Packetize TCP stream
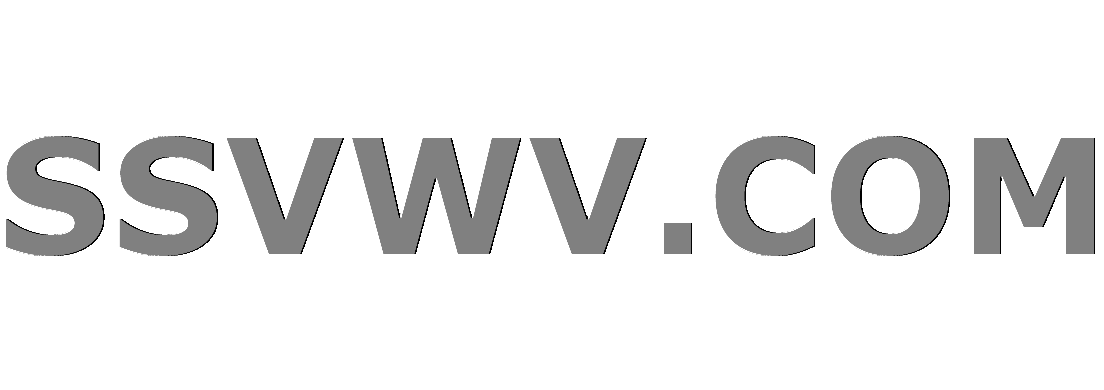
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
In my program, I have the following schema:
Client - intermediate router - server
The client and router are connected with TCP connection. Router and server has UDP connection.
I am sending the data as this:
messagetobesend = "some very long message to be send"
sock = socket(AF_INET,SOCK_STREAM)
# bind my port to the socket
sock.bind((IP,PORT))
sock.connect((routerip, routerport))
# since I'm using TCP, send the data byte by byte
for i in range(len(messagetobesend)):
sock.send(messagetobesend[i])
# now, I have send all of the message
sock.close()
In the router, I have the following:
sock = socket(AF_INET,SOCK_STREAM)
sock.bind((ip, port))
sock.listen(1)
# I have accepted the TCP connection
newsocket, addr = sock.accept()
# now create a UDP socket
udpsock = socket(AF_INET,SOCK_DGRAM)
udpsock.bind((myudpip, myudpport))
while True:
# I want to packetize the data with 128 bytes
data = newsocket.recv(128)
if(data):
data.sendto(udpsock,(serverip,serverport))
else:
#received no data, terminate udp connection
udpsock.close()
newsocket.close()
And the server:
sock = socket(AF_INET,SOCK_DGRAM)
sock.bind((myip, myport))
while True:
data, addr = sock.recvfrom(128)
#print data
Everything I do seems correct. However, the server usually receives packets as 128 bytes, sometimes receives most of them 128bytes and some of them not 128 bytes.
Am I doing something wrong here? I want server to receive all of these packets with length of 128 bytes(all received packets length should be identical)
python tcp udp
add a comment |
In my program, I have the following schema:
Client - intermediate router - server
The client and router are connected with TCP connection. Router and server has UDP connection.
I am sending the data as this:
messagetobesend = "some very long message to be send"
sock = socket(AF_INET,SOCK_STREAM)
# bind my port to the socket
sock.bind((IP,PORT))
sock.connect((routerip, routerport))
# since I'm using TCP, send the data byte by byte
for i in range(len(messagetobesend)):
sock.send(messagetobesend[i])
# now, I have send all of the message
sock.close()
In the router, I have the following:
sock = socket(AF_INET,SOCK_STREAM)
sock.bind((ip, port))
sock.listen(1)
# I have accepted the TCP connection
newsocket, addr = sock.accept()
# now create a UDP socket
udpsock = socket(AF_INET,SOCK_DGRAM)
udpsock.bind((myudpip, myudpport))
while True:
# I want to packetize the data with 128 bytes
data = newsocket.recv(128)
if(data):
data.sendto(udpsock,(serverip,serverport))
else:
#received no data, terminate udp connection
udpsock.close()
newsocket.close()
And the server:
sock = socket(AF_INET,SOCK_DGRAM)
sock.bind((myip, myport))
while True:
data, addr = sock.recvfrom(128)
#print data
Everything I do seems correct. However, the server usually receives packets as 128 bytes, sometimes receives most of them 128bytes and some of them not 128 bytes.
Am I doing something wrong here? I want server to receive all of these packets with length of 128 bytes(all received packets length should be identical)
python tcp udp
add a comment |
In my program, I have the following schema:
Client - intermediate router - server
The client and router are connected with TCP connection. Router and server has UDP connection.
I am sending the data as this:
messagetobesend = "some very long message to be send"
sock = socket(AF_INET,SOCK_STREAM)
# bind my port to the socket
sock.bind((IP,PORT))
sock.connect((routerip, routerport))
# since I'm using TCP, send the data byte by byte
for i in range(len(messagetobesend)):
sock.send(messagetobesend[i])
# now, I have send all of the message
sock.close()
In the router, I have the following:
sock = socket(AF_INET,SOCK_STREAM)
sock.bind((ip, port))
sock.listen(1)
# I have accepted the TCP connection
newsocket, addr = sock.accept()
# now create a UDP socket
udpsock = socket(AF_INET,SOCK_DGRAM)
udpsock.bind((myudpip, myudpport))
while True:
# I want to packetize the data with 128 bytes
data = newsocket.recv(128)
if(data):
data.sendto(udpsock,(serverip,serverport))
else:
#received no data, terminate udp connection
udpsock.close()
newsocket.close()
And the server:
sock = socket(AF_INET,SOCK_DGRAM)
sock.bind((myip, myport))
while True:
data, addr = sock.recvfrom(128)
#print data
Everything I do seems correct. However, the server usually receives packets as 128 bytes, sometimes receives most of them 128bytes and some of them not 128 bytes.
Am I doing something wrong here? I want server to receive all of these packets with length of 128 bytes(all received packets length should be identical)
python tcp udp
In my program, I have the following schema:
Client - intermediate router - server
The client and router are connected with TCP connection. Router and server has UDP connection.
I am sending the data as this:
messagetobesend = "some very long message to be send"
sock = socket(AF_INET,SOCK_STREAM)
# bind my port to the socket
sock.bind((IP,PORT))
sock.connect((routerip, routerport))
# since I'm using TCP, send the data byte by byte
for i in range(len(messagetobesend)):
sock.send(messagetobesend[i])
# now, I have send all of the message
sock.close()
In the router, I have the following:
sock = socket(AF_INET,SOCK_STREAM)
sock.bind((ip, port))
sock.listen(1)
# I have accepted the TCP connection
newsocket, addr = sock.accept()
# now create a UDP socket
udpsock = socket(AF_INET,SOCK_DGRAM)
udpsock.bind((myudpip, myudpport))
while True:
# I want to packetize the data with 128 bytes
data = newsocket.recv(128)
if(data):
data.sendto(udpsock,(serverip,serverport))
else:
#received no data, terminate udp connection
udpsock.close()
newsocket.close()
And the server:
sock = socket(AF_INET,SOCK_DGRAM)
sock.bind((myip, myport))
while True:
data, addr = sock.recvfrom(128)
#print data
Everything I do seems correct. However, the server usually receives packets as 128 bytes, sometimes receives most of them 128bytes and some of them not 128 bytes.
Am I doing something wrong here? I want server to receive all of these packets with length of 128 bytes(all received packets length should be identical)
python tcp udp
python tcp udp
asked Nov 23 '18 at 18:42
m2568475m2568475
1
1
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Your recv
call returns up to 128 bytes. If you need to receive exactly 128 bytes, then you should write your own recv_exactly
:
PACKET_LEN = 128
# ...
def recv_exactly(socket, length):
buf = bytearray()
bytes_left = length
while bytes_left: # equivalent to while bytes_left != 0:
data = socket.recv(bytes_left)
if not data:
return buf # could theoretically be less than length
buf += data
bytes_left -= len(data)
return buf
while True:
data = recv_exactly(newsocket, PACKET_LEN)
if (len(data) == PACKET_LEN): # Data fully received
udpsock.sendto(data, (serverip, serverport))
elif (len(data) > 0): # Data partially received
udpsock.sendto(data, (serverip, serverport))
udpsock.close()
else: # got no data
udpsock.close()
P.S.: You can send whole messagetobesend
, sending it byte by byte is not necessary. OS kernel and network interface would buffer and split your bytes however they need to.
And generally: TCP is a stream of bytes that will end when the socket would be closed. It arrives in unpredictable chunks, independent of how much data was sent in each send
call. TCP guarantees the order and integrity of the stream, but does not have packet boundaries.
UDP is unreliable, can arrive with mangled data, in a wrong packet order, duplicated, or not arrive at all. But UDP is packet oriented, meaning that receiver can determine the boundaries of the packets, exactly how they were sent (if the packet made it over the network).
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451605%2fpacketize-tcp-stream%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your recv
call returns up to 128 bytes. If you need to receive exactly 128 bytes, then you should write your own recv_exactly
:
PACKET_LEN = 128
# ...
def recv_exactly(socket, length):
buf = bytearray()
bytes_left = length
while bytes_left: # equivalent to while bytes_left != 0:
data = socket.recv(bytes_left)
if not data:
return buf # could theoretically be less than length
buf += data
bytes_left -= len(data)
return buf
while True:
data = recv_exactly(newsocket, PACKET_LEN)
if (len(data) == PACKET_LEN): # Data fully received
udpsock.sendto(data, (serverip, serverport))
elif (len(data) > 0): # Data partially received
udpsock.sendto(data, (serverip, serverport))
udpsock.close()
else: # got no data
udpsock.close()
P.S.: You can send whole messagetobesend
, sending it byte by byte is not necessary. OS kernel and network interface would buffer and split your bytes however they need to.
And generally: TCP is a stream of bytes that will end when the socket would be closed. It arrives in unpredictable chunks, independent of how much data was sent in each send
call. TCP guarantees the order and integrity of the stream, but does not have packet boundaries.
UDP is unreliable, can arrive with mangled data, in a wrong packet order, duplicated, or not arrive at all. But UDP is packet oriented, meaning that receiver can determine the boundaries of the packets, exactly how they were sent (if the packet made it over the network).
add a comment |
Your recv
call returns up to 128 bytes. If you need to receive exactly 128 bytes, then you should write your own recv_exactly
:
PACKET_LEN = 128
# ...
def recv_exactly(socket, length):
buf = bytearray()
bytes_left = length
while bytes_left: # equivalent to while bytes_left != 0:
data = socket.recv(bytes_left)
if not data:
return buf # could theoretically be less than length
buf += data
bytes_left -= len(data)
return buf
while True:
data = recv_exactly(newsocket, PACKET_LEN)
if (len(data) == PACKET_LEN): # Data fully received
udpsock.sendto(data, (serverip, serverport))
elif (len(data) > 0): # Data partially received
udpsock.sendto(data, (serverip, serverport))
udpsock.close()
else: # got no data
udpsock.close()
P.S.: You can send whole messagetobesend
, sending it byte by byte is not necessary. OS kernel and network interface would buffer and split your bytes however they need to.
And generally: TCP is a stream of bytes that will end when the socket would be closed. It arrives in unpredictable chunks, independent of how much data was sent in each send
call. TCP guarantees the order and integrity of the stream, but does not have packet boundaries.
UDP is unreliable, can arrive with mangled data, in a wrong packet order, duplicated, or not arrive at all. But UDP is packet oriented, meaning that receiver can determine the boundaries of the packets, exactly how they were sent (if the packet made it over the network).
add a comment |
Your recv
call returns up to 128 bytes. If you need to receive exactly 128 bytes, then you should write your own recv_exactly
:
PACKET_LEN = 128
# ...
def recv_exactly(socket, length):
buf = bytearray()
bytes_left = length
while bytes_left: # equivalent to while bytes_left != 0:
data = socket.recv(bytes_left)
if not data:
return buf # could theoretically be less than length
buf += data
bytes_left -= len(data)
return buf
while True:
data = recv_exactly(newsocket, PACKET_LEN)
if (len(data) == PACKET_LEN): # Data fully received
udpsock.sendto(data, (serverip, serverport))
elif (len(data) > 0): # Data partially received
udpsock.sendto(data, (serverip, serverport))
udpsock.close()
else: # got no data
udpsock.close()
P.S.: You can send whole messagetobesend
, sending it byte by byte is not necessary. OS kernel and network interface would buffer and split your bytes however they need to.
And generally: TCP is a stream of bytes that will end when the socket would be closed. It arrives in unpredictable chunks, independent of how much data was sent in each send
call. TCP guarantees the order and integrity of the stream, but does not have packet boundaries.
UDP is unreliable, can arrive with mangled data, in a wrong packet order, duplicated, or not arrive at all. But UDP is packet oriented, meaning that receiver can determine the boundaries of the packets, exactly how they were sent (if the packet made it over the network).
Your recv
call returns up to 128 bytes. If you need to receive exactly 128 bytes, then you should write your own recv_exactly
:
PACKET_LEN = 128
# ...
def recv_exactly(socket, length):
buf = bytearray()
bytes_left = length
while bytes_left: # equivalent to while bytes_left != 0:
data = socket.recv(bytes_left)
if not data:
return buf # could theoretically be less than length
buf += data
bytes_left -= len(data)
return buf
while True:
data = recv_exactly(newsocket, PACKET_LEN)
if (len(data) == PACKET_LEN): # Data fully received
udpsock.sendto(data, (serverip, serverport))
elif (len(data) > 0): # Data partially received
udpsock.sendto(data, (serverip, serverport))
udpsock.close()
else: # got no data
udpsock.close()
P.S.: You can send whole messagetobesend
, sending it byte by byte is not necessary. OS kernel and network interface would buffer and split your bytes however they need to.
And generally: TCP is a stream of bytes that will end when the socket would be closed. It arrives in unpredictable chunks, independent of how much data was sent in each send
call. TCP guarantees the order and integrity of the stream, but does not have packet boundaries.
UDP is unreliable, can arrive with mangled data, in a wrong packet order, duplicated, or not arrive at all. But UDP is packet oriented, meaning that receiver can determine the boundaries of the packets, exactly how they were sent (if the packet made it over the network).
edited Nov 23 '18 at 19:23
answered Nov 23 '18 at 19:10
Andrew MorozkoAndrew Morozko
931712
931712
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451605%2fpacketize-tcp-stream%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
cvhyYoLaAo43 HYLGnz jwaMioMoYK H,UkZ7HQBPAffaT7 zIh2bJcMRu7mqj8Y2BAj,kN2bajGPBd IjckbWHG21aas6J,L1