Repeating a method call on each direcotry with timer elasped in C#
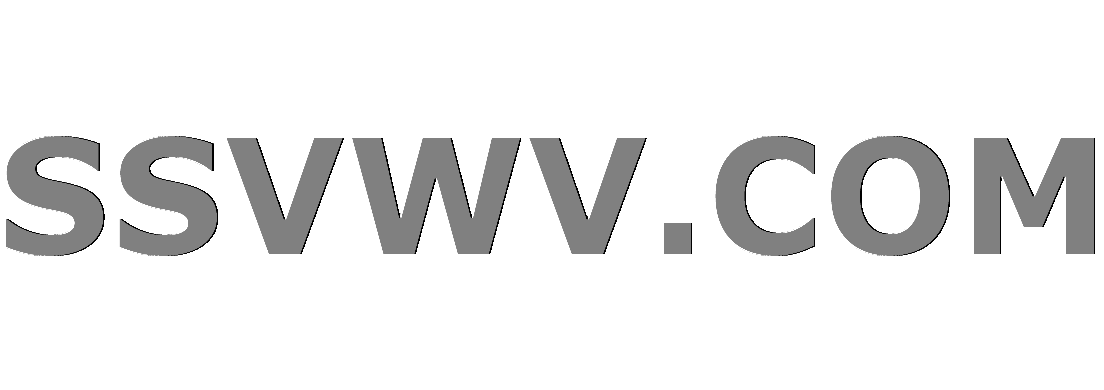
Multi tool use
Overview:
I've created two FileSystemWatcher
to detect sub-folders and its files creation. I also use a Timer
to the second watcher(file watcher), which restart the timer for every file created to any of each folder before calling a zip method dynamically.
I try to make this process to every folder, but it zips all folder at once regardless if there is a file moving into those folders.
Below is part of my code to simulate the timer and zip process... I might have a problem moving the zip file to root folder when calling the zip method.
The Timer inside the file Watcher
namespace ZipperAndWatcher
{
public class Archive
{
public void CreateZip(string path)
{
// zip method code
}
}
public class FileWatcher
{
private FileSystemWatcher _fsw;
private Archive _zip;
private string _path;
private Timer _timer;
public FileWatcher()
{
_fsw = new FileSystemWatcher();
_zip = new Archive();
_path = @"D:Documentsoutput";
_timer = new Timer();
}
public void StartWatching()
{
var rootWatcher = _fsw;
rootWatcher.Path = _path;
rootWatcher.Created += new FileSystemEventHandler(OnRootFolderCreated);
rootWatcher.EnableRaisingEvents = true;
rootWatcher.Filter = "*.*";
}
private void OnRootFolderCreated(object sender, FileSystemEventArgs e)
{
string watchedPath = e.FullPath; // watched path
// create another watcher for file creation and send event to timer
FileSystemWatcher subFolderWatcher = new FileSystemWatcher();
subFolderWatcher.Path = watchedPath + @"";
// Timer setting
var aTimer = _timer;
aTimer.Interval = 20000;
// Lambda == args => expression
// send event to subFolderWatcher
aTimer.Elapsed += new ElapsedEventHandler((subfolderSender, evt) => OnTimedEvent(subfolderSender, evt, subFolderWatcher));
aTimer.AutoReset = false;
aTimer.Enabled = true;
// sub-folder sends event to timer (and wait timer to notify subfolder)
subFolderWatcher.Created += new FileSystemEventHandler((s, evt) => subFolderWatcher_Created(s, evt, aTimer));
subFolderWatcher.Filter = "*.*";
subFolderWatcher.EnableRaisingEvents = true;
}
private void OnTimedEvent(object sender, ElapsedEventArgs evt, FileSystemWatcher subFolderWatcher)
{
subFolderWatcher.EnableRaisingEvents = false;
// Explicit Casting
Timer timer = sender as Timer;
timer.Stop();
timer.Dispose();
// Once time elapsed, zip the folder here?
Console.WriteLine($"time up. zip process at {evt.SignalTime}");
Archive zip = _zip;
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
subFolderWatcher.Dispose();
}
private void subFolderWatcher_Created(object sender, FileSystemEventArgs evt, Timer aTimer)
{
// if new file created, stop the timer
// then restart the timer
aTimer.AutoReset = false;
aTimer.Stop();
aTimer.Start();
Console.WriteLine($"restart the timer as {evt.Name} created on {DateTime.Now.ToString()}");
}
}
}
c# timer filesystems zip filesystemwatcher
|
show 1 more comment
Overview:
I've created two FileSystemWatcher
to detect sub-folders and its files creation. I also use a Timer
to the second watcher(file watcher), which restart the timer for every file created to any of each folder before calling a zip method dynamically.
I try to make this process to every folder, but it zips all folder at once regardless if there is a file moving into those folders.
Below is part of my code to simulate the timer and zip process... I might have a problem moving the zip file to root folder when calling the zip method.
The Timer inside the file Watcher
namespace ZipperAndWatcher
{
public class Archive
{
public void CreateZip(string path)
{
// zip method code
}
}
public class FileWatcher
{
private FileSystemWatcher _fsw;
private Archive _zip;
private string _path;
private Timer _timer;
public FileWatcher()
{
_fsw = new FileSystemWatcher();
_zip = new Archive();
_path = @"D:Documentsoutput";
_timer = new Timer();
}
public void StartWatching()
{
var rootWatcher = _fsw;
rootWatcher.Path = _path;
rootWatcher.Created += new FileSystemEventHandler(OnRootFolderCreated);
rootWatcher.EnableRaisingEvents = true;
rootWatcher.Filter = "*.*";
}
private void OnRootFolderCreated(object sender, FileSystemEventArgs e)
{
string watchedPath = e.FullPath; // watched path
// create another watcher for file creation and send event to timer
FileSystemWatcher subFolderWatcher = new FileSystemWatcher();
subFolderWatcher.Path = watchedPath + @"";
// Timer setting
var aTimer = _timer;
aTimer.Interval = 20000;
// Lambda == args => expression
// send event to subFolderWatcher
aTimer.Elapsed += new ElapsedEventHandler((subfolderSender, evt) => OnTimedEvent(subfolderSender, evt, subFolderWatcher));
aTimer.AutoReset = false;
aTimer.Enabled = true;
// sub-folder sends event to timer (and wait timer to notify subfolder)
subFolderWatcher.Created += new FileSystemEventHandler((s, evt) => subFolderWatcher_Created(s, evt, aTimer));
subFolderWatcher.Filter = "*.*";
subFolderWatcher.EnableRaisingEvents = true;
}
private void OnTimedEvent(object sender, ElapsedEventArgs evt, FileSystemWatcher subFolderWatcher)
{
subFolderWatcher.EnableRaisingEvents = false;
// Explicit Casting
Timer timer = sender as Timer;
timer.Stop();
timer.Dispose();
// Once time elapsed, zip the folder here?
Console.WriteLine($"time up. zip process at {evt.SignalTime}");
Archive zip = _zip;
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
subFolderWatcher.Dispose();
}
private void subFolderWatcher_Created(object sender, FileSystemEventArgs evt, Timer aTimer)
{
// if new file created, stop the timer
// then restart the timer
aTimer.AutoReset = false;
aTimer.Stop();
aTimer.Start();
Console.WriteLine($"restart the timer as {evt.Name} created on {DateTime.Now.ToString()}");
}
}
}
c# timer filesystems zip filesystemwatcher
How do you set up the file system watchers and their timers? I wonder how the event handlers get their extra parameters.
– Klaus Gütter
Nov 17 '18 at 6:58
I set the 2nd watcher and timer inside the OnCreated event handler of the root folder, extended with the code above, which the 2nd watcher sends an event to the timer and also the timer sends an event to the 2nd watcher to interact each other to some extent. I'm not sure how to proceed the zip process to each folder individually.
– Stu_Dent
Nov 17 '18 at 7:37
Could you please add this code to the question?
– Klaus Gütter
Nov 17 '18 at 7:46
@KlausGütter my code might be long and ugly, but you can find it here. My apology.
– Stu_Dent
Nov 18 '18 at 9:30
1
Frankly, I don't get your probem. Is it that your CreateZip method always zips all subdirectories and not only the one where a new file has been added? If you do not want this, just pass the path to the correct directory to the method and remove the foreach loop on the subdirectories from CreateZip.
– Klaus Gütter
Nov 18 '18 at 10:50
|
show 1 more comment
Overview:
I've created two FileSystemWatcher
to detect sub-folders and its files creation. I also use a Timer
to the second watcher(file watcher), which restart the timer for every file created to any of each folder before calling a zip method dynamically.
I try to make this process to every folder, but it zips all folder at once regardless if there is a file moving into those folders.
Below is part of my code to simulate the timer and zip process... I might have a problem moving the zip file to root folder when calling the zip method.
The Timer inside the file Watcher
namespace ZipperAndWatcher
{
public class Archive
{
public void CreateZip(string path)
{
// zip method code
}
}
public class FileWatcher
{
private FileSystemWatcher _fsw;
private Archive _zip;
private string _path;
private Timer _timer;
public FileWatcher()
{
_fsw = new FileSystemWatcher();
_zip = new Archive();
_path = @"D:Documentsoutput";
_timer = new Timer();
}
public void StartWatching()
{
var rootWatcher = _fsw;
rootWatcher.Path = _path;
rootWatcher.Created += new FileSystemEventHandler(OnRootFolderCreated);
rootWatcher.EnableRaisingEvents = true;
rootWatcher.Filter = "*.*";
}
private void OnRootFolderCreated(object sender, FileSystemEventArgs e)
{
string watchedPath = e.FullPath; // watched path
// create another watcher for file creation and send event to timer
FileSystemWatcher subFolderWatcher = new FileSystemWatcher();
subFolderWatcher.Path = watchedPath + @"";
// Timer setting
var aTimer = _timer;
aTimer.Interval = 20000;
// Lambda == args => expression
// send event to subFolderWatcher
aTimer.Elapsed += new ElapsedEventHandler((subfolderSender, evt) => OnTimedEvent(subfolderSender, evt, subFolderWatcher));
aTimer.AutoReset = false;
aTimer.Enabled = true;
// sub-folder sends event to timer (and wait timer to notify subfolder)
subFolderWatcher.Created += new FileSystemEventHandler((s, evt) => subFolderWatcher_Created(s, evt, aTimer));
subFolderWatcher.Filter = "*.*";
subFolderWatcher.EnableRaisingEvents = true;
}
private void OnTimedEvent(object sender, ElapsedEventArgs evt, FileSystemWatcher subFolderWatcher)
{
subFolderWatcher.EnableRaisingEvents = false;
// Explicit Casting
Timer timer = sender as Timer;
timer.Stop();
timer.Dispose();
// Once time elapsed, zip the folder here?
Console.WriteLine($"time up. zip process at {evt.SignalTime}");
Archive zip = _zip;
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
subFolderWatcher.Dispose();
}
private void subFolderWatcher_Created(object sender, FileSystemEventArgs evt, Timer aTimer)
{
// if new file created, stop the timer
// then restart the timer
aTimer.AutoReset = false;
aTimer.Stop();
aTimer.Start();
Console.WriteLine($"restart the timer as {evt.Name} created on {DateTime.Now.ToString()}");
}
}
}
c# timer filesystems zip filesystemwatcher
Overview:
I've created two FileSystemWatcher
to detect sub-folders and its files creation. I also use a Timer
to the second watcher(file watcher), which restart the timer for every file created to any of each folder before calling a zip method dynamically.
I try to make this process to every folder, but it zips all folder at once regardless if there is a file moving into those folders.
Below is part of my code to simulate the timer and zip process... I might have a problem moving the zip file to root folder when calling the zip method.
The Timer inside the file Watcher
namespace ZipperAndWatcher
{
public class Archive
{
public void CreateZip(string path)
{
// zip method code
}
}
public class FileWatcher
{
private FileSystemWatcher _fsw;
private Archive _zip;
private string _path;
private Timer _timer;
public FileWatcher()
{
_fsw = new FileSystemWatcher();
_zip = new Archive();
_path = @"D:Documentsoutput";
_timer = new Timer();
}
public void StartWatching()
{
var rootWatcher = _fsw;
rootWatcher.Path = _path;
rootWatcher.Created += new FileSystemEventHandler(OnRootFolderCreated);
rootWatcher.EnableRaisingEvents = true;
rootWatcher.Filter = "*.*";
}
private void OnRootFolderCreated(object sender, FileSystemEventArgs e)
{
string watchedPath = e.FullPath; // watched path
// create another watcher for file creation and send event to timer
FileSystemWatcher subFolderWatcher = new FileSystemWatcher();
subFolderWatcher.Path = watchedPath + @"";
// Timer setting
var aTimer = _timer;
aTimer.Interval = 20000;
// Lambda == args => expression
// send event to subFolderWatcher
aTimer.Elapsed += new ElapsedEventHandler((subfolderSender, evt) => OnTimedEvent(subfolderSender, evt, subFolderWatcher));
aTimer.AutoReset = false;
aTimer.Enabled = true;
// sub-folder sends event to timer (and wait timer to notify subfolder)
subFolderWatcher.Created += new FileSystemEventHandler((s, evt) => subFolderWatcher_Created(s, evt, aTimer));
subFolderWatcher.Filter = "*.*";
subFolderWatcher.EnableRaisingEvents = true;
}
private void OnTimedEvent(object sender, ElapsedEventArgs evt, FileSystemWatcher subFolderWatcher)
{
subFolderWatcher.EnableRaisingEvents = false;
// Explicit Casting
Timer timer = sender as Timer;
timer.Stop();
timer.Dispose();
// Once time elapsed, zip the folder here?
Console.WriteLine($"time up. zip process at {evt.SignalTime}");
Archive zip = _zip;
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
subFolderWatcher.Dispose();
}
private void subFolderWatcher_Created(object sender, FileSystemEventArgs evt, Timer aTimer)
{
// if new file created, stop the timer
// then restart the timer
aTimer.AutoReset = false;
aTimer.Stop();
aTimer.Start();
Console.WriteLine($"restart the timer as {evt.Name} created on {DateTime.Now.ToString()}");
}
}
}
c# timer filesystems zip filesystemwatcher
c# timer filesystems zip filesystemwatcher
edited Nov 17 '18 at 12:33
Stu_Dent
asked Nov 17 '18 at 5:35


Stu_DentStu_Dent
70110
70110
How do you set up the file system watchers and their timers? I wonder how the event handlers get their extra parameters.
– Klaus Gütter
Nov 17 '18 at 6:58
I set the 2nd watcher and timer inside the OnCreated event handler of the root folder, extended with the code above, which the 2nd watcher sends an event to the timer and also the timer sends an event to the 2nd watcher to interact each other to some extent. I'm not sure how to proceed the zip process to each folder individually.
– Stu_Dent
Nov 17 '18 at 7:37
Could you please add this code to the question?
– Klaus Gütter
Nov 17 '18 at 7:46
@KlausGütter my code might be long and ugly, but you can find it here. My apology.
– Stu_Dent
Nov 18 '18 at 9:30
1
Frankly, I don't get your probem. Is it that your CreateZip method always zips all subdirectories and not only the one where a new file has been added? If you do not want this, just pass the path to the correct directory to the method and remove the foreach loop on the subdirectories from CreateZip.
– Klaus Gütter
Nov 18 '18 at 10:50
|
show 1 more comment
How do you set up the file system watchers and their timers? I wonder how the event handlers get their extra parameters.
– Klaus Gütter
Nov 17 '18 at 6:58
I set the 2nd watcher and timer inside the OnCreated event handler of the root folder, extended with the code above, which the 2nd watcher sends an event to the timer and also the timer sends an event to the 2nd watcher to interact each other to some extent. I'm not sure how to proceed the zip process to each folder individually.
– Stu_Dent
Nov 17 '18 at 7:37
Could you please add this code to the question?
– Klaus Gütter
Nov 17 '18 at 7:46
@KlausGütter my code might be long and ugly, but you can find it here. My apology.
– Stu_Dent
Nov 18 '18 at 9:30
1
Frankly, I don't get your probem. Is it that your CreateZip method always zips all subdirectories and not only the one where a new file has been added? If you do not want this, just pass the path to the correct directory to the method and remove the foreach loop on the subdirectories from CreateZip.
– Klaus Gütter
Nov 18 '18 at 10:50
How do you set up the file system watchers and their timers? I wonder how the event handlers get their extra parameters.
– Klaus Gütter
Nov 17 '18 at 6:58
How do you set up the file system watchers and their timers? I wonder how the event handlers get their extra parameters.
– Klaus Gütter
Nov 17 '18 at 6:58
I set the 2nd watcher and timer inside the OnCreated event handler of the root folder, extended with the code above, which the 2nd watcher sends an event to the timer and also the timer sends an event to the 2nd watcher to interact each other to some extent. I'm not sure how to proceed the zip process to each folder individually.
– Stu_Dent
Nov 17 '18 at 7:37
I set the 2nd watcher and timer inside the OnCreated event handler of the root folder, extended with the code above, which the 2nd watcher sends an event to the timer and also the timer sends an event to the 2nd watcher to interact each other to some extent. I'm not sure how to proceed the zip process to each folder individually.
– Stu_Dent
Nov 17 '18 at 7:37
Could you please add this code to the question?
– Klaus Gütter
Nov 17 '18 at 7:46
Could you please add this code to the question?
– Klaus Gütter
Nov 17 '18 at 7:46
@KlausGütter my code might be long and ugly, but you can find it here. My apology.
– Stu_Dent
Nov 18 '18 at 9:30
@KlausGütter my code might be long and ugly, but you can find it here. My apology.
– Stu_Dent
Nov 18 '18 at 9:30
1
1
Frankly, I don't get your probem. Is it that your CreateZip method always zips all subdirectories and not only the one where a new file has been added? If you do not want this, just pass the path to the correct directory to the method and remove the foreach loop on the subdirectories from CreateZip.
– Klaus Gütter
Nov 18 '18 at 10:50
Frankly, I don't get your probem. Is it that your CreateZip method always zips all subdirectories and not only the one where a new file has been added? If you do not want this, just pass the path to the correct directory to the method and remove the foreach loop on the subdirectories from CreateZip.
– Klaus Gütter
Nov 18 '18 at 10:50
|
show 1 more comment
1 Answer
1
active
oldest
votes
You wrote in your last comment: "what I want is to only zip the specific subdirectories, which has no more files being added after the time elapsed while other subdirectories might still have files adding in, and it is not ready to be zipped"
So in your timer handler (OnTimedEvent), you need to pass the path to the directory that is ready for zipping to CreateZip, not the parent directory, i.e. change
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
to
zip.CreateZip(subFolderWatcher.Path);
Then in your CreateZip method just zip the directory that is passed as parameter, not all subdirectories as you do now.
Thanks, that works, but what If I want each subdirectory zip itself at different time elapsed, Wonder should I remove theforeach
in the zip method?
– Stu_Dent
Nov 18 '18 at 12:43
1
If I understood your code correctly, you have a timer per subdirectory, so these would already operate independently.
– Klaus Gütter
Nov 18 '18 at 12:44
Yes, it is exactly what the timer does, but I want each subdirectory should zip itself once (1)time elapsed and (2)no more files being added in that subdirectory. I still cannot make this works.
– Stu_Dent
Nov 18 '18 at 12:51
1
Don't you already restart the timer whenever a new file arrives? This should handle (1) and (2).
– Klaus Gütter
Nov 18 '18 at 13:45
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348552%2frepeating-a-method-call-on-each-direcotry-with-timer-elasped-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You wrote in your last comment: "what I want is to only zip the specific subdirectories, which has no more files being added after the time elapsed while other subdirectories might still have files adding in, and it is not ready to be zipped"
So in your timer handler (OnTimedEvent), you need to pass the path to the directory that is ready for zipping to CreateZip, not the parent directory, i.e. change
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
to
zip.CreateZip(subFolderWatcher.Path);
Then in your CreateZip method just zip the directory that is passed as parameter, not all subdirectories as you do now.
Thanks, that works, but what If I want each subdirectory zip itself at different time elapsed, Wonder should I remove theforeach
in the zip method?
– Stu_Dent
Nov 18 '18 at 12:43
1
If I understood your code correctly, you have a timer per subdirectory, so these would already operate independently.
– Klaus Gütter
Nov 18 '18 at 12:44
Yes, it is exactly what the timer does, but I want each subdirectory should zip itself once (1)time elapsed and (2)no more files being added in that subdirectory. I still cannot make this works.
– Stu_Dent
Nov 18 '18 at 12:51
1
Don't you already restart the timer whenever a new file arrives? This should handle (1) and (2).
– Klaus Gütter
Nov 18 '18 at 13:45
add a comment |
You wrote in your last comment: "what I want is to only zip the specific subdirectories, which has no more files being added after the time elapsed while other subdirectories might still have files adding in, and it is not ready to be zipped"
So in your timer handler (OnTimedEvent), you need to pass the path to the directory that is ready for zipping to CreateZip, not the parent directory, i.e. change
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
to
zip.CreateZip(subFolderWatcher.Path);
Then in your CreateZip method just zip the directory that is passed as parameter, not all subdirectories as you do now.
Thanks, that works, but what If I want each subdirectory zip itself at different time elapsed, Wonder should I remove theforeach
in the zip method?
– Stu_Dent
Nov 18 '18 at 12:43
1
If I understood your code correctly, you have a timer per subdirectory, so these would already operate independently.
– Klaus Gütter
Nov 18 '18 at 12:44
Yes, it is exactly what the timer does, but I want each subdirectory should zip itself once (1)time elapsed and (2)no more files being added in that subdirectory. I still cannot make this works.
– Stu_Dent
Nov 18 '18 at 12:51
1
Don't you already restart the timer whenever a new file arrives? This should handle (1) and (2).
– Klaus Gütter
Nov 18 '18 at 13:45
add a comment |
You wrote in your last comment: "what I want is to only zip the specific subdirectories, which has no more files being added after the time elapsed while other subdirectories might still have files adding in, and it is not ready to be zipped"
So in your timer handler (OnTimedEvent), you need to pass the path to the directory that is ready for zipping to CreateZip, not the parent directory, i.e. change
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
to
zip.CreateZip(subFolderWatcher.Path);
Then in your CreateZip method just zip the directory that is passed as parameter, not all subdirectories as you do now.
You wrote in your last comment: "what I want is to only zip the specific subdirectories, which has no more files being added after the time elapsed while other subdirectories might still have files adding in, and it is not ready to be zipped"
So in your timer handler (OnTimedEvent), you need to pass the path to the directory that is ready for zipping to CreateZip, not the parent directory, i.e. change
zip.CreateZip(subFolderWatcher.Path.Substring(0, subFolderWatcher.Path.LastIndexOf(@"")));
to
zip.CreateZip(subFolderWatcher.Path);
Then in your CreateZip method just zip the directory that is passed as parameter, not all subdirectories as you do now.
answered Nov 18 '18 at 11:38


Klaus GütterKlaus Gütter
2,40311321
2,40311321
Thanks, that works, but what If I want each subdirectory zip itself at different time elapsed, Wonder should I remove theforeach
in the zip method?
– Stu_Dent
Nov 18 '18 at 12:43
1
If I understood your code correctly, you have a timer per subdirectory, so these would already operate independently.
– Klaus Gütter
Nov 18 '18 at 12:44
Yes, it is exactly what the timer does, but I want each subdirectory should zip itself once (1)time elapsed and (2)no more files being added in that subdirectory. I still cannot make this works.
– Stu_Dent
Nov 18 '18 at 12:51
1
Don't you already restart the timer whenever a new file arrives? This should handle (1) and (2).
– Klaus Gütter
Nov 18 '18 at 13:45
add a comment |
Thanks, that works, but what If I want each subdirectory zip itself at different time elapsed, Wonder should I remove theforeach
in the zip method?
– Stu_Dent
Nov 18 '18 at 12:43
1
If I understood your code correctly, you have a timer per subdirectory, so these would already operate independently.
– Klaus Gütter
Nov 18 '18 at 12:44
Yes, it is exactly what the timer does, but I want each subdirectory should zip itself once (1)time elapsed and (2)no more files being added in that subdirectory. I still cannot make this works.
– Stu_Dent
Nov 18 '18 at 12:51
1
Don't you already restart the timer whenever a new file arrives? This should handle (1) and (2).
– Klaus Gütter
Nov 18 '18 at 13:45
Thanks, that works, but what If I want each subdirectory zip itself at different time elapsed, Wonder should I remove the
foreach
in the zip method?– Stu_Dent
Nov 18 '18 at 12:43
Thanks, that works, but what If I want each subdirectory zip itself at different time elapsed, Wonder should I remove the
foreach
in the zip method?– Stu_Dent
Nov 18 '18 at 12:43
1
1
If I understood your code correctly, you have a timer per subdirectory, so these would already operate independently.
– Klaus Gütter
Nov 18 '18 at 12:44
If I understood your code correctly, you have a timer per subdirectory, so these would already operate independently.
– Klaus Gütter
Nov 18 '18 at 12:44
Yes, it is exactly what the timer does, but I want each subdirectory should zip itself once (1)time elapsed and (2)no more files being added in that subdirectory. I still cannot make this works.
– Stu_Dent
Nov 18 '18 at 12:51
Yes, it is exactly what the timer does, but I want each subdirectory should zip itself once (1)time elapsed and (2)no more files being added in that subdirectory. I still cannot make this works.
– Stu_Dent
Nov 18 '18 at 12:51
1
1
Don't you already restart the timer whenever a new file arrives? This should handle (1) and (2).
– Klaus Gütter
Nov 18 '18 at 13:45
Don't you already restart the timer whenever a new file arrives? This should handle (1) and (2).
– Klaus Gütter
Nov 18 '18 at 13:45
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348552%2frepeating-a-method-call-on-each-direcotry-with-timer-elasped-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
yA 5 7CJl4ex0
How do you set up the file system watchers and their timers? I wonder how the event handlers get their extra parameters.
– Klaus Gütter
Nov 17 '18 at 6:58
I set the 2nd watcher and timer inside the OnCreated event handler of the root folder, extended with the code above, which the 2nd watcher sends an event to the timer and also the timer sends an event to the 2nd watcher to interact each other to some extent. I'm not sure how to proceed the zip process to each folder individually.
– Stu_Dent
Nov 17 '18 at 7:37
Could you please add this code to the question?
– Klaus Gütter
Nov 17 '18 at 7:46
@KlausGütter my code might be long and ugly, but you can find it here. My apology.
– Stu_Dent
Nov 18 '18 at 9:30
1
Frankly, I don't get your probem. Is it that your CreateZip method always zips all subdirectories and not only the one where a new file has been added? If you do not want this, just pass the path to the correct directory to the method and remove the foreach loop on the subdirectories from CreateZip.
– Klaus Gütter
Nov 18 '18 at 10:50