Deleting Files - Skip Files in Use
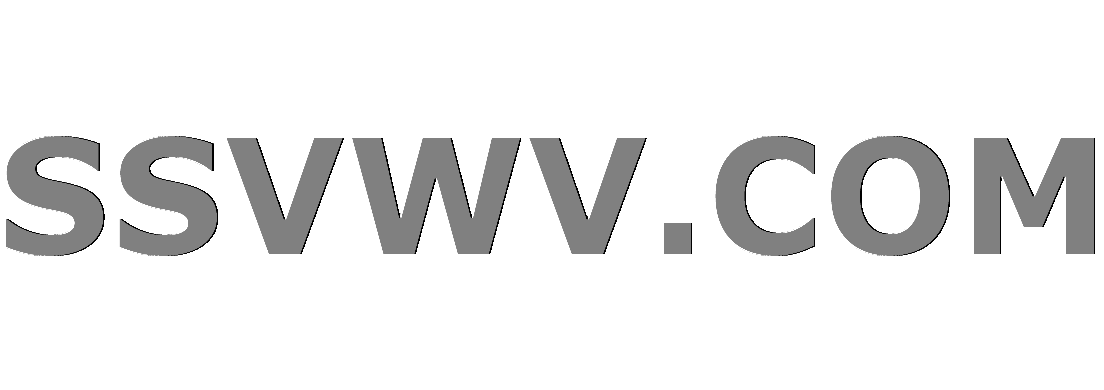
Multi tool use
I'm trying to delete the files in a directory, but I get errors when attempting to remove the files which are currently in use. Is there a way to skip the files that are currently in use and delete the rest of them? Thanks.
foreach(var file in Directory.GetFiles(tempPath))
{
File.Delete(file);
}
That's the code I have so far, not sure how to go about this.
c# file directory
add a comment |
I'm trying to delete the files in a directory, but I get errors when attempting to remove the files which are currently in use. Is there a way to skip the files that are currently in use and delete the rest of them? Thanks.
foreach(var file in Directory.GetFiles(tempPath))
{
File.Delete(file);
}
That's the code I have so far, not sure how to go about this.
c# file directory
I mean, the code won't delete the files that are in use, so it really doesn't even matter. If you don't want errors to show, you could try to catch the exception thrown when it tries deleting files in usage, but it really doesn't matter.
– Frontear
Nov 18 '18 at 14:27
add a comment |
I'm trying to delete the files in a directory, but I get errors when attempting to remove the files which are currently in use. Is there a way to skip the files that are currently in use and delete the rest of them? Thanks.
foreach(var file in Directory.GetFiles(tempPath))
{
File.Delete(file);
}
That's the code I have so far, not sure how to go about this.
c# file directory
I'm trying to delete the files in a directory, but I get errors when attempting to remove the files which are currently in use. Is there a way to skip the files that are currently in use and delete the rest of them? Thanks.
foreach(var file in Directory.GetFiles(tempPath))
{
File.Delete(file);
}
That's the code I have so far, not sure how to go about this.
c# file directory
c# file directory
edited Nov 18 '18 at 15:31
S.Akbari
30.3k93673
30.3k93673
asked Nov 18 '18 at 14:26
xJuicedxJuiced
183
183
I mean, the code won't delete the files that are in use, so it really doesn't even matter. If you don't want errors to show, you could try to catch the exception thrown when it tries deleting files in usage, but it really doesn't matter.
– Frontear
Nov 18 '18 at 14:27
add a comment |
I mean, the code won't delete the files that are in use, so it really doesn't even matter. If you don't want errors to show, you could try to catch the exception thrown when it tries deleting files in usage, but it really doesn't matter.
– Frontear
Nov 18 '18 at 14:27
I mean, the code won't delete the files that are in use, so it really doesn't even matter. If you don't want errors to show, you could try to catch the exception thrown when it tries deleting files in usage, but it really doesn't matter.
– Frontear
Nov 18 '18 at 14:27
I mean, the code won't delete the files that are in use, so it really doesn't even matter. If you don't want errors to show, you could try to catch the exception thrown when it tries deleting files in usage, but it really doesn't matter.
– Frontear
Nov 18 '18 at 14:27
add a comment |
3 Answers
3
active
oldest
votes
I think the easier way is surrounding your code with a try-catch
block. Something like this:
foreach(var file in Directory.GetFiles(tempPath))
{
try
{
File.Delete(file);
}
catch (Exception)
{
//Decide what you want to do here, you can either
//ask user to retry if the file is in use
//Or ignore the failure and continue, or...
}
}
It seems like I was over complicating it in my head, thanks for the help!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
you can check by try catch
private bool IsLocked(string filePath)
{
FileInfo f = new FileInfo(filePath);
FileStream stream = null;
try
{
stream = f.Open(FileMode.Open, FileAccess.Read, FileShare.None);
}
catch (IOException ex)
{
return true;
}
finally
{
if (stream != null)
stream.Close();
}
return false;
}
public void RemoveFile(string folderPath)
{
foreach (var file in Directory.GetFiles(folderPath))
{
if (!IsLocked(file))
{
File.Delete(file);
}
}
}
add a comment |
Wrap File.Delete in a try { } catch block
Oh yeah, this works thanks a lot!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53361930%2fdeleting-files-skip-files-in-use%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
I think the easier way is surrounding your code with a try-catch
block. Something like this:
foreach(var file in Directory.GetFiles(tempPath))
{
try
{
File.Delete(file);
}
catch (Exception)
{
//Decide what you want to do here, you can either
//ask user to retry if the file is in use
//Or ignore the failure and continue, or...
}
}
It seems like I was over complicating it in my head, thanks for the help!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
I think the easier way is surrounding your code with a try-catch
block. Something like this:
foreach(var file in Directory.GetFiles(tempPath))
{
try
{
File.Delete(file);
}
catch (Exception)
{
//Decide what you want to do here, you can either
//ask user to retry if the file is in use
//Or ignore the failure and continue, or...
}
}
It seems like I was over complicating it in my head, thanks for the help!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
I think the easier way is surrounding your code with a try-catch
block. Something like this:
foreach(var file in Directory.GetFiles(tempPath))
{
try
{
File.Delete(file);
}
catch (Exception)
{
//Decide what you want to do here, you can either
//ask user to retry if the file is in use
//Or ignore the failure and continue, or...
}
}
I think the easier way is surrounding your code with a try-catch
block. Something like this:
foreach(var file in Directory.GetFiles(tempPath))
{
try
{
File.Delete(file);
}
catch (Exception)
{
//Decide what you want to do here, you can either
//ask user to retry if the file is in use
//Or ignore the failure and continue, or...
}
}
edited Nov 18 '18 at 17:24
answered Nov 18 '18 at 14:32
S.AkbariS.Akbari
30.3k93673
30.3k93673
It seems like I was over complicating it in my head, thanks for the help!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
It seems like I was over complicating it in my head, thanks for the help!
– xJuiced
Nov 18 '18 at 14:37
It seems like I was over complicating it in my head, thanks for the help!
– xJuiced
Nov 18 '18 at 14:37
It seems like I was over complicating it in my head, thanks for the help!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
you can check by try catch
private bool IsLocked(string filePath)
{
FileInfo f = new FileInfo(filePath);
FileStream stream = null;
try
{
stream = f.Open(FileMode.Open, FileAccess.Read, FileShare.None);
}
catch (IOException ex)
{
return true;
}
finally
{
if (stream != null)
stream.Close();
}
return false;
}
public void RemoveFile(string folderPath)
{
foreach (var file in Directory.GetFiles(folderPath))
{
if (!IsLocked(file))
{
File.Delete(file);
}
}
}
add a comment |
you can check by try catch
private bool IsLocked(string filePath)
{
FileInfo f = new FileInfo(filePath);
FileStream stream = null;
try
{
stream = f.Open(FileMode.Open, FileAccess.Read, FileShare.None);
}
catch (IOException ex)
{
return true;
}
finally
{
if (stream != null)
stream.Close();
}
return false;
}
public void RemoveFile(string folderPath)
{
foreach (var file in Directory.GetFiles(folderPath))
{
if (!IsLocked(file))
{
File.Delete(file);
}
}
}
add a comment |
you can check by try catch
private bool IsLocked(string filePath)
{
FileInfo f = new FileInfo(filePath);
FileStream stream = null;
try
{
stream = f.Open(FileMode.Open, FileAccess.Read, FileShare.None);
}
catch (IOException ex)
{
return true;
}
finally
{
if (stream != null)
stream.Close();
}
return false;
}
public void RemoveFile(string folderPath)
{
foreach (var file in Directory.GetFiles(folderPath))
{
if (!IsLocked(file))
{
File.Delete(file);
}
}
}
you can check by try catch
private bool IsLocked(string filePath)
{
FileInfo f = new FileInfo(filePath);
FileStream stream = null;
try
{
stream = f.Open(FileMode.Open, FileAccess.Read, FileShare.None);
}
catch (IOException ex)
{
return true;
}
finally
{
if (stream != null)
stream.Close();
}
return false;
}
public void RemoveFile(string folderPath)
{
foreach (var file in Directory.GetFiles(folderPath))
{
if (!IsLocked(file))
{
File.Delete(file);
}
}
}
edited Nov 19 '18 at 7:29
answered Nov 18 '18 at 14:38


SAEED REZAEISAEED REZAEI
40424
40424
add a comment |
add a comment |
Wrap File.Delete in a try { } catch block
Oh yeah, this works thanks a lot!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
Wrap File.Delete in a try { } catch block
Oh yeah, this works thanks a lot!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
Wrap File.Delete in a try { } catch block
Wrap File.Delete in a try { } catch block
answered Nov 18 '18 at 14:28


Klaus GütterKlaus Gütter
2,45311321
2,45311321
Oh yeah, this works thanks a lot!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
Oh yeah, this works thanks a lot!
– xJuiced
Nov 18 '18 at 14:37
Oh yeah, this works thanks a lot!
– xJuiced
Nov 18 '18 at 14:37
Oh yeah, this works thanks a lot!
– xJuiced
Nov 18 '18 at 14:37
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53361930%2fdeleting-files-skip-files-in-use%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Xak9,ePZEMx,9Xb9uG nN3s3pAj
I mean, the code won't delete the files that are in use, so it really doesn't even matter. If you don't want errors to show, you could try to catch the exception thrown when it tries deleting files in usage, but it really doesn't matter.
– Frontear
Nov 18 '18 at 14:27