Java Thread Sockets (PrintWriter & BufferedReader)
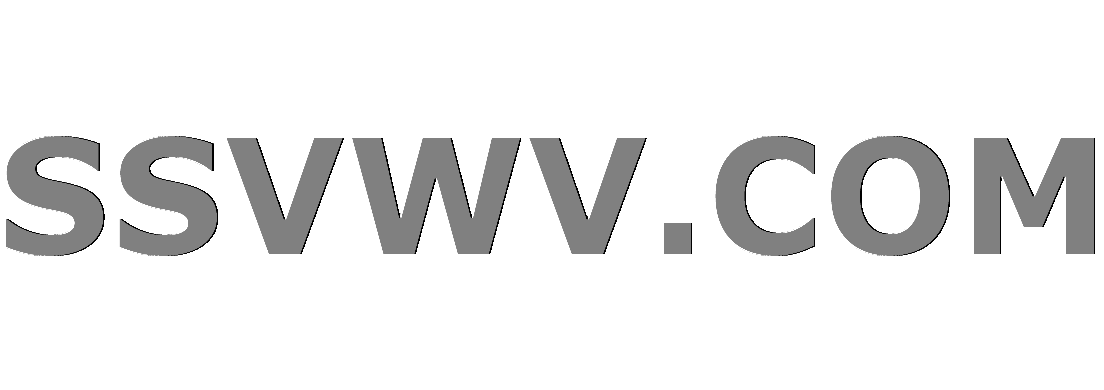
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I'm trying to create a game using only two classes - ServerSocket and Socket. Everything must be at the TCP (not UDP), while architecture is necessarily P2P. The way this game works is that one player chooses a number, then the other and it starts with one of them (no matter which one) counting down. I came across a problem. I establish a connection between two clients and both may enter the selected number. I do not know why I can not send this number to another client. Is the problem that Socket can not send and receive messages at the same time? I've read that there is a possibility to do this using two separate Threads (one receives data, the other sends), but I do not know how exactly.
@Override
public void run() {
BufferedReader br = new BufferedReader(new InputStreamReader(System. in ));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
System.out.println("PICK A NUMBER:");
while (!br.ready()) {
Thread.sleep(500);
}
Integer numberGamePicked = Integer.parseInt(br.readLine());
Thread.sleep(500);
out.println(numberGamePicked);
Thread.sleep(1000);
BufferedReader in =new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
int whatOpponentSend = Integer.parseInt( in .readLine());
System.out.println("RECEIVED " + whatOpponentSend);
}
Of course, up to this point everything is in the run() method. Is the problem that both of them execute the same code at the same time?
java multithreading sockets tcp
add a comment |
I'm trying to create a game using only two classes - ServerSocket and Socket. Everything must be at the TCP (not UDP), while architecture is necessarily P2P. The way this game works is that one player chooses a number, then the other and it starts with one of them (no matter which one) counting down. I came across a problem. I establish a connection between two clients and both may enter the selected number. I do not know why I can not send this number to another client. Is the problem that Socket can not send and receive messages at the same time? I've read that there is a possibility to do this using two separate Threads (one receives data, the other sends), but I do not know how exactly.
@Override
public void run() {
BufferedReader br = new BufferedReader(new InputStreamReader(System. in ));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
System.out.println("PICK A NUMBER:");
while (!br.ready()) {
Thread.sleep(500);
}
Integer numberGamePicked = Integer.parseInt(br.readLine());
Thread.sleep(500);
out.println(numberGamePicked);
Thread.sleep(1000);
BufferedReader in =new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
int whatOpponentSend = Integer.parseInt( in .readLine());
System.out.println("RECEIVED " + whatOpponentSend);
}
Of course, up to this point everything is in the run() method. Is the problem that both of them execute the same code at the same time?
java multithreading sockets tcp
could you give more details? so you open a server socket for one player and a client socket for another player and use the same "run" routine for both? could you post the full code for server and client? It would also be helpful if you post the output messages appearing on both sides when you run it
– mangusta
Nov 24 '18 at 16:52
it is definitely not related to the fact that they can't send/receive at the same time, however if you use the same "run" for both, the "receiver" will not be able to receive the number sent by "sender" until someone enters a number from keyboard on "receiver" side
– mangusta
Nov 24 '18 at 16:54
1
Get rid of those Thread.sleep calls and the br.ready call, they're not doing anything useful for you. The code you've shown only waits for data to be sent from the peer, it doesn't send anything. So, you'll have to show the peer's sending code in order for any debugging to happen here.
– James K Polk
Nov 24 '18 at 17:13
@JamesKPolk but he sends the data using "out.println(numberGamePicked)"
– mangusta
Nov 24 '18 at 17:48
@mangusta: oh, I missed that, thanks!
– James K Polk
Nov 24 '18 at 18:34
add a comment |
I'm trying to create a game using only two classes - ServerSocket and Socket. Everything must be at the TCP (not UDP), while architecture is necessarily P2P. The way this game works is that one player chooses a number, then the other and it starts with one of them (no matter which one) counting down. I came across a problem. I establish a connection between two clients and both may enter the selected number. I do not know why I can not send this number to another client. Is the problem that Socket can not send and receive messages at the same time? I've read that there is a possibility to do this using two separate Threads (one receives data, the other sends), but I do not know how exactly.
@Override
public void run() {
BufferedReader br = new BufferedReader(new InputStreamReader(System. in ));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
System.out.println("PICK A NUMBER:");
while (!br.ready()) {
Thread.sleep(500);
}
Integer numberGamePicked = Integer.parseInt(br.readLine());
Thread.sleep(500);
out.println(numberGamePicked);
Thread.sleep(1000);
BufferedReader in =new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
int whatOpponentSend = Integer.parseInt( in .readLine());
System.out.println("RECEIVED " + whatOpponentSend);
}
Of course, up to this point everything is in the run() method. Is the problem that both of them execute the same code at the same time?
java multithreading sockets tcp
I'm trying to create a game using only two classes - ServerSocket and Socket. Everything must be at the TCP (not UDP), while architecture is necessarily P2P. The way this game works is that one player chooses a number, then the other and it starts with one of them (no matter which one) counting down. I came across a problem. I establish a connection between two clients and both may enter the selected number. I do not know why I can not send this number to another client. Is the problem that Socket can not send and receive messages at the same time? I've read that there is a possibility to do this using two separate Threads (one receives data, the other sends), but I do not know how exactly.
@Override
public void run() {
BufferedReader br = new BufferedReader(new InputStreamReader(System. in ));
PrintWriter out = new PrintWriter(clientSocket.getOutputStream(), true);
System.out.println("PICK A NUMBER:");
while (!br.ready()) {
Thread.sleep(500);
}
Integer numberGamePicked = Integer.parseInt(br.readLine());
Thread.sleep(500);
out.println(numberGamePicked);
Thread.sleep(1000);
BufferedReader in =new BufferedReader(new InputStreamReader(clientSocket.getInputStream()));
int whatOpponentSend = Integer.parseInt( in .readLine());
System.out.println("RECEIVED " + whatOpponentSend);
}
Of course, up to this point everything is in the run() method. Is the problem that both of them execute the same code at the same time?
java multithreading sockets tcp
java multithreading sockets tcp
edited Nov 24 '18 at 18:40


James K Polk
30.6k116997
30.6k116997
asked Nov 24 '18 at 14:34
user5520959
could you give more details? so you open a server socket for one player and a client socket for another player and use the same "run" routine for both? could you post the full code for server and client? It would also be helpful if you post the output messages appearing on both sides when you run it
– mangusta
Nov 24 '18 at 16:52
it is definitely not related to the fact that they can't send/receive at the same time, however if you use the same "run" for both, the "receiver" will not be able to receive the number sent by "sender" until someone enters a number from keyboard on "receiver" side
– mangusta
Nov 24 '18 at 16:54
1
Get rid of those Thread.sleep calls and the br.ready call, they're not doing anything useful for you. The code you've shown only waits for data to be sent from the peer, it doesn't send anything. So, you'll have to show the peer's sending code in order for any debugging to happen here.
– James K Polk
Nov 24 '18 at 17:13
@JamesKPolk but he sends the data using "out.println(numberGamePicked)"
– mangusta
Nov 24 '18 at 17:48
@mangusta: oh, I missed that, thanks!
– James K Polk
Nov 24 '18 at 18:34
add a comment |
could you give more details? so you open a server socket for one player and a client socket for another player and use the same "run" routine for both? could you post the full code for server and client? It would also be helpful if you post the output messages appearing on both sides when you run it
– mangusta
Nov 24 '18 at 16:52
it is definitely not related to the fact that they can't send/receive at the same time, however if you use the same "run" for both, the "receiver" will not be able to receive the number sent by "sender" until someone enters a number from keyboard on "receiver" side
– mangusta
Nov 24 '18 at 16:54
1
Get rid of those Thread.sleep calls and the br.ready call, they're not doing anything useful for you. The code you've shown only waits for data to be sent from the peer, it doesn't send anything. So, you'll have to show the peer's sending code in order for any debugging to happen here.
– James K Polk
Nov 24 '18 at 17:13
@JamesKPolk but he sends the data using "out.println(numberGamePicked)"
– mangusta
Nov 24 '18 at 17:48
@mangusta: oh, I missed that, thanks!
– James K Polk
Nov 24 '18 at 18:34
could you give more details? so you open a server socket for one player and a client socket for another player and use the same "run" routine for both? could you post the full code for server and client? It would also be helpful if you post the output messages appearing on both sides when you run it
– mangusta
Nov 24 '18 at 16:52
could you give more details? so you open a server socket for one player and a client socket for another player and use the same "run" routine for both? could you post the full code for server and client? It would also be helpful if you post the output messages appearing on both sides when you run it
– mangusta
Nov 24 '18 at 16:52
it is definitely not related to the fact that they can't send/receive at the same time, however if you use the same "run" for both, the "receiver" will not be able to receive the number sent by "sender" until someone enters a number from keyboard on "receiver" side
– mangusta
Nov 24 '18 at 16:54
it is definitely not related to the fact that they can't send/receive at the same time, however if you use the same "run" for both, the "receiver" will not be able to receive the number sent by "sender" until someone enters a number from keyboard on "receiver" side
– mangusta
Nov 24 '18 at 16:54
1
1
Get rid of those Thread.sleep calls and the br.ready call, they're not doing anything useful for you. The code you've shown only waits for data to be sent from the peer, it doesn't send anything. So, you'll have to show the peer's sending code in order for any debugging to happen here.
– James K Polk
Nov 24 '18 at 17:13
Get rid of those Thread.sleep calls and the br.ready call, they're not doing anything useful for you. The code you've shown only waits for data to be sent from the peer, it doesn't send anything. So, you'll have to show the peer's sending code in order for any debugging to happen here.
– James K Polk
Nov 24 '18 at 17:13
@JamesKPolk but he sends the data using "out.println(numberGamePicked)"
– mangusta
Nov 24 '18 at 17:48
@JamesKPolk but he sends the data using "out.println(numberGamePicked)"
– mangusta
Nov 24 '18 at 17:48
@mangusta: oh, I missed that, thanks!
– James K Polk
Nov 24 '18 at 18:34
@mangusta: oh, I missed that, thanks!
– James K Polk
Nov 24 '18 at 18:34
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459223%2fjava-thread-sockets-printwriter-bufferedreader%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53459223%2fjava-thread-sockets-printwriter-bufferedreader%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uTAuwgM aFNQtW3oJe,t,ZPZ2dgQy46,zD0JjBu,VYD5N XH,tETaEgt PXT
could you give more details? so you open a server socket for one player and a client socket for another player and use the same "run" routine for both? could you post the full code for server and client? It would also be helpful if you post the output messages appearing on both sides when you run it
– mangusta
Nov 24 '18 at 16:52
it is definitely not related to the fact that they can't send/receive at the same time, however if you use the same "run" for both, the "receiver" will not be able to receive the number sent by "sender" until someone enters a number from keyboard on "receiver" side
– mangusta
Nov 24 '18 at 16:54
1
Get rid of those Thread.sleep calls and the br.ready call, they're not doing anything useful for you. The code you've shown only waits for data to be sent from the peer, it doesn't send anything. So, you'll have to show the peer's sending code in order for any debugging to happen here.
– James K Polk
Nov 24 '18 at 17:13
@JamesKPolk but he sends the data using "out.println(numberGamePicked)"
– mangusta
Nov 24 '18 at 17:48
@mangusta: oh, I missed that, thanks!
– James K Polk
Nov 24 '18 at 18:34