Is it possible to output the numpad's pattern using loops?
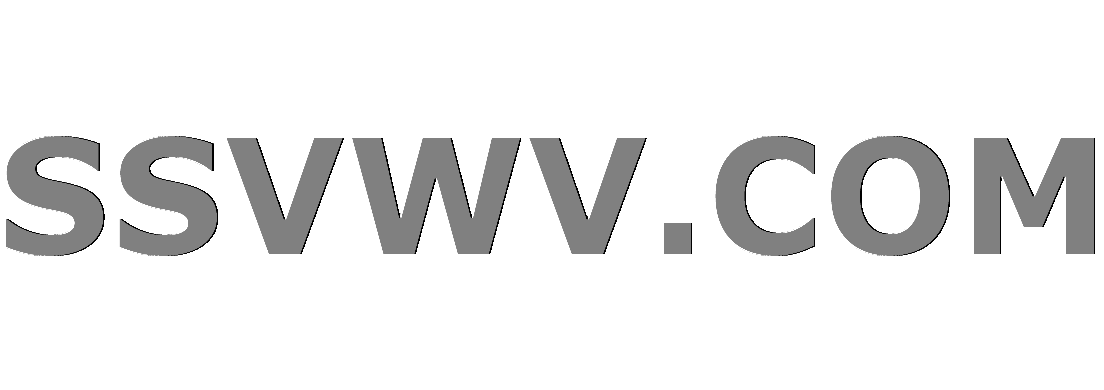
Multi tool use
I just started learning C (coding in general) a few months ago. Earlier today when I was in class, I looked at the numpad and wondered whether I would be able to replicate the pattern using nested loops in C.
7 8 9
4 5 6
1 2 3 // This pattern.
I tried to do it myself for a bit, using for loops primarily. Thanks for any help.
#include<stdio.h>
int main()
{
int row, col, i;
printf("Up to what integer? ");
scanf("%d", &row);
for(i=1; i<=row; i++)
{
for(col=1; col<=10; col++)
{
printf(" %d ", i*col);
}
printf("n");
}
}
Edit: Added supplementary code. Something like this, except to print 3 rows and 3 columns.
c design-patterns printing nested-loops
add a comment |
I just started learning C (coding in general) a few months ago. Earlier today when I was in class, I looked at the numpad and wondered whether I would be able to replicate the pattern using nested loops in C.
7 8 9
4 5 6
1 2 3 // This pattern.
I tried to do it myself for a bit, using for loops primarily. Thanks for any help.
#include<stdio.h>
int main()
{
int row, col, i;
printf("Up to what integer? ");
scanf("%d", &row);
for(i=1; i<=row; i++)
{
for(col=1; col<=10; col++)
{
printf(" %d ", i*col);
}
printf("n");
}
}
Edit: Added supplementary code. Something like this, except to print 3 rows and 3 columns.
c design-patterns printing nested-loops
Sure it is possible. What have you tried so far and were are you stuck?
– Gerhardh
Nov 22 '18 at 11:12
I've tried with integers i, x, y; with for loops i =1. I've tried with the base number being 9 and 1.
– partynextdoor18
Nov 22 '18 at 11:16
1
Please show a MCVE. Without code its pointless to guess that your problem is. Also include the wrong output you got.
– Gerhardh
Nov 22 '18 at 11:17
add a comment |
I just started learning C (coding in general) a few months ago. Earlier today when I was in class, I looked at the numpad and wondered whether I would be able to replicate the pattern using nested loops in C.
7 8 9
4 5 6
1 2 3 // This pattern.
I tried to do it myself for a bit, using for loops primarily. Thanks for any help.
#include<stdio.h>
int main()
{
int row, col, i;
printf("Up to what integer? ");
scanf("%d", &row);
for(i=1; i<=row; i++)
{
for(col=1; col<=10; col++)
{
printf(" %d ", i*col);
}
printf("n");
}
}
Edit: Added supplementary code. Something like this, except to print 3 rows and 3 columns.
c design-patterns printing nested-loops
I just started learning C (coding in general) a few months ago. Earlier today when I was in class, I looked at the numpad and wondered whether I would be able to replicate the pattern using nested loops in C.
7 8 9
4 5 6
1 2 3 // This pattern.
I tried to do it myself for a bit, using for loops primarily. Thanks for any help.
#include<stdio.h>
int main()
{
int row, col, i;
printf("Up to what integer? ");
scanf("%d", &row);
for(i=1; i<=row; i++)
{
for(col=1; col<=10; col++)
{
printf(" %d ", i*col);
}
printf("n");
}
}
Edit: Added supplementary code. Something like this, except to print 3 rows and 3 columns.
c design-patterns printing nested-loops
c design-patterns printing nested-loops
edited Nov 22 '18 at 11:58
partynextdoor18
asked Nov 22 '18 at 11:05
partynextdoor18partynextdoor18
175
175
Sure it is possible. What have you tried so far and were are you stuck?
– Gerhardh
Nov 22 '18 at 11:12
I've tried with integers i, x, y; with for loops i =1. I've tried with the base number being 9 and 1.
– partynextdoor18
Nov 22 '18 at 11:16
1
Please show a MCVE. Without code its pointless to guess that your problem is. Also include the wrong output you got.
– Gerhardh
Nov 22 '18 at 11:17
add a comment |
Sure it is possible. What have you tried so far and were are you stuck?
– Gerhardh
Nov 22 '18 at 11:12
I've tried with integers i, x, y; with for loops i =1. I've tried with the base number being 9 and 1.
– partynextdoor18
Nov 22 '18 at 11:16
1
Please show a MCVE. Without code its pointless to guess that your problem is. Also include the wrong output you got.
– Gerhardh
Nov 22 '18 at 11:17
Sure it is possible. What have you tried so far and were are you stuck?
– Gerhardh
Nov 22 '18 at 11:12
Sure it is possible. What have you tried so far and were are you stuck?
– Gerhardh
Nov 22 '18 at 11:12
I've tried with integers i, x, y; with for loops i =1. I've tried with the base number being 9 and 1.
– partynextdoor18
Nov 22 '18 at 11:16
I've tried with integers i, x, y; with for loops i =1. I've tried with the base number being 9 and 1.
– partynextdoor18
Nov 22 '18 at 11:16
1
1
Please show a MCVE. Without code its pointless to guess that your problem is. Also include the wrong output you got.
– Gerhardh
Nov 22 '18 at 11:17
Please show a MCVE. Without code its pointless to guess that your problem is. Also include the wrong output you got.
– Gerhardh
Nov 22 '18 at 11:17
add a comment |
2 Answers
2
active
oldest
votes
The numpad pattern has the equation 3*i + j
with i
going from 2
to 0
and j
going from 1
to 3
.
So use these values as upper and lower limits of i
and j
in nested for
loops.
#include <stdio.h>
int main(){
for(int i = 2; i >= 0; i--){
for(int j = 1; j <= 3; j++)
printf("%d ", 3 * i + j);
printf("n");
}
return 0;
}
See it live here.
add a comment |
Here's how you can do it:
for(int i = 0; i < 3; ++i){
for(int j = 3; j > 0; --j)
printf("%d ", (10 - j) - i * 3);
printf("n");
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53429560%2fis-it-possible-to-output-the-numpads-pattern-using-loops%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The numpad pattern has the equation 3*i + j
with i
going from 2
to 0
and j
going from 1
to 3
.
So use these values as upper and lower limits of i
and j
in nested for
loops.
#include <stdio.h>
int main(){
for(int i = 2; i >= 0; i--){
for(int j = 1; j <= 3; j++)
printf("%d ", 3 * i + j);
printf("n");
}
return 0;
}
See it live here.
add a comment |
The numpad pattern has the equation 3*i + j
with i
going from 2
to 0
and j
going from 1
to 3
.
So use these values as upper and lower limits of i
and j
in nested for
loops.
#include <stdio.h>
int main(){
for(int i = 2; i >= 0; i--){
for(int j = 1; j <= 3; j++)
printf("%d ", 3 * i + j);
printf("n");
}
return 0;
}
See it live here.
add a comment |
The numpad pattern has the equation 3*i + j
with i
going from 2
to 0
and j
going from 1
to 3
.
So use these values as upper and lower limits of i
and j
in nested for
loops.
#include <stdio.h>
int main(){
for(int i = 2; i >= 0; i--){
for(int j = 1; j <= 3; j++)
printf("%d ", 3 * i + j);
printf("n");
}
return 0;
}
See it live here.
The numpad pattern has the equation 3*i + j
with i
going from 2
to 0
and j
going from 1
to 3
.
So use these values as upper and lower limits of i
and j
in nested for
loops.
#include <stdio.h>
int main(){
for(int i = 2; i >= 0; i--){
for(int j = 1; j <= 3; j++)
printf("%d ", 3 * i + j);
printf("n");
}
return 0;
}
See it live here.
edited Nov 22 '18 at 12:04
answered Nov 22 '18 at 11:57
P.WP.W
16.8k41555
16.8k41555
add a comment |
add a comment |
Here's how you can do it:
for(int i = 0; i < 3; ++i){
for(int j = 3; j > 0; --j)
printf("%d ", (10 - j) - i * 3);
printf("n");
}
add a comment |
Here's how you can do it:
for(int i = 0; i < 3; ++i){
for(int j = 3; j > 0; --j)
printf("%d ", (10 - j) - i * 3);
printf("n");
}
add a comment |
Here's how you can do it:
for(int i = 0; i < 3; ++i){
for(int j = 3; j > 0; --j)
printf("%d ", (10 - j) - i * 3);
printf("n");
}
Here's how you can do it:
for(int i = 0; i < 3; ++i){
for(int j = 3; j > 0; --j)
printf("%d ", (10 - j) - i * 3);
printf("n");
}
answered Nov 22 '18 at 11:38


sstefansstefan
336311
336311
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53429560%2fis-it-possible-to-output-the-numpads-pattern-using-loops%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ERe6Ge3JwcZBnYQo xTW1WpcN 6,C0vXgF0Z
Sure it is possible. What have you tried so far and were are you stuck?
– Gerhardh
Nov 22 '18 at 11:12
I've tried with integers i, x, y; with for loops i =1. I've tried with the base number being 9 and 1.
– partynextdoor18
Nov 22 '18 at 11:16
1
Please show a MCVE. Without code its pointless to guess that your problem is. Also include the wrong output you got.
– Gerhardh
Nov 22 '18 at 11:17