Looping through an object and changing all values
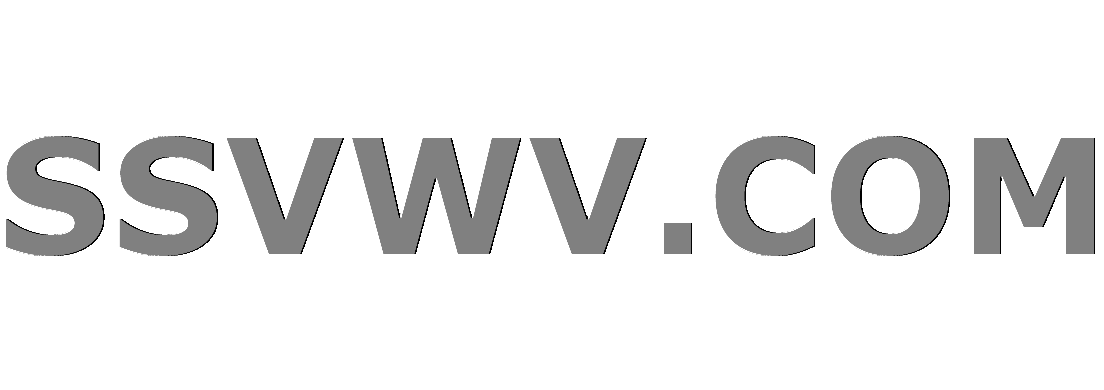
Multi tool use
up vote
1
down vote
favorite
I'm having trouble looping through an object and changing all the values to something else, let's say I want to change all the values to the string "redacted". I need to be able to do this in pure JavaScript.
For example I'd have an object like this...
spy = {
id: 007,
name: "James Bond",
age: 31
};
and the object would look like this after...
spy = {
id: "redacted",
name: "redacted",
age: "redacted"
};
Here is what I have to start with
var superSecret = function(spy){
// Code Here
}
This shouldn't create a new spy object but update it.
javascript
add a comment |
up vote
1
down vote
favorite
I'm having trouble looping through an object and changing all the values to something else, let's say I want to change all the values to the string "redacted". I need to be able to do this in pure JavaScript.
For example I'd have an object like this...
spy = {
id: 007,
name: "James Bond",
age: 31
};
and the object would look like this after...
spy = {
id: "redacted",
name: "redacted",
age: "redacted"
};
Here is what I have to start with
var superSecret = function(spy){
// Code Here
}
This shouldn't create a new spy object but update it.
javascript
for...in
loop
– Rayon
Apr 7 '16 at 5:26
1
You can useObject.keys(spy)
to get the property names, then loop over that using say forEach. Lots of questions and answers here on that.
– RobG
Apr 7 '16 at 5:30
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I'm having trouble looping through an object and changing all the values to something else, let's say I want to change all the values to the string "redacted". I need to be able to do this in pure JavaScript.
For example I'd have an object like this...
spy = {
id: 007,
name: "James Bond",
age: 31
};
and the object would look like this after...
spy = {
id: "redacted",
name: "redacted",
age: "redacted"
};
Here is what I have to start with
var superSecret = function(spy){
// Code Here
}
This shouldn't create a new spy object but update it.
javascript
I'm having trouble looping through an object and changing all the values to something else, let's say I want to change all the values to the string "redacted". I need to be able to do this in pure JavaScript.
For example I'd have an object like this...
spy = {
id: 007,
name: "James Bond",
age: 31
};
and the object would look like this after...
spy = {
id: "redacted",
name: "redacted",
age: "redacted"
};
Here is what I have to start with
var superSecret = function(spy){
// Code Here
}
This shouldn't create a new spy object but update it.
javascript
javascript
asked Apr 7 '16 at 5:25


Dominic
69111
69111
for...in
loop
– Rayon
Apr 7 '16 at 5:26
1
You can useObject.keys(spy)
to get the property names, then loop over that using say forEach. Lots of questions and answers here on that.
– RobG
Apr 7 '16 at 5:30
add a comment |
for...in
loop
– Rayon
Apr 7 '16 at 5:26
1
You can useObject.keys(spy)
to get the property names, then loop over that using say forEach. Lots of questions and answers here on that.
– RobG
Apr 7 '16 at 5:30
for...in
loop– Rayon
Apr 7 '16 at 5:26
for...in
loop– Rayon
Apr 7 '16 at 5:26
1
1
You can use
Object.keys(spy)
to get the property names, then loop over that using say forEach. Lots of questions and answers here on that.– RobG
Apr 7 '16 at 5:30
You can use
Object.keys(spy)
to get the property names, then loop over that using say forEach. Lots of questions and answers here on that.– RobG
Apr 7 '16 at 5:30
add a comment |
5 Answers
5
active
oldest
votes
up vote
13
down vote
accepted
try
var superSecret = function(spy){
Object.keys(spy).forEach(function(key){ spy[key] = "redacted" });
return spy;
}
Man giving me an error of function returned undefined :(
– Dominic
Apr 7 '16 at 5:32
1
Justreturn spy;
– Rayon
Apr 7 '16 at 5:32
Just face palmed myself. I appreciate the help! Been a while since I've played with JavaScript.
– Dominic
Apr 7 '16 at 5:34
@RayonDabre thanks, though OP gave no indication of whether he wants to use the retured value :)
– gurvinder372
Apr 7 '16 at 5:42
@gurvinder372, My comment was for OP as he was expecting something in return ;)
– Rayon
Apr 7 '16 at 5:44
add a comment |
up vote
1
down vote
var superSecret = function(spy){
for(var key in spy){
if(spy.hasOwnProperty(key)){
//code here
spy[key] = "redacted";
}
}
return spy;
}
add a comment |
up vote
1
down vote
You can also go functional.
Using Object.keys
is better as you will only go through the object properties and not it's prototype chain.
Object.keys(spy).reduce((acc, key) => {acc[key] = 'redacted'; return acc; }, {})
add a comment |
up vote
0
down vote
I wrote a little helper function that walks through an object and applies a callback to each entry:
iterateEntries(node, fn) {
const newNode = {};
Object.entries(node).forEach(([key, val]) => (newNode[key] = fn(val)));
return newNode;
}
Usage:
iterateEntries(yourObject, (entry) => {
return entry; // do something with entry here
});
add a comment |
up vote
-1
down vote
Use a proxy:
function superSecret(spy) {
return new Proxy(spy, { get() { return "redacted"; } });
}
> superSecret(spy).id
< "redacted"
add a comment |
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
13
down vote
accepted
try
var superSecret = function(spy){
Object.keys(spy).forEach(function(key){ spy[key] = "redacted" });
return spy;
}
Man giving me an error of function returned undefined :(
– Dominic
Apr 7 '16 at 5:32
1
Justreturn spy;
– Rayon
Apr 7 '16 at 5:32
Just face palmed myself. I appreciate the help! Been a while since I've played with JavaScript.
– Dominic
Apr 7 '16 at 5:34
@RayonDabre thanks, though OP gave no indication of whether he wants to use the retured value :)
– gurvinder372
Apr 7 '16 at 5:42
@gurvinder372, My comment was for OP as he was expecting something in return ;)
– Rayon
Apr 7 '16 at 5:44
add a comment |
up vote
13
down vote
accepted
try
var superSecret = function(spy){
Object.keys(spy).forEach(function(key){ spy[key] = "redacted" });
return spy;
}
Man giving me an error of function returned undefined :(
– Dominic
Apr 7 '16 at 5:32
1
Justreturn spy;
– Rayon
Apr 7 '16 at 5:32
Just face palmed myself. I appreciate the help! Been a while since I've played with JavaScript.
– Dominic
Apr 7 '16 at 5:34
@RayonDabre thanks, though OP gave no indication of whether he wants to use the retured value :)
– gurvinder372
Apr 7 '16 at 5:42
@gurvinder372, My comment was for OP as he was expecting something in return ;)
– Rayon
Apr 7 '16 at 5:44
add a comment |
up vote
13
down vote
accepted
up vote
13
down vote
accepted
try
var superSecret = function(spy){
Object.keys(spy).forEach(function(key){ spy[key] = "redacted" });
return spy;
}
try
var superSecret = function(spy){
Object.keys(spy).forEach(function(key){ spy[key] = "redacted" });
return spy;
}
edited Apr 7 '16 at 5:42
answered Apr 7 '16 at 5:26
gurvinder372
52.8k53355
52.8k53355
Man giving me an error of function returned undefined :(
– Dominic
Apr 7 '16 at 5:32
1
Justreturn spy;
– Rayon
Apr 7 '16 at 5:32
Just face palmed myself. I appreciate the help! Been a while since I've played with JavaScript.
– Dominic
Apr 7 '16 at 5:34
@RayonDabre thanks, though OP gave no indication of whether he wants to use the retured value :)
– gurvinder372
Apr 7 '16 at 5:42
@gurvinder372, My comment was for OP as he was expecting something in return ;)
– Rayon
Apr 7 '16 at 5:44
add a comment |
Man giving me an error of function returned undefined :(
– Dominic
Apr 7 '16 at 5:32
1
Justreturn spy;
– Rayon
Apr 7 '16 at 5:32
Just face palmed myself. I appreciate the help! Been a while since I've played with JavaScript.
– Dominic
Apr 7 '16 at 5:34
@RayonDabre thanks, though OP gave no indication of whether he wants to use the retured value :)
– gurvinder372
Apr 7 '16 at 5:42
@gurvinder372, My comment was for OP as he was expecting something in return ;)
– Rayon
Apr 7 '16 at 5:44
Man giving me an error of function returned undefined :(
– Dominic
Apr 7 '16 at 5:32
Man giving me an error of function returned undefined :(
– Dominic
Apr 7 '16 at 5:32
1
1
Just
return spy;
– Rayon
Apr 7 '16 at 5:32
Just
return spy;
– Rayon
Apr 7 '16 at 5:32
Just face palmed myself. I appreciate the help! Been a while since I've played with JavaScript.
– Dominic
Apr 7 '16 at 5:34
Just face palmed myself. I appreciate the help! Been a while since I've played with JavaScript.
– Dominic
Apr 7 '16 at 5:34
@RayonDabre thanks, though OP gave no indication of whether he wants to use the retured value :)
– gurvinder372
Apr 7 '16 at 5:42
@RayonDabre thanks, though OP gave no indication of whether he wants to use the retured value :)
– gurvinder372
Apr 7 '16 at 5:42
@gurvinder372, My comment was for OP as he was expecting something in return ;)
– Rayon
Apr 7 '16 at 5:44
@gurvinder372, My comment was for OP as he was expecting something in return ;)
– Rayon
Apr 7 '16 at 5:44
add a comment |
up vote
1
down vote
var superSecret = function(spy){
for(var key in spy){
if(spy.hasOwnProperty(key)){
//code here
spy[key] = "redacted";
}
}
return spy;
}
add a comment |
up vote
1
down vote
var superSecret = function(spy){
for(var key in spy){
if(spy.hasOwnProperty(key)){
//code here
spy[key] = "redacted";
}
}
return spy;
}
add a comment |
up vote
1
down vote
up vote
1
down vote
var superSecret = function(spy){
for(var key in spy){
if(spy.hasOwnProperty(key)){
//code here
spy[key] = "redacted";
}
}
return spy;
}
var superSecret = function(spy){
for(var key in spy){
if(spy.hasOwnProperty(key)){
//code here
spy[key] = "redacted";
}
}
return spy;
}
answered Apr 7 '16 at 5:32
Himanshu Tanwar
826414
826414
add a comment |
add a comment |
up vote
1
down vote
You can also go functional.
Using Object.keys
is better as you will only go through the object properties and not it's prototype chain.
Object.keys(spy).reduce((acc, key) => {acc[key] = 'redacted'; return acc; }, {})
add a comment |
up vote
1
down vote
You can also go functional.
Using Object.keys
is better as you will only go through the object properties and not it's prototype chain.
Object.keys(spy).reduce((acc, key) => {acc[key] = 'redacted'; return acc; }, {})
add a comment |
up vote
1
down vote
up vote
1
down vote
You can also go functional.
Using Object.keys
is better as you will only go through the object properties and not it's prototype chain.
Object.keys(spy).reduce((acc, key) => {acc[key] = 'redacted'; return acc; }, {})
You can also go functional.
Using Object.keys
is better as you will only go through the object properties and not it's prototype chain.
Object.keys(spy).reduce((acc, key) => {acc[key] = 'redacted'; return acc; }, {})
answered Aug 6 at 12:39
Harshal
12010
12010
add a comment |
add a comment |
up vote
0
down vote
I wrote a little helper function that walks through an object and applies a callback to each entry:
iterateEntries(node, fn) {
const newNode = {};
Object.entries(node).forEach(([key, val]) => (newNode[key] = fn(val)));
return newNode;
}
Usage:
iterateEntries(yourObject, (entry) => {
return entry; // do something with entry here
});
add a comment |
up vote
0
down vote
I wrote a little helper function that walks through an object and applies a callback to each entry:
iterateEntries(node, fn) {
const newNode = {};
Object.entries(node).forEach(([key, val]) => (newNode[key] = fn(val)));
return newNode;
}
Usage:
iterateEntries(yourObject, (entry) => {
return entry; // do something with entry here
});
add a comment |
up vote
0
down vote
up vote
0
down vote
I wrote a little helper function that walks through an object and applies a callback to each entry:
iterateEntries(node, fn) {
const newNode = {};
Object.entries(node).forEach(([key, val]) => (newNode[key] = fn(val)));
return newNode;
}
Usage:
iterateEntries(yourObject, (entry) => {
return entry; // do something with entry here
});
I wrote a little helper function that walks through an object and applies a callback to each entry:
iterateEntries(node, fn) {
const newNode = {};
Object.entries(node).forEach(([key, val]) => (newNode[key] = fn(val)));
return newNode;
}
Usage:
iterateEntries(yourObject, (entry) => {
return entry; // do something with entry here
});
answered Nov 8 at 14:12
Lukas
2,35943050
2,35943050
add a comment |
add a comment |
up vote
-1
down vote
Use a proxy:
function superSecret(spy) {
return new Proxy(spy, { get() { return "redacted"; } });
}
> superSecret(spy).id
< "redacted"
add a comment |
up vote
-1
down vote
Use a proxy:
function superSecret(spy) {
return new Proxy(spy, { get() { return "redacted"; } });
}
> superSecret(spy).id
< "redacted"
add a comment |
up vote
-1
down vote
up vote
-1
down vote
Use a proxy:
function superSecret(spy) {
return new Proxy(spy, { get() { return "redacted"; } });
}
> superSecret(spy).id
< "redacted"
Use a proxy:
function superSecret(spy) {
return new Proxy(spy, { get() { return "redacted"; } });
}
> superSecret(spy).id
< "redacted"
edited Apr 7 '16 at 6:07
answered Apr 7 '16 at 5:35
user663031
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f36467369%2flooping-through-an-object-and-changing-all-values%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g6it2epbKw0m,j0,Vd jY7YvWEyu ri69P5TooLTugtQ3yiGImQy2byEnpPGYfQGQz21S,X1UC7HwrJPrn8KINV9 QXl
for...in
loop– Rayon
Apr 7 '16 at 5:26
1
You can use
Object.keys(spy)
to get the property names, then loop over that using say forEach. Lots of questions and answers here on that.– RobG
Apr 7 '16 at 5:30