Hibernate integrity constraint violation: NOT NULL check constraint: For onetoOne Mapping using spring boot...
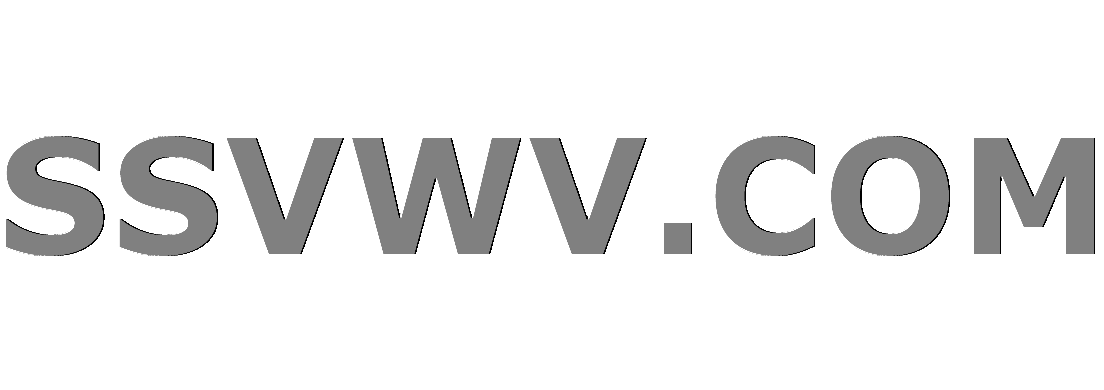
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am trying to create onetoone mapping between two tables where the parent key primary key acts as the primary key for child as well. While trying to save parent I am getting the following error.
Please find the below console log, model classes and service class used for the same. Can someone pls help to resolve the error.
Basically want to transfer the order id from order class to order id under compensation using crud repo.
Parent class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="ORDERS")
public class Order implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@Column(name="ORDER_ID")
private String orderId;
@Column(name="ACCESS_ID")
private String accessId;
@OneToOne(cascade=CascadeType.ALL,mappedBy="order",fetch=FetchType.EAGER)
private Compensation compensation;
//getters & setters
}
Child Class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.MapsId;
import javax.persistence.NamedQuery;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
/*@Id
@Column(name="ORDER_ID")
private String orderId;*/
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
//bi-directional one-to-one association to Order
@Id
@OneToOne(cascade=CascadeType.ALL)
@JoinColumn(name="ORDER_ID")
private Order order;
}
Service class:
package com.sample.service;
import javax.ws.rs.core.Response;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.newModel.Order;
@Component
public class MobilityServiceImpl implements MobilityService {
@Autowired
private MobilityRepository mobilityRepo;
@Override
public Response getOrderDetails(String orderId) {
Order orderDetails=mobilityRepo.findByOrderId(orderId);
return Response.ok(orderDetails).build();
}
@Override
public Response saveOrderDetails(Order orderDetails) {
orderDetails.getCompensation().setOrder(orderDetails);
Order orderResponse =mobilityRepo.save(orderDetails);
String resp=orderResponse.getOrderId()+" is Success";
return Response.ok(resp).build();
}
}
Console log:
Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id2_0_0_, compensati1_.channel_dealer_code as channel_1_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=?
Hibernate: select compensati0_.order_id as order_id2_0_0_, compensati0_.channel_dealer_code as channel_1_0_0_ from compensation compensati0_ where compensati0_.order_id=?
Hibernate: insert into orders (access_id, order_id) values (?, ?)
Hibernate: insert into compensation (channel_dealer_code, order_id) values (?, ?)
2018-11-23 16:13:53.210 WARN 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : SQL Error: -10, SQLState: 23502
2018-11-23 16:13:53.211 ERROR 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
2018-11-23 16:13:53.214 ERROR 17532 --- [nio-8080-exec-1] o.h.i.ExceptionMapperStandardImpl : HHH000346: Error during managed flush [org.hibernate.exception.ConstraintViolationException: could not execute statement]
2018-11-23 16:13:53.244 ERROR 17532 --- [nio-8080-exec-1] o.a.c.c.C.[.[.[/].[dispatcherServlet] : Servlet.service() for servlet [dispatcherServlet] in context with path threw exception [Request processing failed; nested exception is org.springframework.dao.DataIntegrityViolationException: could not execute statement; SQL [n/a]; constraint [null]; nested exception is org.hibernate.exception.ConstraintViolationException: could not execute statement] with root cause
org.hsqldb.HsqlException: integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
JSON Request:
{
"orderId": "1006730",
"accessId": "1810_CRU",
"compensation": {
"channelDealerCode": "ABCD"
}
}
java hibernate spring-boot jpa spring-data-jpa
add a comment |
I am trying to create onetoone mapping between two tables where the parent key primary key acts as the primary key for child as well. While trying to save parent I am getting the following error.
Please find the below console log, model classes and service class used for the same. Can someone pls help to resolve the error.
Basically want to transfer the order id from order class to order id under compensation using crud repo.
Parent class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="ORDERS")
public class Order implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@Column(name="ORDER_ID")
private String orderId;
@Column(name="ACCESS_ID")
private String accessId;
@OneToOne(cascade=CascadeType.ALL,mappedBy="order",fetch=FetchType.EAGER)
private Compensation compensation;
//getters & setters
}
Child Class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.MapsId;
import javax.persistence.NamedQuery;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
/*@Id
@Column(name="ORDER_ID")
private String orderId;*/
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
//bi-directional one-to-one association to Order
@Id
@OneToOne(cascade=CascadeType.ALL)
@JoinColumn(name="ORDER_ID")
private Order order;
}
Service class:
package com.sample.service;
import javax.ws.rs.core.Response;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.newModel.Order;
@Component
public class MobilityServiceImpl implements MobilityService {
@Autowired
private MobilityRepository mobilityRepo;
@Override
public Response getOrderDetails(String orderId) {
Order orderDetails=mobilityRepo.findByOrderId(orderId);
return Response.ok(orderDetails).build();
}
@Override
public Response saveOrderDetails(Order orderDetails) {
orderDetails.getCompensation().setOrder(orderDetails);
Order orderResponse =mobilityRepo.save(orderDetails);
String resp=orderResponse.getOrderId()+" is Success";
return Response.ok(resp).build();
}
}
Console log:
Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id2_0_0_, compensati1_.channel_dealer_code as channel_1_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=?
Hibernate: select compensati0_.order_id as order_id2_0_0_, compensati0_.channel_dealer_code as channel_1_0_0_ from compensation compensati0_ where compensati0_.order_id=?
Hibernate: insert into orders (access_id, order_id) values (?, ?)
Hibernate: insert into compensation (channel_dealer_code, order_id) values (?, ?)
2018-11-23 16:13:53.210 WARN 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : SQL Error: -10, SQLState: 23502
2018-11-23 16:13:53.211 ERROR 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
2018-11-23 16:13:53.214 ERROR 17532 --- [nio-8080-exec-1] o.h.i.ExceptionMapperStandardImpl : HHH000346: Error during managed flush [org.hibernate.exception.ConstraintViolationException: could not execute statement]
2018-11-23 16:13:53.244 ERROR 17532 --- [nio-8080-exec-1] o.a.c.c.C.[.[.[/].[dispatcherServlet] : Servlet.service() for servlet [dispatcherServlet] in context with path threw exception [Request processing failed; nested exception is org.springframework.dao.DataIntegrityViolationException: could not execute statement; SQL [n/a]; constraint [null]; nested exception is org.hibernate.exception.ConstraintViolationException: could not execute statement] with root cause
org.hsqldb.HsqlException: integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
JSON Request:
{
"orderId": "1006730",
"accessId": "1810_CRU",
"compensation": {
"channelDealerCode": "ABCD"
}
}
java hibernate spring-boot jpa spring-data-jpa
add a comment |
I am trying to create onetoone mapping between two tables where the parent key primary key acts as the primary key for child as well. While trying to save parent I am getting the following error.
Please find the below console log, model classes and service class used for the same. Can someone pls help to resolve the error.
Basically want to transfer the order id from order class to order id under compensation using crud repo.
Parent class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="ORDERS")
public class Order implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@Column(name="ORDER_ID")
private String orderId;
@Column(name="ACCESS_ID")
private String accessId;
@OneToOne(cascade=CascadeType.ALL,mappedBy="order",fetch=FetchType.EAGER)
private Compensation compensation;
//getters & setters
}
Child Class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.MapsId;
import javax.persistence.NamedQuery;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
/*@Id
@Column(name="ORDER_ID")
private String orderId;*/
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
//bi-directional one-to-one association to Order
@Id
@OneToOne(cascade=CascadeType.ALL)
@JoinColumn(name="ORDER_ID")
private Order order;
}
Service class:
package com.sample.service;
import javax.ws.rs.core.Response;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.newModel.Order;
@Component
public class MobilityServiceImpl implements MobilityService {
@Autowired
private MobilityRepository mobilityRepo;
@Override
public Response getOrderDetails(String orderId) {
Order orderDetails=mobilityRepo.findByOrderId(orderId);
return Response.ok(orderDetails).build();
}
@Override
public Response saveOrderDetails(Order orderDetails) {
orderDetails.getCompensation().setOrder(orderDetails);
Order orderResponse =mobilityRepo.save(orderDetails);
String resp=orderResponse.getOrderId()+" is Success";
return Response.ok(resp).build();
}
}
Console log:
Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id2_0_0_, compensati1_.channel_dealer_code as channel_1_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=?
Hibernate: select compensati0_.order_id as order_id2_0_0_, compensati0_.channel_dealer_code as channel_1_0_0_ from compensation compensati0_ where compensati0_.order_id=?
Hibernate: insert into orders (access_id, order_id) values (?, ?)
Hibernate: insert into compensation (channel_dealer_code, order_id) values (?, ?)
2018-11-23 16:13:53.210 WARN 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : SQL Error: -10, SQLState: 23502
2018-11-23 16:13:53.211 ERROR 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
2018-11-23 16:13:53.214 ERROR 17532 --- [nio-8080-exec-1] o.h.i.ExceptionMapperStandardImpl : HHH000346: Error during managed flush [org.hibernate.exception.ConstraintViolationException: could not execute statement]
2018-11-23 16:13:53.244 ERROR 17532 --- [nio-8080-exec-1] o.a.c.c.C.[.[.[/].[dispatcherServlet] : Servlet.service() for servlet [dispatcherServlet] in context with path threw exception [Request processing failed; nested exception is org.springframework.dao.DataIntegrityViolationException: could not execute statement; SQL [n/a]; constraint [null]; nested exception is org.hibernate.exception.ConstraintViolationException: could not execute statement] with root cause
org.hsqldb.HsqlException: integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
JSON Request:
{
"orderId": "1006730",
"accessId": "1810_CRU",
"compensation": {
"channelDealerCode": "ABCD"
}
}
java hibernate spring-boot jpa spring-data-jpa
I am trying to create onetoone mapping between two tables where the parent key primary key acts as the primary key for child as well. While trying to save parent I am getting the following error.
Please find the below console log, model classes and service class used for the same. Can someone pls help to resolve the error.
Basically want to transfer the order id from order class to order id under compensation using crud repo.
Parent class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.FetchType;
import javax.persistence.Id;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="ORDERS")
public class Order implements Serializable {
private static final long serialVersionUID = 1L;
@Id
@Column(name="ORDER_ID")
private String orderId;
@Column(name="ACCESS_ID")
private String accessId;
@OneToOne(cascade=CascadeType.ALL,mappedBy="order",fetch=FetchType.EAGER)
private Compensation compensation;
//getters & setters
}
Child Class:
package com.newModel;
import java.io.Serializable;
import javax.persistence.CascadeType;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.Id;
import javax.persistence.JoinColumn;
import javax.persistence.MapsId;
import javax.persistence.NamedQuery;
import javax.persistence.OneToOne;
import javax.persistence.Table;
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
/*@Id
@Column(name="ORDER_ID")
private String orderId;*/
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
//bi-directional one-to-one association to Order
@Id
@OneToOne(cascade=CascadeType.ALL)
@JoinColumn(name="ORDER_ID")
private Order order;
}
Service class:
package com.sample.service;
import javax.ws.rs.core.Response;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import com.newModel.Order;
@Component
public class MobilityServiceImpl implements MobilityService {
@Autowired
private MobilityRepository mobilityRepo;
@Override
public Response getOrderDetails(String orderId) {
Order orderDetails=mobilityRepo.findByOrderId(orderId);
return Response.ok(orderDetails).build();
}
@Override
public Response saveOrderDetails(Order orderDetails) {
orderDetails.getCompensation().setOrder(orderDetails);
Order orderResponse =mobilityRepo.save(orderDetails);
String resp=orderResponse.getOrderId()+" is Success";
return Response.ok(resp).build();
}
}
Console log:
Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id2_0_0_, compensati1_.channel_dealer_code as channel_1_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=?
Hibernate: select compensati0_.order_id as order_id2_0_0_, compensati0_.channel_dealer_code as channel_1_0_0_ from compensation compensati0_ where compensati0_.order_id=?
Hibernate: insert into orders (access_id, order_id) values (?, ?)
Hibernate: insert into compensation (channel_dealer_code, order_id) values (?, ?)
2018-11-23 16:13:53.210 WARN 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : SQL Error: -10, SQLState: 23502
2018-11-23 16:13:53.211 ERROR 17532 --- [nio-8080-exec-1] o.h.engine.jdbc.spi.SqlExceptionHelper : integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
2018-11-23 16:13:53.214 ERROR 17532 --- [nio-8080-exec-1] o.h.i.ExceptionMapperStandardImpl : HHH000346: Error during managed flush [org.hibernate.exception.ConstraintViolationException: could not execute statement]
2018-11-23 16:13:53.244 ERROR 17532 --- [nio-8080-exec-1] o.a.c.c.C.[.[.[/].[dispatcherServlet] : Servlet.service() for servlet [dispatcherServlet] in context with path threw exception [Request processing failed; nested exception is org.springframework.dao.DataIntegrityViolationException: could not execute statement; SQL [n/a]; constraint [null]; nested exception is org.hibernate.exception.ConstraintViolationException: could not execute statement] with root cause
org.hsqldb.HsqlException: integrity constraint violation: NOT NULL check constraint; SYS_CT_10118 table: COMPENSATION column: ORDER_ID
JSON Request:
{
"orderId": "1006730",
"accessId": "1810_CRU",
"compensation": {
"channelDealerCode": "ABCD"
}
}
java hibernate spring-boot jpa spring-data-jpa
java hibernate spring-boot jpa spring-data-jpa
asked Nov 23 '18 at 14:52
user3212324user3212324
53315
53315
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
In yout Compensation
entity you still need to have id
and Order
in separate properties and use MapsId to have the same id
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private String id;
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
@OneToOne(fetch = FetchType.LAZY)
@MapsId
private Order order;
}
Thanks. If I provide like this I am getting exception like org.hibernate.exception.SQLGrammarException: could not extract ResultSet. If I use join column (name="ORDER_ID") I am getting following error. attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 16:44
This means a query has been executed, can you log the query?
– ValerioMC
Nov 23 '18 at 18:28
pls find the below console details.Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id1_0_0_, compensati1_.channel_dealer_code as channel_2_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=? 2018-11-23 14:14:23.268 ERROR 20112 --- [nio-8080-exec-2] o.a.c.c.C.[.[.[/].[dispatcherServlet] [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 18:56
because you are storing Compensation in the same transaction when you have created an Order. Simpler solution is to keep your rest api as it is and have two transaction. Transaction one: store an order Transaction two: get the order and set the compensation. this is just an example to make you understand the problem, then you can find be best solution based on your use case
– ValerioMC
Nov 25 '18 at 8:47
thats right i am trying to store compensation in same order transaction.. is there any restrictions for onetoone mapping since i am able to create transactions for other models using onetomany transactions...
– user3212324
Nov 25 '18 at 15:35
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448843%2fhibernate-integrity-constraint-violation-not-null-check-constraint-for-onetoon%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
In yout Compensation
entity you still need to have id
and Order
in separate properties and use MapsId to have the same id
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private String id;
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
@OneToOne(fetch = FetchType.LAZY)
@MapsId
private Order order;
}
Thanks. If I provide like this I am getting exception like org.hibernate.exception.SQLGrammarException: could not extract ResultSet. If I use join column (name="ORDER_ID") I am getting following error. attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 16:44
This means a query has been executed, can you log the query?
– ValerioMC
Nov 23 '18 at 18:28
pls find the below console details.Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id1_0_0_, compensati1_.channel_dealer_code as channel_2_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=? 2018-11-23 14:14:23.268 ERROR 20112 --- [nio-8080-exec-2] o.a.c.c.C.[.[.[/].[dispatcherServlet] [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 18:56
because you are storing Compensation in the same transaction when you have created an Order. Simpler solution is to keep your rest api as it is and have two transaction. Transaction one: store an order Transaction two: get the order and set the compensation. this is just an example to make you understand the problem, then you can find be best solution based on your use case
– ValerioMC
Nov 25 '18 at 8:47
thats right i am trying to store compensation in same order transaction.. is there any restrictions for onetoone mapping since i am able to create transactions for other models using onetomany transactions...
– user3212324
Nov 25 '18 at 15:35
|
show 1 more comment
In yout Compensation
entity you still need to have id
and Order
in separate properties and use MapsId to have the same id
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private String id;
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
@OneToOne(fetch = FetchType.LAZY)
@MapsId
private Order order;
}
Thanks. If I provide like this I am getting exception like org.hibernate.exception.SQLGrammarException: could not extract ResultSet. If I use join column (name="ORDER_ID") I am getting following error. attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 16:44
This means a query has been executed, can you log the query?
– ValerioMC
Nov 23 '18 at 18:28
pls find the below console details.Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id1_0_0_, compensati1_.channel_dealer_code as channel_2_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=? 2018-11-23 14:14:23.268 ERROR 20112 --- [nio-8080-exec-2] o.a.c.c.C.[.[.[/].[dispatcherServlet] [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 18:56
because you are storing Compensation in the same transaction when you have created an Order. Simpler solution is to keep your rest api as it is and have two transaction. Transaction one: store an order Transaction two: get the order and set the compensation. this is just an example to make you understand the problem, then you can find be best solution based on your use case
– ValerioMC
Nov 25 '18 at 8:47
thats right i am trying to store compensation in same order transaction.. is there any restrictions for onetoone mapping since i am able to create transactions for other models using onetomany transactions...
– user3212324
Nov 25 '18 at 15:35
|
show 1 more comment
In yout Compensation
entity you still need to have id
and Order
in separate properties and use MapsId to have the same id
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private String id;
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
@OneToOne(fetch = FetchType.LAZY)
@MapsId
private Order order;
}
In yout Compensation
entity you still need to have id
and Order
in separate properties and use MapsId to have the same id
@Entity
@Table(name="COMPENSATION")
@NamedQuery(name="Compensation.findAll", query="SELECT o FROM Compensation o")
public class Compensation implements Serializable {
private static final long serialVersionUID = 1L;
@Id
private String id;
@Column(name="CHANNEL_DEALER_CODE")
private String channelDealerCode;
@OneToOne(fetch = FetchType.LAZY)
@MapsId
private Order order;
}
answered Nov 23 '18 at 15:35
ValerioMCValerioMC
1,231511
1,231511
Thanks. If I provide like this I am getting exception like org.hibernate.exception.SQLGrammarException: could not extract ResultSet. If I use join column (name="ORDER_ID") I am getting following error. attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 16:44
This means a query has been executed, can you log the query?
– ValerioMC
Nov 23 '18 at 18:28
pls find the below console details.Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id1_0_0_, compensati1_.channel_dealer_code as channel_2_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=? 2018-11-23 14:14:23.268 ERROR 20112 --- [nio-8080-exec-2] o.a.c.c.C.[.[.[/].[dispatcherServlet] [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 18:56
because you are storing Compensation in the same transaction when you have created an Order. Simpler solution is to keep your rest api as it is and have two transaction. Transaction one: store an order Transaction two: get the order and set the compensation. this is just an example to make you understand the problem, then you can find be best solution based on your use case
– ValerioMC
Nov 25 '18 at 8:47
thats right i am trying to store compensation in same order transaction.. is there any restrictions for onetoone mapping since i am able to create transactions for other models using onetomany transactions...
– user3212324
Nov 25 '18 at 15:35
|
show 1 more comment
Thanks. If I provide like this I am getting exception like org.hibernate.exception.SQLGrammarException: could not extract ResultSet. If I use join column (name="ORDER_ID") I am getting following error. attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 16:44
This means a query has been executed, can you log the query?
– ValerioMC
Nov 23 '18 at 18:28
pls find the below console details.Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id1_0_0_, compensati1_.channel_dealer_code as channel_2_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=? 2018-11-23 14:14:23.268 ERROR 20112 --- [nio-8080-exec-2] o.a.c.c.C.[.[.[/].[dispatcherServlet] [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 18:56
because you are storing Compensation in the same transaction when you have created an Order. Simpler solution is to keep your rest api as it is and have two transaction. Transaction one: store an order Transaction two: get the order and set the compensation. this is just an example to make you understand the problem, then you can find be best solution based on your use case
– ValerioMC
Nov 25 '18 at 8:47
thats right i am trying to store compensation in same order transaction.. is there any restrictions for onetoone mapping since i am able to create transactions for other models using onetomany transactions...
– user3212324
Nov 25 '18 at 15:35
Thanks. If I provide like this I am getting exception like org.hibernate.exception.SQLGrammarException: could not extract ResultSet. If I use join column (name="ORDER_ID") I am getting following error. attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 16:44
Thanks. If I provide like this I am getting exception like org.hibernate.exception.SQLGrammarException: could not extract ResultSet. If I use join column (name="ORDER_ID") I am getting following error. attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 16:44
This means a query has been executed, can you log the query?
– ValerioMC
Nov 23 '18 at 18:28
This means a query has been executed, can you log the query?
– ValerioMC
Nov 23 '18 at 18:28
pls find the below console details.Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id1_0_0_, compensati1_.channel_dealer_code as channel_2_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=? 2018-11-23 14:14:23.268 ERROR 20112 --- [nio-8080-exec-2] o.a.c.c.C.[.[.[/].[dispatcherServlet] [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 18:56
pls find the below console details.Hibernate: select order0_.order_id as order_id1_1_1_, order0_.access_id as access_i2_1_1_, compensati1_.order_id as order_id1_0_0_, compensati1_.channel_dealer_code as channel_2_0_0_ from orders order0_ left outer join compensation compensati1_ on order0_.order_id=compensati1_.order_id where order0_.order_id=? 2018-11-23 14:14:23.268 ERROR 20112 --- [nio-8080-exec-2] o.a.c.c.C.[.[.[/].[dispatcherServlet] [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: attempted to assign id from null one-to-one property
– user3212324
Nov 23 '18 at 18:56
because you are storing Compensation in the same transaction when you have created an Order. Simpler solution is to keep your rest api as it is and have two transaction. Transaction one: store an order Transaction two: get the order and set the compensation. this is just an example to make you understand the problem, then you can find be best solution based on your use case
– ValerioMC
Nov 25 '18 at 8:47
because you are storing Compensation in the same transaction when you have created an Order. Simpler solution is to keep your rest api as it is and have two transaction. Transaction one: store an order Transaction two: get the order and set the compensation. this is just an example to make you understand the problem, then you can find be best solution based on your use case
– ValerioMC
Nov 25 '18 at 8:47
thats right i am trying to store compensation in same order transaction.. is there any restrictions for onetoone mapping since i am able to create transactions for other models using onetomany transactions...
– user3212324
Nov 25 '18 at 15:35
thats right i am trying to store compensation in same order transaction.. is there any restrictions for onetoone mapping since i am able to create transactions for other models using onetomany transactions...
– user3212324
Nov 25 '18 at 15:35
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53448843%2fhibernate-integrity-constraint-violation-not-null-check-constraint-for-onetoon%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7GKurc,SCCLp,SpU7oozC5,ju67flsT3L fCOduPxZSV,DU 9GSUkqaMVjf6K,uT BwOjF7jJit GZ1OR6 cFlGcS048M4a05cgd