Search Text By Value Unity
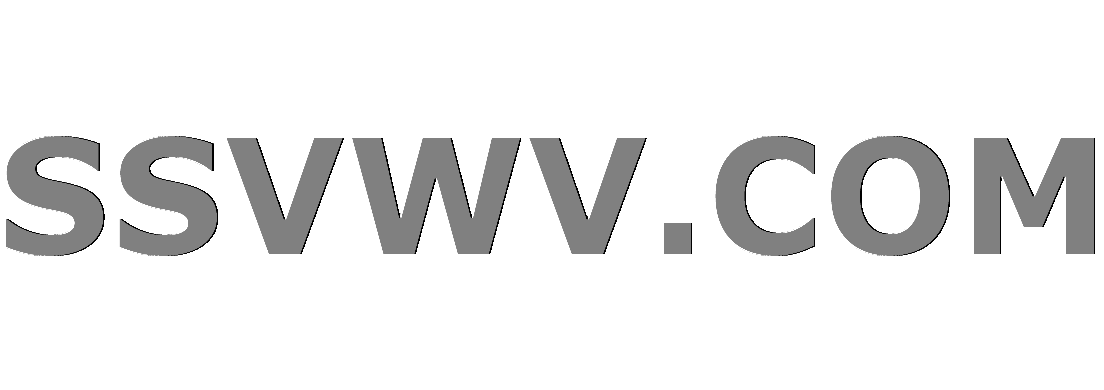
Multi tool use
I need to get a Text GameObject by its value.
For example:
There's a lot of Texts and I only need the one that Says "Hello", NOT THE NAME, the value, i'ts content.
//PseudoCode
string = "Hello";
GameObject.FindObjectsByValue("Hello")
c# unity3d
add a comment |
I need to get a Text GameObject by its value.
For example:
There's a lot of Texts and I only need the one that Says "Hello", NOT THE NAME, the value, i'ts content.
//PseudoCode
string = "Hello";
GameObject.FindObjectsByValue("Hello")
c# unity3d
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 0:19
add a comment |
I need to get a Text GameObject by its value.
For example:
There's a lot of Texts and I only need the one that Says "Hello", NOT THE NAME, the value, i'ts content.
//PseudoCode
string = "Hello";
GameObject.FindObjectsByValue("Hello")
c# unity3d
I need to get a Text GameObject by its value.
For example:
There's a lot of Texts and I only need the one that Says "Hello", NOT THE NAME, the value, i'ts content.
//PseudoCode
string = "Hello";
GameObject.FindObjectsByValue("Hello")
c# unity3d
c# unity3d
asked Nov 14 '18 at 0:05
Sergio MarquezSergio Marquez
417
417
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 0:19
add a comment |
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 0:19
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 0:19
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 0:19
add a comment |
1 Answer
1
active
oldest
votes
There is no built-in function for that, so you need to do it manually. It depends on your situation. In general you must:
- Have reference to all GameObjects that might have that text value
- Check each GameObject's text values with loop
If you need to look for that word once or twice then you can do something like this:
Text array = GameObject.FindObjectsOfType<Text>();
foreach (var x in array)
{
if (string.Compare(x.text,"Hello") == 0)
{
// The stuff to do here
}
}
If you need to keep looking for that word many times then you need store reference to those GameObject
s in some variable and access it anytime you need. This is because FindObjectsOfType<>();
is quite an expensive operation so we need to do it as little as possible. Something like this should work:
private Text array;
private void Awake()
{
Text array = GameObject.FindObjectsOfType<Text>();
}
private void Update()
{
foreach (var x in array)
{
if (string.Compare(x.text, "Hello") == 0)
{
// The stuff to do here
}
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291269%2fsearch-text-by-value-unity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
There is no built-in function for that, so you need to do it manually. It depends on your situation. In general you must:
- Have reference to all GameObjects that might have that text value
- Check each GameObject's text values with loop
If you need to look for that word once or twice then you can do something like this:
Text array = GameObject.FindObjectsOfType<Text>();
foreach (var x in array)
{
if (string.Compare(x.text,"Hello") == 0)
{
// The stuff to do here
}
}
If you need to keep looking for that word many times then you need store reference to those GameObject
s in some variable and access it anytime you need. This is because FindObjectsOfType<>();
is quite an expensive operation so we need to do it as little as possible. Something like this should work:
private Text array;
private void Awake()
{
Text array = GameObject.FindObjectsOfType<Text>();
}
private void Update()
{
foreach (var x in array)
{
if (string.Compare(x.text, "Hello") == 0)
{
// The stuff to do here
}
}
}
add a comment |
There is no built-in function for that, so you need to do it manually. It depends on your situation. In general you must:
- Have reference to all GameObjects that might have that text value
- Check each GameObject's text values with loop
If you need to look for that word once or twice then you can do something like this:
Text array = GameObject.FindObjectsOfType<Text>();
foreach (var x in array)
{
if (string.Compare(x.text,"Hello") == 0)
{
// The stuff to do here
}
}
If you need to keep looking for that word many times then you need store reference to those GameObject
s in some variable and access it anytime you need. This is because FindObjectsOfType<>();
is quite an expensive operation so we need to do it as little as possible. Something like this should work:
private Text array;
private void Awake()
{
Text array = GameObject.FindObjectsOfType<Text>();
}
private void Update()
{
foreach (var x in array)
{
if (string.Compare(x.text, "Hello") == 0)
{
// The stuff to do here
}
}
}
add a comment |
There is no built-in function for that, so you need to do it manually. It depends on your situation. In general you must:
- Have reference to all GameObjects that might have that text value
- Check each GameObject's text values with loop
If you need to look for that word once or twice then you can do something like this:
Text array = GameObject.FindObjectsOfType<Text>();
foreach (var x in array)
{
if (string.Compare(x.text,"Hello") == 0)
{
// The stuff to do here
}
}
If you need to keep looking for that word many times then you need store reference to those GameObject
s in some variable and access it anytime you need. This is because FindObjectsOfType<>();
is quite an expensive operation so we need to do it as little as possible. Something like this should work:
private Text array;
private void Awake()
{
Text array = GameObject.FindObjectsOfType<Text>();
}
private void Update()
{
foreach (var x in array)
{
if (string.Compare(x.text, "Hello") == 0)
{
// The stuff to do here
}
}
}
There is no built-in function for that, so you need to do it manually. It depends on your situation. In general you must:
- Have reference to all GameObjects that might have that text value
- Check each GameObject's text values with loop
If you need to look for that word once or twice then you can do something like this:
Text array = GameObject.FindObjectsOfType<Text>();
foreach (var x in array)
{
if (string.Compare(x.text,"Hello") == 0)
{
// The stuff to do here
}
}
If you need to keep looking for that word many times then you need store reference to those GameObject
s in some variable and access it anytime you need. This is because FindObjectsOfType<>();
is quite an expensive operation so we need to do it as little as possible. Something like this should work:
private Text array;
private void Awake()
{
Text array = GameObject.FindObjectsOfType<Text>();
}
private void Update()
{
foreach (var x in array)
{
if (string.Compare(x.text, "Hello") == 0)
{
// The stuff to do here
}
}
}
edited Nov 14 '18 at 2:41
AustinWBryan
2,33621332
2,33621332
answered Nov 14 '18 at 0:39
rCgLTrCgLT
812
812
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53291269%2fsearch-text-by-value-unity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WidHZ,S 7V9RLI1C,NhCOmR9DZ2uro,3q9VC Xvje0 PHxCz S62TfaI
It would be awesome if you could provide a Minimal, Complete, and Verifiable example.
– mjwills
Nov 14 '18 at 0:19