Calling (and catching) multiple promises
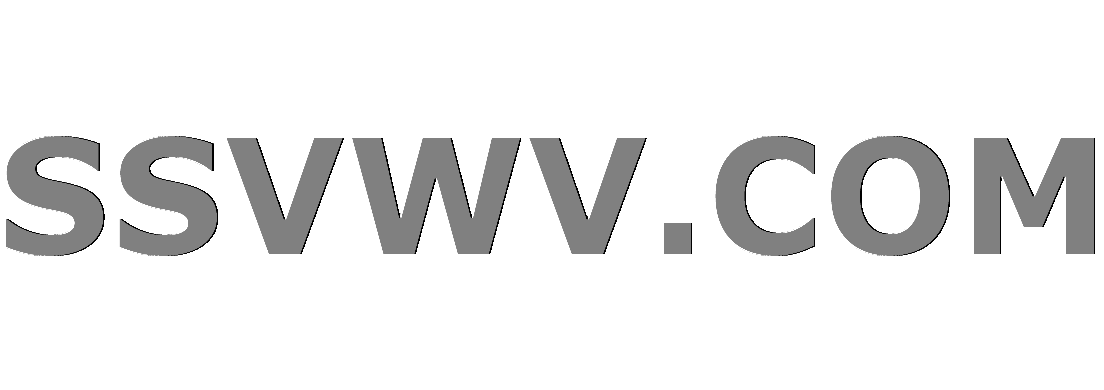
Multi tool use
I am trying to run below code (modified from this link):
let cnt = 1;
var prom = () => new Promise(
function (resolve, reject) {
try {
console.log('enter promise ' + cnt);
if (Math.random() < 0.5) throw new Error('error in promise ' + cnt);
resolve('done in promise ' + cnt);
}
catch (e) {
reject(e);
}
finally{
cnt++;
}
}
);
prom()
.then(prom())
.then(function (fulfilled) {
console.log('all done: ' + fulfilled);
})
.catch(function (error) {
console.log(error.message);
});
Basically I am trying to achieve a catch-all behaviour, i.e. if either promise fails, catch and stop processing. However can't achieve. What am I missing?
node.js promise
add a comment |
I am trying to run below code (modified from this link):
let cnt = 1;
var prom = () => new Promise(
function (resolve, reject) {
try {
console.log('enter promise ' + cnt);
if (Math.random() < 0.5) throw new Error('error in promise ' + cnt);
resolve('done in promise ' + cnt);
}
catch (e) {
reject(e);
}
finally{
cnt++;
}
}
);
prom()
.then(prom())
.then(function (fulfilled) {
console.log('all done: ' + fulfilled);
})
.catch(function (error) {
console.log(error.message);
});
Basically I am trying to achieve a catch-all behaviour, i.e. if either promise fails, catch and stop processing. However can't achieve. What am I missing?
node.js promise
What is happening? Are you getting an error? What error?
– iagowp
Nov 21 '18 at 22:54
Do not pass a promise tothen()
. Pass a callback function instead, e.g..then(prom)
.
– Bergi
Nov 21 '18 at 22:57
this looks like the issue; can you make it an answer?
– paul simmons
Nov 21 '18 at 22:58
Which example exactly from the linked page did you use, and what and why did you modify?
– Bergi
Nov 21 '18 at 22:59
add a comment |
I am trying to run below code (modified from this link):
let cnt = 1;
var prom = () => new Promise(
function (resolve, reject) {
try {
console.log('enter promise ' + cnt);
if (Math.random() < 0.5) throw new Error('error in promise ' + cnt);
resolve('done in promise ' + cnt);
}
catch (e) {
reject(e);
}
finally{
cnt++;
}
}
);
prom()
.then(prom())
.then(function (fulfilled) {
console.log('all done: ' + fulfilled);
})
.catch(function (error) {
console.log(error.message);
});
Basically I am trying to achieve a catch-all behaviour, i.e. if either promise fails, catch and stop processing. However can't achieve. What am I missing?
node.js promise
I am trying to run below code (modified from this link):
let cnt = 1;
var prom = () => new Promise(
function (resolve, reject) {
try {
console.log('enter promise ' + cnt);
if (Math.random() < 0.5) throw new Error('error in promise ' + cnt);
resolve('done in promise ' + cnt);
}
catch (e) {
reject(e);
}
finally{
cnt++;
}
}
);
prom()
.then(prom())
.then(function (fulfilled) {
console.log('all done: ' + fulfilled);
})
.catch(function (error) {
console.log(error.message);
});
Basically I am trying to achieve a catch-all behaviour, i.e. if either promise fails, catch and stop processing. However can't achieve. What am I missing?
node.js promise
node.js promise
asked Nov 21 '18 at 22:47
paul simmonspaul simmons
2,503104672
2,503104672
What is happening? Are you getting an error? What error?
– iagowp
Nov 21 '18 at 22:54
Do not pass a promise tothen()
. Pass a callback function instead, e.g..then(prom)
.
– Bergi
Nov 21 '18 at 22:57
this looks like the issue; can you make it an answer?
– paul simmons
Nov 21 '18 at 22:58
Which example exactly from the linked page did you use, and what and why did you modify?
– Bergi
Nov 21 '18 at 22:59
add a comment |
What is happening? Are you getting an error? What error?
– iagowp
Nov 21 '18 at 22:54
Do not pass a promise tothen()
. Pass a callback function instead, e.g..then(prom)
.
– Bergi
Nov 21 '18 at 22:57
this looks like the issue; can you make it an answer?
– paul simmons
Nov 21 '18 at 22:58
Which example exactly from the linked page did you use, and what and why did you modify?
– Bergi
Nov 21 '18 at 22:59
What is happening? Are you getting an error? What error?
– iagowp
Nov 21 '18 at 22:54
What is happening? Are you getting an error? What error?
– iagowp
Nov 21 '18 at 22:54
Do not pass a promise to
then()
. Pass a callback function instead, e.g. .then(prom)
.– Bergi
Nov 21 '18 at 22:57
Do not pass a promise to
then()
. Pass a callback function instead, e.g. .then(prom)
.– Bergi
Nov 21 '18 at 22:57
this looks like the issue; can you make it an answer?
– paul simmons
Nov 21 '18 at 22:58
this looks like the issue; can you make it an answer?
– paul simmons
Nov 21 '18 at 22:58
Which example exactly from the linked page did you use, and what and why did you modify?
– Bergi
Nov 21 '18 at 22:59
Which example exactly from the linked page did you use, and what and why did you modify?
– Bergi
Nov 21 '18 at 22:59
add a comment |
2 Answers
2
active
oldest
votes
.then()
takes a callback as an argument and you gave it the returned Promise itself.
Use .then(prom)
instead.
add a comment |
Use Promise.all
return Promise.all([
prom(),
prom()
])
.then(([fullfilled1, fulfilled2]) => {
console.log('all done: ' + fulfilled1 + ' ' + fulfilled2);
})
.catch(err => {
console.log(error.message);
});
If one fails, everyone fails.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421498%2fcalling-and-catching-multiple-promises%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
.then()
takes a callback as an argument and you gave it the returned Promise itself.
Use .then(prom)
instead.
add a comment |
.then()
takes a callback as an argument and you gave it the returned Promise itself.
Use .then(prom)
instead.
add a comment |
.then()
takes a callback as an argument and you gave it the returned Promise itself.
Use .then(prom)
instead.
.then()
takes a callback as an argument and you gave it the returned Promise itself.
Use .then(prom)
instead.
answered Nov 21 '18 at 23:04
Sebastian SpeitelSebastian Speitel
4,5682627
4,5682627
add a comment |
add a comment |
Use Promise.all
return Promise.all([
prom(),
prom()
])
.then(([fullfilled1, fulfilled2]) => {
console.log('all done: ' + fulfilled1 + ' ' + fulfilled2);
})
.catch(err => {
console.log(error.message);
});
If one fails, everyone fails.
add a comment |
Use Promise.all
return Promise.all([
prom(),
prom()
])
.then(([fullfilled1, fulfilled2]) => {
console.log('all done: ' + fulfilled1 + ' ' + fulfilled2);
})
.catch(err => {
console.log(error.message);
});
If one fails, everyone fails.
add a comment |
Use Promise.all
return Promise.all([
prom(),
prom()
])
.then(([fullfilled1, fulfilled2]) => {
console.log('all done: ' + fulfilled1 + ' ' + fulfilled2);
})
.catch(err => {
console.log(error.message);
});
If one fails, everyone fails.
Use Promise.all
return Promise.all([
prom(),
prom()
])
.then(([fullfilled1, fulfilled2]) => {
console.log('all done: ' + fulfilled1 + ' ' + fulfilled2);
})
.catch(err => {
console.log(error.message);
});
If one fails, everyone fails.
answered Nov 21 '18 at 23:37
dvsoukupdvsoukup
1,062923
1,062923
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53421498%2fcalling-and-catching-multiple-promises%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
zqqzdF7nr5t6W9aV
What is happening? Are you getting an error? What error?
– iagowp
Nov 21 '18 at 22:54
Do not pass a promise to
then()
. Pass a callback function instead, e.g..then(prom)
.– Bergi
Nov 21 '18 at 22:57
this looks like the issue; can you make it an answer?
– paul simmons
Nov 21 '18 at 22:58
Which example exactly from the linked page did you use, and what and why did you modify?
– Bergi
Nov 21 '18 at 22:59