UICollectionViewCell shifts out of UICollectionView bounds when editing UITextField
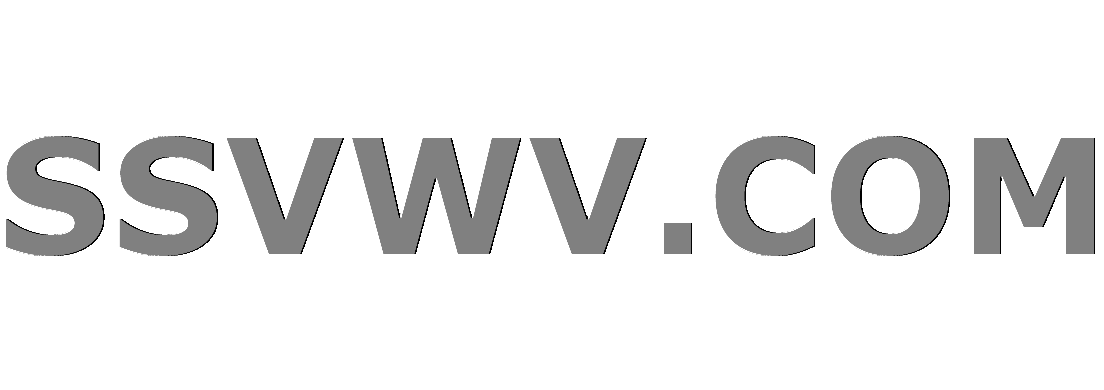
Multi tool use
I have a horizontal UICollectionView
with 5 UICollectionViewCells
that are the width of the screen. On one of my cells I have a few textfields that I would like to be editable, but when I display the soft-keyboard to edit the UITextview
that cell shifts upward for editing but it is now "stuck" at its shifted position instead of the original position I set it at.
I have also noticed that the other cells do not get effected by this, so I at least know it's not the entire UICollectionView
.
NOTE: I apologize for any misused verbiage, I can't attach images yet to help illustrate the issue.
link to screen recording
My code is as follows:
import Foundation
import UIKit
class ProfilePageCell: CVBaseCell {
var profile_ImageVw = UIImageView()
var profile_Img = UIImage()
var email_TxtField = UITextField()
var address_TxtField = UITextField()
var name_TxtField = UITextField()
var location_TxtField = UITextField()
var displayName_TxtField = UITextField()
var email_Lbl = UILabel()
var address_Lbl = UILabel()
var name_Lbl = UILabel()
var location_Lbl = UILabel()
var displayName_Lbl = UILabel()
var txtField_array:[UITextField] =
var label_array:[UILabel] =
var view_Array:[UIView] =
var vw_height = CGFloat()
var vw_width = CGFloat()
var scrollVw = UIScrollView()
override func setupViews(){
super.setupViews()
vw_width = self.frame.width
vw_height = self.frame.height
scrollVw.keyboardDismissMode = .interactive
let fade = CAGradientLayer()
fade.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
fade.colors = [Custom_Colors.color_1.withAlphaComponent(0.5).cgColor, Custom_Colors.color_2.withAlphaComponent(0.5).cgColor, Custom_Colors.color_3.withAlphaComponent(0.5).cgColor, Custom_Colors.color_4.withAlphaComponent(0.5).cgColor, Custom_Colors.color_5.withAlphaComponent(0.5).cgColor]
fade.locations = [0,0.20,0.40,0.75,0.90, 1]
self.layer.addSublayer(fade)
txtField_array = [email_TxtField,address_TxtField, location_TxtField,name_TxtField,location_TxtField,displayName_TxtField]
label_array = [email_Lbl,address_Lbl,location_Lbl,name_Lbl,displayName_Lbl]
scrollVw.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
scrollVw.isScrollEnabled = true
scrollVw.contentSize = CGSize(width: vw_width, height: vw_height*4)
profile_ImageVw.frame = CGRect(x: vw_width/2-(vw_width/6), y: vw_width/5, width: vw_width/3, height: vw_width/3)
profile_ImageVw.clipsToBounds = true
profile_ImageVw.backgroundColor = UIColor.black
profile_ImageVw.alpha = 0.5
profile_Img = UIImage(named: "profile_btn_icon")!
profile_ImageVw.image = profile_Img
profile_ImageVw.contentMode = .scaleAspectFill
profile_ImageVw.layer.cornerRadius = 60
view_Array.append(profile_ImageVw)
email_Lbl.frame = CGRect(x: vw_width/40, y: vw_height/3, width: vw_width*0.70, height: vw_height/20)
email_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: email_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
email_TxtField.placeholder = "email@domain.com"
address_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: email_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: address_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.placeholder = "123 Street Address"
location_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: address_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: location_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.placeholder = "City, State"
name_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: location_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: name_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.placeholder = "First Last"
displayName_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: name_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: displayName_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.placeholder = "YourDisplayName001"
email_Lbl.text = "Email:"
address_Lbl.text = "Address:"
location_Lbl.text = "Location:"
name_Lbl.text = "Name:"
displayName_Lbl.text = "Display Name:"
for i in 0..<label_array.count{
label_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Bold, size: email_Lbl.frame.height/2)
label_array[i].textColor = Custom_Colors.color_gray_compliment
view_Array.append(label_array[i])
}
for i in 0..<txtField_array.count{
txtField_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Regular, size: txtField_array[i].frame.height*0.40)
txtField_array[i].isEnabled = true
txtField_array[i].layer.cornerRadius = 10
txtField_array[i].backgroundColor = UIColor(white: 1, alpha: 0.5)
txtField_array[i].isUserInteractionEnabled = true
view_Array.append(txtField_array[i])
txtField_array[i].endEditing(true)
}
for i in 0..<view_Array.count{
scrollVw.addSubview(view_Array[i])
}
addSubview(scrollVw)
}
//
@objc func keyboardWillChange(notification: Notification){
print (notification.name.rawValue)
guard let keyboardFrame = notification.userInfo![UIKeyboardFrameEndUserInfoKey] as? NSValue else {
return
}
var keyboardHeight: CGFloat
keyboardHeight = keyboardFrame.cgRectValue.height
print(keyboardHeight)
}
}
swift uitextview uicollectionviewcell soft-keyboard
add a comment |
I have a horizontal UICollectionView
with 5 UICollectionViewCells
that are the width of the screen. On one of my cells I have a few textfields that I would like to be editable, but when I display the soft-keyboard to edit the UITextview
that cell shifts upward for editing but it is now "stuck" at its shifted position instead of the original position I set it at.
I have also noticed that the other cells do not get effected by this, so I at least know it's not the entire UICollectionView
.
NOTE: I apologize for any misused verbiage, I can't attach images yet to help illustrate the issue.
link to screen recording
My code is as follows:
import Foundation
import UIKit
class ProfilePageCell: CVBaseCell {
var profile_ImageVw = UIImageView()
var profile_Img = UIImage()
var email_TxtField = UITextField()
var address_TxtField = UITextField()
var name_TxtField = UITextField()
var location_TxtField = UITextField()
var displayName_TxtField = UITextField()
var email_Lbl = UILabel()
var address_Lbl = UILabel()
var name_Lbl = UILabel()
var location_Lbl = UILabel()
var displayName_Lbl = UILabel()
var txtField_array:[UITextField] =
var label_array:[UILabel] =
var view_Array:[UIView] =
var vw_height = CGFloat()
var vw_width = CGFloat()
var scrollVw = UIScrollView()
override func setupViews(){
super.setupViews()
vw_width = self.frame.width
vw_height = self.frame.height
scrollVw.keyboardDismissMode = .interactive
let fade = CAGradientLayer()
fade.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
fade.colors = [Custom_Colors.color_1.withAlphaComponent(0.5).cgColor, Custom_Colors.color_2.withAlphaComponent(0.5).cgColor, Custom_Colors.color_3.withAlphaComponent(0.5).cgColor, Custom_Colors.color_4.withAlphaComponent(0.5).cgColor, Custom_Colors.color_5.withAlphaComponent(0.5).cgColor]
fade.locations = [0,0.20,0.40,0.75,0.90, 1]
self.layer.addSublayer(fade)
txtField_array = [email_TxtField,address_TxtField, location_TxtField,name_TxtField,location_TxtField,displayName_TxtField]
label_array = [email_Lbl,address_Lbl,location_Lbl,name_Lbl,displayName_Lbl]
scrollVw.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
scrollVw.isScrollEnabled = true
scrollVw.contentSize = CGSize(width: vw_width, height: vw_height*4)
profile_ImageVw.frame = CGRect(x: vw_width/2-(vw_width/6), y: vw_width/5, width: vw_width/3, height: vw_width/3)
profile_ImageVw.clipsToBounds = true
profile_ImageVw.backgroundColor = UIColor.black
profile_ImageVw.alpha = 0.5
profile_Img = UIImage(named: "profile_btn_icon")!
profile_ImageVw.image = profile_Img
profile_ImageVw.contentMode = .scaleAspectFill
profile_ImageVw.layer.cornerRadius = 60
view_Array.append(profile_ImageVw)
email_Lbl.frame = CGRect(x: vw_width/40, y: vw_height/3, width: vw_width*0.70, height: vw_height/20)
email_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: email_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
email_TxtField.placeholder = "email@domain.com"
address_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: email_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: address_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.placeholder = "123 Street Address"
location_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: address_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: location_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.placeholder = "City, State"
name_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: location_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: name_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.placeholder = "First Last"
displayName_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: name_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: displayName_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.placeholder = "YourDisplayName001"
email_Lbl.text = "Email:"
address_Lbl.text = "Address:"
location_Lbl.text = "Location:"
name_Lbl.text = "Name:"
displayName_Lbl.text = "Display Name:"
for i in 0..<label_array.count{
label_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Bold, size: email_Lbl.frame.height/2)
label_array[i].textColor = Custom_Colors.color_gray_compliment
view_Array.append(label_array[i])
}
for i in 0..<txtField_array.count{
txtField_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Regular, size: txtField_array[i].frame.height*0.40)
txtField_array[i].isEnabled = true
txtField_array[i].layer.cornerRadius = 10
txtField_array[i].backgroundColor = UIColor(white: 1, alpha: 0.5)
txtField_array[i].isUserInteractionEnabled = true
view_Array.append(txtField_array[i])
txtField_array[i].endEditing(true)
}
for i in 0..<view_Array.count{
scrollVw.addSubview(view_Array[i])
}
addSubview(scrollVw)
}
//
@objc func keyboardWillChange(notification: Notification){
print (notification.name.rawValue)
guard let keyboardFrame = notification.userInfo![UIKeyboardFrameEndUserInfoKey] as? NSValue else {
return
}
var keyboardHeight: CGFloat
keyboardHeight = keyboardFrame.cgRectValue.height
print(keyboardHeight)
}
}
swift uitextview uicollectionviewcell soft-keyboard
Did you change tableView frame or inset when the keyboard appear? If so you have to manually set it back when the keyboard disappears
– Linh Ta
Nov 19 '18 at 2:29
@LinhTa No, I haven't changed the insets manually.
– iGotQuestions
Nov 20 '18 at 4:45
add a comment |
I have a horizontal UICollectionView
with 5 UICollectionViewCells
that are the width of the screen. On one of my cells I have a few textfields that I would like to be editable, but when I display the soft-keyboard to edit the UITextview
that cell shifts upward for editing but it is now "stuck" at its shifted position instead of the original position I set it at.
I have also noticed that the other cells do not get effected by this, so I at least know it's not the entire UICollectionView
.
NOTE: I apologize for any misused verbiage, I can't attach images yet to help illustrate the issue.
link to screen recording
My code is as follows:
import Foundation
import UIKit
class ProfilePageCell: CVBaseCell {
var profile_ImageVw = UIImageView()
var profile_Img = UIImage()
var email_TxtField = UITextField()
var address_TxtField = UITextField()
var name_TxtField = UITextField()
var location_TxtField = UITextField()
var displayName_TxtField = UITextField()
var email_Lbl = UILabel()
var address_Lbl = UILabel()
var name_Lbl = UILabel()
var location_Lbl = UILabel()
var displayName_Lbl = UILabel()
var txtField_array:[UITextField] =
var label_array:[UILabel] =
var view_Array:[UIView] =
var vw_height = CGFloat()
var vw_width = CGFloat()
var scrollVw = UIScrollView()
override func setupViews(){
super.setupViews()
vw_width = self.frame.width
vw_height = self.frame.height
scrollVw.keyboardDismissMode = .interactive
let fade = CAGradientLayer()
fade.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
fade.colors = [Custom_Colors.color_1.withAlphaComponent(0.5).cgColor, Custom_Colors.color_2.withAlphaComponent(0.5).cgColor, Custom_Colors.color_3.withAlphaComponent(0.5).cgColor, Custom_Colors.color_4.withAlphaComponent(0.5).cgColor, Custom_Colors.color_5.withAlphaComponent(0.5).cgColor]
fade.locations = [0,0.20,0.40,0.75,0.90, 1]
self.layer.addSublayer(fade)
txtField_array = [email_TxtField,address_TxtField, location_TxtField,name_TxtField,location_TxtField,displayName_TxtField]
label_array = [email_Lbl,address_Lbl,location_Lbl,name_Lbl,displayName_Lbl]
scrollVw.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
scrollVw.isScrollEnabled = true
scrollVw.contentSize = CGSize(width: vw_width, height: vw_height*4)
profile_ImageVw.frame = CGRect(x: vw_width/2-(vw_width/6), y: vw_width/5, width: vw_width/3, height: vw_width/3)
profile_ImageVw.clipsToBounds = true
profile_ImageVw.backgroundColor = UIColor.black
profile_ImageVw.alpha = 0.5
profile_Img = UIImage(named: "profile_btn_icon")!
profile_ImageVw.image = profile_Img
profile_ImageVw.contentMode = .scaleAspectFill
profile_ImageVw.layer.cornerRadius = 60
view_Array.append(profile_ImageVw)
email_Lbl.frame = CGRect(x: vw_width/40, y: vw_height/3, width: vw_width*0.70, height: vw_height/20)
email_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: email_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
email_TxtField.placeholder = "email@domain.com"
address_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: email_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: address_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.placeholder = "123 Street Address"
location_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: address_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: location_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.placeholder = "City, State"
name_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: location_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: name_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.placeholder = "First Last"
displayName_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: name_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: displayName_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.placeholder = "YourDisplayName001"
email_Lbl.text = "Email:"
address_Lbl.text = "Address:"
location_Lbl.text = "Location:"
name_Lbl.text = "Name:"
displayName_Lbl.text = "Display Name:"
for i in 0..<label_array.count{
label_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Bold, size: email_Lbl.frame.height/2)
label_array[i].textColor = Custom_Colors.color_gray_compliment
view_Array.append(label_array[i])
}
for i in 0..<txtField_array.count{
txtField_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Regular, size: txtField_array[i].frame.height*0.40)
txtField_array[i].isEnabled = true
txtField_array[i].layer.cornerRadius = 10
txtField_array[i].backgroundColor = UIColor(white: 1, alpha: 0.5)
txtField_array[i].isUserInteractionEnabled = true
view_Array.append(txtField_array[i])
txtField_array[i].endEditing(true)
}
for i in 0..<view_Array.count{
scrollVw.addSubview(view_Array[i])
}
addSubview(scrollVw)
}
//
@objc func keyboardWillChange(notification: Notification){
print (notification.name.rawValue)
guard let keyboardFrame = notification.userInfo![UIKeyboardFrameEndUserInfoKey] as? NSValue else {
return
}
var keyboardHeight: CGFloat
keyboardHeight = keyboardFrame.cgRectValue.height
print(keyboardHeight)
}
}
swift uitextview uicollectionviewcell soft-keyboard
I have a horizontal UICollectionView
with 5 UICollectionViewCells
that are the width of the screen. On one of my cells I have a few textfields that I would like to be editable, but when I display the soft-keyboard to edit the UITextview
that cell shifts upward for editing but it is now "stuck" at its shifted position instead of the original position I set it at.
I have also noticed that the other cells do not get effected by this, so I at least know it's not the entire UICollectionView
.
NOTE: I apologize for any misused verbiage, I can't attach images yet to help illustrate the issue.
link to screen recording
My code is as follows:
import Foundation
import UIKit
class ProfilePageCell: CVBaseCell {
var profile_ImageVw = UIImageView()
var profile_Img = UIImage()
var email_TxtField = UITextField()
var address_TxtField = UITextField()
var name_TxtField = UITextField()
var location_TxtField = UITextField()
var displayName_TxtField = UITextField()
var email_Lbl = UILabel()
var address_Lbl = UILabel()
var name_Lbl = UILabel()
var location_Lbl = UILabel()
var displayName_Lbl = UILabel()
var txtField_array:[UITextField] =
var label_array:[UILabel] =
var view_Array:[UIView] =
var vw_height = CGFloat()
var vw_width = CGFloat()
var scrollVw = UIScrollView()
override func setupViews(){
super.setupViews()
vw_width = self.frame.width
vw_height = self.frame.height
scrollVw.keyboardDismissMode = .interactive
let fade = CAGradientLayer()
fade.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
fade.colors = [Custom_Colors.color_1.withAlphaComponent(0.5).cgColor, Custom_Colors.color_2.withAlphaComponent(0.5).cgColor, Custom_Colors.color_3.withAlphaComponent(0.5).cgColor, Custom_Colors.color_4.withAlphaComponent(0.5).cgColor, Custom_Colors.color_5.withAlphaComponent(0.5).cgColor]
fade.locations = [0,0.20,0.40,0.75,0.90, 1]
self.layer.addSublayer(fade)
txtField_array = [email_TxtField,address_TxtField, location_TxtField,name_TxtField,location_TxtField,displayName_TxtField]
label_array = [email_Lbl,address_Lbl,location_Lbl,name_Lbl,displayName_Lbl]
scrollVw.frame = CGRect(x: 0, y: 0, width: vw_width, height: vw_height)
scrollVw.isScrollEnabled = true
scrollVw.contentSize = CGSize(width: vw_width, height: vw_height*4)
profile_ImageVw.frame = CGRect(x: vw_width/2-(vw_width/6), y: vw_width/5, width: vw_width/3, height: vw_width/3)
profile_ImageVw.clipsToBounds = true
profile_ImageVw.backgroundColor = UIColor.black
profile_ImageVw.alpha = 0.5
profile_Img = UIImage(named: "profile_btn_icon")!
profile_ImageVw.image = profile_Img
profile_ImageVw.contentMode = .scaleAspectFill
profile_ImageVw.layer.cornerRadius = 60
view_Array.append(profile_ImageVw)
email_Lbl.frame = CGRect(x: vw_width/40, y: vw_height/3, width: vw_width*0.70, height: vw_height/20)
email_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: email_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
email_TxtField.placeholder = "email@domain.com"
address_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: email_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: address_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
address_TxtField.placeholder = "123 Street Address"
location_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: address_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: location_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
location_TxtField.placeholder = "City, State"
name_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: location_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: name_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
name_TxtField.placeholder = "First Last"
displayName_Lbl.frame = CGRect(x: email_Lbl.frame.minX, y: name_TxtField.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.frame = CGRect(x: email_Lbl.frame.minX, y: displayName_Lbl.frame.maxY, width: email_Lbl.frame.width, height: email_Lbl.frame.height)
displayName_TxtField.placeholder = "YourDisplayName001"
email_Lbl.text = "Email:"
address_Lbl.text = "Address:"
location_Lbl.text = "Location:"
name_Lbl.text = "Name:"
displayName_Lbl.text = "Display Name:"
for i in 0..<label_array.count{
label_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Bold, size: email_Lbl.frame.height/2)
label_array[i].textColor = Custom_Colors.color_gray_compliment
view_Array.append(label_array[i])
}
for i in 0..<txtField_array.count{
txtField_array[i].font = UIFont(name: Custom_Fonts.Google_ProductSans_Regular, size: txtField_array[i].frame.height*0.40)
txtField_array[i].isEnabled = true
txtField_array[i].layer.cornerRadius = 10
txtField_array[i].backgroundColor = UIColor(white: 1, alpha: 0.5)
txtField_array[i].isUserInteractionEnabled = true
view_Array.append(txtField_array[i])
txtField_array[i].endEditing(true)
}
for i in 0..<view_Array.count{
scrollVw.addSubview(view_Array[i])
}
addSubview(scrollVw)
}
//
@objc func keyboardWillChange(notification: Notification){
print (notification.name.rawValue)
guard let keyboardFrame = notification.userInfo![UIKeyboardFrameEndUserInfoKey] as? NSValue else {
return
}
var keyboardHeight: CGFloat
keyboardHeight = keyboardFrame.cgRectValue.height
print(keyboardHeight)
}
}
swift uitextview uicollectionviewcell soft-keyboard
swift uitextview uicollectionviewcell soft-keyboard
edited Nov 17 '18 at 7:16
Vicky_Vignesh
9410
9410
asked Nov 17 '18 at 5:21
iGotQuestionsiGotQuestions
84
84
Did you change tableView frame or inset when the keyboard appear? If so you have to manually set it back when the keyboard disappears
– Linh Ta
Nov 19 '18 at 2:29
@LinhTa No, I haven't changed the insets manually.
– iGotQuestions
Nov 20 '18 at 4:45
add a comment |
Did you change tableView frame or inset when the keyboard appear? If so you have to manually set it back when the keyboard disappears
– Linh Ta
Nov 19 '18 at 2:29
@LinhTa No, I haven't changed the insets manually.
– iGotQuestions
Nov 20 '18 at 4:45
Did you change tableView frame or inset when the keyboard appear? If so you have to manually set it back when the keyboard disappears
– Linh Ta
Nov 19 '18 at 2:29
Did you change tableView frame or inset when the keyboard appear? If so you have to manually set it back when the keyboard disappears
– Linh Ta
Nov 19 '18 at 2:29
@LinhTa No, I haven't changed the insets manually.
– iGotQuestions
Nov 20 '18 at 4:45
@LinhTa No, I haven't changed the insets manually.
– iGotQuestions
Nov 20 '18 at 4:45
add a comment |
1 Answer
1
active
oldest
votes
You have two options:
1) use a third party library to take care of all your views where a keyboard might appear. Take a look at IQKeyboardManager.
2) add a top constraint to your containerView and do smth like this:
func keyboardWillShow(_ n: Notification) {
let keyboardFrame = (n.userInfo![UIKeyboardFrameEndUserInfoKey] as AnyObject).cgRectValue ?? CGRect.zero
let passFrame = self.view.convert(textField1.frame, from: textField1.superview)
if keyboardFrame.intersects(passFrame) {
let diff = passFrame.maxY - keyboardFrame.origin.y;
self.topSpaceConstraint.constant -= (diff + 10);
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
}
func keyboardWillHide(_ n: Notification) {
self.topSpaceConstraint.constant = 94
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
Take note that you will need to know which textField is currently edited, so if the keyboard frame does pass beyond the frame of it, your view should be pushed upwards.
As usual subscribe to UIKeyboardWillShow
and UIKeyboardWillHide
notifications for this to work.
I will suggest going with first option, it's much elegant and works great :]
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348488%2fuicollectionviewcell-shifts-out-of-uicollectionview-bounds-when-editing-uitextfi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
You have two options:
1) use a third party library to take care of all your views where a keyboard might appear. Take a look at IQKeyboardManager.
2) add a top constraint to your containerView and do smth like this:
func keyboardWillShow(_ n: Notification) {
let keyboardFrame = (n.userInfo![UIKeyboardFrameEndUserInfoKey] as AnyObject).cgRectValue ?? CGRect.zero
let passFrame = self.view.convert(textField1.frame, from: textField1.superview)
if keyboardFrame.intersects(passFrame) {
let diff = passFrame.maxY - keyboardFrame.origin.y;
self.topSpaceConstraint.constant -= (diff + 10);
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
}
func keyboardWillHide(_ n: Notification) {
self.topSpaceConstraint.constant = 94
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
Take note that you will need to know which textField is currently edited, so if the keyboard frame does pass beyond the frame of it, your view should be pushed upwards.
As usual subscribe to UIKeyboardWillShow
and UIKeyboardWillHide
notifications for this to work.
I will suggest going with first option, it's much elegant and works great :]
add a comment |
You have two options:
1) use a third party library to take care of all your views where a keyboard might appear. Take a look at IQKeyboardManager.
2) add a top constraint to your containerView and do smth like this:
func keyboardWillShow(_ n: Notification) {
let keyboardFrame = (n.userInfo![UIKeyboardFrameEndUserInfoKey] as AnyObject).cgRectValue ?? CGRect.zero
let passFrame = self.view.convert(textField1.frame, from: textField1.superview)
if keyboardFrame.intersects(passFrame) {
let diff = passFrame.maxY - keyboardFrame.origin.y;
self.topSpaceConstraint.constant -= (diff + 10);
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
}
func keyboardWillHide(_ n: Notification) {
self.topSpaceConstraint.constant = 94
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
Take note that you will need to know which textField is currently edited, so if the keyboard frame does pass beyond the frame of it, your view should be pushed upwards.
As usual subscribe to UIKeyboardWillShow
and UIKeyboardWillHide
notifications for this to work.
I will suggest going with first option, it's much elegant and works great :]
add a comment |
You have two options:
1) use a third party library to take care of all your views where a keyboard might appear. Take a look at IQKeyboardManager.
2) add a top constraint to your containerView and do smth like this:
func keyboardWillShow(_ n: Notification) {
let keyboardFrame = (n.userInfo![UIKeyboardFrameEndUserInfoKey] as AnyObject).cgRectValue ?? CGRect.zero
let passFrame = self.view.convert(textField1.frame, from: textField1.superview)
if keyboardFrame.intersects(passFrame) {
let diff = passFrame.maxY - keyboardFrame.origin.y;
self.topSpaceConstraint.constant -= (diff + 10);
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
}
func keyboardWillHide(_ n: Notification) {
self.topSpaceConstraint.constant = 94
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
Take note that you will need to know which textField is currently edited, so if the keyboard frame does pass beyond the frame of it, your view should be pushed upwards.
As usual subscribe to UIKeyboardWillShow
and UIKeyboardWillHide
notifications for this to work.
I will suggest going with first option, it's much elegant and works great :]
You have two options:
1) use a third party library to take care of all your views where a keyboard might appear. Take a look at IQKeyboardManager.
2) add a top constraint to your containerView and do smth like this:
func keyboardWillShow(_ n: Notification) {
let keyboardFrame = (n.userInfo![UIKeyboardFrameEndUserInfoKey] as AnyObject).cgRectValue ?? CGRect.zero
let passFrame = self.view.convert(textField1.frame, from: textField1.superview)
if keyboardFrame.intersects(passFrame) {
let diff = passFrame.maxY - keyboardFrame.origin.y;
self.topSpaceConstraint.constant -= (diff + 10);
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
}
func keyboardWillHide(_ n: Notification) {
self.topSpaceConstraint.constant = 94
UIView.animate(withDuration: 0.3, animations: {
self.view.layoutIfNeeded()
})
}
Take note that you will need to know which textField is currently edited, so if the keyboard frame does pass beyond the frame of it, your view should be pushed upwards.
As usual subscribe to UIKeyboardWillShow
and UIKeyboardWillHide
notifications for this to work.
I will suggest going with first option, it's much elegant and works great :]
edited Nov 21 '18 at 22:47
answered Nov 19 '18 at 16:59
Deryck LucianDeryck Lucian
1558
1558
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53348488%2fuicollectionviewcell-shifts-out-of-uicollectionview-bounds-when-editing-uitextfi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
P 7LLm2R8w6aCY,sQwSdW1 0Sva,hu7Zw5Z8n3938u3xD kT8 AU wP,q t93sbJHigLVkP,G4e8MTgZgt2 EgFZA3 odgayt4
Did you change tableView frame or inset when the keyboard appear? If so you have to manually set it back when the keyboard disappears
– Linh Ta
Nov 19 '18 at 2:29
@LinhTa No, I haven't changed the insets manually.
– iGotQuestions
Nov 20 '18 at 4:45