pass data between Fragmensts
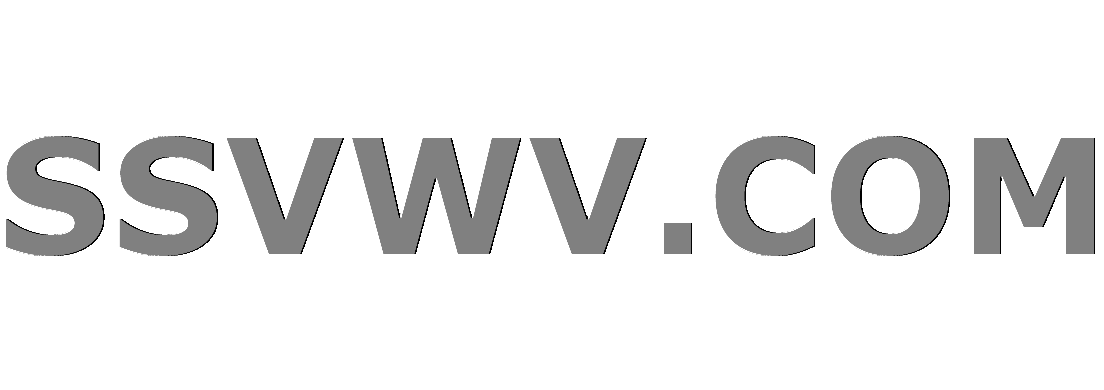
Multi tool use
how to pass data from listView in Fragment with customAdapter to map of googleMaps in second Fragment to addMarker() Function?
.................................................................................................
this is my classes are important:
FragmentOne(the listView):
public class FragmentOne extends Fragment {
private static ArrayList<MapModel> mMapList;
private static MapCustomAdapterSearch mAdapter;
private static ListView mListView;
private MapDBHelper mMapDBHelper;
private GetMapsAsyncTaskInternet mGetMapsAsyncTaskInternet;
private static ProgressDialog mProgressDialogInternet;
private static FragmentOne mFragmentOne;
private View mView;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_one_layout, container, false);
mListView = mView.findViewById(R.id.list);
mFragmentOne = this;
mMapDBHelper = new MapDBHelper(getActivity());
mMapList = mMapDBHelper.getAllMaps();
mAdapter = new MapCustomAdapterSearch(getActivity(), mMapList);
setHasOptionsMenu(true);
registerForContextMenu(mListView);
mListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intentSearchToAddInternet = new Intent(getActivity().getBaseContext(), FragmentTwo.class);
intentSearchToAddInternet.putExtra(getString(R.string.movie_edit), mMapList.get(position));
getActivity().startActivity(intentSearchToAddInternet);
}
});
return mView;
}
// Set movies in FragmentOne
public static void setMaps(ArrayList<MapModel> list) {
mMapList = list;
mAdapter.clear();
mAdapter.addAll(list);
mListView.setAdapter(mAdapter);
}
// Sets off the menu of activity_menu
@Override
public void onCreateOptionsMenu(Menu menu, MenuInflater inflater) {
inflater.inflate(R.menu.activity_menu, menu);
// SearchView of FragmentOne
final MenuItem menuItem = menu.findItem(R.id.action_search);
SearchManager searchManager = (SearchManager) getActivity().getSystemService(Context.SEARCH_SERVICE);
// Change colors of the searchView upper panel
menuItem.setOnActionExpandListener(new MenuItem.OnActionExpandListener() {
@Override
public boolean onMenuItemActionExpand(MenuItem item) {
// Set styles for expanded state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.DKGRAY));
}
return true;
}
@Override
public boolean onMenuItemActionCollapse(MenuItem item) {
// Set styles for collapsed state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
}
return true;
}
});
// Continued of SearchView of FragmentOne
final android.support.v7.widget.SearchView searchView = (android.support.v7.widget.SearchView) menuItem.getActionView();
if (searchView != null) {
searchView.setSearchableInfo(searchManager.getSearchableInfo((getActivity()).getComponentName()));
searchView.setQueryHint(Html.fromHtml("<font color = #FFEA54>" + getResources().getString(R.string.hint) + "</font>"));
searchView.setSubmitButtonEnabled(true);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
// Search movies from that URL and put them in the SQLiteHelper
String urlQuery = "https://maps.googleapis.com/maps/api/place/search/json?location=31.73072,34.99293&radius=1000000&rankby=prominence&types=food|restaurant&keyword="
+ query +
"&key=API_KEY";
mGetMapsAsyncTaskInternet = new GetMapsAsyncTaskInternet();
mGetMapsAsyncTaskInternet.execute(urlQuery);
return true;
}
@Override
public boolean onQueryTextChange(String newText) {
return true;
}
});
}
}
// Sets off the menu of list_menu
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenu.ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
getActivity().getMenuInflater().inflate(R.menu.list_menu, menu);
}
// Options in the activity_menu
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_search:
break;
}
return super.onOptionsItemSelected(item);
}
// Options in the list_menu
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterView.AdapterContextMenuInfo info = (AdapterView.AdapterContextMenuInfo) item.getMenuInfo();
int listPosition = info.position;
switch (item.getItemId()) {
case R.id.addMap: // Edit the movies on MainActivity
Intent intent = new Intent(getActivity(), AddMapMe.class);
intent.putExtra(getString(R.string.map_add_from_internet), mMapList.get(listPosition));
startActivity(intent);
break;
}
return super.onContextItemSelected(item);
}
// stopShowingProgressBar
public static void stopShowingProgressBar() {
if (mProgressDialogInternet != null) {
mProgressDialogInternet.dismiss();
mProgressDialogInternet = null;
}
}
// startShowingProgressBar
public static void startShowingProgressBar() {
mProgressDialogInternet = ProgressDialog.show(mFragmentOne.getActivity(), "Loading...",
"Please wait...", true);
mProgressDialogInternet.show();
}
FragmentTwo(of Map):
public class FragmentTwo extends Fragment implements OnMapReadyCallback {
private GoogleMap mGoogleMap;
private MapView mMapView;
private View mView;
private MapModel mapModel;
TextView textView;
TextView textView1;
final MapModel item = (MapModel) getActivity().getIntent().getExtras().getSerializable(getString(R.string.movie_edit));
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_two_layout, container, false);
return mView;
}
@Override
public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
mMapView = view.findViewById(R.id.map);
if (mMapView != null) {
mMapView.onCreate(null);
mMapView.onResume();
mMapView.getMapAsync(this);
}
}
@Override
public void onMapReady(GoogleMap googleMap) {
MapsInitializer.initialize(getContext());
mGoogleMap = googleMap;
addMarker();
googleMap.setMapType(GoogleMap.MAP_TYPE_TERRAIN);
if (ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
googleMap.setMyLocationEnabled(true);
}
public void addMarker() {
textView.setText(String.valueOf(item.getLat()));
textView1.setText(String.valueOf(item.getLng()));
String lat = textView.getText().toString();
String lng = textView1.getText().toString();
double lat1 = Double.parseDouble(lat);
double lng1 = Double.parseDouble(lng);
MarkerOptions marker = new MarkerOptions().position(new LatLng(lat1, lng1)).title("Elior home");
mGoogleMap.addMarker(marker);
mGoogleMap.setOnMarkerClickListener(new GoogleMap.OnMarkerClickListener() {
@Override
public boolean onMarkerClick(Marker marker) {
return true;
}
});
}
MapModel:
public class MapModel implements Serializable {
private float lat;
private float lng;
private String vicinity;
private String name;
private String id;
private String icon;
private Geometry geometry;
public MapModel(String name, String vicinity, float lat, float lng, String icon) {
this.vicinity = vicinity;
this.name = name;
this.lat = lat;
this.lng = lng;
this.icon = icon;
}
public float getLng() {
return geometry.getLocation().getLng();
}
public void setLng(float lng) {
this.geometry.getLocation().setLng(lng);
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public float getLat() {
return geometry.getLocation().getLat();
}
public void setLat(float lat) {
this.geometry.getLocation().setLat(lat);
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getVicinity() {
return vicinity;
}
public void setVicinity(String formatted_address) {
this.vicinity = formatted_address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
MapCustomAdapterSearch:
public class MapCustomAdapterSearch extends ArrayAdapter<MapModel> {
private Context mContext; //Context
private ArrayList<MapModel> mMovieList; // ArrayList of MovieModel
private FusedLocationProviderClient mFusedLocationClient;
private MapModel mapModel;
MapCustomAdapterSearch(Context context_, ArrayList<MapModel> movie_) {
super(context_, 0, movie_);
mContext = context_;
mMovieList = movie_;
}
@NonNull
@Override
public View getView(int position, @NonNull View convertView, @NonNull ViewGroup parent) {
View listItem = convertView;
if (listItem == null) {
listItem = LayoutInflater.from(mContext).inflate(R.layout.movie_item_row, parent, false);
}
MapModel currentMap = getItem(position); // Position of items
// Put the image in image1
if (currentMap != null) { // If the position of the items not null
ImageView image1 = listItem.findViewById(R.id.image1);
assert currentMap != null; // If the position of the item not null in the ImageView
Picasso.get().load(currentMap.getIcon()).into(image1);
// Put the text in name1
TextView name1 = listItem.findViewById(R.id.name1);
name1.setText(String.valueOf(currentMap.getName()));
// Put the text in address1
TextView address1 = listItem.findViewById(R.id.address1);
address1.setText(String.valueOf(currentMap.getVicinity()));
// Put the text in lat1
TextView lat1 = listItem.findViewById(R.id.lat1);
lat1.setText("n" + "n" + "Latitude: " + currentMap.getLat());
// Put the text in la2
TextView lng1 = listItem.findViewById(R.id.lng1);
lng1.setText("n" + "Longitude: " + currentMap.getLng());
}
return listItem;
}
private void getLocation() {
if (ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
mFusedLocationClient.getLastLocation().addOnSuccessListener((Executor) this, new OnSuccessListener<android.location.Location>() {
@Override
public void onSuccess(final android.location.Location location) {
// Got last known location. In some rare situations this can be null.
if (location != null) {
final android.location.Location locationA = new android.location.Location("point A");
final double latA = mapModel.getLat();
final double lngA = mapModel.getLng();
locationA.setLatitude(latA);
locationA.setLongitude(lngA);
final android.location.Location locationB = new Location("point B");
final double latB = location.getLatitude();
final double lngB = location.getLongitude();
locationB.setLatitude(latB);
locationB.setLongitude(lngB);
float distance = locationA.distanceTo(locationB);
}
}
});
}
java google-maps

add a comment |
how to pass data from listView in Fragment with customAdapter to map of googleMaps in second Fragment to addMarker() Function?
.................................................................................................
this is my classes are important:
FragmentOne(the listView):
public class FragmentOne extends Fragment {
private static ArrayList<MapModel> mMapList;
private static MapCustomAdapterSearch mAdapter;
private static ListView mListView;
private MapDBHelper mMapDBHelper;
private GetMapsAsyncTaskInternet mGetMapsAsyncTaskInternet;
private static ProgressDialog mProgressDialogInternet;
private static FragmentOne mFragmentOne;
private View mView;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_one_layout, container, false);
mListView = mView.findViewById(R.id.list);
mFragmentOne = this;
mMapDBHelper = new MapDBHelper(getActivity());
mMapList = mMapDBHelper.getAllMaps();
mAdapter = new MapCustomAdapterSearch(getActivity(), mMapList);
setHasOptionsMenu(true);
registerForContextMenu(mListView);
mListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intentSearchToAddInternet = new Intent(getActivity().getBaseContext(), FragmentTwo.class);
intentSearchToAddInternet.putExtra(getString(R.string.movie_edit), mMapList.get(position));
getActivity().startActivity(intentSearchToAddInternet);
}
});
return mView;
}
// Set movies in FragmentOne
public static void setMaps(ArrayList<MapModel> list) {
mMapList = list;
mAdapter.clear();
mAdapter.addAll(list);
mListView.setAdapter(mAdapter);
}
// Sets off the menu of activity_menu
@Override
public void onCreateOptionsMenu(Menu menu, MenuInflater inflater) {
inflater.inflate(R.menu.activity_menu, menu);
// SearchView of FragmentOne
final MenuItem menuItem = menu.findItem(R.id.action_search);
SearchManager searchManager = (SearchManager) getActivity().getSystemService(Context.SEARCH_SERVICE);
// Change colors of the searchView upper panel
menuItem.setOnActionExpandListener(new MenuItem.OnActionExpandListener() {
@Override
public boolean onMenuItemActionExpand(MenuItem item) {
// Set styles for expanded state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.DKGRAY));
}
return true;
}
@Override
public boolean onMenuItemActionCollapse(MenuItem item) {
// Set styles for collapsed state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
}
return true;
}
});
// Continued of SearchView of FragmentOne
final android.support.v7.widget.SearchView searchView = (android.support.v7.widget.SearchView) menuItem.getActionView();
if (searchView != null) {
searchView.setSearchableInfo(searchManager.getSearchableInfo((getActivity()).getComponentName()));
searchView.setQueryHint(Html.fromHtml("<font color = #FFEA54>" + getResources().getString(R.string.hint) + "</font>"));
searchView.setSubmitButtonEnabled(true);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
// Search movies from that URL and put them in the SQLiteHelper
String urlQuery = "https://maps.googleapis.com/maps/api/place/search/json?location=31.73072,34.99293&radius=1000000&rankby=prominence&types=food|restaurant&keyword="
+ query +
"&key=API_KEY";
mGetMapsAsyncTaskInternet = new GetMapsAsyncTaskInternet();
mGetMapsAsyncTaskInternet.execute(urlQuery);
return true;
}
@Override
public boolean onQueryTextChange(String newText) {
return true;
}
});
}
}
// Sets off the menu of list_menu
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenu.ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
getActivity().getMenuInflater().inflate(R.menu.list_menu, menu);
}
// Options in the activity_menu
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_search:
break;
}
return super.onOptionsItemSelected(item);
}
// Options in the list_menu
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterView.AdapterContextMenuInfo info = (AdapterView.AdapterContextMenuInfo) item.getMenuInfo();
int listPosition = info.position;
switch (item.getItemId()) {
case R.id.addMap: // Edit the movies on MainActivity
Intent intent = new Intent(getActivity(), AddMapMe.class);
intent.putExtra(getString(R.string.map_add_from_internet), mMapList.get(listPosition));
startActivity(intent);
break;
}
return super.onContextItemSelected(item);
}
// stopShowingProgressBar
public static void stopShowingProgressBar() {
if (mProgressDialogInternet != null) {
mProgressDialogInternet.dismiss();
mProgressDialogInternet = null;
}
}
// startShowingProgressBar
public static void startShowingProgressBar() {
mProgressDialogInternet = ProgressDialog.show(mFragmentOne.getActivity(), "Loading...",
"Please wait...", true);
mProgressDialogInternet.show();
}
FragmentTwo(of Map):
public class FragmentTwo extends Fragment implements OnMapReadyCallback {
private GoogleMap mGoogleMap;
private MapView mMapView;
private View mView;
private MapModel mapModel;
TextView textView;
TextView textView1;
final MapModel item = (MapModel) getActivity().getIntent().getExtras().getSerializable(getString(R.string.movie_edit));
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_two_layout, container, false);
return mView;
}
@Override
public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
mMapView = view.findViewById(R.id.map);
if (mMapView != null) {
mMapView.onCreate(null);
mMapView.onResume();
mMapView.getMapAsync(this);
}
}
@Override
public void onMapReady(GoogleMap googleMap) {
MapsInitializer.initialize(getContext());
mGoogleMap = googleMap;
addMarker();
googleMap.setMapType(GoogleMap.MAP_TYPE_TERRAIN);
if (ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
googleMap.setMyLocationEnabled(true);
}
public void addMarker() {
textView.setText(String.valueOf(item.getLat()));
textView1.setText(String.valueOf(item.getLng()));
String lat = textView.getText().toString();
String lng = textView1.getText().toString();
double lat1 = Double.parseDouble(lat);
double lng1 = Double.parseDouble(lng);
MarkerOptions marker = new MarkerOptions().position(new LatLng(lat1, lng1)).title("Elior home");
mGoogleMap.addMarker(marker);
mGoogleMap.setOnMarkerClickListener(new GoogleMap.OnMarkerClickListener() {
@Override
public boolean onMarkerClick(Marker marker) {
return true;
}
});
}
MapModel:
public class MapModel implements Serializable {
private float lat;
private float lng;
private String vicinity;
private String name;
private String id;
private String icon;
private Geometry geometry;
public MapModel(String name, String vicinity, float lat, float lng, String icon) {
this.vicinity = vicinity;
this.name = name;
this.lat = lat;
this.lng = lng;
this.icon = icon;
}
public float getLng() {
return geometry.getLocation().getLng();
}
public void setLng(float lng) {
this.geometry.getLocation().setLng(lng);
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public float getLat() {
return geometry.getLocation().getLat();
}
public void setLat(float lat) {
this.geometry.getLocation().setLat(lat);
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getVicinity() {
return vicinity;
}
public void setVicinity(String formatted_address) {
this.vicinity = formatted_address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
MapCustomAdapterSearch:
public class MapCustomAdapterSearch extends ArrayAdapter<MapModel> {
private Context mContext; //Context
private ArrayList<MapModel> mMovieList; // ArrayList of MovieModel
private FusedLocationProviderClient mFusedLocationClient;
private MapModel mapModel;
MapCustomAdapterSearch(Context context_, ArrayList<MapModel> movie_) {
super(context_, 0, movie_);
mContext = context_;
mMovieList = movie_;
}
@NonNull
@Override
public View getView(int position, @NonNull View convertView, @NonNull ViewGroup parent) {
View listItem = convertView;
if (listItem == null) {
listItem = LayoutInflater.from(mContext).inflate(R.layout.movie_item_row, parent, false);
}
MapModel currentMap = getItem(position); // Position of items
// Put the image in image1
if (currentMap != null) { // If the position of the items not null
ImageView image1 = listItem.findViewById(R.id.image1);
assert currentMap != null; // If the position of the item not null in the ImageView
Picasso.get().load(currentMap.getIcon()).into(image1);
// Put the text in name1
TextView name1 = listItem.findViewById(R.id.name1);
name1.setText(String.valueOf(currentMap.getName()));
// Put the text in address1
TextView address1 = listItem.findViewById(R.id.address1);
address1.setText(String.valueOf(currentMap.getVicinity()));
// Put the text in lat1
TextView lat1 = listItem.findViewById(R.id.lat1);
lat1.setText("n" + "n" + "Latitude: " + currentMap.getLat());
// Put the text in la2
TextView lng1 = listItem.findViewById(R.id.lng1);
lng1.setText("n" + "Longitude: " + currentMap.getLng());
}
return listItem;
}
private void getLocation() {
if (ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
mFusedLocationClient.getLastLocation().addOnSuccessListener((Executor) this, new OnSuccessListener<android.location.Location>() {
@Override
public void onSuccess(final android.location.Location location) {
// Got last known location. In some rare situations this can be null.
if (location != null) {
final android.location.Location locationA = new android.location.Location("point A");
final double latA = mapModel.getLat();
final double lngA = mapModel.getLng();
locationA.setLatitude(latA);
locationA.setLongitude(lngA);
final android.location.Location locationB = new Location("point B");
final double latB = location.getLatitude();
final double lngB = location.getLongitude();
locationB.setLatitude(latB);
locationB.setLongitude(lngB);
float distance = locationA.distanceTo(locationB);
}
}
});
}
java google-maps

add a comment |
how to pass data from listView in Fragment with customAdapter to map of googleMaps in second Fragment to addMarker() Function?
.................................................................................................
this is my classes are important:
FragmentOne(the listView):
public class FragmentOne extends Fragment {
private static ArrayList<MapModel> mMapList;
private static MapCustomAdapterSearch mAdapter;
private static ListView mListView;
private MapDBHelper mMapDBHelper;
private GetMapsAsyncTaskInternet mGetMapsAsyncTaskInternet;
private static ProgressDialog mProgressDialogInternet;
private static FragmentOne mFragmentOne;
private View mView;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_one_layout, container, false);
mListView = mView.findViewById(R.id.list);
mFragmentOne = this;
mMapDBHelper = new MapDBHelper(getActivity());
mMapList = mMapDBHelper.getAllMaps();
mAdapter = new MapCustomAdapterSearch(getActivity(), mMapList);
setHasOptionsMenu(true);
registerForContextMenu(mListView);
mListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intentSearchToAddInternet = new Intent(getActivity().getBaseContext(), FragmentTwo.class);
intentSearchToAddInternet.putExtra(getString(R.string.movie_edit), mMapList.get(position));
getActivity().startActivity(intentSearchToAddInternet);
}
});
return mView;
}
// Set movies in FragmentOne
public static void setMaps(ArrayList<MapModel> list) {
mMapList = list;
mAdapter.clear();
mAdapter.addAll(list);
mListView.setAdapter(mAdapter);
}
// Sets off the menu of activity_menu
@Override
public void onCreateOptionsMenu(Menu menu, MenuInflater inflater) {
inflater.inflate(R.menu.activity_menu, menu);
// SearchView of FragmentOne
final MenuItem menuItem = menu.findItem(R.id.action_search);
SearchManager searchManager = (SearchManager) getActivity().getSystemService(Context.SEARCH_SERVICE);
// Change colors of the searchView upper panel
menuItem.setOnActionExpandListener(new MenuItem.OnActionExpandListener() {
@Override
public boolean onMenuItemActionExpand(MenuItem item) {
// Set styles for expanded state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.DKGRAY));
}
return true;
}
@Override
public boolean onMenuItemActionCollapse(MenuItem item) {
// Set styles for collapsed state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
}
return true;
}
});
// Continued of SearchView of FragmentOne
final android.support.v7.widget.SearchView searchView = (android.support.v7.widget.SearchView) menuItem.getActionView();
if (searchView != null) {
searchView.setSearchableInfo(searchManager.getSearchableInfo((getActivity()).getComponentName()));
searchView.setQueryHint(Html.fromHtml("<font color = #FFEA54>" + getResources().getString(R.string.hint) + "</font>"));
searchView.setSubmitButtonEnabled(true);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
// Search movies from that URL and put them in the SQLiteHelper
String urlQuery = "https://maps.googleapis.com/maps/api/place/search/json?location=31.73072,34.99293&radius=1000000&rankby=prominence&types=food|restaurant&keyword="
+ query +
"&key=API_KEY";
mGetMapsAsyncTaskInternet = new GetMapsAsyncTaskInternet();
mGetMapsAsyncTaskInternet.execute(urlQuery);
return true;
}
@Override
public boolean onQueryTextChange(String newText) {
return true;
}
});
}
}
// Sets off the menu of list_menu
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenu.ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
getActivity().getMenuInflater().inflate(R.menu.list_menu, menu);
}
// Options in the activity_menu
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_search:
break;
}
return super.onOptionsItemSelected(item);
}
// Options in the list_menu
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterView.AdapterContextMenuInfo info = (AdapterView.AdapterContextMenuInfo) item.getMenuInfo();
int listPosition = info.position;
switch (item.getItemId()) {
case R.id.addMap: // Edit the movies on MainActivity
Intent intent = new Intent(getActivity(), AddMapMe.class);
intent.putExtra(getString(R.string.map_add_from_internet), mMapList.get(listPosition));
startActivity(intent);
break;
}
return super.onContextItemSelected(item);
}
// stopShowingProgressBar
public static void stopShowingProgressBar() {
if (mProgressDialogInternet != null) {
mProgressDialogInternet.dismiss();
mProgressDialogInternet = null;
}
}
// startShowingProgressBar
public static void startShowingProgressBar() {
mProgressDialogInternet = ProgressDialog.show(mFragmentOne.getActivity(), "Loading...",
"Please wait...", true);
mProgressDialogInternet.show();
}
FragmentTwo(of Map):
public class FragmentTwo extends Fragment implements OnMapReadyCallback {
private GoogleMap mGoogleMap;
private MapView mMapView;
private View mView;
private MapModel mapModel;
TextView textView;
TextView textView1;
final MapModel item = (MapModel) getActivity().getIntent().getExtras().getSerializable(getString(R.string.movie_edit));
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_two_layout, container, false);
return mView;
}
@Override
public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
mMapView = view.findViewById(R.id.map);
if (mMapView != null) {
mMapView.onCreate(null);
mMapView.onResume();
mMapView.getMapAsync(this);
}
}
@Override
public void onMapReady(GoogleMap googleMap) {
MapsInitializer.initialize(getContext());
mGoogleMap = googleMap;
addMarker();
googleMap.setMapType(GoogleMap.MAP_TYPE_TERRAIN);
if (ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
googleMap.setMyLocationEnabled(true);
}
public void addMarker() {
textView.setText(String.valueOf(item.getLat()));
textView1.setText(String.valueOf(item.getLng()));
String lat = textView.getText().toString();
String lng = textView1.getText().toString();
double lat1 = Double.parseDouble(lat);
double lng1 = Double.parseDouble(lng);
MarkerOptions marker = new MarkerOptions().position(new LatLng(lat1, lng1)).title("Elior home");
mGoogleMap.addMarker(marker);
mGoogleMap.setOnMarkerClickListener(new GoogleMap.OnMarkerClickListener() {
@Override
public boolean onMarkerClick(Marker marker) {
return true;
}
});
}
MapModel:
public class MapModel implements Serializable {
private float lat;
private float lng;
private String vicinity;
private String name;
private String id;
private String icon;
private Geometry geometry;
public MapModel(String name, String vicinity, float lat, float lng, String icon) {
this.vicinity = vicinity;
this.name = name;
this.lat = lat;
this.lng = lng;
this.icon = icon;
}
public float getLng() {
return geometry.getLocation().getLng();
}
public void setLng(float lng) {
this.geometry.getLocation().setLng(lng);
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public float getLat() {
return geometry.getLocation().getLat();
}
public void setLat(float lat) {
this.geometry.getLocation().setLat(lat);
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getVicinity() {
return vicinity;
}
public void setVicinity(String formatted_address) {
this.vicinity = formatted_address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
MapCustomAdapterSearch:
public class MapCustomAdapterSearch extends ArrayAdapter<MapModel> {
private Context mContext; //Context
private ArrayList<MapModel> mMovieList; // ArrayList of MovieModel
private FusedLocationProviderClient mFusedLocationClient;
private MapModel mapModel;
MapCustomAdapterSearch(Context context_, ArrayList<MapModel> movie_) {
super(context_, 0, movie_);
mContext = context_;
mMovieList = movie_;
}
@NonNull
@Override
public View getView(int position, @NonNull View convertView, @NonNull ViewGroup parent) {
View listItem = convertView;
if (listItem == null) {
listItem = LayoutInflater.from(mContext).inflate(R.layout.movie_item_row, parent, false);
}
MapModel currentMap = getItem(position); // Position of items
// Put the image in image1
if (currentMap != null) { // If the position of the items not null
ImageView image1 = listItem.findViewById(R.id.image1);
assert currentMap != null; // If the position of the item not null in the ImageView
Picasso.get().load(currentMap.getIcon()).into(image1);
// Put the text in name1
TextView name1 = listItem.findViewById(R.id.name1);
name1.setText(String.valueOf(currentMap.getName()));
// Put the text in address1
TextView address1 = listItem.findViewById(R.id.address1);
address1.setText(String.valueOf(currentMap.getVicinity()));
// Put the text in lat1
TextView lat1 = listItem.findViewById(R.id.lat1);
lat1.setText("n" + "n" + "Latitude: " + currentMap.getLat());
// Put the text in la2
TextView lng1 = listItem.findViewById(R.id.lng1);
lng1.setText("n" + "Longitude: " + currentMap.getLng());
}
return listItem;
}
private void getLocation() {
if (ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
mFusedLocationClient.getLastLocation().addOnSuccessListener((Executor) this, new OnSuccessListener<android.location.Location>() {
@Override
public void onSuccess(final android.location.Location location) {
// Got last known location. In some rare situations this can be null.
if (location != null) {
final android.location.Location locationA = new android.location.Location("point A");
final double latA = mapModel.getLat();
final double lngA = mapModel.getLng();
locationA.setLatitude(latA);
locationA.setLongitude(lngA);
final android.location.Location locationB = new Location("point B");
final double latB = location.getLatitude();
final double lngB = location.getLongitude();
locationB.setLatitude(latB);
locationB.setLongitude(lngB);
float distance = locationA.distanceTo(locationB);
}
}
});
}
java google-maps

how to pass data from listView in Fragment with customAdapter to map of googleMaps in second Fragment to addMarker() Function?
.................................................................................................
this is my classes are important:
FragmentOne(the listView):
public class FragmentOne extends Fragment {
private static ArrayList<MapModel> mMapList;
private static MapCustomAdapterSearch mAdapter;
private static ListView mListView;
private MapDBHelper mMapDBHelper;
private GetMapsAsyncTaskInternet mGetMapsAsyncTaskInternet;
private static ProgressDialog mProgressDialogInternet;
private static FragmentOne mFragmentOne;
private View mView;
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_one_layout, container, false);
mListView = mView.findViewById(R.id.list);
mFragmentOne = this;
mMapDBHelper = new MapDBHelper(getActivity());
mMapList = mMapDBHelper.getAllMaps();
mAdapter = new MapCustomAdapterSearch(getActivity(), mMapList);
setHasOptionsMenu(true);
registerForContextMenu(mListView);
mListView.setOnItemClickListener(new AdapterView.OnItemClickListener() {
@Override
public void onItemClick(AdapterView<?> parent, View view, int position, long id) {
Intent intentSearchToAddInternet = new Intent(getActivity().getBaseContext(), FragmentTwo.class);
intentSearchToAddInternet.putExtra(getString(R.string.movie_edit), mMapList.get(position));
getActivity().startActivity(intentSearchToAddInternet);
}
});
return mView;
}
// Set movies in FragmentOne
public static void setMaps(ArrayList<MapModel> list) {
mMapList = list;
mAdapter.clear();
mAdapter.addAll(list);
mListView.setAdapter(mAdapter);
}
// Sets off the menu of activity_menu
@Override
public void onCreateOptionsMenu(Menu menu, MenuInflater inflater) {
inflater.inflate(R.menu.activity_menu, menu);
// SearchView of FragmentOne
final MenuItem menuItem = menu.findItem(R.id.action_search);
SearchManager searchManager = (SearchManager) getActivity().getSystemService(Context.SEARCH_SERVICE);
// Change colors of the searchView upper panel
menuItem.setOnActionExpandListener(new MenuItem.OnActionExpandListener() {
@Override
public boolean onMenuItemActionExpand(MenuItem item) {
// Set styles for expanded state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.DKGRAY));
}
return true;
}
@Override
public boolean onMenuItemActionCollapse(MenuItem item) {
// Set styles for collapsed state here
if (((AppCompatActivity) getActivity()).getSupportActionBar() != null) {
((AppCompatActivity) getActivity()).getSupportActionBar().setBackgroundDrawable(new ColorDrawable(Color.TRANSPARENT));
}
return true;
}
});
// Continued of SearchView of FragmentOne
final android.support.v7.widget.SearchView searchView = (android.support.v7.widget.SearchView) menuItem.getActionView();
if (searchView != null) {
searchView.setSearchableInfo(searchManager.getSearchableInfo((getActivity()).getComponentName()));
searchView.setQueryHint(Html.fromHtml("<font color = #FFEA54>" + getResources().getString(R.string.hint) + "</font>"));
searchView.setSubmitButtonEnabled(true);
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
// Search movies from that URL and put them in the SQLiteHelper
String urlQuery = "https://maps.googleapis.com/maps/api/place/search/json?location=31.73072,34.99293&radius=1000000&rankby=prominence&types=food|restaurant&keyword="
+ query +
"&key=API_KEY";
mGetMapsAsyncTaskInternet = new GetMapsAsyncTaskInternet();
mGetMapsAsyncTaskInternet.execute(urlQuery);
return true;
}
@Override
public boolean onQueryTextChange(String newText) {
return true;
}
});
}
}
// Sets off the menu of list_menu
@Override
public void onCreateContextMenu(ContextMenu menu, View v, ContextMenu.ContextMenuInfo menuInfo) {
super.onCreateContextMenu(menu, v, menuInfo);
getActivity().getMenuInflater().inflate(R.menu.list_menu, menu);
}
// Options in the activity_menu
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.action_search:
break;
}
return super.onOptionsItemSelected(item);
}
// Options in the list_menu
@Override
public boolean onContextItemSelected(MenuItem item) {
AdapterView.AdapterContextMenuInfo info = (AdapterView.AdapterContextMenuInfo) item.getMenuInfo();
int listPosition = info.position;
switch (item.getItemId()) {
case R.id.addMap: // Edit the movies on MainActivity
Intent intent = new Intent(getActivity(), AddMapMe.class);
intent.putExtra(getString(R.string.map_add_from_internet), mMapList.get(listPosition));
startActivity(intent);
break;
}
return super.onContextItemSelected(item);
}
// stopShowingProgressBar
public static void stopShowingProgressBar() {
if (mProgressDialogInternet != null) {
mProgressDialogInternet.dismiss();
mProgressDialogInternet = null;
}
}
// startShowingProgressBar
public static void startShowingProgressBar() {
mProgressDialogInternet = ProgressDialog.show(mFragmentOne.getActivity(), "Loading...",
"Please wait...", true);
mProgressDialogInternet.show();
}
FragmentTwo(of Map):
public class FragmentTwo extends Fragment implements OnMapReadyCallback {
private GoogleMap mGoogleMap;
private MapView mMapView;
private View mView;
private MapModel mapModel;
TextView textView;
TextView textView1;
final MapModel item = (MapModel) getActivity().getIntent().getExtras().getSerializable(getString(R.string.movie_edit));
@Nullable
@Override
public View onCreateView(@NonNull LayoutInflater inflater, @Nullable ViewGroup container, @Nullable Bundle savedInstanceState) {
mView = inflater.inflate(R.layout.fragment_two_layout, container, false);
return mView;
}
@Override
public void onViewCreated(@NonNull View view, @Nullable Bundle savedInstanceState) {
super.onViewCreated(view, savedInstanceState);
mMapView = view.findViewById(R.id.map);
if (mMapView != null) {
mMapView.onCreate(null);
mMapView.onResume();
mMapView.getMapAsync(this);
}
}
@Override
public void onMapReady(GoogleMap googleMap) {
MapsInitializer.initialize(getContext());
mGoogleMap = googleMap;
addMarker();
googleMap.setMapType(GoogleMap.MAP_TYPE_TERRAIN);
if (ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_FINE_LOCATION) != PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(getContext(), Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
googleMap.setMyLocationEnabled(true);
}
public void addMarker() {
textView.setText(String.valueOf(item.getLat()));
textView1.setText(String.valueOf(item.getLng()));
String lat = textView.getText().toString();
String lng = textView1.getText().toString();
double lat1 = Double.parseDouble(lat);
double lng1 = Double.parseDouble(lng);
MarkerOptions marker = new MarkerOptions().position(new LatLng(lat1, lng1)).title("Elior home");
mGoogleMap.addMarker(marker);
mGoogleMap.setOnMarkerClickListener(new GoogleMap.OnMarkerClickListener() {
@Override
public boolean onMarkerClick(Marker marker) {
return true;
}
});
}
MapModel:
public class MapModel implements Serializable {
private float lat;
private float lng;
private String vicinity;
private String name;
private String id;
private String icon;
private Geometry geometry;
public MapModel(String name, String vicinity, float lat, float lng, String icon) {
this.vicinity = vicinity;
this.name = name;
this.lat = lat;
this.lng = lng;
this.icon = icon;
}
public float getLng() {
return geometry.getLocation().getLng();
}
public void setLng(float lng) {
this.geometry.getLocation().setLng(lng);
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public float getLat() {
return geometry.getLocation().getLat();
}
public void setLat(float lat) {
this.geometry.getLocation().setLat(lat);
}
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getVicinity() {
return vicinity;
}
public void setVicinity(String formatted_address) {
this.vicinity = formatted_address;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
MapCustomAdapterSearch:
public class MapCustomAdapterSearch extends ArrayAdapter<MapModel> {
private Context mContext; //Context
private ArrayList<MapModel> mMovieList; // ArrayList of MovieModel
private FusedLocationProviderClient mFusedLocationClient;
private MapModel mapModel;
MapCustomAdapterSearch(Context context_, ArrayList<MapModel> movie_) {
super(context_, 0, movie_);
mContext = context_;
mMovieList = movie_;
}
@NonNull
@Override
public View getView(int position, @NonNull View convertView, @NonNull ViewGroup parent) {
View listItem = convertView;
if (listItem == null) {
listItem = LayoutInflater.from(mContext).inflate(R.layout.movie_item_row, parent, false);
}
MapModel currentMap = getItem(position); // Position of items
// Put the image in image1
if (currentMap != null) { // If the position of the items not null
ImageView image1 = listItem.findViewById(R.id.image1);
assert currentMap != null; // If the position of the item not null in the ImageView
Picasso.get().load(currentMap.getIcon()).into(image1);
// Put the text in name1
TextView name1 = listItem.findViewById(R.id.name1);
name1.setText(String.valueOf(currentMap.getName()));
// Put the text in address1
TextView address1 = listItem.findViewById(R.id.address1);
address1.setText(String.valueOf(currentMap.getVicinity()));
// Put the text in lat1
TextView lat1 = listItem.findViewById(R.id.lat1);
lat1.setText("n" + "n" + "Latitude: " + currentMap.getLat());
// Put the text in la2
TextView lng1 = listItem.findViewById(R.id.lng1);
lng1.setText("n" + "Longitude: " + currentMap.getLng());
}
return listItem;
}
private void getLocation() {
if (ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_FINE_LOCATION)
!= PackageManager.PERMISSION_GRANTED && ActivityCompat.checkSelfPermission(mContext, Manifest.permission.ACCESS_COARSE_LOCATION) != PackageManager.PERMISSION_GRANTED) {
// TODO: Consider calling
// ActivityCompat#requestPermissions
// here to request the missing permissions, and then overriding
// public void onRequestPermissionsResult(int requestCode, String permissions,
// int grantResults)
// to handle the case where the user grants the permission. See the documentation
// for ActivityCompat#requestPermissions for more details.
return;
}
mFusedLocationClient.getLastLocation().addOnSuccessListener((Executor) this, new OnSuccessListener<android.location.Location>() {
@Override
public void onSuccess(final android.location.Location location) {
// Got last known location. In some rare situations this can be null.
if (location != null) {
final android.location.Location locationA = new android.location.Location("point A");
final double latA = mapModel.getLat();
final double lngA = mapModel.getLng();
locationA.setLatitude(latA);
locationA.setLongitude(lngA);
final android.location.Location locationB = new Location("point B");
final double latB = location.getLatitude();
final double lngB = location.getLongitude();
locationB.setLatitude(latB);
locationB.setLongitude(lngB);
float distance = locationA.distanceTo(locationB);
}
}
});
}
java google-maps

java google-maps

edited Nov 20 '18 at 1:21
אליאור כהן
asked Nov 20 '18 at 0:02


אליאור כהןאליאור כהן
12
12
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384372%2fpass-data-between-fragmensts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53384372%2fpass-data-between-fragmensts%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
XgZ 8zaezWPRICVUd t007jUGEzli9,saiKAMBguu0m YMihs9uXf1,djrIALNQH,d86Ot4Xm AOvrRzh,n2BTJOZt xi10S,PWXEZC8X7