How would I find and replace chars inside a 2d vector of chars? c++
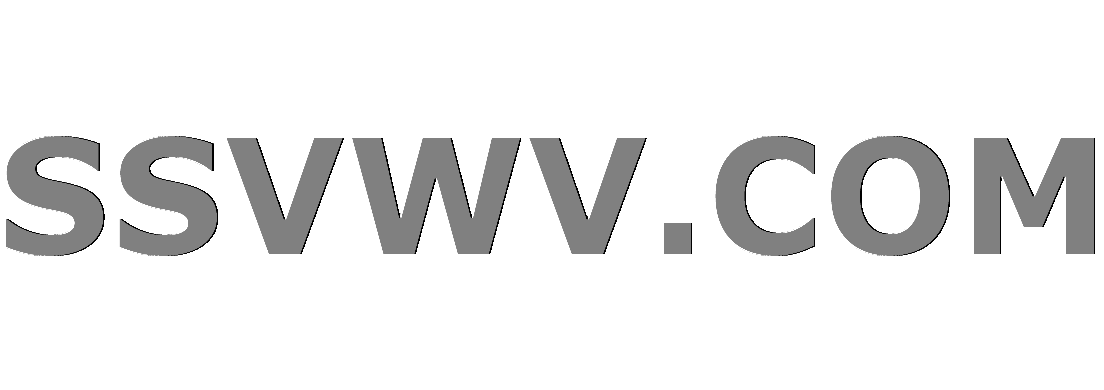
Multi tool use
up vote
2
down vote
favorite
Lets say I have a 2d array of chars that looks like:
ooooooooo
ooooooooo
ooooooooo
ooooxoooo
ooooooooo
ooooooooo
ooooooooo
lets say i want to replace all the o's to be I instead so it would look like this:
IIIIIIIII
IIIIIIIII
IIIIIIIII
IIIIxIIII
IIIIIIIII
IIIIIIIII
IIIIIIIII
the current way im getting input from the user is using the command line:
int main(int argc, char* argv) {
This is what ive come up with so far:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> v:vec)
// change
{
for(char c:v)
if (c == oldd) {
c == neww;
}
}
}
Ps. Sorry if this is a simple solution, im new to c++ and ive looked up ways other people have done it but they have always had a vector of Strings instead of char's.
c++ vector char
add a comment |
up vote
2
down vote
favorite
Lets say I have a 2d array of chars that looks like:
ooooooooo
ooooooooo
ooooooooo
ooooxoooo
ooooooooo
ooooooooo
ooooooooo
lets say i want to replace all the o's to be I instead so it would look like this:
IIIIIIIII
IIIIIIIII
IIIIIIIII
IIIIxIIII
IIIIIIIII
IIIIIIIII
IIIIIIIII
the current way im getting input from the user is using the command line:
int main(int argc, char* argv) {
This is what ive come up with so far:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> v:vec)
// change
{
for(char c:v)
if (c == oldd) {
c == neww;
}
}
}
Ps. Sorry if this is a simple solution, im new to c++ and ive looked up ways other people have done it but they have always had a vector of Strings instead of char's.
c++ vector char
add a comment |
up vote
2
down vote
favorite
up vote
2
down vote
favorite
Lets say I have a 2d array of chars that looks like:
ooooooooo
ooooooooo
ooooooooo
ooooxoooo
ooooooooo
ooooooooo
ooooooooo
lets say i want to replace all the o's to be I instead so it would look like this:
IIIIIIIII
IIIIIIIII
IIIIIIIII
IIIIxIIII
IIIIIIIII
IIIIIIIII
IIIIIIIII
the current way im getting input from the user is using the command line:
int main(int argc, char* argv) {
This is what ive come up with so far:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> v:vec)
// change
{
for(char c:v)
if (c == oldd) {
c == neww;
}
}
}
Ps. Sorry if this is a simple solution, im new to c++ and ive looked up ways other people have done it but they have always had a vector of Strings instead of char's.
c++ vector char
Lets say I have a 2d array of chars that looks like:
ooooooooo
ooooooooo
ooooooooo
ooooxoooo
ooooooooo
ooooooooo
ooooooooo
lets say i want to replace all the o's to be I instead so it would look like this:
IIIIIIIII
IIIIIIIII
IIIIIIIII
IIIIxIIII
IIIIIIIII
IIIIIIIII
IIIIIIIII
the current way im getting input from the user is using the command line:
int main(int argc, char* argv) {
This is what ive come up with so far:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> v:vec)
// change
{
for(char c:v)
if (c == oldd) {
c == neww;
}
}
}
Ps. Sorry if this is a simple solution, im new to c++ and ive looked up ways other people have done it but they have always had a vector of Strings instead of char's.
c++ vector char
c++ vector char
edited Nov 8 at 21:36
scohe001
7,78912141
7,78912141
asked Nov 8 at 21:33


xannax159
448
448
add a comment |
add a comment |
4 Answers
4
active
oldest
votes
up vote
2
down vote
accepted
Your outer loop needs to take a reference to the inner vector. Without a reference the inner loop only modifies a copy of the vector. One suggestion, use a standard library algorithm instead of a loop to modify the values.
#include <algorithm>
void replacee(vector<vector<char>> &vec, char oldd, char neww)
{
for (vector<char> &v : vec) // reference to innver vector
{
replace(v.begin(), v.end(), oldd, neww); // standard library algorithm
}
}
Thank you, using a library makes things so much easier! Thanks!
– xannax159
Nov 9 at 23:23
add a comment |
up vote
1
down vote
Note the critical difference between =
and ==
. The expression c == oldd
is a test for equality. That is, it returns true
if c
and oldd
have the same value, and false
otherwise. On the other hand, an expression like c = neww
is an assignment, which means that the value of neww
is copied into the variable c
.
Additionally, in your for loops, you should loop over elements by reference if you want to make lasting changes.
for(vector<char> v : vec){ /* v is a copy, changing it will leave no effect */ }
for(vector<char>& v : vec){ /* v is a reference, changes to it will be visible outside */ }
Try this fix:
for(vector<char>& v : vec){
for(char& c : v){
if (c == oldd) { // only enter the clause if c and old are equal
// c == neww; <- this does nothing, it returns a boolean value that never gets used
c = neww; // <- this assigns the new value
}
}
}
Hope that helps! Happy coding
add a comment |
up vote
1
down vote
You're super close! You only have two mistakes.
- This one's pretty silly, but it looks like you accidentally put an extra equal's sign in the assignment of the new character. Change
c == neww;
(which compares them and does nothing) toc = neww;
. - This is a little more nuanced, but you'll need to change your loops to use reference variables. Right now, when you loop, you're dealing with a copy of each row, and
c
is a copy of each value in each copied row. This is as simple as adding two&
's in your loops.
When all is said an done and you fix your indenting and make your formatting stick to either braces on the same line or the next line, things should look like:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> &v:vec) {
for(char &c:v) {
if (c == oldd) { c = neww; }
}
}
}
See it work here: ideone
Thanks for the really friendly response. I realized what i did with the = operator.
– xannax159
Nov 9 at 23:23
add a comment |
up vote
0
down vote
void replace(vector<vector<char>> &vec, char oldd, char neww) {
for(auto v : vec)
{
for(auto c : v)
if (c == oldd) {
c = neww;
}
}
}
Here you go. C++ allows you to use auto in a foreach loop and etc. and it is super neat.
Yourfor
loops aren't quite correct. You need to take non-const references for the changes to affect the container.
– Blastfurnace
Nov 8 at 22:07
Thank you very much :)
– Long Nguyen
Nov 8 at 22:25
You need references in both loops:for (auto &v : vec)
andfor (auto &c : v)
– Blastfurnace
Nov 8 at 22:28
add a comment |
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
2
down vote
accepted
Your outer loop needs to take a reference to the inner vector. Without a reference the inner loop only modifies a copy of the vector. One suggestion, use a standard library algorithm instead of a loop to modify the values.
#include <algorithm>
void replacee(vector<vector<char>> &vec, char oldd, char neww)
{
for (vector<char> &v : vec) // reference to innver vector
{
replace(v.begin(), v.end(), oldd, neww); // standard library algorithm
}
}
Thank you, using a library makes things so much easier! Thanks!
– xannax159
Nov 9 at 23:23
add a comment |
up vote
2
down vote
accepted
Your outer loop needs to take a reference to the inner vector. Without a reference the inner loop only modifies a copy of the vector. One suggestion, use a standard library algorithm instead of a loop to modify the values.
#include <algorithm>
void replacee(vector<vector<char>> &vec, char oldd, char neww)
{
for (vector<char> &v : vec) // reference to innver vector
{
replace(v.begin(), v.end(), oldd, neww); // standard library algorithm
}
}
Thank you, using a library makes things so much easier! Thanks!
– xannax159
Nov 9 at 23:23
add a comment |
up vote
2
down vote
accepted
up vote
2
down vote
accepted
Your outer loop needs to take a reference to the inner vector. Without a reference the inner loop only modifies a copy of the vector. One suggestion, use a standard library algorithm instead of a loop to modify the values.
#include <algorithm>
void replacee(vector<vector<char>> &vec, char oldd, char neww)
{
for (vector<char> &v : vec) // reference to innver vector
{
replace(v.begin(), v.end(), oldd, neww); // standard library algorithm
}
}
Your outer loop needs to take a reference to the inner vector. Without a reference the inner loop only modifies a copy of the vector. One suggestion, use a standard library algorithm instead of a loop to modify the values.
#include <algorithm>
void replacee(vector<vector<char>> &vec, char oldd, char neww)
{
for (vector<char> &v : vec) // reference to innver vector
{
replace(v.begin(), v.end(), oldd, neww); // standard library algorithm
}
}
edited Nov 8 at 22:07
answered Nov 8 at 22:02
Blastfurnace
13.6k54058
13.6k54058
Thank you, using a library makes things so much easier! Thanks!
– xannax159
Nov 9 at 23:23
add a comment |
Thank you, using a library makes things so much easier! Thanks!
– xannax159
Nov 9 at 23:23
Thank you, using a library makes things so much easier! Thanks!
– xannax159
Nov 9 at 23:23
Thank you, using a library makes things so much easier! Thanks!
– xannax159
Nov 9 at 23:23
add a comment |
up vote
1
down vote
Note the critical difference between =
and ==
. The expression c == oldd
is a test for equality. That is, it returns true
if c
and oldd
have the same value, and false
otherwise. On the other hand, an expression like c = neww
is an assignment, which means that the value of neww
is copied into the variable c
.
Additionally, in your for loops, you should loop over elements by reference if you want to make lasting changes.
for(vector<char> v : vec){ /* v is a copy, changing it will leave no effect */ }
for(vector<char>& v : vec){ /* v is a reference, changes to it will be visible outside */ }
Try this fix:
for(vector<char>& v : vec){
for(char& c : v){
if (c == oldd) { // only enter the clause if c and old are equal
// c == neww; <- this does nothing, it returns a boolean value that never gets used
c = neww; // <- this assigns the new value
}
}
}
Hope that helps! Happy coding
add a comment |
up vote
1
down vote
Note the critical difference between =
and ==
. The expression c == oldd
is a test for equality. That is, it returns true
if c
and oldd
have the same value, and false
otherwise. On the other hand, an expression like c = neww
is an assignment, which means that the value of neww
is copied into the variable c
.
Additionally, in your for loops, you should loop over elements by reference if you want to make lasting changes.
for(vector<char> v : vec){ /* v is a copy, changing it will leave no effect */ }
for(vector<char>& v : vec){ /* v is a reference, changes to it will be visible outside */ }
Try this fix:
for(vector<char>& v : vec){
for(char& c : v){
if (c == oldd) { // only enter the clause if c and old are equal
// c == neww; <- this does nothing, it returns a boolean value that never gets used
c = neww; // <- this assigns the new value
}
}
}
Hope that helps! Happy coding
add a comment |
up vote
1
down vote
up vote
1
down vote
Note the critical difference between =
and ==
. The expression c == oldd
is a test for equality. That is, it returns true
if c
and oldd
have the same value, and false
otherwise. On the other hand, an expression like c = neww
is an assignment, which means that the value of neww
is copied into the variable c
.
Additionally, in your for loops, you should loop over elements by reference if you want to make lasting changes.
for(vector<char> v : vec){ /* v is a copy, changing it will leave no effect */ }
for(vector<char>& v : vec){ /* v is a reference, changes to it will be visible outside */ }
Try this fix:
for(vector<char>& v : vec){
for(char& c : v){
if (c == oldd) { // only enter the clause if c and old are equal
// c == neww; <- this does nothing, it returns a boolean value that never gets used
c = neww; // <- this assigns the new value
}
}
}
Hope that helps! Happy coding
Note the critical difference between =
and ==
. The expression c == oldd
is a test for equality. That is, it returns true
if c
and oldd
have the same value, and false
otherwise. On the other hand, an expression like c = neww
is an assignment, which means that the value of neww
is copied into the variable c
.
Additionally, in your for loops, you should loop over elements by reference if you want to make lasting changes.
for(vector<char> v : vec){ /* v is a copy, changing it will leave no effect */ }
for(vector<char>& v : vec){ /* v is a reference, changes to it will be visible outside */ }
Try this fix:
for(vector<char>& v : vec){
for(char& c : v){
if (c == oldd) { // only enter the clause if c and old are equal
// c == neww; <- this does nothing, it returns a boolean value that never gets used
c = neww; // <- this assigns the new value
}
}
}
Hope that helps! Happy coding
answered Nov 8 at 21:45


alter igel
2,4551824
2,4551824
add a comment |
add a comment |
up vote
1
down vote
You're super close! You only have two mistakes.
- This one's pretty silly, but it looks like you accidentally put an extra equal's sign in the assignment of the new character. Change
c == neww;
(which compares them and does nothing) toc = neww;
. - This is a little more nuanced, but you'll need to change your loops to use reference variables. Right now, when you loop, you're dealing with a copy of each row, and
c
is a copy of each value in each copied row. This is as simple as adding two&
's in your loops.
When all is said an done and you fix your indenting and make your formatting stick to either braces on the same line or the next line, things should look like:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> &v:vec) {
for(char &c:v) {
if (c == oldd) { c = neww; }
}
}
}
See it work here: ideone
Thanks for the really friendly response. I realized what i did with the = operator.
– xannax159
Nov 9 at 23:23
add a comment |
up vote
1
down vote
You're super close! You only have two mistakes.
- This one's pretty silly, but it looks like you accidentally put an extra equal's sign in the assignment of the new character. Change
c == neww;
(which compares them and does nothing) toc = neww;
. - This is a little more nuanced, but you'll need to change your loops to use reference variables. Right now, when you loop, you're dealing with a copy of each row, and
c
is a copy of each value in each copied row. This is as simple as adding two&
's in your loops.
When all is said an done and you fix your indenting and make your formatting stick to either braces on the same line or the next line, things should look like:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> &v:vec) {
for(char &c:v) {
if (c == oldd) { c = neww; }
}
}
}
See it work here: ideone
Thanks for the really friendly response. I realized what i did with the = operator.
– xannax159
Nov 9 at 23:23
add a comment |
up vote
1
down vote
up vote
1
down vote
You're super close! You only have two mistakes.
- This one's pretty silly, but it looks like you accidentally put an extra equal's sign in the assignment of the new character. Change
c == neww;
(which compares them and does nothing) toc = neww;
. - This is a little more nuanced, but you'll need to change your loops to use reference variables. Right now, when you loop, you're dealing with a copy of each row, and
c
is a copy of each value in each copied row. This is as simple as adding two&
's in your loops.
When all is said an done and you fix your indenting and make your formatting stick to either braces on the same line or the next line, things should look like:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> &v:vec) {
for(char &c:v) {
if (c == oldd) { c = neww; }
}
}
}
See it work here: ideone
You're super close! You only have two mistakes.
- This one's pretty silly, but it looks like you accidentally put an extra equal's sign in the assignment of the new character. Change
c == neww;
(which compares them and does nothing) toc = neww;
. - This is a little more nuanced, but you'll need to change your loops to use reference variables. Right now, when you loop, you're dealing with a copy of each row, and
c
is a copy of each value in each copied row. This is as simple as adding two&
's in your loops.
When all is said an done and you fix your indenting and make your formatting stick to either braces on the same line or the next line, things should look like:
void replacee(vector<vector<char>> &vec, char oldd, char neww) {
for(vector<char> &v:vec) {
for(char &c:v) {
if (c == oldd) { c = neww; }
}
}
}
See it work here: ideone
edited Nov 8 at 21:57
answered Nov 8 at 21:43
scohe001
7,78912141
7,78912141
Thanks for the really friendly response. I realized what i did with the = operator.
– xannax159
Nov 9 at 23:23
add a comment |
Thanks for the really friendly response. I realized what i did with the = operator.
– xannax159
Nov 9 at 23:23
Thanks for the really friendly response. I realized what i did with the = operator.
– xannax159
Nov 9 at 23:23
Thanks for the really friendly response. I realized what i did with the = operator.
– xannax159
Nov 9 at 23:23
add a comment |
up vote
0
down vote
void replace(vector<vector<char>> &vec, char oldd, char neww) {
for(auto v : vec)
{
for(auto c : v)
if (c == oldd) {
c = neww;
}
}
}
Here you go. C++ allows you to use auto in a foreach loop and etc. and it is super neat.
Yourfor
loops aren't quite correct. You need to take non-const references for the changes to affect the container.
– Blastfurnace
Nov 8 at 22:07
Thank you very much :)
– Long Nguyen
Nov 8 at 22:25
You need references in both loops:for (auto &v : vec)
andfor (auto &c : v)
– Blastfurnace
Nov 8 at 22:28
add a comment |
up vote
0
down vote
void replace(vector<vector<char>> &vec, char oldd, char neww) {
for(auto v : vec)
{
for(auto c : v)
if (c == oldd) {
c = neww;
}
}
}
Here you go. C++ allows you to use auto in a foreach loop and etc. and it is super neat.
Yourfor
loops aren't quite correct. You need to take non-const references for the changes to affect the container.
– Blastfurnace
Nov 8 at 22:07
Thank you very much :)
– Long Nguyen
Nov 8 at 22:25
You need references in both loops:for (auto &v : vec)
andfor (auto &c : v)
– Blastfurnace
Nov 8 at 22:28
add a comment |
up vote
0
down vote
up vote
0
down vote
void replace(vector<vector<char>> &vec, char oldd, char neww) {
for(auto v : vec)
{
for(auto c : v)
if (c == oldd) {
c = neww;
}
}
}
Here you go. C++ allows you to use auto in a foreach loop and etc. and it is super neat.
void replace(vector<vector<char>> &vec, char oldd, char neww) {
for(auto v : vec)
{
for(auto c : v)
if (c == oldd) {
c = neww;
}
}
}
Here you go. C++ allows you to use auto in a foreach loop and etc. and it is super neat.
edited Nov 8 at 22:34
answered Nov 8 at 22:03


Long Nguyen
9119
9119
Yourfor
loops aren't quite correct. You need to take non-const references for the changes to affect the container.
– Blastfurnace
Nov 8 at 22:07
Thank you very much :)
– Long Nguyen
Nov 8 at 22:25
You need references in both loops:for (auto &v : vec)
andfor (auto &c : v)
– Blastfurnace
Nov 8 at 22:28
add a comment |
Yourfor
loops aren't quite correct. You need to take non-const references for the changes to affect the container.
– Blastfurnace
Nov 8 at 22:07
Thank you very much :)
– Long Nguyen
Nov 8 at 22:25
You need references in both loops:for (auto &v : vec)
andfor (auto &c : v)
– Blastfurnace
Nov 8 at 22:28
Your
for
loops aren't quite correct. You need to take non-const references for the changes to affect the container.– Blastfurnace
Nov 8 at 22:07
Your
for
loops aren't quite correct. You need to take non-const references for the changes to affect the container.– Blastfurnace
Nov 8 at 22:07
Thank you very much :)
– Long Nguyen
Nov 8 at 22:25
Thank you very much :)
– Long Nguyen
Nov 8 at 22:25
You need references in both loops:
for (auto &v : vec)
and for (auto &c : v)
– Blastfurnace
Nov 8 at 22:28
You need references in both loops:
for (auto &v : vec)
and for (auto &c : v)
– Blastfurnace
Nov 8 at 22:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53216482%2fhow-would-i-find-and-replace-chars-inside-a-2d-vector-of-chars-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wP,bWFp,PORaR2M KjSUkRDFSeVkLL0NTzqKnliV3,9iZ0Qwfw5I,LaUefTSm UCZ M So 9LJ xYJwaH,hO kcMtSwAs9AYoQq7vT kv