Write test to check local setState call with jest and testing-react-library
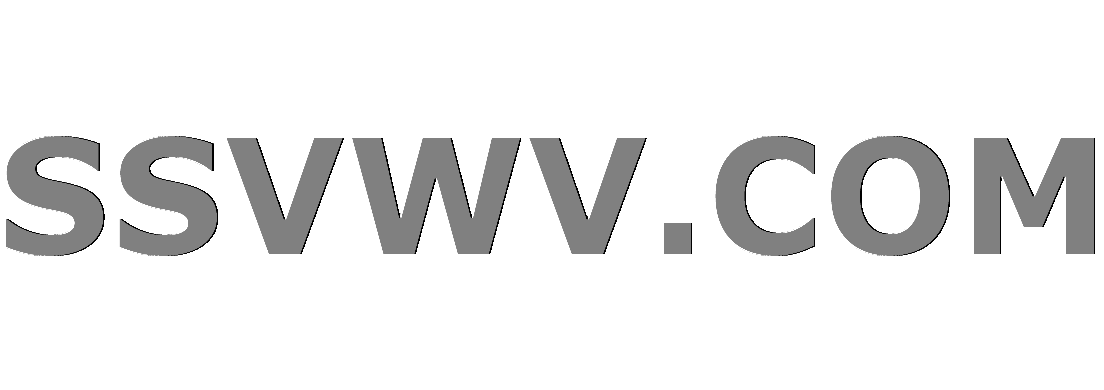
Multi tool use
I am currently using react-testing-library and can't seem to work out how to test setState for components.
In the following example, I am trying to test that the number of items loaded is correct based on the data from the API. Will later on expand this to test things like the interactions between of the items.
Component:
...
componentDidMount() {
this.getModules();
}
getModules () {
fetch('http://localhost:4000/api/query')
.then(res => res.json())
.then(res => this.setState({data : res.data}))
.catch(err => console.error(err))
}
...
render() {
return(
<div data-testid="list">
this.state.data.map((item) => {
return <Item key={item.id} data={item}/>
})
</div>
)
}
Test:
...
function renderWithRouter(
ui,
{route = '/', history = createMemoryHistory({initialEntries: [route]})} = {},) {
return {
...render(<Router history={history}>{ui}</Router>),
history,
}
}
...
test('<ListModule> check list items', () => {
const data = [ ... ]
//not sure what to do here, or after this
const { getByTestId } = renderWithRouter(<ListModule />)
...
//test the items loaded
expect(getByTestId('list').children.length).toBe(data.length)
//then will continue testing functionality
})
I understand this has to do with jest mock functions, but don't understand how to make them work with setting states, or with simulating an API.
Sample Implementation (doesn't work)
const data = [...]
fetchMock.get('http://localhost:4000/api/query', data);
const { getByText } = renderWithRouter(<ListModule />)
const listItem = await waitForElement(() =>
getByText('Sample Test Data Title')
)
javascript reactjs jestjs react-router-dom react-testing-library
add a comment |
I am currently using react-testing-library and can't seem to work out how to test setState for components.
In the following example, I am trying to test that the number of items loaded is correct based on the data from the API. Will later on expand this to test things like the interactions between of the items.
Component:
...
componentDidMount() {
this.getModules();
}
getModules () {
fetch('http://localhost:4000/api/query')
.then(res => res.json())
.then(res => this.setState({data : res.data}))
.catch(err => console.error(err))
}
...
render() {
return(
<div data-testid="list">
this.state.data.map((item) => {
return <Item key={item.id} data={item}/>
})
</div>
)
}
Test:
...
function renderWithRouter(
ui,
{route = '/', history = createMemoryHistory({initialEntries: [route]})} = {},) {
return {
...render(<Router history={history}>{ui}</Router>),
history,
}
}
...
test('<ListModule> check list items', () => {
const data = [ ... ]
//not sure what to do here, or after this
const { getByTestId } = renderWithRouter(<ListModule />)
...
//test the items loaded
expect(getByTestId('list').children.length).toBe(data.length)
//then will continue testing functionality
})
I understand this has to do with jest mock functions, but don't understand how to make them work with setting states, or with simulating an API.
Sample Implementation (doesn't work)
const data = [...]
fetchMock.get('http://localhost:4000/api/query', data);
const { getByText } = renderWithRouter(<ListModule />)
const listItem = await waitForElement(() =>
getByText('Sample Test Data Title')
)
javascript reactjs jestjs react-router-dom react-testing-library
This is exactly same problem, stackoverflow.com/questions/52767157/… .getModules
doesn't return a promise that could be chained in tests.fetch
should be mocked, as the answer suggests.
– estus
Nov 14 '18 at 7:17
add a comment |
I am currently using react-testing-library and can't seem to work out how to test setState for components.
In the following example, I am trying to test that the number of items loaded is correct based on the data from the API. Will later on expand this to test things like the interactions between of the items.
Component:
...
componentDidMount() {
this.getModules();
}
getModules () {
fetch('http://localhost:4000/api/query')
.then(res => res.json())
.then(res => this.setState({data : res.data}))
.catch(err => console.error(err))
}
...
render() {
return(
<div data-testid="list">
this.state.data.map((item) => {
return <Item key={item.id} data={item}/>
})
</div>
)
}
Test:
...
function renderWithRouter(
ui,
{route = '/', history = createMemoryHistory({initialEntries: [route]})} = {},) {
return {
...render(<Router history={history}>{ui}</Router>),
history,
}
}
...
test('<ListModule> check list items', () => {
const data = [ ... ]
//not sure what to do here, or after this
const { getByTestId } = renderWithRouter(<ListModule />)
...
//test the items loaded
expect(getByTestId('list').children.length).toBe(data.length)
//then will continue testing functionality
})
I understand this has to do with jest mock functions, but don't understand how to make them work with setting states, or with simulating an API.
Sample Implementation (doesn't work)
const data = [...]
fetchMock.get('http://localhost:4000/api/query', data);
const { getByText } = renderWithRouter(<ListModule />)
const listItem = await waitForElement(() =>
getByText('Sample Test Data Title')
)
javascript reactjs jestjs react-router-dom react-testing-library
I am currently using react-testing-library and can't seem to work out how to test setState for components.
In the following example, I am trying to test that the number of items loaded is correct based on the data from the API. Will later on expand this to test things like the interactions between of the items.
Component:
...
componentDidMount() {
this.getModules();
}
getModules () {
fetch('http://localhost:4000/api/query')
.then(res => res.json())
.then(res => this.setState({data : res.data}))
.catch(err => console.error(err))
}
...
render() {
return(
<div data-testid="list">
this.state.data.map((item) => {
return <Item key={item.id} data={item}/>
})
</div>
)
}
Test:
...
function renderWithRouter(
ui,
{route = '/', history = createMemoryHistory({initialEntries: [route]})} = {},) {
return {
...render(<Router history={history}>{ui}</Router>),
history,
}
}
...
test('<ListModule> check list items', () => {
const data = [ ... ]
//not sure what to do here, or after this
const { getByTestId } = renderWithRouter(<ListModule />)
...
//test the items loaded
expect(getByTestId('list').children.length).toBe(data.length)
//then will continue testing functionality
})
I understand this has to do with jest mock functions, but don't understand how to make them work with setting states, or with simulating an API.
Sample Implementation (doesn't work)
const data = [...]
fetchMock.get('http://localhost:4000/api/query', data);
const { getByText } = renderWithRouter(<ListModule />)
const listItem = await waitForElement(() =>
getByText('Sample Test Data Title')
)
javascript reactjs jestjs react-router-dom react-testing-library
javascript reactjs jestjs react-router-dom react-testing-library
edited Nov 15 '18 at 21:50
Charklewis
asked Nov 13 '18 at 21:54
CharklewisCharklewis
3417
3417
This is exactly same problem, stackoverflow.com/questions/52767157/… .getModules
doesn't return a promise that could be chained in tests.fetch
should be mocked, as the answer suggests.
– estus
Nov 14 '18 at 7:17
add a comment |
This is exactly same problem, stackoverflow.com/questions/52767157/… .getModules
doesn't return a promise that could be chained in tests.fetch
should be mocked, as the answer suggests.
– estus
Nov 14 '18 at 7:17
This is exactly same problem, stackoverflow.com/questions/52767157/… .
getModules
doesn't return a promise that could be chained in tests. fetch
should be mocked, as the answer suggests.– estus
Nov 14 '18 at 7:17
This is exactly same problem, stackoverflow.com/questions/52767157/… .
getModules
doesn't return a promise that could be chained in tests. fetch
should be mocked, as the answer suggests.– estus
Nov 14 '18 at 7:17
add a comment |
1 Answer
1
active
oldest
votes
You should avoid testing setState
directly since that is an implementation detail of the component. You are on the right path to testing that the correct number of items are rendered. You can mock the fetch
function by either replacing window.fetch
with a Jest mock function or using the fetch-mock library to handle the heavy lifting for you.
// Note that this method does not build the full response object like status codes, headers, etc.
window.fetch = jest.fn(() => {
return Promise.resolve({
json: () => Promise.resolve(fakeData),
});
});
OR
import fetchMock from "fetch-mock";
fetchMock.get(url, fakeData);
I have provided a sample solution that I can't seem to get working. It doesn't call the function in the component, are you able to guide me to where I am going wrong?
– Charklewis
Nov 15 '18 at 21:51
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290085%2fwrite-test-to-check-local-setstate-call-with-jest-and-testing-react-library%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You should avoid testing setState
directly since that is an implementation detail of the component. You are on the right path to testing that the correct number of items are rendered. You can mock the fetch
function by either replacing window.fetch
with a Jest mock function or using the fetch-mock library to handle the heavy lifting for you.
// Note that this method does not build the full response object like status codes, headers, etc.
window.fetch = jest.fn(() => {
return Promise.resolve({
json: () => Promise.resolve(fakeData),
});
});
OR
import fetchMock from "fetch-mock";
fetchMock.get(url, fakeData);
I have provided a sample solution that I can't seem to get working. It doesn't call the function in the component, are you able to guide me to where I am going wrong?
– Charklewis
Nov 15 '18 at 21:51
add a comment |
You should avoid testing setState
directly since that is an implementation detail of the component. You are on the right path to testing that the correct number of items are rendered. You can mock the fetch
function by either replacing window.fetch
with a Jest mock function or using the fetch-mock library to handle the heavy lifting for you.
// Note that this method does not build the full response object like status codes, headers, etc.
window.fetch = jest.fn(() => {
return Promise.resolve({
json: () => Promise.resolve(fakeData),
});
});
OR
import fetchMock from "fetch-mock";
fetchMock.get(url, fakeData);
I have provided a sample solution that I can't seem to get working. It doesn't call the function in the component, are you able to guide me to where I am going wrong?
– Charklewis
Nov 15 '18 at 21:51
add a comment |
You should avoid testing setState
directly since that is an implementation detail of the component. You are on the right path to testing that the correct number of items are rendered. You can mock the fetch
function by either replacing window.fetch
with a Jest mock function or using the fetch-mock library to handle the heavy lifting for you.
// Note that this method does not build the full response object like status codes, headers, etc.
window.fetch = jest.fn(() => {
return Promise.resolve({
json: () => Promise.resolve(fakeData),
});
});
OR
import fetchMock from "fetch-mock";
fetchMock.get(url, fakeData);
You should avoid testing setState
directly since that is an implementation detail of the component. You are on the right path to testing that the correct number of items are rendered. You can mock the fetch
function by either replacing window.fetch
with a Jest mock function or using the fetch-mock library to handle the heavy lifting for you.
// Note that this method does not build the full response object like status codes, headers, etc.
window.fetch = jest.fn(() => {
return Promise.resolve({
json: () => Promise.resolve(fakeData),
});
});
OR
import fetchMock from "fetch-mock";
fetchMock.get(url, fakeData);
answered Nov 14 '18 at 0:05


TylerTyler
1,00117
1,00117
I have provided a sample solution that I can't seem to get working. It doesn't call the function in the component, are you able to guide me to where I am going wrong?
– Charklewis
Nov 15 '18 at 21:51
add a comment |
I have provided a sample solution that I can't seem to get working. It doesn't call the function in the component, are you able to guide me to where I am going wrong?
– Charklewis
Nov 15 '18 at 21:51
I have provided a sample solution that I can't seem to get working. It doesn't call the function in the component, are you able to guide me to where I am going wrong?
– Charklewis
Nov 15 '18 at 21:51
I have provided a sample solution that I can't seem to get working. It doesn't call the function in the component, are you able to guide me to where I am going wrong?
– Charklewis
Nov 15 '18 at 21:51
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53290085%2fwrite-test-to-check-local-setstate-call-with-jest-and-testing-react-library%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
WvJiahyhG7aLjYpF0TXtGx5PyQZXGRV8
This is exactly same problem, stackoverflow.com/questions/52767157/… .
getModules
doesn't return a promise that could be chained in tests.fetch
should be mocked, as the answer suggests.– estus
Nov 14 '18 at 7:17