How to create a method for specific values in a column from a table?
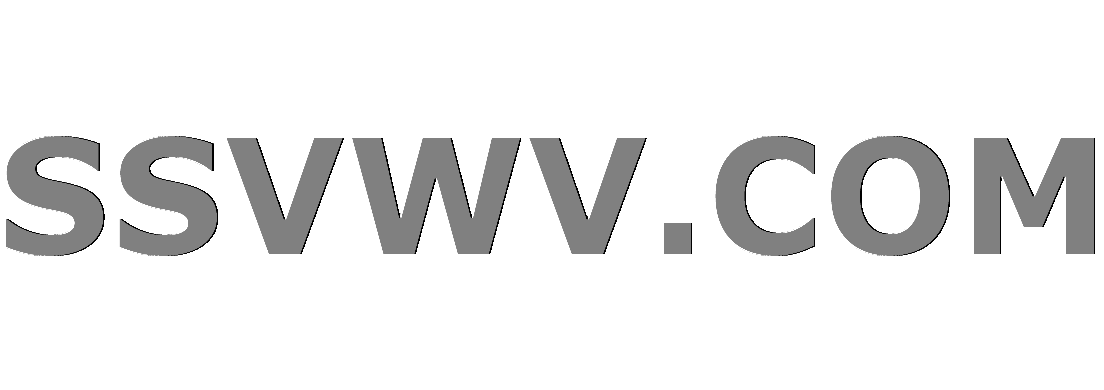
Multi tool use
Issue: I am looking for a way to have separate notices for different values in a tables column.
I have order_status that has 3 separate values, created, cancelled, and charged.
I would like 3 separate notices for each when the columns are changed from a view.
a view will be something like:
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
<%= f.button type:'submit', class: "btn btn-danger" %>
<% end %>
This will change the column to "cancelled".
I then want to create a method like:
def cancel_update
respond_to do |format|
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
end
But this method didn't work how i planned. When i use this method, nothing happens when i click the button, no page reload, redirect, etc. I get the error: "The action 'update' could not be found for OrdersController"
(This was tested by taking out the original update method - which is below).
Now when i use this update method, it works but doesn't pin point the value update on the order_status only.
def update
respond_to do |format|
if @order.update(order_params)
if user_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully uploaded.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
if buyer_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully updated.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
Here is another attempt that somehow didn't function:
def order_cancel
respond_to do |format|
if @order.update(order_status)
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { head :no_content }
end
end
end
private
def order_status
params.permit(:order_status)
end
How can I pin point the order_status values from the controller to allow me to have separate actions and notices when the values are changed by a end user?
ruby-on-rails ruby
add a comment |
Issue: I am looking for a way to have separate notices for different values in a tables column.
I have order_status that has 3 separate values, created, cancelled, and charged.
I would like 3 separate notices for each when the columns are changed from a view.
a view will be something like:
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
<%= f.button type:'submit', class: "btn btn-danger" %>
<% end %>
This will change the column to "cancelled".
I then want to create a method like:
def cancel_update
respond_to do |format|
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
end
But this method didn't work how i planned. When i use this method, nothing happens when i click the button, no page reload, redirect, etc. I get the error: "The action 'update' could not be found for OrdersController"
(This was tested by taking out the original update method - which is below).
Now when i use this update method, it works but doesn't pin point the value update on the order_status only.
def update
respond_to do |format|
if @order.update(order_params)
if user_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully uploaded.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
if buyer_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully updated.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
Here is another attempt that somehow didn't function:
def order_cancel
respond_to do |format|
if @order.update(order_status)
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { head :no_content }
end
end
end
private
def order_status
params.permit(:order_status)
end
How can I pin point the order_status values from the controller to allow me to have separate actions and notices when the values are changed by a end user?
ruby-on-rails ruby
add a comment |
Issue: I am looking for a way to have separate notices for different values in a tables column.
I have order_status that has 3 separate values, created, cancelled, and charged.
I would like 3 separate notices for each when the columns are changed from a view.
a view will be something like:
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
<%= f.button type:'submit', class: "btn btn-danger" %>
<% end %>
This will change the column to "cancelled".
I then want to create a method like:
def cancel_update
respond_to do |format|
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
end
But this method didn't work how i planned. When i use this method, nothing happens when i click the button, no page reload, redirect, etc. I get the error: "The action 'update' could not be found for OrdersController"
(This was tested by taking out the original update method - which is below).
Now when i use this update method, it works but doesn't pin point the value update on the order_status only.
def update
respond_to do |format|
if @order.update(order_params)
if user_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully uploaded.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
if buyer_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully updated.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
Here is another attempt that somehow didn't function:
def order_cancel
respond_to do |format|
if @order.update(order_status)
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { head :no_content }
end
end
end
private
def order_status
params.permit(:order_status)
end
How can I pin point the order_status values from the controller to allow me to have separate actions and notices when the values are changed by a end user?
ruby-on-rails ruby
Issue: I am looking for a way to have separate notices for different values in a tables column.
I have order_status that has 3 separate values, created, cancelled, and charged.
I would like 3 separate notices for each when the columns are changed from a view.
a view will be something like:
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
<%= f.button type:'submit', class: "btn btn-danger" %>
<% end %>
This will change the column to "cancelled".
I then want to create a method like:
def cancel_update
respond_to do |format|
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
end
But this method didn't work how i planned. When i use this method, nothing happens when i click the button, no page reload, redirect, etc. I get the error: "The action 'update' could not be found for OrdersController"
(This was tested by taking out the original update method - which is below).
Now when i use this update method, it works but doesn't pin point the value update on the order_status only.
def update
respond_to do |format|
if @order.update(order_params)
if user_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully uploaded.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
if buyer_signed_in?
format.html { redirect_to @order, notice: 'Order was successfully updated.' }
format.json { render :show, status: :ok, location: @order }
else
format.html { render :edit }
format.json { render json: @order.errors, status: :unprocessable_entity }
end
end
end
end
Here is another attempt that somehow didn't function:
def order_cancel
respond_to do |format|
if @order.update(order_status)
format.html { redirect_to @order, notice: 'Order was successfully cancelled.' }
format.json { head :no_content }
end
end
end
private
def order_status
params.permit(:order_status)
end
How can I pin point the order_status values from the controller to allow me to have separate actions and notices when the values are changed by a end user?
ruby-on-rails ruby
ruby-on-rails ruby
asked Nov 17 '18 at 5:25
unouno
15219
15219
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Given that you use the Rails form builder to generate the form's HTML
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
I would expect that the generated name of the input field is nested under order
. That said you will need to follow these nesting when permitting the params:
def order_status
params.require(:order).permit(:order_status)
end
When you are unsure how the parameters really look like you might want to have a look at the generated HTML structure of the form or you can look at the Rails logs for the update
request to the application.
add a comment |
So, those three lines are wrong:
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
Should be:
if user_signed_in?
if @order.update(status: params[:order_status])
if @order.order_status == "cancelled"
But actually should be @order.update!(status: :cancelled)
in a cancel
action, or at least have a state machine to validate that the user is not messing up the states of the orders.
Or like is expected by your form, those should be in a update
method (not cancel_update
)
The update
method you posted doesn't make sense, it has a minimum of 2 renders, I think you meant to not have the buyer_signed_in
section.
Thanks! ill give this a try in a few!
– uno
Nov 17 '18 at 12:02
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348517%2fhow-to-create-a-method-for-specific-values-in-a-column-from-a-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Given that you use the Rails form builder to generate the form's HTML
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
I would expect that the generated name of the input field is nested under order
. That said you will need to follow these nesting when permitting the params:
def order_status
params.require(:order).permit(:order_status)
end
When you are unsure how the parameters really look like you might want to have a look at the generated HTML structure of the form or you can look at the Rails logs for the update
request to the application.
add a comment |
Given that you use the Rails form builder to generate the form's HTML
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
I would expect that the generated name of the input field is nested under order
. That said you will need to follow these nesting when permitting the params:
def order_status
params.require(:order).permit(:order_status)
end
When you are unsure how the parameters really look like you might want to have a look at the generated HTML structure of the form or you can look at the Rails logs for the update
request to the application.
add a comment |
Given that you use the Rails form builder to generate the form's HTML
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
I would expect that the generated name of the input field is nested under order
. That said you will need to follow these nesting when permitting the params:
def order_status
params.require(:order).permit(:order_status)
end
When you are unsure how the parameters really look like you might want to have a look at the generated HTML structure of the form or you can look at the Rails logs for the update
request to the application.
Given that you use the Rails form builder to generate the form's HTML
<%= form_for @order, remote: true do |f| %>
<%= f.hidden_field :order_status, value: "cancelled" %>
I would expect that the generated name of the input field is nested under order
. That said you will need to follow these nesting when permitting the params:
def order_status
params.require(:order).permit(:order_status)
end
When you are unsure how the parameters really look like you might want to have a look at the generated HTML structure of the form or you can look at the Rails logs for the update
request to the application.
answered Nov 17 '18 at 7:47


spickermannspickermann
59.4k65676
59.4k65676
add a comment |
add a comment |
So, those three lines are wrong:
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
Should be:
if user_signed_in?
if @order.update(status: params[:order_status])
if @order.order_status == "cancelled"
But actually should be @order.update!(status: :cancelled)
in a cancel
action, or at least have a state machine to validate that the user is not messing up the states of the orders.
Or like is expected by your form, those should be in a update
method (not cancel_update
)
The update
method you posted doesn't make sense, it has a minimum of 2 renders, I think you meant to not have the buyer_signed_in
section.
Thanks! ill give this a try in a few!
– uno
Nov 17 '18 at 12:02
add a comment |
So, those three lines are wrong:
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
Should be:
if user_signed_in?
if @order.update(status: params[:order_status])
if @order.order_status == "cancelled"
But actually should be @order.update!(status: :cancelled)
in a cancel
action, or at least have a state machine to validate that the user is not messing up the states of the orders.
Or like is expected by your form, those should be in a update
method (not cancel_update
)
The update
method you posted doesn't make sense, it has a minimum of 2 renders, I think you meant to not have the buyer_signed_in
section.
Thanks! ill give this a try in a few!
– uno
Nov 17 '18 at 12:02
add a comment |
So, those three lines are wrong:
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
Should be:
if user_signed_in?
if @order.update(status: params[:order_status])
if @order.order_status == "cancelled"
But actually should be @order.update!(status: :cancelled)
in a cancel
action, or at least have a state machine to validate that the user is not messing up the states of the orders.
Or like is expected by your form, those should be in a update
method (not cancel_update
)
The update
method you posted doesn't make sense, it has a minimum of 2 renders, I think you meant to not have the buyer_signed_in
section.
So, those three lines are wrong:
if @order.update(params[:order_update])
if user_signed_in?
if @order.order_status = "cancelled"
Should be:
if user_signed_in?
if @order.update(status: params[:order_status])
if @order.order_status == "cancelled"
But actually should be @order.update!(status: :cancelled)
in a cancel
action, or at least have a state machine to validate that the user is not messing up the states of the orders.
Or like is expected by your form, those should be in a update
method (not cancel_update
)
The update
method you posted doesn't make sense, it has a minimum of 2 renders, I think you meant to not have the buyer_signed_in
section.
answered Nov 17 '18 at 11:58


DorianDorian
12.9k37385
12.9k37385
Thanks! ill give this a try in a few!
– uno
Nov 17 '18 at 12:02
add a comment |
Thanks! ill give this a try in a few!
– uno
Nov 17 '18 at 12:02
Thanks! ill give this a try in a few!
– uno
Nov 17 '18 at 12:02
Thanks! ill give this a try in a few!
– uno
Nov 17 '18 at 12:02
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348517%2fhow-to-create-a-method-for-specific-values-in-a-column-from-a-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
pARNfbcdtZgkGXWOju iyHoop0GR5 iyNN,tak6rxuio0wRUWXZl ri 7Q9x jF,MKY3l