How to specify size for bernoulli distribution with pymc3?
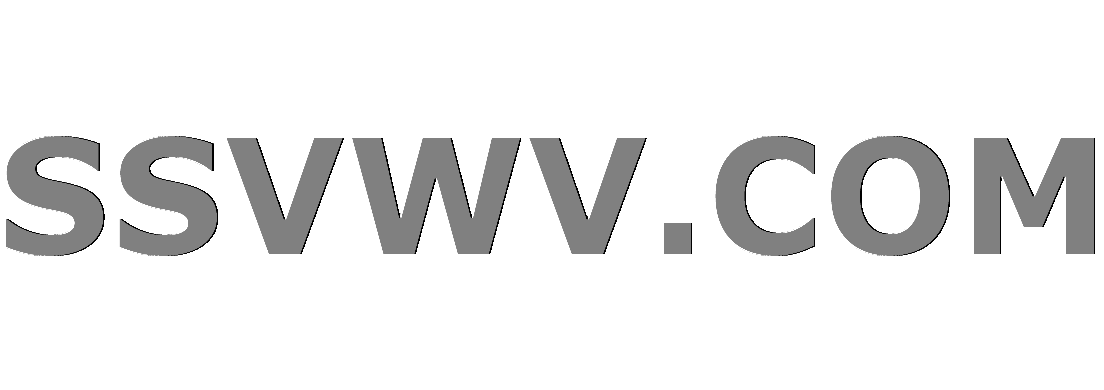
Multi tool use
up vote
3
down vote
favorite
In trying to make my way through Bayesian Methods for Hackers, which is in pymc, I came across this code:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, size=N)
I've tried to translate this to pymc3 with the following, but it just returns a numpy array, rather than a tensor (?):
first_coin_flips = pm.Bernoulli("first_flips", 0.5).random(size=50)
The reason the size matters is that it's used later on in a deterministic variable. Here's the entirety of the code that I have so far:
import pymc3 as pm
import matplotlib.pyplot as plt
import numpy as np
import mpld3
import theano.tensor as tt
model = pm.Model()
with model:
N = 100
p = pm.Uniform("cheating_freq", 0, 1)
true_answers = pm.Bernoulli("truths", p)
print(true_answers)
first_coin_flips = pm.Bernoulli("first_flips", 0.5)
second_coin_flips = pm.Bernoulli("second_flips", 0.5)
# print(first_coin_flips.value)
# Create model variables
def calc_p(true_answers, first_coin_flips, second_coin_flips):
observed = first_coin_flips * true_answers + (1-first_coin_flips) * second_coin_flips
# NOTE: Where I think the size param matters, since we're dividing by it
return observed.sum() / float(N)
calced_p = pm.Deterministic("observed", calc_p(true_answers, first_coin_flips, second_coin_flips))
step = pm.Metropolis(model.free_RVs)
trace = pm.sample(1000, tune=500, step=step)
pm.traceplot(trace)
html = mpld3.fig_to_html(plt.gcf())
with open("output.html", 'w') as f:
f.write(html)
f.close()
And the output:
The coin flips and uniform cheating_freq
output look correct, but the observed
doesn't look like anything to me, and I think it's because I'm not translating that size
param correctly.
python statistics pymc3
add a comment |
up vote
3
down vote
favorite
In trying to make my way through Bayesian Methods for Hackers, which is in pymc, I came across this code:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, size=N)
I've tried to translate this to pymc3 with the following, but it just returns a numpy array, rather than a tensor (?):
first_coin_flips = pm.Bernoulli("first_flips", 0.5).random(size=50)
The reason the size matters is that it's used later on in a deterministic variable. Here's the entirety of the code that I have so far:
import pymc3 as pm
import matplotlib.pyplot as plt
import numpy as np
import mpld3
import theano.tensor as tt
model = pm.Model()
with model:
N = 100
p = pm.Uniform("cheating_freq", 0, 1)
true_answers = pm.Bernoulli("truths", p)
print(true_answers)
first_coin_flips = pm.Bernoulli("first_flips", 0.5)
second_coin_flips = pm.Bernoulli("second_flips", 0.5)
# print(first_coin_flips.value)
# Create model variables
def calc_p(true_answers, first_coin_flips, second_coin_flips):
observed = first_coin_flips * true_answers + (1-first_coin_flips) * second_coin_flips
# NOTE: Where I think the size param matters, since we're dividing by it
return observed.sum() / float(N)
calced_p = pm.Deterministic("observed", calc_p(true_answers, first_coin_flips, second_coin_flips))
step = pm.Metropolis(model.free_RVs)
trace = pm.sample(1000, tune=500, step=step)
pm.traceplot(trace)
html = mpld3.fig_to_html(plt.gcf())
with open("output.html", 'w') as f:
f.write(html)
f.close()
And the output:
The coin flips and uniform cheating_freq
output look correct, but the observed
doesn't look like anything to me, and I think it's because I'm not translating that size
param correctly.
python statistics pymc3
1
Could you include a link to the original code you're trying to replicate? Also, the notebooks are already all converted for PyMC3. E.g., each chapter folder has a notebook for PyMC2, PyMC3, and TF Probability, which is what PyMC4 will use.
– merv
Nov 7 at 18:31
Oh wow, didn't realize that there were already translations for pymc3. That answers my question, I'll create an answer for it. Thanks!
– Marcus Buffett
Nov 7 at 19:23
add a comment |
up vote
3
down vote
favorite
up vote
3
down vote
favorite
In trying to make my way through Bayesian Methods for Hackers, which is in pymc, I came across this code:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, size=N)
I've tried to translate this to pymc3 with the following, but it just returns a numpy array, rather than a tensor (?):
first_coin_flips = pm.Bernoulli("first_flips", 0.5).random(size=50)
The reason the size matters is that it's used later on in a deterministic variable. Here's the entirety of the code that I have so far:
import pymc3 as pm
import matplotlib.pyplot as plt
import numpy as np
import mpld3
import theano.tensor as tt
model = pm.Model()
with model:
N = 100
p = pm.Uniform("cheating_freq", 0, 1)
true_answers = pm.Bernoulli("truths", p)
print(true_answers)
first_coin_flips = pm.Bernoulli("first_flips", 0.5)
second_coin_flips = pm.Bernoulli("second_flips", 0.5)
# print(first_coin_flips.value)
# Create model variables
def calc_p(true_answers, first_coin_flips, second_coin_flips):
observed = first_coin_flips * true_answers + (1-first_coin_flips) * second_coin_flips
# NOTE: Where I think the size param matters, since we're dividing by it
return observed.sum() / float(N)
calced_p = pm.Deterministic("observed", calc_p(true_answers, first_coin_flips, second_coin_flips))
step = pm.Metropolis(model.free_RVs)
trace = pm.sample(1000, tune=500, step=step)
pm.traceplot(trace)
html = mpld3.fig_to_html(plt.gcf())
with open("output.html", 'w') as f:
f.write(html)
f.close()
And the output:
The coin flips and uniform cheating_freq
output look correct, but the observed
doesn't look like anything to me, and I think it's because I'm not translating that size
param correctly.
python statistics pymc3
In trying to make my way through Bayesian Methods for Hackers, which is in pymc, I came across this code:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, size=N)
I've tried to translate this to pymc3 with the following, but it just returns a numpy array, rather than a tensor (?):
first_coin_flips = pm.Bernoulli("first_flips", 0.5).random(size=50)
The reason the size matters is that it's used later on in a deterministic variable. Here's the entirety of the code that I have so far:
import pymc3 as pm
import matplotlib.pyplot as plt
import numpy as np
import mpld3
import theano.tensor as tt
model = pm.Model()
with model:
N = 100
p = pm.Uniform("cheating_freq", 0, 1)
true_answers = pm.Bernoulli("truths", p)
print(true_answers)
first_coin_flips = pm.Bernoulli("first_flips", 0.5)
second_coin_flips = pm.Bernoulli("second_flips", 0.5)
# print(first_coin_flips.value)
# Create model variables
def calc_p(true_answers, first_coin_flips, second_coin_flips):
observed = first_coin_flips * true_answers + (1-first_coin_flips) * second_coin_flips
# NOTE: Where I think the size param matters, since we're dividing by it
return observed.sum() / float(N)
calced_p = pm.Deterministic("observed", calc_p(true_answers, first_coin_flips, second_coin_flips))
step = pm.Metropolis(model.free_RVs)
trace = pm.sample(1000, tune=500, step=step)
pm.traceplot(trace)
html = mpld3.fig_to_html(plt.gcf())
with open("output.html", 'w') as f:
f.write(html)
f.close()
And the output:
The coin flips and uniform cheating_freq
output look correct, but the observed
doesn't look like anything to me, and I think it's because I'm not translating that size
param correctly.
python statistics pymc3
python statistics pymc3
asked Nov 7 at 17:00
Marcus Buffett
592422
592422
1
Could you include a link to the original code you're trying to replicate? Also, the notebooks are already all converted for PyMC3. E.g., each chapter folder has a notebook for PyMC2, PyMC3, and TF Probability, which is what PyMC4 will use.
– merv
Nov 7 at 18:31
Oh wow, didn't realize that there were already translations for pymc3. That answers my question, I'll create an answer for it. Thanks!
– Marcus Buffett
Nov 7 at 19:23
add a comment |
1
Could you include a link to the original code you're trying to replicate? Also, the notebooks are already all converted for PyMC3. E.g., each chapter folder has a notebook for PyMC2, PyMC3, and TF Probability, which is what PyMC4 will use.
– merv
Nov 7 at 18:31
Oh wow, didn't realize that there were already translations for pymc3. That answers my question, I'll create an answer for it. Thanks!
– Marcus Buffett
Nov 7 at 19:23
1
1
Could you include a link to the original code you're trying to replicate? Also, the notebooks are already all converted for PyMC3. E.g., each chapter folder has a notebook for PyMC2, PyMC3, and TF Probability, which is what PyMC4 will use.
– merv
Nov 7 at 18:31
Could you include a link to the original code you're trying to replicate? Also, the notebooks are already all converted for PyMC3. E.g., each chapter folder has a notebook for PyMC2, PyMC3, and TF Probability, which is what PyMC4 will use.
– merv
Nov 7 at 18:31
Oh wow, didn't realize that there were already translations for pymc3. That answers my question, I'll create an answer for it. Thanks!
– Marcus Buffett
Nov 7 at 19:23
Oh wow, didn't realize that there were already translations for pymc3. That answers my question, I'll create an answer for it. Thanks!
– Marcus Buffett
Nov 7 at 19:23
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
accepted
The pymc3 way to specify the size of a Bernoulli distribution is by using the shape
parameter, like:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, shape=N)
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
accepted
The pymc3 way to specify the size of a Bernoulli distribution is by using the shape
parameter, like:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, shape=N)
add a comment |
up vote
1
down vote
accepted
The pymc3 way to specify the size of a Bernoulli distribution is by using the shape
parameter, like:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, shape=N)
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
The pymc3 way to specify the size of a Bernoulli distribution is by using the shape
parameter, like:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, shape=N)
The pymc3 way to specify the size of a Bernoulli distribution is by using the shape
parameter, like:
first_coin_flips = pm.Bernoulli("first_flips", 0.5, shape=N)
answered Nov 7 at 19:25
Marcus Buffett
592422
592422
add a comment |
add a comment |
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53194272%2fhow-to-specify-size-for-bernoulli-distribution-with-pymc3%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
u5,jed4 LJGB0iG1
1
Could you include a link to the original code you're trying to replicate? Also, the notebooks are already all converted for PyMC3. E.g., each chapter folder has a notebook for PyMC2, PyMC3, and TF Probability, which is what PyMC4 will use.
– merv
Nov 7 at 18:31
Oh wow, didn't realize that there were already translations for pymc3. That answers my question, I'll create an answer for it. Thanks!
– Marcus Buffett
Nov 7 at 19:23