QueryDict withmyquery = QueryDict(mutable=True)
and then updating that mutable query with the new data withmyquery.update(newdata)
.newdata
being the modified data where you add your city.
– Kevin Hernandez
Nov 8 at 18:15
1
1
You can try making a new Queryset and set it to
mutable=True
then update the queryset with the new city data and then save that updated data to the database. If this sounds close to what you're looking for I can provide a snippet as an answer.– Kevin Hernandez
Nov 8 at 14:24
You can try making a new Queryset and set it to
mutable=True
then update the queryset with the new city data and then save that updated data to the database. If this sounds close to what you're looking for I can provide a snippet as an answer.– Kevin Hernandez
Nov 8 at 14:24
1
1
Try this discussion. It contains some interesting ideas.
– Fotis Sk
Nov 8 at 17:10
Try this discussion. It contains some interesting ideas.
– Fotis Sk
Nov 8 at 17:10
Thanks for commenting! @KevinHernandez The thing is that I don't want to save this city X in the database. I am connecting this city table to other tables in my db, and I wouldn't want it to show X as an option anywhere else. It should just be a sign for me to do the calculations in the backend when the user chooses the city X. But, this mutable=True does sound interesting, maybe it could help...
– GileBrt
Nov 8 at 17:40
Thanks for commenting! @KevinHernandez The thing is that I don't want to save this city X in the database. I am connecting this city table to other tables in my db, and I wouldn't want it to show X as an option anywhere else. It should just be a sign for me to do the calculations in the backend when the user chooses the city X. But, this mutable=True does sound interesting, maybe it could help...
– GileBrt
Nov 8 at 17:40
@FotisSk I saw this discussion, but I am looking for a way to edit the queryset in the code, not to create a union of two querysets.
– GileBrt
Nov 8 at 17:40
@FotisSk I saw this discussion, but I am looking for a way to edit the queryset in the code, not to create a union of two querysets.
– GileBrt
Nov 8 at 17:40
@GileBrt You can try importing QueryDict from
django.http
as from django.http import QueryDict
Then creating your own QueryDict with myquery = QueryDict(mutable=True)
and then updating that mutable query with the new data with myquery.update(newdata)
. newdata
being the modified data where you add your city.– Kevin Hernandez
Nov 8 at 18:15
@GileBrt You can try importing QueryDict from
django.http
as from django.http import QueryDict
Then creating your own QueryDict with myquery = QueryDict(mutable=True)
and then updating that mutable query with the new data with myquery.update(newdata)
. newdata
being the modified data where you add your city.– Kevin Hernandez
Nov 8 at 18:15
|
show 1 more comment
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53209568%2fadding-manually-an-entry-to-the-django-queryset%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
BqLH YD qK rtS0d,NM7g,7cjlXBF1XzabS z,n1 z1CPdKs
1
You can try making a new Queryset and set it to
mutable=True
then update the queryset with the new city data and then save that updated data to the database. If this sounds close to what you're looking for I can provide a snippet as an answer.– Kevin Hernandez
Nov 8 at 14:24
1
Try this discussion. It contains some interesting ideas.
– Fotis Sk
Nov 8 at 17:10
Thanks for commenting! @KevinHernandez The thing is that I don't want to save this city X in the database. I am connecting this city table to other tables in my db, and I wouldn't want it to show X as an option anywhere else. It should just be a sign for me to do the calculations in the backend when the user chooses the city X. But, this mutable=True does sound interesting, maybe it could help...
– GileBrt
Nov 8 at 17:40
@FotisSk I saw this discussion, but I am looking for a way to edit the queryset in the code, not to create a union of two querysets.
– GileBrt
Nov 8 at 17:40
@GileBrt You can try importing QueryDict from
django.http
asfrom django.http import QueryDict
Then creating your own QueryDict withmyquery = QueryDict(mutable=True)
and then updating that mutable query with the new data withmyquery.update(newdata)
.newdata
being the modified data where you add your city.– Kevin Hernandez
Nov 8 at 18:15