JsonConvert.DeserializeObject Returns null list
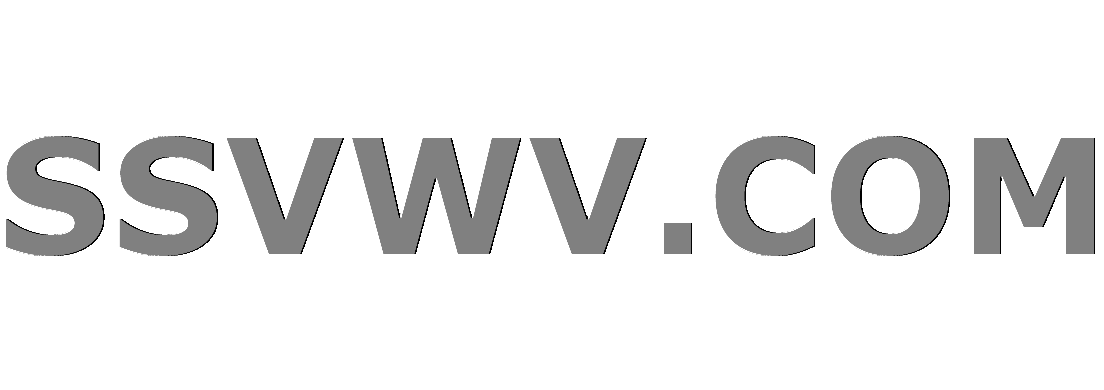
Multi tool use
Hoping someone can catch what I'm doing wrong. CardCollection.cs just contains a List called cards, with a public methods Cards to act as getter/setter.
I should point out that I'm using Visual Studio 2017 for development, and when I inspect the string "jsonString" in debug mode using the built-in JSON Visualizer it displays properly in that window. Unfortunately JsonConvert.DeserializeObject does not properly set my CardCollection object and results in an empty list of cards
From "Form.cs"
private async void SelectFileButton_ClickAsync(object sender, EventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
dialog.Filter = "JSON Files|*.json";
dialog.Title = "Select a JSON File";
if (dialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string line = "";
string jsonString = "";
string fileName = dialog.FileName;
StringBuilder builder = new StringBuilder("");
using (StreamReader reader = File.OpenText(dialog.FileName))
{
while ((line = await reader.ReadLineAsync()) != null)
{
line = line.Replace("t", "");
line = line.Replace("n", "");
builder.Append(line);
}
}
jsonString = builder.ToString();
var settings = new JsonSerializerSettings
{
NullValueHandling = NullValueHandling.Ignore,
MissingMemberHandling = MissingMemberHandling.Ignore
};
CardCollection cardList = JsonConvert.DeserializeObject<CardCollection>(jsonString, settings);
}
}
Card.cs
public class Card
{
string colorIdentity;
string colors;
double convertedManaCost;
ForeignData foreignData;
bool isReserved;
string layout;
Legality legalities;
string loyalty;
string manaCost;
string name;
string power;
string printings;
Ruling rulings;
string subtypes;
string supertypes;
string text;
string toughness;
string type;
string types;
string uuid;
public string ColorIdentity { get => colorIdentity; set => colorIdentity = value; }
public string Colors { get => colors; set => colors = value; }
public double ConvertedManaCost { get => convertedManaCost; set => convertedManaCost = value; }
public bool IsReserved { get => isReserved; set => isReserved = value; }
public string Layout { get => layout; set => layout = value; }
public string Loyalty { get => loyalty; set => loyalty = value; }
public string ManaCost { get => manaCost; set => manaCost = value; }
public string Name { get => name; set => name = value; }
public string Power { get => power; set => power = value; }
public string Printings { get => printings; set => printings = value; }
public string Subtypes { get => subtypes; set => subtypes = value; }
public string Supertypes { get => supertypes; set => supertypes = value; }
public string Text { get => text; set => text = value; }
public string Toughness { get => toughness; set => toughness = value; }
public string Type { get => type; set => type = value; }
public string Types { get => types; set => types = value; }
public string Uuid { get => uuid; set => uuid = value; }
internal ForeignData ForeignData { get => foreignData; set => foreignData = value; }
internal Legality Legalities { get => legalities; set => legalities = value; }
internal Ruling Rulings { get => rulings; set => rulings = value; }
}
Here is sample of the JSON file
{
"1999 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "1999 World Championships Ad",
"printings": [
"WC99"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "8635dea0-985a-4c02-a02b-8b08d96fb2dd"
},
"2004 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "2004 World Championships Ad",
"printings": [
"WC04"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "cbd6a762-f3c2-4b29-a07e-f0c9c81cf1ec"
},
"A Display of My Dark Power": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "scheme",
"legalities": {},
"name": "A Display of My Dark Power",
"printings": [
"ARC"
],
"rulings": [
{
"date": "2010-06-15",
"text": "The ability affects all players, not just you."
},
{
"date": "2010-06-15",
"text": "The effect doesn’t wear off until just before your next untap step (even if an effect will cause that untap step to be skipped)."
},
{
"date": "2010-06-15",
"text": "The types of mana are white, blue, black, red, green, and colorless."
},
{
"date": "2010-06-15",
"text": "If a land produces more than one type of mana at a single time (as Boros Garrison does, for example), the land’s controller chooses which one of those types of mana is produced by the delayed triggered ability."
},
{
"date": "2010-06-15",
"text": "If a land is tapped for mana but doesn’t produce any (for example, if you tap Gaea’s Cradle for mana while you control no creatures), the delayed triggered ability won’t trigger."
}
],
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "When you set this scheme in motion, until your next turn, whenever a player taps a land for mana, that player adds one mana of any type that land produced.",
"type": "Scheme",
"types": [
"Scheme"
],
"uuid": "c5287e77-890d-41bd-acc6-7a8f1866426d"
}
}
c# json json-deserialization
add a comment |
Hoping someone can catch what I'm doing wrong. CardCollection.cs just contains a List called cards, with a public methods Cards to act as getter/setter.
I should point out that I'm using Visual Studio 2017 for development, and when I inspect the string "jsonString" in debug mode using the built-in JSON Visualizer it displays properly in that window. Unfortunately JsonConvert.DeserializeObject does not properly set my CardCollection object and results in an empty list of cards
From "Form.cs"
private async void SelectFileButton_ClickAsync(object sender, EventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
dialog.Filter = "JSON Files|*.json";
dialog.Title = "Select a JSON File";
if (dialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string line = "";
string jsonString = "";
string fileName = dialog.FileName;
StringBuilder builder = new StringBuilder("");
using (StreamReader reader = File.OpenText(dialog.FileName))
{
while ((line = await reader.ReadLineAsync()) != null)
{
line = line.Replace("t", "");
line = line.Replace("n", "");
builder.Append(line);
}
}
jsonString = builder.ToString();
var settings = new JsonSerializerSettings
{
NullValueHandling = NullValueHandling.Ignore,
MissingMemberHandling = MissingMemberHandling.Ignore
};
CardCollection cardList = JsonConvert.DeserializeObject<CardCollection>(jsonString, settings);
}
}
Card.cs
public class Card
{
string colorIdentity;
string colors;
double convertedManaCost;
ForeignData foreignData;
bool isReserved;
string layout;
Legality legalities;
string loyalty;
string manaCost;
string name;
string power;
string printings;
Ruling rulings;
string subtypes;
string supertypes;
string text;
string toughness;
string type;
string types;
string uuid;
public string ColorIdentity { get => colorIdentity; set => colorIdentity = value; }
public string Colors { get => colors; set => colors = value; }
public double ConvertedManaCost { get => convertedManaCost; set => convertedManaCost = value; }
public bool IsReserved { get => isReserved; set => isReserved = value; }
public string Layout { get => layout; set => layout = value; }
public string Loyalty { get => loyalty; set => loyalty = value; }
public string ManaCost { get => manaCost; set => manaCost = value; }
public string Name { get => name; set => name = value; }
public string Power { get => power; set => power = value; }
public string Printings { get => printings; set => printings = value; }
public string Subtypes { get => subtypes; set => subtypes = value; }
public string Supertypes { get => supertypes; set => supertypes = value; }
public string Text { get => text; set => text = value; }
public string Toughness { get => toughness; set => toughness = value; }
public string Type { get => type; set => type = value; }
public string Types { get => types; set => types = value; }
public string Uuid { get => uuid; set => uuid = value; }
internal ForeignData ForeignData { get => foreignData; set => foreignData = value; }
internal Legality Legalities { get => legalities; set => legalities = value; }
internal Ruling Rulings { get => rulings; set => rulings = value; }
}
Here is sample of the JSON file
{
"1999 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "1999 World Championships Ad",
"printings": [
"WC99"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "8635dea0-985a-4c02-a02b-8b08d96fb2dd"
},
"2004 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "2004 World Championships Ad",
"printings": [
"WC04"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "cbd6a762-f3c2-4b29-a07e-f0c9c81cf1ec"
},
"A Display of My Dark Power": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "scheme",
"legalities": {},
"name": "A Display of My Dark Power",
"printings": [
"ARC"
],
"rulings": [
{
"date": "2010-06-15",
"text": "The ability affects all players, not just you."
},
{
"date": "2010-06-15",
"text": "The effect doesn’t wear off until just before your next untap step (even if an effect will cause that untap step to be skipped)."
},
{
"date": "2010-06-15",
"text": "The types of mana are white, blue, black, red, green, and colorless."
},
{
"date": "2010-06-15",
"text": "If a land produces more than one type of mana at a single time (as Boros Garrison does, for example), the land’s controller chooses which one of those types of mana is produced by the delayed triggered ability."
},
{
"date": "2010-06-15",
"text": "If a land is tapped for mana but doesn’t produce any (for example, if you tap Gaea’s Cradle for mana while you control no creatures), the delayed triggered ability won’t trigger."
}
],
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "When you set this scheme in motion, until your next turn, whenever a player taps a land for mana, that player adds one mana of any type that land produced.",
"type": "Scheme",
"types": [
"Scheme"
],
"uuid": "c5287e77-890d-41bd-acc6-7a8f1866426d"
}
}
c# json json-deserialization
Hi, have you tried dynamic json = JsonConvert.DeserializeObject and see if that works
– saj
Nov 21 '18 at 21:43
As an aside, if you're using C# 3.0 or later. yourCard
could use auto properties to save on some boilerplate, see: docs.microsoft.com/en-us/dotnet/csharp/programming-guide/…
– Evan Trimboli
Nov 21 '18 at 21:46
add a comment |
Hoping someone can catch what I'm doing wrong. CardCollection.cs just contains a List called cards, with a public methods Cards to act as getter/setter.
I should point out that I'm using Visual Studio 2017 for development, and when I inspect the string "jsonString" in debug mode using the built-in JSON Visualizer it displays properly in that window. Unfortunately JsonConvert.DeserializeObject does not properly set my CardCollection object and results in an empty list of cards
From "Form.cs"
private async void SelectFileButton_ClickAsync(object sender, EventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
dialog.Filter = "JSON Files|*.json";
dialog.Title = "Select a JSON File";
if (dialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string line = "";
string jsonString = "";
string fileName = dialog.FileName;
StringBuilder builder = new StringBuilder("");
using (StreamReader reader = File.OpenText(dialog.FileName))
{
while ((line = await reader.ReadLineAsync()) != null)
{
line = line.Replace("t", "");
line = line.Replace("n", "");
builder.Append(line);
}
}
jsonString = builder.ToString();
var settings = new JsonSerializerSettings
{
NullValueHandling = NullValueHandling.Ignore,
MissingMemberHandling = MissingMemberHandling.Ignore
};
CardCollection cardList = JsonConvert.DeserializeObject<CardCollection>(jsonString, settings);
}
}
Card.cs
public class Card
{
string colorIdentity;
string colors;
double convertedManaCost;
ForeignData foreignData;
bool isReserved;
string layout;
Legality legalities;
string loyalty;
string manaCost;
string name;
string power;
string printings;
Ruling rulings;
string subtypes;
string supertypes;
string text;
string toughness;
string type;
string types;
string uuid;
public string ColorIdentity { get => colorIdentity; set => colorIdentity = value; }
public string Colors { get => colors; set => colors = value; }
public double ConvertedManaCost { get => convertedManaCost; set => convertedManaCost = value; }
public bool IsReserved { get => isReserved; set => isReserved = value; }
public string Layout { get => layout; set => layout = value; }
public string Loyalty { get => loyalty; set => loyalty = value; }
public string ManaCost { get => manaCost; set => manaCost = value; }
public string Name { get => name; set => name = value; }
public string Power { get => power; set => power = value; }
public string Printings { get => printings; set => printings = value; }
public string Subtypes { get => subtypes; set => subtypes = value; }
public string Supertypes { get => supertypes; set => supertypes = value; }
public string Text { get => text; set => text = value; }
public string Toughness { get => toughness; set => toughness = value; }
public string Type { get => type; set => type = value; }
public string Types { get => types; set => types = value; }
public string Uuid { get => uuid; set => uuid = value; }
internal ForeignData ForeignData { get => foreignData; set => foreignData = value; }
internal Legality Legalities { get => legalities; set => legalities = value; }
internal Ruling Rulings { get => rulings; set => rulings = value; }
}
Here is sample of the JSON file
{
"1999 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "1999 World Championships Ad",
"printings": [
"WC99"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "8635dea0-985a-4c02-a02b-8b08d96fb2dd"
},
"2004 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "2004 World Championships Ad",
"printings": [
"WC04"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "cbd6a762-f3c2-4b29-a07e-f0c9c81cf1ec"
},
"A Display of My Dark Power": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "scheme",
"legalities": {},
"name": "A Display of My Dark Power",
"printings": [
"ARC"
],
"rulings": [
{
"date": "2010-06-15",
"text": "The ability affects all players, not just you."
},
{
"date": "2010-06-15",
"text": "The effect doesn’t wear off until just before your next untap step (even if an effect will cause that untap step to be skipped)."
},
{
"date": "2010-06-15",
"text": "The types of mana are white, blue, black, red, green, and colorless."
},
{
"date": "2010-06-15",
"text": "If a land produces more than one type of mana at a single time (as Boros Garrison does, for example), the land’s controller chooses which one of those types of mana is produced by the delayed triggered ability."
},
{
"date": "2010-06-15",
"text": "If a land is tapped for mana but doesn’t produce any (for example, if you tap Gaea’s Cradle for mana while you control no creatures), the delayed triggered ability won’t trigger."
}
],
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "When you set this scheme in motion, until your next turn, whenever a player taps a land for mana, that player adds one mana of any type that land produced.",
"type": "Scheme",
"types": [
"Scheme"
],
"uuid": "c5287e77-890d-41bd-acc6-7a8f1866426d"
}
}
c# json json-deserialization
Hoping someone can catch what I'm doing wrong. CardCollection.cs just contains a List called cards, with a public methods Cards to act as getter/setter.
I should point out that I'm using Visual Studio 2017 for development, and when I inspect the string "jsonString" in debug mode using the built-in JSON Visualizer it displays properly in that window. Unfortunately JsonConvert.DeserializeObject does not properly set my CardCollection object and results in an empty list of cards
From "Form.cs"
private async void SelectFileButton_ClickAsync(object sender, EventArgs e)
{
OpenFileDialog dialog = new OpenFileDialog();
dialog.Filter = "JSON Files|*.json";
dialog.Title = "Select a JSON File";
if (dialog.ShowDialog() == System.Windows.Forms.DialogResult.OK)
{
string line = "";
string jsonString = "";
string fileName = dialog.FileName;
StringBuilder builder = new StringBuilder("");
using (StreamReader reader = File.OpenText(dialog.FileName))
{
while ((line = await reader.ReadLineAsync()) != null)
{
line = line.Replace("t", "");
line = line.Replace("n", "");
builder.Append(line);
}
}
jsonString = builder.ToString();
var settings = new JsonSerializerSettings
{
NullValueHandling = NullValueHandling.Ignore,
MissingMemberHandling = MissingMemberHandling.Ignore
};
CardCollection cardList = JsonConvert.DeserializeObject<CardCollection>(jsonString, settings);
}
}
Card.cs
public class Card
{
string colorIdentity;
string colors;
double convertedManaCost;
ForeignData foreignData;
bool isReserved;
string layout;
Legality legalities;
string loyalty;
string manaCost;
string name;
string power;
string printings;
Ruling rulings;
string subtypes;
string supertypes;
string text;
string toughness;
string type;
string types;
string uuid;
public string ColorIdentity { get => colorIdentity; set => colorIdentity = value; }
public string Colors { get => colors; set => colors = value; }
public double ConvertedManaCost { get => convertedManaCost; set => convertedManaCost = value; }
public bool IsReserved { get => isReserved; set => isReserved = value; }
public string Layout { get => layout; set => layout = value; }
public string Loyalty { get => loyalty; set => loyalty = value; }
public string ManaCost { get => manaCost; set => manaCost = value; }
public string Name { get => name; set => name = value; }
public string Power { get => power; set => power = value; }
public string Printings { get => printings; set => printings = value; }
public string Subtypes { get => subtypes; set => subtypes = value; }
public string Supertypes { get => supertypes; set => supertypes = value; }
public string Text { get => text; set => text = value; }
public string Toughness { get => toughness; set => toughness = value; }
public string Type { get => type; set => type = value; }
public string Types { get => types; set => types = value; }
public string Uuid { get => uuid; set => uuid = value; }
internal ForeignData ForeignData { get => foreignData; set => foreignData = value; }
internal Legality Legalities { get => legalities; set => legalities = value; }
internal Ruling Rulings { get => rulings; set => rulings = value; }
}
Here is sample of the JSON file
{
"1999 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "1999 World Championships Ad",
"printings": [
"WC99"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "8635dea0-985a-4c02-a02b-8b08d96fb2dd"
},
"2004 World Championships Ad": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "token",
"legalities": {},
"name": "2004 World Championships Ad",
"printings": [
"WC04"
],
"rulings": ,
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "",
"type": "Card",
"types": [
"Card"
],
"uuid": "cbd6a762-f3c2-4b29-a07e-f0c9c81cf1ec"
},
"A Display of My Dark Power": {
"colorIdentity": ,
"colors": ,
"convertedManaCost": 0.0,
"foreignData": ,
"isReserved": false,
"layout": "scheme",
"legalities": {},
"name": "A Display of My Dark Power",
"printings": [
"ARC"
],
"rulings": [
{
"date": "2010-06-15",
"text": "The ability affects all players, not just you."
},
{
"date": "2010-06-15",
"text": "The effect doesn’t wear off until just before your next untap step (even if an effect will cause that untap step to be skipped)."
},
{
"date": "2010-06-15",
"text": "The types of mana are white, blue, black, red, green, and colorless."
},
{
"date": "2010-06-15",
"text": "If a land produces more than one type of mana at a single time (as Boros Garrison does, for example), the land’s controller chooses which one of those types of mana is produced by the delayed triggered ability."
},
{
"date": "2010-06-15",
"text": "If a land is tapped for mana but doesn’t produce any (for example, if you tap Gaea’s Cradle for mana while you control no creatures), the delayed triggered ability won’t trigger."
}
],
"starter": true,
"subtypes": ,
"supertypes": ,
"text": "When you set this scheme in motion, until your next turn, whenever a player taps a land for mana, that player adds one mana of any type that land produced.",
"type": "Scheme",
"types": [
"Scheme"
],
"uuid": "c5287e77-890d-41bd-acc6-7a8f1866426d"
}
}
c# json json-deserialization
c# json json-deserialization
edited Nov 21 '18 at 22:46


rene
33.7k1181108
33.7k1181108
asked Nov 21 '18 at 21:38


Ian ClaymanIan Clayman
11
11
Hi, have you tried dynamic json = JsonConvert.DeserializeObject and see if that works
– saj
Nov 21 '18 at 21:43
As an aside, if you're using C# 3.0 or later. yourCard
could use auto properties to save on some boilerplate, see: docs.microsoft.com/en-us/dotnet/csharp/programming-guide/…
– Evan Trimboli
Nov 21 '18 at 21:46
add a comment |
Hi, have you tried dynamic json = JsonConvert.DeserializeObject and see if that works
– saj
Nov 21 '18 at 21:43
As an aside, if you're using C# 3.0 or later. yourCard
could use auto properties to save on some boilerplate, see: docs.microsoft.com/en-us/dotnet/csharp/programming-guide/…
– Evan Trimboli
Nov 21 '18 at 21:46
Hi, have you tried dynamic json = JsonConvert.DeserializeObject and see if that works
– saj
Nov 21 '18 at 21:43
Hi, have you tried dynamic json = JsonConvert.DeserializeObject and see if that works
– saj
Nov 21 '18 at 21:43
As an aside, if you're using C# 3.0 or later. your
Card
could use auto properties to save on some boilerplate, see: docs.microsoft.com/en-us/dotnet/csharp/programming-guide/…– Evan Trimboli
Nov 21 '18 at 21:46
As an aside, if you're using C# 3.0 or later. your
Card
could use auto properties to save on some boilerplate, see: docs.microsoft.com/en-us/dotnet/csharp/programming-guide/…– Evan Trimboli
Nov 21 '18 at 21:46
add a comment |
2 Answers
2
active
oldest
votes
"1999 World Championships Ad": {
What you have here is an object, not an array. You should deserialize it as a dictionary:
JsonConvert.DeserializeObject<Dictionary<string, Card>>(...);
add a comment |
Your class Card
does not match your json in several ways. There are several good ways to create classes for serializing/deserializing json directly.
Method 1:
- Copy your json example containing all possible keys.
- In Visual Studio 2017. Edit->Paste Special->Paste Json as classes
This will create the classes you need to deserialize your data.
Method 2:
Use Quicktype.io. Paste you json and get the classes needed in your favorite language.
One extra problem with you class Card
is that the property names are case sensitive! ColorIdentity != colorIdentity.
If you want to keep PascalCasing in your code, but have camelCasing in your Json you need to decorate each property like this:
[JsonProperty("colorIdentity")]
public object ColorIdentity { get; set; }
Also the names of the competitions in your json are objects that are not present in your classes. If you follow method 1 or 2 you will see what I mean.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53420831%2fjsonconvert-deserializeobjectt-returns-null-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
"1999 World Championships Ad": {
What you have here is an object, not an array. You should deserialize it as a dictionary:
JsonConvert.DeserializeObject<Dictionary<string, Card>>(...);
add a comment |
"1999 World Championships Ad": {
What you have here is an object, not an array. You should deserialize it as a dictionary:
JsonConvert.DeserializeObject<Dictionary<string, Card>>(...);
add a comment |
"1999 World Championships Ad": {
What you have here is an object, not an array. You should deserialize it as a dictionary:
JsonConvert.DeserializeObject<Dictionary<string, Card>>(...);
"1999 World Championships Ad": {
What you have here is an object, not an array. You should deserialize it as a dictionary:
JsonConvert.DeserializeObject<Dictionary<string, Card>>(...);
answered Nov 21 '18 at 22:07
Xiaoy312Xiaoy312
11.4k12234
11.4k12234
add a comment |
add a comment |
Your class Card
does not match your json in several ways. There are several good ways to create classes for serializing/deserializing json directly.
Method 1:
- Copy your json example containing all possible keys.
- In Visual Studio 2017. Edit->Paste Special->Paste Json as classes
This will create the classes you need to deserialize your data.
Method 2:
Use Quicktype.io. Paste you json and get the classes needed in your favorite language.
One extra problem with you class Card
is that the property names are case sensitive! ColorIdentity != colorIdentity.
If you want to keep PascalCasing in your code, but have camelCasing in your Json you need to decorate each property like this:
[JsonProperty("colorIdentity")]
public object ColorIdentity { get; set; }
Also the names of the competitions in your json are objects that are not present in your classes. If you follow method 1 or 2 you will see what I mean.
add a comment |
Your class Card
does not match your json in several ways. There are several good ways to create classes for serializing/deserializing json directly.
Method 1:
- Copy your json example containing all possible keys.
- In Visual Studio 2017. Edit->Paste Special->Paste Json as classes
This will create the classes you need to deserialize your data.
Method 2:
Use Quicktype.io. Paste you json and get the classes needed in your favorite language.
One extra problem with you class Card
is that the property names are case sensitive! ColorIdentity != colorIdentity.
If you want to keep PascalCasing in your code, but have camelCasing in your Json you need to decorate each property like this:
[JsonProperty("colorIdentity")]
public object ColorIdentity { get; set; }
Also the names of the competitions in your json are objects that are not present in your classes. If you follow method 1 or 2 you will see what I mean.
add a comment |
Your class Card
does not match your json in several ways. There are several good ways to create classes for serializing/deserializing json directly.
Method 1:
- Copy your json example containing all possible keys.
- In Visual Studio 2017. Edit->Paste Special->Paste Json as classes
This will create the classes you need to deserialize your data.
Method 2:
Use Quicktype.io. Paste you json and get the classes needed in your favorite language.
One extra problem with you class Card
is that the property names are case sensitive! ColorIdentity != colorIdentity.
If you want to keep PascalCasing in your code, but have camelCasing in your Json you need to decorate each property like this:
[JsonProperty("colorIdentity")]
public object ColorIdentity { get; set; }
Also the names of the competitions in your json are objects that are not present in your classes. If you follow method 1 or 2 you will see what I mean.
Your class Card
does not match your json in several ways. There are several good ways to create classes for serializing/deserializing json directly.
Method 1:
- Copy your json example containing all possible keys.
- In Visual Studio 2017. Edit->Paste Special->Paste Json as classes
This will create the classes you need to deserialize your data.
Method 2:
Use Quicktype.io. Paste you json and get the classes needed in your favorite language.
One extra problem with you class Card
is that the property names are case sensitive! ColorIdentity != colorIdentity.
If you want to keep PascalCasing in your code, but have camelCasing in your Json you need to decorate each property like this:
[JsonProperty("colorIdentity")]
public object ColorIdentity { get; set; }
Also the names of the competitions in your json are objects that are not present in your classes. If you follow method 1 or 2 you will see what I mean.
edited Nov 21 '18 at 22:44


rene
33.7k1181108
33.7k1181108
answered Nov 21 '18 at 22:12


torsantorsan
16139
16139
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53420831%2fjsonconvert-deserializeobjectt-returns-null-list%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5tXOT,A7ei5FZROGIXn6fv04y,CNX4,KRTcH Xj6YY7s03YoIWZaYkaro,6iVSOoA mLA,bGZTe7
Hi, have you tried dynamic json = JsonConvert.DeserializeObject and see if that works
– saj
Nov 21 '18 at 21:43
As an aside, if you're using C# 3.0 or later. your
Card
could use auto properties to save on some boilerplate, see: docs.microsoft.com/en-us/dotnet/csharp/programming-guide/…– Evan Trimboli
Nov 21 '18 at 21:46