jdbcTemplate.batchUpdate not inserting rows
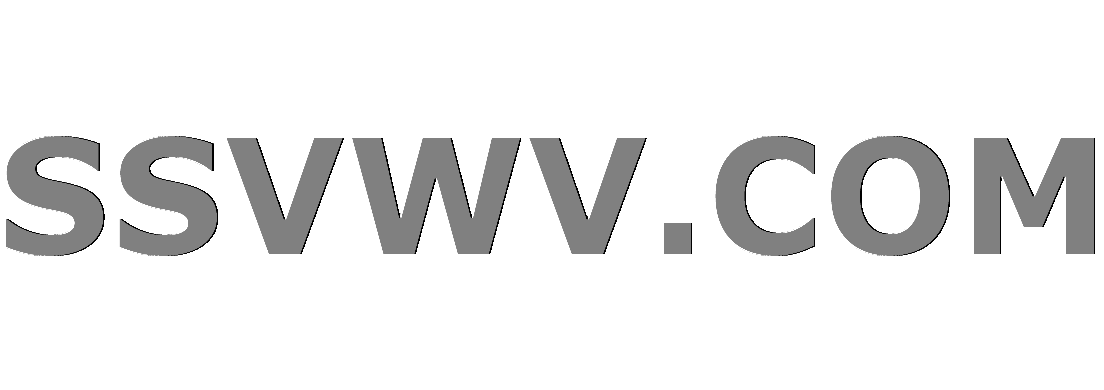
Multi tool use
I'm trying to do a batch insert, but when run in Tomcat the app seems to hang, and no new rows inserted. Below is my code:
public class MatchDAOImpl implements MatchDAO {
private JdbcTemplate jdbcTemplate;
public MatchDAOImpl(DataSource dataSource) {
jdbcTemplate = new JdbcTemplate(dataSource);
}
@Override
public void batchInsert(final List<Match> matches){
String sql = "INSERT INTO matches (id, status, home_team_id, away_team_id, home_goals, away_goals) VALUES (?, ?, ?, ?, ?, ?)";
jdbcTemplate.batchUpdate(sql, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
Match match = matches.get(i);
System.out.println("Inserting values for #" + match.getId());
ps.setInt(1, match.getId());
ps.setString(2, match.getStatus());
ps.setInt(3, match.getHomeTeam().getId());
ps.setInt(4, match.getAwayTeam().getId());
ps.setInt(5, match.getHomeGoals());
ps.setInt(6, match.getAwayGoals());
}
@Override
public int getBatchSize() {
return matches.size();
}
});
}
Here is an example of it being called:
matchesDAO.batchInsert(matches);
Also, you'll see I have a println command so I know that it's at least using this method. Below is a sample output from this:
Inserting values for #233398
Inserting values for #233399
Inserting values for #233400
Inserting values for #233401
Inserting values for #233402
...
However, when I check the database for any new rows there are none (empty table). I'm not getting any exception thrown to indicate what the issue is. Does my method look OK?
UPDATE
MvcConfig.java
@Configuration
@ComponentScan(basePackages="com.example.budget")
@EnableWebMvc
public class MvcConfiguration extends WebMvcConfigurerAdapter{
//...
@Bean
public DataSource getDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
// dataSource.setDriverClassName("oracle.jdbc.driver.OracleDriver");
dataSource.setDriverClassName( OracleDriver.class.getName() );
dataSource.setUrl("jdbc:oracle:thin:@localhost:1521:xe");
dataSource.setUsername("budget_admin");
dataSource.setPassword("budg3t");
return dataSource;
}
@Bean
public MatchDAO getMatchDAO() {
return new MatchDAOImpl(getDataSource());
}
Here is where batchInsert is being called for now:
@Controller
public class MatchesController {
@Autowired
private FootballData footballData;
@Autowired
private MatchDAO matchDAOImpl;
@RequestMapping(value = "/api/matches")
public ModelAndView matches(ModelAndView model) {
List<Match> matches = footballData.requestMatches();
// write to db
matchDAOImpl.batchInsert(matches);
model.addObject("matches", matches);
model.setViewName("matches");
return model;
}
java jdbctemplate
|
show 3 more comments
I'm trying to do a batch insert, but when run in Tomcat the app seems to hang, and no new rows inserted. Below is my code:
public class MatchDAOImpl implements MatchDAO {
private JdbcTemplate jdbcTemplate;
public MatchDAOImpl(DataSource dataSource) {
jdbcTemplate = new JdbcTemplate(dataSource);
}
@Override
public void batchInsert(final List<Match> matches){
String sql = "INSERT INTO matches (id, status, home_team_id, away_team_id, home_goals, away_goals) VALUES (?, ?, ?, ?, ?, ?)";
jdbcTemplate.batchUpdate(sql, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
Match match = matches.get(i);
System.out.println("Inserting values for #" + match.getId());
ps.setInt(1, match.getId());
ps.setString(2, match.getStatus());
ps.setInt(3, match.getHomeTeam().getId());
ps.setInt(4, match.getAwayTeam().getId());
ps.setInt(5, match.getHomeGoals());
ps.setInt(6, match.getAwayGoals());
}
@Override
public int getBatchSize() {
return matches.size();
}
});
}
Here is an example of it being called:
matchesDAO.batchInsert(matches);
Also, you'll see I have a println command so I know that it's at least using this method. Below is a sample output from this:
Inserting values for #233398
Inserting values for #233399
Inserting values for #233400
Inserting values for #233401
Inserting values for #233402
...
However, when I check the database for any new rows there are none (empty table). I'm not getting any exception thrown to indicate what the issue is. Does my method look OK?
UPDATE
MvcConfig.java
@Configuration
@ComponentScan(basePackages="com.example.budget")
@EnableWebMvc
public class MvcConfiguration extends WebMvcConfigurerAdapter{
//...
@Bean
public DataSource getDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
// dataSource.setDriverClassName("oracle.jdbc.driver.OracleDriver");
dataSource.setDriverClassName( OracleDriver.class.getName() );
dataSource.setUrl("jdbc:oracle:thin:@localhost:1521:xe");
dataSource.setUsername("budget_admin");
dataSource.setPassword("budg3t");
return dataSource;
}
@Bean
public MatchDAO getMatchDAO() {
return new MatchDAOImpl(getDataSource());
}
Here is where batchInsert is being called for now:
@Controller
public class MatchesController {
@Autowired
private FootballData footballData;
@Autowired
private MatchDAO matchDAOImpl;
@RequestMapping(value = "/api/matches")
public ModelAndView matches(ModelAndView model) {
List<Match> matches = footballData.requestMatches();
// write to db
matchDAOImpl.batchInsert(matches);
model.addObject("matches", matches);
model.setViewName("matches");
return model;
}
java jdbctemplate
Do you have a transaction wrapping all of this, and being committed at some point?
– JB Nizet
Nov 18 '18 at 17:47
No I don't think so. I've been following along this tutorial - mkyong.com/spring/spring-jdbctemplate-batchupdate-example
– Martyn
Nov 18 '18 at 18:09
Can you share your datasource JDBC URL with us ?
– ygor
Nov 18 '18 at 18:19
1
And also, how do you execute thebatchInsert
method ? From amain
, web controller? Perhaps from aJUnit
test ? We need to know, whether there is perhaps an enclosing transaction.
– ygor
Nov 18 '18 at 18:28
1
Read the Spring documentation. You need a DataSourceTransactionManager, and your DAO method at least, or your controller method calling it, must be transactional.
– JB Nizet
Nov 18 '18 at 19:39
|
show 3 more comments
I'm trying to do a batch insert, but when run in Tomcat the app seems to hang, and no new rows inserted. Below is my code:
public class MatchDAOImpl implements MatchDAO {
private JdbcTemplate jdbcTemplate;
public MatchDAOImpl(DataSource dataSource) {
jdbcTemplate = new JdbcTemplate(dataSource);
}
@Override
public void batchInsert(final List<Match> matches){
String sql = "INSERT INTO matches (id, status, home_team_id, away_team_id, home_goals, away_goals) VALUES (?, ?, ?, ?, ?, ?)";
jdbcTemplate.batchUpdate(sql, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
Match match = matches.get(i);
System.out.println("Inserting values for #" + match.getId());
ps.setInt(1, match.getId());
ps.setString(2, match.getStatus());
ps.setInt(3, match.getHomeTeam().getId());
ps.setInt(4, match.getAwayTeam().getId());
ps.setInt(5, match.getHomeGoals());
ps.setInt(6, match.getAwayGoals());
}
@Override
public int getBatchSize() {
return matches.size();
}
});
}
Here is an example of it being called:
matchesDAO.batchInsert(matches);
Also, you'll see I have a println command so I know that it's at least using this method. Below is a sample output from this:
Inserting values for #233398
Inserting values for #233399
Inserting values for #233400
Inserting values for #233401
Inserting values for #233402
...
However, when I check the database for any new rows there are none (empty table). I'm not getting any exception thrown to indicate what the issue is. Does my method look OK?
UPDATE
MvcConfig.java
@Configuration
@ComponentScan(basePackages="com.example.budget")
@EnableWebMvc
public class MvcConfiguration extends WebMvcConfigurerAdapter{
//...
@Bean
public DataSource getDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
// dataSource.setDriverClassName("oracle.jdbc.driver.OracleDriver");
dataSource.setDriverClassName( OracleDriver.class.getName() );
dataSource.setUrl("jdbc:oracle:thin:@localhost:1521:xe");
dataSource.setUsername("budget_admin");
dataSource.setPassword("budg3t");
return dataSource;
}
@Bean
public MatchDAO getMatchDAO() {
return new MatchDAOImpl(getDataSource());
}
Here is where batchInsert is being called for now:
@Controller
public class MatchesController {
@Autowired
private FootballData footballData;
@Autowired
private MatchDAO matchDAOImpl;
@RequestMapping(value = "/api/matches")
public ModelAndView matches(ModelAndView model) {
List<Match> matches = footballData.requestMatches();
// write to db
matchDAOImpl.batchInsert(matches);
model.addObject("matches", matches);
model.setViewName("matches");
return model;
}
java jdbctemplate
I'm trying to do a batch insert, but when run in Tomcat the app seems to hang, and no new rows inserted. Below is my code:
public class MatchDAOImpl implements MatchDAO {
private JdbcTemplate jdbcTemplate;
public MatchDAOImpl(DataSource dataSource) {
jdbcTemplate = new JdbcTemplate(dataSource);
}
@Override
public void batchInsert(final List<Match> matches){
String sql = "INSERT INTO matches (id, status, home_team_id, away_team_id, home_goals, away_goals) VALUES (?, ?, ?, ?, ?, ?)";
jdbcTemplate.batchUpdate(sql, new BatchPreparedStatementSetter() {
@Override
public void setValues(PreparedStatement ps, int i) throws SQLException {
Match match = matches.get(i);
System.out.println("Inserting values for #" + match.getId());
ps.setInt(1, match.getId());
ps.setString(2, match.getStatus());
ps.setInt(3, match.getHomeTeam().getId());
ps.setInt(4, match.getAwayTeam().getId());
ps.setInt(5, match.getHomeGoals());
ps.setInt(6, match.getAwayGoals());
}
@Override
public int getBatchSize() {
return matches.size();
}
});
}
Here is an example of it being called:
matchesDAO.batchInsert(matches);
Also, you'll see I have a println command so I know that it's at least using this method. Below is a sample output from this:
Inserting values for #233398
Inserting values for #233399
Inserting values for #233400
Inserting values for #233401
Inserting values for #233402
...
However, when I check the database for any new rows there are none (empty table). I'm not getting any exception thrown to indicate what the issue is. Does my method look OK?
UPDATE
MvcConfig.java
@Configuration
@ComponentScan(basePackages="com.example.budget")
@EnableWebMvc
public class MvcConfiguration extends WebMvcConfigurerAdapter{
//...
@Bean
public DataSource getDataSource() {
DriverManagerDataSource dataSource = new DriverManagerDataSource();
// dataSource.setDriverClassName("oracle.jdbc.driver.OracleDriver");
dataSource.setDriverClassName( OracleDriver.class.getName() );
dataSource.setUrl("jdbc:oracle:thin:@localhost:1521:xe");
dataSource.setUsername("budget_admin");
dataSource.setPassword("budg3t");
return dataSource;
}
@Bean
public MatchDAO getMatchDAO() {
return new MatchDAOImpl(getDataSource());
}
Here is where batchInsert is being called for now:
@Controller
public class MatchesController {
@Autowired
private FootballData footballData;
@Autowired
private MatchDAO matchDAOImpl;
@RequestMapping(value = "/api/matches")
public ModelAndView matches(ModelAndView model) {
List<Match> matches = footballData.requestMatches();
// write to db
matchDAOImpl.batchInsert(matches);
model.addObject("matches", matches);
model.setViewName("matches");
return model;
}
java jdbctemplate
java jdbctemplate
edited Nov 18 '18 at 19:18
Martyn
asked Nov 18 '18 at 17:37
MartynMartyn
1,58571863
1,58571863
Do you have a transaction wrapping all of this, and being committed at some point?
– JB Nizet
Nov 18 '18 at 17:47
No I don't think so. I've been following along this tutorial - mkyong.com/spring/spring-jdbctemplate-batchupdate-example
– Martyn
Nov 18 '18 at 18:09
Can you share your datasource JDBC URL with us ?
– ygor
Nov 18 '18 at 18:19
1
And also, how do you execute thebatchInsert
method ? From amain
, web controller? Perhaps from aJUnit
test ? We need to know, whether there is perhaps an enclosing transaction.
– ygor
Nov 18 '18 at 18:28
1
Read the Spring documentation. You need a DataSourceTransactionManager, and your DAO method at least, or your controller method calling it, must be transactional.
– JB Nizet
Nov 18 '18 at 19:39
|
show 3 more comments
Do you have a transaction wrapping all of this, and being committed at some point?
– JB Nizet
Nov 18 '18 at 17:47
No I don't think so. I've been following along this tutorial - mkyong.com/spring/spring-jdbctemplate-batchupdate-example
– Martyn
Nov 18 '18 at 18:09
Can you share your datasource JDBC URL with us ?
– ygor
Nov 18 '18 at 18:19
1
And also, how do you execute thebatchInsert
method ? From amain
, web controller? Perhaps from aJUnit
test ? We need to know, whether there is perhaps an enclosing transaction.
– ygor
Nov 18 '18 at 18:28
1
Read the Spring documentation. You need a DataSourceTransactionManager, and your DAO method at least, or your controller method calling it, must be transactional.
– JB Nizet
Nov 18 '18 at 19:39
Do you have a transaction wrapping all of this, and being committed at some point?
– JB Nizet
Nov 18 '18 at 17:47
Do you have a transaction wrapping all of this, and being committed at some point?
– JB Nizet
Nov 18 '18 at 17:47
No I don't think so. I've been following along this tutorial - mkyong.com/spring/spring-jdbctemplate-batchupdate-example
– Martyn
Nov 18 '18 at 18:09
No I don't think so. I've been following along this tutorial - mkyong.com/spring/spring-jdbctemplate-batchupdate-example
– Martyn
Nov 18 '18 at 18:09
Can you share your datasource JDBC URL with us ?
– ygor
Nov 18 '18 at 18:19
Can you share your datasource JDBC URL with us ?
– ygor
Nov 18 '18 at 18:19
1
1
And also, how do you execute the
batchInsert
method ? From a main
, web controller? Perhaps from a JUnit
test ? We need to know, whether there is perhaps an enclosing transaction.– ygor
Nov 18 '18 at 18:28
And also, how do you execute the
batchInsert
method ? From a main
, web controller? Perhaps from a JUnit
test ? We need to know, whether there is perhaps an enclosing transaction.– ygor
Nov 18 '18 at 18:28
1
1
Read the Spring documentation. You need a DataSourceTransactionManager, and your DAO method at least, or your controller method calling it, must be transactional.
– JB Nizet
Nov 18 '18 at 19:39
Read the Spring documentation. You need a DataSourceTransactionManager, and your DAO method at least, or your controller method calling it, must be transactional.
– JB Nizet
Nov 18 '18 at 19:39
|
show 3 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53363712%2fjdbctemplate-batchupdate-not-inserting-rows%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53363712%2fjdbctemplate-batchupdate-not-inserting-rows%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zVXf 9NG4hfJbUP,cROLaE,AZX y,T2ab xpqZ TCmL,d69ZimO,Lyiwq PcFqcU,fa4vf3QjGK,9Fs d8r,r0GPX V7Xclva5RVIWcJNO
Do you have a transaction wrapping all of this, and being committed at some point?
– JB Nizet
Nov 18 '18 at 17:47
No I don't think so. I've been following along this tutorial - mkyong.com/spring/spring-jdbctemplate-batchupdate-example
– Martyn
Nov 18 '18 at 18:09
Can you share your datasource JDBC URL with us ?
– ygor
Nov 18 '18 at 18:19
1
And also, how do you execute the
batchInsert
method ? From amain
, web controller? Perhaps from aJUnit
test ? We need to know, whether there is perhaps an enclosing transaction.– ygor
Nov 18 '18 at 18:28
1
Read the Spring documentation. You need a DataSourceTransactionManager, and your DAO method at least, or your controller method calling it, must be transactional.
– JB Nizet
Nov 18 '18 at 19:39