Vue + Puppeteer: evaluate specified scripts only?
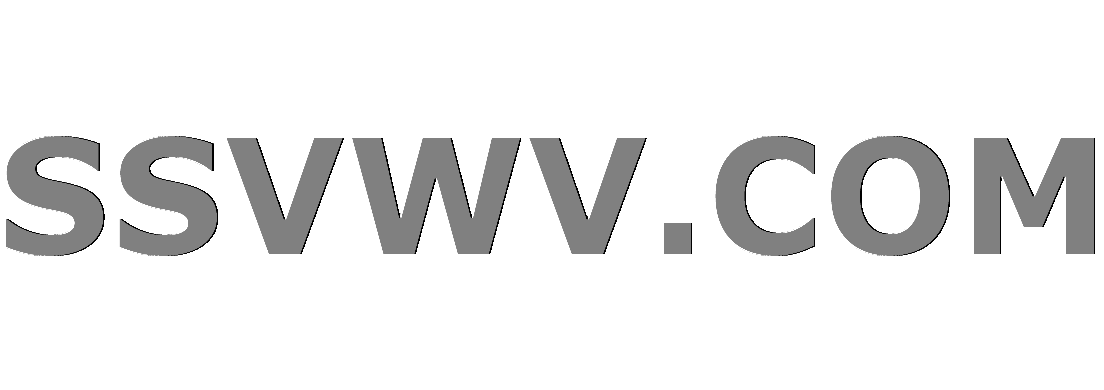
Multi tool use
up vote
-1
down vote
favorite
I'm using Vue to create an SPA (single-page app) and would like to render some semi-static pages. "Semi-static" meaning I'd like specific parts of the page to be rendered as their eventual HTML markup, while other parts of the page should remain dynamic just like the original Vue SPA.
A very basic example of one of my Vue templates:
<template>
<div>{{ topHeadline }}</div>
<div>
<widget
v-for="(w, index) in getWidgetList"
v-bind:key="w.id"
v-bind:name="w.widgetName"
v-bind:obj="w"></widget>
<div>
</template>
<script>
import { stateholder } from '../state/index.js'
export default {
data () {
return {
headlineText: stateholder.state.headline ,
versionNum: stateholder.state.versionNumber
}
} ,
methods: {
topHeadline: function() {
return `{$headlineText} v.{$versionNum}`
} ,
getWidgetList: async function() {
axios.get(...).then((response) => {
return response.data
});
}
}
}
</script>
In this case, I'd like Puppeteer to render the topHeadline as text in a simple DIV tag, while leaving the getWidgetList un-evaluated, so the end-user would still perform the axios request and then render a list of components dynamically.
Is this possible? How do I instruct puppeteer to evaluate some scripts but leave others alone?
vue.js vuejs2 puppeteer
add a comment |
up vote
-1
down vote
favorite
I'm using Vue to create an SPA (single-page app) and would like to render some semi-static pages. "Semi-static" meaning I'd like specific parts of the page to be rendered as their eventual HTML markup, while other parts of the page should remain dynamic just like the original Vue SPA.
A very basic example of one of my Vue templates:
<template>
<div>{{ topHeadline }}</div>
<div>
<widget
v-for="(w, index) in getWidgetList"
v-bind:key="w.id"
v-bind:name="w.widgetName"
v-bind:obj="w"></widget>
<div>
</template>
<script>
import { stateholder } from '../state/index.js'
export default {
data () {
return {
headlineText: stateholder.state.headline ,
versionNum: stateholder.state.versionNumber
}
} ,
methods: {
topHeadline: function() {
return `{$headlineText} v.{$versionNum}`
} ,
getWidgetList: async function() {
axios.get(...).then((response) => {
return response.data
});
}
}
}
</script>
In this case, I'd like Puppeteer to render the topHeadline as text in a simple DIV tag, while leaving the getWidgetList un-evaluated, so the end-user would still perform the axios request and then render a list of components dynamically.
Is this possible? How do I instruct puppeteer to evaluate some scripts but leave others alone?
vue.js vuejs2 puppeteer
It sounds like you're looking for server side rendering. Puppeteer isn't designed to do that kind of thing. Vue has a guide for setting it up.
– jfadich
Nov 8 at 23:06
add a comment |
up vote
-1
down vote
favorite
up vote
-1
down vote
favorite
I'm using Vue to create an SPA (single-page app) and would like to render some semi-static pages. "Semi-static" meaning I'd like specific parts of the page to be rendered as their eventual HTML markup, while other parts of the page should remain dynamic just like the original Vue SPA.
A very basic example of one of my Vue templates:
<template>
<div>{{ topHeadline }}</div>
<div>
<widget
v-for="(w, index) in getWidgetList"
v-bind:key="w.id"
v-bind:name="w.widgetName"
v-bind:obj="w"></widget>
<div>
</template>
<script>
import { stateholder } from '../state/index.js'
export default {
data () {
return {
headlineText: stateholder.state.headline ,
versionNum: stateholder.state.versionNumber
}
} ,
methods: {
topHeadline: function() {
return `{$headlineText} v.{$versionNum}`
} ,
getWidgetList: async function() {
axios.get(...).then((response) => {
return response.data
});
}
}
}
</script>
In this case, I'd like Puppeteer to render the topHeadline as text in a simple DIV tag, while leaving the getWidgetList un-evaluated, so the end-user would still perform the axios request and then render a list of components dynamically.
Is this possible? How do I instruct puppeteer to evaluate some scripts but leave others alone?
vue.js vuejs2 puppeteer
I'm using Vue to create an SPA (single-page app) and would like to render some semi-static pages. "Semi-static" meaning I'd like specific parts of the page to be rendered as their eventual HTML markup, while other parts of the page should remain dynamic just like the original Vue SPA.
A very basic example of one of my Vue templates:
<template>
<div>{{ topHeadline }}</div>
<div>
<widget
v-for="(w, index) in getWidgetList"
v-bind:key="w.id"
v-bind:name="w.widgetName"
v-bind:obj="w"></widget>
<div>
</template>
<script>
import { stateholder } from '../state/index.js'
export default {
data () {
return {
headlineText: stateholder.state.headline ,
versionNum: stateholder.state.versionNumber
}
} ,
methods: {
topHeadline: function() {
return `{$headlineText} v.{$versionNum}`
} ,
getWidgetList: async function() {
axios.get(...).then((response) => {
return response.data
});
}
}
}
</script>
In this case, I'd like Puppeteer to render the topHeadline as text in a simple DIV tag, while leaving the getWidgetList un-evaluated, so the end-user would still perform the axios request and then render a list of components dynamically.
Is this possible? How do I instruct puppeteer to evaluate some scripts but leave others alone?
vue.js vuejs2 puppeteer
vue.js vuejs2 puppeteer
edited Nov 8 at 23:00


Boussadjra Brahim
4,2003628
4,2003628
asked Nov 8 at 22:09


ExactaBox
2,579717
2,579717
It sounds like you're looking for server side rendering. Puppeteer isn't designed to do that kind of thing. Vue has a guide for setting it up.
– jfadich
Nov 8 at 23:06
add a comment |
It sounds like you're looking for server side rendering. Puppeteer isn't designed to do that kind of thing. Vue has a guide for setting it up.
– jfadich
Nov 8 at 23:06
It sounds like you're looking for server side rendering. Puppeteer isn't designed to do that kind of thing. Vue has a guide for setting it up.
– jfadich
Nov 8 at 23:06
It sounds like you're looking for server side rendering. Puppeteer isn't designed to do that kind of thing. Vue has a guide for setting it up.
– jfadich
Nov 8 at 23:06
add a comment |
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53216905%2fvue-puppeteer-evaluate-specified-scripts-only%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
poI,1DAjFD8JqGIjZtpmK0DqVIz0gBV7gIFgadzQNIwJKiz,WJo5l 1V34juS jYHoP3BGst 6Pzc53
It sounds like you're looking for server side rendering. Puppeteer isn't designed to do that kind of thing. Vue has a guide for setting it up.
– jfadich
Nov 8 at 23:06