Download of jQuery Ajax request contents is empty
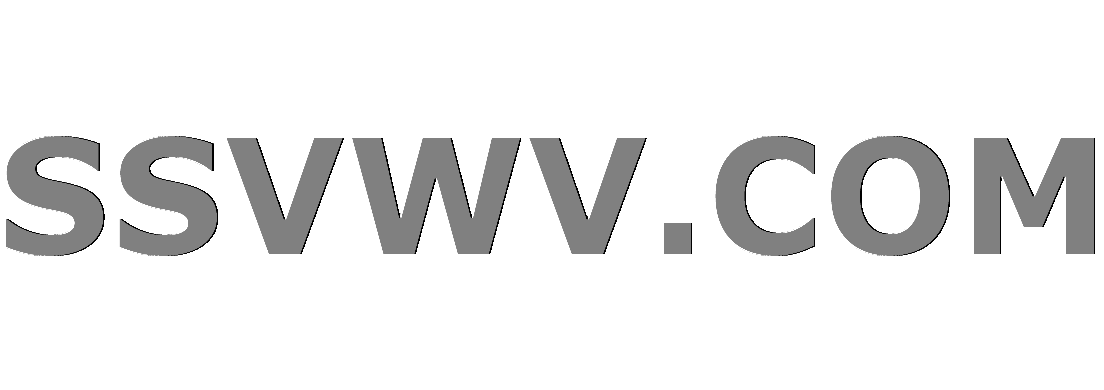
Multi tool use
I have a PHP file that returns output in PDF - Works fine if I access the file directly.
I'd like to retrieve the PDF file through AJAX.
In native Javascript, it works fine:
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
};
req.send();
But I guess I'd use jQuery to ensure cross browser compatibility (although the snippet above works in Edge, Chrome and Firefox on pc, I haven't tested it in other browsers/on other platforms)
So I tried to rewrite the function:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
url: url,
method: 'POST',
responseType: 'blob',
success: function(data)
{
var filename='test.pdf';
var blob=new Blob([data]);
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
The jQuery equivalent allows me to download a PDF file but … the PDF file is empty.
So I guess I am doing something wrong, probably in the DATA to BLOB conversion. But what? I hope somebody can see what I am doing wrong.
I've been using ages on StackOverflow, read many suggestions - but didn't find any answer. I simply can't see the forest for the trees.
javascript php jquery ajax xmlhttprequest
add a comment |
I have a PHP file that returns output in PDF - Works fine if I access the file directly.
I'd like to retrieve the PDF file through AJAX.
In native Javascript, it works fine:
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
};
req.send();
But I guess I'd use jQuery to ensure cross browser compatibility (although the snippet above works in Edge, Chrome and Firefox on pc, I haven't tested it in other browsers/on other platforms)
So I tried to rewrite the function:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
url: url,
method: 'POST',
responseType: 'blob',
success: function(data)
{
var filename='test.pdf';
var blob=new Blob([data]);
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
The jQuery equivalent allows me to download a PDF file but … the PDF file is empty.
So I guess I am doing something wrong, probably in the DATA to BLOB conversion. But what? I hope somebody can see what I am doing wrong.
I've been using ages on StackOverflow, read many suggestions - but didn't find any answer. I simply can't see the forest for the trees.
javascript php jquery ajax xmlhttprequest
add a comment |
I have a PHP file that returns output in PDF - Works fine if I access the file directly.
I'd like to retrieve the PDF file through AJAX.
In native Javascript, it works fine:
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
};
req.send();
But I guess I'd use jQuery to ensure cross browser compatibility (although the snippet above works in Edge, Chrome and Firefox on pc, I haven't tested it in other browsers/on other platforms)
So I tried to rewrite the function:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
url: url,
method: 'POST',
responseType: 'blob',
success: function(data)
{
var filename='test.pdf';
var blob=new Blob([data]);
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
The jQuery equivalent allows me to download a PDF file but … the PDF file is empty.
So I guess I am doing something wrong, probably in the DATA to BLOB conversion. But what? I hope somebody can see what I am doing wrong.
I've been using ages on StackOverflow, read many suggestions - but didn't find any answer. I simply can't see the forest for the trees.
javascript php jquery ajax xmlhttprequest
I have a PHP file that returns output in PDF - Works fine if I access the file directly.
I'd like to retrieve the PDF file through AJAX.
In native Javascript, it works fine:
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
};
req.send();
But I guess I'd use jQuery to ensure cross browser compatibility (although the snippet above works in Edge, Chrome and Firefox on pc, I haven't tested it in other browsers/on other platforms)
So I tried to rewrite the function:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
url: url,
method: 'POST',
responseType: 'blob',
success: function(data)
{
var filename='test.pdf';
var blob=new Blob([data]);
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
The jQuery equivalent allows me to download a PDF file but … the PDF file is empty.
So I guess I am doing something wrong, probably in the DATA to BLOB conversion. But what? I hope somebody can see what I am doing wrong.
I've been using ages on StackOverflow, read many suggestions - but didn't find any answer. I simply can't see the forest for the trees.
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
};
req.send();
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
};
req.send();
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
url: url,
method: 'POST',
responseType: 'blob',
success: function(data)
{
var filename='test.pdf';
var blob=new Blob([data]);
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
url: url,
method: 'POST',
responseType: 'blob',
success: function(data)
{
var filename='test.pdf';
var blob=new Blob([data]);
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
javascript php jquery ajax xmlhttprequest
javascript php jquery ajax xmlhttprequest
edited Nov 22 '18 at 22:07
miken32
24.2k95073
24.2k95073
asked Nov 22 '18 at 20:52
KairosKairos
63
63
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
As binary data is not possible to retrieve through jQuery.ajax, Native is the only way, at least for now. The following method works in Edge, Firefox, Chrome and Opera - tested on WIndows 10.
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.setAttribute("type", "hidden"); // make it hidden if needed
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
document.body.appendChild(link);
link.click();
link.remove();
var file = new File([blob], filename, { type: 'application/force-download' });
//window.open(URL.createObjectURL(file));
}
};
req.send();
add a comment |
Looking at the documentation for the jQuery.ajax()
function, we see there's no setting called responseType
, so you need to use xhrFields
to directly set a property of the XHR object. And, since you're only setting the URL and success callback, we can just use the shorter jquery.post()
function.
So the data is returned, we make a Blob
and then a URL to download it. I'm not on Windows so I can't test if that link I constructed will work as expected, but figured I'd do it the jQuery way.
var url = './api/pdftest.php?wpid=' + wpid;
$.post({
url: url,
xhrFields: {responseType: "blob"},
success: function(data) {
// don't set the MIME type to pdf or it will display
var blob = new Blob([data], {type: "application/octet-stream"});
// build a blob URL
var bloburl = window.URL.createObjectURL(blob);
// trigger download for edge
var link = $("<a>").attr({href: bloburl, download: "test.pdf"}).click();
// trigger download for other browsers
window.open(bloburl);
}
});
Tried your solution in Chrome and Firefox, and that allows me to download a PDF - but it is still an empty PDF. In Edge, it won't download at all. The reason I create a link and put a ".click()" on it, AND try to force download the file is because Firefox does not accept the "click" method, while Edge does not accept the force download method (at least not the one I used)
– Kairos
Nov 23 '18 at 7:52
Here is a live version of the two scripts: kairosplanner.com/pdftest.php
– Kairos
Nov 23 '18 at 12:49
Then I'd suggest your original download is a problem. Put in some debugging likedata.length
to ensure you're actually getting something. I tested this code and it worked fine (in Firefox.)
– miken32
Nov 23 '18 at 17:12
Ok I tried your live version. First off, saying a downloaded file is "empty" means something very specific when you're talking to a programming crowd. It means empty, as in zero bytes. Your problem is that the PDF is a blank page, and that's absolutely down to the response of the server. You'll need to debug why your server is returning a PDF without text under certain circumstances.
– miken32
Nov 23 '18 at 17:37
A simple check with the web inspector reveals that your native Javascript version, for some reason, is not sending two headers:X-Requested-With: XmlHttpRequest
andTE: Trailers
. Otherwise the two requests are identical.
– miken32
Nov 23 '18 at 17:37
|
show 7 more comments
Probably double!
This is the solution I found thanks to Hisham at Download pdf file using jquery ajax:
First, add the following plugin that can be used to the XHR V2 capabilities missing in JQuery: https://github.com/acigna/jquery-ajax-native
Then:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
dataType: 'native',
url: url,
xhrFields:
{
responseType: 'blob'
},
success: function(blob)
{
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
This seems to be working.
Note: the window.open() is to make download possible in Firefox, the link.click() method Works in Edge, Chrome and Opera
Thanks to miken32 for pointing into the right direction.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437849%2fdownload-of-jquery-ajax-request-contents-is-empty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
As binary data is not possible to retrieve through jQuery.ajax, Native is the only way, at least for now. The following method works in Edge, Firefox, Chrome and Opera - tested on WIndows 10.
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.setAttribute("type", "hidden"); // make it hidden if needed
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
document.body.appendChild(link);
link.click();
link.remove();
var file = new File([blob], filename, { type: 'application/force-download' });
//window.open(URL.createObjectURL(file));
}
};
req.send();
add a comment |
As binary data is not possible to retrieve through jQuery.ajax, Native is the only way, at least for now. The following method works in Edge, Firefox, Chrome and Opera - tested on WIndows 10.
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.setAttribute("type", "hidden"); // make it hidden if needed
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
document.body.appendChild(link);
link.click();
link.remove();
var file = new File([blob], filename, { type: 'application/force-download' });
//window.open(URL.createObjectURL(file));
}
};
req.send();
add a comment |
As binary data is not possible to retrieve through jQuery.ajax, Native is the only way, at least for now. The following method works in Edge, Firefox, Chrome and Opera - tested on WIndows 10.
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.setAttribute("type", "hidden"); // make it hidden if needed
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
document.body.appendChild(link);
link.click();
link.remove();
var file = new File([blob], filename, { type: 'application/force-download' });
//window.open(URL.createObjectURL(file));
}
};
req.send();
As binary data is not possible to retrieve through jQuery.ajax, Native is the only way, at least for now. The following method works in Edge, Firefox, Chrome and Opera - tested on WIndows 10.
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.setAttribute("type", "hidden"); // make it hidden if needed
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
document.body.appendChild(link);
link.click();
link.remove();
var file = new File([blob], filename, { type: 'application/force-download' });
//window.open(URL.createObjectURL(file));
}
};
req.send();
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.setAttribute("type", "hidden"); // make it hidden if needed
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
document.body.appendChild(link);
link.click();
link.remove();
var file = new File([blob], filename, { type: 'application/force-download' });
//window.open(URL.createObjectURL(file));
}
};
req.send();
var req = new XMLHttpRequest();
req.open("POST", "./api/pdftest.php?wpid="+wpid, true);
req.responseType = "blob";
req.onreadystatechange = function ()
{
if (req.readyState === 4 && req.status === 200)
{
var blob=req.response;
var filename = "test.pdf";
var link = document.createElement('a');
link.setAttribute("type", "hidden"); // make it hidden if needed
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
document.body.appendChild(link);
link.click();
link.remove();
var file = new File([blob], filename, { type: 'application/force-download' });
//window.open(URL.createObjectURL(file));
}
};
req.send();
answered Nov 24 '18 at 19:59
KairosKairos
63
63
add a comment |
add a comment |
Looking at the documentation for the jQuery.ajax()
function, we see there's no setting called responseType
, so you need to use xhrFields
to directly set a property of the XHR object. And, since you're only setting the URL and success callback, we can just use the shorter jquery.post()
function.
So the data is returned, we make a Blob
and then a URL to download it. I'm not on Windows so I can't test if that link I constructed will work as expected, but figured I'd do it the jQuery way.
var url = './api/pdftest.php?wpid=' + wpid;
$.post({
url: url,
xhrFields: {responseType: "blob"},
success: function(data) {
// don't set the MIME type to pdf or it will display
var blob = new Blob([data], {type: "application/octet-stream"});
// build a blob URL
var bloburl = window.URL.createObjectURL(blob);
// trigger download for edge
var link = $("<a>").attr({href: bloburl, download: "test.pdf"}).click();
// trigger download for other browsers
window.open(bloburl);
}
});
Tried your solution in Chrome and Firefox, and that allows me to download a PDF - but it is still an empty PDF. In Edge, it won't download at all. The reason I create a link and put a ".click()" on it, AND try to force download the file is because Firefox does not accept the "click" method, while Edge does not accept the force download method (at least not the one I used)
– Kairos
Nov 23 '18 at 7:52
Here is a live version of the two scripts: kairosplanner.com/pdftest.php
– Kairos
Nov 23 '18 at 12:49
Then I'd suggest your original download is a problem. Put in some debugging likedata.length
to ensure you're actually getting something. I tested this code and it worked fine (in Firefox.)
– miken32
Nov 23 '18 at 17:12
Ok I tried your live version. First off, saying a downloaded file is "empty" means something very specific when you're talking to a programming crowd. It means empty, as in zero bytes. Your problem is that the PDF is a blank page, and that's absolutely down to the response of the server. You'll need to debug why your server is returning a PDF without text under certain circumstances.
– miken32
Nov 23 '18 at 17:37
A simple check with the web inspector reveals that your native Javascript version, for some reason, is not sending two headers:X-Requested-With: XmlHttpRequest
andTE: Trailers
. Otherwise the two requests are identical.
– miken32
Nov 23 '18 at 17:37
|
show 7 more comments
Looking at the documentation for the jQuery.ajax()
function, we see there's no setting called responseType
, so you need to use xhrFields
to directly set a property of the XHR object. And, since you're only setting the URL and success callback, we can just use the shorter jquery.post()
function.
So the data is returned, we make a Blob
and then a URL to download it. I'm not on Windows so I can't test if that link I constructed will work as expected, but figured I'd do it the jQuery way.
var url = './api/pdftest.php?wpid=' + wpid;
$.post({
url: url,
xhrFields: {responseType: "blob"},
success: function(data) {
// don't set the MIME type to pdf or it will display
var blob = new Blob([data], {type: "application/octet-stream"});
// build a blob URL
var bloburl = window.URL.createObjectURL(blob);
// trigger download for edge
var link = $("<a>").attr({href: bloburl, download: "test.pdf"}).click();
// trigger download for other browsers
window.open(bloburl);
}
});
Tried your solution in Chrome and Firefox, and that allows me to download a PDF - but it is still an empty PDF. In Edge, it won't download at all. The reason I create a link and put a ".click()" on it, AND try to force download the file is because Firefox does not accept the "click" method, while Edge does not accept the force download method (at least not the one I used)
– Kairos
Nov 23 '18 at 7:52
Here is a live version of the two scripts: kairosplanner.com/pdftest.php
– Kairos
Nov 23 '18 at 12:49
Then I'd suggest your original download is a problem. Put in some debugging likedata.length
to ensure you're actually getting something. I tested this code and it worked fine (in Firefox.)
– miken32
Nov 23 '18 at 17:12
Ok I tried your live version. First off, saying a downloaded file is "empty" means something very specific when you're talking to a programming crowd. It means empty, as in zero bytes. Your problem is that the PDF is a blank page, and that's absolutely down to the response of the server. You'll need to debug why your server is returning a PDF without text under certain circumstances.
– miken32
Nov 23 '18 at 17:37
A simple check with the web inspector reveals that your native Javascript version, for some reason, is not sending two headers:X-Requested-With: XmlHttpRequest
andTE: Trailers
. Otherwise the two requests are identical.
– miken32
Nov 23 '18 at 17:37
|
show 7 more comments
Looking at the documentation for the jQuery.ajax()
function, we see there's no setting called responseType
, so you need to use xhrFields
to directly set a property of the XHR object. And, since you're only setting the URL and success callback, we can just use the shorter jquery.post()
function.
So the data is returned, we make a Blob
and then a URL to download it. I'm not on Windows so I can't test if that link I constructed will work as expected, but figured I'd do it the jQuery way.
var url = './api/pdftest.php?wpid=' + wpid;
$.post({
url: url,
xhrFields: {responseType: "blob"},
success: function(data) {
// don't set the MIME type to pdf or it will display
var blob = new Blob([data], {type: "application/octet-stream"});
// build a blob URL
var bloburl = window.URL.createObjectURL(blob);
// trigger download for edge
var link = $("<a>").attr({href: bloburl, download: "test.pdf"}).click();
// trigger download for other browsers
window.open(bloburl);
}
});
Looking at the documentation for the jQuery.ajax()
function, we see there's no setting called responseType
, so you need to use xhrFields
to directly set a property of the XHR object. And, since you're only setting the URL and success callback, we can just use the shorter jquery.post()
function.
So the data is returned, we make a Blob
and then a URL to download it. I'm not on Windows so I can't test if that link I constructed will work as expected, but figured I'd do it the jQuery way.
var url = './api/pdftest.php?wpid=' + wpid;
$.post({
url: url,
xhrFields: {responseType: "blob"},
success: function(data) {
// don't set the MIME type to pdf or it will display
var blob = new Blob([data], {type: "application/octet-stream"});
// build a blob URL
var bloburl = window.URL.createObjectURL(blob);
// trigger download for edge
var link = $("<a>").attr({href: bloburl, download: "test.pdf"}).click();
// trigger download for other browsers
window.open(bloburl);
}
});
edited Nov 25 '18 at 4:44
answered Nov 22 '18 at 22:06
miken32miken32
24.2k95073
24.2k95073
Tried your solution in Chrome and Firefox, and that allows me to download a PDF - but it is still an empty PDF. In Edge, it won't download at all. The reason I create a link and put a ".click()" on it, AND try to force download the file is because Firefox does not accept the "click" method, while Edge does not accept the force download method (at least not the one I used)
– Kairos
Nov 23 '18 at 7:52
Here is a live version of the two scripts: kairosplanner.com/pdftest.php
– Kairos
Nov 23 '18 at 12:49
Then I'd suggest your original download is a problem. Put in some debugging likedata.length
to ensure you're actually getting something. I tested this code and it worked fine (in Firefox.)
– miken32
Nov 23 '18 at 17:12
Ok I tried your live version. First off, saying a downloaded file is "empty" means something very specific when you're talking to a programming crowd. It means empty, as in zero bytes. Your problem is that the PDF is a blank page, and that's absolutely down to the response of the server. You'll need to debug why your server is returning a PDF without text under certain circumstances.
– miken32
Nov 23 '18 at 17:37
A simple check with the web inspector reveals that your native Javascript version, for some reason, is not sending two headers:X-Requested-With: XmlHttpRequest
andTE: Trailers
. Otherwise the two requests are identical.
– miken32
Nov 23 '18 at 17:37
|
show 7 more comments
Tried your solution in Chrome and Firefox, and that allows me to download a PDF - but it is still an empty PDF. In Edge, it won't download at all. The reason I create a link and put a ".click()" on it, AND try to force download the file is because Firefox does not accept the "click" method, while Edge does not accept the force download method (at least not the one I used)
– Kairos
Nov 23 '18 at 7:52
Here is a live version of the two scripts: kairosplanner.com/pdftest.php
– Kairos
Nov 23 '18 at 12:49
Then I'd suggest your original download is a problem. Put in some debugging likedata.length
to ensure you're actually getting something. I tested this code and it worked fine (in Firefox.)
– miken32
Nov 23 '18 at 17:12
Ok I tried your live version. First off, saying a downloaded file is "empty" means something very specific when you're talking to a programming crowd. It means empty, as in zero bytes. Your problem is that the PDF is a blank page, and that's absolutely down to the response of the server. You'll need to debug why your server is returning a PDF without text under certain circumstances.
– miken32
Nov 23 '18 at 17:37
A simple check with the web inspector reveals that your native Javascript version, for some reason, is not sending two headers:X-Requested-With: XmlHttpRequest
andTE: Trailers
. Otherwise the two requests are identical.
– miken32
Nov 23 '18 at 17:37
Tried your solution in Chrome and Firefox, and that allows me to download a PDF - but it is still an empty PDF. In Edge, it won't download at all. The reason I create a link and put a ".click()" on it, AND try to force download the file is because Firefox does not accept the "click" method, while Edge does not accept the force download method (at least not the one I used)
– Kairos
Nov 23 '18 at 7:52
Tried your solution in Chrome and Firefox, and that allows me to download a PDF - but it is still an empty PDF. In Edge, it won't download at all. The reason I create a link and put a ".click()" on it, AND try to force download the file is because Firefox does not accept the "click" method, while Edge does not accept the force download method (at least not the one I used)
– Kairos
Nov 23 '18 at 7:52
Here is a live version of the two scripts: kairosplanner.com/pdftest.php
– Kairos
Nov 23 '18 at 12:49
Here is a live version of the two scripts: kairosplanner.com/pdftest.php
– Kairos
Nov 23 '18 at 12:49
Then I'd suggest your original download is a problem. Put in some debugging like
data.length
to ensure you're actually getting something. I tested this code and it worked fine (in Firefox.)– miken32
Nov 23 '18 at 17:12
Then I'd suggest your original download is a problem. Put in some debugging like
data.length
to ensure you're actually getting something. I tested this code and it worked fine (in Firefox.)– miken32
Nov 23 '18 at 17:12
Ok I tried your live version. First off, saying a downloaded file is "empty" means something very specific when you're talking to a programming crowd. It means empty, as in zero bytes. Your problem is that the PDF is a blank page, and that's absolutely down to the response of the server. You'll need to debug why your server is returning a PDF without text under certain circumstances.
– miken32
Nov 23 '18 at 17:37
Ok I tried your live version. First off, saying a downloaded file is "empty" means something very specific when you're talking to a programming crowd. It means empty, as in zero bytes. Your problem is that the PDF is a blank page, and that's absolutely down to the response of the server. You'll need to debug why your server is returning a PDF without text under certain circumstances.
– miken32
Nov 23 '18 at 17:37
A simple check with the web inspector reveals that your native Javascript version, for some reason, is not sending two headers:
X-Requested-With: XmlHttpRequest
and TE: Trailers
. Otherwise the two requests are identical.– miken32
Nov 23 '18 at 17:37
A simple check with the web inspector reveals that your native Javascript version, for some reason, is not sending two headers:
X-Requested-With: XmlHttpRequest
and TE: Trailers
. Otherwise the two requests are identical.– miken32
Nov 23 '18 at 17:37
|
show 7 more comments
Probably double!
This is the solution I found thanks to Hisham at Download pdf file using jquery ajax:
First, add the following plugin that can be used to the XHR V2 capabilities missing in JQuery: https://github.com/acigna/jquery-ajax-native
Then:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
dataType: 'native',
url: url,
xhrFields:
{
responseType: 'blob'
},
success: function(blob)
{
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
This seems to be working.
Note: the window.open() is to make download possible in Firefox, the link.click() method Works in Edge, Chrome and Opera
Thanks to miken32 for pointing into the right direction.
add a comment |
Probably double!
This is the solution I found thanks to Hisham at Download pdf file using jquery ajax:
First, add the following plugin that can be used to the XHR V2 capabilities missing in JQuery: https://github.com/acigna/jquery-ajax-native
Then:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
dataType: 'native',
url: url,
xhrFields:
{
responseType: 'blob'
},
success: function(blob)
{
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
This seems to be working.
Note: the window.open() is to make download possible in Firefox, the link.click() method Works in Edge, Chrome and Opera
Thanks to miken32 for pointing into the right direction.
add a comment |
Probably double!
This is the solution I found thanks to Hisham at Download pdf file using jquery ajax:
First, add the following plugin that can be used to the XHR V2 capabilities missing in JQuery: https://github.com/acigna/jquery-ajax-native
Then:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
dataType: 'native',
url: url,
xhrFields:
{
responseType: 'blob'
},
success: function(blob)
{
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
This seems to be working.
Note: the window.open() is to make download possible in Firefox, the link.click() method Works in Edge, Chrome and Opera
Thanks to miken32 for pointing into the right direction.
Probably double!
This is the solution I found thanks to Hisham at Download pdf file using jquery ajax:
First, add the following plugin that can be used to the XHR V2 capabilities missing in JQuery: https://github.com/acigna/jquery-ajax-native
Then:
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
dataType: 'native',
url: url,
xhrFields:
{
responseType: 'blob'
},
success: function(blob)
{
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
This seems to be working.
Note: the window.open() is to make download possible in Firefox, the link.click() method Works in Edge, Chrome and Opera
Thanks to miken32 for pointing into the right direction.
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
dataType: 'native',
url: url,
xhrFields:
{
responseType: 'blob'
},
success: function(blob)
{
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
url='./api/pdftest.php?wpid='+wpid;
$.ajax(
{
dataType: 'native',
url: url,
xhrFields:
{
responseType: 'blob'
},
success: function(blob)
{
var filename = "test.pdf";
var link = document.createElement('a');
link.href = window.URL.createObjectURL(blob);
link.download = "test.pdf";
link.click();
var file = new File([blob], filename, { type: 'application/force-download' });
window.open(URL.createObjectURL(file));
}
});
answered Nov 25 '18 at 10:21
KairosKairos
63
63
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437849%2fdownload-of-jquery-ajax-request-contents-is-empty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
tstyOhYO0Az6S 2dbT9azNYNBp6RuqApq2,DZ,4 i6,kjC,G0agw1,ffC,6 0ihvDcb Hw4OrxR